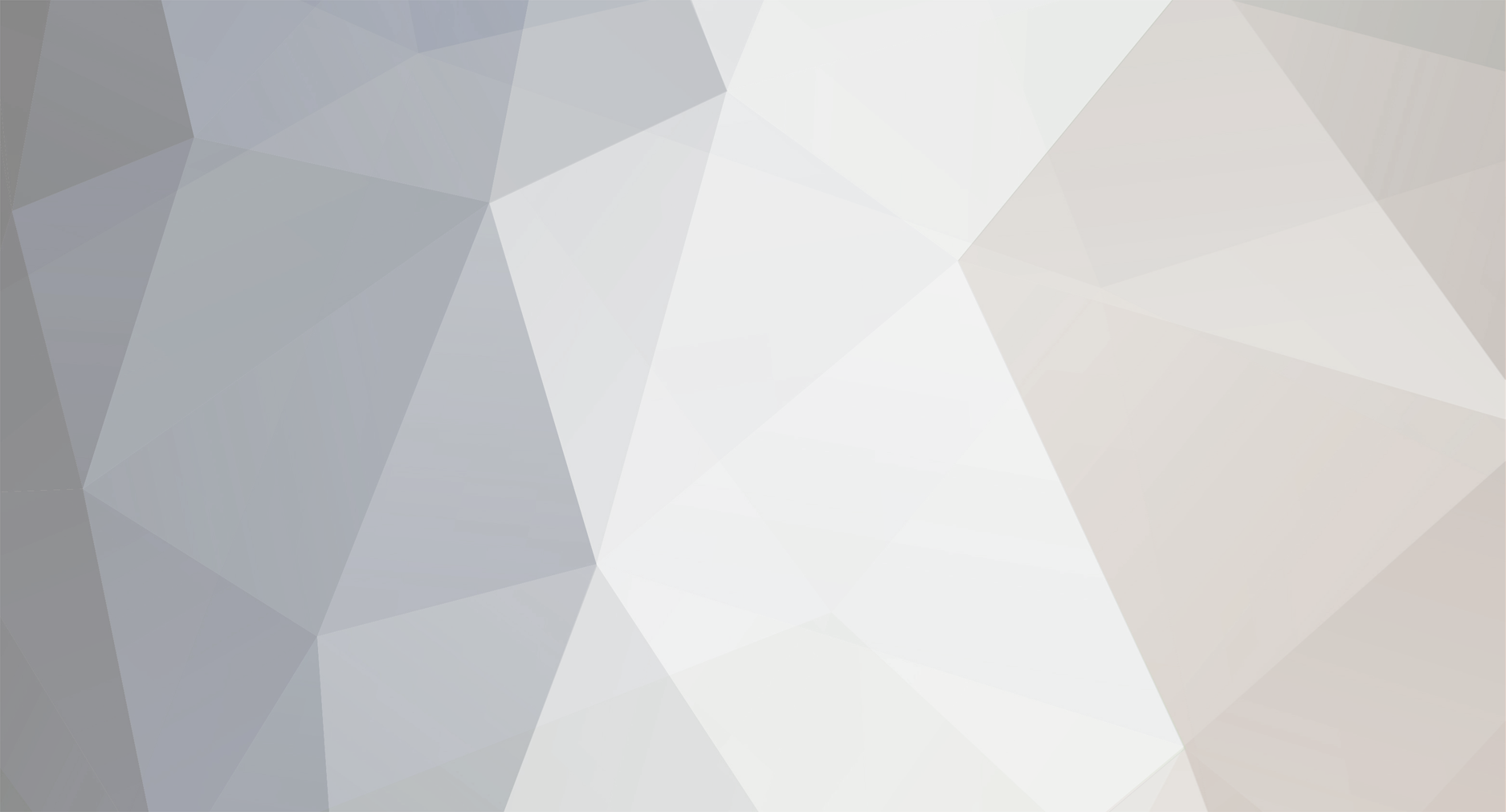
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
When running queries - especially dynamic ones (i.e. they have variables) I would highly encourage you to create the queries as string variables first. That way if there is an error you can echo the query out for debugging purposes. Also, there is absolutely no reason to use this: mysql_real_escape_string((int)$id) If you have forced the value to be an integer, then mysql_real_escape_string() is not doing anything of value. using (int) is already making the value safe for a DB query Based upon the partial error you posted the only query which that would come from is this one: $query = mysql_query("SELECT * FROM sub_nav WHERE subNav_ID=" . mysql_real_escape_string((int)$id)); BUt, you don't have any error handling that would have displayed that error! Are you sure that is the correct code that is producing the error? Try changing to the following $id = (int) $id; $sql = "SELECT * FROM sub_nav WHERE subNav_ID = {$id}"; $query = mysql_query($sql); if(!$query) { die("Query: {$sql}<br>Error: " . mysql_error()); }
-
You still haven't given the exact table details (at least the relevant fields). But, you say that " . . . they keep track of just the amount of cleaner they use and when." So, I would assume that the records have a date on them. So, you do not need to use an arbitrary 22 to get results per month as you can get ACTUAL per month values using the query with a GROUP BY using the month.
-
My guess would be that one of the queries is not returning any records. But, that logic is kinda inefficient. Why do you need to do this if ($row['playerName'] == $playerName) { return $rank; } Just make the query so it only returns records where that condition is true. Go take a look here: http://stackoverflow.com/questions/3614666/mysql-get-row-position-in-order-by
-
The number of records should be irrelevant. Please post the table structure and try to explain the logic accordingly. Why are you using some arbitrary number such as 22 when you should be able to easily calculate the average per month using real data?
-
Hmm . . . when I provide code I don't expect the person receiving the code to use it verbatim. But, I do expect the recipient to try and understand the code. And, if something is not understood then to ask questions. If you had used the code as I submitted it it probably would have worked perfectly assuming there were no minor typos. What you implemented makes no sense. You have two while loops to process the query results with one embedded in another. The first while loop will extract the first record and create the header (which I showed a better method). Then the inner while loop will process the individual records. But, it will start at record #2 since the first record was already consumed.
-
Again, it all depends on what you need. There is no one-size-fits-all solution. Just off the top of my head, I would probably do this in the query. Primarily based on the requirement to calculate an amount "per month" would be very easy in a query by utilizing a GROUP BY clause.
-
Where's your code? If the results are off by 1, I typically see this when a mysql_fetch is called before the loop starts. The only other thing I can think of is the use of mysql_result() before the loop. But, the manual doesn't state that using mysql_result() will move the pointer ahead a record. So, that doesn't make sense.
-
Did you verify if all the records you expect were included in the result set (hint, echo the value of mysql_num_rows() to the page). Did you inspect the HTML source to see if the records were there but not displayed due to a problem with the table formatting? Did you do any debugging and, if so, what did you find?
-
Your "mock" data is not of much value. I assume that both tables have at least two columns (i.e. fields) which you are displaying above. So, record 1 in the first table have values of "A" and "B". But, what is not apparent is what the relationship is between the records in table 1 and the records in table 2. Typically you would have a unique primary ID in one table and then use that as a foreign key in another table. Examples: Table 1 id | letter1 | letter2 6 A B 7 C D 8 E F Table 2 letter_id | number1 | number2 6 1 2 7 3 4 It's impossible to provide a solution without more info.
-
From my perspective it would depend on how elaborate the calculations need to be and what you plan to do with them. If I was doing some simple calculations I'd do it in the query. But, if I was calculating tax, shipping, etc. I'd probably do that in PHP.
-
Not tested, but logic should be good <?php //Set number of columns to use $maxCols = 3; error_reporting(E_ALL ^ E_NOTICE); ini_set("display_errors", 1); require ('includes/config.inc.php'); include ('./includes/header.html'); require (MYSQL); include ('./includes/main.html'); if($id = isset($_GET['catID'])) { //Create and run query to get product category names for the selected cat ID $query = "SELECT `product`.`product`, `category`.`cat`, `product`.`prod_descr`, `category`.`cat_descr`, `product`.`price`, `product`.`image` FROM `product` LEFT JOIN `category` ON `product`.`catID` = `category`.`catID` WHERE `product`.`catID`='{$_GET['catID']}' ORDER BY `product`.`product`"; $r = mysqli_query($dbc, $query); if(!$f) { echo "Database query failed"; } else { //Create flag variable to determine if header needs to be displayed $catHead = mysql_result($r, 0, 'cat'); $cat_descrHead = mysql_result($r, 0, 'cat_descr'); //Open div echo "<div id='right'>"; //Display header echo "<h1><span># </span>{$row['cat']}<span> #</span></h1>\n"; echo "<h2>{$row['cat_descr']}</h2>\n"; //Open table echo "<table cellpadding='5' cols='2'>"; //Set index var to track record count $recIdx = 0; while($row = mysqli_fetch_array($r)) { $recIdx++; //Open new row if needed if($recIdx % $maxCols == 1) { echo "<tr>\n"; } //Display product echo "<td>"; echo "<img src='db/images/{$row['image']}' height=50px width=50px /><br>"; echo "<a>{$row['product']}</a><br>"; echo "<span class='price'>£{$row['price']}</span>"; echo "</td>\n"; //Close row if needed if($recIdx % $maxCols == 0) { echo "</tr>\n"; } } //Close last row if needed if($recIdx % $maxCols == 0) { echo "</tr>\n"; } //Close table & div echo "</table>"; echo "</div>"; } } include ('./includes/footer.html'); ?>
-
Well, that doesn't tell us if you want the records to be output left to right or top to bottom 1 2 3 4 5 6 7 8 9 Or 1 4 7 2 5 8 3 6 9 The first option is much easier to implement, so I'll provide a sample for that in a few minutes
-
Submit Form -> Radio Buttons left unselected don't send
Psycho replied to Bhayes21's topic in PHP Coding Help
Well, as you've seen an unselected radio button will simply not be included in the POST data. Therefore, you need to create a method to handle it. Here are some options: I assume the processing script is only processing based upon what is sent. You could instead change that to process based upon what is EXPECTED. So, if you expect 10 questions, then loop through those 10 questions and check if there was a response. If not, you know it wasn't answered. If you still want to reply upon the submitted data for your processing logic, you could add a hidden field for each question for the purposes of processing. E.g. <input type="hidden" name="questions[1_01]" /> <input type="hidden" name="questions[1_02]" /> <input type="hidden" name="questions[1_03]" /> . . .[code] You can then simply loop through the "questions" array and look for the corresponding answer if one exists. Lastly, you could use some JavaScript to handle this. But, I'd advise against it since it would not be as reliable. -
You can rename the file on the server to whatever you want. I even recommend it. Whatever name, whatever directory. What the user will see is what you show them. As long as you store the original information (eg, filename) somewhere then you can show it to them later. For example, download scripts. The URL can be anything because the (suggested) name of the downloaded file is given by your script in the Content-Disposition header. Thanks for the advice. How do I go about renaming the file back to the original when It's downloaded? And also thanks to The Little Guy for the link but I'm trying to not use any outside programs requiring a license. Just store the original file name in your database, then look for some example download scripts - most of them should show how you can dynamically set the file name for the download. Also, the getID3() class is a free for non-commercial use. And, depending on how you implement it you can probably use it for commercial use without payment. look at the info at the bottom of the page.
-
I agree with both of the above.
-
Marking topic solved.
-
In the item.php page you are creating the links to the products like so echo "<td>". "<li>". "<a href='item.php?product={$row['product']}'>" . "<img src='db/images/".$row['image']."' height=50px width=50px />" . "</a>" . "</li>" . "</td>"; echo "<td>". "<li>" . "<a href='item.php?product={$row['product']}'>" . $row['product'] . "</a>" . "</li>" . "</td>"; note the href='item.php?product={$row['product']}' Then in the item.php page you are trying to reference the id using if($id = isset($_GET['prodID'])) You are setting the value using the variable name 'product', but trying to reference it as 'prodID'. The two need to be the same.
-
Whether a value should be stored in a session or not is dependent on many factors. If the data needs to persists across sessions, then you will likely want to store it in a cookie or in a database. Typical things to store in a session are data to indicate that the user is logged in, shopping cart data, some preferences, etc. It's really hard to give you an answer without knowing what the variable is used for.
-
Let's not do that. The reason is that understanding where the data is coming from and what the ultimate disposition of that data can have significant impact on the "best" solution. One thing that really irritates me is when someone on these forums asks for a solution without providing all the relevant information. Then, once a solution is provided, they provide some tidbit of information that they *thought* was not pertinent but which, consequently, completely changes the solution needed. You don't even state "how" the user is inputting the information or how it is being received. Is the user entering data into individual fields for each piece of data for each user? Is there a csv or some other type of file input? And, again, what are you planning to DO with this data. having a user enter 120+ records of data for the sole purposes of sorting it and displaying it on screen without actually saving it sounds pretty worthless. By the way, if this is a homework problem, which I'm thinking it is, we will be glad to help, but you have to at least show some attempt at the problem.
-
You need to be more specific. You say you want three columns with data in the format Name Age Name Age Name Age But, that looks like data in two columns. So, are you wanting data in 6 columns (2 per record) or is the name and age in the same TD cell? Not really clear what you need/want.
-
Well, you don't show the full content you are working with. It's quite possible you don't need the regex you already have - is there other content on the page with "Code.Number" that is outside the UL tags? if not, you just need one regex pattern for the page. preg_match_all("#Code.number\('([^']*)#", $text, $matches); print_r($matches[1]); Output: Array ( [0] => 4.236173.USD.EN.057880560561287921.386595955 [1] => 4.236173.USD.EN.057880560561287921.386595955 [2] => 4.1957403.USD.EN.057880560561287921.458090474 [3] => 4.1957403.USD.EN.057880560561287921.458090474 [4] => 4.4801652.USD.EN.057880560561287921.1469771421 [5] => 4.4801652.USD.EN.057880560561287921.1469771421 )
-
Right, you should ONLY store the minimum data necessary in order to identify the product. Things like descriptions, price, etc. can change. Therefore, you always want to get the freshest data using the ID.
-
Match is a reserved word in MySQL. Either change the field name or use backticks around the field name in your queries: `match`
-
Using cookies to store shopping cart data is a pretty standard practice. If you are running into problems then you are likely storing way more data than you need to. For a standard shopping cart you would just need a simple array such as $_COOKIE['cart'] ( [2] => 10, [4] => 1, [9] => 3, [12] => 1 ) where the numeric index is the product id and the value is the quantity. It might require a more complex format if the products can have more variables such as size and color, but not to the extent that you would have a problem with the cookie size.
-
FYI: Here is a case-insensitive glob() solution to find ".jar" files $files = glob("/path/to/dir/*.".sql_regcase('jar')); BUt, to the OP's problem, since the data is created externally the array_filter() function is probably the correct solution.