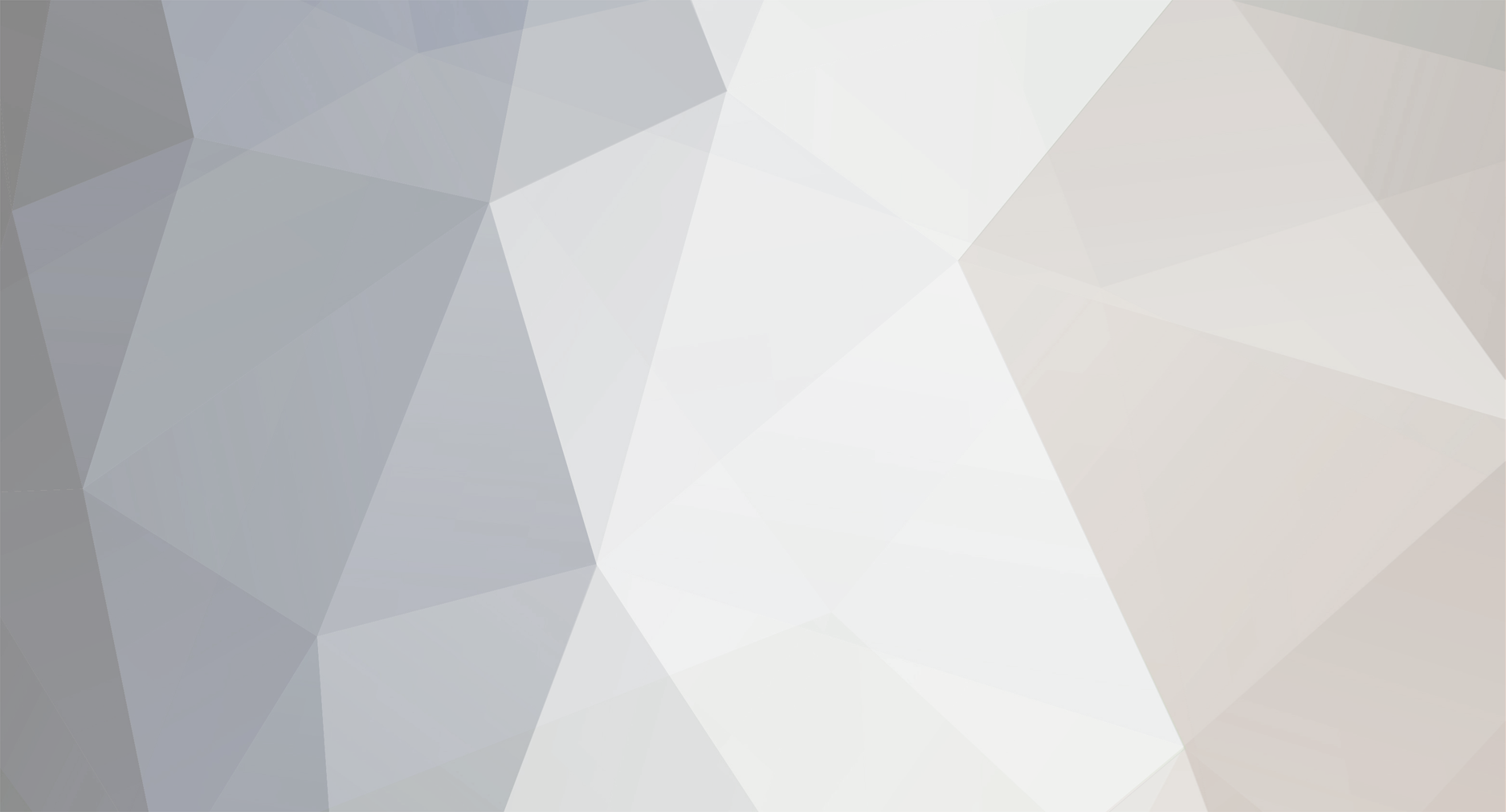
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
heh... Psycho, my thoughts are that the OP stated he is grabbing files from a directory and looping through them to display them each in a new tbl row. I'm thinking he can use glob to grab all of the .jar files from the directory and foreach through them that way, something like this: Unless one of the files happens to be named .JAR, .Jar, etc. Then, using "*.jar" in the glob() function will not work. glob() doesn't accept true regex expressions, but you can build a case-insensitive expression using the function I posted above.
-
@AyKay47: Note that glob() is case sensitive. There is a function that can convert a string into a pattern that will make glob() work in a case insensitive manner, but I cannot find it. EDIT: Found it sql_regcase()
-
Where is this data coming from exactly? There may be better ways to get the data initially that would not require any post-processing. But, to answer your question, you can filter an array using the oddly named function array_filter().
-
You are trying to loop through the results two times. That is not necessary, simply create a flag variable to determine if the header needs to be displayed. //Create and run query to get product category names for the selected cat ID $query = "SELECT `product`.`product`, `category`.`cat` FROM `product` LEFT JOIN `category` ON `product`.`catID` = `category`.`catID` WHERE `product`.`catID`='{$_GET['catID']}' ORDER BY `product`.`product`"; $result = mysqli_query($dbc, $query); //Create flag variable to determine if header needs to be displayed $showHeader = true; echo "<div id='right'>\n"; while($row = mysqli_fetch_array($r)) { if($showHeader) { //Display category header echo "<h1>{$row['cat']}</h1>\n"; $showHeader = false; } //Display product name echo "<p>{$row[2]}<br /></p>\n"; } echo "</div>\n"; } Alternatively, since you will have only one header, you could use mysql_result() to pull the header name out of the result set without moving the pointer ahead //Create and run query to get product category names for the selected cat ID $query = "SELECT `product`.`product`, `category`.`cat` FROM `product` LEFT JOIN `category` ON `product`.`catID` = `category`.`catID` WHERE `product`.`catID`='{$_GET['catID']}' ORDER BY `product`.`product`"; $result = mysqli_query($dbc, $query); $cat_header = mysql_result($result, 0, 'cat'); echo "<div id='right'>\n"; echo "<h1>{$cat_header}</h1>\n"; while($row = mysqli_fetch_array($r)) { //Display product name echo "<p>{$row[2]}<br /></p>\n"; } echo "</div>\n";
-
OK, so what is the current code doing and how do you want it changed? What have you already tried and what were the results? Are you expecting someone to simply modify the code for you?
-
I don't believe either of those strings would cause SQL Injection unless you were doing some really bad practices such as eval() on that data before creating the query. Now, I can definitely see those strings causing other problems not related to SQL injection. What problems did you experience that makes you think this is SQL Injection and not XSS attack or some other problem?
-
Repeatedly updating and displaying sql query results
Psycho replied to kehaglund's topic in PHP Coding Help
You would want to use AJAX for this. Basically the page with have a JavaScript function that will trigger every n seconds to get the new data. The JavaScript will make a request to the server (e.g. PHP code) to get the new data. The PHP code will get the data and return it back to the JavaScript code. The JavaScript code will then take the returned data and repopulate the appropriate content on the page. There are tons of tutorials available out there on how to do this. trying to explain the entire process in a forum post is not realistic. -
Logical Sequence Mistake or Variable Assignment Mistake
Psycho replied to cosmic_sniper's topic in PHP Coding Help
I DID cover that in my first response, did I not? Even so, as I stated in my previous post a switch() statement is a more appropriate solution than a bunch of if/else statements in this scenario. -
Logical Sequence Mistake or Variable Assignment Mistake
Psycho replied to cosmic_sniper's topic in PHP Coding Help
EDIT: DavidAM already provided the same solution, but I'll leave this anyway since I included the use of switch() which is more appropriate for this. The form name is not included in the POST data. Only the fields are passed. What you will want to do is create a hidden field in each form to determine which processing to do. And, if the input fields for each form is the same, then make the field names the same. Form <form action="output.php" method="post" name="a"> req1: <input type="text" name="req_1"> req2: <input type="text" name="req_2"> <input type="hidden" name="form" value="a"> . . . <input type="submit"> </form> ------ . . . <form action="output.php" method="post" name="b"> req1: <input type="text" name="req_1"> req2: <input type="text" name="req_2"> <input type="hidden" name="form" value="b"> . . . <input type="submit"> </form> Processing code switch($_POST['form']) { case 'a': //Insert processing logic for form a here break; case 'b': //Insert processing logic for form b here break; case 'c': //Insert processing logic for form c here break; case 'd': //Insert processing logic for form d here break; } -
Logical Sequence Mistake or Variable Assignment Mistake
Psycho replied to cosmic_sniper's topic in PHP Coding Help
Don't just slap things into the code hoping it will work. Make sure you understand what you are doing. using a single equal sign is an assignment operator. It assigns the second argument to the first argument. So, if you have if($foo = 'bar') That will always return true because the PHP parser can successfully assign the value of 'bar' to the variable $foo. However,a double equal sign is a comparison operator - which is what you would normally use in an if() statement. So, if this if($form_name == "a") { is always returning false, that is because $form_name does not equal the string 'a'. You are assigning the value to $form_name using $form_name = $_POST['a']; but there is no input field with the name of 'a', so I would assume $form_name is always equal to NULL. I see fields called 'a_req_1' and 'a_req_2' though. I really can't provide any specific code help because I have no idea what fields you should be using or what your ultimate goal is. -
You never stated you were using their API - which would have been pertinent information if someone was trying to help you.
-
What you are trying to do violates YouTube's Terms of Service
-
How are you "forwarding" from the processing page to the display page? You shouldn't be forwarding the user and in stead should have separate scripts to break up the functionality and INCLUDE the scripts. I'm guessing you are doing a header() redirect or something similar which wipes out any post data. Here is an example of one way: process_page.php //Do some validation to ensure you have the necessary info to actually do the processing if(!isset($_POST['foo']) { echo "Error: Unable to process your request"; } else { include('get_data_page.php'); if(!$result) { echo "Error: unable to get data<br>" . mysql_error(); } else { include('display_page'); } } get_data_page.php //Create/run query $fooVar = mysql_real_escape_string($_POST['foo']); $query = "SELECT * FROM table WHERE foo = '$fooVar'"; $result = mysql_query($query); display_page.php while($row = mysql_fetch_assoc($result) { //Create output }
-
Hmm, I've never put spaces between the table name and the table field like that. So, don't know if that is the cause. But, I would definitely suggest using "proper" joins. It makes it more logical to separate the JOIN logic from the WHERE logic. Try the following: $q = "SELECT a.col_1, a.col_2, a.col_3, b.col_1, b.col_2, b.col_3, c.col_1, c.col_2, c.col_3, d.col_1, d.col_2, d.col_3 FROM table_A AS a LEFT JOIN table_B AS b ON b.f_uid = a.uid LEFT JOIN table_C AS c ON c.f_uid = a.uid LEFT JOIN table_D AS d ON d.f_uid = a.uid WHERE a.signed_up = '1' ORDER BY a.some_col";
-
I agree with The Little Guy's solution, but I wanted to comment on part of your current code above: $rows = mysqli_num_rows($r); for ($j = 0 ; $j < $rows ; ++$j) { $row = mysqli_fetch_row($r); echo '<div id="right">'; echo '<h1>'. $row[1] . '</h1>' . '<br />'; echo '<br />'; echo '</div>'; } That is an inefficient solution. No need to calculate the number of rows and then create a for loop. Instead, just use a while loop. That is the standard for extracting the records from a query result. Also, I would highly advise using mysqli_fetch_assoc() to get the fields by name instead of the numeric index. If you make changes to the DB structure or queries later you will have a lot of code that needs to be "fixed" if you use the numeric index rather than the name. I also changed the output to use double quotes for the string delimiter so you can add linebreaks to the HTML output. Makes a world of difference when debugging the HTML output. while($row = mysqli_fetch_assoc($r)) { echo "<div id='right'>\n"; echo "<h1>{$row['cat']}</h1><br /><br />\n"; echo "</div>\n"; }
-
mysql_fetch_assoc() only pulls one record at a time from the result set. You will need a loop to get all the records. The manual for mysql_fetch_assoc() has an example script on how to do this. http://php.net/manual/en/function.mysql-fetch-assoc.php
-
Need help to extract data from the webpage and insert to my database
Psycho replied to erankaw's topic in PHP Coding Help
We cannot help you with that as doing so would violate the terms of service for that site: http://www.wunderground.com/members/tos.asp -
Inserting multiple values into the same mysql column
Psycho replied to ebotelho's topic in PHP Coding Help
The drawback with that is that every time you create a new "game" you have to populate the intermediary (i.e. bridge) table with records for all the users. Then if you add players you have to also add records to the intermediary table for all the existing games. That requires a lot more overhead and is not a normalized database. Any new players that are added shouldn't require you to add records to associate that player with all the existing games with a default of unconfirmed. The lack of a confirmation records implies that they are unconfirmed. -
Inserting multiple values into the same mysql column
Psycho replied to ebotelho's topic in PHP Coding Help
I agree with smerny, except that I would suggest using a proper JOIN in the query. The above query has a problem in that it would not return a result if there were no confirmed players. So, if you create the game first, then go to another page to show the game and the confirmed players it wouldn't even show the game details. Also, if you need the confirmed AND unconfirmed players you need to do a second JOIN using the RIGHT parameter. The following example would return the details of the match and the confirmed players SELECT j.data, j.criado, u.player_name FROM jogos AS j LEFT JOIN confirmed AS c ON j.id = c.jogos_id RIGHT JOIN usuários AS u ON u.player_id = c.player_id WHERE j.id = '$game_id' This is just off the top of my head and not tested, but it should be pointing you in the right direction. -
Not sure about dragon_sa's solution, but I decided to give it a whack and came up with the following. I've tested it and confirmed it works. It returns an array of all the prime numbers up to and including the target number function displayPrime($target) { if((int)$target != $target || (int)$target < 2) { return false; } $primes = array(2); for($num = 3; $num <= $target; $num+=2) { $isprime = true; foreach($primes as $prime) { if($num % $prime == 0) { $isprime = false; break; } } if($isprime) { $primes[] = $num; } } return $primes; } $result = displayPrime(55);
-
Yeah, that's not going to work. The only way I think would work would be to start at 2 and work your way up. Then for each number you need to test if it is divisible by any of the previous prime numbers. If not, then that number is prime and add it to your result. Then move to the next number up.
-
Although the logic in your function is correct, you certainly used an over abundance of parens! however, there's no need to write your own logic for this. You can use PHP's checkdate() or even the date() function to do it for you. function leap_year($year) { if(checkdate(2, 29, $year)) { echo "Leap Year"; } else { echo "Not a leap year"; } } or,more simply function leap_year($year) { echo (checkdate(2, 29, $year)) ? "Leap Year" : "Not a leap year"; }
-
I'm not understanding your database structure from what is provided, but I'll try to offer some suggestions based on what I *think* you may have. I assume your records have a start date. But, do they also have an end date? If not, you should consider creating such a filed. So, just leave the end date NULL if that record is supposed to be used indefinitely. Then if you add a new record for the same prescription set the start date for that record and also set the end date for the previous record. Then, when you run your query, just grab all records for the user where the start date is before the end of the calendar period you are showing AND where the end date is NULL or greater than the start date for the calendar. Lastly, while processing the records you can use the end date value to determine if a particular record should be displayed on any given day.
-
Just start the URL with a "/", that will always be relative to the root of the site. <a href='/filename.php'>go to that page</a> That will load the file "filename.php" from the root of the site regardless of the location that was called to create that page.
-
Yes, but you need to be more specific on what. EXACTLY, you need to extract. You will have to create rules to parse the data. For example, one possibility would be to create a process to extract each LI element into a name/value pair where the name is determined by the text within the label tag and the value is the text within the div that immediately follows the label tag. But, if the content was ever changed, or if it could be in a different format, the rules would not work. Here is a quick example: $pattern = "#<li[^>]*><label[^>]*>([^<]*)</label><div>([^<]*)#"; preg_match_all($pattern, $input, $matches, PREG_SET_ORDER); //Clean up matches and put into //data array with named keys $data = array(); foreach($matches as $match) { $data[trim($match[1])] = trim($match[2]); } //Output results print_r($data); Using your example as the input, this would be the result: Array ( [Title] => Expatriate Tax Director [Contract type] => Permanent [Market sector] => [Country] => [Location] => Reading [salary] => 120.00 - 120.00 United Kingdom Pounds/Month [Description] => Expat Tax Director [Expires on] => February 28, 2013 [ideal candidate] => Expatriate Tax ) Note that there is no value for "Market sector" or "Country". That is because those values are enclosed in anchor tags. To get those values the pattern would need to be further modified to support values that are enclosed in anchor tags.