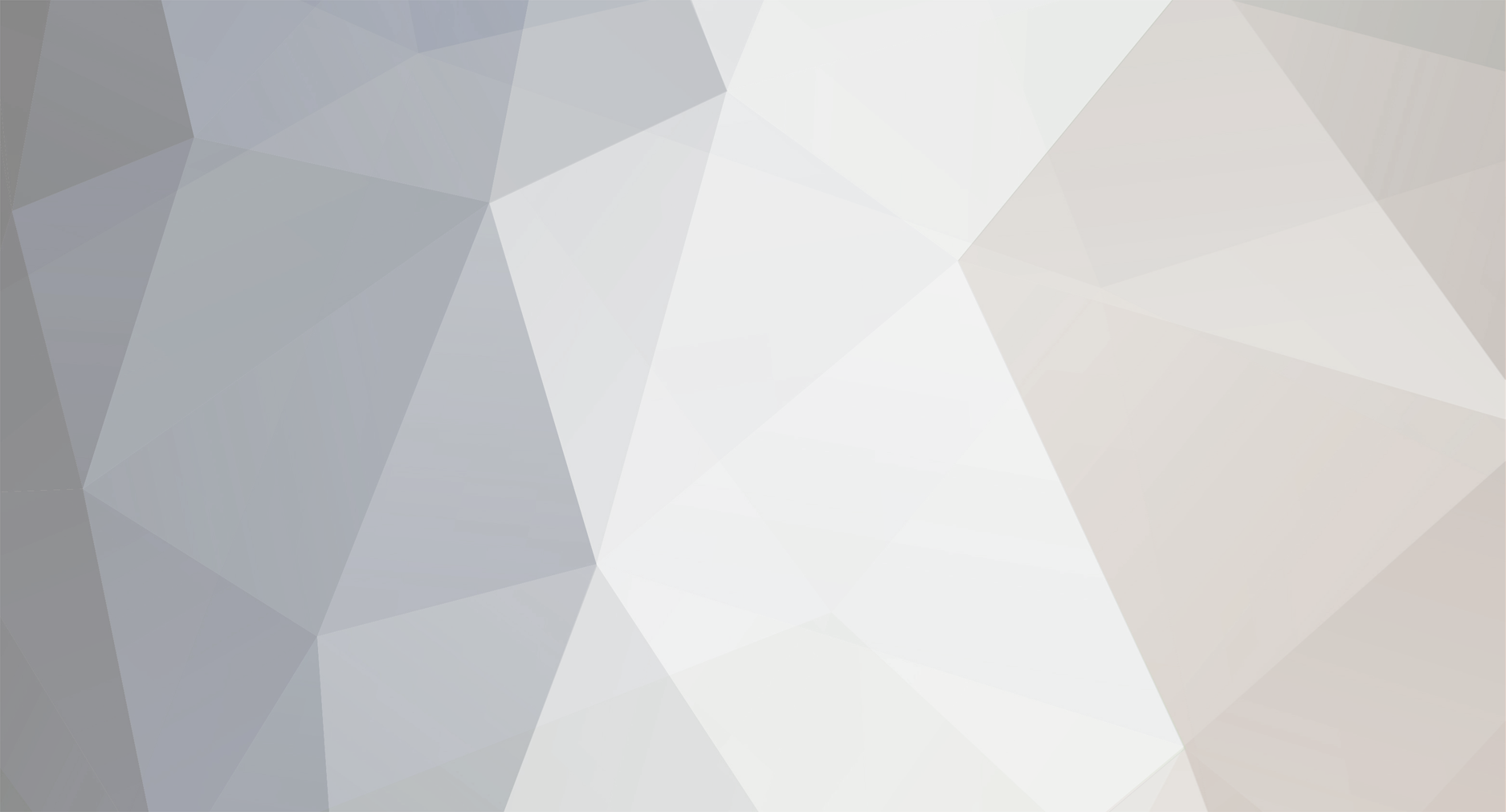
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
I don't see anything in that code that would necessarily cause a significant performance problem. It does not show how the entire query is created. It only shows where some additional clauses (e.g. LIMIT) are added. And, it seems like the query would only be run one (unless that file is included multiple times). There does seem to be some 'potentially' unnecessary post-processing of the code. For example, there is code to eliminate rugs without any stock from the results. That would be better handled in the WHERE clause of the query. But, that again does not "appear" to be something that would cause significant performance problems. If you did not write this code and do not understand it and this is for a production site, I would really advise you bring someone in to fix this for you. We can only point out some suggestions on particular pieces of code we see. Without a full understanding of how the whole thing works there is the very real possibility that suggested changes could create other, more serious problems. Some may become readily apparent while others may not be easily exposed but are nonetheless significant. This forum is for people to get help with code they have written. That's not because we don't want to help, it's because the person writing the code should have enough knowledge to post the relevant section of code they are having difficulty with and will understand how any changes would impact other areas of the application.
-
My guess is they are selecting something, then looping through it and selecting something else for each result. Zactly! I've seen this before where the output needed to have several sets of subheadings by company, vendors and purchases. The developer did a query to get the list of companies and then did a nested loop running a new query each time to get the vendors for that company. Then did another nested loop to do queries to get the data for those vendors for that company. So the page needed to run the number of queries based on: # companies X # vendors X # purchases. After a couple of months in use the amount of data was causing the report to time out. I fixed it by simply creating a single query that joined the records and used "flag" variables while processing the data to determine when to display new headers. BAD Example (Many queries based upon number of records) //Run query to get types $query_types = "SELECT type_id, type_name FROM carpet_types"; $result_types = mysql_query($query_types); while($row1 = mysql_fetch_assoc($result_types)) { //Display type name echo "<h1>Type: {$row1['type_name']}</h1>"; //Run query to get carpets matching this type $query_carpets = "SELECT carpet_name, price FROM carpets WHERE type_id = {$row1['type_id']}"; $result_carpets = mysql_query($query_carpets); while($row2 = mysql_fetch_assoc($result_carpets)) { //Display carpet info echo "Name: {$row2['carpet_name']}, Price: {$row2['price']}<br>"; } } GOOD Example (One Query) //Run ONE query to get ALL carpet information - including the type info //Ensure records are sorted appropriately $query = "SELECT c.carpet_name, c.price, t.type_id, t.type_name FROM carpets AS c LEFT JOIN carpet_types AS t ON c.type_id = t.type_id ORDER BY t.type_id"; $result = mysql_query($query); $current_type = false; //Flag to detect change in type while($row = mysql_fetch_assoc($result)) { //Display type if different than last if($current_type != $row['type_id']) { echo "<h1>Type: {$row1['type_name']}</h1>"; } //Display carpet info echo "Name: {$row2['carpet_name']}, Price: {$row2['price']}<br>"; }
-
Yet you felt the need to write a couple paragraphs to defend it? jk But, I agree with josh. The difficulty in trying to craft a RegEx to find "valid" dates is not worth the effort. Simply use the regex to pull "possible" date matches and then validate that they are valid using some other means, e.g. checkdate() Besides, by trying to inject validation into the expression it is actually modifying invalid inputs into valid inputs. If a user wanted to enter a date of 2/3/2011 and accidentally entered 2/3/20011, the RegEx you provided would not capture the last '1' and would find a "valid" date of 2/3/2001. The same problem exists with the beginning of the matches. Following josh's method, just capture any possible matches THEN use a proper method to validate the matches. Building upon josh's expression, I made two changes. 1) I used \d instead of 0-9 and 2) I captured the month, day, year parts as separate values expressly for the purpose of running them through checkdate() //Find possible matches preg_match_all("#([\d]+)/([\d]+)/([\d]+)#", $INPUT, $matches, PREG_SET_ORDER); //Find matches that are VALID dates and put into array $valid_dates = array(); //Array to hold all valid dates foreach($matches as $match) { if(checkdate ($match[1], $match[2], $match[3])) { //Save the date to the array of valid dates. //Can convert to timestamp or specific format as needed $valid_dates[] = $match[0]; } } Also, if you wanted to also allow dates that use a dash as the delimiter (e.g. 2-15-2003) you could use this expression "#([\d]+)[/|-]([\d]+)[/|-]([\d]+)#"
-
You take the input submitted by the user and: 1. Validate that the values are appropriate (e.g. was a topping of wingnuts or anything which you do not provide). 2. Sanitize the values so that they are safe for use in a query (some validation can do this as well, such as verifying the amount is a valid number). 3. Create an insert query. Since you are wanting to insert multiple records you should "add up" all the records to build one insert query such as INSERT INTO table_name (field1, field2, filed3) VALUES ('record1-value1', 'record1-value2', 'record1-value3'), ('record2-value1', 'record2-value2', 'record2-value3'), ('record3-value1', 'record3-value2', 'record3-value3')
-
Perhaps, but the question wasn't about what new technology can I learn to solve this problem, it was about "how do I solve this problem". AJAX has many benefits, but it also has it's drawbacks. If you use it to populate "linked" select lists a request must be sent back to the server each time a primary select list is changed and then the results are sent back to the client to be used to update the child select list(s). That process will take some time. Depending on many factors that process can be very quick - so much so it is imperceptible to the user - or the process can take a second or more. A second or two may not seem like much, but from a user's perspective it makes the site seem slow or buggy. In fact, if there are times when the whole process may hang due to a blip in transmission. In addition, since there will be some delay that you cannot know ahead of time you would have to implement some checks and balances into the page. For example, what if a user changes the first option and then, before the 2nd list has been updated by the AJAX call, the user changes the value in the 2nd list? You would have to disable the 2nd list before the AJAX call is made and then re-enable after the list is updated. I have personally seen where this had to be done because the AJAX call could take a couple seconds to complete. If you have a somewhat finite list of options the best option, in my opinion, is to simply implement it completely client-side. The performance will be instantaneous and you don't have to worry about all the other problems.
-
Well, you don't necessarily need to use AJAX, but you definitely want to use JavaScript. If the lists of all possible options is not excessive, then you can simply load all the data into the page as JavaScript data and have all the functionality happen client-side. However, if the list of all options is very large or if the available options can change based upon other activities then you would want to use AJAX. I know I have several posts in these forums providing a JavaScript only solution. Here is one: http://www.phpfreaks.com/forums/index.php?topic=148720.msg640073#msg640073
-
Pretty much, but I would make a few comments. Give tables and fields meaningful names that will provide context to the purpose and relationship of the data. It will make a world of difference when you are coding - especially when you need to go back and change things. I would change the "Recipients" table to "Message_Recipients" which implies that it is dependent on the messages. Also, the recipients table does not need a unique ID field since it would not be needed for anything. The recipient records are associated with the messages table using the message id. Lastly, for the foreign key references don't give them a name that is completely different than the primary key name. For example, I would use "from_member_id" for the "member_id" FK. (in fact I, personally, would never name a field simply "id" even as the primary key). Here is the layout I would suggest: Member table: - id - first_name - username - email Message table: - id - from_member_id (FK to id in Member table) - sent_on - subject - body Message Recipient table: - message_id - to_member_id - read_on Then to get the data for a message and all the recipients you would use SELECT * FROM messages LEFT JOIN members AS m1 ON messages.from_member_id = m1.id LEFT JOIN recipients ON messages.id = recipients.message_id LEFT JOIN members AS m2 ON recipients.to_member_id = m2.id WHERE message.id = '$messageid' That's only a rough example, you would only want to explicitly incude the necessary fields in the select list
-
Is it an array with just one record or would you have multiple "POSTS" in the array? If you have multiple posts, the output format will not work - or, at least, you need to show an example of what you want for a mufti-record output because it doesn't make sense to me. Plus, why would you want to make the tags elements separate subarrays with only one element. That really makes no sese. I can see making the tags a subarray, but not with each one as a single element array. I was going to provide a solution, but after looking at what you are really asking for I would rather see "why" you think you need that. I think we can probably provide a better solution for processing the data than what you may be doing now.
-
Images are not uploading onto webpage from phpmyadmin
Psycho replied to jayjay1234's topic in PHP Coding Help
I don't see anyhing in your output that would generate an image in the output. Typically, the image path/name of the image is stored in the database and then when generating the output you would do something such as echo "<img str='{$row['product_image']}' /> But, again, I don't even see a field referenced in the output that would seem to be an image. Also, you *can* store the actual image data in the DB and dynamically create the images, but that's a little more complicated and not what I think you want at this time. So, what is the field in the DB where you are storing the image info and what do those contents contain? -
Agreed. strtotime() will always interpret the same input as the same value. Note, there *could*be some differences on how one server would interpret based upon the locale settings of that server (not 100% sure), but each server would always interpret the same input as the same value. For me, running "01 July 2012" through strtotime() interprests it correctly as the first day of July in the year 2012. So, your problem, must be due to some other problem. If the user is entering the dates directly the interpretation of strtotime() can be affected by certain formatting. You can easily verify whether strtotime() is generating the right result by adding some debugging code. For example: $user_input = $_POST['new_date']; $new_date = strtotime($user_input); echo "Date from user: {$user_input}<br>\n"; echo "Date from strtotime: " . date('F j, Y', $new_date);
-
Then you don't need the if/else for the row color either function perDetails($var1, $result, $var2) { $dft_img = 'lib/images/name.png'; echo '<div class="desc">'.$var1.'</div>'; echo '<table align="center" cellpadding="2" cellspacing="1">'; while($row = mysql_fetch_array($result)) { $img = ($row['pphoto_localurl']!='') ? $row['pphoto_localurl'] : $dft_img; $poster = str_replace("./", "lib/", $img); $color = ($color=='#FFC600') ? '#C6FF00' : '#FFC600'; echo "<tr bgcolor='{$color}'>\n"; echo "<td><img src='{$poster}' width='32' height='42' alt='{$row['pname']}'</td>\n"; echo "<td><a href='persons.php?det={$var2}&perid={$row['pid']}'> {$row['pname']} </a></td>\n"; echo "</tr>\n"; } echo '</table>'; }
-
You are putting the if/else in the wrong place. You should use it to define the image function perDetails($var1, $result, $var2) { $dft_img = 'lib/images/name.png'; echo '<div class="desc">'.$var1.'</div>'; echo '<table align="center" cellpadding="2" cellspacing="1">'; while($row = mysql_fetch_array($result)) { $img = ($row['pphoto_localurl']!='') ? $row['pphoto_localurl'] : $dft_img; $poster = str_replace("./", "lib/", $img); echo "<tr bgcolor='#FFC600'>\n"; echo "<td><img src='{$poster}' width='32' height='42' alt='{$row['pname']}'</td>\n"; echo "<td><a href='persons.php?det={$var2}&perid={$row['pid']}'> {$row['pname']} </a></td>\n"; echo "</tr>\n"; } echo '</table>'; }
-
You could look into finding a permutation script (there have been many posts on these forums) to generate all the different ordering of the letters. Then compare those to the possible words. Although, if you have a target of words, then you could come up with a solution that counts the different letters and can compare that way. That would probably be more efficient. So, if the anagram and the target word have the same number of 'a', 'r', 's', etc. Then it is a solution. I'd probably order the letters in the words from a-z and then compare.
-
The code you last posted has the same problem I advised you to fix. You are naming the checkboxes as "pizzaID", where "ID" is the ID number of that value. That is NOT needed and actually makes the problem more difficult to solve. You also didn't solve the illogical if/else at the start of the page that is the same as the other one I pointed out. Here is how I would code this page. Note none of this is tested as I don't have your database. I expect you to find and fix any minor errors. <? session_start(); if (!isset($_SESSION['userName'])) { header('location:../index.php'); } mysql_connect("localhost", "root", "")or die("cannot connect"); mysql_select_db("deliverpizza")or die("cannot select DB"); $sql="SELECT * FROM `pizzatopping`"; $result= mysql_query($sql) or die(mysql_error()); $orderForm = ''; while($row =mysql_fetch_assoc($result)) { $orderForm .= "<tr>\n"; $orderForm .= "<td>{$row['id']}</td>\n"; $orderForm .= "<td>{$row['type']}</td>\n"; $orderForm .= "<td>{$row['size']}</td>\n"; $orderForm .= "<td>{$row['topping']}</td>\n"; $orderForm .= "<td>{$row['cost']}</td>\n"; $orderForm .= "<td>{$row['info']}</td>\n"; $orderForm .= "<td><input type='checkbox' name='pizzas[]' value='{$row['id']}' /></td>\n"; $orderForm .= "</tr>\n"; } ?> ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Deliver-Pizza Topping</title> <link href="css/pizza-topping.css" rel="stylesheet" type="text/css" /> </head> <body> <div id="container"> <div id="header"> <img src="images/logo.jpg" alt="logo" /> <a href="log-off.php" id="logg-off" >log off</a> <ul id="nav"> <li><a href="home.php" target="_self">Home</a></li> <span> | </span> <li><a href="Pizza-Topping.php" target="_self">Pizza Topping</a></li> <span> | </span> <li><a href="order.php" target="_self">Order</a></li> <span> | </span> <li><a href="Account.php" target="_self">Account Details</a></li> <span> | </span> </ul> </div> <div id="Featured"> <img src="images/banner1.jpg" alt="banner" name="banner"" ID="banner"></div><!--end Featured--> <div class="featuredimage" id="main"> <div id="content" class="tiles"> <h1>Pizza-Topping</h1><hr /> <p>The list bello are the pizzas available to order</p> <div id ="staff"><div style="width:920px; height:autopx; overflow:auto; heightmax:300px"> <table> <tr> <th>pizza number</th> <th>Type</th> <th>Size</th> <th>Topping</th> <th>Cost</th> <th>Information</th> <th><form name="pizza-order" action="order.php" method="post"><input type="submit" name="submit" Value="Order"></th> </tr> <?php echo $orderForm; ?> </table> </div> <!--<form name="pizza-order" action="order.php"> Pizza Number<input type="text" Name="pizza-1">Quanity<input type="text" Name="quanit-1y"><br/> Order 2<input type="text" name="order-2"><br/> <input type="submit" name="submit" Value="Order"> </form>--> </div> </div> </div> <!--end content--> <div id="footer"><span><p>Created By Ryan Williams</p></span></div><!--end footer--> </div><!--end container--> </body> </html>
-
If you are not going to take any of the advice that is given, why are you asking for help? The way that you are currently creating your checkboxes is flawed and making the whole process much more difficult than it should be. You should not be giving all the of the checkboxes unique names. You will already know which checkboxes are checked based upon the passed values. Name all the checkboxes the same (as an array).
-
This topic has been moved to PHP Regex. http://www.phpfreaks.com/forums/index.php?topic=356497.0
-
Well, here is the while loop while($row = mysql_fetch_array($results)){ And the error states So, apparently $results is a string. Let's look where it is defined $qstring = $Fixit.",".$offset.",".$rowsperpage; $results = $qstring; I have a feeling you meant to RUN the query and assign the results to $results like this $qstring = $Fixit.",".$offset.",".$rowsperpage; $results = mysql_query($qstring) or die(mysql_error());
-
$regex = "#(\[[^\]]*\]=)|{|}#e"; $output = preg_replace($regex, '', $string);
-
This topic has been moved to PHP Regex. http://www.phpfreaks.com/forums/index.php?topic=356424.0
-
I recently posted in a thread here with a link to a page that provided those calculations. Was that your thread? I am definitely no expert in calculating distances using latitude and longitude, so I suggest looking for the appropriate resources. Not really a PHP question though.
-
Probably using mysql_real_escape_string() on inserted values that were first run through magic quotes.
-
As a result, would the \ be inserted into the salt value in the database as well? Fail That's the whole point of mysql_real_escape_string() - it escapes characters so they will be interpreted as literal characters in the query and not control characters (e.g. quotes around a value) Example: $value = "foo'bar"; $sql_value = mysql_real_escape_string($value); $query = "INSERT INTO `table` (`field`) VALUES ('$sql_value')"; echo $query; //Output: INSERT INTO `table` (`field`) VALUES ('foo\'bar') If that query is run the actual value inserted into the database will be foo'bar, without the backslash. It's not really any different than escaping quotemarks that are included in a quoted string echo "<input type=\"text\" name=\"email\" />";
-
The same as you would for any data that may contain content that could foul the query: mysql_real_escape_string()
-
Yes No. That's not to say you DON'T use AJAX, just start with making it work, THEN add the AJAX if you feel it is worthwhile. Adding AJAX into a process you've never done will only increase the complexity.
-
I've seen some before, but I have no clue what the titles were to search on. Try something along the line of paginated or multi page forms.