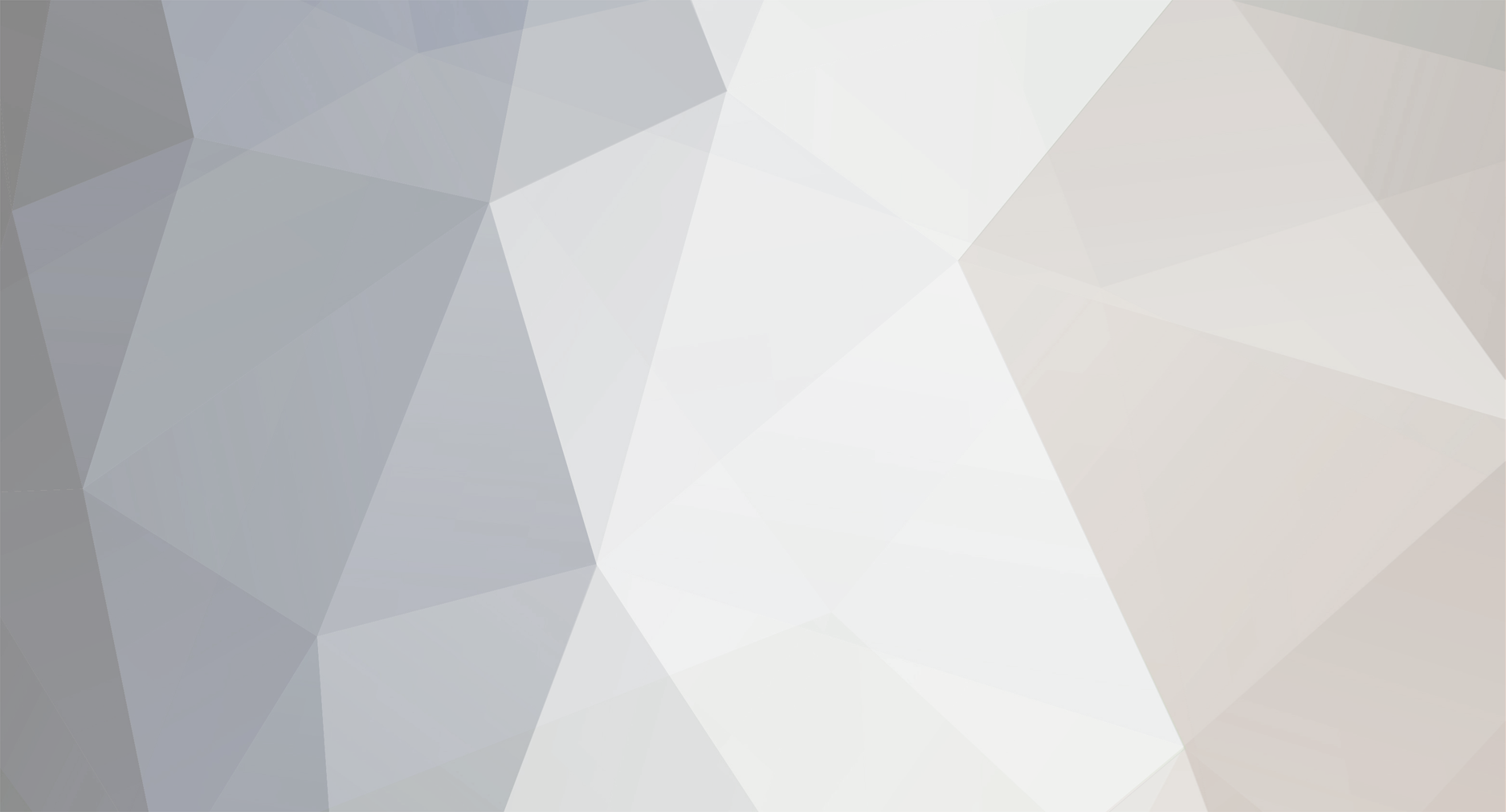
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
I have no idea. Your query is failing and you have no error handling. I'm guessing that the value of $totalpower is not what you expect or is not what you need. You should always have code in your scripts to elegantly handle errors. For a development environment, you can just use something like $result = mysql_query($query) or die("Query: {$query}<br>Error: " . mysql_error()); However, for a production environment you would never want to display the raw error to the user. In this particular situation, is $totalpower the VALUE for the user's total power? If so, you don't need a variable, just put in the field name that you want to sort by. The only reason to use a variable is if you wanted to sort by different fields.
-
Check if firstname and lastname exist before inserting records
Psycho replied to php-newbies's topic in PHP Coding Help
Well, I was suggesting Firstname+Lastname OR Phone Number. But, use whatever combinations make sense for your scenario. To implement this should be quite easy. As I said, if there are any possible matches, list them out with a button to take the user to the "update.php" page. In fact, I would create a form for each possible match with hidden fields containing the data the user has just entered. Then on the update.php page you could modify the page to check if data was posted to it from the add page. If so, run a query on the passed record id, then create the update form with the data from the database - but overwrite anything (in the form fields) with what the user entered (and passed) from the add page. Then the user just needs to click the submit button for the edit script to submit the changes. -
Check if firstname and lastname exist before inserting records
Psycho replied to php-newbies's topic in PHP Coding Help
I agree 100%. In addition to different people with the same names, what about people that use different versions of their name? For example, you might have someone that uses "Michael" one time and then "Mike" another time. You then have two records for the same person. I understand what your concern is, but it is ill advised to automatically update a user's data because you *think* it is them. Pretty dangerous. I'll offer a suggestion for an alternative though, which does run contrary to your requirements - but it is a better approach. When a user signs up do a search for any records that match ((FirstName AND LastName) OR emailAddress). You can also do phone number, or whatever makes sense for your application. If there are no matches then create a new record. If there are any matches, then provide the user some text such as: Your data appears to match one or more existing users below. If your record is listed below and you are wanting to update your data , click the Update button next to your record. If you do not have an existing record, click the Add button. Then list out each possible match providing enough detail for the user to recognize that it is their record: name, address, email, phone, etc. If the user clicks the "Update" button take them to a page to update thier data (you can even pre-poipulate the modified data that they chnaged on the previous page). Not sure what you mean by But, if you mean a different page for updating, then this will work elegantly. -
Sorting Numerically and then Alphabetically in Certain Format
Psycho replied to abney317's topic in PHP Coding Help
I was going to suggest adding a new column for the numeric value of the dataID to use for sorting purposes. That is easier. However, if you are going to give the dynamic field a reference, might as well use it in the ORDER BY clause. SELECT *, dataID * 1 AS ord FROM data ORDER BY ord, dataID -
I think he is wanting to display the records four across, then start a new row. I.e. Record 1 | Record 2 | Record 3 | Record 4 -------------------------------------------- Record 6 | Record 6 | Record 7 | Record 8 -------------------------------------------- Record 9 | Record 10 | Record 11 | Record 12 Here you go. Made the number of columns configurable using the variable $max_columns. Also cleaned up some of the logic. <?php include "config.php"; $con = mysql_connect($dbhost, $dbusr, $dbpass); if (!$con) { die('Could not connect:'. mysql_error()); } mysql_select_db($dbname, $con); $query = "SELECT * FROM venues"; $result = mysql_query($query); $output = ''; if (!$result) { $output .= "<tr><td>Query to show fields from table failed</td></tr>\n"; } elseif(mysql_num_rows($result)==0) { $output .= "<tr><td>No records returned</td></tr>\n"; } else { $max_columns = 4; $record_count = 0; while($row = mysql_fetch_assoc($result)) { $record_count++; //Start new row if 1st record or $max_columns if($record_count%$max_columns==1) { $output .= "<tr>\n"; } //Display record $output .= "<td>{$row['id']}</td>\n"; $output .= "<td>{$row['logonname']}</td>\n"; $output .= "<td>{$row['locationname']}</td>\n"; //Add additional fields as needed //Close row if last record or $max_columns if($record_count%$max_columns==0) { $output .= "</tr>\n"; } } //Close last row if still open if($record_count%$max_columns!=0) { $output .= "</tr>\n"; } } ?> <table> <?php echo $output; ?> </table>
-
This will ensure the password has at least one letter (upper or lower case) and at least 1 number. It can contain any other characters in addition to that validation. function validPassword($password) { return preg_match("#^.*(?=.*\d)(?=.*[a-z]).*$#i", $password); } I can't take full credit for this. I modified it from the examples on this page: http://nilangshah.wordpress.com/2007/06/26/password-validation-via-regular-expression/ Amazing what you can find using Google, isn't it?
-
Well, first off, are you POSITIVE that the variable $totalpower is defined as you expect it to? I would suggest NEVER creating your queries inside the mysql_query() function. Instead, create the queries as a variable - then if there are any problems you can echo the query to the page to validate it is built how you think it should. However, even if $totalpower is defined correctly, your problem is that you enclosed in in single quotes. That tells MySQL that it is a string - not a field. You can enclose it in back-quotes or none at all. Again, this probably would have been more obvious had you echo'd the query tot he page <?php $query = "SELECT `Username` FROM members ORDER BY {$totalpower}"; $result = mysql_query($query); $output = ''; $rank = 0; while($row = mysql_fetch_assoc($result)) { $no++; $output .= "<tr>\n"; $output .= "<td>{$rank }</td>"; $output .= "<td>{$row['Username']}</td>\n"; $output .= "</tr>\n"; } ?> <table border='1' cellpadding='10'> <tr> <th>Rank</th> <th>Username</th> </tr> <?php echo $output; ?> </table>"; A couple other points. 1) Only select the fields you need in our query - it is more efficient. Don't use '*' unless you need everything. Also, I would highly suggest you start learning to break the logic (i.e. PHP logic code) and the presentation (i.e. HTML code) in your scripts. I modified the code so that the logic to get the records is separated from the display of the records. It is easier to maintain code in this fashion.
-
htmlentities() and/or htmlspecialchars() http://php.net/manual/en/function.htmlentities.php http://www.php.net/manual/en/function.htmlspecialchars.php
-
Why are you using a random number? You should be using an auto-incrementing ID field in the database. Then you do not need to specify the id it will be generated for you and you will ensure that there is never a duplicate. But, on a purely academic basis, what you are asking is pretty simple. Use rande() to create an array from 1-15, then use chuffle() to randomize the array, then use array_pop() or array_shift() to get a value from the array and remove that value from the array. Then use that same function to get the next value and the next, until the array is empty. $randomIDs = range(1, 15); shuffle($randomIDs); //Call this when you need an ID $id = array_pop($randomIDs);
-
I'm not sure what the problem is, but I'm not going to go through 300+ lines of code looking for it. But, I'll give you some advice on how to find it yourself. 1. Indent your code to match the logical code blocks. It looks like you attempted to do this, but it's not quite right. For example: if($foo==$bar) { //do something //Do something else if($x==$y) { //execute something } else { //Execute another thing here } } You have code blocks that put the conditional code on the same indent as the condition while($row=mysql_fetch_array($resultj)) { $vjogador = $row['Valor'] ; } Also, why do you have all those while() loops that do nothing bug assign a value to a variable. If there should only be one record returned from the query then you don't need a while loop. If you expect many records then you are only left with the last value when the loop ends. There is no reason for you to be running all those queries. Plus, you have no error handling on your query calls. So if there is a DB error you won't know it. In any event that page is a complete mess - sorry to be blunt. I'm guessing you are new to programming and are trying to work it out as you write it. You need to take a step back and figure out the logic first (i suggest doing a rough draft on paper) then coding it. For example, I see where you are apparently doing a query to get the password based upon a user name. You then assign the result from that query to the variable $test and check if that value matches the one in the cookie. But, that logic assumes that the user name is in the database. If it doesn't then $test will not even be defined. Then the second query you have is before the test you have for the password. If that test does not pass you don't even use the results of that second query. So, that query would go after the test of the password. But, I see that all you do with the value from that query is use it is a subsequent query. So, instead of two queries, you should be using one. Instead of (modified slightly) $resultj = mysql_query("SELECT IDJogador FROM Propostas WHERE IDProposta = '$idprop' ", $db) ; $row=mysql_fetch_array($resultj) $Jogador = $row['IDJogador']; $resultjo = mysql_query("SELECT IDEquipa FROM Jogadores WHERE IDJogador = '$Jogador' ", $db) ; $fu=mysql_fetch_array($resultjo)); $eqjog = $fu['IDEquipa'] ; You should use one query such as $query = "SELECT Jogadores.IDEquipa FROM Jogadores JOIN Propostas USING (IDJogador) WHERE Propostas.IDProposta = '$idprop'"; $resultjo = mysql_query($query, $db) or die(mysql_erro()); if(!$resultjo) { echo "Could not find IDEquipa"; } else { $fu=mysql_fetch_array($resultjo)); $eqjog = $fu['IDEquipa'] ; } You are running lots of queries to get only one field of data, where you should instead be getting multiple pieces of data.
-
If this is your friends site it would be a pretty trivial task for your friend to create a web service to allow you to get the full list of categories/subcategories. But, let's say you are only doing this as an educational exercise on how to screen-scape the data from a web-page. But, as stated above you may need to obtain permission first. based on your explanation it is not clear "how" the subcategories are getting displayed. Is the menu system a javascript controlled thing and all the data is in the current page? If so, it may be easy or difficult (even impossible) to differentiate the categories/subcategories. However, if the subcategories are displayed on a page refresh after selecting a category, then you could do this using cURL. In either case you need to analyze the layout of how categories/subcategories are constructed and build the logic to decipher it. That means your code will be very "fragile" and can break any time the site owner changes content/structure.
-
I'm sorry, but I'm not sure if you are asking for the "last" value or the "highest" value. I know that in the data you presented that the last value is also the highest value, but it is easy enough to code for either the last value or the highest value - so you need to decide. You really should start looking through the manual or do some google searching as either of these solutions are the simple implementation of a single function. To get the last value: $lastValue = array_pop($matches[1]); To get the highest value: $maximumValue = max($matches[1]);
-
Just a comment, but it is not necessary to add ALL that content. Just enough for us to understand the problem. Having said that, I think you are taking the wrong approach. My understanding is that you want the decimal numbers from that content, correct? Instead of removing all the other content using preg_replace() I would instead use preg_match_all() to find all the decimal numbers in that text. preg_match_all("#\d+\.\d+#", $emailInput, $matches); You will now have an array ($matches) that contains all the decimal numbers from the email input. However, I see there are some decimal numbers in the header text at the top of that text. I *assume* you only want the decimals that are in the lines such as 20080224000000 0.48 0. If that is true, then you can get only those by adding some criteria to look for a space before the decimal number. preg_match_all("# (\d+\.\d+)#", $input, $matches); Note: this second solution will create a mulch-dimensional array that includes two sets of all matches. The first one (index 0) contains the matches with the leading space and the second (index 1) contains only the decimal matches. Just to be clear, if the input contains these lines: 20080224000000 0.48 0 20080224001500 0.477 0 20080224003000 0.477 0 20080224004500 0.475 0 The regex above would return: 0.48, 0.477, 0.477 & 0.475
-
This topic has been moved to PHP Regex. http://www.phpfreaks.com/forums/index.php?topic=344381.0
-
Well, I know I didn't test the code, so you may have needed to make some changes. But, you changes some things that shouldn't have been changed. You changed this $query = "SELECT `id`, `Name`, `Location`, `Fault` FROM orders"; To this $query = "SELECT 'id', 'Name', 'Location', 'Fault' FROM orders"; The backquotes ` where changed to normal single quotes '. The backquoates are a way to distinguish field names in your query. They are absolutely necessary unless you have fields that use reserved words, but it's not a bad habit to use them anyways. By using the strait single quotes you are telling the MySQL engine to select the literal strings. So, instead of getting the values for the fields `id`, `Name`, `Location`, and `Fault`, you are instead getting the actual text strings of 'id', 'Name', 'Location', and 'Fault'. I'm surprised you didn't see this on the page that displayes the records. That is why you query is failing on the other page. You are passing the text "id" instead of the id value from the database. Also, you used this line: echo '<p>Update query failed<br>Query: {$query}<br>Error: ' . mysql_error(); Which generated the error You are seeing the literal string of "{$query}" instead of the value associated with the variable $query. That is because you defined that string using single quotes. When using double quotes to define a string, any variables will be interpreted. In other words, you would have seen the actual query in the error message if you had used double quotes.
-
C'mon Webstyles, based on your posts I've seen I'm disappointed. I'm sure you are aware that only CHECKED checkboxes are passed in the post data, right? Therefore, the array you receive only contains the checked options. No need to loop over the array. Anyway, I'm not going to write you a solution, but I will give you two pieces of information that should allow you to get started. 1. As Webstyles suggested, create your checkboxes as arrays with the ID of the records as the values. <input type="checkbox" name="selectedReceipts[]" value="<?php echo $receiptID; ?>" > 2. On your receiving page you can use the array of checked receipts to generate a query of all the selected receipts using the IN operator. //Converts ids of selected receipts to comma separated string $receiptIDs = implode(',', $_POST['selectedReceipts']); //Create query $query = "SELECT * FROM table WHERE id IN($receiptIDs)"; Note: I left out a lot of validation that would need to be done.
-
Help with insert multiple lines from different textarea into database
Psycho replied to IreneLing's topic in PHP Coding Help
The layout of your table in the database is irrespective to the user input. The user can submit the import file with the three fields in any order. You then use the selections they made to determine which column goes where. Example //Get the user's selections for which columns are for what data $column1 = $_POST['col1']; $column2 = $_POST['col2']; $column3 = $_POST['col3']; //process the data for input into the database //Assume datafile was converted into an array by lines foreach($lines as $line) { $data = explode(',' $line); //Data now consists of an array with three value in the //order that they exist in the user's data file //But we don't know if col 1 is the Cell, First name, or last name //Put values into array $values[] = "('{$data[0}', '{$data[1]}', '{$data[2]}')"; } //So we have the data in VALUEs for DB input but we still don't know which field is what //Using the user input for the columns build the INPUT query to match the column to the appropriate field $query = "INSERT INTO table_name (`{$column1}`, `{$column2}`, `{$column3}`) VALUES " . implode(', ', $values); -
Php problem- Syntax error, unexpected t_string line 3
Psycho replied to Weakwill's topic in PHP Coding Help
the fopen() function expects at least two parameters. Those first two parameters must be string. You can either pass a string as a quoted piece of text in double or single quotes or as a variable: "foo" 'foo' $var = "foo"; In your line of code you have the first parameter using two single quote marks followed by the text lovell.txt. For the second parameter you have the letter a enclosed in two sets of single quote marks. You should either user one set of single quote marks or one set of double quote marks. Two concurrent single-quote marks is not the same as a double-quote mark $handle = fopen('lovell.txt', 'a'); Or $handle = fopen("lovell.txt", "a"); -
I have no idea what you are wanting. Give a visual example. Also, are you using two queries or one?
-
I agree that separation of functions is a good idea. I don't know that there have ever been claims that it improves performance or not, but for sheer maintainability and future development it makes things much easier.
-
Help with insert multiple lines from different textarea into database
Psycho replied to IreneLing's topic in PHP Coding Help
Please specify which field each column in your upload file is for Column 1: <select name="col1"> <option value="Cell">Cell</option> <option value="FirstName">First Name</option> <option value="LastName">Last Name</option> </select> Column 2: <select name="col2"> <option value="Cell">Cell</option> <option value="FirstName">First Name</option> <option value="LastName">Last Name</option> </select> Column 3: <select name="col3"> <option value="Cell">Cell</option> <option value="FirstName">First Name</option> <option value="LastName">Last Name</option> </select> -
I have no idea why you have a "Compare" field in your database. Here is a brief outline of how I would do a compare process: Int he pages where I list products I would provide a checkbox for each product. The checkboxes would be an array and each checkbox value would be the ID of the record. Compare <input type="checkbox" name="compare[]" value="<?php echo $recordID; ?>" /> Then when you submit the page you will have an array of all the products the user wants to compare in the POST data. You just use that to then query the database for those products. Example: $compareIDs = implode(', ', $_POST['compare']); $query = "SELECT * FROM table WHERE id IN ($compareIDs)"; Note that I left off a lot of validation that should be done, but that is the rough process.
-
The first code block is simply the building of a query. What will be more important is database normalization, use of indexes, etc. But, I do have a few comments on that one. 1. What is the purpose of "1=1" in your WHERE clause: Where 1=1 and si.Active = 1 2. In this code, there is no need to do TWO comparisons when only one will do the trick using empty() (but there's no need for that - see next comment). Also, you should almost always trim user input. Otherwise an input with just spaces can cause problems. if(!$categoryId==0 and !$categoryId==""){ $sql = $sql."And CategoryID = ".mysql_real_escape_string($categoryId); } 3. Also on that bit of code, why are you using mysql_real_escape_string() on the category ID? Just convert it to an int. Since 0 is not a valid value to use you can enforce the value to an integer (ensure it is SQL safe) and use that for the validation. $categoryID = (int) $categoryId; if($categoryID!=0){ $sql = $sql."And CategoryID = $categoryID"; } 4. Also, I'm not sure, but I think that using $sql = $sql . "new text" would be less efficient than using $sql .= "new text" As for your display code, the one thing I would change are the two lines to display the div with the image. Those two lines are EXACTLY the same except for the image. So instead of rewriting both lines, just define a variable for the difference. This will make the code much more maintainable. If you have to change the output in the future you don't have to change both lines - only one. if(!$row['Url']==NULL){ $imageFile = htmlspecialchars($row['Front']);; } else{ $imageFile = "no-img.jpg"; } echo "<div id='centered'><a href='".WEBSITE_DOMAIN."shops/".$row['ShopUrl']."/".$row['ItemUrl']."'><img alt='".htmlspecialchars($row['Name'])."' title='".htmlspecialchars($row['Name'])."' height='135' width='170' src='images/$imageFile'/></a></div>";
-
Help with insert multiple lines from different textarea into database
Psycho replied to IreneLing's topic in PHP Coding Help
If you allow the user to import a file it is up to you to define the business requirements and functionality you will provide. On one end you can be very rigid. For example, you assume that the user will be putting the data in a specific order. You then process each line doing a minimal validation that each line contains at least three pieces of data and insert that record accordingly. This approach typically makes for a quick implementation. The other extreme is giving users the ability to define headers, change what columns go where etc. For example, when the user uploads the file you could (programmatically or through user input) determine if the file has headers or not. Then display the data for the file on the screen for the user to review. You could highlight records that have potential errors, allow the user to specify which columns are for which data fields, do manual edits, etc. Then once the user is done they could finally have the imported data processed. Or you could do something in-between - it's up top you. But, if your immediate problem is ONLY that users are getting the column orders incorrect, then an easy solution is on the upload page provide three select fields: Column 1, Column 2, Column 3. For each field the user will select what field that column of data will be for (First Name, Last Name, Cell). Of course you would need to implement some validation so the user doesn't select the same field for two different columns. -
Help with insert multiple lines from different textarea into database
Psycho replied to IreneLing's topic in PHP Coding Help
That's a bad idea, IMHO, to associate data between records using line-breaks. It would be a better idea to have the user upload a csv file. But, if you really want to do that: //Process the input textareas into arrays $cells = array_map('mysql_real_escape_string', explode("\n", $_POST['cells'])); $firstNames = array_map('mysql_real_escape_string', explode("\n", $_POST['firstnames'])); $lastNames = array_map('mysql_real_escape_string', explode("\n", $_POST['lastnames'])); //Determine the values with the least amoutn of elements $min_count = min($cells, $firstNames, $lastNames); //Create array to hold INSERT values $values = array(); //Create the INSERT values for($index=0; $index<$min_count; $index++) { $values[] = "('{$cells[$index]}', '{$firstNames[$index]}', '{$lastNames[$index]}')"; } //Create the INSERT query $query = "INSERT INTO table_name (`Cell`, `FirstName`, `LastName`) VALUES " . implode(', ', $values);