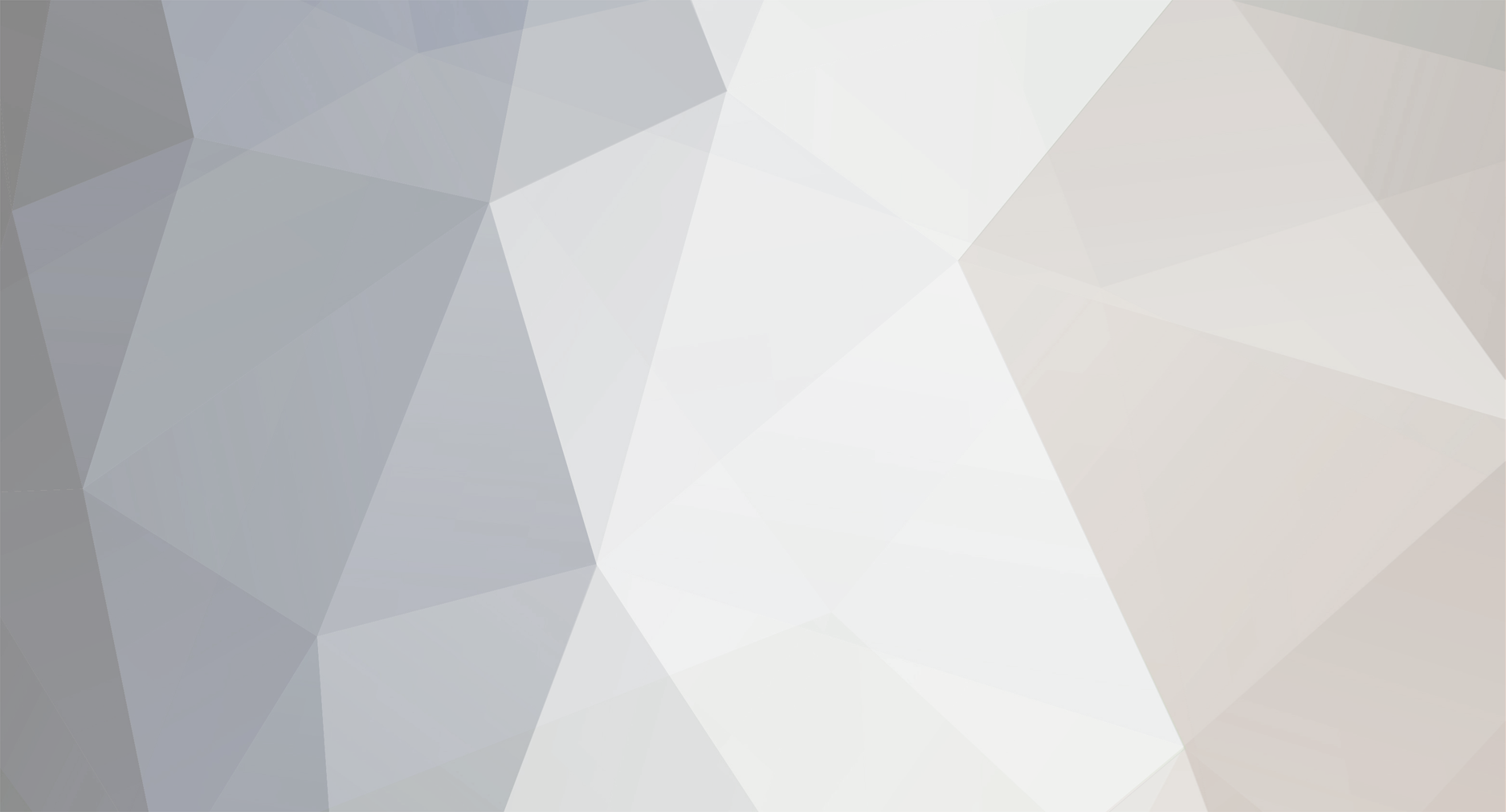
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Huh? Where in your requirements does it state that the points for friends will be dynamic based upon their points. Your requirements state that the points for friends is 20 points each. That issue aside, looking at some of the "tasks" I would assume some of them will be tracked via existing DB records (friends, votes, blogs, etc.) I would think those tasks could be programmatically determined by the tables for friends, votes, blogs, etc. For other "tasks", if they are not tracked via existing records, you would need to add a separate table (or tables) to track those tasks. Calculating the points based upon fixed points to tasks is fairly stritforward - add up the tasks and multiple by the points. But, this concept of variable points for a friend will need serious analysis. For example, if I become friends with "Bob" and my points for that friendship are supposed to be variable based upon how "valuable" Bob is, are the points fixed based upon how popular Bob is at the time the friendship is made. Or, do my points for that friendship change as Bobs "value" changes. The former would be fairly straitforward. At the time the friendship is made calculate how many points to assign and create a record for that relationship and the points awarded. If you want to go with the latter it will get fairly complex. In fact, it could lead to circular references if my points are partially determined by my friends' values and their values are determined by their points - which are in turn partially derived from their friends values, etc.
-
Help incorporating email validator with field check
Psycho replied to sphinx's topic in PHP Coding Help
No, it is not complicated - it is just structured. Trying to dump a bunch of validation into a single line is problematic. You stated you wanted to add the email validation into the one you already have. But, the error message states So, how would that message be appropriate if all the fields are filled in and the email validation fails? Plus, there are also the other problems with your code that I pointed out (e.g. not trimming the POST values). But, you are free to cut corners as you wish. if(empty($name)|| empty($visitor_email)|| empty($user_message) || !is_valid_email($visitor_email)) { $errors .= "<div id='contact_form_errorloc' class='err1'>Some of the above fields have not been filled in or the email is not valid.</div><br>"; } -
Create a foreach loop to "write out" the array values into the page in the format you want for the JavaScript array. YOU have to decide how you want the JavaScript array to be structured. JS arrays do not allowed named keys. You can use named keys - but then it is an "object" in JS and not an array - which changes how you can use it.
-
You can't "run" JavaScript via PHP. PHP is run on the server and JavaScript is run on the client. I would think you should be looking for a PHP solution to generate the results. But, if you cannot find one, then one option would be to use PHP to generate a page with ALL the records you need to process, then create a JavaScript function to process each record on the page. But, instead of trying to update each record in the database as it is processed, you could instead have the results stored in form fields on the page. Then once all the records are processed, submit the page one time and have a PHP page to update all the records simultaneously.
-
There is no way for us to really give you any good advice. There are a multitude of variables that would affect "how" you should set up your database. Besides, this is the PHP Coding forum - not the database forum. Some things that would dictate the appropriate methods: Do specific actions have specific points assigned? Are the points for the actions variable? If so, on what basis are they variable. You will need to give a complete overview of how the point system works to get any useful information.
-
It doesn't look like you need an if/else. You can simply calculate the price based upon the base plus the "overage" times the rate. the overage being the amount of the $charge_mass over the minimum. If the $charge_mass is less than the minimum, the overage would be 0. Here is the verbose solution: $data = "SELECT *, ($volume_mass/`volume`) as totalv FROM rates WHERE hub_from = 'nxd' AND hub_to = 'auk'"; $result = mysql_query($data) or die ("Error in query: $data. " . mysql_error()); while($row = mysql_fetch_object($result)) { $charge_mass = max($actual_mass, $row['totalv']); $overage = max($charge_mass - $row['min_kg'], 0); $total_cost = $row['base'] + ($overage * $row['per_kg']); echo "{$row['service']}: {$total_cost}<br>\n"; } Or you can make it more compact as follows (use the query from above): while($row = mysql_fetch_object($result)) { $total_cost = $row['base'] + (max(max($actual_mass, $row['totalv']) - $row['min_kg'], 0) * $row['per_kg']); echo "{$row['service']}: {$total_cost}<br>\n"; } Personally, I'd go with the verbose code with comments
-
Help incorporating email validator with field check
Psycho replied to sphinx's topic in PHP Coding Help
True, but unfortunately there are still plenty of hosts that have not upgraded to PHP5. -
Help incorporating email validator with field check
Psycho replied to sphinx's topic in PHP Coding Help
Well, you could incorporate it into your current "global" validation - but the message would be problematic. Also, you need to trim() the post data - otherwise a field with only spaces would pass your validation. Plus, that function is using outdated functions. I prefer to individually check each field as appropriate and display error messages for all the errors. I assume the two email fields are supposed to match - so you need to validate that as well. function is_email($email) { $formatTest = '/^[\w!#$%&\'*+\-\/=?^`{|}~]+(\.[\w!#$%&\'*+\-\/=?^`{|}~]+)*@[a-z\d]([a-z\d-]{0,62}[a-z\d])?(\.[a-z\d]([a-z\d-]{0,62}[a-z\d])?)*\.[a-z]{2,6}$/i'; $lengthTest = '/^(.{1,64})@(.{4,255})$/'; return (preg_match($formatTest, $email) && preg_match($lengthTest, $email)); } if(isset($_POST['submit'])) { //Create array to track errors $errors = array(); //Pre-process post data $name = trim($_POST['name']); $visitor_email = trim($_POST['email']); $emailconfirm = trim($_POST['emailconfirm']); $user_message = trim($_POST['message']); //Validate post data if(empty($name)) { $errors[] = "Name is required"; } if(empty($user_message)) { $errors[] = "Message is required"; } if(empty($visitor_email) || empty($emailconfirm)) { $errors[] = "Email and confirmation are required"; } elseif($emailconfirm!=$emailconfirm) { $errors[] = "Email and confirmation do not match"; } elseif(!is_email($visitor_email)) { $errors[] = "Email is not valid"; } if(count($errors)) { //Create error message $errors .= "<div id='contact_form_errorloc' class='err1'>"; $errors .= "The following errors occured:\n"; $errors .= "<ul>\n"; foreach($errors as $error) { $errors .= "<li>{$error}</li>\n"; } $errors .= "<ul>\n"; $errors .= "</div><br>"; } else { //No errors occured } } Here is the full documentation of the email validation function I provided: // NOTES: // // Format test // - Username: // - Can contain the following characters: // - Uppercase and lowercase English letters (a-z, A-Z) // - Digits 0 to 9 // - Characters _ ! # $ % & ' * + - / = ? ^ ` { | } ~ // - May contain '.' (periods), but cannot begin or end with a period // and they may not appear in succession (i.e. 2 or more in a row) // - Must be between 1 and 64 characters // - Domain: // - Can contain the following characters: 'a-z', 'A-Z', '0-9', '-' (hyphen), and '.' (period). // - There may be subdomains, separated by a period (.), but the combined domain may not // begin with a period and they not appear in succession (i.e. 2 or more in a row) // - Domain/Subdomain name parts may not begin or end with a hyphen // - Domain/Subdomain name parts must be between 1-64 characters // - TLD accepts: 'a-z' & 'A-Z' (2 to 6 characters) // // Note: the domain and tld parts must be between 4 and 255 characters total // // Length test // - Username: 1 to 64 characters // - Domain: 4 to 255 character //===================================================== // Function: is_email ( string $email ) // // Description: Finds whether the given string variable // is a properly formatted email. // // Parameters: $email the string being evaluated // // Return Values: Returns TRUE if $email is valid email // format, FALSE otherwise. //===================================================== -
Looping through array and printing HTML blocks
Psycho replied to willboudle's topic in PHP Coding Help
I don't believe so. But, it can be used to define functions differently based upon certain parameters. With OOP it would be similar to dynamically loading subclasses to extend the main class. Here is a ROUGH example of how you could use it (not that this is a good example) function createSayThankYou($language) { switch($language) { case 'sp': function sayThankYou() { echo "gracias."; } break; case 'ge': function sayThankYou() { echo "danke."; } break; case 'en': default: function sayThankYou() { echo "thank you."; } break; } } //Dynamically create the function to say thank you createSayThankYou($users_language); sayThankYou(); //Will output thank you based on function created above -
You should check for DB errors and provide a means to see the errors. In a dev environment you can just do this: $result = mysql_query($command) or die(mysql_error()); I don't see anything wrong with the syntax of your query. Although you don't need the semi-colon at the end of the query, but I don't think that will cause an error. Could be you have a typo in the field names. Runt he above to see what the actual error is.
-
Looping through array and printing HTML blocks
Psycho replied to willboudle's topic in PHP Coding Help
Yes. Absolutely. Of course, they would only have scope within the function that defines them - just like variables. EDIT: I was wrong. You can define a function within a function and it will have global scope. BUT - you must call the function that defines the "sub" function before it becomes available. -
Looping through array and printing HTML blocks
Psycho replied to willboudle's topic in PHP Coding Help
Well, your code is a bit confusing as to what you are really trying to accomplish. Plus, you are putting functions within functions. There is typically no reason to do that. It definitely makes no sense in your scenario. In fact, you are defining the products within a function and not doing anything with them IN that function. I'll give you an example script which I think is close to what you want and you can modify it as needed <?php //Put the array is a separate file and include it here. //Makes management much easier $products = array ( '101' => array( 'name' => 'RIDDICK /TSHIRT', 'price' => '25.95', 'sizes' => array('S', 'M', 'L', '1X', '2X', '3X', '4X') ), '102' => array( 'name' => 'MAFIA / TSHIRT', 'price' => '15.95', 'sizes' => array('S', 'M', 'L', '1X', '2X', '3X', '4X') ) ); //Only need to specify band name. You can programatically create //all uppercase or all lower case as needed $band_name = "Apocalypse"; $sku = "FGD"; function createProductForm($bandname, $sku, $productArray) { $BANDCAPS = strtoupper($bandname); $BANDLOWER = strtolower($bandname); foreach($productArray as $skunum => $prodInfo) { $prodID = $sku.$skunum; $sizeOptions = ''; foreach($prodInfo['sizes'] as $size) { $sizeOptions .= "<option value='{$prodID}{$size}'>{$size}</option>\n"; } echo "<!-- PRODUCT BEGIN -->\n"; echo "<li class='product'>\n"; echo "<a href='product_files/large/{$prodID}.jpg' rel='lightbox'><img src='product_files/{$prodID}.png' alt='{$bandname} Merch T-shirts'></a>\n"; echo "<br>\n"; echo "<strong>{$prodInfo['name']}</strong><br>({$prodID})<br>\${$prodInfo['price']}<br>\n"; echo "<form name='{$prodID}' method='GET' target='_blank' action='http://www.jsrdirect.com/cgi-bin/Make-a-Store.cgi'>\n"; echo "<input type='hidden' name='band' value='{$BANDCAPS}'>\n"; echo "<input type='hidden' name='back' value='http://www.jsrdirect.com/bands/{$BANDLOWER}/index.html'>\n"; echo "<strong>Qty:</strong>\n"; echo "<input type='text' name='quantity' size='1' value='1'>\n"; echo "<strong>Size:</strong> \n"; echo "<select name='item'>\n"; echo $sizeOptions; echo "</select>\n"; echo "<br><br>\n"; echo "<input class='css3button' value='Add To Cart' type='image'>\n"; echo "</form>\n"; echo "</li>\n"; echo "<!-- Product End -->\n"; } } ?> <html> <head></head> <body> <h1>Order Form</h1> <?php createProductForm($band_name, $sku, $products); ?> </body> </html> -
There is no need to post your entire script - only the part that is relevant to the issue at hand. You should be able to determine what that is based upon what you have written and what your issue is. Posting the entire thing makes it difficult for those of us having to pick through the entire thing trying to figure out what's going on. After hunting down the query in your code, the problem is obvious. By the way, I would highly suggest writing your queries in a structured format for easy reading - it makes debugging these types of errors much easier. Here is your query in a formatted fashion: (SELECT postcode FROM freelistings) UNION (SELECT postcode FROM basicpackage) UNION (SELECT postcode FROM premiumuser WHERE postcode LIKE '%$trimmed%') ORDER BY postcode Look at the WHERE clause and see if you can tell how that WHERE clause would be applied. Do you see the problem? Hint: each SELECT statement is interpreted individually. Well, I would like to point out that I have NEVER actually used a UNION in a query. However, I found the solution to your problem in less than a minute by Googling "Mysql Union". I skipped the first result, which was the MySQL manual as it is not the most strait-forward documentation, and instead clicked the second link which was a tutorial for MySQL UNION. Although it didn't show an example with a WHERE clause the tutorial was illustrative enough that I could see how they would be implemented. A good programmer is not one who has memorized every function and how it works. A good programmer is one that can (among other things) easily find the functions and how to implement them. EDIT: Actually, I just looked at the MySQL manual for UNION and the very first example query showed exactly how you would utilize UNION with WHERE clauses. So, you came here asking a question on how to accomplish something and I gave you the answer - use UNION. You then expected me to write code for you instead of you taking a few minutes out of your day to learn how to use UNION. Again, I have never used UNION myself - I only knew the general concept of what it can accomplish - which is what I provided to you in my first response. So, we both had the exact same information but I was able to find the solution by doing a little work. The only reason I did respond to your last post was that you at least made an effort. That is all I expect from someone asking for my FREE help - that you show some effort.
-
The script is generating an error if you have no values - or if you only enter invalid values - because there is no validation before the foreach statement that the array still has values. Just do an if/else statement right before the foreach() statement such as if(!count($links)) { //Display error message that no valid values were entered } else { //Perform the processing of the $links } As to your second question. If you are going to have "sets" of fields to be processed together you will want to link the fields to gether. Some people would just tell you that you can associate the first value (0 index) from $_POST['links'] with the first value from $_POST['names'], etc. But, I would advise against it. If you were to ever add checkboxes that will no longer work because only checked checkboxes are passed in post data. Also, I think it just makes it less problematic if you have a more definitive way to link the fields. In this case I would add an index to the form field names. This will be easy using the previous code I provided above. Example: $link_count = 10; for($link_index=1; $link_index<$link_count; $link_index++) { $link_no = $link_index+1 echo "<tr> <td width='140' style='padding:4px;'>link {$link_no}:</td> <td >URL: <input type='text' class='edit bk' name='links[{$link_index}]' style='width:350px;'></td> <td >Name: <input type='text' class='edit bk' name='names[{$link_index}]' style='width:350px;'></td> </tr>\n"; } Now, you can process the sets of fields using something like foreach($_POST['links'] as $index => $link_url) { $link_name = $_POST['names'][$index]; } With this approach you would want to move the validations inside the loop since you need to validate the "sets" of records as a whole.
-
In my opinion, the structure of your page really needs a makeover. You have a class that does nothing but a simple calculation. You then have a lot of error handling outside the class. If that is all your class is going to do, I wouldn't even use a class. But, you could move the validations into the class to make it more useful. Also, your error handling is not very elegant since it uses exit(). Instead you should always display a complete page. Also, your class defines two properties for the class - but never uses them. The variables you use in the add() method are not the same as the properties you defined for the class. I have made some major modifications below. I think it is all correct - but since the code has no comments and the output is not english I'm not entirely sure. <?php class calc { var $number1 = ''; var $number2 = ''; var $errors = array(); var $response = ''; function add($value1, $value2) { $this->set_number1($value1); $this->set_number2($value2); if(count($this->errors)) { $this->response = "The following errors occured:\n"; $this->response .= "<ul>\n"; foreach($this->errors as $error) { $this->response .= "<li>{$error}</li>\n"; } $this->response .= "</ul>\n"; return false; } else { $result = $this->number1 - ($this->number2*0.98); $this->response ="Du kommer til med ad spare $result dkk<br>"; return true; } } function set_number1($value) { $this->number1 = trim($value); if(empty($this->number1)) { $this->errors[] = "Du skal tast ind nuværende måndlig udgift!"; } elseif(!ctype_digit($this->number1)) { $this->errors[] = "De skal være nummer!"; } return; } function set_number2($value) { $this->number2 = trim($value); if(empty($this->number2)) { $this->errors[] = "Du skal tast ind Forbrug p/md.!"; } elseif(!ctype_digit($this->number2)) { $this->errors[] = "De skal være nummer!"; } elseif($this->number2>299) { $this->errors[] = "Månedlig forbrug skal være 300 eller mere"; } return; } } $calc = new calc(); if(isset($_POST['value1']) && isset($_POST['value2'])) { $calc->add(($_POST['value1'], $_POST['value2']); } ?> <html> <head> <title>PHP Calculator v1</title> </head> <body> <form name="calc" action="" method="post"> Nuværende månedlig udgift: <input type=text name="value1" value="<?php echo $calc->number1; ?>"><br> Forbrug af kopper/md.: <input type=text name="value2" value="<?php echo $calc->number2; ?>"><br> <input type=submit value="Calculate"><br><br> <?php echo $calc->response; ?> </form> </body> </html>
-
Looping through array and printing HTML blocks
Psycho replied to willboudle's topic in PHP Coding Help
If you have a variable declared outside a function that you need to use inside a function, then pass that variable as one of the parameters for the function! Although, I would suggest changing the format of your array. Creating different subsets for sku's, name, sizes, etc. as you have them make it difficult to work with. Especially if you need to manually edit them. I would suggest an array where the sku number id the index and the value is an array of the parameters for that sku item. '101' => array( 'name' => 'RIDDICK /TSHIRT', 'price' => '15.95', 'sizes' => array('S', 'M', 'L', '1X', '2X', '3X', '4X') ), '102' => array( 'name' => 'MAFIA / TSHIRT', 'price' => '15.95', 'sizes' => array('S', 'M', 'L', '1X', '2X', '3X', '4X') ), -
Yes, it is. It is to get help on code you have written. If you had provided enough information for me to work with I might have been able to try and throw something together. But, I don't know what fields you need, the field types, etc. etc. As I stated above, there are some specific requirements for using UNION. It probably would have taken several posts back and forth for me to get the information I need to create the query for you - and you wouldn't have really learned much in the process. However, if you were to read a tutorial on how to use UNION and figured out how to implement it yourself you would learn a great deal. So, give it a try. If you have problems then post what you have and the problems you are encountering.
-
This topic has been moved to Miscellaneous. http://www.phpfreaks.com/forums/index.php?topic=345120.0
-
It's certainly not a PHP question. This forum is for people looking for help on PHP code they have written. Moving topic to an appropriate forum.
-
Aww, I just wrote out a post to point you in the right direction. I knew what the issue was as soon as I saw the page with the array displayed. As for Just to clarify, it wasn't that the date format in PHP was different - it was that you built it to be different. But, I'm happy to see you actually worked on the problem and found the solution yourself. Far too many people come here without putting in an ounce of effort. EDIT: By the way, echoing the array to the page was a good debugging step. The next step should have been to look to the if() and foreach() statements that echo's the events to the page. if(isset($events[$event_day])) { foreach($events[$event_day] as $event) { I would have done something as follows if(isset($events[$event_day])) { #### DEBUG LINE #### $calendar.= "events[$event_day] IS set. Count: " . count($events[$event_day]) . "<br>\n"; foreach($events[$event_day] as $event) { if($event['category_id'] !=2){ $calendar.= '<div class="events">♦ <a href="' . $event['ShoWareEventLink'] . '">'.$event['start_time'].' - '.$event['EventTitle'].'</a></div>'; //^this is where we put the performance info } } } else { #### DEBUG LINE #### $calendar.= "events[$event_day] NOT set<br>\n"; $calendar.= str_repeat('<p> </p>',2); //^this is where we have blanks if an event_day <> list_day }
-
$result = <<<EOD <font face="Arial" size=2> <p>Member Name</font> <font face="Arial" size=2>no2</font> <p> <font face="Arial" size=2>My name is 'Kate' and im fine.</font> <p> <font face="Arial" size=2>/member.php</font><font face="Arial" size=2> today</font> EOD; $pattern = "/My name is '([^']*)'/i"; preg_match($pattern , $result, $matches); print_r($matches); Output: Array ( [0] => My name is 'Kate' [1] => Kate )
-
This topic has been moved to PHP Regex. http://www.phpfreaks.com/forums/index.php?topic=345218.0
-
Work's fine for me when I copied that text into a variable called $result. However, that expression could be simplified to $pattern = "/My name is '([^']*)'/i";
-
What do you mean they are not in the same order? In the order they are listed with the other fields in the table? It won't matter as long as you select the same number of fields from the tables and the field types match. As I already stated above. You should be SELECTing the actual fields you want to query. Using '*' is a waste since it returns all the fields from the associated tables. If you only need certain fields, then you shoudl be listing them in your query SELECT field1, field2, field3 FROM sometable You have a computer and you have access to the internet. Did you even do one search to look for information on using UNION? There are many pages available out there that will do a superior job of explaining how to use UNION than I can do within the confines of a forum post. It's not that I don't want to be helpful, but you should at least put forth some effort to solving your problem instead of expecting others to do it for you.
-
What makes you think those are PDFs? Looks like the online content is made up of images to me.