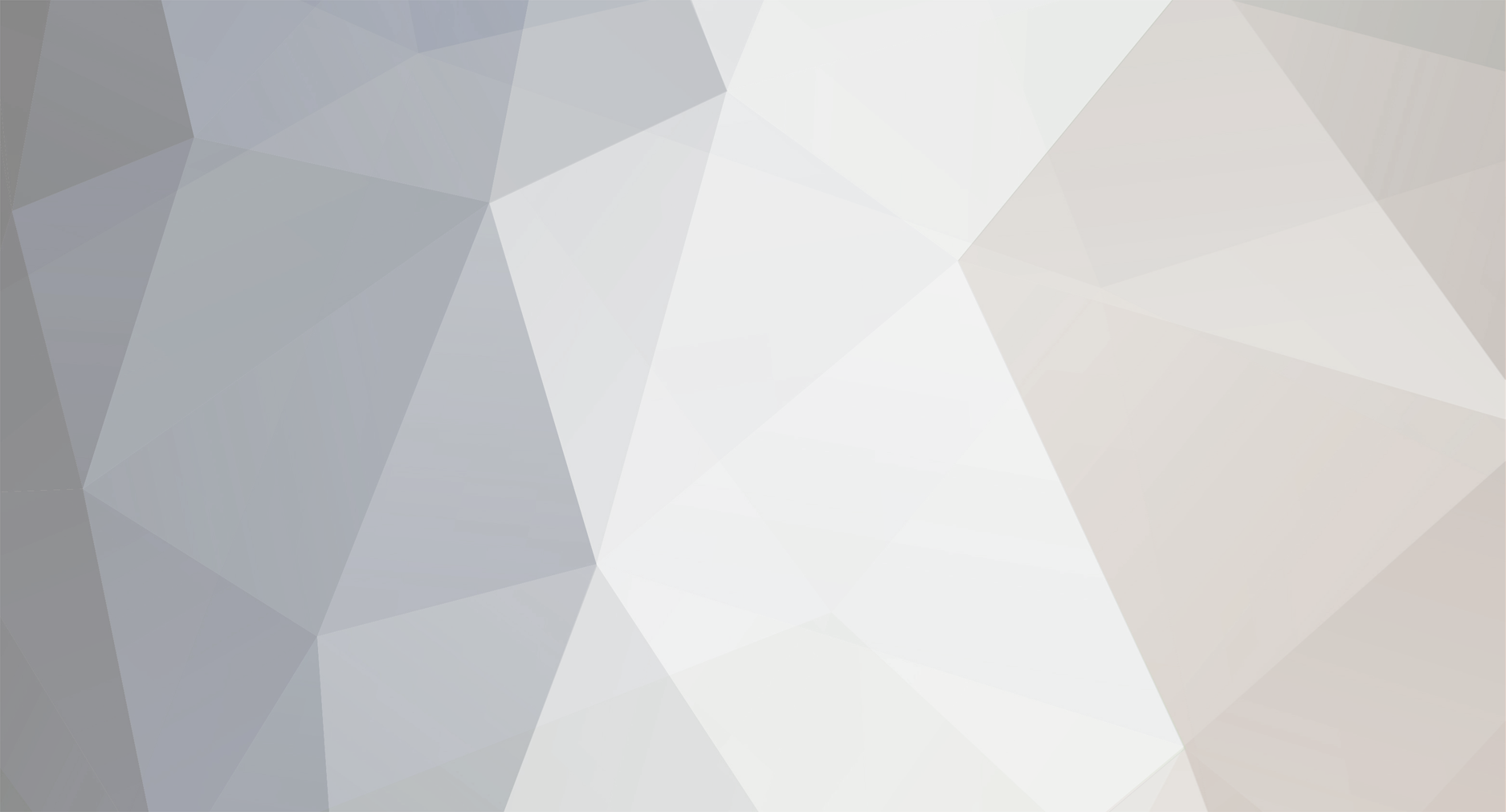
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
As AbraCadaver stated a checkbox is either checked or not and it only has one value. But, if the fact the checkbox is supposed represent multiple selections, then you could handle that on the server-side completely. For example, let's say you have the following checkboxes Pick a Color: <input type="checkbox" name="all_colors" value="all"> All <input type="checkbox" name="colors[]" value="blue"> Blue <input type="checkbox" name="colors[]" value="red"> Red <input type="checkbox" name="colors[]" value="yellow"> Yellow <input type="checkbox" name="colors[]" value="green"> Green You could do something like this if(isset($_POST['all_colors'])) { $user_colors = array('blue', 'red', 'yellow', 'green'); } else { $user_colors = isset($_POST['all_colors']) ? $_POST['all_colors'] : array(); } Not exactly the most elegant solution, but it could improved based upon your specific need.
-
Of course, once you make the genre an option you lose the visual "grouping". But, you can use styles to simulate the same effect. But, I can't validate that the stylings will look the same from browser to browser. You'd have to test for yourself. This will give you a "similar" look without using the OPTGROUP tag (at least in FF). Define the following classes in your style sheet .genreOption { font-weight: bold; font-style: italic;} .subgenreOption { margin-left: 10px; } Then change the code above to instead apply different styles based upon the value type foreach($genre_list as $genre => $subgenre_list) { echo "<option class='genreOption' value='{$genre}'>{$genre}</option>\n"; foreach($subgenre_list as $subgenre) { echo "<option class='subgenreOption' value='{$genre}-{$subgenre}'>{$subgenre}</option>\n"; } } NOTE: The style are only applied when you view the values with the list expanded. Once a value is selected and the select list closes it is displayed 'normally'
-
PHP question about using dates with if statements
Psycho replied to jdurden's topic in PHP Coding Help
OK, looking at your code a little closer I see there is no date returned only $thumbnail["thumb"], which I assume to be the path to the thumbnail. In that case you could define variables for this month and next month, then get the value for the file using filemtime() and do your comparison. Also, you say you are doing this for multiple thumbnail images. So, you must be running some of this code in a loop - which you didn't provide. Just make sure you put anything outside the loop that doesn't need to be in the loop. For example all the variable assignments I would assume should be outside the loop. ### Outside the loop ### $width = 940; $height = 277; $classtext = ''; $titletext = get_the_title(); $date = print(Date("l F d, Y")); //Defne this and next month $thisMonth = date('Y-m'); $nextMonth = date('Y-m', strtotime('next month', strtotime("($thisMonth)-1"))); ### Inside the loop ### $thumbnail = get_thumbnail($width, $height, $classtext, $titletext, $titletext, false); $thumb = $thumbnail["thumb"]; //Get Year-Month of the thumbnail file $thumbMonth = date('Y-m', filemtime($thumbnail["thumb"])); if($thumb != '' && ($thumbMonth == $thisMonth || $thumbMonth == $nextMonth) ) { -
PHP question about using dates with if statements
Psycho replied to jdurden's topic in PHP Coding Help
Well, what format is the date returned from your function get_thumbnail()? Is it s UNIX timestamp a string format (if so, what format is it YYYY-MM-DD, MM-DD-YYYY, MonthName DD, YYYY, or what)? -
One clarification to what WebStyles stated. The mysql_fecth_assoc() reads one record from the result set of the query that was performed - it is not reading the data from the database table. There's an important distinction there. Although you can think of the result set as a temporary table created from the query. Then, as he stated, that function will get the 'next' record from the result set and returns the values of that record an an array. So, when you use somethign like $row = mysql_fetch_assoc($result) The variable $result will be an array of the values from the record returned by the function. That type of line is typically run in a while loop such as while ($query = mysql_fecth_assoc($result)) { so it will get the next record, perform some actions on that record, and repeat (i.e. get the next record). So, the loop will continue as long as the result of $row = mysql_fetch_assoc($result) is not false - as long as there are records being returned. As soo, as you have reached the last record, that condition will return false and the loop ends. So, basically, that while loop is an efficient means of looping through all the records in a query result set. And, as stated above, your variable names are messed up. The code should look something like this (added some error handling) //Define the query to run as the variable $query $query = 'SELECT `Name`, `Username` FROM `users`'; //Executre the query and assign the result set to the variable $result $result = mysql_query($query); if(!$result) { echo "The query failed so a FALSE value was assigned to $result<br>"; echo "The error was " . mysql_error(); } elseif(mysql_num_rows($result)==0) { echo "There were no rows (i.e. records) in the result set"; } else { //Records were returned, let's process them echo "Here are the results of the query:<br>\n"; while ($row = mysql_fecth_assoc($result)) { //Get values from the row/record based on the field name as the array index //And assign to variable to be used in the processign code $name = $row['Name']; $username = $row['Username']; //Output the results echo "Name: {$name}, Username: {$username}<br>\n"; } }
-
Another option is to build an array in a more logical format using the category/group name as the index and the genre's as sub-arrays. This is much more logical IMHO $genre_list = array ( "ACTION" => array("First Person Shooter", "Third Person Shooter", "Tactical Shooter", "Fighting", "Arcade"), "ADVENTURE" => array("Adventure", "Platformer", "Point and Click") ) echo "<select id='genres' name='genres'>\n"; foreach($genre_list as $genre => $subgenre_list) { echo "<optgroup label='{$genre}'>\n"; foreach($subgenre_list as $subgenre) { echo "<option value='{$genre}-{$subgenre}'>{$subgenre}</option>\n"; } } echo "</select>\n";
-
where do i start in creating a sitemap for my shopping cart?
Psycho replied to jasonc's topic in PHP Coding Help
OK, so you don't want a sitemap for the shopping cart you want a sitemap for your products. But, then again, it doesn't sound like that is what you really want either. It sounds as if you want a page of all your products (via their dynamic links) such that search engines can index your products. If you are specifically interested in just Google then look into the information they provide for product searching: http://www.google.com/intl/en_us/products/submit.html http://www.google.com/support/mini/bin/answer.py?hl=en&answer=15745 Although here is a more generic reference regarding search engine optimization for variable content sites: http://www.seo-consultant-services.co.uk/static-html-vs-dynamic-urls.html -
Do not run queries in loops - it is horribly inefficient. You should run one query with a JOIN to get all the data. Also, don't use * in your SELECT statement unless you absolutely need all the fields. Just list out the fields you need. Lastly, I would recommend not giving fields in different tables the same name (unless one is a foreign key of the other). It makes it more difficult when you do need to use JOINs. Anyway, the problem you are having is that when you process each "network" you are appending new records to the $games array and then assigning that value to the $network array. So, on the second network, you are adding those new games to the $games array (which still holds the records from the first network). Anyway, you are doing this wrong as it is and should be using a single query with a JOIN statement. Thsi may have a typo or two, but should work as you need it $query = "SELECT network.id as n_id, network.title as n__title, games.id as g_id, games.title as g_title FROM ".DB_PREFIX."roster_networks AS network JOIN ".DB_PREFIX."roster_games AS games ON games.networkID = network.id ORDER BY network.title, games.title"; $result = mysql_query($query) or die(mysql_error()); $networks = array(); //Create the variable with default value while($row = mysql_fetch_assoc($result)) { if(!isset($networks[$row['n_id']])) { //Network ID is different than last record $network_id = $row['n_id']; $networks[$network_id] = array(); $networks[$network_id]['title'] = $row['n_title']; $networks[$network_id]['games'] = array(); } //Add game data to current network index $game_id = $row['g_id']; $networks[$network_id]['games'][$game_id] = array( 'id' => $game_id, 'title' => $row['g_title'], 'icon' => '', 'record' => '', 'members' => '', 'status' => '' ); }
-
Well, I already stated that I did not understand your question as asked. The fact that no one else has responded probably means no one else understood it either. Why don't you try doing what I asked, give some examples of the input you wouold have and the expected output.
-
This topic has been moved to Miscellaneous. http://www.phpfreaks.com/forums/index.php?topic=345678.0
-
where do i start in creating a sitemap for my shopping cart?
Psycho replied to jasonc's topic in PHP Coding Help
Well, no the format does not need to be XML. But, what are you really asking? A "shopping cart" is a group of products that a user has selected for purchase. You need a site-map for their cart? -
What - specifically - are you having trouble understanding? Email is, in my opinion, can be one of the more complex tasks IF you need to make it complex. But, for a simple plain-text email the description and examples in the manual are easy enough to follow. I don't know that anyone could provide a more thorough explanation in a forum post. I assume you understand the first three parameters (which are required): $to , $subject , $message. The manual gives a good explanation of how the data in those parameters should be formatted. The last two parameters ($additional_headers and $additional_parameters) are probably where your confusion is. The first one shuld typically be considered required because you must set a "from" address. If it is not set in the php.ini file, then you must set it is this parameter. The manual also explains some other headers that can be set: CC, BCC, Reply-To, etc. But, there are numerous headers and parameters that can be set. To be honest I have never found a definitive list of what options can be set. That is probably because those values are not dictated by PHP but by the original classifications for email communications. In fact, I would guess that some parameters may be valid for some email servers and not others.
-
Here is what I would use to define those three variables that should make the logic much easier //Create function to get value from GET or POST function getVarFromPostOrURL($var_name, $default=false) { //Reverse if and elseif conditions based upon //whether POST or GET should take precedence $value = ''; if(isset($_POST[$var_name])) { $value = trim($_POST[$var_name]); } elseif($_GET[$var_name])) { $value = trim($_GET[$var_name]); } return (!empty($value)) ? $value : $default; } $user = getVarFromPostOrURL('user'); $fnt = getVarFromPostOrURL('fnt', 'arial'); $img = getVarFromPostOrURL('img', 'blue'); if(!$user) { exit('No user data available!'); }
-
Your logic really needs some cleaning up. You have this block which will set the default font if the font parameter is passed through the GET data. if(isset($_GET['fnt'])) { if(!empty($_GET['fnt'])) { $fnt = $_GET['fnt']; } else{$fnt='arial';} } Then you have this block of code which defined font based upon POST data if(isset($_POST['user'],$_POST['img'],$_POST['fnt'])) { if(!empty($_POST['user'])) { $user=$_POST['user']; } if(!empty($_POST['img'])) { $img = $_POST['img']; } if(!empty($_POST['fnt'])) { $fnt=$_POST['fnt']; } } So, if the font variable is passed through POST data - but is empty - $fnt never gets defined. So, I have to assume that it is only passed through POST data (or perhaps either)? Why don't you just define the default when you first define $fnt at the top of the page? $user = "arial"; $img = ""; $fnt = ""; In fact, you could do the same for the $img which seems it should have a default of "blue"
-
This topic has been moved to PHP Regex. http://www.phpfreaks.com/forums/index.php?topic=345658.0
-
The "rules" as you have stated them are not specific enough to provide anything. Can you please provide a couple examples of the inputs and the expected outputs?
-
OK, I went back and read some of your comments and think I have a much simpler solution for you. You state: So, a week starts on a Sunday and ends on a Saturday and the same is true for your month periods. So, there is no need to determine the range of the week and test against the range of the months. You only need to test the end of the previous week to see what month it falls into (or you could just test the beginning of the previous week). But, if you need to get the start and end of the previous week you should use something like this $prev_week_end = date('Y-m-d', strtotime('last Saturday')); $prev_week_start = date('Y-m-d', strtotime('last Sunday', $prev_week_end)); Note how I reused the variable $prev_week_end to calculate $prev_week_start instead of using nested strtotime() and having to recalculate. Anyway, with just the $prev_week_end variable you can determine which month to apply. Based upon your previous statement, the Saturday following the last Friday in a month is the end of that month's period. So, logically, the Friday's are always in the actual month that the month period applies to. It is only with some Saturday's where the Saturday is actually in the month after the one it is supposed to apply to. To make a long story short you can determine the applicable month to use for the report by checking what month of the Friday in the previous week. $prev_week_end = date('Y-m-d', strtotime('last Saturday')); $prev_week_start = date('Y-m-d', strtotime('last Sunday', $prev_week_end)); $prev_week_friday = date('-1 day', $prev_week_end); $report_month = date('m', $prev_week_friday); $report_year = date('Y', $prev_week_friday); All that other code you had would be unnecessary. This is based upon what I understood from your previous statements. If it is not correct, please elaborate.
-
OK, even though the strtotime() function isn't working for me with those strings, looking at how your data values are being calculated I was able to determine that your variables should be getting the following values. $sunday = '2011-10-02'; $saturday = '2011-10-08'; $firstday = '2011-08-28'; $lastday = '2011-10-01'; $thisfirstday = '2011-10-02'; $thislastday = '2011-10-30'; Then analyzing your if/else conditions with those values, the third one would be the correct result. Which is what you got. However, I will reiterate that your logic for determining those dates is over complicated. I think you can make the entire script (determining the dates and the if/else statements) much, much simpler. Please provide and explanation of what you are trying to achieve and I'll be happy to give it a try.
-
With the data you have posted I can only validate that the first if() condition should resolve to false, and it is since you are getting the results from the third condition. Need the values for the other variables used to see why the third condition is resulting in true. Need values for $saturday, $thisfirstday, and $thislastday.
-
Well, I'm on 5.1.6 and those strtotime() values don't work for me. I don't know that there have been updates to the parsing for strtotime() or not in those smaller updates. But, unless your production server is on the same version as you, using that would likely not work. But, as I stated previously you are over complicating things. Here is one simpler solution that would get you the Saturday immediately following the last Friday of the previous month $sat_following_last_friday_of_last_month =strtotime(date('Y-m').'-2 last Saturday'); It basically starts on the 2nd of the current month and looks for the previous (i.e. last) Saturday. EDIT: Could you please post the values for all the variables you are using in your if/else statements, and what results you are getting for $month and $year?
-
Hmm. . . I ran your exact code and got the results above. $firstday and $lastday are set in 1969! You are saying you are getting different results? Some simple testing found that "first day of last month" is not a valid string to use with strtotime() (at least in my PHP install). What version are you using? As I stated, your nested strtotime() functions seem overly complicated for determining the days. Is the Saturday following the last Friday of the previous month the only day you really need?
-
If that script is working fine on many pages and just not one, then obviously there is something different happening on that one page. Plus, if you are using that same code on multiple pages you should create a function and include that function on the multiple pages!!! That way you don't have to maintain multiple copies of the same code. But, I think I see your problem. You have a foreach loop to apparently try and access the multiple files: foreach($slike as $slika){ $image =$_FILES[$slika]["name"]; $uploadedfile = $_FILES[$slika]['tmp_name']; But, look at the $slike array $slike = array(file, file1, file2, file3, file4); Those are not the strings of 'file', 'file1', 'file2', etc. Since you did not enclose the values in quote marks the PHP parser will interpret those as constants. Since I assume you didn't define those constants the parser is probably using a null value. (Although I'm surprised the first one, 'file', is not producing an error, because that is a function name). Your array should probably look like this $slike = array('file', 'file1', 'file2', 'file3', 'file4'); But, really, that code is horribly inefficient. I'm surprised that you've been using this code for years and haven't made it into a function to make it reusable.
-
Using your example above, here is what the data would look like in a valid CSV format name1,subj1,abcd , ,efgh ,subje2,test1 ,,test2 Here is some sample code that would get all the "valid" values from that file $csv_file = "data.csv"; $handle = fopen($csv_file, "r"); if ($handle === FALSE) { //Unable to read file echo "Unable to open file"; } else { //Create result array to put valid values $results = array(); //Process the data line by line while (($data = fgetcsv($handle, 1000, ",")) !== FALSE) { //Trim the values and remove empty $data = array_filter(array_map('trim', $data)); //Add to result array $results = array_merge($results, $data); } //Close the file handler fclose($handle); } //The result array now holds just the valid values foreach($results as $value) { echo "$value<br>\n"; } Output name1 subj1 abcd efgh subje2 test1 test2
-
The data you showed in your first post is not the data from a CSV file - it looks more like tabular/positional data. Either that, or you modified the data for your post. It is hard to help you when you are not showing the actual data you are working with. Also, since you are using a custom class to read the data it is quite possible the problem is with that code. But, if you have problems such as this, the first step is to validate the data - which is one debugging strategy. Are you getting any errors with your code? Did you validate that an array was returned? Etc., etc.