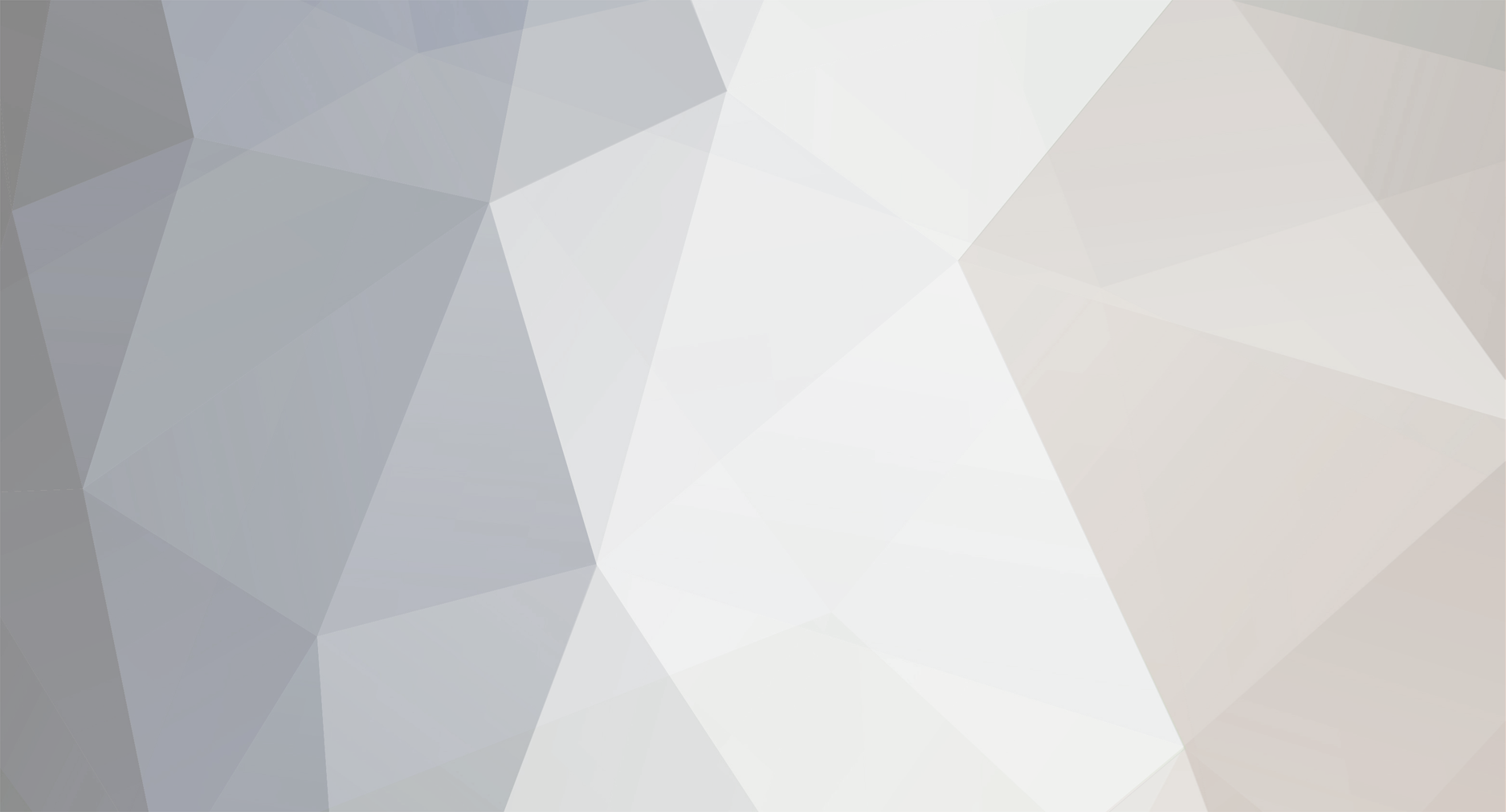
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
These are lines 25 - 27 <?php include(`includes/header.php); ?> <?php include(includes/nav.php); ?> The first include is using a "back tick" before the file name and is missing a quote at the end of the file name and the second include is missing both quotes around the file name. You need to enclose the file names in single or double quotes ( ' or "). The division by zero is probably because the incorrect or missing quotes is causing it to try an interpret the '/' as a division. Try: <?php include('includes/header.php'); ?> <?php include('includes/nav.php'); ?>
-
That loop will continue indefinitely! You need to run the query ONE TIME and then do a while loop over the RESULTS. You are currently running a new query on each iteration of the loop and only getting the first result. if($_GET['place']==addnetwork) { //Create/execute query $query = "SELECT name, url FROM s_links"; $result = mysql_query($query) or die("Error running query: " . mysql_error()); //display current networks echo "<table border=1>\n"; echo "<tr><th>Network Name</th><th>URL</th></tr>\n"; //Loop through results while ($row = mysql_fetch_assoc$result()) { echo "<tr><td>{$row['name']}</td><td>{$row['url']}</td></tr>\n"; } echo "</table>\n"; }
-
If the value is a boolean (i.e. True/False) a checkbox is the appropriate input. The first solution I showed above is very simple to implement when you want to allow the user to ONLY update that one field for multiple records. And, if they are editing multiple fields on a single record, then you just set the value to true/false based upon whether the checkbox field isset() in the post data.
-
Yes, you *should* say thanks. You came here asking for assistance and I provided it, along with some constructive criticism. I'm sorry if the manner in which I provided that criticism damaged your fragile sensibilities. Yes, I have not always written code as I do now, but I always strived to write code that was logical and adhered to some sort of comprehensible format. Sorry, but unless someone started writing code yesterday I don't buy the "I'm new to programming" defense. You don't have to be a good programmer to write clean code. But, it is just criticism, do with it what you will. And, I could really give a rats ass if you thank me or not. I don't come here and invest my time and energy to get validation of my self worth. I do it because I enjoy helping people. Running queries in loops is incredibly inefficient. It uses up a lot of CPU/Memory resources and as your application and/or user base grows will create performance issues. True, you probably won't have any significant issues with this particular implementation if you used a loop, but why build it the wrong way when 1) The right way is much simpler and 2) Doing it the wrong way instills bad practices that would then get implemented in functionality where is would be a problem. If it were me, I would have one page where a user can edit the "Active" status for all records, but not allow editing of the Name or PDF (on that page). I would make the user click a link/button to go to a page to edit that information individually for each record (in addition to the active status if they wish). That's just a preference of mine, but there are technical/usability reasons why I would do that. For one, if you allow the user to submit all those changes for multiple records on one page you have to validate the input for all the records (which you don't appear to be doing and are completely open to SQL Injection!). Then if there is a validation error for one record it becomes more difficult to handle. Also, if the user is only needing to update one or a couple records you still have to process ALL the records, that's just inefficient. There are other reasons too, but I'm not going to write a book here. Ok, so let's say you do want to have a page to update all the information for all the records in one swoop. That can be done - again without running queries in a loop. In addition to implementing validation and error handling which you are currently lacking, you would implement a process to gather all the data to be updated and create a single query using a MySQL CASE statement. You could use your current code as a base for this. But, you would still have to fix the problem you have. In my last post I stated: But, I guess you don't know that because that is the cause of your problem. Currently your input fields are being created as arrays (good), but you are not defining an index for them (bad). You assumed that the first checkbox in the post data would belong to the first text field and the first file field. But, if you only check the 3rd and 4th checkboxes then the 1st and 2nd aren't included in the POST data, so the first checkbox value in the POST data will belong tho the 3rd record and the 2nd checkbox value will belong to the 4th record. For a multi-record form, you should always explicitly assign an index for each "group" of records. If you are editing records, then set the index for each input to the record ID. <input type="checkbox"<?php echo "$active"; ?>name="active[<?php echo $id; ?>]" /> <input type="text" style="padding: 0px;" name="name[<?php echo $id; ?>]" value="<? echo "$name"; ?>" /> <input type="file" name="menu_file[<?php echo $id; ?>]" /> Now, you do not need a hidden field to store the record ID, because you will have it as the array index for each value! Also, just an FYI, you are creating the field for "MAX_FILE_SIZE" in your loop. You only need it once on your form, so create it outside the loop. Now, in your processing logic you want to go over each "group" of inputs. Since the unchecked checkboxes are not sent in the POST data, you can't use those. But, all the text inputs will be sent in the POST data even if they are empty. So, you can use that array to loop over each record set like this: foreach($_POST['name'] as $id => $name) { $active = (isset($_POST['active'])) ? 'on' : 'off' ; $file_name = $menu_file["name"]["$id"]; //name of file on users machine $file_type = $menu_file["type"]["$id"]; //type of file being uploaded $file_size = $menu_file["size"]["$id"]; //size of the uploaded file in bytes $file_error = $menu_file["error"]["$id"]; //returned PHP error codes for upload $file_temp = $menu_file["tmp_name"]["$id"]; //temporary name on server //Process the record data and VALIDATE } Now, instead of running the UPDATE query on each loop, you can use the data to prepare a single query to update all the records. foreach($_POST['name'] as $id => $name) { //All procesign and validation has been done prior to this $activeUpdates .= " WHEN {$id} THEN {$active}\n"; $menuUpdates .= " WHEN {$id} THEN {$name}\n"; $locationUpdates .= " WHEN {$id} THEN {$upload_name}\n"; } //Loop is complete, create ONE query to update all records $query = UPDATE menus SET active = CASE {$activeUpdates} ELSE active END, SET menu = CASE {$menuUpdates} ELSE active END, SET location = CASE {$locationUpdates} ELSE active END";
-
Not sure what your issue is with understanding foreach() loops, they simply iterate through each record in an array. But, let me be blunt - your is very disorganized and just plain messy. I could spend a while going over all the issues I see (i.e. don't use PHP short tags). I'm not saying this to be mean. You need to step back and plan out what you are doing and then execute it. I can tell you are creating the logic as you write the code - and some of the logic is just plain poor. For example: $active = $row["active"]; if($active=="on") { $active = " checked=\"checked\" "; } elseif($active!=="on") { $active = " "; } Why do you use an elseif() and why are you using a string variable for a true/false value? You should be using a tinyint field with a 1/0. That logic should look something like this: $active = ""; if($row["active"]=='on') { $active = 'checked="checked" '; } Or just use the ternary operator: $active = ($row["active"]=='on') ? 'checked="checked" ' : ''; I've looked briefly over your code and am not going to try and "fix" it because you are taking the wrong approach. You should NEVER run queries in loops. There is always a better way. Anyway, this is the approach you should be taking: For the form, create the checkboxes with a name as an array and the value as the ID of the record: <input type="checkbox" name="active[]" value="<?php echo $id; ?> Now, as you should know only "checked" checkbox inputs are passed in the POST data. So, you will not get a list of only checked records and not the unchecked records. You can easily update all the records with just TWO queries. Example: $checkedIDsAry = $_POST['active']; //Convert values to INTs for DB security array_walk($checkedIDsAry, 'intval'); //Convert to comma separated string $checkedIDsStr = implode(',', $checkedIDsAry); //Create and run one query to update checked records $query = "UPDATE table SET active = 1 WHERE id IN ($checkedIDsStr)"; mysql_query($query); //Create and run one query to update unchecked records $query = "UPDATE table SET active = 0 WHERE id NOT IN ($checkedIDsStr)"; mysql_query($query);
-
What? You are running a query of all records to determine the distance and then run another query using the records within the specified distance? Why do that. Just run one query of all the records and determine the ones you need while processing them. That is going to be more efficient than running two queries.
-
Then you should have stated that from the beginning. I did..... No, you stated you want to include/exclude PHP code on the server based upon whether JavaScript was enabled. But, when you say you want your site to be seamless for users with/without JavaScript then you are talking about "Unobtrusive JavaScript", which is a concept that has been around for years and does not include making server-side changes to support And I don't see how this is a needless exercise? The rest of your post was not very helpful at all. It is obviously not as straight-forward as you say and your example has nothing to do with what I am trying to do at all. I didn't mean that it is a needless exercise in that the goal you are trying to accomplish is needless or not worthwhile, only that the method you are trying to utilize to meet that goal is the wrong method. So, any further discussions in trying to make that method work are needless. As I stated above, the concept of "unobtrusive JavaScript" (utilizing JavaScript that does not break the user experience for users with JS enabled) has been around for years. In addition, to solving the JS disabled issue, "unobtrusive JavaScript" also addresses inconsistencies on how JS is interpreted between different browsers as well as backward comparability for functions not supported in older browsers. But, one of the core concepts of this process is a separation of the "behavior" layer (i.e. the JavaScript) and the presentation layer (the HTML). The example I gave was just an off-the-cuff example of one very specific scenario. But, the same principles can be applied for any number of scenarios. There are a ton of resources regarding unobtrusive JavaScript that you could take a look at to obtain the goal you are after. Or, you can just disregard everything we have provided and do it your own way.
-
Bring back the <blink> tag! But, seriously, when the frack are people going to stop using the <font> tag?
-
OK, now that you have at least attempted it, I'll show you a more efficient method. You should avoid using loops for things when there are other methods at your disposal. That function is inefficient as it need to run a loop for each and every character - especially since it has to repeat whenever a duplicate character is encountered. That is just a waste. Also, I would suggest not using variable names such as "$norepeat" - when the value is FALSE you have a double negative (by virtue of the no) which results in a true. If a variable is supposed to be true/false, name it such that it is more logical (i.e. $repeat). You can always check for a negative. lastly, I would suggest making the list of characters as an additional parameter for the function. That give's you more flexibility. Anyway, this will do the same thing in a much more efficient manner function randomString($length, $charList, $repeat=false) { if(!$repeat && $length>strlen($charList)) { throw new Exception('Non repetitive random string can\'t be longer than charset'); } //Create an array of the characters (add additional if needed) $charsAry = str_split(str_repeat($charList, ceil($length/strlen($charList)))); //Randomize the array shuffle($charsAry); //Return the random string return implode(array_slice($charsAry, 0, $length)); } $chars = 'abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789!@#$%^&*'; echo randomString(32, $chars);
-
Why don't you tell us what is happening that shouldn't or what is not happening that should so we have some place of reference to look for a problem.
-
Then you should have stated that from the beginning. If that is your goal then this is a needless exercise. It is a fairly strait-forward task to implement unobtrusive JavaScript so it is only used if the user has JS enabled. Let's take an example: Let's say you have a paginated report of records. At the bottom of the page you have a select list for the user to "jump" to a selected page. Many such controls use JS to automatically do a submission when the value is changed so the user doesn't have to click a submit button. But, if the user doesn't have JS then you need a submit button (or you can remove the control completely and just let them use the other navigation links). The solution: figure out how you want the page to behave when the user does not have JS enabled and build that FIRST. In this case we will give the user a submit button next to the page selection list. Pretty simple, just build a mini form with just that one select list (maybe a hidden field or two as needed) and a submit button. Once you have that working THEN you can implement some JS to enrich the user experience. So, now - if the user has JS enabled - we want the form to submit as soon as the user changes the select list value and we don't want to display the submit button since it has no purpose. Simple. Create an onload even that will do two things: 1) Change the style property of the submit button so it is not displayed and 2) create an onchange trigger for the select list that will call a function to submit the form. That is a very simplistic scenario, but it is the same process you should take for anything like this. Build the HTML only solution first, then add JS features on top of that and include code that will hide features/content that are not needed when JS is enabled.
-
Only adding the id and distance to the array doesn't make sense. I have to imagine that you want to output more data than just the ID and the distance (name, address, url, etc.???). By creating an array with just the distance and ID it will be difficult to match the data up with the records. You should create a multidimensional array with all of the photographer data you need and ADD the distance. Then you can use usort() along with a custom function to sort the array in any manner you want. In the solution below it sorts first by distance then by "name". So, if two records have the same distance they will be sorted by name. I just guessed on the field name, but you can change it to whatever you want and/or modify the sorting function however you need. $photographers_inrange = array(); while ($row = mysql_fetch_array($result)) { //get the photographers postcode and split it into 2 pieces $p_postcode = strstr($row['address_post_code'], ' ', TRUE); //get the photographers postcode and split it into 2 pieces $u_postcode_a = strstr($u_postcode, ' ', TRUE); //run the two postcodes throught the function to determin the deistance $distance = return_distance($u_postcode_a, $p_postcode); //if the distance is <= to the distance the photographer covers if($distance <= $row['cover_distance']) { //Add entire record to array $photographers_inrange[$row['ID']] = $row; //Add distance to array $photographers_inrange[$row['ID']]['distance'] = $distance; } } //Custom function for sorting by distance, then by "name"? function sortPhotographers($a, $b) { if ($a['distance'] != $b['distance']) { return ($a['distance'] < $b['distance']) ? -1 : 1; } if($a['name'] != $b['name']) { return ($a['distance'] < $b['distance']) ? -1 : 1; } return 0; } //Sort the records usort($photographers_inrange, 'sortPhotographers'); foreach ($photographers_inrange as $id => $record) { echo "{$record['name']} ({$record['ID']}): {$record['distance']}KM<br>\n"; }
-
OK, after reviewing the query further, it doesn't make sense. Here is the query in a readable format: SELECT post.username, post.dateline, post.postid, post.threadid, thread.threadid, thread.forumid FROM post LEFT JOIN thread ON post.threadid=thread.threadid WHERE thread.forumid='$id' ORDER by postid DESC LIMIT $default_limit OK, the "LEFT JOIN" tells the query to include records from the LEFT table (i.e. posts) even if there are no corresponding records in the RIGHT table (i.e. thread). First off, it looks as if the post records are dependent on the thread (i.e. multiple posts can be associated with a thread). So, why are you using a LEFT JOIN? Do you really have posts that are not associated with a thread? Further, you have a WHERE clause that is checking for threads that match a specific value. But, the JOIN is trying to include posts that are not associated with a thread - yet you put a specific condition on the records using a value in the thread. This is illogical. I don't see any reason to use a LEFT JOIN (if my understanding is correct). just use a normal JOIN.
-
This topic has been moved to MySQL Help. http://www.phpfreaks.com/forums/index.php?topic=342696.0
-
You would have to provide more details regarding your database structure. And, this post belongs in the MySQL forum [moving] - not the PHP forum. You can also do an "EXPLAIN" on your query in MySQL to see what it returns (google it). EDIT: I see nothing in that query on the face of it that should cause a problem. Are you running this query in a loop?
-
But, the OP is trying to include a file in the PHP code.
-
Never EVER run queries in loop!!! 99% of the time you can get the information you want with a single query. The other 1% of the time you are doing something you shouldn't. You need to learn how to do JOINs. Your code is very unorganized making this difficult to resolve. But, it comes down to the fact that you only need ONE query to get all the relationships between the sender and the receiver (without having to do a loop of all employees). I think the following will work based upon what I think I am able to understand of your database structure. If this isn't correct ,please post the full details of the tables involved along with an explanation of exactly what you are trying to achieve. if (!$con) { die('Could not connect: ' . mysql_error()); } mysql_select_db("Book", $con); $sender_email = mysql_real_escape_string(trim($_POST['email'])); $query = "SELECT firstName, lastName FROM employeelist WHERE Email_id = '$sender'"; $result = mysql_query($query); if(!$sender = mysql_fetch_assoc($messenger)) { echo "No user found for the email adrress: {$_POST['email']}"; } else { $sender_fname = $message ['firstName']; $sender_lname = $message ['lastName']; $query = "SELECT firstName, lastName, count(recipientinfo.rvalue) as `count` FROM employeelist JOIN message ON employeelist.Email_id = .sender JOIN recipientinfo ON message.mid = recipientinfo.mid WHERE message.sender = '$sender_email' AND recipientinfo.rtype = 'TO' GROUP BY recipientinfo.rvalue"; $result = mysql_query($query) or die("Query:<br>{$query}<br>Error:<br>".mysql_error()); echo "<br/>"; while($row = mysql_fetch_array($result)) { $receiver_fname = $row['firstName']; $receiver_lname = $row['lastName']; $count = $row['count']; echo "The number of ties between the sender {$sender_fname} {$sender_lname} and the receiver {$receiver_fname} {$receiver_lname} is: {$count}<br>\n"; } } mysql_close($con);
-
I would forgo the PDF portion at this point and just make an HTML table with the letters. If you are learning, there is no need to over-complicate this. Once you can do that - then you can learn the process of making PDFs. As for making the word search, that is an interesting problem. Trying to program the logic to dynamically determine the positions of the words will take some effort. You have to be able to get words to cross-over each other and share some letters. To do that would take some analysis of the words before you start, otherwise you may only get a handful of words in the grid and none of the remaining words would fit. The only suggestion I can think of would be to start with the longest words and work backwards. I'm sure this is possible, but it is not a trivial task by any means. I would highly suggest that before you even start coding that you get out a piece of paper and write out the logic you want to use to determine placement of the words. If you can't come up with the logic, no amount of functions are going to help. If I were doing this I would probably use a multidimensional array to store the letters.
-
You can't use JavaScript to determine what pages are include()ed in PHP! And, it is possible to determine if JavaScript is enabled on the user's browser. All you need to do is simply have some JavaScript code when the user first access your site to set a cookie (e.g. 'javascript' = 1). Then in the PHP code you can check if that cookie is set. If so, then you know JS is enabled on the user's browser. Otherwise, you can assume it is not. Alternatively, you could implement an AJAX call that sets a variable for the user's session. The drawback to both methods is that you need to have the page with the JS code loaded before any pages that need to react to whether the user has JS enabled or not. One solution would be to validate JS on the first page load when a users access your site. Here's a rough example: if(!isset($_COOKIE['javascript'])) { //redirect to page to confirm JS or not header('http://www.mysite.com/jscheck.php'); exit; } Then the page 'jscheck.php' would load and attempt to set the cookie with JS. Also, that page could utilize a META tag to refresh after 1-2 seconds. Upon refresh the PHP code could determine if the JS cookie was set or not. If not, it would set the cookie itself with a false value (i.e. 0) and then it would redirect the user (using the header() function) back to the original page requested. So, the end result is that the very first time the users access the site, there may be a few seconds and a page refresh before they get redirected back to the original page. But then, after that, you can reference the cookie as needed.
-
To add to .josh's statement about rethinking this, how do you plan to "protect" a tutorial with an image? You can't just slap an image on top of other content and even remotely think it is "protected". It is not possible to "protect" any content you make available on the web, the only things you can do is 1) Put watermarks *into* content so anyone reusing the content would be seen as potentially stealing it (or at least it would give credit to you). 2) Put measures in place to make it difficult for someone to copy your content. Both of the above have significant problems. For using watermarks, they are only useful if embedded as part of the content (i.e. images and videos, they are only somewhat effective in PDFs). The problem with watermarks is that you have to balance making the watermark useful vs. obscuring the content. A small, unobtrusive watermark can be removed or covered. But, you don't want the watermark so obtrusive that it gets in the way of users consuming it. It really is difficult to pull off, IMO. As for putting measures in place to prevent users from copying content. This is an exercise in futility. The methods involved can utilize many different methods (with JavaScript being one of the more common). But, in the end these methods only prevent copying by novice users. Anyone with any a modicum of ability/knowledge in web technology can easily get the content. And, to make it worse, many of the methods implemented can prevent legitimate users from accessing the content due to browser incompatibilities. So, you should really take a step back and ask yourself if trying to protect your content is worth all the trouble considering that it will probably impact your legitimate users more than those wishing to copy the content and that, in the end, you really can't protect it with any certainty.
-
After second thought, there is an easy way for the query to return 0 when sum() returns a null value. Also, I just realized, that those queries will not work because there is no GROUP BY clause! Don't know how "it worked" for you. Here is an updated query that has the requisite GROUP BY and will return 0 for records that don't have any associated points records $sqlquery="SELECT IFNULL(SUM(points.promo), 0) AS score, members.name FROM members LEFT JOIN points ON members.id = points.memberid GROUP BY members.id ORDER BY members.name ASC";
-
Go and find a tutorial or two on how to use JOINs in queries. It may be a little daunting at first, but knowing how to really use JOINs is a very powerful skill. Here is a revised query. Although, note that 'score' will be returned as a null value, so be sure to add some handling to convert to 0. Also, IMHO it is advantageous to put line breaks in the query for readability. It is difficult to look at a long query all on one line and "see" any errors. When you put line breaks to separate the logical parts it is much more intuitive. $sqlquery="SELECT Sum(points.promo) AS score, members.name FROM members LEFT JOIN points ON members.id = points.memberid ORDER BY members.name ASC"; Note the "LEFT JOIN" which means include the records on the LEFT (i.e. the first table) even if there are no records to join on the RIGHT (i.e. the second table)
-
Yeah I attempted it using mt_rand like voip03 did, but his is far more elegant. I'll get started on this now Well, it will get somewhat complicated (or inefficient) to use that logic while enforcing the requirement for no repeating characters. I have a fairly easy implementation in mind, but I want to at least see you make an attempt rather than just writing it for you.
-
I totally missed that. I thought he was simply appending the result to an array - didn't see he was calling another method. Doh! gizmola provided a solution for you: public function getAll() { $db = $this->_db; $sql = "SELECT * FROM $this->_table"; $db->query($sql); while($data = $db->fetch_array()) { $this->_friends[$data['id']] = $data; //<--Changed line } return $this->_friends; }
-
The PHP code is simply executing the command. If you have validated that you are passing the exact same values and getting different results then it is likely a problem with ffmpeg. Perhaps you have an earlier process that had not yet complete when you call another and the application can't handle that. Or, perhaps, when you are checking the file the process had not yet completed - it won't be instantaneous. I suggest you check into the documentation for ffmpeg. However, Clarkeez's solution will not work. Your code is just calling the application to start the process. It certainly won't be done by the time PHP gets to the next line in the code. You would have to implement a process for PHP to continually check for the file and the file size every x seconds, with a maximum execute time of y seconds. So, if the file isn't created (with a filesize > 0) by the max timeout you can guesstimate that the process failed. Certainly not an ideal solution.