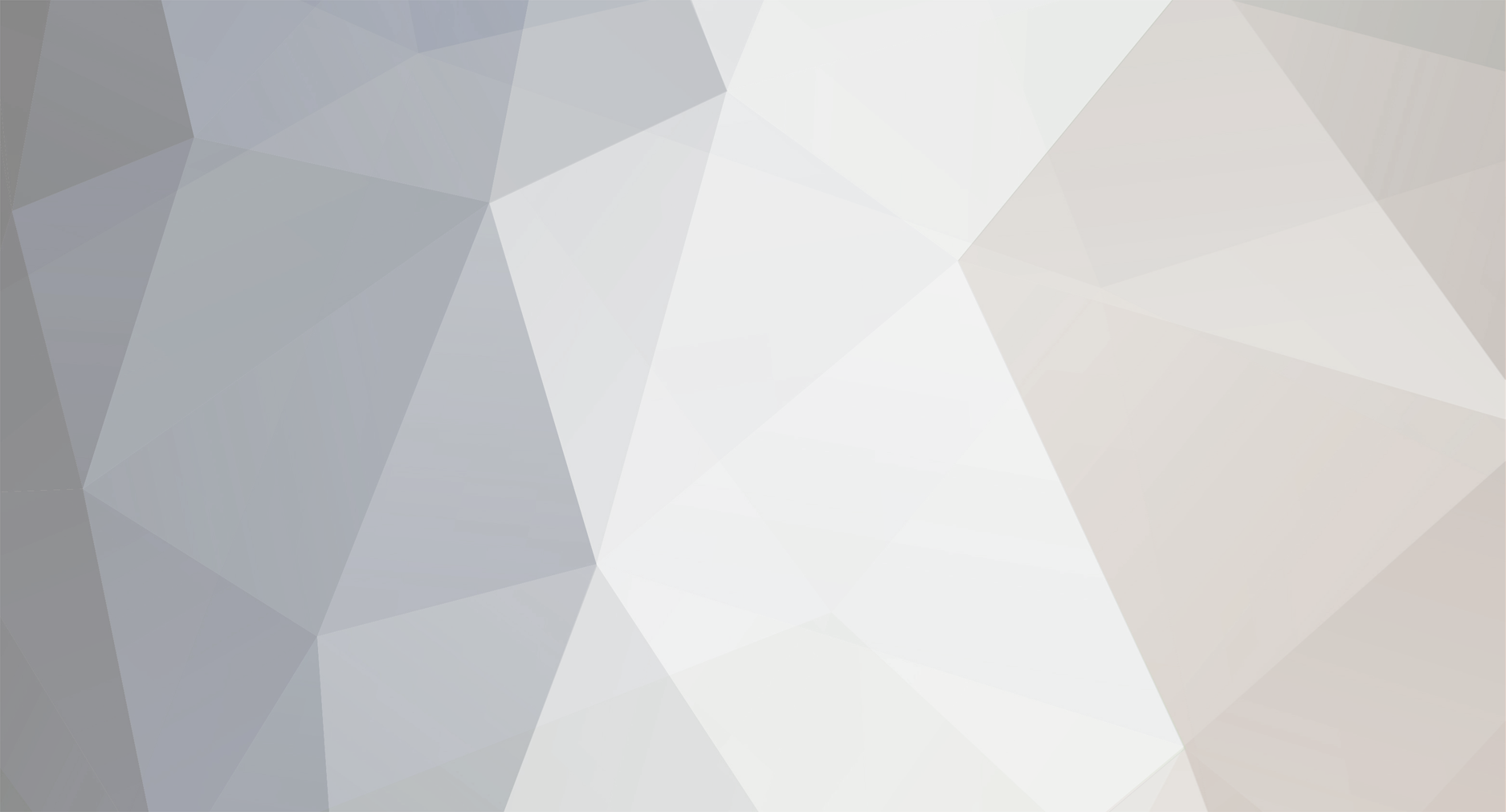
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
You don't need sessions. Again, to determine if a checkbox was checked you just check if the field was passed int he POST data, i.e. isset(). In fact, you can do the same for all the fields. Personally, I hate putting PHP "logic" code within the HTML, but since that is what you are doing: <form action="<?php echo $PHP_SELF;?>" method="post" name="reg1"> <tr> <td> <input name="noemail" type="checkbox" value="noemail"<?php if (isset($_POST["noemail"]) { echo ' checked="checked"'; } ?>> Do not want to get the E-mail address </td> </tr> <tr> <td nowrap>Enter email - 1 <input name="email__1" type="text" value="<?php if (isset($_POST['email1'])) { echo $_POST['email1']; ?>" /> </td> <td nowrap>Enter email - 2 <input name="email_2" type="text" value="<?php if (isset($_POST['email_2'])) { echo $_POST['email_2']; ?>" /> </td> </tr>
-
OK, I didn't read through all of your post, but I think I read enough to at least provide some suggestions. I think the function cleanQuery() is problematic for several reasons: First off, if magic quotes are turned on, then you need to be running strip_slashes on the input regardless if you are going to use it in a query or not. You state that you are having the form post to itself. I do that all the time because I can then repopulate the form with the user's input if there was a validation error. If magic quotes are on you need to unescape the input but NOT run it through the mysql_real_escape_string(). The way I look at it - is there any reason you want the escaped value that is generated via magic quotes? I can't think of any. Therefore, I prefer to put a function that is called on load of every page to unescape ALL input (POST, GET, etc.) if magic quotes is turned on. Then you are always dealing with the raw user input. Second, I see no reason to try and support mysql_escape_string(). That function was introduced in 4.0.1 (the year 2000) and mysql_real_escape_string() was introduced in 4.3.0 (the year 2002). If anyone is running a version of PHP that doesn't support mysql_real_escape_string() they should upgrade. Trying to be backward compatible to a decade old technology is not worthwhile. Third, mysql_real_escape_string() is for 'string' data. You should not rely upon it for any other type of input: date and/or time, ints, floats, etc. Running values through mysql_real_escape_string() that are supposed to be numeric is a pointless process. I see you are running $_POST['scouts1'] through the cleanQuery() function and then checking if the value is numeric. If the value is numeric then the cleanQuery() wouldn't do anything to the value anyway. So, you just performed an operation that did nothing. Also, I don't think is_numeric() is the right validation. I assume the value is the primary index for a db value? If so, you should be checking that the value is an integer. is_numeric() will return true for negative and float values. Lastly, unless you have a good reason not to (on a case y case basis) you should always be trimming the user input to remove leading/trailing white space. So, I suggest having an initial process that runs strip_slashes() on the initial input in case you need to repopulate the form with the submitted data or use it in another manner outside of a query. Then use a second process to escape/validate the data for use in a db query. You can create individual functions for each type of data you need to process (string, date, int, float, etc.) or you can create one function that you pass the value and a second parameter to determine the correct processing.
-
300 millisecond seems a little aggressive to me. I would probably go with every second or, at a minimum, every 500ms. But, be sure to set your request up so that if a request is pending it doesn't send another one. There are always instances where due to traffic on the net that a request may take a second or two.
-
ALL the data? You should only have it retrieve the NEW data. When the client makes a request to the server it should send a timestamp of the last time a request was made. Then the server would query for any messages with a newer timestamp. You'll need to add another field to your table to store the timestamp of when messages are saved. When a response is made back to the user (whether there was new data or not) you should return the timestamp used to query the DB. Don't use a JS timestamp or you could have a message missed due to a slight variance
-
What security risks are you concerned with? If it is that someone could exploit the code to access the data, then that is just something you have to code against just as any site with a database. If you want the data deleted then delete the messages when someone retrieves a message meant for them. BUt, that limits your ability to resolve errors if they occur. As for sending too many requests, you should only be returning the new information to the user. So, if there are no new messages, the request will take very little bandwidth and resources. That assumes the application is built to be efficient.
-
Why is this causing Escape characters in my DB?
Psycho replied to doubledee's topic in PHP Coding Help
You need to be careful before converting input into a display dependent format. There are a several drawbacks to doing that you need to either be aware of or actively code around. For one, if you ever wanted to use that data for some other purpose than in a web page you would have to unencode it, which is not a 100% guaranteed process. 2) You will have to create your database fields to be much larger than the maximum allowed length for the user. There is no way to tell how many characters the user will enter that may be converted to five/six character encoded values. So, you either need to make the DB fields excessively large or risk losing some of the user input. -
Why is this causing Escape characters in my DB?
Psycho replied to doubledee's topic in PHP Coding Help
Echo the values before and after each operation to determine if something is adding the backslashes or if it is coming from the source. -
Just add some simple debugging to see what error is occurring $result = mysql_query($query) or die (mysql_erro());
-
It's pretty difficult for us to tell you how to set this up. All of this will be dependent upon the business requirements. Duration: I have no idea what this is for really. What exactly are the user's buying? YOU need to decide how you want to sell the product. Should you be selling it for three months (Jan-Mar) or should you be selling it for 90 day durations? Don't create your database to decide your business model. Choose your business model then build your database to fit. Amount: Again, this is YOUR decision. What currency makes sense for you. Are you going to use a service to process payments? If so, what is the common demonination that the service uses> Or do you want the currency in your home denomination? Upgrade: how the hell should I know. I don't know what the product is or the complexity of how upgrades would apply. Again, figure out the business requirements, then build to fit.
-
Always set the session regardless of whether the user wants to be "remembered" or not. I assume you have a login? When the user first hits your site you should check if the cookie value is set. If so, authenticate against that cookie value and then set the session value. If no, then have the user log in and set the session (and also set the cookie if they choose to be remembered). While the user is on your site always use the session value.
-
Use cURL: http://php.net/manual/en/book.curl.php To get started look up some tutorials
-
Looping problem (dropping a record every 6th row)
Psycho replied to shamwowy's topic in PHP Coding Help
Not to knock the others' solutions, I'd prefer using a modulus operator in this situation. Also, I see there was no handling to close the last row if the results weren't an exact multiple of 5. I don't like having mis-formatted HTML. Here's my solution. you can easily change how many columns are used by changing the value of $cols_per_row. Also, the last row will always be properly closed even if there are only 1-4 record on the last row $query1 ="SELECT * FROM jitem JOIN jloc ON jloc.jloc_id IN(jitem.jitem_location) WHERE jitem.jitem_display = 1 ORDER BY jitem_listorder"; if( $r = mysql_query($query1) ) { $cols_per_row = 5; $column = 0; while( $row = mysql_fetch_assoc($r) ) { $column++; if ($column % $cols_per_row == 1) { echo "<tr>\n"; //start table row } print "<td width=20% valign=top align=center>"; print "<a href='slides/{$row['jitem_slide']}' title='{$row['jitem_title']}' border=0 onclick=\"window.open(this.href, '','height=545,width=500');return false;\">"; print "<img border=0 src='thumbs/{$row['jitem_thumb']}' alt='{$row['jitem_title']}'/><br/>"; print "<font size=2 color=white><strong>{$row['jitem_title']}</strong></a><br/>{$row['jitem_desc']}<br/>"; print "\${$row['jitem_price']}</font>"; print "<br/><font size=2><a href=cart.php?itemid={$row['jitem_id']}&action=add>Add To Cart</a></font>"; print "</td>\n"; if ($column % $cols_per_row == 0) { echo "</tr>\n"; //end table row } } //Close last row if not already closed if ($column % $cols_per_row != 0) { echo "</tr>\n"; //end last table row } } else { print "Database returned no records. query1 is {$query1}"; } mysql_close(); -
Hmm, I can't replicate that error using that code no matter what value I provide for $username - even if I use an explicit integer. Are you absolutely sure that is the right file and not some other file that is include()ed in that page? Go ahead and post the first 40 lines from registration_script.php
-
I was curious about this and decided to do some research. Here are the W3C specs on checkboxes http://www.w3.org/TR/html4/interact/forms.html#h-17.2.1
-
It is standard HTML behavior that checkboxes that are not checked are not passed in the POST data. But, it has nothing to do with being "empty". An empty text field IS passed in the POST data because an empty string IS a value. Unchecked checkboxes are not passed because, well, what would be passed? When a form field is passed in the POST data you get the field name and the field value. If the checkbox is checked you get the checkbox name and the checkbox value. So, if it is not checked you would get the field name and what? They could have passed an empty string, but the value could have been an empty string so you still wouldn't know if it was checked or not. A null value might have made sense, but I think the problem there is that different programming languages handle null differently. So, I assume, the decision was to not pass the field at all. In 90% of cases the value of the checkbox is not needed since you are typically concerned with whether it is simply checked or not [using isset()]. Where I am usually concerned with the value is when I provide a list of checkboxes for the user to select from. In those instances I will create the checkboxes as an array. For example a list of categories with the category ID as the value. I can then determine which categories were checked using the values of the array passed in the POST data.
-
Which is why there is a manual! If you go to the manual page for in_array() the left hand side of the page lists all the functions related to arrays. Also, each manual page lists similar/related functions under the "See Also" section right after the examples. Whenever you are having an issue with a function not doing what you expect or it doesn't "quote" do what you want always check the "See Also" section. In this case, array_intersect() isn't listed in the See Also section for in_array(), but it is also a good idea to browse through the other functions available. I have stumbled upon many useful functions by just browsing the manual. array_intersect() has five different variations and there are also 5 variations of array_diff(). You should take a look.
-
You can use an array as the needle. But, if you do it is checking if that array exists as a subarray of the haystack - not the individual values of the needle array. You could loop through each element in the needle and check it against the haystack using in_array(), but there is a simpler solution: array_intersect(). array_intersect() will find all instances of the values from the first array that exist in any of the other arrays and returns the result as an array. In this case it would return an array of all the values from $conf['guild']['reqAchievements'] that exist in $charAchievements. If that resulting array has 0 elements then none of the values from $conf['guild']['reqAchievements'] exist in $charAchievements. if(!count(array_intersect($conf['guild']['reqAchievements'], $charAchievements))) { $charMeetsAchievement = false; } else { $charMeetsAchievement = true; } However, since you are simply setting a value to true/false based upon whether the condition is true/false; you can simplify this by setting the value TO the condition. Just change the condition to return true if results were found. $charMeetsAchievement = (count(array_intersect($conf['guild']['reqAchievements'], $charAchievements)));
-
Yes, it absolutely can be used in a for loop. But it seems that $charAchievements[$i] is an actual value of the array and not an array itself. If $charAchievements[$i] was a subarray then it would work. The point of in_array() is to check the value against all the values in the array - there is no need to iterate through the array to use in_array() - it does it automatically. The exception is if you need to check a value against a mulch-dimensional array. So, if my assumption is correct that $charAchievements is a single-dimensional array then you don't need a for loop at all. just if(!in_array($conf['guild']['reqAchievements'], $charAchievements)): // <== Removed [$i] $charMeetsAchievement = false; else: $charMeetsAchievement = true; endif; If you are not understanding this or you think I may be misunderstanding this, please provide the output of print_r($charAchievements); so we can see the structure of the array.
-
Maybe I used the wrong description. It's not that it won't work, it is just not the logically correct test. The test is to determine whether the checkbox was checked or not. If it is not checked then that POST value will not be set. So, isset() is the logically correct test. As you pointed out a value of "0" (or an empty string) would cause empty() to fail. Yes, it would be foolish to use "0" (or an empty string) for the value of a checkbox, but using isset() will work regardless of the value of the checkbox. You also have to consider that the person writing the code today isn't the person that will always be the person working on the code. Someone could come along and set the value of the checkbox to "0" in an attempt to use that value for some other logic. My point is, if you want to know if something is empty use empty(), if you want to know if it is set use isset(). As for the reordering of the conditions, that wasn't directed to you. That was just a general statement based upon the original order. I have spent countless hours when going through old code to try and re-understand what I have done, so I try to always make my code logical and as easy to comprehend as I can (within reason). The little bit of extra effort save much more time on the back end. And, as to the "performance" benefit. Yes, absolutely, you would never notice it in this instance. But, that doesn't mean it would never be a benefit, which is why I pointed it out. If, for example, you have a script that needs to process hundreds/thousands of records from a DB query or an import file that need to be processed than any tweak you can make to be more efficient is desireable.
-
When a user registers you can just generate a unique code for that user, store it in the database and then send the user an email with a url using that unique code as a parameter of the url. Here is an outline of the steps you could take: 1. User registers on your site. 2. Create a "random" code. For example you could create an MD5 hash of their username and/or email address. Just make sure it will be unique 3. Store that value in a column in the user table (e.g. registration code). 4. Create a url (e.g. www.mysite.com/confirm.php?code=[random_code]) with that code as a parameter and send it to the user in an email 5. Create a page for that URL to point to (confrm.php). In that page grab the code using $_GET and do a MySQL query(ies) to update that user record to be "confirmed" You also need to determine how to differentiate between confirmed/unconfirmed users. One easy method is to simply remove the confirmation code when the user confirms their account. Then if a user attempts to log in after checking the username/password you would check to see if there was a value for the confirmation code. If so, you provide a message that they need to confirm their account before logging in. Although, you would need to consider if you need to provide a means for someone to regenerate the confirmation email. Or even change the email if they mistyped it.
-
You should not run queries in loops. You should be able to get all the data you need with a single query. Provide some details of the tables you are pulling data from, what data you want, and the format you want the data to be displayed.
-
Right, your query is failing. Change your mysql_query() line to this $done = mysql_query ($sql) or die("Query:<br>{$query}<br>Error:<br>".mysql_error()); However, I see why your query is failing. Here it is in a more readable format: $sql = "SELECT * FROM message, recipientinfo WHERE message.message_id = recipientinfo.mid AND ( message.sender = 'kevin.hyatt@enron.com' AND recipientinfo.rvalue = 'michelle.lokay@enron.com' AND recipientinfo.rtype = TO )"; It is failing because of the "TO" in the last condition. If you are trying to compare against a string then the value need to be in single quotes: 'TO'. If TO is a variable or constant then you will need to define it appropriately - if it is a string then it still needs to be in quotes. Personally I prefer to use explicit joins. I don't know if it is more efficient, but it is easier to see the relationships. This is how I would have prepared that query $sql = "SELECT * FROM message JOIN recipientinfo ON message.message_id = recipientinfo.mid WHERE message.sender = 'kevin.hyatt@enron.com' AND recipientinfo.rvalue = 'michelle.lokay@enron.com' AND recipientinfo.rtype = 'TO'"; Don't be afraid to add line breaks and spacing to your code. It makes the code much, much more readable and logical for a human.
-
@xyph, that is incorrect logic. A checkbox that is not checked does not get passed in the POST data. So, even if empty() works it is the wrong test. Since this is a single checkbox and you only want to know if it was checked or not, the value is insignificant. You simply need to see if it was is set. Although there was no problem with the order of the conditions, I changed them up slightly to what, I think, is a more logical order for comprehension. It is also more efficient from a code perspective. If the checkbox IS checked the other conditions won't be tested because of the AND clause. if( !isset($_POST['checkbox']) && (empty($_POST['email']) || !check_text($_POST['email'])) ) { echo 'You have not entered an email or hit the check box';
-
If you are talking about the "default" property for fields, yes that is the default value to be applied when a new record is created if one is not set when the record is created. So, if I have a field called 'active' with a default value of '1' then I can create new records without setting a value for that field and they will default to 1. I don't know about your version of PHPMyAdmin, but in mine if I set a field type to "timestamp" there is a checkbox that appears right below the default value text box which states states "CURRENT_TIMESTAMP". Just check that field and the field will automatically be set to the timestamp at the time the record is created - assuming you don't pass a value for the field.
-
Didn't I provide a sample query above? If you saved the value as a text value instead of a numeric value, that query won't work though. But, in my opinion, that is still the wrong way to do this. I would suggest to instead add a new record to the transactions table for the interest. But, that obviously would require a complete overhaul of what you have now.