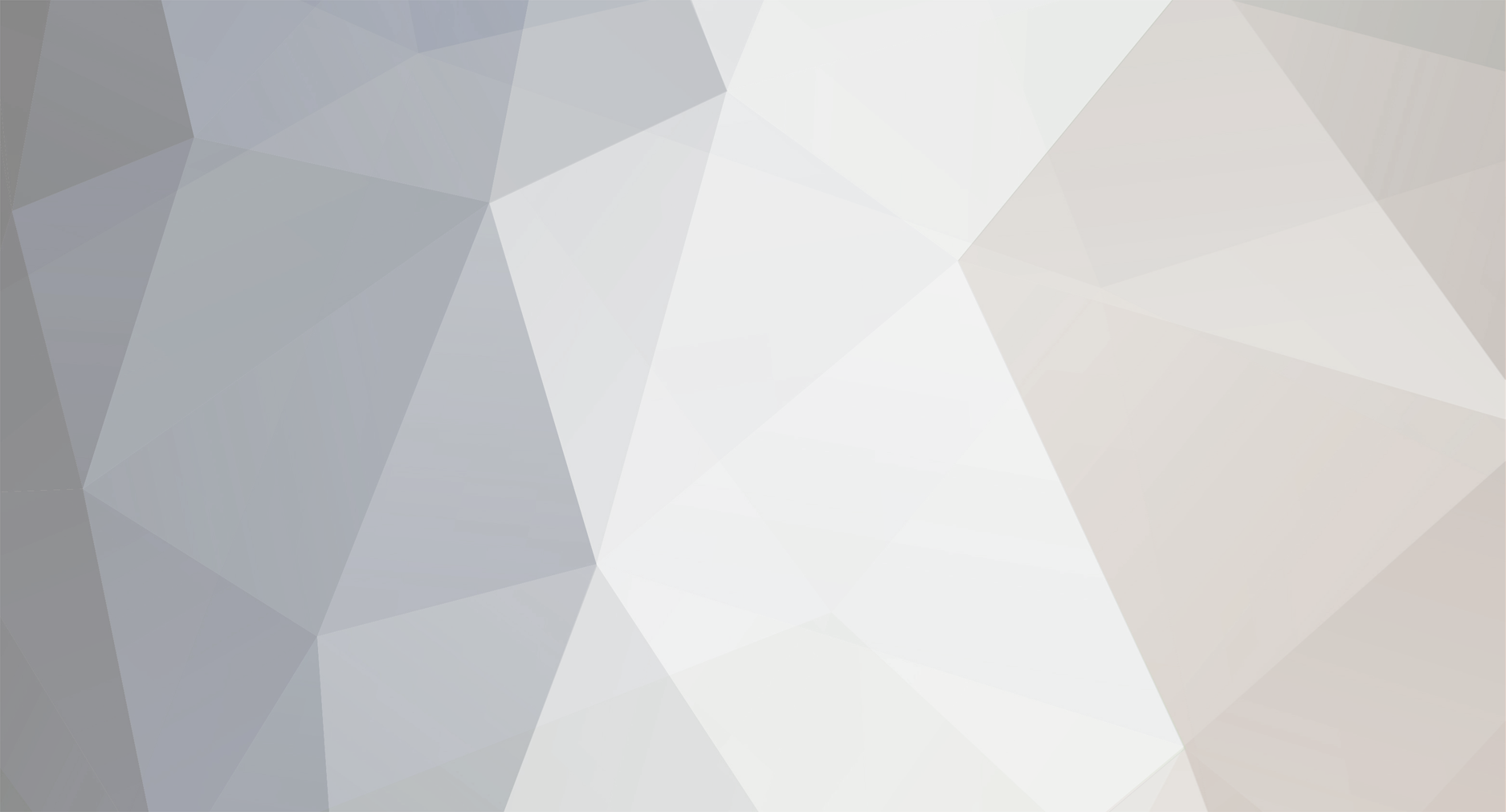
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Works in IE9
-
You should not store the user's password in a cookie. Anyone with access to the user's computer could access the cookie and see the password. Use something else, such as a hash of the password. Granted, someone could copy the cookie to their PC and access the site as that user, but they would not have the user's password. Since so many people reuse passwords among different sites - exposing the password is much worse than someone getting access as that user to just one site. And, for that matter, I wouldn't store the username either. Just set a value for the user id or something that would have no meaning to someone who analyzes the cookie data.
-
Don't use PHP to sort the records, use the MySQL ORDER BY to sort the options. Also, don't use '*' to get all the fields if you only need model $query = "SELECT model FROM cars ORDER BY model"; $result = mysql_query($query); while($row = mysql_fetch_assoc($result)) { echo "{$row['mode']}<br>\n"; }
-
$query = "SELECT message FROM memberMail WHERE userIDFrom='92' AND unread='1'"; $result = mysql_query($query) or die(mysql_error()); //Display number of unread messages $num_rows = mysql_num_rows($result); echo "You have ($num_rows) unread message(s)."; //If there are unread messages, display them if (mysql_num_rows($result) > 0) { while($row = mysql_fetch_assoc($result)) { echo "<p>{$row['message']}<p><br>\n"; } }
-
EDIT:requinix beat me to it, but I have additional useful info, so I'll leave this post anyway. You have an array - use foreach() instead of while() with a variable you have to increment. Your while() condition is while($i<=$count) and $i starts at 0. So, if the file contains 10 lines you start with $i = 0 and continue the loop while $i <=10. That would mean the loop would go 11 times. That extra iteration will lead to problems. The specific problem is that you have to process the WHOLE file before you can determine that it was not found! $search_id = "meyersg"; $file = "bio.txt"; $lines = file(file); $member_found = false; foreach($lines as $line) { $row = explode(";", $line); if($row[0]==$search_id) { $member_found = true; echo "{$row[1]}</br>{$row[2]}</br>{$row[3]}</br>\n"; } } if(!$member_found) { echo "Member not found."; }
-
Mysql Question: Loading database info from more than one table.
Psycho replied to Hall of Famer's topic in PHP Coding Help
If the passwords are already hashed with MD5() how do you plan to update them since you don't (or shouldn't) have the original value? The only way I can see this working is if the first part of your new process is to do an MD5() hash and then do additional modifications. So, when you update all the current passwords you would perform just the additional modifications and not the initial MD5(). By the way, from my understanding, running multiple hashes on the same value can lead to a less secure value. So, you might want to check some sites for best practice processes before you go through with this change. I just thought of something. If doing a mass update will not work for the new process you want to implement there is another solution you can do. Update your login script to update the hashed passwords when the user's log in. 1. Create a new column in the user table called "updated_hash" set the default value to 1, but then modify all the current records to have a value of 0. That way when new user accounts are created they will have a value of 1 (since they will be hashed with the new method) but existing users will have a value of 0. 2. Modify the login script so it converts the entered passwords in both formats and checks for a matching record using both values AND have it return the value for "updated_hash". 3. If authentication passes and the value of "updated_hash" is 1 no addition steps are needed. But, if the value is 0, then you continue as follows: 4. Update the stored password hash for the user record with the hashed value using the new method and change the value of "updated_hash" to a 1. As users log in their passwords will be updated to the new hashed format automatically. You could then wait a few weeks/months to see if all of the records have been updated and then remove the "updated_hash" column and the additional functionality in the authentication script. -
How do you figure that? If your url is loaded in an iframe it is still being "visited" by the user. The user's browser is still making the request and displaying the page. But, ok, let's just say you want to prevent your page from being loaded in an iframe. Just do a Google search for "javascript break out of frames". There are plenty of ready made script to make a page "break out" of a frame if the browser loads it into one. Is it foolproof? Not be any means, but it should prevent the majority of users that want to do that. But, be assured that someone with even a modicum of programming skills would be able to programmatically load the content of your page without that javascript code being implemented.
-
I don't use MVC, so I can't speak to that. But, using your customer form as an example, this is the process I would use: The user points their browser to newcustomer.php. We will call that the controller file for this process. Typically, I just set some parameters in the pages that the user accesses and then include a "master" controller file, but this will do. OK, this controller file will first do a check to see if the form has been submitted. If not, it will include a separate file that has the actual HTML form (with maybe some minor PHP logic). And then it ends. However, if the form was submitted the controller file can then validate the form data and attempt to create the client. When writing procedural code I will, of course, do the validation in that page before attempting to create the new record. But, if I am using a class I will do most/all of the validation in the class. I would just pass the variables from the form post to the class method to create the customer. If the method determines that there is an error it will generate an appropriate error message back to the controller script. OK, no matter how I do the validation, there are two possibilities. Either validation fails or it passes and the record is created. If validation fails, I will again include the form page. Some of the logic I spoke of previously would repopulate the form with the values the user previously submitted and also display an error message as to what needs to change. If validation passes, then I may do something entirely different. Perhaps I display a confirmation page or I might display a summary list of customers. Either way, the controller script will determine that the customer was created and include the appropriate page to be displayed (which might also be a controller script). One note about the form page. I could also use that page for editing purposes. That is why it may include some logic. Based upon the parameters set it may need to include a hidden field for the customer ID begin edited and there might need to be some minor text differences on the page based upon whether the user is creating or editing. You can only edit your posts for a certain amount of time after creating them
-
But, that is not how the OP is using "closed". Closed is not the field with a 1 representing that the doors are closed (true). He is using a boolean for the field "status" and then the logic in the code uses a TRUE value for "status" to represent "Close" and a FALSE "status" value to represent "Open". I'll concede that there could possibly be a logical justification for that. but, I'd be willing to bet that is not the case here.
-
while loop mysql_fetch_array single output
Psycho replied to undertaker333's topic in PHP Coding Help
No. That line does not output anything. It simply defined a variable called $comments. So, on the first iteration of the loop it sets $contents equal to the variables from the first record. On the second iteration it OVERWRITES the contents fo the first record with the content of the second records. So, when the loop completes it holds the contents of only one record - the last one! So, if you are only using the variables in $comments, why do you have those other variables defined in the loop (e.g. $serials) that are not used? Anyway, you can "concatenate" the output for each record onto the results of the previous record using ".=" when defining the variable. That means to add the text to the end of the current value. $query = "SELECT name, msg, date FROM comment"; $result = mysql_query($query); $comments= ''; while($row = mysql_fetch_assoc($result)) { $comments .= "{$row['name']}<br />{$row['msg']}<br />{$row['date']}<br /><br />\n"; } -
This topic has been moved to MySQL Help. http://www.phpfreaks.com/forums/index.php?topic=342459.0
-
What kind of MySQL field are you using to store the value? I fusing one of the date type fields then the format has to be very specific. http://dev.mysql.com/doc/refman/5.1/en/datetime.html [Moving to MySQL forum]
-
This has obviously no impact, and as long as you remember your own logic everything will always work, but I just thought I would mention that when using Booleans (value 1 or 0), the "normal" logic is that 0 is false, closed, inactive, no, negative, etc... and 1 is true, open, active, yes, positive, ... You seem to be using the inverse: 1 for closed, 0 for open. That really depends on the context of the output. If "closed" is the desired value, or the result of a successfull operation then 1 would be more applicable. "Desired value", what's that have to do with it? Booleans are logical values that have specific meanings. I agree with WebStyles on this one. A '0' for closed makes much more sense.
-
Mysql Question: Loading database info from more than one table.
Psycho replied to Hall of Famer's topic in PHP Coding Help
I think you need to explain what, exactly, you are trying to achieve. If you have tow separate tables that are not related and you need a record from each table, then why do you not want the data in two separate variables? As to your original question: That is why you SHOULD NOT use variables such as $row1, $row2, etc. Give your variables meaningful names so that you, or anyone else, will ahve a good idea of what the variable holds. And, mysql_fetch_array() returns more than an "associative array indexed by column names". It also contains all the fields numerically indexed. I've never seen a reason to use mysql_fetch_array(), IMHO I think mysql_fetch_assoc() is a better alternative - it ONLY returns an array indexed by the field names. Using your example above, I would do something like this: $query = "SELECT * FROM {$usertable} WHERE user_id = '{$userid}'"; $result = mysql_query($query); $userdata = mysql_fetch_assoc($result); $query = "SELECT * FROM {$clienttable} WHERE client_id = '{$clientid}'"; $result = mysql_query($query); $clientdata = mysql_fetch_assoc($result); If you have multiple people working in the code all of them should understand the difference between $userdata and $clientdata. That is why it is important to have coding standards in an organization. Although using classes would make all of this pointless. -
For web development the preferred approach is to separate the various components of the application. You can do this a little or a lot. I'm terrible at terminology, but I'll try to give a basic explanation. There are tons of tutorials and resources on the net that would do a better job than I. Just be sure to check a few different sites as not all the info out there is good. Here is a typical structure: Controller File: this file will be used to determine what the user has selected and what they are trying to do (user has requested a list of users, user is trying to add a product to their order, etc.) That file will determine the "logic" file to load to actually perform the action. Logic file, this file contains the code to perform the actions that the user requested. This file may also utilize other types of files (data abstraction layer, classes, etc.) Using a Data abstraction layer provides many benefits. For example, you can change to a different database with little effort. Classes (part of OOP) allow you to centralize the functions for working with specific objects (clients, users, orders, etc.) Presentation: These are the files that create the actual output to the browser. By separating the presentation from the logic you can use teh same logic and repurpose it to different "presentation" outputs: web page, rss feed, xml, smart phone, etc.
-
This topic has been moved to JavaScript Help. http://www.phpfreaks.com/forums/index.php?topic=342456.0
-
while loop mysql_fetch_array single output
Psycho replied to undertaker333's topic in PHP Coding Help
Well, you didn't show the code that outputs the data, so I don't know what to put inside the loop. You simply need to move the code that outputs the data inside the loop. -
Like/dislike buttons - Harder than it sounds!
Psycho replied to mcfmullen's topic in Javascript Help
I didn't put anything in to save changes to the Like/Dislike options. Since this will happen in real-time you will need to implement AJAX to save those selections without refreshing the page. Sorry, but I'm not going to write all this for you. -
Like/dislike buttons - Harder than it sounds!
Psycho replied to mcfmullen's topic in Javascript Help
Pull the data from the database and when you create the input fields set their values accordingly. For example, for the posts I would assume you are pulling the data from a database query and creating the post text and the radio button options in a while loop. Here is an example of how you could create the radiio buttons and select the appropriate one. $postIndex = 0; while($row = mysql_fetch_assoc($result)) { $postIndex++; $likeChecked = ($row['like']==1) ? ' checked="checked"' : ''; $dislikeChecked = ($row['like']==0) ? ' checked="checked"' : ''; echo "<div id=\"post_{$postIndex}\" class=\"post\">\n"; echo " <b>{$row['title']}</b><br>\n"; echo "{$row['post_content']}<br>\n"; echo " <input type=\"radio\" name=\"like_{$postIndex}\" value=\"1\" onclick=\"toggleLayer(this.form);\"{$likeChecked}>Like\n"; echo " <input type=\"radio\" name=\"like_{$postIndex}\" value=\"0\" onclick=\"toggleLayer(this.form);\"{$dislikeChecked}> Dislike\n"; echo "</div>\n"; } -
Whew! That's a relief. I don't think I could go on living if you weren't able to add arrays to integers.
-
What? You stated "Both of my variables are integers.". But, now you say you are trying to add an array to a number. I don't even know what that means. You might as well state you are trying to add the color 'blue' to a school of fish. It is illogical. You can add a "value", or "values", in the array to a number. You'd better show what the contents of the array are and what your expectations when "adding" the array to the number are.
-
Use var_dump() on the variables being added and post what the results are for each one.
-
Like/dislike buttons - Harder than it sounds!
Psycho replied to mcfmullen's topic in Javascript Help
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.1//EN" "http://www.w3.org/TR/xhtml11/DTD/xhtml11.dtd"> <html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en-NZ"> <head> <style> .post { border-bottom: 1px solid black; margin-bottom: 10px; } </style> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8" /> <title>Unhide on checkboxes/radio buttons</title> <script type="text/javascript"> function radioGroupValue(groupObj) { //Check if there is only one option (i.e. not an array) if (!groupObj.length) { return (groupObj.checked) ? groupObj.value : false; } //Multiple options, iterate through each option for (var i=0; i<groupObj.length; i++) { if (groupObj[i].checked) { return groupObj[i].value; } } //No option was selected return false; } function toggleLayer(formObj) { var showLikes = document.getElementById('show_likes').checked; var showDislikes = document.getElementById('show_dislikes').checked; var postIndex = 1; while(document.getElementById('post_'+postIndex)) { var liked = radioGroupValue(formObj.elements['like_'+postIndex]) var display = ((!showLikes && liked==='1') || (!showDislikes && liked==='0')) ? 'none' : ''; document.getElementById('post_'+postIndex).style.display = display; postIndex++; } } </script> </head> <body> <form action="" method="post"> <fieldset> <legend>Unhide Layer Form</legend> <ul> <li><label for="b1">Show Likes:</label> <input id="show_likes" name="show_likes" type="checkbox" value="1" checked="checked" onclick="toggleLayer(this.form);" /></li> <li><label for="b1">Show Disikes:</label> <input id="show_dislikes" name="show_dislikes" type="checkbox" value="1" checked="checked" onclick="toggleLayer(this.form);" /></li> </ul> </fieldset> <br><br> <div id="post_1" class="post"> <b>Post #1</b><br> Content of post #1<br> <input type="radio" name="like_1" value="1">Like <input type="radio" name="like_1" value="0" onclick="toggleLayer(this.form);"> Dislike </div> <div id="post_2" class="post"> <b>Post #2</b><br> Content of post #2<br> <input type="radio" name="like_2" value="1">Like <input type="radio" name="like_2" value="0" onclick="toggleLayer(this.form);"> Dislike </div> <div id="post_3" class="post"> <b>Post #3</b><br> Content of post #3<br> <input type="radio" name="like_3" value="1">Like <input type="radio" name="like_3" value="0" onclick="toggleLayer(this.form);"> Dislike </div> <div id="post_4" class="post"> <b>Post #4</b><br> Content of post #4<br> <input type="radio" name="like_4" value="1">Like <input type="radio" name="like_4" value="0" onclick="toggleLayer(this.form);"> Dislike </div> <div id="post_5" class="post"> <b>Post #5</b><br> Content of post #5<br> <input type="radio" name="like_5" value="1">Like <input type="radio" name="like_5" value="0" onclick="toggleLayer(this.form);"> Dislike </div> <div id="post_6" class="post"> <b>Post #6</b><br> Content of post #6<br> <input type="radio" name="like_6" value="1">Like <input type="radio" name="like_6" value="0" onclick="toggleLayer(this.form);"> Dislike </div> <div id="post_7" class="post"> <b>Post #7</b><br> Content of post #7<br> <input type="radio" name="like_7" value="1">Like <input type="radio" name="like_7" value="0" onclick="toggleLayer(this.form);"> Dislike </div> </form> </body> </html> -
Using a separate table is the proper way to do it. It does not make the database un-tidy; it makes it normalized. You are not going to find an efficient or accurate way to query the tags if they are stored in a delimited field that way. Once they are in a separate table, it is very simple to determine the number of posts associated with each tag. As it is, you will have to read every post in the database to get a tag count, and you will have to read every post in the database to find all posts associated with a specific tag. This will put an unnecessary load on the database. Having a normalized database will actually result in less of a load when looking for tag counts, or posts associated with a tag, or any other query against the tags. Yeah, what he ^^ said
-
while loop mysql_fetch_array single output
Psycho replied to undertaker333's topic in PHP Coding Help
Use freaking CODE or PHP tags to post your code! $comments= "";$result = mysql_query("SELECT * FROM comment"); while($row = mysql_fetch_array($result)) { $serials= $row['serial']; $names= $row['name']; $emails= $row['email']; $msges= $row['msg']; $date_added= $row['date']; $comments= "$names <br /> $msges <br /> $date_added"; } Well, you are looping through the results of your query and assigning the values to variables. But, you are not using those variables in the loop, so on each subsequent iteration of the loop it is overwriting the values using the current record. So, you must have some code after that loop that is echoing the $comment. But, since you are doing that AFTER the loop it is only showing you the last value.