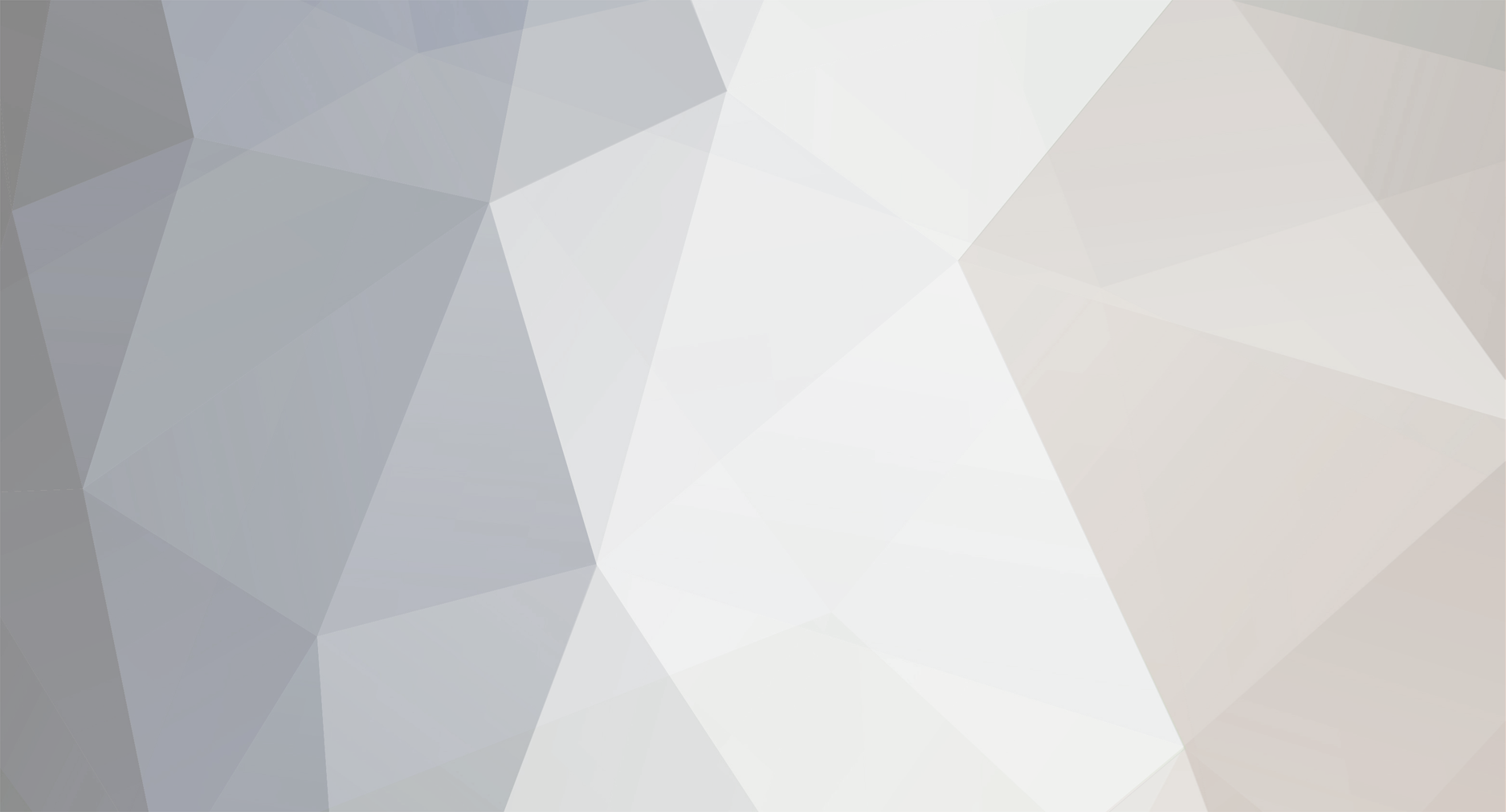
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
There are lots of problems with your code. I have gone through and completely reworked the structure, but not resolved all the problems. Here are some issues: 1. You have a die() clause for the main success scenario! Redirect the user to a confirmation page that their account was created 2. The verification error check and the actual error messages are widely separated. Don't make it hard on yourself put the error messages where the error check is. 3. FONT tags have been deprecated for YEARS! Stop using them and use style properties. 4. No validations for processing errors (query errors or send mail errors) I also changed the form so it will repopulate with the submitted data if there are validation errors> there is a lot more I would change, but don't have the time. But, the structure is much more logical and easier to read. I haven't tested it (since I don't have your DB), so there might be some typos/syntax errors <?php $title = "Register"; $errors = array(); $errorMsg = ''; if (isset($_POST['submitbtn'])) { $firstname = fixtext($_POST['firstname']); $lastname = fixtext($_POST['lastname']); $username = fixtext($_POST['username']); $email = fixtext($_POST['email']); $password = fixtext($_POST['password']); $repassword = fixtext($_POST['repassword']); $youtube = fixtext($_POST['youtube']); $bio = fixtext($_POST['bio']); $name = $_FILES['avatar']['name']; $type = $_FILES['avatar']['type']; $size = $_FILES['avatar']['size']; $tmpname = $_FILES['avatar']['tmp_name']; $ext = substr($name, strrpos($name, '.')); //Validate input if ($firstname && $lastname && $username && $email && $password && $repassword) { $errors[] = "You did not fill in all the required fields."; } elseif ($password == $repassword) { $errors[] = "Your passwords did not match."; } elseif (strstr($email, "@") && strstr($email, ".") && (strlen($email) >= 6)) { $errors[] = "You did not enter a valid email."; } else { //Initial input has been validated, check for duplicates require("scripts/connect.php"); $query = "SELECT * FROM users WHERE username='$username'"; $result = mysql_query($query) or die(mysql_error()); if (mysql_num_rows($resutl) != 0) { $errors[] = "That username is already taken."; } $query = "SELECT * FROM users WHERE email='$email'"; $result = mysql_query($query) or die(mysql_error()); if(mysql_num_rows($resutl) != 0) { $errors[] = "That email is already taken."; } } //If there were errors define error message(s) if(count($errors)>0) { //Define the error message $errorMsg = "The following errors occured:<ul>\n"; foreach($errors as $errorTxt) { $errorMsg .= "<li>{$errorTxt}</li>\n"; } $errorMsg .= "</ul>\n"; } else { //There were no errors, process the data $pass = md5(md5($password)); $date = date("F d, Y") $avatar = "defavatar.png"; if ($name) { $avatar = $username.$ext; move_uploaded_file($tmpname, "avatars/$avatar"); } $code = rand(23456789,98765432); $query = "INSERT INTO users VALUES ('', '$firstname', '$lastname', '$username', '$email', '$pass', '$avatar', '$bio', '$youtube', '', '0', '$code', '0', '$date')"; $result = mysql_query($query) or die(mysql_error()); //gets the next id in the users table $lastid = mysql_insert_id(); $to = $email; $subject = "Activate your Account"; $headers = "From: admin@mywebsite.com"; $body ="Hello $firstname,\n\n You need to activate your account with the link below: http://www.mywebsite.com/activate.php?id=$lastid&code=$code \n\n Thanks"; //function to send email if(mail ($to, $subject, $body, $headers)) { //Email was sent header("successpage.php"); } else { $errorMsg = "Unable to send registration email."; } } } ?> <?php require("styles/top.php"); ?> <div id='full'> <span style="color:red;"><?php echo $errorMsg; ?></span> <br><br> <form action='register.php' method='post' enctype='multipart/form-data'> <table cellspacing='10px'> <tr> <td></td> <td><font color='red'>*</font> are required</td> </tr> <tr> <td>First Name:</td> <td><input type='text' name='firstname' class='textbox' size='35' value='<?php echo htmlentities($_POST['firstname']); ?>'><font color='red'>*</font></td> </tr> <tr> <td>Last Name:</td> <td><input type='text' name='lastname' class='textbox' size='35' value='<?php echo htmlentities($_POST['lastname']); ?>'><font color='red'>*</font></td> </tr> <tr> <td>Username:</td> <td><input type='text' name='username' class='textbox' size='35' value='<?php echo htmlentities($_POST['username']); ?>'><font color='red'>*</font></td> </tr> <tr> <td>Email:</td> <td><input type='text' name='email' class='textbox' size='35' value='<?php echo htmlentities($_POST['email']); ?>'><font color='red'>*</font></td> </tr> <tr> <td>Password:</td> <td><input type='password' name='password' class='textbox' size='35'><font color='red'>*</font></td> </tr> <tr> <td>Confirm Password:</td> <td><input type='password' name='repassword' class='textbox' size='35'><font color='red'>*</font></td> </tr> <tr> <td>Avatar:</td> <td><input type='file' name='avatar'></td> </tr> <tr> <td>Youtube Username:</td> <td><input type='text' name='youtube' class='textbox' size='35' value='<?php echo htmlentities($_POST['youtube']); ?>'></td> </tr> <tr> <td>Bio/About:</td> <td><textarea name='bio' cols='35' rows='5' class='textbox' value='<?php echo htmlentities($_POST['bio']); ?>'></textarea></td> </tr> <tr> <td></td> <td><input type='submit' name='submitbtn' value='Register' class='button'></td> </tr> </table> </form> </div> <?php require("styles/bottom.php"); ?>
-
I'm not sure what the code above is supposed to illustrate with regards to your request. But, here is a simple function that would allow you to insert a new value into an array at a specified position. You can use positive values to determine position from beginning of array or negative values to determine position from end of array function array_insert(&$array, $newValue, $index) { $index = (int) $index; $array = array_merge( array_slice($array, 0, $index), (array) $newValue, array_slice($array, $index) ); } Usage: $test = array('one', 'two', 'three'); echo "Before:<br>\n"; print_r($test); array_insert($test, 'foo', 1); echo "After:<br>\n"; print_r($test); Output Before: Array ( [0] => one [1] => two [2] => three ) After: Array ( [0] => one [1] => foo [2] => two [3] => three )
-
Sorry, I'm not going to read through all that code. But, just looking at the first code block and your response, I will make the following suggestion. Don't use PHP timestamps as your value for the records. You then lose the ability to use the built-in functions within MySQL to work with data by date. You are making this harder than it needs to be. Just create a MySQL datetime or timestamp field that is autopopulated with the current timestamp when the record is created. Then you don't even need to include the field in your INSERT statements. But, if you want to stick with what you have: This will delete all records older than $mins mysql_query("DELETE FROM `main` WHERE `Timestamper` < '$mins'"); This will SELECT all records older than $mins $result = mysql_query("SELECT * FROM main WHERE `Timestamper` < '$mins'", $link); If you are running the DELETE before the SELECT then you will get nothing because you just deleted all the records you are trying to select. But, going back to my original statements in this thread, why are you trying to delete all the records older than 10 minutes? Let them stay and only select the records that are UP TO 10 minutes old. Then - if you need to - implement a weekly (?) process to purge old records.
-
OK, I would use PHP to do all the logic and remove the dynamic creation of 'Timespent' from the database query - you can get what you need just using $row['date_created']. Here is a function that you can use to get a text description for when the record was created. Just pass $row['date_created'] to the function. The function will return an appropriate value based upon whether the record was created seconds, minutes, hours or days ago. Currently, it will show 1 day (or n days), but you can change that the the date by un-commenting the line shown and removing the one after it. function timeSince($timestamp) { $minute = 60; $hour = $minute * 60; $day = $hour * 24; $secondsSince = time() - $timestamp; if($secondsSince >= $day) { $daysSince = floor($secondsSince/$day); $s = ($daysSince>1) ? 's' : ''; //return date('F d Y', $row['date_created']) return "{$daysSince} day{$s} ago."; } if($secondsSince >= $hour) { $hoursSince = floor($secondsSince/$hour); $s = ($hoursSince>1) ? 's' : ''; return "{$hoursSince} hour{$s} ago."; } if($secondsSince >= $minute) { $minutesSince = floor($secondsSince/$minute); $s = ($minutesSince>1) ? 's' : ''; return "{$minutesSince} minute{$s} ago."; } $s = ($secondsSince>1) ? 's' : ''; return "{$secondsSince} second{$s} ago."; }
-
If I am understanding you correctly, what you want can be achieved by using a JOIN with the appropriate conditions. I am by no means an expert, but I consider myself very competent in database queries. To say "I know how to use JOIN" really doesn't indicate one's level of expertise. There are many types of JOINs and virtually a limitless numbers of ways to use them. It is using JOINs in the right way that indicates a persons level of expertise. I asked you to provide an explanation of your database in hopes that I could provide a properly constructed query to get the data you want. Instead you decided to get an attitude and state "I know how to use join". Well, you obviously don't know how to use a while loop, so good luck to you.
-
You don't need to test every input - you only need to check one of them. Just put ALL of your form processing code within an IF condition if (isset($_POST['vnaam'])) { //Form was posted, process the POST data } else { //Form was not posted, do something else }
-
Then try harder. Don't cop out by making a lame excuse. I asked you to 1) show your table layout and 2) explain your requirements better. You only attempted to provide #2. Yes, a JOIN is what you want to do here. But, I can't provide good advice without knowing your database structure. I will provide a sample query using some wild guesses on your database structure and what I think your requirements are SELECT * FROM $datebase1 LEFT JOIN $memberreview_table ON $datebase1.reviewID = $memberreview_table.reviewID WHERE series_name LIKE '$start_letter%' AND $memberreview_table.memberName <> $loggedInUserName ORDER BY series_name
-
What is the value of $row['TimeSpent']? It is a mysql Timestamp, a PHP timestamp or what? I'm not sure I even understand what it's purpose is. You have a field called $row['date_created'] - isn't that the value you should be using for your calculations? Edit: Same question for $row['date_created'] as to field type/value.
-
A while loop will only continue as long as the conditions for the loop are true. With the loop you have above the loop will continue until either one of the mysql_fetch_assoc() calls returns false. So, if one result has 5 records and the other has 5,000 records, the loop will exit after five records are extracted from both because the first one will return false. You say I really don't see how you are able to make that distinction using two separate, unrelated queries. You likely need to use a JOIN and just do ONE query.But, since I really don't understand your requirements or your table stricture I can't provide any suggestions. Please show the table layout and restate your requirement above and/or give an example.
-
if true, $var = this image. if false, $var = that image.
Psycho replied to RyanSF07's topic in PHP Coding Help
Why do you need $favorite and $fav? It seems all you are doing is defining $fav to be the exact same value as $favorite with some added line breaks. Just use $favorite in your template or use the if/else to define $fav. But, looking at the code provided, I see no reason why you would get two images. The problem is elsewhere. Do you possibly have both $favorite and $fav in your template? -
What kind of field is 'Timestamper'? A MySQL timestamp field is a different type of value than a PHP timestamp - so you can't compare them directly, you will need to convert one or the other. Also, that logic seems backwards. That query is trying to pull all records LESS than '$mins' (which is now - 60 seconds) - which would be all records OLDER than '$mins'. Please state what field type 'Timestamper' is and show the code you are using to set it or are you just using the MySQL auto-opulate with the date the record is created?
-
you are using a frame for this content, you are not using PHP (or some other server-side technology) that allows you to dynamically control content. Therefore, the snippets of code you have above will not help you. Frames have been discouraged by those knowledgeable in web development for many, many years now. You should really reconsider rebuilding what you have. However, I'm not going to rebuild your site in this post, so I'll try to provide a solution - although it is sort of a hack. On the main page that includes the iframe add a line of PHP code to save a cookie. setcookie("ShowFile", 1); Do not set an expiration for the cookie. That way it should expire as soon as they close their browser. Then on the page that is loaded ito the iframe, just check if the cookie is set or not if(isset($_COOKIE['ShowFile']) { echo "You must access this content from the main page."; } else { //Show normal content } Now, this is by no means secure. Someone could get around this if they really wanted to, but it should prevent direct access for the majority of users. Also, anyone could directly load the file IF they have already loaded the main page.
-
I'm not sure I totally follow your workflow, but I *think* I see the problem. It would have been helpful if you provided the names of the files that those scripts are in so we could understand what code was being included()ed where. But, this is what I think is happening. The first script above has a form with an action tag that submits to the third script above. The third script has an include tag for the 2nd script. If that's the case, the problem is here: if (($_SERVER['REQUEST_METHOD'] == 'POST' && (empty($_POST['naam']) || !empty($familienaam_fout) || ... You POSTed the form from the first script so, "$_SERVER['REQUEST_METHOD'] == 'POST'" is true. I assume that check is to see if you POSTed the form in the second script not the form from the first script. The solution should be pretty simple. Instead of checking if ANYTHING was POSTed check to make sure something from that second form is posted if ((isset($_POST['vnaam']) && (empty($_POST['naam']) || !empty($familienaam_fout) || ...
-
if ($pos_x < 30 || $pos_x > 240 || $pos_y < 30 || $pos_y > 240) { echo "You hit a wall."; }
-
Hmm, the first one doesn't work and the second one does. I wonder which one is correct? When using and AND or OR, the parts on each side of that clause are interpreted individually. So, in this case: if($action !== 'delete' || 'edit') It is saying if [$action !== 'delete' ] is true OR if ['edit'] is true. It doesn't know you wanted to compare 'edit' to $action. Each clause of the condition stands on it's own. In this case (with only two options), a more simple approach would be to simply check for one value and if not that value then choose the other. I would default to the edit. $action = (isset($_POST['action']) && $_POST['action']=='delete') ? 'delete' : 'edit'; Or, to make your code easier to read you could use in_array() which is also useful wehn you have more than two values if(!in_array($action, array('edit', 'delete'))) { echo "ERROR- you must choose an appropriate form Action. Please try again." }
-
What does your form look like? Doing the nested foreach loops probably is the wrong process, but the right approach would depend on the "context" of the data as it is entered onto the form.
-
insert multiple database table row from dynamic checkboxes from db
Psycho replied to sir_amin's topic in PHP Coding Help
I was thinking about that when you stated that these were checkboxes. I wasn't sure if this was a one-time operation or if you wanted to be able to edit the selections. If these are to be editable, then you should have the page create the page to create all the checkboxes and automatically have the ones checked where there are corresponding records. Now, when the page is submitted you should do a two-step process. First DELETE all the associated records for the lid (lesson ID?) then INSERT new records for the checkboxes that are checked. -
Fetch array from SQL database, sort, then add autoincrement column.
Psycho replied to delambo's topic in PHP Coding Help
OK, here you go. This is a completely functional script. The output is without any style, but I trust you can work on that. You can load this page at any time. It will give you a list of all the entrants and their currently assigned raft numbers (if they have been assigned one). If they have not been assigned a raft number it will show "UNASSIGNED". If there are any unassigned rafts there will be a button to assign raft numbers. You should only click that button when you are ready to generate those numbers (i.e. race day) otherwise if new entrants are added they will not be in the order by category. But, if you do get last minute entrants you can load this page and see that there are entrants that have not been given a number. The button will be available again and you can then assign a number to those last minute entrants that will start after the previous last number. Make sure you either change the variables at the top of the script of include your own code for database connection (and don't forget the table name). EDIT: For testing purposes just do a single update query to reset all the raft_number values to 0 to start over. <?php $server = 'localhost'; $uname = 'root'; $pword = ''; $dbName = 'test'; $tableName = 'entrants'; $link = mysql_connect($server, $uname, $pword); if (!$link) { die('Could not connect: ' . mysql_error()); } if (!mysql_select_db($dbName)) { die('Could not select database: ' . mysql_error()); } //Check if user selected to assign raft numbers if(isset($_POST['assign'])) { //Create and run query to get the highest currently assigned raft number $query = "SELECT MAX(raft_number) FROM {$tableName}"; $result = mysql_query($query) or die(mysql_error() . " <br>\n{$query}"); //Define last assigned raft number $raftNo = mysql_result($result, 0); //Create run query to get all unassigned rafts $query = "SELECT id FROM {$tableName} WHERE raft_number = 0 ORDER BY category, id"; $result = mysql_query($query) or die(mysql_error() . " <br>\n{$query}"); //Process results to generate list of "when" //clauses to be used in a single update query $WHEN_CLAUSES = ''; while($row = mysql_fetch_assoc($result)) { $raftNo++; $WHEN_CLAUSES .= " WHEN {$row['id']} THEN {$raftNo} \n"; } //Create and run query to assign raft numbers $query = "UPDATE {$tableName} SET raft_number = CASE id {$WHEN_CLAUSES} ELSE raft_number END"; $result = mysql_query($query) or die(mysql_error() . " <br>\n{$query}"); } //Display the current list of entrants $query = "SELECT raftname, category, raft_number FROM {$tableName} ORDER BY category, id"; $result = mysql_query($query) or die(mysql_error() . " <br>\n{$query}"); //Create output of all entrants $output = ''; while($row = mysql_fetch_assoc($result)) { $raftNo = ($row['raft_number']==0) ? 'UNASSIGNED' : $row['raft_number']; $output .= "<tr>\n"; $output .= "<td>{$row['raftname']}</td>\n"; $output .= "<td>{$row['category']}</td>\n"; $output .= "<td>{$raftNo}</td>\n"; $output .= "</tr>"; } //Run query for ONLY the rafts that have not been //assigned numbers and order the results by catory/id $query = "SELECT id FROM {$tableName} WHERE raft_number = 0 ORDER BY category, id"; $result = mysql_query($query) or die(mysql_error() . " <br>\n{$query}"); $unassigned_count = mysql_num_rows($result); //Create button to assign raft numbers (if needed) if($unassigned_count==0) { $assignButton = ''; } else { $assignButton = "<form method='post' action=''>\n"; $assignButton .= "<input type='hidden' name='assign' value='1'>\n"; $assignButton .= "<button type='submit'>Assign Raft Numbers</button>\n"; $assignButton .= "</form>\n"; } ?> <html> <head></head> <body> <table border="1"> <tr> <th>Raft Name</th> <th>Category</th> <th>Raft Number</th> </tr> <?php echo $output; ?> </table> <?php echo $assignButton; ?> </body> </html> -
Fetch array from SQL database, sort, then add autoincrement column.
Psycho replied to delambo's topic in PHP Coding Help
An auto-incrementing id is used for referential integrity of the database - especially when a foreign key is needed. You should not use the primary index for a sorting value. Now, I can provide a solution, but I see a problem with the requirements. As I understand the requirements the boat numbers will be ordered by category then by the order they registered. So, if you have four boats in category A they will be 1, 2, 3, 4. Then the boats in category B would start at 5. But, what happens when you need to add another boat in group A? Are there no "last minute" entries? If so, you would either have to assign that entry a number at the end of all the previously assigned numbers OR you would have to assign them a number in the order defined above and reassign numbers for all the ones that follow. If you can provide some information on how "last minute" entries should be handled I can help, but that decision will affect the solution I build. -
Do you know the timezone for the user? If so, converting a time to another timezone is a simple task. Ideally, I would store the time from GMT, but even if you have it stored in a different timezone you can still convert from that to another. But, the real question is whether you have captured the timezone for the user, such as having them select their timezone when they create an account. If you have not provided a means for the user to select their timezone, there are ways to auto-detect it via JavaScript, but I'm not sure how foolproof that is.
-
insert multiple database table row from dynamic checkboxes from db
Psycho replied to sir_amin's topic in PHP Coding Help
This part of the code is very inefficient: foreach ($_POST['gradeid'] as $i) { $insertSQL = sprintf("INSERT INTO term_lesson (lid, gradeid) VALUES (%s, %s)", GetSQLValueString($_POST['lid'], "int"), GetSQLValueString($i, "int")); mysql_select_db($database_register, $register); $Result1 = mysql_query($insertSQL, $register) or die(mysql_error()); } You have a loop to do multiple INSERTs individually. Running queries in loops is always a bad idea. Even so, there is no need to select the database each time or to rerun the validation for $_POST['lid'] each time. That code can be much more efficient. $lid = GetSQLValueString($_POST['lid'], "int"); $insertValues = array(); foreach ($_POST['gradeid'] as $gradeid) { $insertValues[] = sprintf("(%s, %s)", $lid, GetSQLValueString($gradeid, "int")); } mysql_select_db($database_register, $register); //Create ONE query to insert all the records $insertSQL = "INSERT INTO term_lesson (lid, gradeid) VALUES " . implode(', ', $insertValues); $Result1 = mysql_query($insertSQL, $register) or die(mysql_error()); -
Check that the $_POST['cat_to_chg'] variable actually has a value and that it exactly matches a value in the array. You also don't need to do a foreach loop, just use array_search() to see if the value exists $OLDcategory = trim($_POST['cat_to_chg']); $NEWcategory = trim($_POST['new_cat']); $cats = file("categories.txt"); echo "Old Category: '$OLDcategory'<br>\n"; echo "New Category: '$NEWcategory'<br>\n"; echo "Array Before<pre>".print_r($cats, true)."</pre><br>\n"; $cats = array_merge(array($NEWcategory), $cats); $oldIndex = array_searc($OLDcategory, $cats); if($oldIndex!==false) { unset($cats[$oldIndex]); } echo "Array After<pre>".print_r($cats, true)."</pre><br>\n";
-
If you need to re-purpose this functionality you need to build a function that will take parameters and perform the necessary operations accordingly. If you need to do the same thing more than once, build it once so that it can meet the needs of the multiple scenarios you need. Otherwise your code become hard to maintain. For example, if you have copied the same logic multiple times and you later find a bug in a certain module you will have to try and remember where you used that same functionality. Anyway, as to "how the array works" I'm not sure what you are asking. The real "work" in this code is done through the queries and I provided comments to explain what each query was doing. But, with respect to the arrays, they are simply a variable to hold the values from the queries using the record ID as the key and the populating of the value. Then later in the code it iterates through the respective arrays to display the values on the page. The one array that was built slightly different was the last one. For the records where the population is less than the max,I first query for those records. Then when building the array I calculate what the new population will be by using the lower of the two values (currentpopulation + towninc) or (maxpopulation). Then, I run a query to set those new values using the same logic in the MySQL query. Now, that is the most efficient method that I could think of, but it does have a problem. Although I am using the same logic to calculate what the new values will be in PHP (for display purposes) and in MySQL for the actual update - those are two different processes. To be 100% accurate you should probably run three queries: one to get the values that need to be updated, a second to update the values, and a third to do a select on the records from the first query to get their updated values.
-
OK, looking at your code, this is what I came up with. I basically see three "classes" of records: 1. Ones that are already at max population and should not be changed 2. Ones that are over max population and need to be reduced 3. Ones that are under max population and should be increased by 'towninc' UNLESS that would take them over the max population and in that case the population should be set at the max. None of this is tested since I don't have your database so there may be some syntax/typo errors, but the logic should be sound <?php function populationtick() /**Begin pull of all rows, then proceed to update each row for certain fields**/ { //Get list of towns already at max population $query = "SELECT `id`, `maxpop` FROM `users` WHERE `currentpop` = `maxpop`"; $result = mysql_query($query) or die (mysql_error()); $atMaxPopAry = array() while($town = mysql_fetch_assoc($result)) { $atMaxPopAry[$town['id']] = $town['maxpop']; } //Get list of towns over the max population $query = "SELECT `id`, `maxpop` FROM `users` WHERE `currentpop` > `maxpop`"; $result = mysql_query($query) or die (mysql_error()); $overMaxPopAry = array() while($town = mysql_fetch_assoc($result)) { $overMaxPopAry[] = [$town['id']] = $town['maxpop']; } //Reset current pop to max pop for those over max $query = "UPDATE `users` SET `currentpop` = `maxpop` WHERE `currentpop` > `maxpop`"; $result = mysql_query($query) or die (mysql_error()); //Get list of towns under the max population $query = "SELECT `id`, `currentpop`, `towninc`, `maxpop` FROM `users` WHERE `currentpop` < `maxpop`"; $result = mysql_query($query) or die (mysql_error()); $underMaxPopAry = array() while($town = mysql_fetch_assoc($result)) { $underMaxPopAry[$town['id']] = min(($town['currentpop']+$town['towninc']),$town['maxpop']); } //Increate current pop to (current pop + towninc) or max pop, whichever is less $query = "UPDATE `users` SET `currentpop` = IF(`currentpop`+`towninc`>`maxpop`, `maxpop`, `currentpop`+`towninc`) WHERE `currentpop` < `maxpop`"; $result = mysql_query($query) or die (mysql_error()); echo "The following towns were already at max population:<br>"; echo "<ul>\n"; foreach($atMaxPopAry as $townId => $pop) { "<li>{$townId} = {$pop}</li>\n"; } echo "<ul>\n"; echo "<br><br>\n"; echo "The following towns were over max population and were reduced to the max population:<br>"; echo "<ul>\n"; foreach($overMaxPopAry as $townId => $pop) { "<li>{$townId} = {$pop}</li>\n"; } echo "<ul>\n"; echo "<br><br>\n"; echo "The following towns under max population and were increased:<br>\n"; echo "<ul>\n"; foreach($underMaxPopAry as $townId => $pop) { "<li>{$townId} = {$pop}</li>\n"; } echo "<ul>\n"; } /**End Function **/ ?>
-
OK, I see a lot of things going on in that code and most of it is probably unnecessary. Can you explain in plain English what you are trying to accomplish? You don't need to run queries in loops, you can probably do all you need with just a couple of queries.