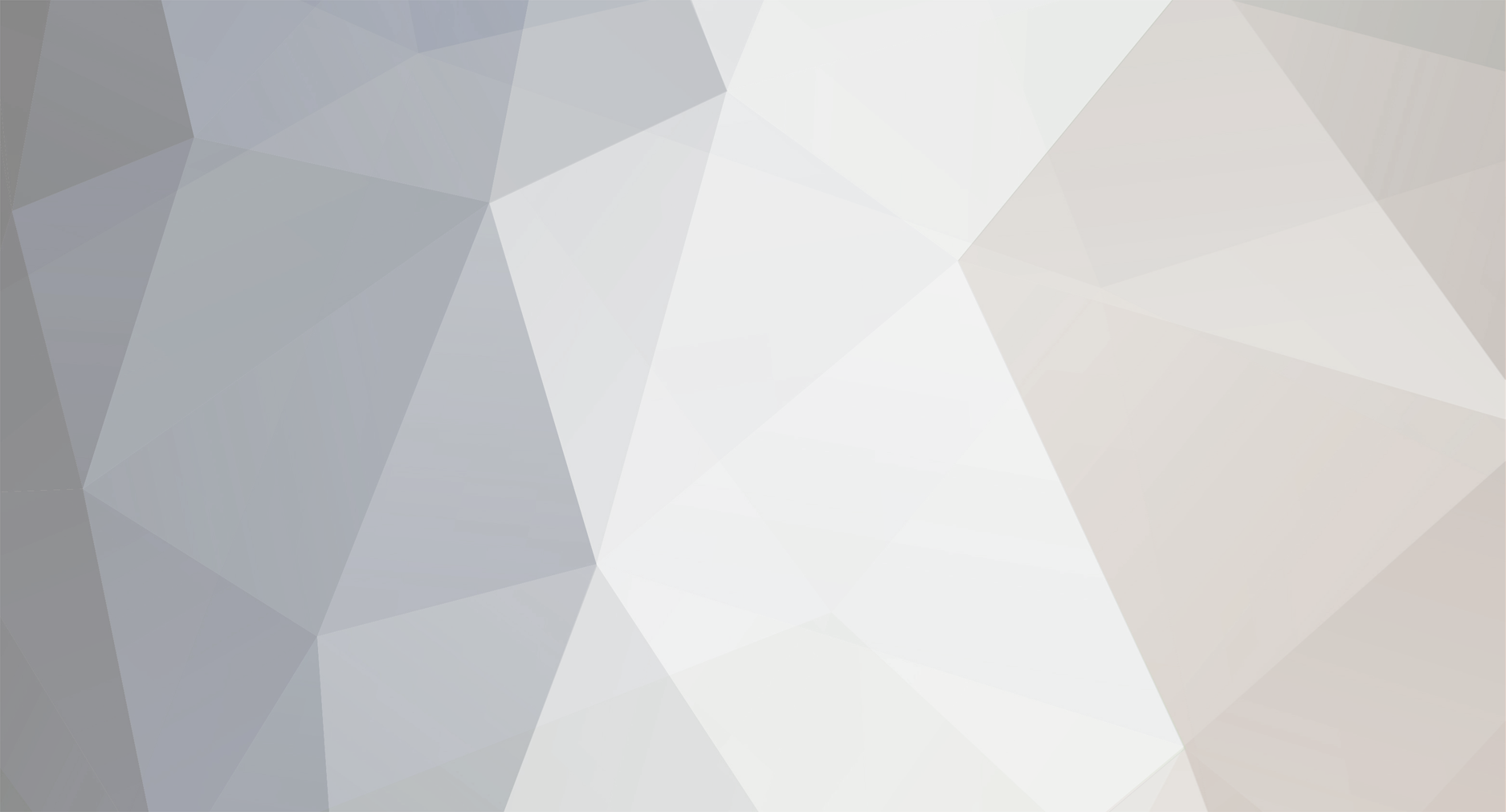
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
dynamically populate a form with database data
Psycho replied to esoteric's topic in PHP Coding Help
Yeah, but don't use $data to define $data. The only time that makes sense is if you are modifying a value to be of the same type. Anyway, I'm surprised you didn't get any errors. Also, do your products not have a unique ID? You should be passing THAT value and not the product name. And, when you populate the form you should have a hidden field for the product ID. If you use the product name as the key and the user changes the name how do you know what record to update!? So, change your select list so the ID is the value <?php $sql="SELECT ProductID, ProductName FROM Products"; $result =mysql_query($sql); $productOptions = ''; while ($data=mysql_fetch_assoc($result)) { $productOptions .= "<option value =\"{$data['ProductID']}\">{$data['ProductName']}</option>\n"; } ?> <select name="ProductID" id="ProductID"> <?php echo $productOptions; ?> </select> Then change your form script to actually use the value that is passed $productID = intval($_POST['productID']); $query = "SELECT * FROM Products WHERE ProductID = {$productID}"; $result = mysql_query($query) or die("Couldn't execute query. ". mysql_error()); $product_data = mysql_fetch_assoc($result); If you still get an empty form, do a print_r($_POST) and echo your query to the page to make sure they are what you expect. -
You should not run queries in loops! It is very inefficient. Looking for code to do what you want isn't a bad idea, but don't use it as a crutch. You should analyze the code to understand what it is doing. Then you can learn to write your own code when you need it. I have had several instances where I didn't know how to approach a problem and searched for some existing code to do what I needed. Then when I found some code I would analyze it step by step to understand the logic. In doing so I would usually find some error in the code. But, it was a good learning experience. The code you last posted is no different than what I proposed except you are running one query for each record instead of one query to insert all the records. Also, you should not use mysql_real_escape_string() on EVERY input. You should only use it on input for string values. If you are expecting a numeric value then validate the value accordingly. Likewise, you need to validate date fields properly if they are going to be stored as date and/or time values in the database (which they should). I didn't change the validations. I changed some of the validations. You shoudl create a function for validating the date input and converting to a mysql date value. And, you should include a switch to skip a record if it does not contain valid data. $insertVals = array(); foreach($_POST['student_id'] as $id => $Act) { $student_id = intval($Act); $student_name = mysql_real_escape_string($_POST['student_name'][$id]); $student_email = mysql_real_escape_string($_POST['student_email'][$id]); $teacher_name = mysql_real_escape_string($_POST['teacher_name'][$id]); $grp = mysql_real_escape_string($_POST['grp'][$id]); $date = mysql_real_escape_string($_POST['date'][$id]); $date_dd = mysql_real_escape_string($_POST['date_dd'][$id]); $date_mm = mysql_real_escape_string($_POST['date_mm'][$id]); $date_yy = mysql_real_escape_string($_POST['date_yy'][$id]); $att = intval($_POST['att'][$id]); //Need to add check to skip record if any required data is missing or is invalid $insertVals[] = "('$student_id', '$student_name', '$student_email', '$teacher_name','$grp','$date','$date_dd','$date_mm','$date_yy','$att')"; } //Create query to insert all the records $query = "INSERT INTO table (`student_id`,`student_name`,`student_email`,`teacher_name`, `grp`, `date`, `date_dd`, `date_mm`, `date_yy`, `att` ) VALUES " . implode(', ', $insertVals); mysql_query($query);
-
Give each input an array name such that it uses the student ID as the index. Then you can process all the fields associated with the student. And, by doing that you don't need the student ID field <input type="hidden" name="student_name[1]" value="'.$st_name.'" /> <input type="hidden" name="student_email[1]" value="'.$st_email.'" /> Then in the processing code $insertVals = array(); foreach($_POST['attend'] as $studentID => $attended) { $student_name = $_POST['student_name']['$studentID]; $student_email = $_POST['student_email']['$studentID]; $insertVals[] = "('$studentID', '$student_name', '$student_email', '$attended')"; } I only showed a couple of fields, but that should give you the idea. But, I don't see what you need those values in the processing code since I would assume they already exist in the DB. You should only need the student ID and the data that is new.
-
dynamically populate a form with database data
Psycho replied to esoteric's topic in PHP Coding Help
OK, to make this easy, create two pages: "select_product.php" and "edit_product.php". On the select_product.php page you would create a small form with nothing but a select list. Do a DB query to get a list of products names and their IDs. use those values to create the select list. That form should have the edit_product.php page as it's action. So, when the user selects a product from the select list and submits the form they will be redirected to the edit_product.php page along with the POST data (i.e. the selected product). Then on the edit_product.php page you would get the selected product from the POST data and do a DB query to get all the values for the product that you want to populate the form with. $query = "SELECT * FROM products WHERE product_id = $selectedProduct"; $result = mysql_query($query); $product_data = mysql_fetch_assoc(); For simple text inputs you would just set the value using what you retrieved from the DB, but be sure to run it through htmlentiteis(). $prod_name = htmlentities($product_data['name']); <input type="text" name="prod_name" value="<?php echo $prod_name; ?>" /> For radio buttons, checkboxes, or select lists you will need to dynamically check the DB value to determine how the form value should be set //Dynamically create select options for category $cat_list = array("Garden", "Home", "Gifts", "Pets"); $cat_options = ''; foreach($cat_list as $cat) { //Dynamically select the option already saved $selected = ($cat==$prod_cat) ? ' slected="selected"' : ''; $cat_options = "<option value=\"{$cat}\"{$selected}>{$cat}</option>\n" } <td><select name="cat"> <?php echo $cat_options; ?> </select></td> -
dynamically populate a form with database data
Psycho replied to esoteric's topic in PHP Coding Help
Well, if you want it truly dynamic then you will need to implement AJAX. But, I would suggest just implementing a PHP solution to start. So, you would have to select a value from the drop down and then submit the selection to get your form with all the values populated. I'll review your form and provide some sample code. -
I made this a function so you can reuse it and modify the output if needed. I don't think using <SUP> tags is the "proper" way to do this any more. function superscriptAllButFirst($string) { $index = strpos($string, ' ') + 1; $firstWord = substr($string, 0, $index); $remainingWords = substr($string, $index); return "$firstWord<sup>{$remainingWords}</sup>"; } $text = "Hello world I love you"; echo superscriptAllButFirst($text);
-
First off, let's start with your form. The radio buttons should look something like this: Student 1 <input type="radio" name="attend[1] value="1" /> Yes <input type="radio" name="attend[1] value="0" /> No Student 2 <input type="radio" name="attend[2] value="1" /> Yes <input type="radio" name="attend[2] value="0" /> No The number inside the name parameter will be the student ID. Then in your processing code you can iterate through all the POST data and generate a single INSERT statement to create all the records. $insertVals = array(); foreach($_POST['attend'] as $studentID => $attended) { $insertVals[] = "('$studentID', '$attended')"; } //Create and run INSERT query $query = "INSERT INTO attendance (`studentID`, `attended`) VALUES " . implode(', ', $insertVals); $result = mysql_query($query) or die (mysql_error()); This assumes the table has a date field that default to the current date when new records are created. Otherwise you need to generate the date in PHP and add it to the values.
-
How many colors are you allowing the user to select? Just one or are you going to have an advanced color picker. Also, what is the default color?
-
Um, no, that doesn't make sense either. That requires you to update that value whenever the member has an event that changes their credits. There is no need for that as long as you have a record of all the credits for the members. You can then determine the totals dynamically. You should have to track the ame values (or even the sum of the same values) in different places.
-
You just need a column for the account_type and another for the "modifier" or whatever makes sense for you. Looking at your sample code, the modifier for the 'abhimani_plus' account type would be (1/3000) or whatever that mathematical result is. So, why do you need an account balance field? You should be able to determine the account balance at any time by using the transactions. And, then you would just add the interest to the transactions table. Using your logic of updating the value of account balance with the interest amount how does anyone ever validate that the interest was applied since you would have no record of it?
-
I would agree that the switch statement might be a solution, but it would require a slightly unintuitive implementation since the switch value is compared to the case values. You can't, AKAIK, do a mathematical comparison between the value in the switch to the value in the case. But, there is a workaround that I have seen used before that will work: switch(true) { case ($val < 1000): echo "1"; break; case ($val > 3000): echo "2"; break; case ($val > 8000): echo "3"; break; case ($val > 16000): echo "4"; break; } However, a switch statement is kind of all or nothing. Once it finds a match it will perform all the code in the remaining case statements unless there is a break in which case it will stop. So, you can't have it find a match with one and then check if there is a match with the remaining. Per the original post he wants it to continue checking after the first match.
-
Your change will not produce different results than what you have. You state you want it to continue after the first condition is met. So, just have a list of IF statemetns (not ifelse) and each one will be interpreted individually. If you want more help give an example of some input values and what you need for the output.
-
Looking at your sample code it looks like the account type is used as a modifier for determining the interest. I would suggest creating an separate table to associate account types with the "modifier". Then you do not need to do all those IF statements and you can calculate ALL the interest changes in ONE query. Such as UPDATE account SET `account_balance`= ( `account_balance`+`account_balance`*`account_interest`* ( SELECT modifier FROM modifiers WHERE modifiers.account_type=account.account_type ) ) But, you are doing this wrong. You shouldn't be simply adding the interest to the account balance. Heck, you shouldn't have an account balance at all. You should have a table to track transactions. You would then calculate the account balance using the sum of the transactions. Then you can run the script to calculate the interest on the last day of the month or even several days after the end of the month and you could still calculate the correct interest based upon their account balance as of midnight on the last day of the month.
-
You're losing me. I understand that you want to get a list of users, that were referred by a specific user, and the sum of their credits. All you need is a single query to get the data you need. You don't need any arrays. I could provide a sample query, but the one thing that doesn't make sense is your reference above to "Jim's Referrals Table". You shouldn't have a different table for each person's referrals. Just use one table with a column for referrerID. You are makign this way harder than it needs to be. Assuming you had a referrerID column you would be able to get the data you want using a query such as SELECT members.name, SUM(events.amount) as earned FROM members JOIN events USING member_id WHERE members.referrerID = $referrerID GROUP BY members.memberID
-
I don't know of a good method to check for number of line breaks since they can be different between OSes and such. Your example above has "REGUK01" after the very first percent. I could provide a regex to find the very first percent in the file, but I have a suspicion that might not always be correct. Someone might have a regex solution for you, but I'm not sure how to work it out. The only solution I can come up with would be to iterate through each line. $lines = file('filename.txt'); foreach($lines as $index => $line) { if(strpos($line, 'REGUK01')!==false) { $percent = trim($lines[$index-2]); break; } } echo "Percent: {$percent}";
-
Without more info, I can't be certain this will fulfill your needs, but this might work for you: preg_match("#REGUK01.*?(\d{1,2}(\.\d+)?%)#s", $text, $match); $percent = $match[1]; Notes: 1. It finds the first "percentage" that follows after "REGUK01". So if you have a percentage on the same line it will find that one instead of the one on the next line. For that matter if the first match if after the next line it will find that as well. 2. It will match a "percentage" that is in any of the following formats: 1% 12% 1.2% 1.23% (or any number of digits following the decimal) 12.3% 12.34% (or any number of digits following the decimal) There must be one or two digits at the beginning. The decimal is optional and when it exist there must be one or more digits that follow after it.
-
If you could provide a couple of examples of the text that will be parsed it would be helpful. Is the percentage the only thing on the next line or is it embedded in other text. Is the percentage always in the format dd.d% or can it be a single digit (or no digits) before the decimal? Is there always 1 digit after the decimal? Can there be a percentage on the same line as "REGUK01"?
-
Good luck to you. I'm done with this post.
-
OK, I did not test that code - how could I since I don't have your database - so there may be some syntax/typos. I expect the person receiving the code I provide to be able to debug those types of errors. Assuming there were no syntax errors, or you fixed them, that error may be from MySQL. That is what has been stated several times now - that your query is failing. You have been asked several times to provide that error. If you want help, please provide the error. Even if it isn't the MySQL error we would probably be able to provide a solution to that error.
-
As was already stated previously you have no error handling on your query. Therefore, if the query fails there is no report that there was an error and the script continues on as if there were no problems. That is why you need to add error handling to your db queries. Displaying the raw output of mysql_error() to a user is not advised. But, for now, with developing your script go ahead and check if there was an error. If so, display the error to the page (and the query) to the page. You can work on implementing a more elegant error reporting system later. But, for now, I would say there is a 99% chance your query is failing. The error message generated would tell you what the problem is or at least point you in the right direction. But, since you are not displaying it or telling us what the error is we have no way of knowing what the error is. It could be something as simple as a typo of a field name or something more complex.
-
Define the function outside the loop then call it within the loop
-
PHP array checkbox help xD storing in database
Psycho replied to rmariano02's topic in PHP Coding Help
Your query is probably failing and you don't see it because you have no error handling. The reason it is probably failing is that checkboxes that aren't checked do not pass a value. So, you have some values in the query that are missing. Don't give your fields names such as 1, 2, 3. Give them names that correspond to their field names. That makes it much easier to debug/fix code. Also, don't store the value "check". That is a string value that you would have to constantly compare against a string. Use 1 (true) and 0 (false). Those values are logically handled as Boolean values. Here are some changes to make: 1. Change the checkboxes to something like this <input name="service[]" value="Facial" type="checkbox"> Facial</input> <input name="service[]" value="Diamond_Microderma" type="checkbox"> Diamond Microderma</input><br/> I forget if you should have quotes around the indexes in the HTML field names, but I don't think so. 2. In your processing of the input, be sure to generate a COMPLETE list of values for all services so you have an appropriate value for the query //Create an array of ALL services $services = array('Facial', 'Diamond_Microderma', 'PeelingsProcedure', ....); //use above array to create new array using services as key and default value of 0 $svc = array_fill_keys($services, 0); //Loop through each POST service and updates the values as needed foreach($_POST['services'] as $checkedService) { $svc[$checkedService] = 1; } Lastly change your query to use the new values and add some error handling $query = "INSERT INTO ff_tblreserve (Facial, Diamond_Microderma, PeelingsProcedure, Pimple_Treatment, Pimple_Injection, Acne_Surgery, Acne_prone_skin_microneeding, Advanced_stem_cell_microneeding_therapy, Needle_less_stem_cell_infusion, Needle_less_mesolift, Botox, Bio_hair_growth_stem_cell_infusion, RF_Figure_countour, Slimming_Massage, PT_Metabolic_Accelerator, Mesotherapy, Ultrasonic_Liposuction, Filler, Hair_Removal, Photorejuvenation, Hyperpigmentation, Acnecontrol, time, date, id) VALUES ('$svc['Facial']','$svc['Diamond_Microderma']','$svc['PeelingsProcedure']', . . ."; $result = mysql_query($query) or die ("Query:<br>\n{$query}\n<br>Error:<br>\n" . mysql_error()); -
Nope, he is defining the function in a while() loop Organize your code to prevent these types of errors.
-
Is that error with "timespent" or "timesince". "timespent" is the dynamically created MySQL value that you are suing which I suggested was not necessary. "timesince" is the function I provided. So, I am assuming the error is with that. But, I only see you defining that function once in the code above.