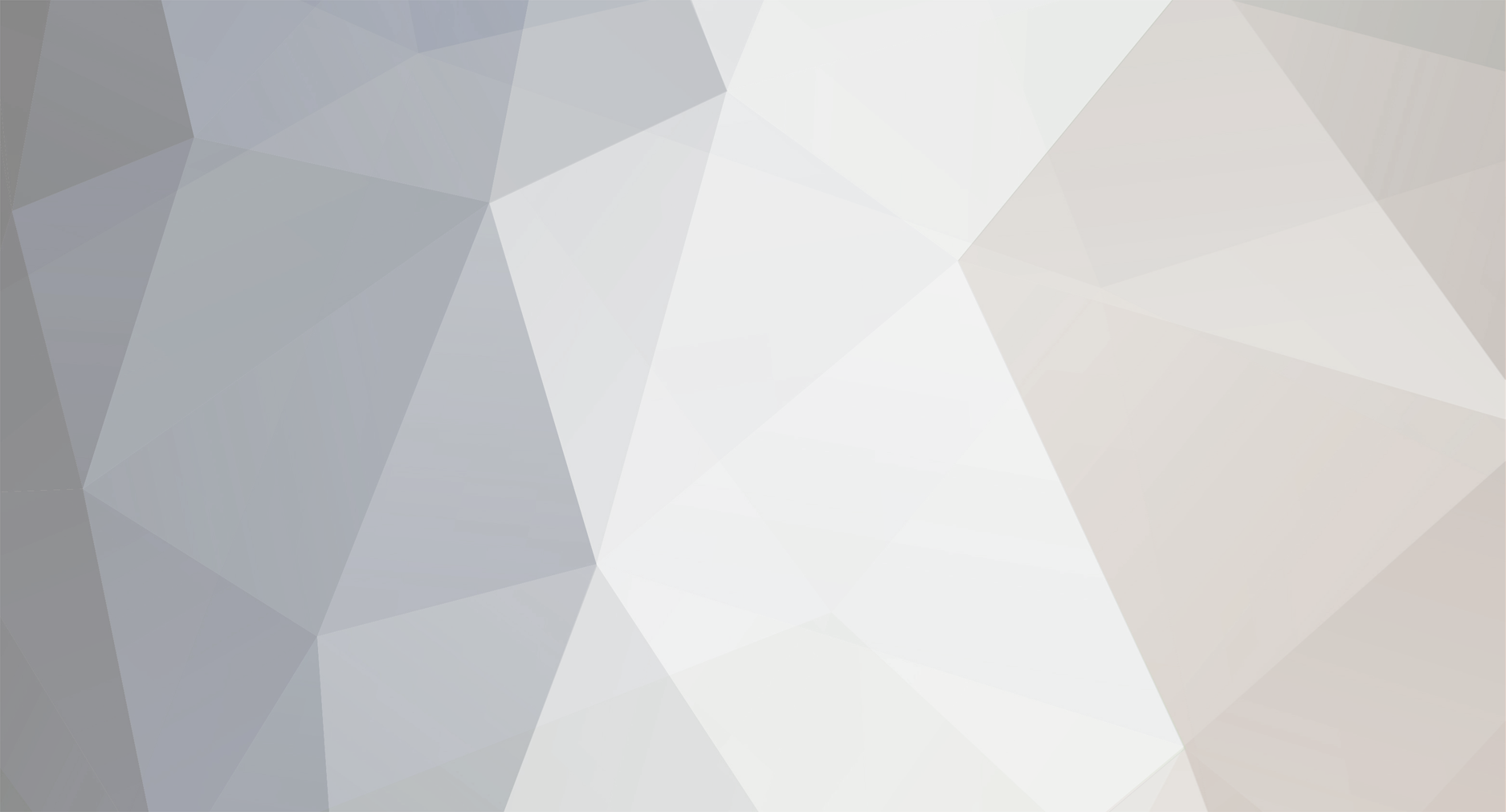
Psycho
Moderators-
Posts
12,159 -
Joined
-
Last visited
-
Days Won
130
Everything posted by Psycho
-
how do i get a variable stored on another page?
Psycho replied to Fenhopi's topic in PHP Coding Help
If the 2nd page is accessed directly after the first, such as with a link or a redirect. You can append the variable to the URL (e.g. somepage.php?foo=bar). But, if the pages are not accessed sequentially and/or the value needs to persist over time, then you need to save the value somewhere. The most common solution would be a database. But, if you don't have access to a database and your need is limited, you can simply write the value to a flat-file on your server. Then read it on the pages where you need to access it. Tutorial for file writing and reading: http://www.tizag.com/phpT/filewrite.php -
OK, I understand this problem very well, but find that wikipedia entry confusing. Quite simply, after you have processed the POST/GET data, simply redirect the user to a "success" page (e.g. Thank you for your submission") using "header('Location: success.php');". When the user is redirected to that page, all POST/GET data is lost. So, if the user refreshes the page they will just get a refresh of that page, not the processing page. I hope that didn't make it more confusing.
-
No, that is inefficient. You only need to do a single query. The exact same question was asked just a couple days ago and a solution provided: http://www.phpfreaks.com/forums/index.php/topic,300778.msg1423631.html#msg1423631 SELECT * FROM (SELECT * FROM chat_messages ORDER BY message_id DESC LIMIT 10) as last10 ORDER BY last10.message_id ASC
-
Multiple Select Checkboxes, retrieve data from db and write to excel
Psycho replied to smilla's topic in PHP Coding Help
Here is a tested script based upon your previous posts. Note that since the file name is "static" for each day that there could be a problem if two users request an export at the same time. You can avoid this problem by utilizing a random name for the temp file stored on the server but still use the "static" name in the two disposition headers. <?php //Sanitize and prepare the input $recordIDs = array(); foreach ($_POST['row'] as $id) { $recordIDs[] = (int) $id; } $idList = implode(',', $recordIDs); //Query the data $query = "SELECT cm.nominee, co.company, cm.department FROM comments cm INNER JOIN company co ON cm.cid=co.id WHERE cm.id IN ($idList)"; $result = mysql_query($query); if(!$result) { echo "Problem running query"; } else if(mysql_num_rows($result)<1) { echo "There were no records returned"; } else { //Create the output file (will get rewritten for each user). $outputFileName = "Export_".date('Y-m-d').".csv";; $outputFileObj = fopen($outputFileName, 'w+'); //Create header row fputcsv($outputFileObj, array('Nominee', 'Company', 'Department')); //Ouput records while($record = mysql_fetch_assoc($result)) { fputcsv($outputFileObj, $record); } fclose($outputFileObj); //Output file to user header("Content-type: application/force-download"); header("Content-Disposition: inline; filename={$outputFileName}"); header("Content-Transfer-Encoding: Binary"); // header("Content-length: ".filesize($outputFileName)); header('Content-Type: application/excel'); header("Content-Disposition: attachment; filename={$outputFileName}"); readfile($outputFileName); //Delete temp file unlink($outputFileName); } ?> -
Multiple Select Checkboxes, retrieve data from db and write to excel
Psycho replied to smilla's topic in PHP Coding Help
No need to rebuild the wheel here. Creating a CSV file (you can give it an xls extension) will be much easier than a true Excel file. But, you don't have to create it programatically. PHP has the built in function fputcsv() which will output a record in array format as a properly formatted line. By trying to build your own CSV creation routine you are going to run into problems to overcome if the data has commas or quote marks. http://php.net/manual/en/function.fputcsv.php -
No need. The first two conditions will return false. So, if either of the failures occur it will never get to the return true. Only if the first two error confitions don't apply will the return true get processed.
-
You are not checking the "results" of the query. You are merely checking to see if the query ran successfully, i.e. without errors: public function validateUser($eMail, $Password) { $query = "SELECT * FROM regUsers WHERE eMail='$eMail' AND Password='$Password'"; $result = mysql_query($query); if(!$validResult) { //Query did not run $msg = $this->err_prefix."There was an error running the query. ".$this->mysql->error; throw new Exception($msg); return false; } else if (mysql_num_rows($result)!=1) { //Query ran, but there were no results $msg = $this->err_prefix."Your e-mail and/or Password do not match."; throw new Exception($msg); return false; } return true; }
-
So annoying! - After clicking submit, nothing happens
Psycho replied to 3raser's topic in PHP Coding Help
To be honest, that code has so much wrong with it I don't know where to start. It doesn't seem to be in any logical order making it difficult to debug. Here's one example. It seems the code is written so that variables can be passed via POST or via GET. $id = $_GET['id']; $id2 = $_POST['id2']; Then there is subsequent code to see if either of those are set. A much easier approach would be something like $id = isset($_POST['id']) ? $_GET['id'] : $_GET['id']; And then there is this while ($row = mysql_fetch_assoc($extract)) { $dpasscode = $row['passcode']; } Why would you use a while loop when you would expect only one record? -
Working on event calendar better way to store dates.
Psycho replied to bobby317's topic in PHP Coding Help
Using the first example from that page, you can convert a php timestamp to a MySQL date using $mysqldate = date( 'Y-m-d H:i:s', $phpdate ); And you can convert a MySQL date value to a PHP timestamp using $phpdate = strtotime( $mysqldate ); So, you only have two issues left. 1) Converting the user entered date to a PHP timestamp and converting a PHP timestampt to the appropriate string for display purposes. Not knowing how you want the date displayed or how the user inputs the date, I can't say how it should be done. What is the format of the user entered date and what is the format you want it displayed? E.g. 2-15-2010 Feb. 15th, 2010 2010-15-2 ???? -
So annoying! - After clicking submit, nothing happens
Psycho replied to 3raser's topic in PHP Coding Help
What do you mean it doesn't work? You stated that the goal is to allow the user to edit thier review. That requires that the posted data is sent to a page which will run an UPDATE query. That code does not have any update queries, therefore no updates occur. The form is posting to the page 'edit.php' if that is another page you need to be looking at that code. If it is the same page, then there is nothing in this page to handle an update. -
I hate checkboxes; please help me process them.
Psycho replied to bluegray's topic in PHP Coding Help
We Well, I don't know how the $data variable is created/set. but, if there is any logic to match it up against a known set of "possible" values, that might be the problem. If you have 10 checkboxes and only 8 are checked, then the post data only contains 8 fields. The two unchecked fields are not part of the post data. That might explain your results. -
So annoying! - After clicking submit, nothing happens
Psycho replied to 3raser's topic in PHP Coding Help
Well, back on topic. I don't see any UPDATE queries in your code, so there is no way to, well, update the existing posts. If you are posting that data to a different page you need to check that the submitted data is what you expect. If so, then the problem lies somewhere in the coding of that page. -
So annoying! - After clicking submit, nothing happens
Psycho replied to 3raser's topic in PHP Coding Help
Edit your post NOW to remove the database server info. -
AJAX. You will need to use a combination of JavaScript and PHP. There is no way to "push" data to the web browser with common implementations. The JavaScript will need to make a request to the server every n seconds to see if there is any new data to display and then update the user's dislplay accordingly.
-
I hate checkboxes; please help me process them.
Psycho replied to bluegray's topic in PHP Coding Help
Well, makes sense to me. You're making the code more complex than it needs to be. But here is the specific problem: foreach ($boxdata as $bd) { $key = key ($boxdata) ; key() "...returns the index element of the current array position" So, on the first iteration of the foreach() loop you get the first value of the array assigned to $bd. But at that same instance, the array pointer is shifted to the next element. So, the $key value you get is for the second value in the array, not the first. You don't need to go to all that trouble. That loop does nothing more than get the keys, which you can easily do with array_keys(); $boxdata = isset($data) ? $data : array(); var_dump($boxdata); $keys = array_keys($boxdata); var_dump ($keys); $fields = implode (', ', $keys) ; //return $fields ; echo "<p>$fields</p>" ; By the way, you should be using the VALUES of the checkboxes not the names (which is what it seems you are doing). Just give all the checkboxes the same name such as "fields[]". Then you can reference all of the checked values using the post value $_POST['fields'] without all this extra effort. $fields = implode (', ', $_POST['fields']) ; -
Working on event calendar better way to store dates.
Psycho replied to bobby317's topic in PHP Coding Help
You should definitely be using "date" fields to store the data. Here is an article on the subject that might help: http://www.richardlord.net/blog/dates-in-php-and-mysql -
No, that would be overkill. If you had simply stated in your first post what you were trying to achieve this would have been solved days ago. <?php $banList = array( 'Hacking' => "Banned for hacking into someone elses account and/or farming/deleting the user.", 'Farming' => "Banned for merging two or more of your accounts together into one account.", 'Multiple Accounts' => "Having more than one account constitutes an immediate ban of all the users account's. If you feel this has happened unjustly, contact support.", 'Glitch abuse' => "Banned for abusing in-game glitches and using them to your advantage without informing Admin.", 'Verbal Abuse' => "Banned for using foul or inappropriate language within the game.", 'Proxy' => "Banned for use of proxy's and potential multiple account usage.", 'Other' => "Banned at Admin discretion." ); $userBanReasons = array(); foreach($banList as $banTitle => $banReason) { if(preg_match("/$banTitle/i", $info[92])>1) { $userBanReasons[] = $banReason; } } if(count($userBanReasons)>0) { $reasons = "You were banned for the following reason(s):<br />\n" . implode("<br />\n", $userBanReasons); } ?>
-
For multi-dimensional arrays I prefer to use usort() over array_multisort() because array_multisort() requires you to recreate the array as multiple arrays in order to work. usort() allows you to create a function to be as simple or complex as you need. In this case: function sortTitles($a, $b) { if($a['title']==$b['title']) { return 0; } return ($a['title'] > $b['title']) ? 1 : -1; } usort($theArray, 'sortTitles');
-
I think this is what you are after: SELECT * FROM (SELECT * FROM table ORDER BY field DESC LIMIT 30) as last30 ORDER BY last30.field ASC
-
You are using the URL value to define a file to include!
-
How are you going to know what to delete if the ID isn't passed? If you are concerned about malicious users deleting records that shoulnd't be then you shoudll validate on the delete script that the user requesting the delete has the appropriate authorization to do so.
-
Just to beat a dead horse: The only thing that irritates me worse than seeing "looping" queries is when people write the SQL statement directly in the mysql_query() function instead of create a string var. By writing directly in the query function you lose the ability to easliy debug database errors.
-
According to the mysql documentation using addcshlashes() after using mysql_real_escape_string() is the preferred method of sanitizing for LIKE statements. However, very important, you would use the optional parameter in addcslashes() to only escape the '%' and '_' characters; $cleanValue = addcslashes(mysql_real_escape_string($userValue), “%_”) See page 78 in this document: http://dev.mysql.com/tech-resources/articles/guide-to-php-security-ch3.pdf Here is your code revised: function Safe($data, $Like=false) { if (get_magic_quotes_gpc()) { $data = stripslashes($data); } $data = mysql_real_escape_string($data); //If the Data is going to be used in a MySQL LIKE statement then Escape these characters as MySQL won't like them if($Like) { $data = addcslashes(mysql_real_escape_string($data), “%_”); } return $data; } Although, I don't know that it is even necessary to check if it is a LIKE statement. In other words, it may be valid to always escape those two values. I'm too busy to validate that right now.
-
It's difficult to debug your code without seeing all the applicable code. Could be you are using the wrong field names or something. But, your code has more "logic" than it needs. For example you check passowrd function does not need an else. The IF will exit the function of validation fails, so you simply need to return true after the IF code. Assuming the code is referncing the correct fields and the values are what you expect, the code looks like it should work, but here is a rewrite that is more efficient and logical, IMO. function ConfirmRegPass() { var frm = document.forms["register"]; if(frm.password.value != frm.password2.value) { sfm_show_error_msg("The Password and verified password don't match!", frm.password1); return false; } return true; } function Companyfield() { var frm = document.forms["register"]; if(frm.companyfield.value == "") { sfm_show_error_msg("Please select a company field.",frm.companyfield); return false; } else if(frm.companyfield.value=="Other" && frm.companyfieldother.value == "") { sfm_show_error_msg("Please type your company field.", frm.companyfieldother); return false; } return true; }
-
Is there any consistency in "how" the data is corrupted? It might be easier to fix the corruption than to build your own XML parser.