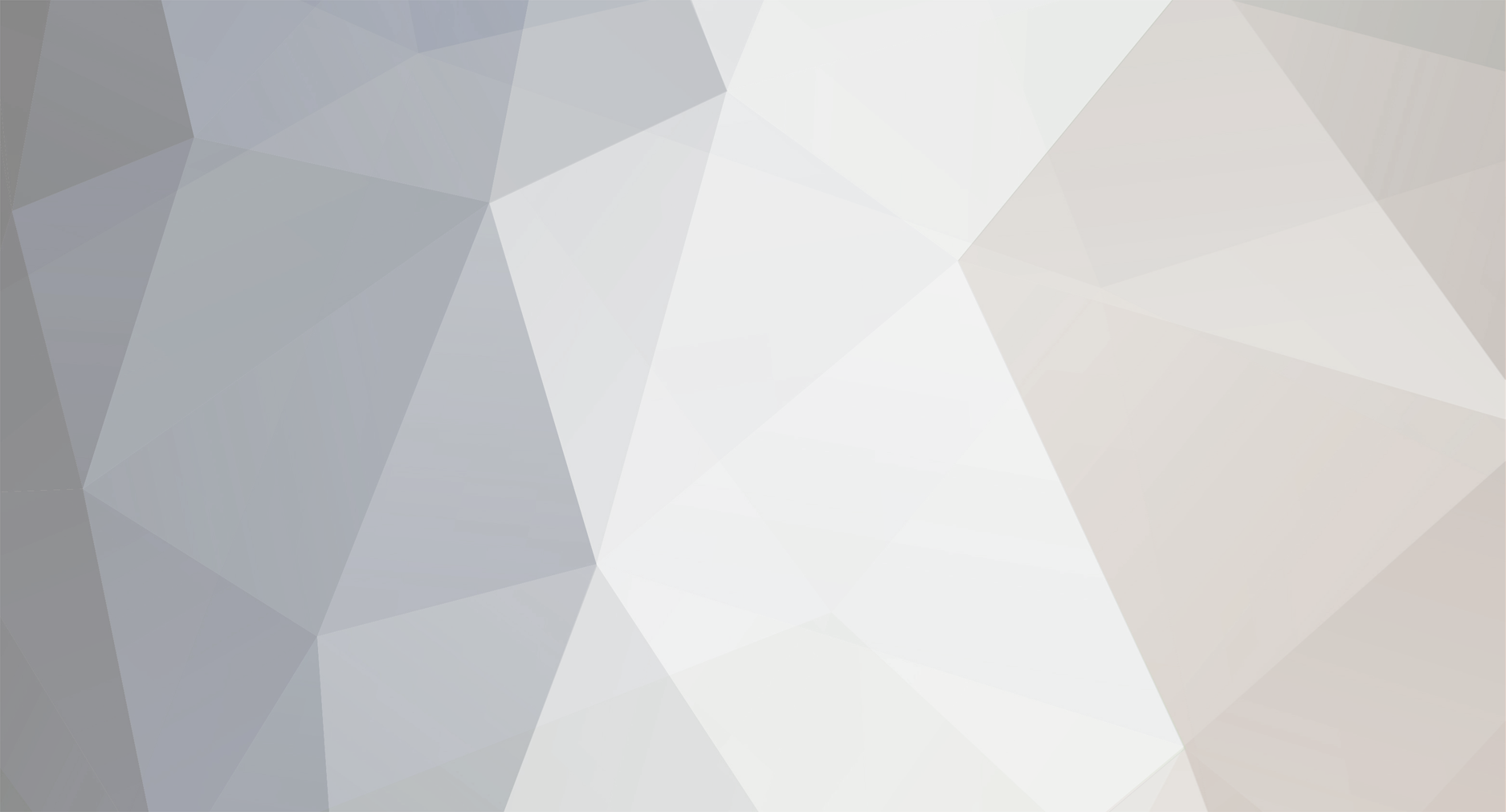
Psycho
Moderators-
Posts
12,159 -
Joined
-
Last visited
-
Days Won
130
Everything posted by Psycho
-
No, not at all. But, the information you needed was in the manual. It was down in the comments section, but if you are spending hours researching a problem the best place to start is to fully read the manual for the particular function. Sorry if I offended you, but I believe in teaching people to fish rather than giving them a fish.
-
You are making this more difficult than it needs to be. The records should have a unique ID which doesn't have to have anything to do with the sort order. Records should be ordered by the timestamp the record was created (as thorpe suggests). You can then add an index number to be "displayed" to the user within the PHP code that creates the HTML (as miancu suggests).
-
With all due respect, JavaScript is not required <html> <head> <meta http-equiv="refresh" content="5;url=http://yoursite.com/file.pdf"> </head> <body> Your file will download in 5 seconds. If it does not start automatically, click <a href="http://yoursite.com/file.pdf">here</a>. </body> </html>
-
Yes, it is possible. But, why not specify a max width and a max height. So, for example, if the max size is 150 x 150 the following images would be resized as follows: 1000 x 1000 = 150 x 150 1000 x 3000 = 50 x 150 3000 x 1000 = 150 x 50 You could even "fill in" the unused proportions with black or white. Distorting the image is a bad route IMHO.
-
The link should take the user to another page where the logo is displayed. That page could also use a meta refresh tag to start the download after 5 seconds
-
You are making it harder than it needs to be by trying to replace characters before and after the "v". Just do a simple replace of '/watch?v=' with '/v/' using str_replace(). $link = str_replace('/watch?v=', '/v/', $link);
-
js novice needs help consolidating simple (but long) script
Psycho replied to glennn.php's topic in Javascript Help
There is a problem with your form. There is a question for "Do you drink alcohol?" with four checkbox options: No, Beer, Wine, Liquor. So, the form allows the user to select No and one of the three Yes options concurrently. The question should be something such as "Select the types of alcohol that you drink" and just drop the No option as that would be assumed if the user didn't select any options. -
I guess reading the manual is too much work? To escape a type specifier withint sprintf() you use a percent character. Therefore, if you want to literally use the percent character, you would use it twice. WHERE fname LIKE %s%% OR lname LIKE %s%% But, you can't do that here. Your GetSQLValueString() functions is apparently returning the string with the value already enclosed in parens. So, you can't add the percent sign within the format string. Otherwise you would end up with: WHERE fname LIKE 'd'% OR lname LIKE 'd'% You have to add it to the value passed in the GetSQLValueString() function.
-
One line, using mt_rand(): $no = (mt_rand(0,1)) ? mt_rand(20, 50) : mt_rand(-50, -20);
-
Just read the manual. I'm sure it will do a better job of explaining the functions than I can.
-
Ideally, you should store these values as separate records in an associated table instead of dumping them into a single field. It seems like more work, but it will make your life much easier in the long run. Well, now that you show all the possible options, it is evident that you cannot use a regular expression for this since there is no common rule to apply, if all the options began with "no", then you could use a regular expression: preg_match_all("#(\bno .+?(?=no|$))#", $text, $textToArray); But the options for "vegetarian" and "gluten free" make that impossible. You will have to go with a much more inefficient approach. <?php //Value from database $database_value = "no wheat no sugar gluten free no pork no nuts (allergy) vegetarian"; //Array of all possible options $optionsAry = array('no wheat', 'no sugar', 'no red meat', 'no cheese', 'no dairy', 'no pork', 'vegetarian', 'gluten free', 'no fish', 'no nuts (allergy)'); //parse database string using available options $userOptionsAry = array(); foreach($optionsAry as $optionStr) { if(strpos($database_value, $optionStr)!==false) { $userOptionsAry[] = $optionStr; } } //Convert database options array to string with separator $userOptionsStr = implode('|', $userOptionsAry); echo $userOptionsStr; //Output: no sugar|no pork|vegetarian|gluten free|no nuts (allergy) ?>
-
That would do absolutely nothing since those two values are being set right before that IF condition. Even using isset() on the POST values would be pointless because even if the user left them blank, they will still be set. Here is a better approach: $username = trim($_POST['username']); $password = trim($_POST['password']); if(empty($username) || empty($password)){ However, because of the current logic, the user would always see that error message on the first loading of the page. You should wrap all the logic for processing the POST data in an if statement such as: if(isset($_POST) { //PHP logic for processing form data } That way, the first time the user accesses the page the PHP logic won't run and only the form will be displayed.
-
I don't think the problem has anything to do with image dimensions. You are suppressing errors on your database functions. So, if there are any errors you won't see them. You are also not referencing the field correctly in the results. The field name should be in quotes. But, even though it is not correct, it has been my experience it will work anyway. Try the following: $user_id = 10; mysql_connect("localhost", "user", "pass"); mysql_select_db("my_db"); $q = "SELECT * FROM my_table WHERE id = '$user_id'"; $r = mysql_query($q); if(!$result) { echo "Error running query:<br />{$q}<br />Error:<br />" . mysql_error(); } else { $rset = mysql_fetch_assoc($r); $imagebytes = $rset['arb_photo']; header("Content-type: image/jpeg"); print $imagebytes; } If that doesn't work, do a print_r() on $rset to verify the database results
-
To be honest, that code is kind of rough and unorganized. Take this for instance: $query = ("SELECT user.userID, user.gender, user.bd_year, user.city, user.state, user.zip, photos.userID, photos.photo_1 FROM user, photos WHERE user.bd_year <= $year1 AND user.bd_year >= $year2") or die(mysql_error()); Why do you have an "or die" on a statement that is defining a string? Also, the code to display that there are no records is dependant on this if ($result = mysql_query($query)) That will tell you when the query failed - not when there are no records. Also, I don't see anything in your query to join the two tables, so I expect you will always get results. Plus, you are trying to echo $age, but it has not been defined and there are fields in the query that aren't used. You still need to address some of the problems above, but this should get you pointed in the right direction. <?php //Set variable for columns in grid $max_columns = 4; //Function for creating row output function createRow($records) { if(count($records)==0) { return false; } $rowOutput = "<tr>\n"; foreach($records as $record) { $rowOutput .= "<td width=\"175\">"; $rowOutput .= "<div id=\"profiles\">"; $rowOutput .= "<a href=\"profile.php\">"; $rowOutput .= "<div id=\"name\">{$record['userID']}</div>"; $rowOutput .= "<div id=\"image\">"; $rowOutput .= "<img src=\"uploads/{$record['photo_1']}\" width=\"100\" height=\"100\" border=\"0\">"; $rowOutput .= "</div></a>"; $rowOutput .= "<div id=\"status\">{$record['age']} - {$record['city']}, {$record['state']}</div>"; $rowOutput .= "</div>"; $rowOutput .= "</td>\n"; } $rowOutput .= "<tr>\n"; return $rowOutput; } $query = "SELECT user.userID, user.gender, user.bd_year, user.city, user.state, user.zip, photos.userID, photos.photo_1 FROM user, photos WHERE user.bd_year <= $year1 AND user.bd_year >= $year2" $result = mysql_query($query) or die(mysql_error()); if (!$result) { //Query failed $output = "There was a problem. Please try again later."; } else if(mysql_num_rows($result)<1) { //No records in query $output = " Nothing found."; } else { $output = "<table cellspacing=\"0\" cellpadding=\"3\" border=\"0\" width=\"700\">\n"; $output .= "<tr>\n"; $rowRecords = array(); while ($rowRecords[] = mysql_fetch_assoc($result)) { if (count($rowRecords)==$max_columns) { //Create output for current row & reset array $output .= createRow($rowRecords); $rowRecords = array(); } } //Process last row (if uneven) $output .= createRow($rowRecords); //Close table $output .= "</table>"\n; } ?> <html> <body> <?php echo $output; ?> </body> </html>
-
EDIT PFMaBiSmAd already answered this. But, I'll post this nonetheless since I provided a code solution Well, a non-breaking space (i.e. ) is a different character than a breaking-space. The typical space character that allows line breaking is ASCII code 32, wherease the converted to a single character space is ASCII character 160. So you could either echo a non-breaking space character to the page and copy/paste that into your str_replace, or use the character code equivalent. str_replace(chr(160), "", $string)
-
Does a-z in regex validate european charachters?
Psycho replied to shadiadiph's topic in Javascript Help
<html> <head> <script type="text/javascript"> function invalidInput(text) { var invalidChars = /[$%@!]/ return invalidChars.test(text); } function checkInput() { var inputText = document.getElementById('input').value; if(invalidInput(inputText)) { alert('Input was NOT valid'); } else { alert('Input was valid'); } return; } </script> </head> <body> Input: <input type="text" name="input" id="input" /> <br /> <button onclick="checkInput();">Test</button> </body> </html> -
Another thought is to use AJAX and process the requests asynchronously. However, once the user navigates to another page - either on their own or through header() the javascript request cannot "report back" to the user once the process is complete.
-
Not tested, so there might be some syntax errors. BUt, logic shoudl be sound. <?php //Variable to determine number of columns $records_per_row = 4; //Function for creating a row function create_row($row_data) { if(count($row_data)<1) { return false; } $output = "<tr>\n"; foreach($row_data as $record) { $output = "<td><img src=\"{$record['src']}\" /><br />{$record['name']}</td>\n"; } $output = "</tr>\n"; return $output; } //Create & run query $query = "SELECT name, src FROM images"; $result = mysql_query($query); //Process the data $records = array(); $tableOutput = ''; while($records[] = mysql_fetch_assoc($result)) { if(count($records)==$records_per_row) { //Create row HTML and reset array $tableOutput .= create_row($records); $records = array(); } } //Process last row $tableOutput .= create_row($records); ?> <html> <body> <table> <?php echo $tableOutput; ?> </table> </body> </html>
-
There are ways to do this. For example you could store the form data in a queue table and have a cron job that runs every x minutes to process any submissions. The problem with this approach is that the submission/processing is no longer part of the user's session since the processing is done in a separate process. How long is the script taking to run? You could display a page with a message such as "Your data is being processed" with an indeterminate progress indicator. But, more importantly, have you investigated the code to see that it is running as efficiently as it can? For example, do you have any database queries running in a loop? Those are extremely inefficient and can almost always be done with a single query.
-
Use double equal signs for comparisons, use single equal sign for assignment.
-
It seems all of your "Play" links go tot he same page and have an optional parameter to identify the track to be played. Here are two examples: http://www.hostrox.com/proj/songs.php?movie=Movii1# http://www.hostrox.com/proj/songs.php?movie=Film123# I assume this is all in a adatabase. So, one option would be to have a column in the songs table for "times_played" or somethign similar. Then, just add some code on the songs.php page to increment that value by one whenever the page is called. If the value of movie ont he query string is the unique ID for the record int he database, then the code might look something like this: $song_id = mysql_real_escape_string($_GET['movie']); $query = "UPDATE songs SET times_played = times_played + 1 WHERE song_id = $song_id"; $result = mysql_query($query);
-
Corrected the regular expressions, also I'm pretty sure you want to use \t and \n $a = "<table border=0><tr bgcolor='#D6D6D6'><td align='center'>Volume 1</td><td align='center'>Volume 2</td></tr></table>"; $a = preg_replace("/<table[^>]*>(.*?)<\/table>/i", "$1", $a); $a = preg_replace("/<tr[^>]*>(.*?)<\/tr>/i", "\n$1", $a); $a = preg_replace("/<td[^>]*>(.*?)<\/td>/i", "\n\t$1", $a); echo $a; //Output: // // Volume 1 // Volume 2 NOTE: moved topic to RegEx forum.
-
Multiple inserts from a variable that has comma seperated values
Psycho replied to jetsettt's topic in MySQL Help
Why do you need to query the db for information regarding the selected IDs? You should only need to save records with the selected IDs since they will point back to the relevant records anyway. However, to answer your questin directly (even though it is not the right approach in this situation), you don't need to so a select query and then multiple inserts. You could simply do an insert using a select as the values Example: INSERT INTO table1 (field1, field2) VALUES (SELECT fieldA, fieldB FROM table2 WHERE something='$value') -
Counting the amount of directories in a directory?
Psycho replied to sw0o0sh's topic in PHP Coding Help
Try reading the manual. http://us3.php.net/manual/en/function.glob.php glob() accepts an optional secondary parameter. There is one specifically for what you want. -
I've got a solution that takes only two lines $no = rand (1, 62); $no = ($no<32) ? ($no-51) : ($no-12);