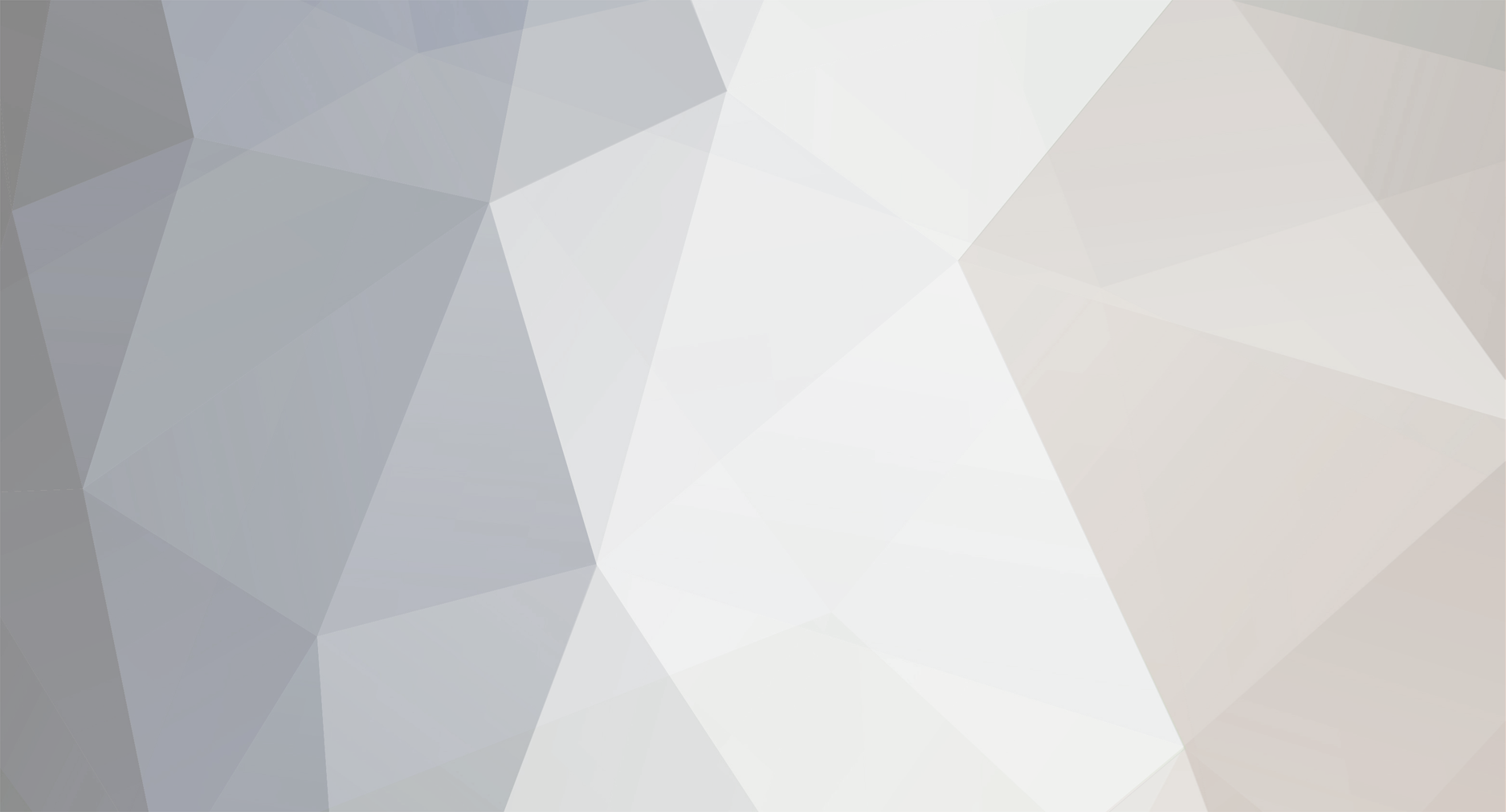
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Well, you are doing it all wrong. You need to normalize your database (too much to try and explain here). The "items" should be stored in a separate table with a foreign key back to the user table. Creating nine (times two) serparate columns is a poor design. Tables would look something like this: Users user_id username items user_id item index name
-
Then you shoudl be using a switch() stement instead of all the If/Esle statements. Not 100% sure what your questin is. What is the problem you are having with that code?
-
Ok, you really need to start using the "logic" that is available in PHP instead of brute force coding. I see your error, but let's go over a couple of things that would make your life so much easier first. Instead of this while($row = mysql_fetch_array( $yep )) { $name1 = $row['name1']; $name2 = $row['name2']; $name3 = $row['name3']; $name4 = $row['name4']; $name5 = $row['name5']; $name6 = $row['name6']; $name7 = $row['name7']; $name8 = $row['name8']; $name9 = $row['name9']; } You could just to this: extract($row); And, you should modify your query to only pull the fields you want. Then there is the mess of if/else statements if ($wdnumber = "1") { echo "You chose 1"; } elseif ($wdnumber = "2") { echo "You chose 2"; } elseif ($wdnumber = "3") { echo "You chose 3"; } elseif ($wdnumber = "4") { echo "You chose 4"; } elseif ($wdnumber = "5") { echo "You chose 5"; } elseif ($wdnumber = "6") { echo "You chose 6"; } elseif ($wdnumber = "7") { echo "You chose 7"; } elseif ($wdnumber = "8") { echo "You chose 8"; } elseif ($wdnumber = "9") { echo "You chose 9"; } Why not just do this: echo "You chose {$wdnumber}"; Anyway, this is your problem if ($wdnumber = "1") { You are doing an assignment NOT a comparison. Since the PHP parser has no problem assigning the value of 1 to the variable the condition always passes. You use double equal signs for comparisons. if ($wdnumber == "1") {
-
The problem is because you have three different criteria using an AND and an OR. The order of precedence on how those are processed is causing the issue. AND's are processed before OR's. http://dev.mysql.com/doc/refman/5.0/en/operator-precedence.html As the query is interpreted now it is pulling records where: `product_name` LIKE '%$searchString%' OR `product_description` LIKE '%$searchString%' AND `in_stock`='Y' You obviously want records where one of the first two conditions is true AND the last condition is true. So, you should use parens to force how the line is interpreted. SELECT * FROM `fcpv3_products` WHERE (`product_name` LIKE '%$searchString%' OR `product_description` LIKE '%$searchString%') AND `in_stock`='Y' ORDER BY `id`
-
I'm having a difficult time really understanding your request. It would have been helpful if you also showed what you expected the result to be when the user selects "3" instead of just showing what you are getting. I assume you want the result to be such that the selected page is indicated in some manneer (e.g. bold) and that the avaialble pages - up to 10 - are displayed to the left and the right of the selected page. That page has some other problems with inefficiency and other things (e.g. a function to return a static variable?!). Here is some code based upon what I think you want along with other improvements. This is NOT tested. The logic should be good, but I didn't go to the trouble of create a database table for this. <?php //Config variables $records_per_page = 10; $max_page_links = 10; //Connect to DB $db_conn = new MySQLi('localhost', 'root', '', 'db'); //Determine total records and total pages $query = "SELECT COUNT(`content`) as total FROM `link`"; $result = $db_conn->query($query); $row = $result->fetch_assoc(); $total_records = $row['total']; $total_pages = (ceil($total_records/$records_per_page)); //Determine the current page $page = (isset($_GET['page'])) ? (int) $_GET['page'] : 1; if($page<1 || $page>$total_pages) { $page = 1; } //Detemine the start for the limit in query $limit_start = ($page - 1) * $records_per_page; //Get & display data for currently selected page $query = "SELECT `content` FROM `link` ORDER BY `no` DESC LIMIT {$limit_start}, {$records_per_page}"; $thumbnail = $db_conn->query($query); while($row = $thumbnail->fetch_assoc()) { $page_output = "{$row['content']}<br />\n"; } //Create array of the navigation links //--------------------------- $nav_links_ary = array(); $first_page_link = max(1, $page-$max_page_links); $last_page_link = min($total_pages, $max_page_links); //Add page links to array if($page>1) { $nav_links_ary[] = "<a href=\"?page=" . ($page-1) . ">Prev</a>"; } for ($pg=$first_page_link; $pg<$last_page_link; $pg++) { $nav_links_ary[] = "<a href=\"?page={$pg}\">{$pg}</a>"; } if($page<$total_pages) { $nav_links_ary[] = "<a href=\"?page=" . ($page+1) . ">Next</a>"; } //Create final output for navigation links $nav_links = implode(' | ', $nav_links_ary); ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Untitled Document</title> </head> <body> <?php echo $page_output; ?> <p> </p> <?php $nav_links; ?> </body> </html>
-
Rather simple question.. array_search() or other!!
Psycho replied to Zugzwangle's topic in PHP Coding Help
It looks like the values in the array actually contain a name and the actual valule. So, Building upon AlexWD's post, here is a function you could use. Just pass the function the "name" you are looking for and it will return the value: function getValue($array, $name) { foreach($array as $key => $value) { if(substr($value, 1, strlen($name))==$name) { return substr($value, strlen($name)+2); } } //No match found return false; } //Usage echo getValue($withoutBreaks, 'EventDate'); //Ouput: 2010.06.18 Edit: Now that I think of it, it might be better to just create a function to conver the array to where the names are the keys and the values are the actual values: Array ( ['Event'] => 90m + 5s, rated ['Site Room'] => 1 ['Date'] => 2010.06.18 ['Round'] => ? ... -
Well, I can't determine what is wrogn without seeing the data since the queery looks fine. Try posting the table structure for the two tables and provide a few sample records that are being returned from the query which you feel shouldn't be.
-
The problem is that you are putting the second "rp" in single quotes!!! That tells MySQL you wat to set the value to a STRING. Since the field is obviously a numeric field the string is being interpreted as 0. You need to reference the MySQL field to be added to the $winnings, not the string "rp" UPDATE `Member` SET `rp` = `rp` + $winnings WHERE `username` = '$username' Of course, you would see the errors if you had added error handling to your database calls.
-
I just realized I added code that was unnecessary. Instead of this: $success = rename($userfile_name, $newName); if($success) I should have just done this: if(rename($userfile_name, $newName))
-
So confused ! PHP CODE! I can't figure out whats missing.
Psycho replied to canadian_angel's topic in PHP Coding Help
<?php if(isset($_POST['miles'])) { $miles = $_POST['miles']; $stops = $_POST['stops']; $condition = $_POST['condition']; $mph = ($condition=='stormy') ? 40 : 50; $total_minutes = ($miles/$mph)*60 + (5 * $stops); $hours = floor($total_minutes/60); $minutes = floor($total_minutes%60); $result = "To travel {$miles} mile(s) in {$condition} weather with {$stops} stop(s) would take approximately {$hours} hour(s) and {$minutes} minute(s)."; } ?> <!DOCTYPE HTML PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-Transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1"/> <title>Passenger Train</title> </head> <body> <!-- Script 6.3 - passenger_train.php --> Fill out form to determine time to required destination: <br /> <form action="test.php" method="post"> <br /> Miles: <input type="text" name="miles" size="5" value="<?php echo $miles; ?>" /> <br /> Stops: <input type="text" name="stops" size="5" value="<?php echo $stops; ?>" /> <br /> Weather Conditions: <input type="radio" name="condition" value="clear" checked="checked" /> Clear <input type="radio" name="condition" value="stormy" /> Stormy <br /><br /> <input type="submit" name="submit" value="Calculate" /> </form> <br /><br /> <?php echo $result; ?> </body> </html> -
Well, you state you want to use the project_id to "sort" the records, but your query is currently filtering the records. Other than that, I see no obvious problems. Although, if there are other columsn with duplicate names between the tables that would cause an error. You don't state if you are getting any errors. If so, please post them. If you really do want to sort instead of filter the query would look something like this: SELECT * FROM contact JOIN project_contact USING (contact_id) ORDER BY project_id
-
No it wouldn't; it is perfectly acceptable to have an IF statement without an ELSE. In this case, you don't want an ELSE unless there is a problem with the rename process. But, your ELSE is currently running whenever the POST value isn't set to a certain value. Instead you should be checking the response of rename() to see if the process succeeds or not. <?php //when user submits form, cd to upload directory, rename the file and echo that it completed or failed if ($_POST['action']=="change") { $newName = trim($_POST['newName']); chdir($upload_directory); $success = rename($userfile_name, $newName); if($success) { echo "The file has been renamed.<br>"; //destroy the session so the user can go back and upload another file session_destroy(); } else { echo "The file rename failed.<br>"; } } ?>
-
Yeah, I'm pretty sure that works for ASP as well, but it's been years since I used it. The one thing to be aware of with that is that referencing those fields in JavaScript is somewhat counter-intuitive. They are still an array, but not how you might think. Example: var checkboxGroup = document.forms['formname'].elements['groups[]']; Note the use of "[]" in the referenced name.
-
Instead of naming the checkboxes in sequential order, they should be named as an array <input type="checkbox" name="groups[]" value="Choir" /> <input type="checkbox" name="groups[]" value="Chorale" /> Then in the processing page you would just do this if(isset($_POST['groups'])) //Only run if checkboxes were selected { foreach($_POST['groups'] as $groupName) { echo "{$groupName} was selected<BR>\n"; } }
-
how to get directory/folder size and delete files inside
Psycho replied to Dss.Lexius's topic in PHP Coding Help
Well, a quick look in the manual: http://www.php.net/manual/en/function.filesize.php#94566 provides the following solution for getting the "size" of a folder, i.e. the sum of the sizes of all the files in the folder. Folders don't actually have a size. function dirSize($directory) { $size = 0; foreach(new RecursiveIteratorIterator(new RecursiveDirectoryIterator($directory)) as $file){ $size+=$file->getSize(); } return $size; } So, do a check, and then if > 500MB (remember the above function returns bytes) then use unlink() to delete the file -
I don't think you can do what you are asking with your current structure. I'm not sure I even understand what the current structure is as it doesn't make sense to me. I would expect users may have multiple favorites under one genre (e.g. country) but non under another genre (e.g. barbershop). You should modify your database structure. I would suggest the following: 1) a user table with basic user info, name, age, etc, 2. A genre with genre_id and genre_name. You would have one record for each genre you want to track 3. Finally a user_genre table to link the two above. The table could have fields for user_id, genre_id, artist_name, date_added. You could then grab the most curretly added artist record for a user or know if there are none.
-
Why, then, did yu post in the JavaScript forum? I don't think your problem can be fixed in PHP either. The page gets sent to the printer and it prints what is sent to it whther it takes 1 page, 3 pages or more. You need to look at the content and structure of your page and modify it to fit in a standard printed page. You can use CSS to have the format of the page different for print output rather than browser output. So, you could choose to have some elements not display in a printed output if they are not needed and are causing the html "page" to print on multiple physical printed pages.
-
Well, if the user is logged in then you should have a session variable set to know who the user is on subsequent pages. Also, you should also be using a userID field in the table instead of relying upon the username. So, using the sample code I posted before, you would get the username from the session variable and instead of an ISERT you would want to do an update. Something like: $username = $_SESSION['username']; $sql = "UPDATE Member SET rd = rd + $winnings}"; Since you want to limit the user to a number of rolls per day, you have a couple of options. 1) You could store every roll into an associated table with fields such as username, roll, date. That would allow you to capture historical data. If you are absolutely sure you won't need that historical data, then you could add two fields to the Member table: lastrolldate, rollcount Then when a user makes a roll you can check if the rolldate is today - if so, add the rollcount by one. If the rolldate is not today, then set it to today and set rollcount to 1.
-
Simple timedate question: adding times together.
Psycho replied to gergy008's topic in PHP Coding Help
date("dmyHis", strtotime("+1 day")); -
Well, aside from the fact that you don't needs all those IF statements, your query would simply create a record with teh value 500 in it. I would think you need a value to identify that the record belongs to this user. Not knowing what th eintention is for the database record I can't state how this should work. For example, should there be duplicate records in the database for eadch user if they win multiple times or should there be one record with the total winnings? Here is revised code with all the IF statments removed and the query adjusted based upon some assumptions of the available data and fields in the table <?php $dice = rand(1,6); echo "You rolled a<br /><b>{$dice}</b>\n"; if($dice == 6); { $winnings = "500"; include("haha.php"); $user_id = $_SESSION['user_id']; $cxn = mysqli_connect($dbhost,$dbuser,$dbpassword,$dbdatabase); $sql = "INSERT INTO Member (user_id, rp) VALUES ('$user_id', '$winnings')"; mysqli_query($cxn,$sql); } ?>
-
use the usort() function and create a function for the sorting. http://us.php.net/manual/en/function.usort.php You can even sort by multiple fields.Here is an example with sorting of three different fields. function sortEntries($a, $b) { //If J_J_Num is not same, sort by that field if($a['J_J_num']!=$b['J_J_num']) { return ($a['J_J_num']<$b['J_J_num'])? 1 : -1; } //If J_J_Num was the same, sort by field2, if not the same if($a['field2']!=$b['field2']) { return ($a['J_J_num']<$b['J_J_num'])? 1 : -1; } //If J_J_Num and field2 were the same, sort by field3, if not the same if($a['field3']!=$b['field3']) { return ($a['J_J_num']<$b['J_J_num'])? 1 : -1; } //All sort fields were the same return 0 return 0; } usort($theArray, 'sortEntries'); Note: the order of 1 and -1 determine whether that field is sorted in ascending or descending order.
-
i've got an expression :) just needs...correcting
Psycho replied to GoneNowBye's topic in Regex Help
The issue has nothing to do with your expression. It has to do with how the PHP function returns matches. I assume you are using preg_match_all(): http://us.php.net/manual/en/function.preg-match-all.php Please read the documentation on how values are populated into the $matches parameter and the available flags to modify the returned values. The default is to I don't believe you can get what you are after, but you can try experimenting with the other flags. -
In the index.php page do something like this <?php if ($_SERVER['REQUEST_METHOD'] == "POST") { include('lookup.php'); } else { ?> <form action="lookup.php" method="POST" class="form"> <label for="domain">Domain Name</label> <div class="domain_div"> <input name="domain" type="text" class="domain_tb" id="domain" value="domain" /> </div> <div class="button_div"> <input name="Submit" type="button" value="Look Up" class="buttons" /> </div> </form> <?php } ?>
-
This may work. Since I don't have your database and am too lazy to create one, I can't test it. If I am correct the generated query should return a result set with each user ID and their appropriate total. (be sure to replace "table" with the appropriate table name) $country = "USA"; $number = "1"; $query = "SELECT user_id, SUM ( IF (country='{$country}' AND bool='Yes', 1, 0), IF (country='{$country}' AND bool='Yes' AND number='{$number}', 1, 0) ) as total FROM (SELECT user_id, value as country FROM table WHERE field_id = 1) as t1 JOIN (SELECT value as bool FROM table WHERE field_id = 2) as t2 ON t1.user_id = t2.user_id JOIN (SELECT value as number FROM table WHERE field_id = 3) as t3 ON t1.user_id = t3.user_id";
-
Not quite. The query was looking for a specific staff ID. If you are wanting to show all the staff records, then you should remove the WHERE clause from your query entirely: $query_rsStaff = "SELECT * FROM cms_staff";