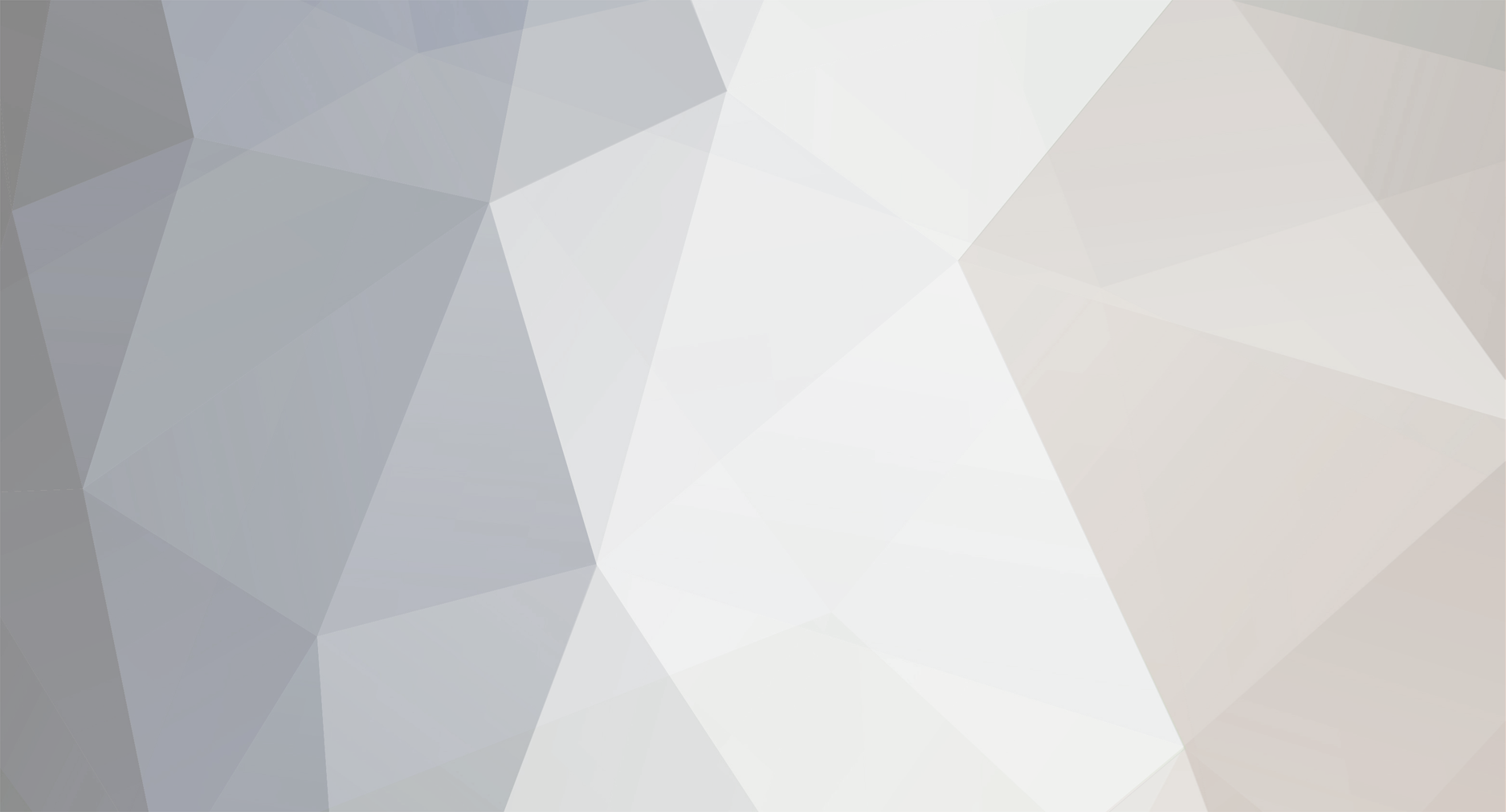
Psycho
Moderators-
Posts
12,159 -
Joined
-
Last visited
-
Days Won
130
Everything posted by Psycho
-
You page has many different forms and I'm not sure what validation should be run for one or another. But, as Nightslyr stated, you just need on onsubmit trigger for the form. The function that is called should ultimately return true (to submit the form) or false (do not submit the form) whenthere is a problem. Here is a simple example <html> <head> <script type="text/javascript"> function validateForm(formObj) { if (formObj.uname.value=='') { alert('You must enter a name'); return false; } //No errors occured return true; } </script> </head> <body> <form name="test" onsubmit="validateForm(this);"> Name: <input type="text" name="uname"> <br /> <button type="submit">Submit</button> </form> </body>
-
You need to provide more information and some of your actual code. There's nothing about posting data that will strip the spaces by default, so you have an error in your code - which you didn't post. Even the code you did post isn't correct. On the output you only show code that echos the Last name and the age, but you show the first name displayed. Did you check the generated HTML code to see if the entire value is there and possibly not shown because of an HTML format problem?
-
To expand on what DanielO provided, PHP5 allows for an optional parameter when using microtime() which will return the value as a float. Otherwise, microtime returns a string in the format: "[milliseconds in decimal format] [seconds since epoc]" Which is not as useful, IMHO. If you are using PHP5, then add the optional parameter when using microtime. Otherwise, create a function to do it for you. <?php function mtime_to_float($mtime) { list($microseconds, $seconds) = explode(' ', $mtime); return ($seconds + $microseconds); } $start = microtime(); usleep(500000); $end = microtime(); $time_passed = (mtime_to_float($end) - mtime_to_float($start)); if (($time_passed) > .5) { echo "Process took more than 1/2 second passed."; } else { echo "Process took 1/2 second or less"; } ?> Note: For accuracy do not call microtime() within functionality to do the calculations/comparisons. Instead set variables to microtime() at the points in the code to be evaluated, then do the calculations.
-
I took a different approach and processed the value as a string (first converting the value to an int and then itno a string to remove decimal content): <?php function convertNumToParts($number) { $numberStr = (string) (int) $number; $result = array(); for($i=0; $i<strlen($numberStr); $i++) { $result[pow(10, strlen($numberStr)-$i-1)] = substr($numberStr, $i, 1); } return $result; } $number = 525; $parts = convertNumToParts($number); print_r($parts); //Output: //Array //( // [100] => 5 // [10] => 2 // [1] => 5 //) ?>
-
I think you were reading something into my post that wasn't there. I asked why you didn't reply in your original post since this new post is technically against the forum rules And, in fact, that post also violated the rules I'm not trying to be an ass, but the rules are there for a reason. They help you to get the answers you need and make it efficient for those providing support. As for the "rewrite" comment, it was simply a factual statement. The code you have now was not meant for how you are using it. A tutorial is not meant for you to simply copy-paste the code, it is meant for you to learn a concept and then implement the concept, not necessarily the exact code, into your project. As I said, I would not use the code as you have it. There is logic in there that is no longer relevant. I would encourage you to make this a learning experience and to read through the code line-by-line to understand what is happening on each line and to determine what changes, if any, are warranted. If I was trying to be mean I would point out that your usage of the word "ignorance" is incorrect. Perhaps you meant "arrogance". Oh wait, I guess I did point that out. Seriously, though, no ill will intended.
-
Nice tip Neil. bassguru, If I may, I think your pattern has some holes. For one, it does not allow the plus sign in the username, which is perfectly valid for an email address. Also, for the TLD you are only accepting 2 or 3 letter values. There are plenty of valid TLDs that are more than 3 characters in length (.MUSEUM and .TRAVEL being the longest ones I know of). Here is the function I use for email validation. It validates the format and the length. Feel free to point out any flaws: //===================================================== // Function: is_email ( string $email ) // // Description: Finds whether the given string variable // is a properly formatted email. // // Parameters: $email the string being evaluated // // Return Values: Returns TRUE if $email is valid email // format, FALSE otherwise. //===================================================== function is_email($email) { $formatTest = '/^[-\w+]+(\.[-\w+]+)*@[-a-z\d]{2,}(\.[-a-z\d]{2,})*\.[a-z]{2,6}$/i'; $lengthTest = '/^(.{1,64})@(.{4,255})$/'; return (preg_match($formatTest, $email) && preg_match($lengthTest, $email)); } // NOTES: // // Format test // - Username accepts: 'a-z', 'A-Z', '0-9', '_' (underscore), '-' (dash), '+' (plus), & '.' (period) // Note: cannot start or end with a period (and connot be in succession) // - Domain accepts: 'a-z', 'A-Z', '0-9', '-' (dash), & '.' (period) // Note: cannot start or end with a period (and connot be in succession) // - TLD accepts: 'a-z' & 'A-Z' // // Length test // - Username: 1 to 64 characters // - Domain: 4 to 255 character
-
Why didn't you just reply to your original thread? The fix is as simple as it gets. These lines only add a condition if the checkbox was set // grab the search types. $types = array(); $types[] = isset($_GET['ptitle'])?"`title` LIKE '%{$searchTermDB}%'":''; $types[] = isset($_GET['pdate'])?"`date` LIKE '%{$searchTermDB}%'":''; $types[] = isset($_GET['pindustry'])?"`industry` LIKE '%{$searchTermDB}%'":''; Just change them so they are set by default // grab the search types. $types = array(); $types[] = "`title` LIKE '%{$searchTermDB}%'"; $types[] = "`date` LIKE '%{$searchTermDB}%'"; $types[] = "`industry` LIKE '%{$searchTermDB}%'"; Personally, I would change a lot more, but I'm not in a mood to rewrite your code.
-
[SOLVED] tried redirect with headers and get loop
Psycho replied to bigbenbuilders's topic in PHP Coding Help
Are you expecting values to be passed through any $_REQUEST variable or just $_GET? Don't see why you are using the $_REQUEST variable. I would just use GET myself. But anyway, just replace this line: $option = trim( strtolower( mosGetParam( $_REQUEST, 'option' ) ) ); With this one: $option = (isset($_REQUEST['option'])) ? trim(strtolower(mosGetParam($_REQUEST,'option'))) : 'com_content'; Do the same for the other variables. Since I don't know what mosGetParam() this might not work, but should give you an idea. -
[SOLVED] tried redirect with headers and get loop
Psycho replied to bigbenbuilders's topic in PHP Coding Help
Didn't I just show you? Set a variable (i.e. $option) to the value of $_GET['option'] if it is set, otherwise set it to a default value, in this example 'com_content' $option = (isset($_GET['option']))?$_GET['option']:'com_content'; Then just use that variable in your code. -
If the value being searched is ONLY in the format you entered and is not embedded in a larger body of text (specifically with other < or >), then this will work just fine: preg_match("/>(.*)</", $link, $matches); $username = $matches[1]; If the username is within larger bodies of text then you can use this preg_match("/<a href=\"member\.php\?u=\d+\">(.*)<\/a>/", $link, $matches); $username = $matches[1]; The will grab the 'text' of an anchor only if it is exactly formatted as you have above - also the ID must consist of one or more numbers.
-
That code IS PHP - what exactly are you wanting?
-
[SOLVED] tried redirect with headers and get loop
Psycho replied to bigbenbuilders's topic in PHP Coding Help
Isn't that the same page, just with different parameters on the query string? No wondering it is looping. Are you wanting to redirect it to the same page (with the parameters) because the page is dependant on the parameters? If so, no need to redirect, just set defaults for the parameters. Example: $option = (isset($_GET['option']))?$_GET['option']:'com_content'; $task = (isset($_GET['task']))?$_GET['task']:'category'; $sectionid = (isset($_GET['sectionid']))?$_GET['sectionid']:'16'; $id = (isset($_GET['id']))?$_GET['id']:'293'; $Itemid = (isset($_GET['Itemid']))?$_GET['Itemid']:'351'; -
[SOLVED] Select * from table where value = this or that
Psycho replied to clay1's topic in PHP Coding Help
Its just like PHP in that each condiftion must stand on it's own. You can't do where x = 1 or 2, you have to do where x = 1 or x = 2. I don't use postgresql, but this should be right if ($_POST['select'] == 'washington dc'){ $sql = "SELECT * from \"leads\" WHERE \"event city\" ~* '{$_POST['select']}' OR \"event city\" ~* 'alexandria' and \"date\" {$_POST['operator']} '{$y1}-{$m1}-{$d1}'"; -
Problem getting function to update Total box
Psycho replied to The.Pr0fess0r's topic in Javascript Help
If you want help with a JavaScript problem you are much more likely to get a response if you post the "JavaScript" and not the PHP code. You have JavaScript code that is dynamically generated based upon database results. Not knowing what is in your database I have no idea what that rendered code would look like. Please post code from the rendered HTML output from the script. If the error is in the core javascript we should be able to help. If the error has to do with the dynamic code we should be abble to identify that and then give next steps to further debug. -
On a second look, stripslashes() will un-escape single quotes in the content. But, if the content has a double quote your javascript will fail (becuase the js elements are defined with double quotes). I would suggest not using strpslashes and defining the javascript elements with single quotes - that way the escaped single quotes will be needed: PHP code while ($row = mysql_fetch_array($result)) { $states[] = "'{$row['name']}'"; } $jsStateList = implode(',', $states)
-
PHP code while ($row = mysql_fetch_array($result)) { $states[] = '"'.stripslashes($row['name']).'"'; } $jsStateList = implode(',', $states) Where you create the js code function StateSuggestions() { this.states = [ <?php echo wordwrap($jsStateList);?> ]; }
-
Search function functionality off a bit, quick fix, need a push
Psycho replied to bossman's topic in PHP Coding Help
The answer is right there in the comments of your script // grab the search types. $types = array(); $types[] = isset($_GET['ptitle'])?"`title` LIKE '%{$searchTermDB}%'":''; $types[] = isset($_GET['pdate'])?"`date` LIKE '%{$searchTermDB}%'":''; $types[] = isset($_GET['pindustry'])?"`industry` LIKE '%{$searchTermDB}%'":''; $types = array_filter($types, "removeEmpty"); // removes any item that was empty (not checked) if (count($types) < 1) $types[] = "`title` LIKE '%{$searchTermDB}%'"; // use the body as a default search if none are checked It states "use the body as a default search if none are checked". I don't see any way to "check" those three options in your form - therefore those fields are never used in the search criteria. I can make yoru page search by the year or by industry if I manually modify the URL. Either add checkboxes for the user to select those options or just include them in the search criteria by default. Search records with year value of 2009: http://www.adoberegistrations.com/ResourceCenters/OYilmaz/search_test/search.php?search=2009&pdate=true -
Why are you allowing users to add the same item to their cart multiple times? If the user tries to add an item to their cart which already exists in the cart then you should either add the new quantity to the existing quantity (typically seen in most online shopping sites) or provide some error condition. But, the answer to your question is to use a SUM() on the quantity field and do a GROUP BY based upon the item id.
-
Yes it is a lot of code and I doubt anyone is going to read through all of that. You need to take some time and perform some debugging to narrow down the problem. If you have verified that the POST data is received there is no reason to continue analyzing the form. Here are some things to test/verify: Verify the POST data is received by the processing page. Have you checked the spam folder for the email account you are sending to? Echo the mail() variables ($to,$subject,$message,$headers) to the page to ensure they are what you expect. If so, then you know everything is ok up till that point. This is probably the most important piece of information. If the variables aren't what they should be, then you need to trace back to the source of the problem.
-
You didn't state if that fixed your problem or not. I'm guessing not based upon the problems I see. Personally using the with() is a waste of time to just crete references you would only use once. Instead I would pass the form object to the script. But, one minor problem I see is the last test which checks if the query field is empty but then puts focus on the email field (using a bad reference). Also, all the Else If statements are unnecessary, you just need IF statements since you will do a return if any are true anyway. I have modified the code so that it will check for ALL errors and then alert the user. Much better user experience IMHO instead of having the user fix one error only to get another one on the next submission attempt. I also added a function called isEmpty() which will trim() the value in a field (removing leading and trailing spaces) before checking if the field is empty. Otherwise, someone could enter spaces and it would be accepted as valid input. <html> <head> <script language="JavaScript" type="text/javascript"> function isEmpty(fieldObj) { //Trims leading/trailing spaces fieldObj.value = fieldObj.value.replace(/^\s+|\s+$/g,''); //Return true/false on whether field is empty return (fieldObj.value==''); } function checkform(formObj) { var errors = new Array(); var focusObj = false; //perform all validations if(isEmpty(formObj.Name)) { errors[errors.length] = 'Name cannot be empty'; if(!focusObj) focusObj = formObj.Name; } if(isEmpty(formObj.Email)) { errors[errors.length] = 'Email cannot be empty'; if(!focusObj) focusObj = formObj.Email; } if(isEmpty(formObj.Phone)) { errors[errors.length] = 'Phone number cannot be empty'; if(!focusObj) focusObj = formObj.Phone; } if(isEmpty(formObj.Address)) { errors[errors.length] = 'Address cannot be empty'; if(!focusObj) focusObj = formObj.Address; } if(isEmpty(formObj.Query)) { errors[errors.length] = 'Query cannot be empty'; if(!focusObj) focusObj = formObj.Query; } //Check if any errors occured if(errors.length!=0) { var errorMsg = 'The following errors occured:\n'; errorMsg += '\n - ' + errors.join('\n - '); alert(errorMsg); focusObj.focus(); return false; } else { return true; } } </script> </head> <body> <form action="contact.php" method="get" onsubmit="return checkform(this);"/> <table> <tr> <td align="left" class="bodyText style12" width="100"> Name :</td> <td align="right" width="350"><p><input type="text" name="Name" size="28" maxlength="100" /></p></td> </tr> <tr> <td align="left" class="bodyText style12" width="100">Address :</td> <td align="right" width="350"><p><input type="text" name="Address" size="28" maxlength="100" /></p></td> </tr> <tr> <td align="left" class="bodyText style12" width="100">Phone:</td> <td align="right" width="350"><p><input type="text" name="Phone" size="28" maxlength="100" /></p></td> </tr> <tr> <td align="left" class="bodyText style12" width="100">Email:</td> <td align="right" width="350"><p><input type="text" name="Email" size="28" maxlength="100" /></p></td> </tr> <tr> <td align="left" class="bodyText style12" width="100">Your Query :</td> <td align="right" width="350"></p><textarea name="Query" cols="30" rows="2"></textarea></td></p></tr> <tr> <td align="right" colspan="2"> <p><input type="submit" name ="Submit" value="Email"/><input type="reset" name ="reset" value="Reset" /></p> </td> </tr> </table> </form> </body> </html>
-
[SOLVED] Hide a table row from one of its td's values
Psycho replied to guyfromfl's topic in Javascript Help
No need to make the code more complex than it need to be by having it read the content. I would go with your second option. If you can't modify the class in an effective manner you could also set an id for each row that is complete in a numerical sequence: c_1, c_2, c_3, etc; Then when the user selects complete, just create a loop that sets the display property for each row. You would use a while loop such that the loop will exit when there are no more IDs that match Ex: var idx = 1; while (document.gertElementById('c_'+idx)) { //set row object display property idx++; } -
I see a couple things wrong. 1. You have two while loops for pulling records from the query. Unless you reset the pointer to the first record after the first while loop, there are no records for the 2nd while loop to process. Besides you should just process the reocrds one. 2. Inthe query you are pulling the products.product_price field as ' price' - (note the space). I assume that is a typo. <?php function email() { require("class.phpmailer.php"); include "config.php"; mysql_connect("localhost","storaapu_admin","admin"); mysql_select_db("storaapu_storageinstyle"); $arremails='jacof@spign.co.za'; $sessid = session_id(); $a = $_SESSION['cart']['a']; $id = $_SESSION['cart']['id']; //Get the order details $order_data = ''; $grand_total = 85; $query = "SELECT cart.product_id as 'id', sum(cart.cart_qty) as 'a', cart.cart_qty, cart.cart_session_id, cart.cart_id, products.product_name as 'name', products.product_price as 'price',(products.product_price * cart.cart_qty) as 'total' FROM cart, products WHERE cart.cart_session_id LIKE '$sessid' AND products.product_id = cart.product_id GROUP BY cart.product_id"; $result = mysql_query($query); while($record = mysql_fetch_assoc($result)) { $extprice = number_format($record['price'] * $record['a'], 2); $grand_total += $record['total']; $order_data .= " <tr> <td align=\"center\" valign=\"center\">{$record['a']}</td> <td align=\"center\">{$record['id']}</td> <td align=\"center\">{$record['name']}</td> <td align=\"center\">{$record['price']}</td> <td>{$extprice}</td> </td><td align=\"center\">{$record['total']}</td> </tr>"; } //Format the grand total $grand_total = number_format($grand_total, 2); //Build the body of the email $body = "<table width=\"600\" border=\"0\" cellpadding=\"5\" cellspacing=\"1\"> <tr><td width=\"150\">First name</td><td class=\"content\">{$_SESSION['firstname']}</td></tr> <tr><td width=\"150\">Surname</td><td class=\"content\">{$_SESSION['surname']}</td></tr> <tr><td width=\"150\">Address</td><td class=\"content\">{$_SESSION['address']}</td></tr> <tr><td width=\"150\">Suburb</td><td class=\"content\">{$_SESSION['suburb']}</td></tr> <tr> <td width=\"150\">City</td><td class=\"content\">{$_SESSION['city']}</td></tr> <tr><td width=\"150\">Postal Code</td><td class=\"content\">{$_SESSION['pcode']}</td></tr> <tr><td width=\"150\">Postal Address</td><td class=\"content\">{$_SESSION['postaladdress']}</td></tr> <tr><td width=\"150\">Postal Suburb</td><td class=\"content\">{$_SESSION['postalsuburb']}</td></tr> <tr><td width=\"150\">Postal City</td><td class=\"content\">{$_SESSION['postalcity']}</td></tr> <tr><td width=\"150\">Postal Code</td><td class=\"content\">{$_SESSION['postalcode']}</td></tr> <tr><td width=\"150\">Telephone Number(W)</td><td class=\"content\">{$_SESSION['telw']}</td></tr> <tr><td width=\"150\">Telephone Number(H)</td><td class=\"content\">{$_SESSION['telh']}</td></tr> <tr><td width=\"150\">Cellphone Number</td><td class=\"content\">{$_SESSION['cell']}</td></tr> <tr><td width=\"150\">Email Address</td><td class=\"content\">{$_SESSION['email']}</td></tr> <tr><td width=\"150\">Fax Number</td><td class=\"content\">{$_SESSION['fax']}</td></tr> </table> <br> <table border=\"0\" width=\"600\" align=\"center\" cellpadding=\"5\"> <tr><td colspan=\"7\" bgcolor=\"#BAC6E3\">Product/Order Information</td></tr> <tr> <td>Quantity</td> <td>Product ID</td> <td>Item Name</td> <td>Price Each</td> <td>Extended Price</td> <td></td> <td></td> </tr> {$order_data} <tr> <td> </td> <td colspan=\"4\" align=\"left\" class=\"cart-table\"> Your total including shipping is:</td> <td align=\"center\" class=\"cart-table\">R {$grand_total}</td> <td> </td> </tr> </table>"; //Send the email $mail = new PHPMailer(); $mail->IsSMTP(); // telling the class to use SMTP $mail->Host = "mail.spign.co.za"; // SMTP server $mail->From = "info@something.com"; $mail->AddAddress($arremails); $mail->Subject = "Order Form"; $mail->IsHTML(true); $mail->Body = $body; if(!$mail->Send()) { } else { } } ?>
-
Is there a SIMPLE Active Directory PHP interface?
Psycho replied to Moron's topic in PHP Coding Help
So, look at the link I posted. Seems fairly straitforward to me -
Is there a SIMPLE Active Directory PHP interface?
Psycho replied to Moron's topic in PHP Coding Help
Think about what you are asking. You are wanting a PHP solution to get the user's AD username & password - without actually connecting to the AD server. Can you imagine how big of a security hole that would be if random websites could do that? A web app can't just grab information off the user's computer. I don't know of any way for a PHP app to grap the username and password for the user's AD session. You could have the user enter their AD username/password upon first login and authenticate them against AD as in this tuorial: http://www.davidschultz.org/2008/04/10/how-to-authenticate-against-active-directory-using-php/ However, if you want it so users don't have to always log in you could implement additional processes - at the expense of security. And, I'm sure your IT Admin would have a say about this. Here is one possibility: Have the user log in one time using their AD credentials. Upon successful authentication store their username & password in the database (encrypted for at least the password). Then also create a random hash value to be stored int he DB and as a cookie on the user's machine. Upon a subsequent access to the PHP application you would grab their credentials from the DB using the cookie value. Of course if the cookie expires or is deleted the user would have to log in again. Of course this opens a security risk in that anyone with access to that computer could get the value from the cookie and then use it to get logged in to the website as that person. -
There's no reason you can query the database and then construct the email based upon that query. Something must be wrong in the code you are using. Here is an example of how I would think it should be done (can't say for sure as I don't know enough about your app). I think your function is way too dependant upon session data. For an order I would expect all the pertinent data about an order to be stored in the DB. But, anyway, this is just an example - can't guarantee it will work, but should point you in the right direction. function email() { require("class.phpmailer.php"); include "config.php"; $arremails='blah@something.com'; $sessid = session_id(); $a = $_SESSION['cart']['a']; $id = $_SESSION['cart']['id']; //Get the order details $order_data = ''; $query = "SELECT * FROM orders WHERE id='$id'"; $result = mysql_query($query); while($record = mysql_fetch_assoc($result)) { $order_data .= " <tr> <td align=\"center\" valign=\"center\">{$a}</td> <td align=\"center\">{$id}</td> <td align=\"center\">{$record['name']}</td> <td align=\"center\">{$record['price']}</td> <td align=\"center\"></td> </tr>"; } //Build the body of the email $body = "<table width=\"600\" border=\"0\" cellpadding=\"5\" cellspacing=\"1\"> <tr><td width=\"150\">First name</td><td class=\"content\">{$_SESSION['firstname']}</td></tr> <tr><td width=\"150\">Surname</td><td class=\"content\">{$_SESSION['surname']}</td></tr> <tr><td width=\"150\">Address</td><td class=\"content\">$addess</td></tr> <tr><td width=\"150\">Suburb</td><td class=\"content\">{$_SESSION['suburb']}</td></tr> <tr> <td width=\"150\">City</td><td class=\"content\">{$_SESSION['city']}</td></tr> <tr><td width=\"150\">Postal Code</td><td class=\"content\">{$_SESSION['pcode']}</td></tr> <tr><td width=\"150\">Postal Address</td><td class=\"content\">{$_SESSION['postaladdress']}</td></tr> <tr><td width=\"150\">Postal Suburb</td><td class=\"content\">{$_SESSION['postalsuburb']}</td></tr> <tr><td width=\"150\">Postal City</td><td class=\"content\">{$_SESSION['postalcity']}</td></tr> <tr><td width=\"150\">Postal Code</td><td class=\"content\">{$_SESSION['postalcode']}</td></tr> <tr><td width=\"150\">Telephone Number(W)</td><td class=\"content\">{$_SESSION['telw']}</td></tr> <tr><td width=\"150\">Telephone Number(H)</td><td class=\"content\">{$_SESSION['telh']}</td></tr> <tr><td width=\"150\">Cellphone Number</td><td class=\"content\">{$_SESSION['cell']}</td></tr> <tr><td width=\"150\">Email Address</td><td class=\"content\">{$_SESSION['email']}</td></tr> <tr><td width=\"150\">Fax Number</td><td class=\"content\">{$_SESSION['fax']}</td></tr> </table> <br> <table border=\"0\" width=\"600\" align=\"center\" cellpadding=\"5\"> <tr><td colspan=\"7\" bgcolor=\"#BAC6E3\">Product/Order Information</td></tr> <tr> <td>Quantity</td> <td>Product ID</td> <td>Item Name</td> <td>Price Each</td> <td>Extended Price</td> <td></td> <td></td> </tr> {$order_data} </table>"; //Send the email $mail = new PHPMailer(); $mail->IsSMTP(); // telling the class to use SMTP $mail->Host = "mail.something.com"; // SMTP server $mail->From = "info@something.com"; $mail->AddAddress($arremails); $mail->Subject = "Order Form"; $mail->IsHTML(true); $mail->Body = $body; if(!$mail->Send()) { echo "<script language=JavaScript>window.location=\"mailfail.html\"</script><br>"; } else { echo "<script language=JavaScript>window.location=\"thankyou.html\"</script><br>"; } }