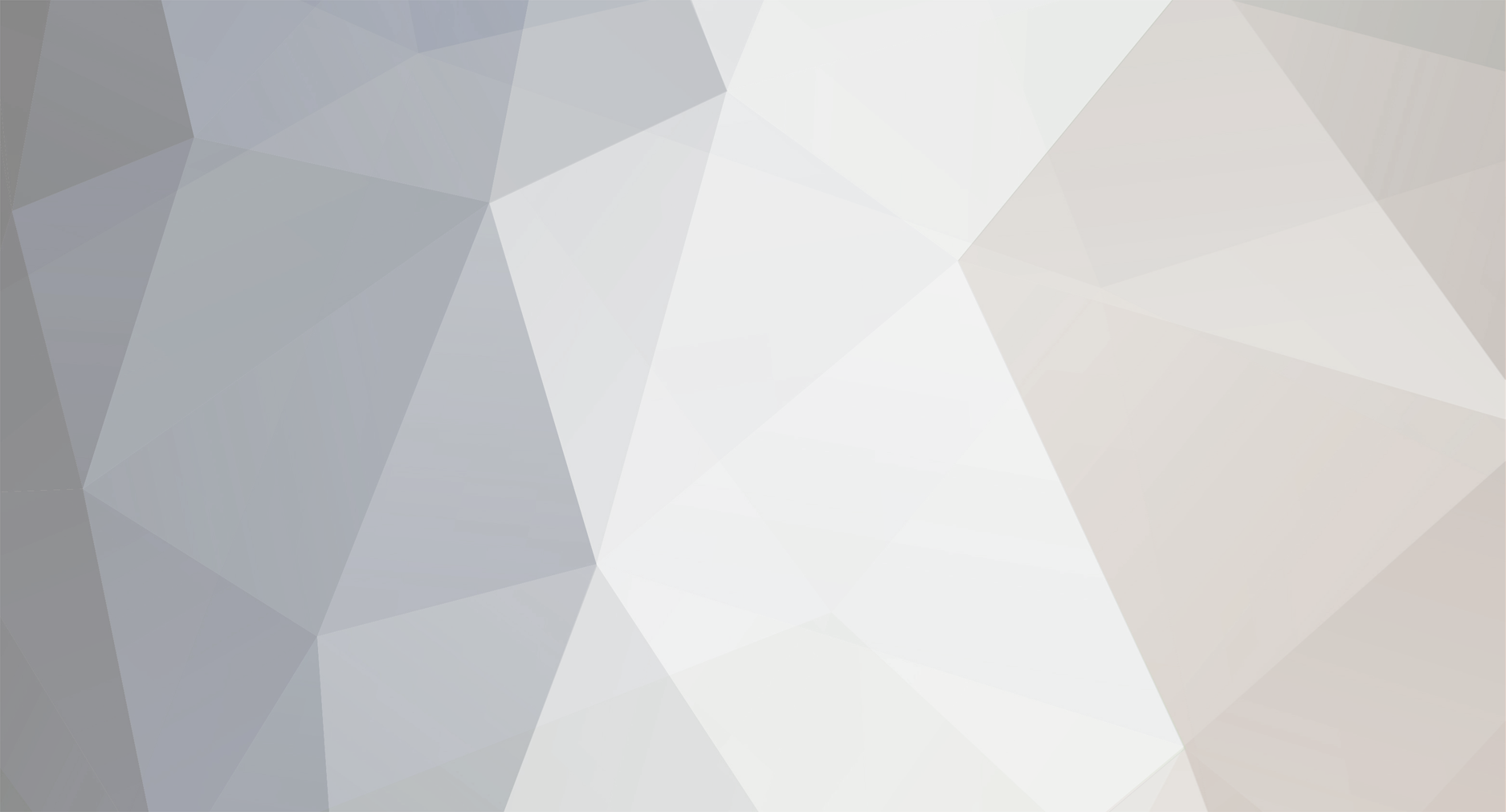
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
From your statements I am assuming you are posting the data twice. Are you populating the first post data into hidden fields? Are the parameters of those hidden fields enclosed in single quotes? If so, that secondary page would have input fields that look like this: <input type='hidden' name='Men's Roller Blades' value='0' /> So, when it gets to the apostrophe in "Men's" it thinks that is the end of the name value for that field. You should do one or more of the following: 1) Use double quotes (but ensure you don't have any values with double quotes) 2) Escape any quotes in the parameter values 3) Don't "double post", instead save the first post data to session variables.
-
I'm getting blank emails using php contact forms
Psycho replied to phpfan28's topic in PHP Coding Help
Do you realize even though you have some error checking for name, email and comments that you do absolutely nothing different if there are errors? If someone submits the form with nothing entered you will get a blank email. You should not rely on JavaScript for validation since people can have it turned off. It's a nice user feature, but it should be used as an add-on to server-side validation. By the way, your blocks of code to validate have an else that echo's an empty string. Why? Try something like this: <?php function safe($string) { $pattern = "/ | |%0a|%0d|Content-Type:|bcc:|to:|cc:/i"; return preg_replace($pattern, '', $string); } $errors = array(); if(empty($_POST['name'])){ $errors[] = 'Please enter your name'; } if(empty($_POST['email'])){ $errors[] = 'Please enter your email'; } if(empty($_POST['comments'])){ $errors[] = 'Please enter your comments'; } if (count($errors)>0) { //Only a very basic error handler //You could alternatively send user back to the form echo "The follwing errors occured:<br> "; foreach ($errors as $error) { echo " - $error<br> "; } } else { $emailPattern = '/^[^@s]+@([-a-z0-9]+.)+[a-z]{2,}$/i'; $to = "service@rodriguezstudios.com"; //$to ="atlnycdude23@gmail.com"; // ----> Use this $to email declation just in case in a emergency. $subject = 'Sales Inquiries, Potential/ReturningCustomer Related Questions'; $from = 'Rodriguez Studios'; $name = safe (stripslashes( $_POST['name']) ); $email = safe($_POST['email'] ); $phone = safe($_POST['phone'] ); $reasons = safe ($_POST['reasons']); $search = safe( $_POST['search']); $facebook = safe($_POST['facebook'] ); $wsb = safe ( $_POST['wsb']); $other = safe( $_POST['other']); $comments = safe(stripslashes($_POST['comments']) ); $headers = "From: ". $from . "<" . $to. "> "; $headers .= "Reply-To: " . $email . " "; $headers .= "Return-path: ". $email; $message .= "Name: " . $name . " "; $message .= "Email: " . $email . " "; $message .= "Phone Number: " . $number . " "; $message .= "Reasons: " . $reasons . " "; $message .= "Facebook: " . $facebook . " ";; $message .= "WSB-TV: " . $wsb . " "; $message .= "Other: " . $other . " "; $message .= "Comments: " . $comments . " "; if (mail($to,$subject,$message,$headers)){ echo "Thank you so much for your Inquiry. I'm looking forward in serving you soon!"; } else { echo "&Result=error"; } } ?> -
[SOLVED] Help with dates and dates datatype with sql and php.
Psycho replied to Eggzorcist's topic in PHP Coding Help
Look at your query a little closer. You are not appending $filtertime to the query; the periods are within the double quotes that you are using to define your query. The result would look something like this SELECT * FROM events WHERE user = '[username]' and fromslashes > '.[filtertime].' If you were to have done a simple debug to echo the query out you would have found the error. When using double quotes to define a string there is no need to exit the quote to append a variable, but I like to encapsulate $filtertime = date("Y-m-d",mktime(0, 0, 0, date("m") , date("d")+5, date("Y"))); $query = "SELECT * FROM events WHERE user = '{$user}' and fromslashes > '{$filtertime}"; -
function checkAllBox(formObj, fieldName, checkedState) { if(formObj[fieldName].length) { var fieldLen = formObj[fieldName].length; for(var i=0; i<fieldLen; i++) { formObj[fieldName][i].checked = checkedState; addToList(formObj[fieldName][i], 'txt1'); } } else { formObj[fieldName].checked = checkedState; addToList(formObj[fieldName], 'txt1'); } return; }
-
Here is a better structure. have th emain page check if form was submitted, is so check for errors. If the form was not submitted or there were post errors, display the form (with the previously submitted values) If there were no errors send the email and then redirect (with header() to the appropriate page) Point the user to contact.php, form.php is just an include page contact.php <?php if (isset($_POST)) { // get posted data into local variables $EmailFrom = "myemail@gmail.com"; $EmailTo = "myemail@gmail.com"; $Subject = "My Subject"; $FirstName = Trim(stripslashes($_POST['FirstName'])); $LastName = Trim(stripslashes($_POST['LastName'])); $Company = Trim(stripslashes($_POST['Company'])); $Street1 = Trim(stripslashes($_POST['Street1'])); $Street2 = Trim(stripslashes($_POST['Street2'])); $City = Trim(stripslashes($_POST['City'])); $State = Trim(stripslashes($_POST['State'])); $PostCode = Trim(stripslashes($_POST['PostCode'])); $Tel = Trim(stripslashes($_POST['Tel'])); $email = Trim(stripslashes($_POST['email'])); $update = Trim(stripslashes($_POST['update'])); if (empty($FirstName) || empty($LastName) || empty($Company) || empty($Street1) || empty($City) || empty($State) || empty($PostCode) || empty($Tel) || empty($email)) { $error = "All fields are required, please try again."; } else { // prepare email body text $Body = "FirstName: $FirstName\n"; $Body .= "LastName: $LastName\n"; $Body .= "Company: $Company\n"; $Body .= "Street1: $Street1\n"; $Body .= "Street2: $Street2\n"; $Body .= "City: $City\n"; $Body .= "State: $State\n"; $Body .= "PostCode: $PostCode\n"; $Body .= "Tel: $Tel\n"; $Body .= "email: $email\n"; $Body .= "update: $update\n"; // send email $success = mail($EmailTo, $Subject, $Body, "From: <$EmailFrom>"); // redirect to success page if ($success){ header("Location: thankyou.html"); } else{ header("Location: header.php"); } } } //If data is not posted or if there was //an error is submisstion, display the form include("form.php"); ?> form.php <?php $stateAry = array( 'AL' => 'Alabama', 'AK' => 'Alaska', 'AZ' => 'Arizona', 'AR' => 'Arkansas', 'CA' => 'California', 'CO' => 'Colorado', 'CT' => 'Connecticut', 'DE' => 'Delaware', 'DC' => 'District of Columbia', 'FL' => 'Florida', 'GA' => 'Georgia', 'HI' => 'Hawaii', 'ID' => 'Idaho', 'IL' => 'Illinois', 'IN' => 'Indiana', 'IA' => 'Iowa', 'KS' => 'Kansas', 'KY' => 'Kentucky', 'LA' => 'Louisiana', 'ME' => 'Maine', 'MD' => 'Maryland', 'MA' => 'Massachusetts', 'MI' => 'Michigan', 'MN' => 'Minnesota', 'MS' => 'Mississippi', 'MO' => 'Missouri', 'MT' => 'Montana', 'NE' => 'Nebraska', 'NV' => 'Nevada', 'NH' => 'New Hampshire', 'NJ' => 'New Jersey', 'NM' => 'New Mexico', 'NY' => 'New York', 'NC' => 'North Carolina', 'ND' => 'North Dakota', 'OH' => 'Ohio', 'OK' => 'Oklahoma', 'OR' => 'Oregon', 'PA' => 'Pennsylvania', 'RI' => 'Rhode Island', 'SC' => 'South Carolina', 'SD' => 'South Dakota', 'TN' => 'Tennessee', 'TX' => 'Texas', 'UT' => 'Utah', 'VT' => 'Vermont', 'VA' => 'Virginia', 'WA' => 'Washington', 'WV' => 'West Virginia', 'WI' => 'Wisconsin', 'WY' => 'Wyoming', ); $stateOptions = ''; foreach($stateAry as $stateAbbrv => $stateName) { $selected = ($stateAbbrv==$_POST['State']) ? ' selected="selected"' : '' ; $stateOptions .= " <option value=\"{$stateAbbrv}\"{$selected}>{$stateName}</option>"; } ?> <html> <body> <div style="color:red;"><?php echo $error; ?></div> <form id="InformationRequest" name="InformationRequest" method="post" action="<?php echo $_SERVER[php_SELF]; ?> " onSubmit="return checkform(this)"> <table width="340" border="0" cellspacing="0" cellpadding="2" align="center"> <tr> <td class="info">First Name*:</td> <td class="infoInput"><input type="text" name="FirstName" id="FirstName" value="<?php echo $_POST['FirstName']; ?>" tabindex="0" /></td> </tr> <tr> <td class="info">Last Name*:</td> <td class="infoInput"><input type="text" name="LastName" id="LastName" value="<?php echo $_POST['LastName']; ?>" tabindex="1" /></td> </tr> <tr> <td class="info">Company*:</td> <td class="infoInput"><input type="text" name="Company" id="Company" value="<?php echo $_POST['Company']; ?>" tabindex="2" /></td> </tr> <tr> <td class="info">Address*:</td> <td class="infoInput"><input type="text" name="Street1" id="Street1" value="<?php echo $_POST['Street1']; ?>" tabindex="3" /></td> </tr> <tr> <td class="info">Address 2:</td> <td class="infoInput"><input type="text" name="Street2" id="Street2" value="<?php echo $_POST['Street2']; ?>" tabindex="4" /></td> </tr> <tr> <td class="info">City*:</td> <td class="infoInput"><input type="text" name="City" id="City" value="<?php echo $_POST['City']; ?>" tabindex="5" /></td> </tr> <tr> <td class="info">State*:</td> <td class="infoInput"><select name="State" id="State"> <option value="">Pick your state</option> <?php echo $stateOptions; ?> </select> </td> </tr> <tr> <td class="info">Zip*:</td> <td class="infoInput"><input type="text" name="PostCode" id="PostCode" value="<?php echo $_POST['PostCode']; ?>" tabindex="6" /></td> </tr> <tr> <td class="info">Email*:</td> <td class="infoInput"><input type="text" name="email" id="email" value="<?php echo $_POST['email']; ?>" tabindex="7" /></td> </tr> <tr> <td class="info">Phone*:</td> <td class="infoInput"><input type="text" name="Tel" id="Tel" value="<?php echo $_POST['Tel']; ?>" tabindex="8" /></td> </tr> <tr> <td class="info"><input type="reset" name="reset" id="reset" value="Reset" /></td> <td class="infoInput"><input type="submit" name="submit" id="submit" value="Submit" /></td> </tr> </table> </form> </body> </html>
-
If I have a table created like this <table class="build"> Is there a way, in my style sheet, to reference TH cells in that table? Something like this (although it is wrong): table.build.th { color: #ff0000; } EDIT: Damn, I knew I'd find it right after posting table.build th
-
Good thing I made the checkboxes an array by givign them the same name. Here's a simple function to do that. I also made some other corrections: 1. Do not use FONT tags, they have been deprecated for amny years. Use inline style properties or, even better, a style sheet and classes. 2. Modifies readonly attribue in textarea 3. Added closing "/" to input fields to make them compliant. <html> <head> <title>Fruits</title> <script type="text/javascript"> function addToList(checkObj, outputObjID) { var checkGroup = checkObj.form[checkObj.name]; var checkGroupLen = checkGroup.length; var valueList = new Array(); for (var i=0; i<checkGroupLen; i++) { if (checkGroup[i].checked) { valueList[valueList.length] = checkGroup[i].value; } } document.getElementById(outputObjID).value = valueList.join('\r\n'); return; } function checkAllBox(formObj, fieldName, checkedState) { if(formObj[fieldName].length) { var fieldLen = formObj[fieldName].length; for(var i=0; i<fieldLen; i++) { formObj[fieldName][i].checked = checkedState; } } else { formObj[fieldName].checked = checkedState; } return; } </script> </head> <body> <form name="myform"> <input type="checkbox" name="checkAll" value="all" onClick="checkAllBox(this.form, 'fruit[]', this.checked);" /><b>Check All</b><br> <input type="checkbox" name="fruit[]" value="Oranges" onClick="addToList(this, 'txt1');" /><span style="color:#808080">Oranges</span><br> <input type="checkbox" name="fruit[]" value="Apples" onClick="addToList(this, 'txt1');" /><span style="color:#808080">Apples</span><br> <input type="checkbox" name="fruit[]" value="Grapes" onClick="addToList(this, 'txt1');" /><span style="color:#808080">Grapes</span><br> <textarea rows="4" cols="10" name="txt1" id="txt1" style="color:#808080" readonly="readonly"></textarea> </form> </body> </html>
-
I would not use regex for this. I think the problem is due to how IE and FF interpret new lines. But, anyway I wouldl completely repopulate the field on every checkbox change: <html> <head> <title>Fruits</title> <script type="text/javascript"> function addToList(checkObj, outputObjID) { var checkGroup = checkObj.form[checkObj.name]; var checkGroupLen = checkGroup.length; var valueList = new Array(); for (var i=0; i<checkGroupLen; i++) { if (checkGroup[i].checked) { valueList[valueList.length] = checkGroup[i].value; } } document.getElementById(outputObjID).value = valueList.join('\r\n'); return; } </script> </head> <body> <form name="myform"> <input type="checkbox" name="fruit[]" value="Oranges" onClick="addToList(this, 'txt1')"><font color="#808080">Oranges</font><br> <input type="checkbox" name="fruit[]" value="Apples" onClick="addToList(this, 'txt1')"><font color="#808080">Apples</font><br> <input type="checkbox" name="fruit[]" value="Grapes" onClick="addToList(this, 'txt1')"><font color="#808080">Grapes</font><br> <textarea rows="4" cols="10" name="txt1" id="txt1" style="color:#808080" readonly></textarea> </form> </body> </html>
-
The issue you are having is with what is called variable scope (google for more info). A variable created in a function is not available outside the function, unless you specify it as such. Personally, I would write your code like this function add($a,$b) { return ($a+$b); } $a=5; $b=8; $total = add($a, $b); echo "$a + $b = $total<br />";
-
Utterly terrible database design. The "ranks" should be stored in a separate table with a reference back to the parent record. But, if you are going to use this crap, this should work: $uquery = mysql_query("select userid,username,membergroupids from user") or die(mysql_error()); $ranks = array(); while ($uresult = mysql_fetch_array($uquery)) { $groupIDs = explode(',', $uresult['membergroupids']); if (in_array('32', $groupIDs)) { $ranks['Officer'] = $uresult['username']; } elseif (in_array('31', $groupIDs)) { $ranks['Junior Officer'] = $uresult['username']; } } foreach($ranks as $rankName => $members) { echo "<b>{$rankName}</b><br /> "; foreach($members as $memberName) { echo "{$memberName}<br /> "; } }
-
Any suggestions for what, it works right? That is the ternary operator, which some refer to as the shorthand notation for an if/else. Yes you can use an IF/ELSE statement, but if you don't understand it that should be an incentive to learn something new instead of not using it because you don't understand it. The pice of code is multiplying the second value by 1 id the operation is add or by -1 if the operation is subtract. The total calculation is val1 + val2 * (1 or -1) So the calcualtion is always adding the values, but in the case of a subtract operation it will simply change the sign of the second value. Here is the long version of the total calculation: if (op=='add') { var total = param1 + param2; } else { var total = param1 1 param2; }
-
1. When you define type, you are only creaating a reference to the radio GROUP. A GROUP does not have a value, the options do. You could either iterate through each option in the group and find which one is checked and then get the value of that option OR, even easier, pass a parameter to the function. 2. You never define the result object to assign the innerHTML value to. <html> <head> <script type="text/javascript"> function calculator(op, resultID) { var param1 = parseFloat(document.calcform.box1.value); var param2 = parseFloat(document.calcform.box2.value); var total = param1 + param2 * ((op=='add')?1:-1); document.getElementById(resultID).innerHTML = total; } </script> </head> <body> <form name="calcform"> <label> <input name="box1" type="text" id="num" value="4"> </label> <label> <input name="box2" type="text" id="num" value="5"> </label> <label> <input type="radio" name="radio" id="radio" value="add" onClick="calculator('add', 'result');"> add </label> <label> <input type="radio" name="radio" id="radio" value="subtract" onClick="calculator('sub', 'result');"> subtract </label> <div id="result">output is here</div> </form> </body> </html>
-
I'm only going to address the issue you present regarding the upload of images before form submission. This is how I would approach that problem. I woulld have two forms on the page. The main form would be for the advert details and the second form at the bottom would be for uploading images. When the page is first displayed I would use some randomly generated value that is inserted into hidden fields in the forms when the page is created by PHP. Then I would use AJAX when using the form to upload images. The AJAX call would submit the data (i.e. File) and return back a path to the image so you can use JavaScript to display the image without reloading the page. When saving the file details to the database you would use the randomly generated value to identify those images. Then when the user finally submits the advert the process to add the advert to the DB would get the ID of the new advert record and then perform a query on the images table to change the random code to the actual ID. (Actually I'd probably use a separate table for the temp images until the advert is finalized)
-
Also, the logic in your while loop will not work. Not sure what you are trying to accomplish. Added comments to your code to explain why you aren't getting any results. //Assigns the VALUE of the current element in the array while ($new = current($row)) { //key() will attempt to return the KEY of the current element of the array //However, $new is the extracted VALUE, so key() will return nothing in this instance echo key($new).'<br />'; next($row); }
-
From WikiPedia: I could be wrong, but it looks pretty obvious to me that what he is wanting to accomplish is exactly like a pyramid scheme. Granted, it may not be illegal where he is from, but it would be shady nonetheless.
-
I agree. It is also illegal in many places: Pyramid Scheme
-
There are also 3rd party utilities that will convert HTML code into an image representatin of the web page - they don't take a screen grap of the page, they dynamically create an image based upon the code. I don't know if there are any free ones. Here's one that you could use on a web server and utilize through PHP: http://www.converthtmltoimage.com/ (assuming you are not on a shared host) And another: http://www.guangmingsoft.net/htmlsnapshot/help.htm
-
What is the point of that. If you want the "text" of the select field, then just make the value the same as the text. Trying to manipulate the values on submission is completely unnecessary and would fail for anyone without javascript enabled
-
Um, GET data is no more/less secure than POST data. A user can modify POST data just as easily than GET data. If you are not validating/cleansing ALL user data (GET, POST & COOKIE) you are not secure.
-
Yeah, that was different than I thought too, but you know, I think the problem is even simpler than that. Just find the max horizontal or vertical distance! The shorter distance will be completed first, but you still need to complete the longer distance. It wasn't until I saw your code above that it dawned on me. //Total moves needed between start and end in rows and columns $distance = max(abs($row-$dr), abs($col-$dc)); By the way, why so long since your last post?
-
Was just going to suggest that. On each iterration of the while loop move 1 step vertically (if there is space) and one step horizontally (again, if there is space). But, consider each iteration of the loop as one move.
-
Start Option values and end options values are now offset by one hour <?php function createTimeOptions($start, $end) { $options = ''; for ($time=$start; $time<=$end; $time+=.25) { $hours = floor($time); $minutes = ($time-$hours) * 60; $timeStr = date("g:i a", mktime($hours, $minutes, 0, 1, 1, 2000)); $options .= "<option value=\"{$timeStr}\">{$timeStr}</option>\n"; } return $options; } ?> <html> <head> <script type="text/javascript"> function updateEndTime(beginTimeSelectObj) { var beginTimeIdx = beginTimeSelectObj.selectedIndex; var endTimeSelectObj = document.getElementById('end'); endTimeSelectObj.selectedIndex = beginTimeIdx; var optionColor; for (var endTimeIdx=0; endTimeIdx<endTimeSelectObj.options.length; endTimeIdx++) { optionColor = (endTimeIdx<beginTimeIdx) ? '#cecece' : ''; endTimeSelectObj.options[endTimeIdx].style.backgroundColor = optionColor; } return; } function validateEndTime(endTimeSelectObj) { var beginTimeSelectObj = document.getElementById('start'); if (endTimeSelectObj.selectedIndex<beginTimeSelectObj.selectedIndex) { endTimeSelectObj.selectedIndex = beginTimeSelectObj.selectedIndex; } return; } </script> </head> <body> Start: <select name="start" id="start" onchange="updateEndTime(this);"> <?php echo createTimeOptions(8, 22); //8am to 10pm ?> </select> <br> End: <select name="end" id="end" onchange="validateEndTime(this);"> <?php echo createTimeOptions(9, 23); //9am to 11pm ?> </select> </body> </html>
-
At first I didn't see anything, but on second look I can see two things that would cause it to fail: 1. Character case: if the case of the word in your database didn't match the case of the word in the text, it wouldn't be replaced 2. Incorrect logic in your loop. You start with the variable "$string". Then on the first pass you define the variable $str using str_replace() on $string. So, $str would have the replacement of the first bad word. But, on the next pass you would be doing a str_replace() again on $string (the original text) so you would have lost any replacement from the first pass. So, in the end you would ONLY get the result of the last word processed against the original $string value.
-
Does the swear word get displayed on the page in the first example from this line? echo $word . "<br/>"; If not, are you sure the query returns any results and/or that the results have a column named 'word'? In any event, the process above is not very efficinet and, worse, will make improper replacements. For example, this "shitzu dogs" would become this "***zu dogs". You need to use preg_replace(). Add all the bad words to an array as a regular expression using the word boundry metacharacter to find whole words, along with the case insensitive switch. This will ensure that only whole word matches are replaced. <?php include('../../includes/inc.conn.php'); function swear_filter($string) { $words = array(); $query = "SELECT word FROM bad_words"; $result = mysql_query($query) or die(mysql_error()); while($row = mysql_fetch_array($result)){ $words[] = '/\b' . $row['word'] . '\b/i'; } return preg_replace($words, '***', $string); } $string_to_check = "My shitzu took a Shit on the carpet."; echo swear_filter($string_to_check); //Assuming the word 'shit' is in the db results //Output: "My shitzu took a *** on the carpet" ?>
-
Yeah, I'm sure they won't mind you doing that.