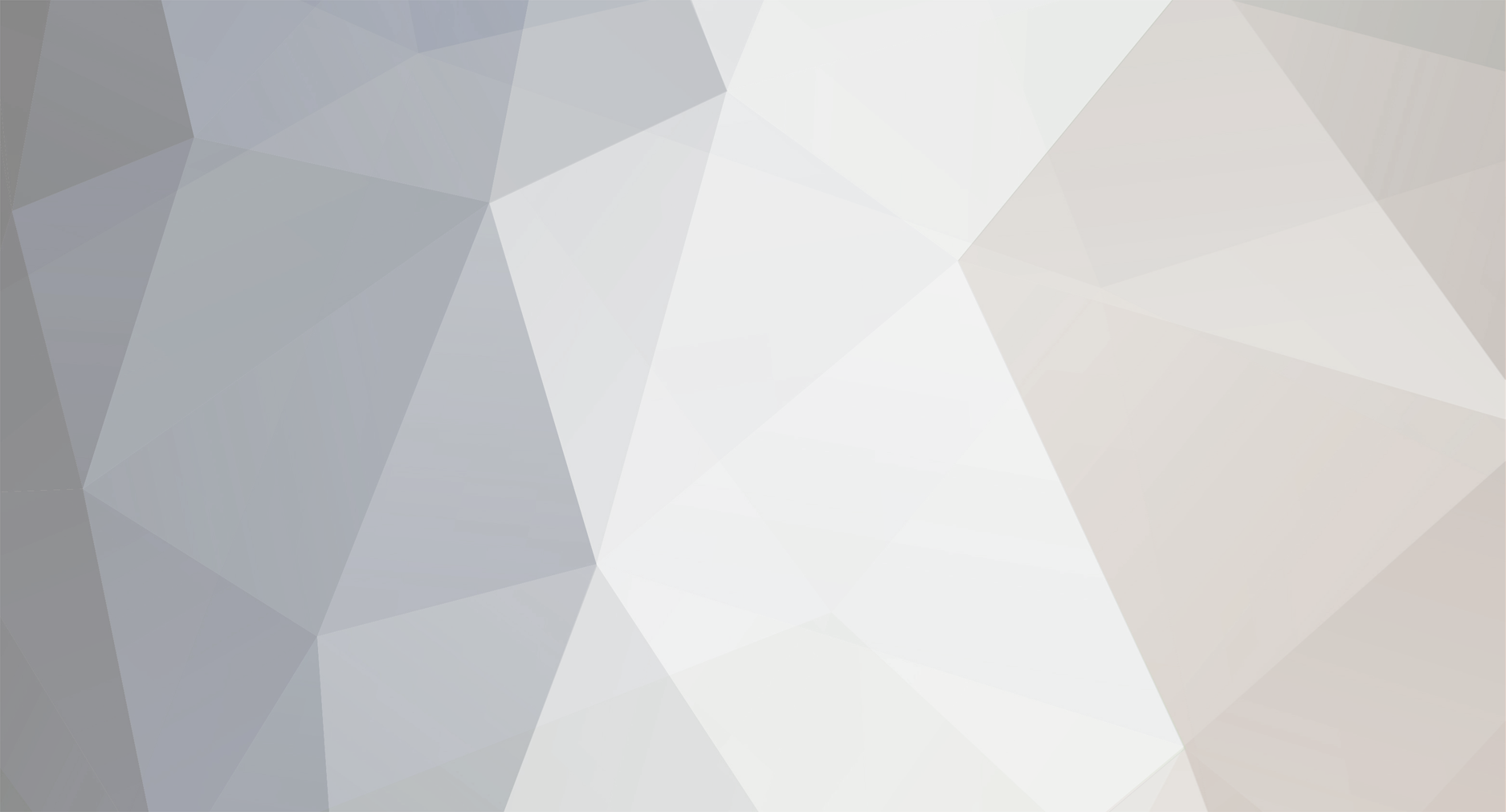
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Well, MD5 is not encryption. It could work - assuming VB Script supports the MD5 algorithm. But, there are two potential problems with that: 1) MD5 cannot be undone. So, if you are planning to pass an MD5 value from one place to another and to be able to read the original value, that will not work. 2) If you are only using the value as a checksum (i.e. check the MD5 value passed to the MD5 of another known value) then it might work. But, if you are passing the value on the URL, others will see it. As long as they do not know it is the MD5 of a date you are fine, but if they were to figure it out they could generate MD5 values as they need them. I'm not really clear on how you plan to use this. But, option #2 - if applicable - could work for you, just use a salt in the process. However, if you need a value that can be decrypt, then I would suggest creating your own process for encrypting/decrypting. If this was for protecting government or business secrets then I would suggest looking for a true encryption process that was cross-platform compatible. But, since this is about server abuse I think you could come up with some method that will sufficiently deter abuse. Here's a simple example: Date: 10-05-2009 Reverse each group of characters: 01-05-9002 remove dashes: 01059002 Replace numbers with character equivalent: ABFAJAAC You could then write the reverse process in a different language to get the original value. Now that is only a very simple example and in no way a method to secure data. But, I think it may be a sufficient deterrent for server abuse.
-
What's wrong with using a standard auto-increment INT field and start it at whatever value you want. It's true that it won't be padded to 8 characters, but that is a display issue. By keeping the field as an INT type you get much more flexibility; e.g. select where field > [some_value]. Then whenever you want to display the field simply use string_pad() or some other methos to display with it padded to eight characters
-
[SOLVED] How to randomize mysql_fetch_array($result) ?
Psycho replied to deko's topic in PHP Coding Help
I can't see how having multiple tables is worthwhile. Yes, using RAND() is not a speedy process, but what is the performance (or lack of) using a number of records that you expect to have? How often will you run that process? Does it need to be randomized each time or can it only be randomized say once per day? All of these should play into your decision. Good luck -
[SOLVED] How to randomize mysql_fetch_array($result) ?
Psycho replied to deko's topic in PHP Coding Help
I forget the correct terminology, but in a query you can give a table or field an alternate name. This is a necessity when trying to join the same table on itself. So, in the query with 'image1' and 'image2' I referenced the image table two times and gave each an alternate name or reference to differentiate betweent he two. I use table references a lot. I typically like to give my table descriptive names so I know what they are, but then in my query I will use short references for ease of implementation -
[SOLVED] How to randomize mysql_fetch_array($result) ?
Psycho replied to deko's topic in PHP Coding Help
Once you have the array of all elements, randomized the array, and spliced the array, why do you need to loop over them again? I thought the whole idea was to get a random set of records. Once you have that array of 25 elements, just do another query to get the records for those 25 using implode on the array. I noticed I did have an error in creating those queries. When using implode you need to have the array elements separated by a comma. If you go with option 3, this code will result in $result contain the 25 random records: //Get 25 random IDs from the DB $query = "SELECT id FROM image"; $result = mysql_query($query); //Populate IDs into an array while ($record = mysql_fetch_assoc($result)) { $allIDs[] = $record['id']; } //Randomize the array shuffle($allIDs); //Get the first 25 records/IDs $randIDs = array_slice($allIDs, 0, 25); //Query for the full records to the 25 random IDs $query = "SELECT * FROM image WHERE id IN (" . implode(',', $randIDs) . ")"; $result = mysql_query($query); //$result will contain a record set of the full records for the 25 IDs Not tested so there may be other syntax errors. -
[SOLVED] How to randomize mysql_fetch_array($result) ?
Psycho replied to deko's topic in PHP Coding Help
I'm not sure what the most efficient method would be. I'll give you three and you can see which is fastest by doing some tests: Option 1: Using a JOIN query. Actually I'm not sure if this would be any faster than doing a RAND on the original query, but it's worth trying SELECT image1.id AS randID, image2.* FROM image m1 LEFT JOIN image image2 ON image1.id = image2.id ORDER BY RAND() LIMIT 25 Option 2: Do one query to get the IDs and a second to get the data $query = "SELECT id FROM image ORDER BY RAND() LIMIT 25"; $result = mysql_query($query); while ($record = mysql_fetch_assoc($result)) { $randIDs[] = $record['id']; } $query = "SELECT * FROM image WHERE id IN (" . implode('', $randIDs) . ")"; $result = mysql_query($query); Option 3: Get ALL ids and use PHP to get 25 random ones $query = "SELECT id FROM image"; $result = mysql_query($query); while ($record = mysql_fetch_assoc($result)) { $allIDs[] = $record['id']; } //Randomize the array shuffle($allIDs); //Get the first 25 records $randIDs = array_slice($allIDs, 0, 25); $query = "SELECT * FROM image WHERE id IN (" . implode('', $randIDs) . ")"; $result = mysql_query($query); -
The way I understood the request he wants to trigger an event if the cursor moves from one specific div directly to another specific div. If so, the logic above work unless you put an onmouseout or over on every other element on the page to reset the global variable. Suppose the user mouses out of the fixed div into "white space" on the page and then selects the second div. Unless there was an event to reset the var "lastVisited" it won't make a difference if the user took 1 millisecond or 1 hour to go from one div to the other since "lastVisited" will still be true. Here is some code I put together. This may be a little more extravagant than you need. Basically, it allows you to do a test to see if the user has gone directly from one object to another. For all of the "from" objects you will have to add an onmouseout event to call "setOutTime(this)" so the system can capture the out time from that object. Then for the onmouseover objects in the funtion you want to trigger you will need to pass the ID of the from object you are testing for. Then call "onMouseFromObj(obj2ID, 5)" to test whether the user moused directly from that object. I used '5' for 5 milliseconds. In testing I noticed that the number of milliseconds recorded from moving from one object to another, which were directly next to each other, varied from 0 to 1. But, if the objects are not directly next to each other you may need to modify that value. Here is the test page <html> <head> <style> #obj1 {width:100px;height:100px;background-color:#cc0000;} #obj2 {width:100px;height:100px;background-color:#00cc00;} #obj3 {width:100px;height:100px;background-color:#0000cc;} </style> <script type="text/javascript"> var outTimes = new Array(); function setOutTime(obj) { var now = new Date(); outTimes[obj.id] = now.valueOf(); } function onMouseFromObj(obj2ID, limit) { var now = new Date(); var objOnTime = now.valueOf(); //For debugging only (can be removed in final implementation) var output = (objOnTime-outTimes[obj2ID]) + ' milliseconds passed between object 2 and current object'; document.getElementById('output').innerHTML = output; return ((objOnTime-outTimes[obj2ID])<=limit); } function showAlert(obj1, obj2ID) { if (onMouseFromObj(obj2ID, 5)) { alert ('true'); } } </script> </head> <body> A mouseover from 2 to 1 or from 2 to 3 will trigger an alert<br /><br /> <div id="obj1" onmouseout="setOutTime(this);" onmouseover="showAlert(this, 'obj2');"><br /><br />Object 1<br /><br /></div> <div id="obj2" onmouseout="setOutTime(this);" ><br /><br />Object 2<br /><br /></div> <div id="obj3" onmouseout="setOutTime(this);" onmouseover="showAlert(this, 'obj2');"><br /><br />Object 3<br /><br /></div> <br /><br /> <div id="output"></div> </form> </body> </html>
-
Yeah, that's an odd one. An onmouseout and onmouseover are events, they are not states. You will have to build your own logic for this. I would create some global variables to track the onover/out state of the elements. But, I'm not entirely sure how the out/over events take place. I would assume that the out event triggers before the over event. So, let's say the user moves from object 1 to object 2. On the over event of object 2 you could check the saved state of object 1, but it would always be "out" because you have to leave object 1 to get to object 2. But, you are interested in knowing that object 1 was the last object that was moused over before getting to object 1. The only was to do this definitively would be to put an onmouseover event on every single object on the page. There might be a way to script that, but I'm not sure - and it seems overkill. I would just record the onmouse event of object 1 and put a timestamp on it. Then when the user mouses over object 2 check the onmouseout time for object 1. If it is less than x milliseconds trigger your custom event.
-
[SOLVED] How to randomize mysql_fetch_array($result) ?
Psycho replied to deko's topic in PHP Coding Help
I don't know if your method is more efficient or not, but this is how I think the query should be written SELECT * FROM image WHERE id IN (SELECT id FROM image ORDER BY RAND() LIMIT 25) Additional WHERE clauses should go in the subquery. Otherwise the sub query would get 25 records and the WHERE clause for the main query might exclude some of those because of additional criteria -
2400 was the way we always referred to midnight when I was in the military. Wikipedia seems to suggest that 24:00 represents the end of the day and 0:00 represents the beginning of the day. Makse sense if you are talking about work schedules. For eaxmple: 00:00 to 08:00 versus 24:00 to 08:00 or 16:00 to 24:00 versus 16:00 to 00:00 http://upload.wikimedia.org/wikipedia/en/4/44/Railtime.png[/img]
-
A more compact regex function valid_time($time) { return (preg_match("/^((([01][0-9])|([2][0-3]))[0-5][0-9]))|(24:00)$/", $time)); }
-
I was going to try and do reg ex as well, but decided I didn't want to invest the time. But I did use reg ex to validate the initial pattern. This function will validate that the input is two sets of two digits separated by a colon (and no other content). It then validates that the two groups of digits make a valid time. I made it to allow 00:00 and 24:00. Note: You can change it to allow a single digit for the hour by changing the first "{2}" to "{1,2}" function valid_time($time) { //Verify there are two sets of two numbers separated by colon if (!preg_match("/^\d{2}:\d{2}$/", $time)) { return false; } //Return true/false based upon hour/minute values list($hours, $minutes) = explode(':', $time); return ($hours<24 && $minutes<60 || ($hours==24 && $minutes==0)); }
-
strtotime() still seems to have some problems based upon the OPs original request. 1. It accepts seconds as well, in fact it found 23:59:60 as valid 2. It accepts 00:00 as valid, but not 24:00 (which is what most people I know use for midnight) 3. It accepts negaive times I think I could craft a regular expression for this, but would need very specific criteria.
-
With that approach you will need to do something different to sort the array since it is multi-dimensional. Actually I see two possible solutions: 1. If you are only going to use the filesize once (such as when displaying the content of the array), then you don't need to store that info at the time you create the array. You could simply calculate fiel size at the time you generate the content. 2. If you need the filesize data as part of the array, then use SoN9ne's solution to create a multi-dimensional array, then use the following to sort the new array: function sortFileArray($a, $b) { if ($a['name'] == $b['name']) { return 0; } return ($a['name'] < $b['name']) ? -1 : 1; } usort($narray, 'sortFileArray');
-
Yeah, I thought about that after I had posted. It is ordering the custom value as a string. No problem, we will just take a different approach. We will create a different dynamic field which will include the parent ID for sub-boards but will contain the record ID for parents. We can use that to sort first to get all the "boards" (parent and childs) grouped together. Then we will next sort by the parent ID field. Beause the parents will always have a 0, they will be at the top of each group. Lastly we will sort by the board name so the child boards are ordered in alphabetical order. Give this a try (not tested): SELECT *, IF(parent=0, id, parent) AS `board` FROM `boards` ORDER BY `board` ASC, `parent` ASC, `name` ASC
-
1. Create a design with layers such that the "slot machine" is on a top layer and the images moving behind the slot machine are not visible except through the small windows for the three slots. 2. Create individual images for each item (same sizes) 3. Create the script to rotate the images and randomly select one for each slot. Here is how I would approach the script: When the event is triggered I would first run a function to select the winning items for each slot. Then I would run a secondary function to start the image rotating process. I would write this function so that all images in each slot rotate at the same speed for a set number of seconds (3-5). Then the function would slow down the image rotations slot number 1 and ultimately stop. Then I would do the same for slot two and three in turn. The image rotation logic would need to have the order of images for each slot - they are not supposed to be in the same order for each. Then it would need to determine which images was currently being displayed (image 1) in a slot and place the next image (image 2) directly above it (which should be hidden behind another layer). Next it would move image 1 and image 2 down a set number of pixels based upon the speed you want to display. The function would be recursive (calling itself using setTimeout() so it can repeat the process). Once image 1 has past out of view, image 2 should be fully in view. The script would then remove image 1 from the page and place image 3 directly above image 2 and start the process over again. Here is a link to a tutorial on moving images in JS - not sure how good it is: http://www.c-point.com/javascript_tutorial/MMTutorial/lMoveImage.htm There are a lot of different things that would need to be done, but none of them are extremely difficult. Just break it down into specific pieces of functionality and work on one at a time. Once that piece works, move on to the next. If you tried to do it all-at-once I suspect it would be overwhelming.
-
Tested in IE only <html> <head> <script type="text/javascript"> function colorFields(fieldIdx) { fieldIdx = (!fieldIdx) ? 0 : fieldIdx; var fields = document.getElementById('theForm').elements; fields[fieldIdx].style.backgroundColor='#ff0000'; fieldIdx++; if(fieldIdx<fields.length) { setTimeout('colorFields('+fieldIdx+')', 500); } return true; } window.onload=colorFields; </script> </head> <body> <form id="theForm"> <input type="text" name="field1" /><br /> <input type="text" name="field2"/><br /> <input type="text" name="field3" /><br /> <textarea></textarea><br /> <input type="checkbox"><br /> <input type="radio"><br /> <input type="radio"><br /> </form> </body> </html>
-
Just create a dynamic field for sorting purposes. Did you have any logic in mind that you wanted to use for the ordering of the sub boards? The following will order them as you asked - sub boards will be further ordered simply by their ID SELECT * , IF(parent=0, id, CONCAT(parent, '.', id)) AS sort FROM `boards` ORDER BY sort ASC +----+-----------------+-----+--------+------+ | id | name | cat | parent | sort | +----+-----------------+-----+--------+------+ | 1 | Board 1 | 1 | 0 | 1 | | 2 | Board 2 | 1 | 0 | 2 | | 3 | Board 3 | 1 | 0 | 3 | | 6 | Board 3's Sub 1 | 0 | 3 | 3.6 | | 7 | Board 3's Sub 2 | 0 | 3 | 3.7 | | 4 | Board 4 | 2 | 0 | 4 | | 5 | Board 5 | 2 | 0 | 5 | +----+-----------------+-----+--------+------+
-
I haven't tested that, but I'm not sure it would work. Well, at least not 100%. The expression I posted looked for a single or double quote and then would look for the same character to end the paramter value. By using an or in both places it would start at a single or double quote and then would end at a single quote or double quote or space. So, this href href="http://www.mysite.com/users?name=O'Donnel" would only return "http://www.mysite.com/users?name=O", but my script will always get the entire value for the paramter delimited with single or double quotes. I don't consider myself a regex expert and I'm sure there is a bette expression though. To make the presented text into hyperlinks, just change the echo statement accordingly echo "<li><a href=\"{$match}\">{$match}</a></li>"; As for your Wordpress problems I can't really help you as I've never used it. but the googling I've done indicates that the_permalink() is used to display the link to the current post being displayed. So, I'm not sure how that applies to what you are doing.
-
If you were doing str_replace you'd do something like this: $text = str_replace(Array('[b]', '[/b]'), Array('<b>', '</b>'), $text); Now say if someone entered this text: [b]Hello world There would be no closing </b> tag, which can cause problems (be it display problems or validation issues). Not only that, there is no way you could use str_replace() to parse something like "Go here" into a valid link without regular expressions. Well, I suppose you could do it with a lot of various scripts to process the text multiple times, but it would be pointless since you can do it very easily with regular expressions.
-
Well, I was easily able to adapt it for double or single quotes, but not no quotes. Note, the only changes are the preg_match() AND the $matches array index used in the foreach() loop <?php $url = "http://www.somesite.com"; $allowedDomains = array ( 'domain1', 'domain2' ); $page = file_get_contents($url); preg_match_all("/<a.*?href=(\"|\')(.*?)\\1.*?>.*?<\/a>/is", $page, $matches); echo "<pre>"; //print_r($matches); echo "<pre>"; echo "Links at $url<br /> "; echo "<ul> "; foreach ($matches[2] as $match) { $validDomain = false; foreach ($allowedDomains as $domain) { if (strpos($match, $domain)) { $validDomain = true; break; } } if ($validDomain) { echo "<li>$match</li>"; } } echo "</ul> "; ?>
-
Here is some sample code I have laying around. You can add your own options by modifying $patters and $replacements accordingly <?php //bbcode $patterns = array( //BB Code "/\[[b]\](.*?)\[\/b\]/is", "/\[[i]\](.*?)\[\/i\]/is", "/\[[u]\](.*?)\[\/u\]/is", "/\[[s]\](.*?)\[\/s\]/is", "/\[marquee\](.*?)\[\/marquee\]/is", "/\[url\](.*?)\[\/url\]/is", "/\[url=(.*?)\](.*?)\[\/url\]/is", "/\[img\](.*?)\[\/img\]/is", "/\[quote\](.*?)\[\/quote\]/is", "/\[(size|color)=(.*?)\](.*?)\[\/\\1\]/is", "/\[br\]/i", //Emoticons "/ \:\) /", "/ \:\( /", "/ \ /", "/ \ /", "/ \:\| /", "/ \ /", "/ \:\? /", "/ \;\) /"); $replacements = array( //BB Code "<b>\\1</b>", "<i>\\1</i>", "<u>\\1</u>", "<s>\\1</s>", "<marquee>\\1</marquee>", "<a href=\"\\1\">\\1</a>", "<a href=\"\\1\" target=\"_blank\">\\2</a>", "<img border=\"0\" src=\"\\1\">", "<div><b>Quote:</b> <i>\\1</i></div>", "<font \\1=\"\\2\">\\3</font>", "<br />", //Emoticons " <img src=\"smilies/happy.gif\" border=\"0\"> ", " <img src=\"smilies/angry.gif\" border=\"0\"> ", " <img src=\"smilies/omg.gif\" border=\"0\"> ", " <img src=\"smilies/tounge.gif\" border=\"0\"> ", " <img src=\"smilies/dry.gif\" border=\"0\"> ", " <img src=\"smilies/biggrin.gif\" border=\"0\"> ", " <img src=\"smilies/confused.gif\" border=\"0\"> ", " <img src=\"smilies/wink.gif\" border=\"0\"> " ); $string = "[b]This is bold text[/b] [i]this is italic text[/i] [b][i]This is bold, italic text[/b][/i]"; $result = preg_replace($patterns,$replacements,$string); echo $result; ?> EDIT: had to remove the BBCode optins for [ code ] as it was screwing up my post.
-
Odd, the forum accidentally removed a double quote. I had actually tested the code and it was working fine. I just copy/pasted in to the forum. cags has it correct.
-
Note that this only works if they put the href content in double quotes. They could use single quotes or no quotes. I just didn't have the time to code for all three scenarios. <?php $url = "http://www.somesite.com"; $allowedDomains = array ( 'rapidshare', 'megaupload' ); $page = file_get_contents($url); preg_match_all("/<a.*?href="([^"]*).*?>.*?</a>/is", $page, $matches); echo "Links at $URL<br /> "; echo "<ul> "; foreach ($matches[1] as $match) { $validDomain = false; foreach ($allowedDomains as $domain) { if (strpos($match, $domain)) { $validDomain = true; break; } } if ($validDomain) { echo "<li>$match</li>"; } } echo "</ul> "; ?>
-
mod_rewrite is what you are looking for. http://articles.sitepoint.com/article/guide-url-rewriting Note: I can't speak to the quality of that tutorial, it was just the first one when I did a google search.