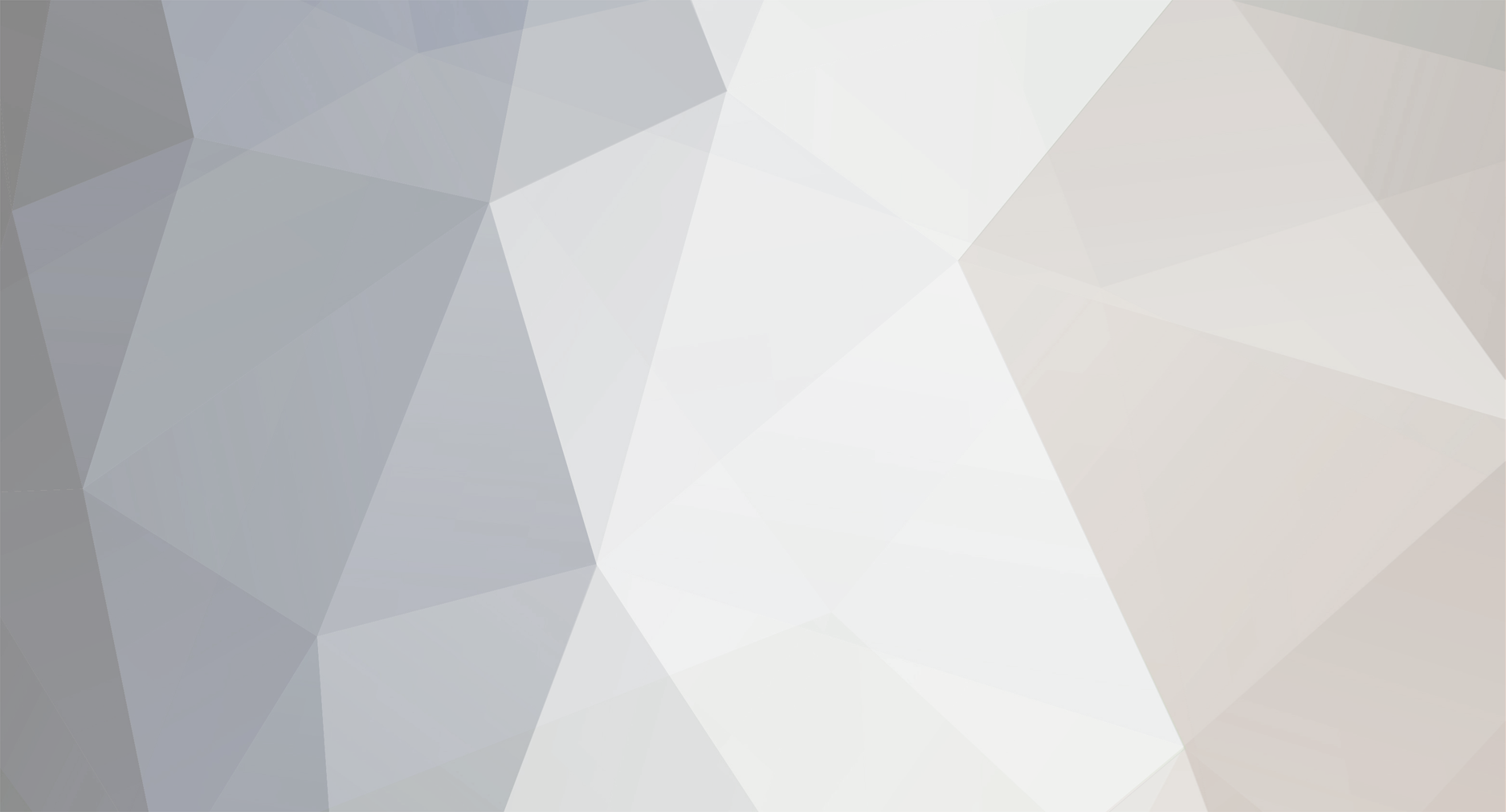
Psycho
Moderators-
Posts
12,159 -
Joined
-
Last visited
-
Days Won
130
Everything posted by Psycho
-
Hmm... you really didn't provide enough informatin as to what you are trying to accomplish. I will rephrase what I think you are trying to accomplish and ask some questions to validate: You are starting with a select list with a single empty item (value of 1, text is empty) - or does that select list have multiple values and you are only showing part of the code. You then want to change the "value" of the select list. The question is, do you want to change the value of the currently selected option or do you want to add an option with the new value and then select that value (leaving the original option(s) in place)? Try explaining what you want to happen from a user perspective.
-
Um, query the database for the values. Run them through the function to generate the new values, and put them back in the DB.
-
Rhodesa's code will work fine for a single pass, but will have problems on subsequent passes without accounting for the value not being in the middle of the range. From your description the min/max values do not change each time the number is processed. I think you need a function where you can pass those variables. Here is a function that I think does what you ask. I also included some code at the end to validate if it is what you want. The functin takes four inputs which should be self explanatory <?php function changeValue($current, $min, $max, $step) { //Determine the direction of movement if($current==$max) { $direction = -1; } else if($current==$min) { $direction = 1; } else { $direction = (rand(0,1)==1)?1:-1; } //Determine the maximum range to the min or max based on direction $rangeToEnd = ($direction==1) ? ($max-$current) : ($current-$min); //Detemine the maximum chnage which will be the minimum of $rangeToEnd or 1/2 the (max-min) $maxChange = floor(min($rangeToEnd, (($max-$min)/2))/$step); //Get a random change value $change = rand(1, $maxChange); //Apply change to current and return the value $newValue = ($current + ($change * $step * $direction)); return $newValue; } //Test the code. $value = 7000000; echo "Start Value: ".number_format($value)."<br><br>"; //Run function ten times to test the output for ($i = 0; $i<9; $i++) { $value = changeValue ($value, 4000000, 10000000, 1000000); echo "Step $i: ".number_format($value)."<br>"; } ?> The output of that would look something like this: Start Value: 7,000,000 Step 1: 5,000,000 Step 2: 6,000,000 Step 3: 7,000,000 Step 4: 5,000,000 Step 5: 4,000,000 Step 6: 6,000,000 Step 7: 9,000,000 Step 8: 7,000,000 Step 9: 8,000,000 Step 10: 6,000,000
-
Yeah, you can't put an array variable within double quotes like that. It needs to either be appended to the string using something like this $string .= "<dt>$i++ - " . $row['category_name'] . "</dt>\n"; Or you can enclose in brackets like this: $string .= "<dt>$i++ - {$row['category_name']}</dt>\n"; I prefer the latter.
-
Well, again, you need to providfe more information. If IE is reporting an error, it would be indicating the line number of the error. What is the code that is areound that line number? Assuming the IDs referenced in the getElementByID() calls are valid and are of the type that accept a value, the code above should be fine. But, why would you redefine the passed variables to the function? Just use this: function add_quote(message, id) { document.getElementById('reply_txtbox').value = '<QUOTE>' + message + '</QUOTE>'; document.getElementById('hidden_q_number').value = id; } There's really nothing more I can provide to help based upon the info given. If you want additional help I would suggest copying the code from the entire rendered page into a flat html file and attaching to this thread. That way I, or someone else, can replicate the error.
-
Looking at the first code block in your last post, I see nothing wrong. where is the function being called? You're making this a lot more difficult for us to help you. With a Javascript error we need to see the rendered page code, NOT the PHP code. The problem could be somethign as simple as the code generated through PHP includes a quote mark that is fouling up the page. Can you provide a link to the page that is giving you the problem?
-
Also, you have some bad code in your pages. Whn I clicked one of the image links, it opened a new browser window. That window had quite a bit of "lag". I thin notices that IE was taking up 20% of my CPU cycles while that window is sitting idle. The browser should be taking up 0% if the user is not loading a page with the rare exception where you have a script running some type of timer loop.
-
YOu don't need to send out a PM everytime you post. Most users will check the "Show new replies to your posts." link at the top of the page to see where there have been responses that they may need to reply to. As to your question of "is there any where to override the hiding of the div?" I'm not sure I follow. I already showed you how you could have one div set to be displayed. Are you wanting the ability to show all the divs? If so, I would just modify the previous function and allow all the div to be displayed by passing a 'true' parameter: function showDiv(selectedIndex) { var divIndex = 0; while (document.getElementById('game_'+divIndex)) { var displayStyle = (divIndex==selectedIndex || selectedIndex===true)?'inline':'none'; document.getElementById('game_'+divIndex).style.display = displayStyle; divIndex++; } } This would hide all the divs showDiv(false) This would show all the divs showDiv(true) This would hide all the divs except the one with the id 'game_3' showDiv(3)
-
Yes, I meant to write "style". You don't need another function. If you called the function like this showDiv(false) it will hide ALL the divs. However, if you call the function like this showDiv(2) it will hide ALL the divs except the div "game_2".
-
You can't give multiple elements on a page the same ID. You can give them similar names that can be processed programatically. Just create all your divs with a common name, but with a unique number. Such as: "game_1", "game_2", "game_3", etc. Then you can create a simple loop to hide all the divs (and alternatively unhide one specific div): Note: to hide ALL divs, pass 'false' to the function. Or pass an index number and all divs will be hidden except the specified div function showDiv(selectedIndex) { var divIndex = 0; while (document.getElementById('game_'+divIndex)) { var displayStyle = (divIndex==selectedIndex)?'inline':'none'; document.getElementById('game_'+divIndex).styke.display = displayStyle; divIndex++; } }
-
[SOLVED] Displaying table content skips first row
Psycho replied to rubbertoad's topic in PHP Coding Help
Then you have a line of code somewhere that is using up that first record. Show the code that starts from the actual query to where you actually start displaying the records. I revised your code above slightly and there is no reasons it shouldn't work. This worked fine for me if (mysql_num_rows($rsTable)) { //Open the table echo "<table border=\"1\">\n"; //Print the table headers echo "<tr>"; for($i=0; $i< mysql_num_fields($rsTable); $i++) { echo "<td>".mysql_field_name($rsTable, $i)."</td>"; } echo "<tr>\n"; //Print the records while($record = mysql_fetch_assoc($rsTable)) { //Print table contents echo "<tr>"; foreach($record as $value) { echo "<td>$value</td>"; } echo "</tr>\n"; } //Close the table echo "</table>\n"; } -
[SOLVED] Displaying table content skips first row
Psycho replied to rubbertoad's topic in PHP Coding Help
It could be that he is using this code to display the results of various database queries - such as for debugging purposes. If not, then I agree, hard code the column names. You could do somthing like this: if (mysql_num_rows($query)) { echo "<table border=\"1\">\n"; $first_record = true; while($record = mysql_fetch_assoc($query)) { //Print table headers if first record if ($first_record) { $first_record = false; echo "<tr>"; foreach($record as $label => $value) { echo "<td>$label</td>"; } echo "</tr>\n"; } //Print table contents echo "<tr>"; foreach($record as $value) { echo "<td>$value</td>"; } echo "</tr\n>"; } } -
1. Don't use short tags (i.e. <? ), use te full php tag ( <?php ). 2. Is this a javascript error or a PHP error? I'm assuming a JS error absed upon the forum you posted in. In that case you sheed to show the processed output. In otherwords, what is the result after being processed by PHP? And, as a side note, why are you echoing a value in PHP and enclosign in quotes? PHP will first interpret the content in the quotes as a string and then have to revert any variables to their value. Just use echo $variable
-
[SOLVED] Problem with script submitting/radio buttons
Psycho replied to fudmonkey's topic in Javascript Help
You can't put double quotes within a strng delimited with double quotes unless you escape them. This is the problem line: echo"<br><input type=radio name=note value=4 onclick="this.form.submit()">GREAT"; When the line is parsed it thinks that the double quote at the beginning of the onclick is ending the string you want to echo. Change that line to thie echo"<br><input type=radio name=note value=4 onclick=\"this.form.submit()\">GREAT"; -
<html> <head> <script type="text/javascript"> <!-- This script and many more are available free online at --> <!-- The JavaScript Source!! http://javascript.internet.com --> <!-- Begin var timerID = null; var timerRunning = false; function stopclock () { if(timerRunning) clearTimeout(timerID); timerRunning = false; } function showtime () { var now = new Date(); var hours = (now.getHours()%12==0) ? '12' : (now.getHours()%12); var minutes = padZero(now.getMinutes()); var seconds = padZero(now.getSeconds()); var ampm = (hours >= 12) ? " P.M." : " A.M." var timeValue = hours+':'+minutes+':'+seconds+' '+ampm;; document.getElementById('clock').innerHTML = timeValue; document.getElementById('message').innerHTML = (hours==5) ? 'haha' : ''; timerID = setTimeout("showtime()", 1000); timerRunning = true; } function padZero(value) { value += '0' + value; return value.substr(value.length-2); } function startclock() { stopclock(); showtime(); } // End --> </script> <body onLoad="startclock()"> <center> <div id="clock"></div> <div id="message"></div> </center> </body> </html>
-
AJAX to replace the use of header("Location: ")
Psycho replied to jBooker's topic in Javascript Help
Yes it is possible, but - in my opinion (and many others) - using AJAX (i.e. JavaScript) as the only means of doing this would be bad practice. You would be preventing the use of your site to anyone without JS enabled. Plus, you could run in to incompatability issues between how different browsers parse that JS code. That being said, I would say that implementing an AJAX solution would be a good "additional" feature. In other words, the page should work without JS enabled through normal means of a form POST but, if JS is enabled, then utilize AJAX. There are literally hundreds of tutorial on the net about AJAX. Trying to teach someone in a forum post would not make sense. -
You can't use one set of JavaScript tags to include an external file AND to include in-line code. <!-- LOAD THE EXTERNAL TWEEN CLASS --> <script language="text/javascript" src="Tween.js"></script> <!-- CREATE IN-LINE JAVASCRIPT CODE --> <script language="text/javascript"> function loadSwish() { t1 = new Tween(document.getElementById('titleMenu').style,'top',Tween.elasticEaseOut,-100,27,2,'px'); t1.start(); } </script>
-
The problem is that a radio group of multiple options is treated as an array, whereas a group with just one option is not an array. Therefore the for() loop fails due to the fact that buttons.length has no value. The solution is to check if buttons.length is a valid parameter. Try this function checkTest(form) { var checked = false; var buttons = form.elements.TestRadioOption; if (buttons.length) { //Array: iterate through each option for (var i=0; i<buttons.length; i++) { if (buttons[i].checked) { return true; } } } else { //Not an array Array: check the single value checked = buttons.checked; } if(checked == false) { alert("Please Select a Radio BUtton"); } return checked; }
-
[SOLVED] Problem with script submitting/radio buttons
Psycho replied to fudmonkey's topic in Javascript Help
Works for me. Did you check if any errors are being thrown? By the way, use some line breaks in your HTML code. It's a pain to try and rework the HTML into a readable format when trying to help someone. -
Um, not trying to ruin your "issue" for the rest of the readers, but what you are asking is not far-fetched. It has already been done: http://www.free-press-release.com/news/200901/1232966171.html
-
Well, I've tested it and it works for me. I inserted a file into the database using a mediumblob type. Then I had two pages: one for the HTML page that would display the content (including the image tag) and a second page that would be used as the SRC for the image tag. content page <html> <body> Image:<br /> <img src="userimage.php"> </body> </html> Image Page (i.e. userimage.php) <?php // Connect to DB $dbh = mysql_connect('localhost', 'root', ''); mysql_select_db('testDB'); $query = "SELECT `image` FROM `userimage`"; $result = mysql_query($query) or die(mysql_error()); if(mysql_num_rows($result) == 1) { $record = mysql_fetch_assoc($query); $img_content = $record['image']; } else { $img_content = readfile('profile_blank_gray.jpg'); } header('Content-type: image/jpeg'); echo $img_content; ?> The problem may be in how you are saving/storing the image in the database. Take a look at this tutorial: http://www.php-mysql-tutorial.com/wikis/mysql-tutorials/uploading-files-to-mysql-database.aspx Then why do it. What is your reasoning for wanting to make something simple into something complex?
-
I think you are confusing the difference of including an image on a page (i.e. using the IMG tags) versus handing the image to the browser as an image. The code you have will either "serve" the image to the browser as a file or try to insert an image tag intothe HTML page - two entirely different things. In the page that you want to "display" the image, do somethign like this: <p>Here is some text that comes before the image</p> <br /> <img src="getuserimage.php"> <br /> <p>Some text that comes after the image</p> Then create the page "getuserimage.php" so it will serve the image to the browser. <?php include ("blogconnect.php"); $sql = "SELECT * FROM userimage"; $query = mysql_query($sql, $connect) or die(mysql_error()); if(mysql_num_rows($query)== 1) { $row = mysql_fetch_assoc($query); $img_content = $row['image']; } else { $img_content = readfile("../images/profile_blank_gray.jpg"); } header('Content-type: image/jpeg'); echo $img_content; ?> However, I see absolutely no reason to use a database to store only ONE image!? If you are trying to "protect" the image from hot-linking there are much better ways of doing that. And, the method you have does not protect the image from being copied if that is what you are after.
-
Regarding your calendar error, that is a different problem and should have been created as a different post in the forum. However, did you check the actual HTML output of the script to see if the code is being generated correctly with the quotes? I ran a test with just the bit of code you tried to highlight in red and received the following output which "looks" correct to me - but I don't know the correct parameters for your function, so it is only a guess <input type="text" id="hours2" name="hours" size="10" )></input> <a href="javascript:NewCssCal('hours2','ddmmyyyy')"> <img src="sample/images/cal.gif" width="16" height="16" alt="Pick a date"></a> So, assuming the output looks correct, what DOES happen when you click the link? Do you get an error message? If there is a JS error, there should be a yellow triangle in the bottom left of the window in IE (just double click it to see the error).
-
It's late and I'm not up to reviewing the code, but I will answer this question You are correct which is why the code I provided will present the suer a list of Companies by name BUT the values of those items will be the company IDs!
-
Well, your form has a generally correct format for creating the list, but I don't see where you actually run the query. Plus, you are not populating the value into the text of the option. Also, if the records int he company table have IDs, you should be using that as the value of the option. lastly, you would want to include that value in the processing page to include a value for the companyID into the new record being created. This is just an example for the form page <select name="Select Company Name"> <option value="">--Select Company Name--</option> <?php $query = "SELECT id, company_name FROM company_table"; $result = mysql_query($query) or die (mysql_error()); while ($record = mysql_fetch_assoc(result)) { echo "<option value=\"{$record['id']}\">{$record['company_name']}</option>\n"; } ?> </select>