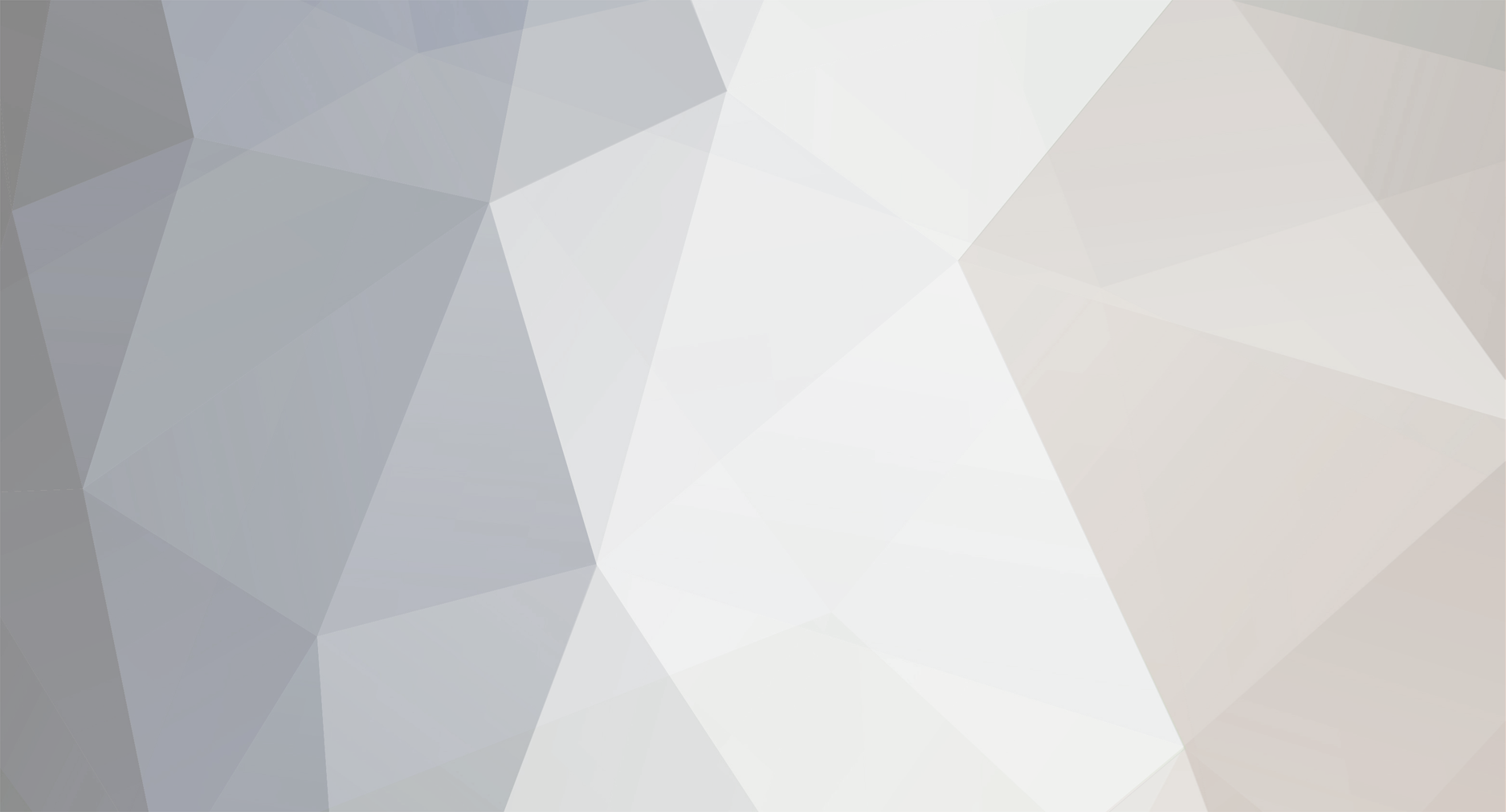
Psycho
Moderators-
Posts
12,159 -
Joined
-
Last visited
-
Days Won
130
Everything posted by Psycho
-
You should never use the same name for anything even if they are structurally different objects (e.g. a function and a field name). It will only cause confusion even if it does work. In any event, the second block of code should work - at least it did for me when I tested it with a field and function named the same (both IE & FF).
-
My "example" was just that - an example. The logic still holds true no matter what method you are using to generate the output. I still contend that the most appropriate method of solving this issue is with modifying the code that generates the output not in manipulating the data. If you were to provide the code used to generate the output then we could take a look at modifying that. But, if the code is a completely self-contained 3rd party function it may not be worthwhile to do so and modifying the data may be the most "efficient" solution - while not the optimal solution.
-
In my opinion you are doing this backwards. Your array should just contain your data and the process of outputting the array to the page should take care of the rest. You should not be adding empty array elements to create a blank row, Example: <?php //Create array of JUST the data $data = array ( array ("SL","121","9"), array ("SL","121","9"), array ("SL","121","10"), array ("SL","121","11"), array ("SL","121","16") ); function displayArrayData($arrayValues) { //Start the table echo "<table border=\"1\">\n"; echo " <tr>\n"; echo " <th>Column 1</th>\n"; echo " <th>Column 2</th>\n"; echo " <th>Column 3</th>\n"; echo " </tr>\n"; $lastRowID = ''; //Iterrate through each record foreach ($arrayValues as $dataRow) { //Show blank row if this record is different ID than last if($dataRow[2]!=$lastRowID && $lastRowID != '') { echo "<tr><td colspan=\"3\"> </td></tr>\n"; } //Set last ID value $lastRowID = $dataRow[2]; //Display current record echo " <tr>\n"; echo " <td>{$dataRow[0]}</td>\n"; echo " <td>{$dataRow[1]}</td>\n"; echo " <td>{$dataRow[2]}</td>\n"; echo " </tr>\n"; } //Close the table echo "</table>\n"; } displayArrayData($data); ?> The data array only contains the data and the logic parses the data and outputs it as needed. The output would look like this: SL 121 9 SL 121 9 SL 121 10 SL 121 11 SL 121 16
-
Upload Security- help with protecting against executable files
Psycho replied to clkwebdesign's topic in PHP Coding Help
I always advocate using "White Lists" (list of acceptable values) instead of using "Black Lists" (list of unacceptable values). The problem with a black list (disallowing certain file extensions) is that it you can't be 100% certain there are not other items that could be a problem. If you were to only allow .txt files to be uploaded it probably wouldn't be an issue. But, if you are only excluding some file types are you sure there are not others that can be executed by the web server (e.g. .php4)? Just set a list of safe file types and only allow those to be uploaded. Secondly, wherever these files are uploaded you should restrict direct access to those files by the user. If the user needs access to the files you should identify the file by ID and only allow the user to download the file using a download script. -
You "supposed" to be able to set the printed page size using the CSS properties for paged media. But, the tests I ran in IE and FF seem to indicate they don't support it. The browser always goes with the default page size (which in my case is 8.5" x 11" - not A4). So, I'm not sure where it gets the default from.
-
Well, if you want to take accessability in mind as well as compatability for user without JS enabled I would start go with a max number of input boxes and then show/hide the appropriate number. Then if the user does not have JS enabled they will just get the max allowable. If you can't go with a max value then you will have to create the fields dynamically which will prevent anyone without JS enabled from using your page. Plus, you will have to think about what to do if the user wants to reduce the number of fields (if anything). Here's a script I alredy have which starts with four fields and then as you get to the end will continue to add fields one at a time automatically. I like this better than adding a predetermined set of fields specified by the user as it only adds fields as they need them. But, this could be easily modified to do exactly as you asked in your post <html> <head> <script> function addTiming(field) { thisFieldIdx = field.id.substr(6); newFieldIdx = thisFieldIdx*1 + 1; if (!document.getElementById('timing'+newFieldIdx)) { timingDiv = document.getElementById('timings'); timingDiv.innerHTML = timingDiv.innerHTML + 'Timing '+newFieldIdx+': <input type="text" name="timing'+newFieldIdx+'" id="timing'+newFieldIdx+'" onfocus="addTiming(this);"><br>'; } document.getElementById('timing'+thisFieldIdx).select(); document.getElementById('timing'+thisFieldIdx).focus(); return; } </script> </head> <body> <form name="formname"> <div id="timings"> Timing 1: <input type="text" name="timing1" id="timing1"><br> Timing 2: <input type="text" name="timing2" id="timing2"><br> Timing 3: <input type="text" name="timing3" id="timing3"><br> Timing 4: <input type="text" name="timing4" id="timing4" onfocus="addTiming(this);"><br> </form> </div> </body> </html>
-
kickstart beat me to it, but I created a function (plus his has a typo because of the [ code ] tag), so I'll post mine anyway: <?php function getHexRange($start, $end) { $startDec = hexdec($start); $endDec = hexdec($end); $hexArray = array(); for($dec=$startDec; $dec<=$endDec; $dec++) { $hexArray[] = dechex($dec); } return $hexArray; } $range = getHexRange('9CF90000', '9CF910CB'); print_r($range); ?>
-
Assuming your permission check is secure & comprehensive then, yes, I would say this is overkill. You wouldn't be displaying the links to unauthorized users and you wouldn't process the links from unauthorized users. So, I'm not sure what you are trying to prevent with the encrypted parameters on the URL
-
You wouldn't. But, in my opinion, you are approaching this the wrong way. Instead of encrypting the parameters of the link to prevent unauthorized users from performing an action (e.g. delete a record) you should just leave the links in plain text (i.e. itemid=1) and then do a permission check on the processing page to ensure the user trying to perform the action has the appropriate rights to do so. That will also make debugging MUCH easier. While the current approach would be very difficult for someone to break, it IS possible. Checking the user's permissions would be definitive.
-
OK, so the first button really isn't a submit button at all! In this situation you don't want to use two "submit" buttons anyway. If you had simply stated your objective in the beginnign we wouldn't have wasted this time. If you want to do JavaScript validation you simply need to create an onsubmit event that will perform the validation and send the form if validation passes and if validation fails halt the fiorm submission and display the errors. You just need to create a javascript function to perform the valiaftion and return true if there are no errors and return false if there are errors. Then in the FORM tab put an onsubmit trigger like this <form name="theform" onsubmit="return validate();">
-
Once you click submit the page is submitted and will reload. You could have the buttin show as soon as you click submit, but it will only display for a fraction of a second before the page reloads. I think you need to clearly explain what you are trying to accomplish.
-
Do you really need to show/hide a second submit button? Your example code would seem to indicate you only need to change the value of the current submit button. Anyway the code below will show/hide the second submit button: <html> <head> <script type="text/javascript"> function checkFields() { if (document.getElementById('field1').value!='' && document.getElementById('field2').value!='' && document.getElementById('field3').value!='') { document.getElementById('submit2').style.visibility='visible'; } else { document.getElementById('submit2').style.visibility='hidden'; } return; } </script> </head> <body> Field 1: <input type="text" name="field1" id="field1" onkeyup="checkFields();" /><br /> Field 2: <input type="text" name="field2" id="field2" onkeyup="checkFields();" /><br /> Field 3: <input type="text" name="field3" id="field3" onkeyup="checkFields();" /><br /> <input type="submit" name="submit" id="Submit" value="submit" /> <input type="submit" name="submit2" id="Submit" value="1" style="visibility:hidden;" /> </body> </html>
-
Ken2k7's option is probably the most efficient, but just for the sake of learning here's another approach <?php $query = "SELECT * FROM category WHERE cat_name = '$curr_cat'"; $result = mysql_query($query) or die(mysql_error()); while($record = mysql_fetch_assoc($result)) { $catNames = $record['area']; } echo implode(', ', $catNames); ?>
-
Why do you need to set the value between the quote marks? You could only do that during the page load with a write() command. Makes much more sense to just set the value of the object as your function is doing above. Is that function not working? In any event it is inefficient. function validateIt() { var quan= document.getElementById('fname'); var amount = document.getElementByName('amount_1'); var howMany = document.getElementByName('quantity_1'); if(quan.value>=0 && quan.value<=1000) { amount.value="0.19"; howMany.value=quan.value; } else if(quan.value>1000 && quan.value<=5000) { amount.value="0.18"; howMany.value=quan.value; } else if(quan.value>5000 && quan.value<=10000) { amount.value="0.17"; howMany.value=quan.value; } }
-
Or just do one query $query = "SELECT MIN(id) as min, MAX(id) as max FROM table"; $result = mysql_query($query); $record = mysql_fetch_assoc($result); $random_number = rand($record['min'], $record['max']); Note, as of v4.2.0 srand() is not necessary
-
And, the reason your code didn't work is that you are using the name "numCounter" for the isset() test, but everywhere else you are using "numCount"
-
This will give you the page that was called by the browser - i.e. not the current page if it was included - $_SERVER['REQUEST_URI']
-
Use __FILE__ http://www.php.net/manual/en/language.constants.predefined.php Edit: Whoops, that will give you the same thing. Use this. $_SERVER['REQUEST_URI']
-
Except for the first few header lines it looks to be in a basic XML format. Just use an XML parser. Many tutorials out there.
-
[SOLVED] adding text before/after all images in the page
Psycho replied to SchweppesAle's topic in Javascript Help
Can you at least require that the images be enclosed in SPAN tags (without any parameters)? If so, this script will add the hyperlinks needed: <html> <head> <script type="text/javascript"> function linkImages(image) { var minImageWidth = 450; var imageList = document.images; var imageCount = imageList.length; for (var idx=0; idx<imageCount; idx++) { if (imageList[idx].width > minImageWidth) { var parentSpan = imageList[idx].parentNode; var imageHTML = parentSpan.innerHTML; var imageSrc = imageList[idx].src; var newHTML = '<a href="'+imageSrc+'" rel="lightbox">'+imageHTML+'</a>'; parentSpan.innerHTML = newHTML; } } return; } window.onload = linkImages; </script> </head> <body> <span><img SRC="small.jpg" /></span><br><br> <span><img SRC="lighthouse.jpg" /></span><br><br> <span><img SRC="rock_island.jpg" /></span> </body> </html> -
[SOLVED] adding text before/after all images in the page
Psycho replied to SchweppesAle's topic in Javascript Help
Well, you could try to add a rel="lightbox" to the images as well. if (imageList[idx].width > minImageWidth) { imageList[idx].rel = 'lightbox'; imageList[idx].onclick = openImage; } Or, if that doesn't work, then put an anchor tag around every image before the Javascript runs, but make the href value "#". The image and anchor tags should have similar IDs. Then change the href to the img src using the JS. HTML when page loads <a href="#" rel="lightbox" id="href_1"><img src="http://www.mydomain.com/image.jpg" id= id="img_1"></a> Then in the script above replace the loop with this (not tested) if (imageList[idx].width > minImageWidth) { idNum = imageList[idx].id.substr(4); document.getElementById('href_'+idNum).href=imageList[idx].src } -
[SOLVED] adding text before/after all images in the page
Psycho replied to SchweppesAle's topic in Javascript Help
No need to "add" anchor tags - just dynamically give the images an onclick event. This should do what you want (tested in IE7 & FF3): <script type="text/javascript"> function linkImages() { var minImageWidth = 450; var imageList = document.images; var imageCount = imageList.length; for (var idx=0; idx<imageCount; idx++) { if (imageList[idx].width > minImageWidth) { imageList[idx].onclick = openImage; } } return; } function openImage() { window.location = this.src; } window.onload = linkImages; </script> -
Here is a sample script that will display the server time and clinet time on the page. <html> <head> <script type="text/javascript"> var serverTime = new Date(<?php echo (time() * 1000); ?>); var clientTime = new Date(); var serverOffset = serverTime - clientTime; function showTime() { // alert(serverOffset); var client = new Date(); var server = new Date(client - serverOffset); document.getElementById('client').innerHTML = client; document.getElementById('server').innerHTML = server; setTimeout('showTime()', 1000); } </script> </head> <body onload="showTime();"> Client Time: <span id="client"></span><br> Server Time: <span id="server"></span> </body> </html>
-
And, the URL I posted to the other thread shows you how to do that. There are two problems with what you have done. 1. You are only outputting a number (the hour) from the server and you are trying to use it as a date object. 2. The rest of your javascript has other problems based upon the limited testing I did. If you can provide a complete working page where you "hard code" a server-time, I can provide a solution. But, I can't determine what you are trying to do in your code, so I can't provide a solution.
-
Yes and no. There's no reason you cannot dynamically create JavaScript using PHP just like you can dynamically create HTML with PHP. However, you are right in that <?php date('G');?>; is bugs. Well, it's not technically bogus, but it won't do anything! You need to put an echo in there to have it actually written to the page. <?php echo date('G');?>; I have not analyzed the rest of the code. But, you shouldn't need to write that same variable three times to the page.