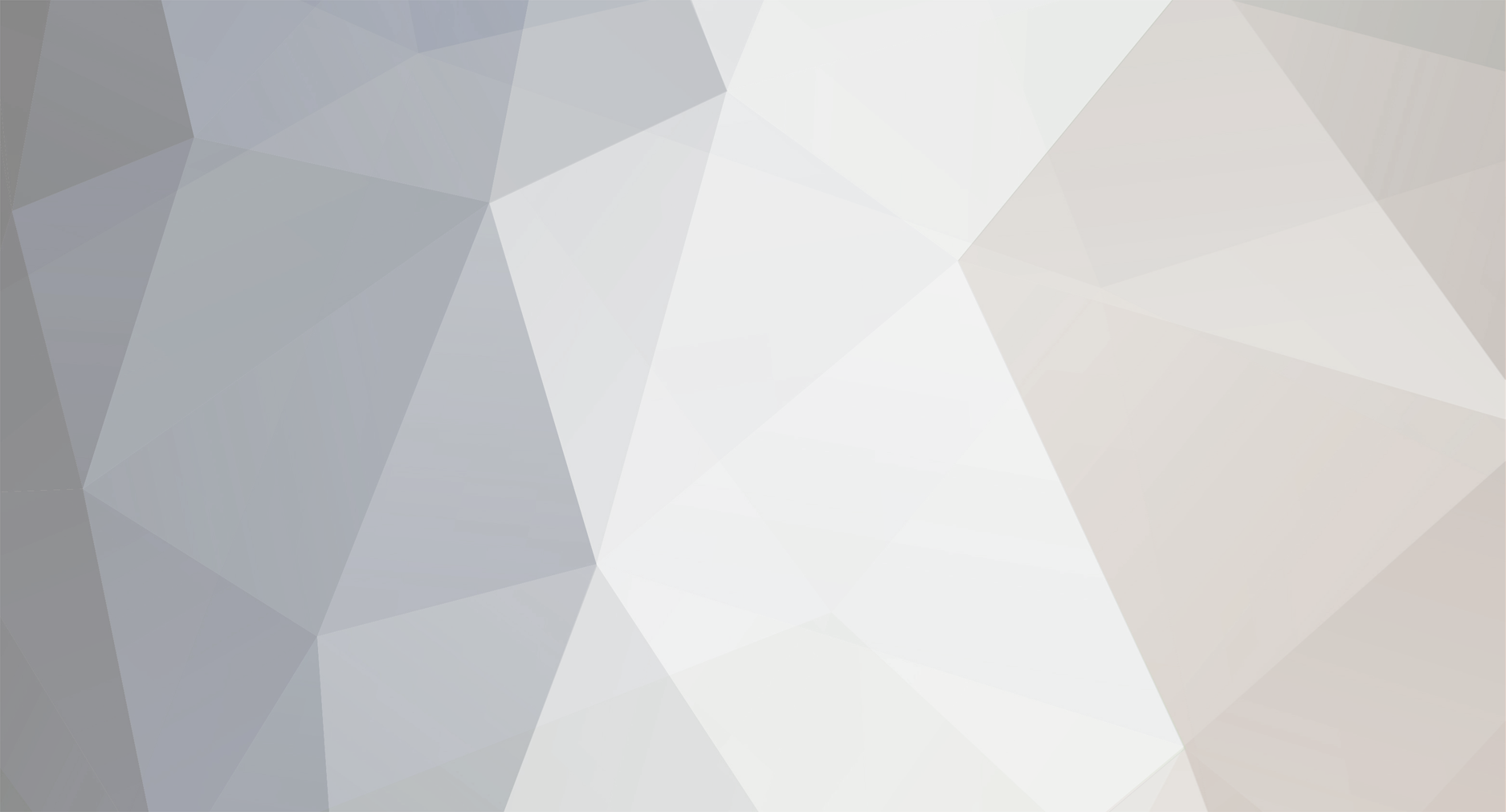
Psycho
Moderators-
Posts
12,159 -
Joined
-
Last visited
-
Days Won
130
Everything posted by Psycho
-
if you mean combined them into the members table, I can't really think of a way to logically do that. I asked in case I may be misunderstanding something about what you are trying to achieve. Now one thing I am not sure of is do you track movies that members have watched IF they have not rated them? If so, then you don't need to track if they have watched the movie as you can simply deduce that by the movies they have rated. If you are tracking those separately you *could* possibly combine the watched and rating value for each member in a single table (movieID | watched | rating).
-
No, I'm saying you want four tables: Members: ID--username--password movies: ID--title--rating watched: movieID--memberID member_rating movieID--memberID--member_rating In your current schema, how were you tracking multiple movies that a member had watched or multuple movies a member had rated? Anyway, with the above schema you could get a list of every movie a member had watched and/or rated with a query such as this SELECT * FROM movies LEFT JOIN watched ON watched.movieID = movies.ID LEFT JOIN rating ON rating.movieID = movies.ID JOIN members ON members.id = watched.memberID OR members.id = rating.memberID WHERE members.name = '$memberName'
-
Or, you can do it with a regular expression function removeZeroRecords($string) { $string = ';'.$string; $string = preg_replace('/;0,[^;]*/', '', $string); return substr($string, 1); } $string = "324,3;65,6;0,17;93,10;"; echo removeZeroRecords($string); //Output: 324,3;65,6;93,10;
-
function removeZeroRecords($string) { //Separate the string into an array on semicolon $records = explode(';', $string); //Iterate through each record in the array foreach ($records as $index => $record) { //Separate record into the first/second numbers based on comma $parts = explode(',', $record); if ($parts[0]=='0') { //Remove records that have a 0 as starting number unset($records[$index]); } } //Combine the remaining records into a strin and return it return implode(';', $records); } $string = "324,3;65,6;0,17;93,10;"; echo removeZeroRecords($string); //Output: 324,3;65,6;93,10;
-
[SOLVED] prevent the user from copying a specific paragraph
Psycho replied to yami007's topic in Javascript Help
@darkfreaks: I think the OP is echoing a random number to the screen and then expecting the user to enter that random number into the field as a verification that the user is a human and not a spambot. So, he is not trying to prevent the user from seeing source code, just want to enforce the user to type the random number in rather than copy and paste. By the way, who is "Maj" ??? @yami007 : You could probably do something where an onkeydown has to be registered on the field, but I don't beleive spambots would even have JS enabled. I'd suggest making the field disabled by default and then enabling it onclick(). Of course anyone without JS enabled will be out of luck. -
I am *assuming* the members table is a table of members. If that is the case, then ratings and "watched" movies should be in separate tables (along with another table for the movie records).
-
This page describes the problem in better detail, but the solutioin is the same as I provided. http://blog.unidev.com/index.php/2008/10/10/math-error-in-javascript/
-
I forget why this happens, but just add a toFixed() to the result to get the correct value. Updated function: function Calculate() { with(document.forms['myform'].elements) { var subTotal = parseFloat(Qty1.value) * parseFloat(Price1.value); Cost1.value = subTotal; subtotal.value = subTotal; var totalValue = parseFloat(subtotal.value) + parseFloat(shipping.value) + parseFloat(tax1.value) + parseFloat(tax2.value) + parseFloat(tax3.value); total.value = totalValue.toFixed(2); } return; }
-
[SOLVED] prevent the user from copying a specific paragraph
Psycho replied to yami007's topic in Javascript Help
Not sure what you are asking (either you don't want them to copy a paragraph displayed on the page or you have some verification code that you don't want copied). But, the solution is the same, which is there is nothing you can do. Yes, there are "right-click" hacks that attempt to prevent a user from usign features such as "view-source", but they do not work in that they are easily circumvented. If it is text displayed on the page you are trying to "protect" the best option, IMHO, is to make the text an image and use a watermark. It doesn't prevent anyone from using that image, but they won't be able to do so without people seeing your watermark and knowing it wasn't theirs. But, since it's text they could simply rekey that text into a text editor. And, again, if we are talking about the code, there is really nothign you can do except obfuscate the code, which only makes it more difficult for someone to assemble into a readable format. Besides, have you not benefited from other's code? Why are you being stingy? -
Yes, I am suggesting you use one table for ALL of the workout programs. Having mutiple tables doesn't make sense. Part of the power of a database is the ability to extract ONLY the data that you want. In this case you could query the one table with all the workout programs and filter it by goal, level, etc. In fact, I would imagine that you have some of the same workouts for different goals, levels, etc. In that case to "normalize" the database you would have one table of each unique workout. Then in separate tables you would associate each workout to goals, levels, days. as appropriate. For example, the bench press exercise could be associated with weight loss & strength trainign goals, beginner, intermediate & advanced levels, and 3 & 5 day workouts. The table structure would look like this exercises id | name | sets | reps | minutes 1 | bench press | 10 | 10 | 20 goals exercise_id | goal 1 | weight loss 1 | strength training (note: you could have a separate table to translate the goal_ids into their names or handle in the PHP code) levels exercise_id | level 1 | beginner 1 | intermediate 1 | advanced days exercise_id | days 1 | 3 1 | 5 Of course this may mean a lot of rework. So, decide if its worth it to you to take this approach. Also, if you have a field named sets, do not put "3 sets" as the value. Just use 3.
-
[SOLVED] Concatenation of IF and FOR EACH statement...
Psycho replied to Thetcm's topic in PHP Coding Help
To be honest, I still don't completely understand what exactly you are doing. My suggestion above was only that - it can be modified to fit your particular needs (if it makes sense). For example, you don't have to use Div1, Div2, etc. You could have descriptive names for them if need be. But, building the entire page should be straitforward as well. I guess I'm just not following "why" you need to create the text in a single line of code. Could you not do somthing like this: $responseText = '<td>'; $relatedRecords = $record->getRelatedSet("relatedSetName"); if (FileMaker::isError($relatedRecords) === false) { $master_record = $record; foreach ($relatedRecords as $record) { $responseText .= nl2br($record->getField('fieldName::Language', 0)); } $record = $master_record; } $responseText .= '<td>'; //Perform additional operations as needed and add text to the //$responseText variable to be returned through the AJAX call //Output the entire response text for AJAX response echo $responseText -
[SOLVED] Concatenation of IF and FOR EACH statement...
Psycho replied to Thetcm's topic in PHP Coding Help
Yes, but... You can send back multiple responses as one response! I've done something similar in the past. So, let's say you have 10 divs on the page: div1, div2, etc. Then let's say there is a button on the page that will make an AJAX call and the response from that AJAX call needs to update one or more of the divs but the JS code won't know ahead of time how many or which ones - it will be determined when teh AJAX call is received by the server-side code and is processed. Here is one solution: When the call is made to the server-side page and logic is run to determine what needs to be returned to the JavaScript, the server-side code will determine which divs need to be updated and return a single sting that the javascript will parse and populate the appropriate divs. Here's an example: The PHP code will determine which Divs need to be updated and will return the entire set of data in this format: div1:div 1 content|div3:This content goes in div 3|div8:And of course,this goes in div8 The JavaScript will then parse the return value and populate the divs accordingly such as this // returnVal is the value returned fromt eh AJAX call //Split the return value into an array of name/value pairs for each div var divs = returnVal.split('|'); //Iterate through each div name/value pair for(var i=0; i<divs.length; i++) { //Split the div name/value pair into it's parts divParts = divs[i].split(':'); //Poulate the div with it's content document.getElementById(divParts[0]).innerHTML = divParts[1]; } This was just an example. Youwould need to make sure that whatever characters you use to deliniate the data would not be included in your data. You could always use quotes around the text, control characters, etc. Hope this helps. -
[SOLVED] Concatenation of IF and FOR EACH statement...
Psycho replied to Thetcm's topic in PHP Coding Help
I've reread your post a few times and I'm wondering "why" you need to take this approach? I always appreciate compact code, but not at the expense of readability and useability. If this is server-side code to generate the response for an AJAX call you don't have to echo all of the text in one line. However, if you need to run nl2br() on more than one array, you may want to create a function to do that and return the array as a single string using implode function arrayToString($array) { foreach ($array as $key => $value) { $array[$key] = nl2br(); } return implode('', $array); } -
[SOLVED] Concatenation of IF and FOR EACH statement...
Psycho replied to Thetcm's topic in PHP Coding Help
I *think* I understand your problem. Try using the ternary operator (If/Else shorthand) $var = 'foo'; $string='Start'.(($var=='foo')?'-TRUE-TEXT-':'-FALSE-TEXT-').'End'; echo $string; //Output: Start-TRUE-TEXT-End $var = 'bar'; $string='Start'.(($var=='foo')?'-TRUE-TEXT-':'-FALSE-TEXT-').'End'; echo $string; //Output: Start-FALSE-TEXT-End -
Having multiple files should not make a perceptible difference. I would guess there would be some additional time involved due to the renegotiation to find the file and start the transfer, but I think we're talking microseconds. If you really want to know the impact - do a test. Assign getTime() to a variable before you load the external files and then assign getTime() to another variable after you load them - then check the difference. Then for comparison, put all the JS into one external file and run the test again.
-
Why not just send out a new temp password instead of using an unsecure method of storing the data. It's just good practice. Any site can get infiltrated. That woudl include the database and the PHP files that are used to encrypt/decrypt data in your database. Because many users use the same usernames, passwords, etc. on several sites if someone can get the info from one site they can try that info on other sites such as banking. It's just good practice, IMHO, to use an undecryptable method using a good salt.
-
[SOLVED] kinda complicated output results
Psycho replied to willrockformilk's topic in Javascript Help
Please mark the topic as solved. And, I was not implying that you did expect me to write the code for you. I was just commenting on the fact that you, unlike many posters, took responsibility for reviewing the code that was providied and providing constructive feedback on what didn't work right. For that I thank you. Far too often I see replies from people simply stating that the code doesn't work without providing an error message, let alone actually doing the simplest of debugging. -
Hiding where your downloading the file from
Psycho replied to _OwNeD.YoU_'s topic in PHP Coding Help
Note iarp's script will provide a dialog to Open/Save/Cancel the file instead of attempting to directly open the file (as requested by the OP). That script can be used to attempt to open the file automatically by commenting out this line: header('Content-Disposition: attachment; filename="'.$name.'"') -
Hiding where your downloading the file from
Psycho replied to _OwNeD.YoU_'s topic in PHP Coding Help
Well, the actual solution will depend upon "how" you want to access the files. Are the file names/paths stored in a database and you will pass an ID to find the file details int he database. Or perhaps you will simply pass the file name without the path to the file so the user can't directly link to the file. Here's an example //Get the file name and path in some manner //e.g. pass an id ot name to the page such as //http://www.mydomain.com/showpdf.php?id=4 //Generate a variable with the full path and name to the file on the server $filename = "/pdffiles/mypdf.pdf"; if (file_exists ($filename)) { header("Cache-Control: public"); header("Content-Description: File Transfer"); header("Content-Type: application/pdf"); header("Content-Transfer-Encoding: binary"); header('Content-Length: '. filesize($filename)); readfile($filename); } else { echo "File not found"; } -
[SOLVED] kinda complicated output results
Psycho replied to willrockformilk's topic in Javascript Help
I typically do not test the code I post and instead consider it a "guide" to the poster (ergo the text in my sig). I appreciate the fact that you found/resolved the minor errors - typically people just post back "your code didn't work". Anyway, I'm not an AJAX expert, but I think the problem is that the original code was designed to expect XML data. After looking at a tutorial it looks like there are different ways to receive the response data. Try changing this var divContent = ajaxObject.responseXML.documentElement; To this var divContent = ajaxObject.responseText; -
[SOLVED] kinda complicated output results
Psycho replied to willrockformilk's topic in Javascript Help
You're making this too complicated. When you do the AJAX call simply query for the results then build the formatted output within the PHP code and deliver the formatted results back to the JavaScript to display on the page. The results of an AJAX call do not have to be XML data. function ajaxResponse() { // this function will handle the processing // N.B. - in making your own functions like this, please note // that you cannot have ANY PARAMETERS for this type of function!! if (ajaxObject.readyState == 4) // if ready state is 4 (the page is finished loading) { if (ajaxObject.status == 200) // if the status code is 200 (everything's OK) { // here is where we will do the processing var divContent = ajaxObject.responseXML.documentElement; document.getElementById('descriptionarea').innerHTML = divContent; } else // if the status code is anything else (bad news) { alert('There was an error. HTTP error code ' + ajaxObject.status.toString() + '.'); return; // exit } } // end if // if the ready state isn't 4, we don't do anything, just // wait until it is... } // end function ajaxResponse <?php // Error Display ini_set('display_errors', 1); ini_set('log_errors', 1); ini_set('error_log', dirname(__FILE__) . '/error_log.txt'); error_reporting(E_ALL & ~E_NOTICE); //Connect to Server and Select Database $con = mysql_connect("localhost","root","*******"); if (!$con) { die('Could not connect: ' . mysql_error()); } mysql_select_db("phonedirectory", $con) or die ('Could not select db: ' . mysql_error());; // Start script $firstName = mysql_real_escape_string($_GET['fname']); $queryfirstname = "SELECT * FROM employee WHERE EmpFName LIKE '%{$firstName}%'"; $firstname = mysql_query($queryfirstname) or die(mysql_error()); $rows = mysql_num_rows($firstname); if (mysql_num_rows($firstname)==0) { echo "<table>"; echo "<tr><th>Last Name</th><th>First Name</th></tr>"; while($row = mysql_fetch_array($firstname)) { echo "<tr><td>{$row['EmpLName']}</td><td>{$row['EmpFName']}</td></tr>"; } echo "</table>"; } else { echo "No results found."; } ?> NOTE: Modified the query to 1) escape the user value to prevent SQL injection/data corruption and 2) use a LIKE clause so the user can find partial matches -
Only if you can determine how the records are related. The current code has two seperate lists of records. How are they related? As I stated, if there is a relation you "should" be able to get the data with a single query (including the difference value).
-
Ah, yes. I was thinking about the "reading" of a file and didn't consider XML. You could, of course, read/parse an XML file with JavaScript. If there is a way to read/parse fiels with AJAX that are not XML, please elaborate. I still contend that a server-side language is the way to go due to cross-browser issue whenever dealing with javascript.
-
Yep, byt he time you get to the math operation, you have already run through all of the records from the first query and all the records from the second query. Since you use teh same variable name for the records ($row), the value for $row1['Weight'] from teh first query is wiped out when you get to the calculation. If you were to use different variable names in those while loops you could get the difference between the LAST weight and the LAST score using something like: $diff=($row2['Score'] - $row1['Weight']); But, I'm not sure that is what you are trying to accomplish. Are you trying to get the difference of the total scores and the total weights? That seems doable. but, if you are wanting the difference on a record by record level, I don't know how the records are supposed to match up. If there is some way to correlate the records you could get it all with one query.
-
The '===' means an identical comparison - not just evaluated equal. In other words, the values are just not "evaluated" as equal - they are exactly identical. A few examples should explain clearly: if (1 == true) //result is true (a 1 is evaluated as true) if (1 == 1) //result is true if (1 === true) //result is false (they are not the same type) if (1 === 1) //result is true This PHP manual page should help as well: http://us3.php.net/operators.comparison As for the code tags, you can simply select the PREVIEW button to get your post in a form where you can insert them with the available tools, or just put these around your code (without spaces) and it will display like my examples above: [ c o d e ]code goes here[ / c o d e ]