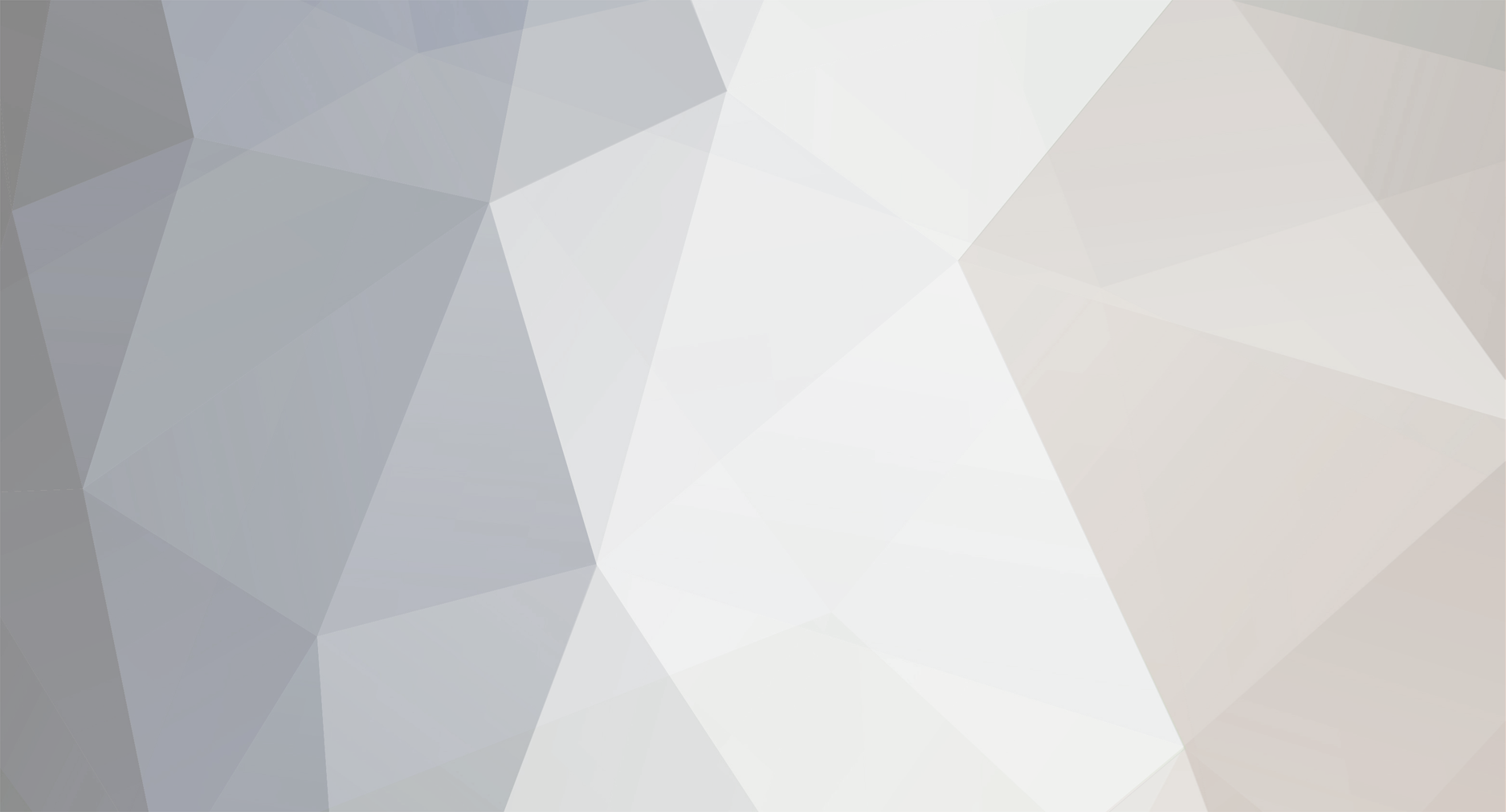
Psycho
Moderators-
Posts
12,159 -
Joined
-
Last visited
-
Days Won
130
Everything posted by Psycho
-
Where "string" is the string variable: string.replace(/clubs$/i, 'club'); That will change "clubs" or "Clubs" (or any case variations, i.e. it is case insensitive) to "club" if those matches occur at the very end of the string. If you maintain the case of the word, someone else might have a simple replace() solution. I could do it, but would take a few more lines of code using a match() and then rebuilding the string. If you need to maintiain case, please state so.
-
Please be specific. Are you wanting to remove the letter "s" in any string where the word "clubs" exists? Are you wanting to remove the last letter from a string? Are you wanting to remove the letter "s" from any string? Give some details as to what you are trying to accomplish - exactly. substr() would be one possible solution based upon certain parameters. In others it may be inefficient or not even appropriate to use.
-
[SOLVED] Textarea info put into separate rows
Psycho replied to shakingspear's topic in PHP Coding Help
I got this to work! That's almost a mirror image of the solution I posted way back on reply #3. However, in my humble opinion, I think mine was a little more efficient because it would 1) Not insert empty values into the database if the user had empty lines and 2) created a single insert quert as opposed to multiple queries. There were some syntax errors in that code, but the logic was sound. I went ahead and cleaned it up - with comments. //Ensure the POST value is set if (isset($_POST['textarea'])) { //Explode the POST value into an array $lines = explode("\n", $_POST['textarea']); //Iterate through each line foreach ($lines as $line) { //Trim leading and trailing spaces $line = trim($line); //If line not empty add to array of values if(!empty($line)) { $values[] = '(' . mysql_real_escape_string($line) . ')'; } } //If array of values has elements create SQL statement & run if (count($values)) { $query = "INSERT INTO random_prompts (prompt) VALUES (" . implode(', ', $values) . ")"; //echo "<pre>$query</pre>"; mysql_query($query) or die(mysql_erorr()); } } -
<html> <head> <script type="text/javascript"> // State lists var states = new Array(); states['Canada'] = ['Alberta','British Columbia','Ontario']; states['Mexico'] = ['Baja California','Chihuahua','Jalisco']; states['United States'] = ['California','Florida','New York']; // City lists var cities = new Array(); cities['Canada'] = new Array(); cities['Canada']['Alberta'] = ['Edmonton','Calgary']; cities['Canada']['British Columbia'] = ['Victoria','Vancouver']; cities['Canada']['Ontario'] = ['Toronto','Hamilton']; cities['Mexico'] = new Array(); cities['Mexico']['Baja California'] = ['Tijauna','Mexicali']; cities['Mexico']['Chihuahua'] = ['Ciudad Juárez','Chihuahua']; cities['Mexico']['Jalisco'] = ['Guadalajara','Chapala']; cities['United States'] = new Array(); cities['United States']['California'] = ['Los Angeles','San Francisco']; cities['United States']['Florida'] = ['Miami','Orlando']; cities['United States']['New York'] = ['Buffalo','New York']; function setStates(){ cntrySel = document.getElementById('country'); stateSel = document.getElementById('state'); stateList = states[cntrySel.value]; changeSelect(stateSel, stateList); setCities(); } function setCities(){ cntrySel = document.getElementById('country'); stateSel = document.getElementById('state'); citySel = document.getElementById('city'); cityList = cities[cntrySel.value][stateSel.value]; changeSelect(citySel, cityList); } function changeSelect(fieldObj, valuesAry, optTextAry, selectedValue) { //Clear the select list fieldObj.options.length = 0; //Set the option text to the values if not passed optTextAry = (optTextAry)?optTextAry:valuesAry; //Itterate through the list and create the options for (var i=0; i<valuesAry.length; i++) { selectFlag = (selectedValue && selectedValue==valuesAry[i])?true:false; fieldObj.options[fieldObj.length] = new Option(optTextAry[i], valuesAry[i], false, selectFlag); } } </script> </head> <body onload="setStates();"> <form name="test" method="POST" action="processingpage.php"> Country: <select name="country" id="country" onchange="setStates();"> <option value="Canada">Canada</option> <option value="Mexico">Mexico</option> <option value="United States">United States</option> </select> <br><br><br> State: <select name="state" id="state" onchange="setCities();"> <option value="">Please select a Country</option> </select> <br><br><br> City: <select name="city" id="city"> <option value="">Please select a Country</option> </select> </form> </body> </html>
-
if($_POST['province']!='all') { $whereList[] = "province = '".mysql_real_escape_string($_POST['province'])."'"; } if($_POST['city']!='all') { $whereList[] = "city = '".mysql_real_escape_string($_POST['city'])."'"; } if($_POST['suburb']!='all') { $whereList[] = "suburb = '".mysql_real_escape_string($_POST['suburb'])."'"; } $whereClause = (count($whereList)) ? ' WHERE ' . implode(' OR ', $whereList) : ''; $query = "SELECT * FROM table $whereClause";
-
[SOLVED] Textarea info put into separate rows
Psycho replied to shakingspear's topic in PHP Coding Help
Try this: <?php $textarea = $_POST['textarea']; $lines = explode("\n", $textarea); foreach ($lines as $line) { if(!empty(trim($line))) { $values[] = '(' . mysql_real_escape_string((trim($line)) . ')'; } } $query = "INSERT INTO random_prompts (prompt) VALUES (" . implode(', ', $values) . ")"; mysql_query($query) or die(mysql_erorr()); ?> -
$code = "<a href=\"test.html\">test</a>"; preg_match_all("/href=\"(.*)\"/i", $code, $array); echo '<pre>'; print_r($array); echo '</pre>'; EDIT: DO not "bump" after only 10 minutes.
-
How to pass php variable containing quote to JS function
Psycho replied to blogfisher's topic in PHP Coding Help
Try this. I used addslashes() and then replaced \" with " <?php $single = "sing'le"; $double = 'dou"ble'; $slash = "sla\sh"; ?> <html> <head> <SCRIPT LANGUAGE="JavaScript"> function readText (form, data) { TestVar = form.inputbox.value; alert ("You typed: " + TestVar + "... and ..." + data); } </SCRIPT> </head> <body> <form name=""> Input Box:<br /> <input type="text" name="inputbox"> <br /> <button onclick="readText (this.form, '<?php echo str_replace ('\"', '"', addslashes($single)); ?>');">Single Quote test</button><br /> <button onclick="readText (this.form, '<?php echo str_replace ('\"', '"', addslashes($double)); ?>');">Double Quote test</button><br /> <button onclick="readText (this.form, '<?php echo str_replace ('\"', '"', addslashes($slash)); ?>');">Slash test</button><br /> </form> </body> </html> -
How to pass php variable containing quote to JS function
Psycho replied to blogfisher's topic in PHP Coding Help
What ^ he ^ said echo "<INPUT TYPE=\"button\" NAME=\"button1\" Value=\"Read\" onClick=\"javascript:readText(this.form, \"".addslashes($myvar)."\")\">"; -
Only checkboxes that are "checked" are passed in the form data. So, if you make the boxes an array using a name such as "u[]" then the first "checked" item will have an index of 0, the second 1, etc. Regardless of where they were positioned in the form. You shouldn't rely upon the index of the values in any case. None of the code you posted was really very complete. From your first post, it looks as if you are getting records from a database and creating a chekbox option for each. If that is the case you should set the value of each checkbox to the ID of the record. Then the PHP page that receives the POST data will be able to determine which records were selected. Something like this: <?php while($row=mysql_fetch_array($res)) { echo "<tr align=\"center\">\n"; echo " <td><input type=\"checkbox\" name=\"u[]\" value="{$row['id']}" /></td>\n"; echo " <th>{$row['name']}</th>\n"; echo " <th>{$row['username']}</th>\n"; echo " <td><input type=\"password\" name=\"pwd_{$row['id']}\" /></td>\n"; echo "</tr>\n"; } ?>
-
For this line: header("Location: ".$at."member.php"); I will assume you have a check on that page to ensure the user is already logged in AND that you are checking the value for $_SESSION['access_type']. Otherwise, users can simply change the URL to see pages they are not supposed to see. Just a suggestion, but instead of having specific pages for each accesstype you could create a single initial page which checks the value of $_SESSION['access_type'] and then includes the correct content page. Also, another suggestion, I typically like to trim() the input values for text fields so spaces are not considered. In this case the username field. But, that would also require that the values are trimmed during the creation process. Otherwise you could end up with a username created with leading or trailing spaces, but the user would never be able to log in! I never trim() passwords.
-
You're welcome. Please mark the topic as solved.
-
<SCRIPT LANGUAGE="JavaScript"> function Check(chkOptions, chkState) { for (i=0; i<chkOptions.length; i++) { chkOptions[i].checked = chkState; } return; } </script> Here is the code for body part . <form name="modifyform" action="1.php" method="post"> <b>Sample Program</b><br> <input type="checkbox" name="master" value="yes" onClick="Check(document.modifyform['u[]'], document.modifyform.master.checked)"><b>Check Control</b> <br/> <?php for($i=0;$i<5;$i++) {?> <input type="checkbox" name='u[]' value='<?php echo $i ?>'>ASP <?php echo $i ?><br> <?php } ?> <input type="submit" value="submit" name="gen"/> </form> <?php foreach($_POST['u'] as $checkValue) { echo "$checkValue<br />"; } ?>
-
Then see if you can find something with the features they need that you feel you can modify. Unless they are wanting some really non-standard functionality (which it doesn't sound like from your explanation) I find it hard that there is nothing available to meet their needs. More likely they didn't like the "look" of those ready made solutions. But, they are typically built to be customized for the look and feel - which sounds like something right up your alley. I would start by getting a list of requirements from them and I bet you will find that there are plenty of ready-made solutions that fit their needs.
-
It all depends on the level of functionality that is required. For an extremely basic site, it is totally doable. But, even for a basic site, I would be VERY hesitant to have someone with little experience putting it together. We are talking abut monetary transaction. Even if the CC transactions are being taken care of by a 3rd party souce, the site developer is required to create the code needed to connect to that source. And, even putting that aside, the developer is responsible for creating all the code for creating the cart, totally the order with shipping, taxes, etc. One mistake can cause many problems in lost revenue or customer perception. There are many low cost ecomerce ready solutions available. I'd suggest finding one with the features you need and see about customizing it.
-
OI, your problem stems from teh fact that your IF condition is SETTING the value insted of TESTING the value. Wrong: if ($row1['time'] = "08:00") Right: if ($row1['time'] == "08:00") But, a better solution would be to add the 'time' column to your WHERE clause <?php $time = '8:00'; $query1= "SELECT time, description FROM cal_events WHERE date = '$finaldate1' AND user_id = '$employee' AND time = '$time'"; $result1=mysql_query($query1); while($row1=mysql_fetch_assoc($result1)) { ?> <th scope="col">8:00am</th> <th height="48" scope="col"><?php echo $row1['description']; ?></th>
-
[SOLVED] using getelementbyname instead of getelementid
Psycho replied to M.O.S. Studios's topic in Javascript Help
1. JavaScript (which is what we are talking about) is not Java. Two different things. 2. No need to go back to a select list. Just need to incorporate the function I posted. Try this: <html> <head> <script type="text/javascript"> function radioGroupValue(groupObj) { //Check if radio group has multiple options (i.e. is an array) if (groupObj.length) { //Iterrate through each option for (var i=0; i<groupObj.length; i++) { //Check if option is checked if (groupObj[i].checked) { //Return value of the checked radio button return groupObj[i].value; } } } //There is only one option in the radio group else if (groupObj.checked==true) { return groupObj.value; } //No option was selected return false; } function sendToPageOne() { var thisValue = radioGroupValue(document.form2.image1); window.opener.form1.image1.value = thisValue; } </script> </head> <body> <table width="100%" height="100%" cellpadding="0" cellspacing="0" frame="0" border="0"> <form method="POST" action="account.php?actions=addpic" enctype="multipart/form-data"> <tr width='100%'><td width='100%' height='5'><a href='account.php?actions=addpic'><font size='2'><i>root</i></font></a></td></tr> <tr width='100%'><td width='100%' height='5' bgcolor='#a4b9f7'><input type='submit' name='directory' value='upload/..' id='submitb'></td></tr> <tr width='100%'><td width='100%' height='5' bgcolor='#779ff7'><input type='submit' name='directory' value='../../upload/cards' id='submitb'></td></tr> <tr width='100%'><td width='100%' height='5' bgcolor='#779ff7'><input type='submit' name='directory' value='../../upload/test' id='submitb'></td></tr> </form> <form name="form2" action="browse.php" method="POST"> <tr width='100%'><td width='100%'> <input type="radio" name="image1" id=image1 value="" checked>None<br> <input type="radio" name="image1" id=image1 value="../../upload/1.jpg">1.jpg<br> <input type="radio" name="image1" id=image1 value="../../upload/2.jpg">2.jpg<br> <input type="radio" name="image1" id=image1 value="../../upload/2472178932_060c51e5a7[1].jpg">2472178932_060c51e5a7[1].jpg<br> <input type="radio" name="image1" id=image1 value="../../upload/Crater.jpg">Crater.jpg<br> <input type="radio" name="image1" id=image1 value="../../upload/clistheader[1].jpg">clistheader[1].jpg<br> <input type="radio" name="image1" id=image1 value="../../upload/cube.jpg">cube.jpg<br> <input type="radio" name="image1" id=image1 value="../../upload/gcube.jpg">gcube.jpg<br> <input type="radio" name="image1" id=image1 value="../../upload/test.jpg">test.jpg<br> <input type="submit" onclick="sendToPageOne();self.close();"; value="Send to page 1"> </td> </tr> </form> </table> </body> </html> -
[SOLVED] using getelementbyname instead of getelementid
Psycho replied to M.O.S. Studios's topic in Javascript Help
The problem has nothing to do with getElementById. The problem is that you are dealing with a radio group. With a select list you can find the value of the select list object. But, with a radio group each option has a value and there is no direct way to reference the selected value. To add to the problem, a radio group is treated differently if it has multiple options or only one option. I have a small custom function that will return that selected value of a radio group regardless of the number of options: function radioGroupValue(groupObj) { //Check if radio group has multiple options (i.e. is an array) if (groupObj.length) { //Iterrate through each option for (var i=0; i<groupObj.length; i++) { //Check if option is checked if (groupObj[i].checked) { //Return value of the checked radio button return groupObj[i].value; } } } //There is only one option in the radio group else if (groupObj.checked==true) { return groupObj.value; } //No option was selected return false; } -
Your table description is either wrong or it just won't work. You state that the LID field is what links table1 and table2 data and that there are many records in table2 that link to records in table1. But, you also state that LID is not unique in table1 (the user table). If LID is not unique in table1 how do you know which records in table2 link to which records in table1? I did have a typo in the code I posted previusly - but I did link to the page with an explanation. You could have read that page and figured it out for yourself. Assuming that LID is unique in table 1 (but not table2) than this should work for you: (same as I provided above, but with different field names) SELECT table1.ID, table1.LID, MAX(table2.aa0) aa0, table2.aa1 FROM table2 JOIN table1 on table1.LID = table2.LID GROUP BY table2.LID
-
It would have been MUCH better to use some real-world names/data instead of table1, table2, etc. For instance you could have said you had a situation similar to a customer table and an order table and you were looking to get the details for the last order for each customer. Anyway, this might work for you, give it a try: SELECT table1.number1, table1.status, MAX(table2.info1), table2.info2 FROM table2 JOIN table1 on table1.number1 = table2.number2 GROUP BY table2.number1 Reference: http://www.tizag.com/mysqlTutorial/mysqlmax.php
-
"$result" is a database result set. You can't print it to the page and it's value is meaningless for comparison purposes. You need to extract the records from the result set. Also, you aren't even using closing tags on your anchors. Sloppy code will always be errror prone. Try this: if($session->isAdmin()) { $query = "SELECT locked FROM forumtutorial_posts WERE postid = $id"; print "Query: $query<br /><br />\n"; //For debugging only $result = mysql_query($query) or die(mysql_error());; $record = mysql_fetch_assoc($result); print"  -<A href='deletetopic.php?id=$id'>Delete Topic</a> \n"; if ($record['locked'] < 1) { // if locked is set to 0 (unlocked) print"  -<A href='locktopic.php?id=$id'>Lock Topic</a>\n"; } else { //otherwise show unlock print"  -<A href='unlocktopic.php?id=$id'>Unlock Topic</a>\n"; } }
-
The solutino will depend upon several factors. If you need to have it show dynamically (i.e. the user does not have to submit the page to get the value) then it will require JavaScript. You could either do it totally within JavaScript by doing a DB query of all the ID/name pairs and creating JavaScript variables or you could do an AJAX request each time the user changes the select list. The first option is typically much faster, but if you have many, many records it might be a problem. AJAX would require a hit to the database each time the user changes the select list, but only for the specific record. It can also have a slight delay. One very easy way to do it in this situation would be for the select field to have the ID as the label for the options and the name as the value for the options. That field would pass the name with the form - but you could also use the value (the name) to populate a text field. But, you would not have the ID in any field - so just populate a hidden field with the label of the option selected. <html> <head> <script> function populateUserName(selObj) { var selOption = selObj.options[selObj.selectedIndex]; if (selOption.value) { document.getElementById('user_name').value = selOption.value; document.getElementById('user_id').value = selOption.text; } else { document.getElementById('user_name').value = ''; document.getElementById('user_id').value = ''; } } </script> </head> <body> User ID:<br> <select name="user_sel" onchange="populateUserName(this);"> <option value="">--Select--</option> <option value="Bob Smith">4</option> <option value="Thomas Edison">12</option> <option value="Wilber Johnson">27</option> </select> <br></b><br> User Name:<br> <input type="text" name="user_name" id="user_name" /> <br></b><br> User ID [Make this hidden]:<br> <input type="text" name="user_id" id="user_id" /> </body> </html>
-
Sorry, but I still don't see why you are having such difficulty. The code I posted worked fine for me. As for not "init" the first field I assume you mean you want the first field to be checked if none of them are checked. I didn't pick that up from your code. It is not that it is difficult, just that it is not something I would normally see as a requirement. As for the problems you mentioned: 1. Boxes/Buttons do not init as checked or on What does that mean? Are you saying you want a checkbox or button to be checked by default if none was selected by the user.? Simple enough. 2. Radio Button will not uncheck. You can't "uncheck" radio buttons - that is by their nature. 3. Check boxes click up in value but will not decrement unless highest box value is checked. It looks as if you are creating multiple checkboxes to act as a radio group. Why, just use a radio group. The code last posted has a lot of problems with basic logic: $show_me = 0; if ($show_me==1) { foreach ($_POST as $key => $val) { echo "K=> $key V=> $val <br>"; } } What is the purpose of that code. You first set the value of $show_me to 0 and then have a condition to test if it is equal to 1. That code has absolutely no purpose. if (!isset($_POST['one'])) { $chk_stat = 'checked="checked"'; } $chk_stat = (isset($_POST['one'])) ? 'checked="checked"' : ''; $dsp_lin = (isset($_POST['one'])) ? 'Box Checked!' : 'Box UnChecked!'; Not sure what the purpose of $chk_stat is since you set it to the same value no matter if it was checked previously or not. Most of those lines are totally unneeded. Just have this: $chk_stat = 'checked="checked"'; $dsp_lin = (isset($_POST['one'])) ? 'Box Checked!' : 'Box UnChecked!'; Also, the radio groups field names were changed such that they are no longer treated as arrays - which they are supposed to be. Also, the three checkboxes should also be given names such that they are treated as arrays based upon your usage of them. I'm really not understanding what you are tryin to accomplish here. This is apparently more of an academic exercise rather than a real-world problem. A form is typically filled out and submitted so that information can be processed. This page seems to be one where it is continually submitted to "mirror" the functionality that would exist if you were not submitting the form on every click.
-
Well, I can think of one way where you would only have to test "some" of the values. Don't know how efficient this would be. function includedValues($array, $min, $max) { sort($array, SORT_NUMERIC); //Remove values less than the min while($array[0]<$min) { array_shift($array); } //Remove values greater than the max while($array[count($array)-1]>$max) { array_pop($array); } return array; }
-
To be honest I don't have a clue as to what problem you are trying to explain. Processing checkboxes and radio buttons is not difficult. Your code looks to be way more complicated than it needs to be: creation of variable that are not needed, hard coding when loops should be used, etc. I reduced your 58 lines of PHP code to process the data to about 20 lines and it all works. <?php $chk_stat = (isset($_POST['one'])) ? ' checked="checked"' : ''; $dsp_lin = (isset($_POST['one'])) ? 'Box Checked!' : 'Box UnChecked!'; if (isset($_POST['two'])) { foreach($_POST['two'] as $field) { $chkVar = 'chk_vl'.$field; $$chkVar = ' checked="checked"'; $dsp_ary[] = "Checkbox $field is checked"; } $dsp_str = implode(', ', $dsp_ary); } $rad_stat = (isset($_POST['rd1'])) ? ' checked="checked"' : ''; $rad_lin = (isset($_POST['rd1'])) ? 'Button Checked!' : 'Button UnChecked!'; if (isset($_POST['rd2'])) { foreach($_POST['rd2'] as $field) { $radVar = 'rad_vl'.$field; $$radVar = ' checked="checked"'; $rad_ary[] = "Button $field is checked"; } $rad_str = implode(', ', $rad_ary); } ?> <html> <head> <script> function checkedAll (id, checked) { var el = document.getElementById(id); for (var i = 0; i < el.elements.length; i++) { el.elements[i].checked = checked; } } // document.form['oneclick'].submit(); </script> </head> <body> <!-- PHP section --> <form name=oneclick id="form_one" method=post action="<?php echo $_SERVER['PHP_SELF']; ?>" > <font size='+3' color='blue'><b>PHP Section</b></font><br> <p> <table border='0' cellspacing='0' cellpading='0'> <td> <font size='+2'><b>Check Boxes</b></font><br> <input type="checkbox" name="one" onclick="document.oneclick.submit()"<?php echo $chk_stat; ?> /> <font size='+1'><b>Single Toggling Checkbox</b></font><br> <p> <?php echo "<p> $dsp_lin"; ?> <p> <font size='+1'><b>Multiple Checkboxes</b></font><br> <p> <input type="checkbox" name="two[]" id='1' value='1' onclick="document.oneclick.submit()"<?php echo $chk_vl1; ?> /> Checkbox One <input type="checkbox" name="two[]" id="2" value=2 onclick="document.oneclick.submit()"<?php echo $chk_vl2; ?> /> Checkbox Two <input type="checkbox" name="two[]" id="3" value=3 onclick="document.oneclick.submit()"<?php echo $chk_vl3; ?> /> Checkbox Three <p> <?php echo "<p> $dsp_str"; ?> </td> <td width='100'> </td> <td> <font size='+2'><b>Radio Buttons</b></font><br> <input type="radio" name="rd1" onclick="document.oneclick.submit()" <?php echo $rad_stat; ?> /> <font size='+1'><b>Single Toggling Button</b></font><br> <p> <?php echo "<p> $rad_lin"; ?> <p> <font size='+1'><b>Multiple Buttons</b></font><br> <p> <input type="radio" name="rd2[]" id='1' value='1' onclick="document.oneclick.submit()"<?php echo $rad_vl1; ?> /> Button One <input type="radio" name="rd2[]" id=2 value=2 onclick="document.oneclick.submit()"<?php echo $rad_vl2; ?> /> Button Two <input type="radio" name="rd2[]" id=3 value=3 onclick="document.oneclick.submit()"<?php echo $rad_vl3; ?> /> Button Three <p> <?php echo "<p> $rad_str"; ?> </td> </tr> </table> </form> <p> <hr width='100%' align='center'> <!-- JavaScript section --> <form name='twoclick' id="form_two" method='post' action="<?php echo $_SERVER['PHP_SELF']; ?>" > <font size='+3' color='blue'><b>JavaScript Section</b></font><br> <p> <table border='0' cellspacing='0' cellpading='0'> <td> <font size='+2'><b>Check Boxes</b></font><br> <input type="checkbox" name="cb1" id='1' onclick="javascript:toggleAll('form_two', cb1)"> <font size='+1'><b>Single Toggling Checkbox</b></font><br> <p> <?php echo "<p> $jav_lin"; ?> <p> <font size='+1'><b>Multiple Checkboxes</b></font><br> <p> <input type="checkbox" name="cb2" id='1' onclick="javascript:toggleItem('form_two', cb2)"> Checkbox One <input type="checkbox" name="cb2" id=2 onclick="javascript:toggleIten('form_two', cb2)"> Checkbox Two <input type="checkbox" name="cb2" id=3 onclick="javascript:toggleItem('form_two', cb2)"> Checkbox Three <p> <?php echo "<p> $jav_str"; ?> </td> <td width='100'> </td> <td> <font size='+2'><b>Radio Buttons</b></font><br> <input type="radio" name="jv1" onclick="javascript:toggleAll('form_two', jv1)"> <font size='+1'><b>Single Toggling Button</b></font><br> <p> <?php echo "<p> $jav_out"; ?> <p> <font size='+1'><b>Multiple Buttons</b></font><br> <p> <input type="radio" name="jv2" id='1' value='1' onclick="javascript:toggleItem('form_two', jv2)"> Button One <input type="radio" name="jv2" id=2 value=2 onclick="javascript:toggleItem('form_two', jv2)"> Button Two <input type="radio" name="jv2" id=2 value=2 onclick="javascript:toggleItem('form_two', jv2)"> Button Three <p> <?php echo "<p> $jav_sel"; ?> </td> </tr> <tr> <td> <font size=+2><b>Select/Unselect All</b></font><br> <p> <input type="checkbox" name="foo" id='1'/> <input type="checkbox" name="bar" id=2/> <a href="javascript:checkedAll('form_two', true)">all</a> <a href="javascript:checkedAll('form_two', false)">none</a> </td> </tr> </table> </form> </body> </html>