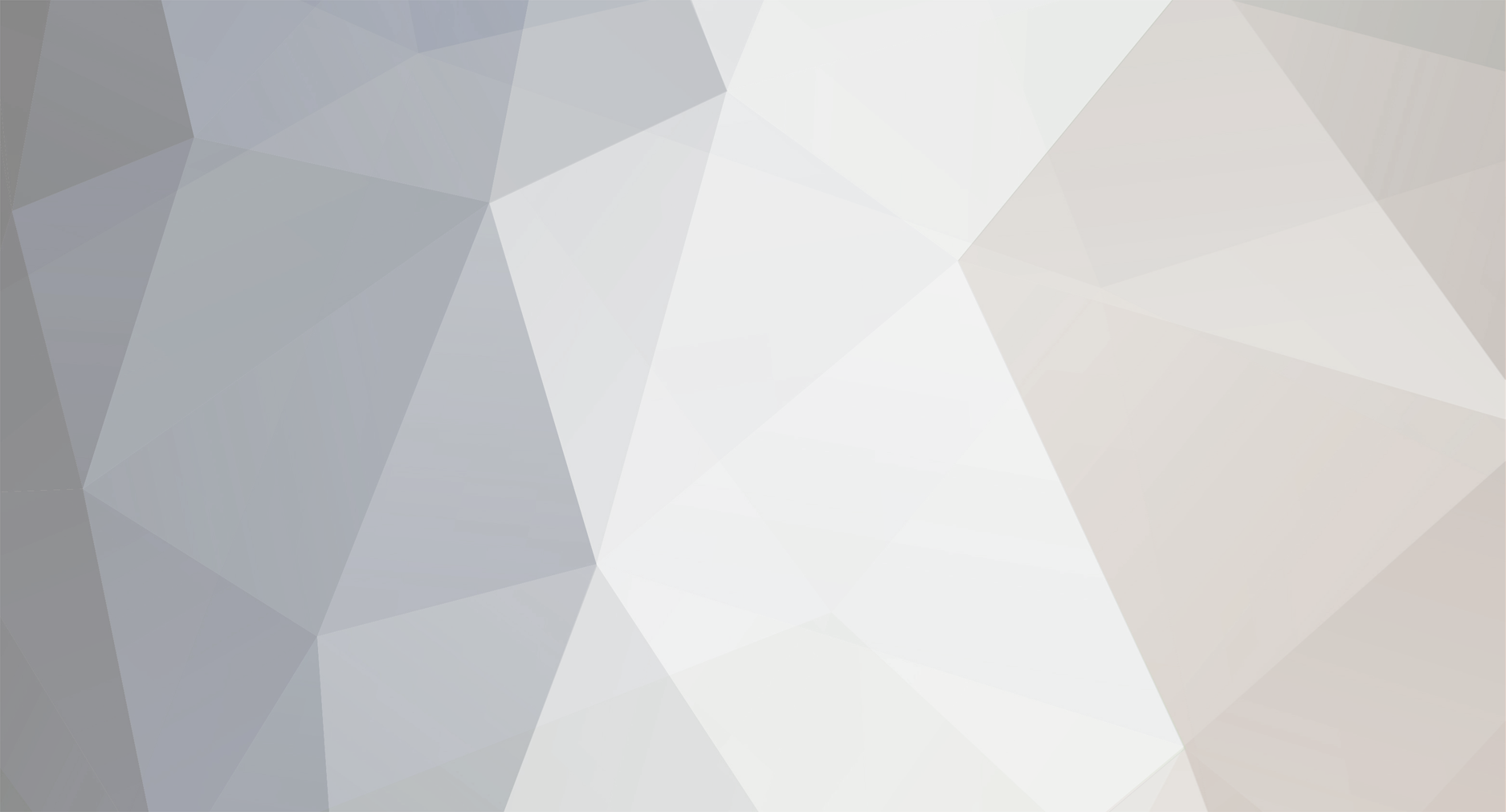
Psycho
Moderators-
Posts
12,159 -
Joined
-
Last visited
-
Days Won
130
Everything posted by Psycho
-
Why would you not allow '+' (plus) or '.' period? those are perfectly acceptable characters for an email - a period is a very common character for an email. But, you can modify it if you wish. This shoudl do it as you asked, but I havent tested it: function is_email($email) { $formatTest = '/^[a-z0-9][-\w]+@[-a-z\d]{2,}(\.[-a-z\d]{2,})*\.[a-z]{2,6}$/i'; $lengthTest = '/^(.{1,64})@(.{4,255})$/'; return (preg_match($formatTest, $email) && preg_match($lengthTest, $email)); }
-
Yes, I forgot to add that (I also used a '=' instead of '+' when creating the array_to_string to add it at then end of that) - thanks. Fixed: function in_array(searchArray, searchString) { var array_to_string = '~' + searchArray.join('~') + '~'; return ( (array_to_string.search('~'+searchString+'~')!=-1) ? true : false); }
-
The above example seems more complex than it needs to be in my opinion (couldn't open the external link). Here is a simple function I created to ensure an email is properly formatted. function is_email($email) { $formatTest = '/^[-\w+]+(\.[-\w+]+)*@[-a-z\d]{2,}(\.[-a-z\d]{2,})*\.[a-z]{2,6}$/i'; $lengthTest = '/^(.{1,64})@(.{4,255})$/'; return (preg_match($formatTest, $email) && preg_match($lengthTest, $email)); } The above validates the following: Format test - Username accepts: 'a-z', 'A-Z', '0-9', '_' (underscore), '-' (dash), '+' (plus), & '.' (period) Note: cannot start or end with a period (and connot be in succession) - Domain accepts: 'a-z', 'A-Z', '0-9', '-' (dash), & '.' (period) Note: cannot start or end with a period (and connot be in succession) - TLD accepts: 'a-z', 'A-Z', & '0-9' Length test - Username: 1 to 64 characters - Domain: 4 to 255 character
-
I'm sure there's some reason why you want to change a button type to a submit button, but I can't think of any good ones. Can you explain the reason behind what you are trying to accomplish? There might be a better solution. Besides, doing what you describe above would probably block any usesrs w/o JS enabled.
-
I'd just add a couple of comments: 1) If you do build a function to do this, be sure to "break" out of the loop whne you find the value to prevent unnecessary execution. 2) You can also do this without looping through the array with a little slight of hand. However, it may or may not be more efficient based upon the size of the array. You would also want to use delimiters that would not appear in the data.: (array is the array to be searched) function in_array(searchArray, searchString) { var array_to_string = '~' + searchArray.join('~') = '~'; return ( (array_to_string.search(searchString)!=-1) ? true : false); }
-
I think you're taking an overly complex approach here. Just create BOTH the select field AND the text field. Then use DHTML to determine which one to display. The server side code would also need to check which value to use from the POST. The way you explain how you want it to work, the server-side code would just need to check if the text field has a value - if so use it - otherwise use the select field. One very good benefit of this approach would be for users that do not have JS enabled. They would see both fields. Then, if they need a custom value, they would enter it in. Here's one solution <html> <head> <script type="text/javascript"> function displayCustom(selID, fieldID) { selObj = document.getElementById(selID); fieldObj = document.getElementById(fieldID); if (selObj.selectedIndex==0) { fieldObj.disabled = false; document.getElementById(fieldID+'_span').style.visibility = ''; } else { fieldObj.disabled = true; document.getElementById(fieldID+'_span').style.visibility = 'hidden'; } return; } window.onload = function() { displayCustom('category', 'custom_cat'); } </script> </head> <body> Category: <select name="category" id="category" onchange="displayCustom(this.id, 'custom_cat');"> <option value="">Custom</option> <option value="1" selected="selected">Category A</option> <option value="2">Category B</option> <option value="3">Category C</option> </select> <br /> <span id="custom_cat_span">Custom Category: <input type="text" name="custom_cat" id="custom_cat"></span> </body> </html>
-
That's some interesting code you have there. I would just create a single form and let the user update one item or all six at the same time. I'll leave it to you to change the descriptive text at thte top, but this should be pretty intuitive [Note, you should never copy & paste code when you have the same exact functionality. It makes future changes suceptible to bugs] <html> <body> <p> Jim or Tom: on this page, just enter in the new text that you want to replace </p> <p>the old text (what's currently "live" on the site is shown in the boxes) and hit submit.</p> <br /><br /> <?php if ($_POST['Submit']=='Change') { //Create form with current values echo "<form action=\"".$PHP_SELF."\" method=\"post\">\n"; for($special_no = 1; $special_no<=6; $special_no++) { echo "<b>Special #{$special_no} Description</b><br />\n"; echo "<textarea Name=\"update{$special_no}\" cols=\"40\" rows=\"4\">"; $text = file_get_contents("includes/special_{$special_no}_title.txt"); echo $text; echo "</textarea><br /><br />\n"; } echo "<input type=\"submit\" name=\"Submit\" value=\"Submit Changes\">"; echo "</form>\n"; } else { //Display the current/changed value for($special_no = 1; $special_no<=6; $special_no++) { if($_POST['Submit']=='Submit Changes') { $file = fopen("includes/special_{$special_no}_title.txt","w+"); $text = $_POST['update'.$special_no]; fwrite($file, $text); fclose($file); } $text = file_get_contents("includes/special_{$special_no}_title.txt"); echo "<b>Special #{$special_no} Description</b><br />\n"; echo nl2br($text) . "<br /><br />\n"; } echo "<form action=\"".$PHP_SELF."\" method=\"post\">"; echo "<input type=\"submit\" name=\"Submit\" value=\"Change\">"; echo "<form action=\"".$PHP_SELF."\" method=\"post\">"; } ?> </body> </html>
-
Why don't you provide a couple examples of input text and the expected output with an explanation of how the output is to be derived.
-
I didn't correct your code. I only formatted it so the prolem was apparent - which is there is a closing curly bracket "}" without a corresponding opening bracket. I don't know how your class and functions are supposed to be configured.
-
I'm too lazy to check at the moment if JavaScript can "test" if a file exists via a URL. But, even if it did, that seems like a complex solution for something simple. You could just set a single value of the total number of banners there are. Then the script to change banners will start with 1 and keep incrementing programatically until it reaches the max number and the start over at 1 again.
-
I have to agree with waynewex, I took a few minutes to "properly" format the code and the error was redily apparent. Here is your code with logical indentation down to line 82. It looks like you closed the class too early <?php define("EZXEZ_LIB", "EzXez Unlimited2"); define("EZXEZ_VER", "v 1.1 beta"); define("EZXEZ_BUILD", "20081202"); define("EZXEZ_AUTH", "by www.nabrak.biz.tm"); define("EZXEZ_LOGIN", "http://www.nabrak.biz.tm/ezxez/index.php"); define("EZXEZ_KIRIM", "http://www.nabrak.biz.tm/ezxez/index.php"); define("EZXEZ_CLEFT", "http://www.nabrak.biz.tm/ezxez/index.php"); Class EZXEZ { var $cookie, $ch, $is_init, $cleft, $newurl, $cLoaded; function LibName() { return array(EZXEZ_LIB,EZXEZ_VER,EZXEZ_BUILD,EZXEZ_AUTH); } function UserAgent() { /* $agent = EZXEZ_LIB . '/' . EZXEZ_VER . '(' . EZXEZ_BUILD . '-' . EZXEZ_AUTH; */ return $_SERVER[HTTP_USER_AGENT]; } function Login($user, $pass, $tujuan, $pesan){ $no = $this->Nomer($user); if ($no == 0) { return 0; } else { $cid = $no[0]; $pid = $no[1]; } $loginPost = "user=".$this->tujuan($user)."&pass=".$pass."&tujuan=".$tujuan."&pesan=".$pesan; $login = $this->useCurl(EZXEZ_LOGIN,$loginPost); if ($this->newurl == 'index.php') return 1; return 0; } //########################## //Ends the Class EZXEZ //########################## function kirimSms ($user, $pass, $tujuan, $pesan,$nick="",$nolimit=1) { $pesan = ''; if (!empty($nick)) { $pesan .= $nick.'%3A%0D'; } $pesan .= rawurlencode($pesan); $hasil = ''; if ($nolimit == 1) $pesan = substr($pesan,0,160); $panjang = strlen($pesan); if (strlen($pesan)>160) $panjang = 160; $kirimPost = "user=".$this->tujuan($user)."&pass=".$pass."&tujuan=".$tujuan."&pesan=".$pesan; $kirim = $this->useCurl(EZXEZ_KIRIM,$kirimPost); //index.php?p=sendsms if ($this->newurl == 'index.php' ) { $hasil .= $tujuan.' => OK! '; } else { $hasil .= $tujuan.' => Gagal Terkirim! '; $err = 1; } } //########################## //Ends the function kirimSms //########################## if ($err == 1) return array(0,'Failed'); return array(1,$hasil); } // ????????????
-
OK, sorry. your problem is that you do not have a single quote mark following the topbar or content values. Add the single quote marks in red below: $SQL = "UPDATE `pgContent` SET `topBar`='{$top}' `content`='{$content}' WHERE `pageId`='{$id}'";
-
[SOLVED] Just curious if this is possible with PHP...
Psycho replied to galvin's topic in PHP Coding Help
<html> <head> <script type="text/javascript"> function updateText(inputObj, outputID) { document.getElementById(outputID).innerHTML = inputObj.value; } </script> </head> <body> Type your input here: <input type="text" id="text" name="text" onkeyup="updateText(this, 'spanID');" /> <br /><br /> The text you are typing is: <span id="spanID"><span> </body> </html> -
What is the full error message witht he line number?
-
Although I see many things I would change, your problem is on line 11 of the 2nd script you posted $id = $_GET['id']; You are POSTing data to that page, not sending it on the URL. Change that to $id = $_POST['id']; and create a hidden field on the form with the ID. I don't think you can POST and GET data at the same time. I would recommend adding an on die() handler to all of your db calls. I typically have the error echo the full query to the page when it fails. In this case you would have seen that the value for the id was empty.
-
<input type="text" onfocus="this.style.width='200px';" onblur="this.style.width='50';" />
-
Maybe I'm not understanding this 100%. But, I just took the code you posted and created a new file on my desktop. When I run the page in a browser, the word "Hello" is displayed. Since that text does not appear in the code I assume that is the text returned by the AJAX call and populated into the DIV. Although there is an error on this line reSizeLeftRight(); because that function is not within the code you provided. I don't see any parameters being used in that code such as you explained, so I'm not sure what should or should not be happening here. If the remote sites are going to include the entire AJAX code, it should work fine. But, you cannot include external javascript files from remote sites.
-
No disrespect intended. I was just making a correction (you can't use count() on a result set as posted in Reply #2, was not referring to the array solution) and showing another alternative to get both all the records and the last record independantly without dumping the results into an array and without a 2nd query.
-
mysql_fetch_array() fetches one record as an array - not the entire result set. The OP is looking for the last record int he result set.
-
Actually, I don't think dclamps code would work. It is using a count() on a mysql result resource. Should be using a mysql_num_rows(). But, you could use something like that without dumping the results into an array first: $result = mysql_query("SELECT * FROM news WHERE date <= NOW() ORDER BY id ASC") or die(mysql_error()); //Add last record to a new array $last_record = mysql_result($result, mysql_num_rows($result)-1); //Run through all results from query while ($record = mysql_fetch_assoc($result)) { // Do something }
-
OK, after a more thorough look at the code - it is not amkign any sense. The same query is being run twice - with nothing being doine with the results from the first query not being used. Plus, after the second query, there is a while loop that does nothing inside the loop which leaves only the last record being assinged to the array variable $plans. Then, that avaraible is only used once for $plans['mcharge']. I have no clue what is trying to be accomplished here. And, why wuld you turn off error reporting when there are problems you are trying to debug? Also, I always create my queries as string variables so I can echo them to the page when there is a problem. It helps to determine that variables have the values I expect them to. Lastly, you are running nested queries. That can be done MUCH more efficiently by using JOINs in your queries. I think much of the problem is due to the fact that the very first query is only returning the id column, but the second query is trying to use the 'plan' value. The first query will need to return that column if you want to use it. I would make an attempt at fixing the code, but again I'm not 100% sure of what the goal is.
-
Unless "plan" is a constant, you can't use $id[plan]. The index name must have quotes around it. Try this: $query = "SELECT * FROM `plan` WHERE `id` = '{$id['plan']}'" $plan1_sql = mysql_query($query) or die("Error!");
-
<html> <head> <script type="text/javascript"> function addValue(fieldID, addAmount) { var fieldObj = document.getElementById(fieldID); var newValue = (parseInt(fieldObj.value) + addAmount); fieldObj.value = (newValue<0) ? 0 : newValue; return; } </script> </head> <body> Count: <input type="text" name="count" id="count" readonly="readonly" value="5" /> <button onclick="addValue('count', 1)">+1</button> <button onclick="addValue('count', -1)">-1</button> <br /><br /> <button type="submit">Submit</button> </body> </html>
-
Yes, please elaborate. I have no clue what you are asking.
-
calling a function multiple times with different param's
Psycho replied to nicktherockstar's topic in Javascript Help
Try this: function runOnLoad() { getLiveData('index.php?cont=1','myDiv1'); getLiveData('index.php?cont=2','myDiv2'); getLiveData('index.php?cont=3','myDiv3'); } <body onload="runOnLoad();">