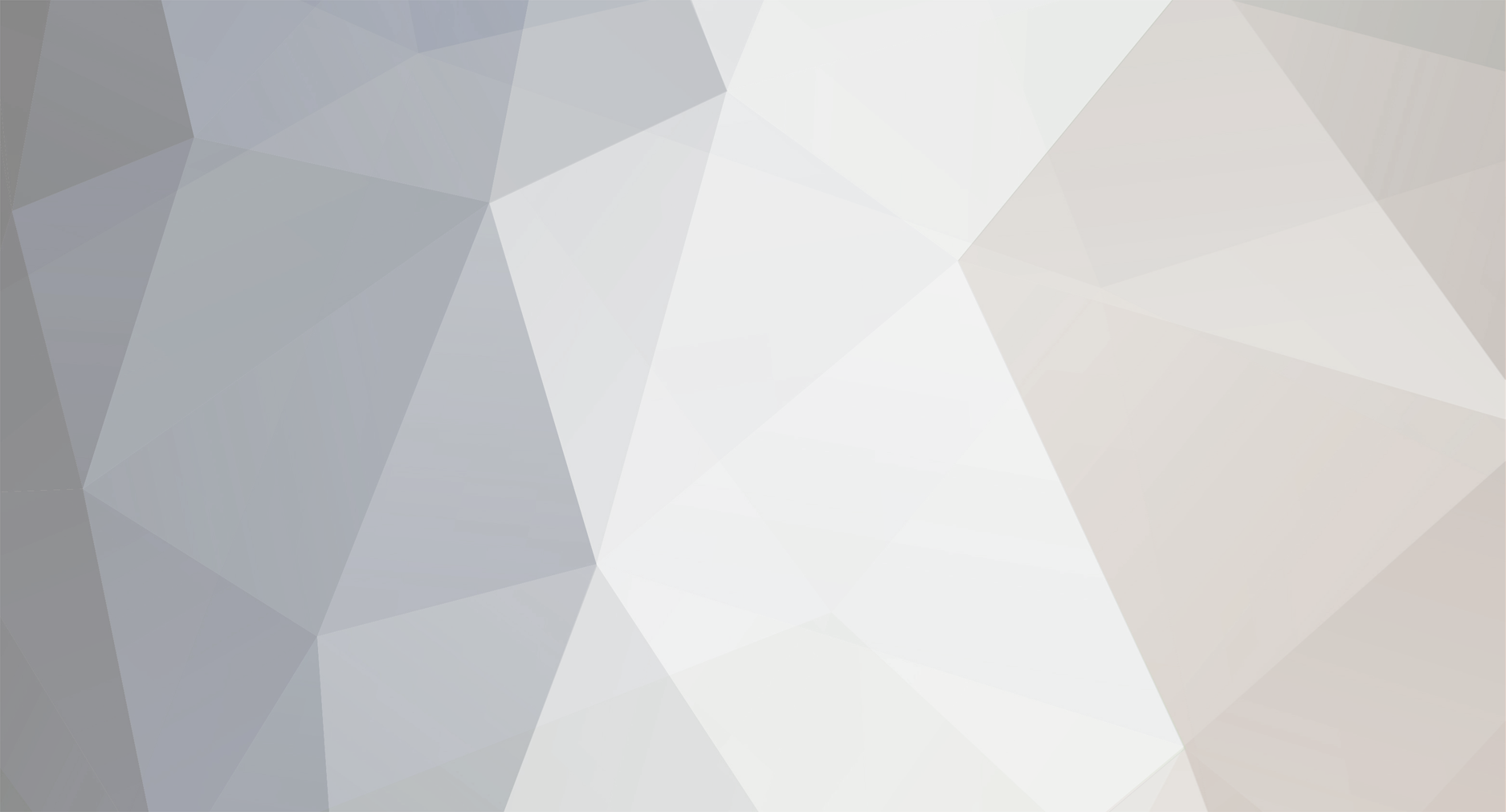
Psycho
Moderators-
Posts
12,159 -
Joined
-
Last visited
-
Days Won
130
Everything posted by Psycho
-
You should really post the source text in it's original form to get a proper solution. There might be line break characters or other data that may make the solution easier or more difficult. But, yes you can do it by extracting the data between two known values: $text = "194 WTNT32 KNHC 290231 TCPAT2 BULLETIN TROPICAL STORM GUSTAV ADVISORY NUMBER 17 NWS TPC/NATIONAL HURRICANE CENTER MIAMI FL AL072008 1100 PM EDT THU AUG 28 2008"; preg_match('/BULLETIN(.*)NWS/', $text, $matches); $advisory = trim($matches[1]); echo "Advisory: $advisory"; //Output: "Advisory: TROPICAL STORM GUSTAV ADVISORY NUMBER 17"
-
First thing you need to decide is what to do IF both are submitted. You can use JavaScript to try and limit the user, but that is not foolproof. So you can either 1) Not accept the input and redisplay the form or 2) Consider one as the primary and eaccept that. I will assume that if the user submitted both that the user really meant to submit a new value if (isset($_POST['new_category']) && trim($_POST['new_category'])!='') { $category = mysql_real_escape_string(trim($_POST['new_category'])); } else if (isset($_POST['category']) && trim($_POST['category'])!='') { $category = mysql_real_escape_string(trim($_POST['category'])); } else { $category = false; } if (!$category) { echo "No category submitted!"; } else { $query = "INSERT INTO dw_items (item_category) VALUES ('$category')"; //Run query }
-
A foreach() is used on things such as an array which has elements that the foreach() loop will iterrate through. You want a for() loop that willcontinue until a certain condition is met - in this case whern the counter reaces a certain limit, for ($counter=0; $counter<20; $counter++) { //Do comething 20 times } The first expression ($counter=0) is evaluated once (sets the value to 0) The 2nd expression is evaluated on each iterration - if it returns true the loop is run. If it returns false the loop ends. The 3rd expression is evaluated at the end of each iterration (increates the value of $counter by 1) EDIT: I see MasterACE14 has suggested using a while loop. It is valid, but I prefer a for() loop as you can see all the logic in one place - and with a while loop it is easier to forget the step of increasing the counter and you end up with an infinite loop. Your choice.
-
String is not evaluating correctly. Anyone help?
Psycho replied to AndreGuatemala's topic in PHP Coding Help
Are you even sure that TBL_MEMB is being evaluated correctly? What is the error you get??? I always create my queries as a string variable so I can echo them to the page if there is an error. $getTitle = urldecode(substr($_SERVER['REQUEST_URI'], 10)); $query = "SELECT id FROM ".TBL_MEMB." WHERE title='$getTitle'"; $realID = $db->get_var($query); You don't need to "break out" of the quotes for the $getTitle variable since you are using double quotes. -
A plain text file? //Path tot he file $file_name = 'directory/file.txt'; //Read fiel into an array $lines = file($file_name); //Extract the 6th line of text $sixth_line = $lines[5]; //Output the data echo $sixth_line; Although your example appears to have the data on line five - unless there is an empty line at the top.
-
What is the source of this text? If it is from an HTML page/file you could simply look for the underline tags. Or, is it from a rich text file, or what?
-
Here's a quick sample script showing one way this can be done. When you click the button it repopulates the list with a new set of random options from a list. So, there is a possibility that the value you have already selected will be in the new list. If it is, that value will be selected. If not, the list defaults to the first item in the list. <html> <head> <script type="text/javascript"> function changeSelect(selectField, newList, selectedVal) { selectField.options.length = 0; for (i=0; i<newList.length; i++) { selected = (selectedVal==newList[i]) ? true : false ; selectField.options[selectField.length] = new Option(newList[i], newList[i], selected, selected); } } function randArray(){ return (Math.round(Math.random())-0.5); } function changeColors() { //Array of all possible options colorArray = new Array('Blue','Green','Yellow','Orange','Red','Purple'); //Randomize Array colorArray.sort( randArray ); //Select first 5 options newOptions = colorArray.slice(0,4); selObj = document.getElementById('color'); currentSelected = selObj.options[selObj.selectedIndex].value; changeSelect(selObj, newOptions, currentSelected); } </script> </head> <body onload="changeColors();"> <form name="test" method="POST" action="processingpage.php"> Color: <select name="country" id="color"> <option></option> </select> <br> <button onclick="changeColors();">Change Colors In List</button> </form> </body> </html>
-
The data can only populate the form if you specifically write code for it to do so. However, I am going to make an assumption that you want the form to be populated with the POSTed data in the event there is a validation error. Well, I would still suggest writing your code such that the form is only re-populated when a validation fails, but a "quick fix" would be to use unset() unset($_POST);
-
True, but the OP stated Which would lead me to belive he was unsuccessful in doing so.
-
[SOLVED] Using readir and only store jpg in array
Psycho replied to ifubad's topic in PHP Coding Help
You could, but it would probably be easier to just check the last 4 characters to see if they equal '.jpg' if (str_to_lower(substr($filename, -4))=='.jpg') { echo "It's a jpeg!"; } else { echo "It's NOT a jpeg."; } -
It sounds as if you are wanting to display the select list with an option pre-selected based upon what waws saved int he database. You shouldn't be using AJAX for this. As Ken2k7 alluded to you should be using PHP for this. Example: //Create the options for the select list $colorOptions = array('Blue', 'Red', 'Green', 'Yellow'); //Query db for the saved value $query = "SELECT favorite_color FROM users WHERE user_id = $userID"; $result = mysql_query($query) or die(mysql_error()."<br>Query: $query"); //Extract saved value if (mysql_num_rows($result)) { $userData = mysql_fetch_assoc($result); } //Create select list and "select" the saved value echo "<select name=\"favoriteColor\">"; foreach($colorOptions as $color) { $selected = ($color == $userData['favorite_color']) ? ' selected="selected"' : '' ; echo "<option value=\"$color\"$selected>$color</option"; } echo "</select>";
-
Try replancing your assignment of the $search value with this: $search = (isset($_POST['search'])) ? $_POST['search'] : $_GET['search']; $search = mysql_real_escape_string($search);
-
And, you will be doing yourself a BIG favor if you were to create your queries as string variables so you can echo them to the page when they fail. The SQL error that is returned only contains part of the query making it difficult to debug. Example: $query = "select * from person where $type like '%$search%'"; $result = mysql_query($query) or die (mysql_error ()."<br>Query: $query");
-
I agree with Wildteen and Darkwater that you can convert the value to an integer and then just validate that it is greater than 0. However, in some instances changing the users entered value like that can be problematic and you should have them correct it themselves. The easiest solution for that (in my opinion) would be a simple regular expression check <?php if (!preg_match("/[^\d]/", $_POST['thevalue'])) { echo "Valid"; } else { echo "Not Valid"; } ?> The value must contain only numbers (0-9) and cannot contain decimals, negative signs, etc.
-
That is not enough to give you a solution. Somewhere in the script that value is used as a timer for hiding the submenu. you would need to modify the script to hide the sub-menu based upon the onclick trigger of another submenu.
-
The problem is that you are setting the disabled state to the STRING values "true" or "false" not the boolean values of true and false. Incorrect: document.forms[0].EF1.disabled="false"; Correct: document.forms[0].EF1.disabled=false; Also, you can cut down the amount of code needed a great deal with a little more logic. It appears than many "sections" are either being enabled or disabled completely. You could simply create a function to enable/diable those sections and call them accordingly. function disableBlock2(disableBool) { document.forms[0].B1A.disabled =disableBool; document.forms[0].B1B.disabled =disableBool; document.forms[0].B2A.disabled =disableBool; document.forms[0].B2B.disabled =disableBool; document.forms[0].uB1A.disabled=disableBool; document.forms[0].uB1B.disabled=disableBool; document.forms[0].uB2A.disabled=disableBool; document.forms[0].uB2B.disabled=disableBool; } function disableBlock4(disableBool) { document.forms[0].osmoseV.disabled=disableBool; document.forms[0].cediV.disabled =disableBool; document.forms[0].vf1V.disabled =disableBool; document.forms[0].vf2V.disabled =disableBool; document.forms[0].vf3V.disabled =disableBool; document.forms[0].vf4V.disabled =disableBool; document.forms[0].vf8V.disabled =disableBool; document.forms[0].vf10V.disabled =disableBool; document.forms[0].vf9V.disabled =disableBool; document.forms[0].vf11V.disabled =disableBool; } function disableBlock5(disableBool) { document.forms[0].osmoseL.disabled=disableBool; document.forms[0].cediL.disabled =disableBool; document.forms[0].vf1L.disabled =disableBool; document.forms[0].vf2L.disabled =disableBool; document.forms[0].vf3L.disabled =disableBool; document.forms[0].vf4L.disabled =disableBool; document.forms[0].vf8L.disabled =disableBool; document.forms[0].vf10L.disabled =disableBool; document.forms[0].vf9L.disabled =disableBool; document.forms[0].vf11L.disabled =disableBool; } function disableBlock6(disableBool) { document.forms[0].osmoseN.disabled=disableBool; document.forms[0].cediN.disabled =disableBool; document.forms[0].vf1N.disabled =disableBool; document.forms[0].vf2N.disabled =disableBool; document.forms[0].vf3N.disabled =disableBool; document.forms[0].vf4N.disabled =disableBool; document.forms[0].vf8N.disabled =disableBool; document.forms[0].vf10N.disabled =disableBool; document.forms[0].vf9N.disabled =disableBool; document.forms[0].vf11N.disabled =disableBool; } function checkploeg(){ var ploeg = document.forms[0].ploeg.options[document.forms[0].ploeg.selectedIndex].text; if(ploeg == "vroeg"){ document.forms[0].EF1.disabled=false; document.forms[0].EF2.disabled=false; disableBlock2(true); document.forms[0].DeNOx.disabled=false; document.forms[0].ammoniak.disabled=false; document.forms[0].H2Oput.disabled=true; document.forms[0].H2Otank.disabled=true; disableBlock4(false); disableBlock5(true); disableBlock6(true); disableBlock7(true); document.forms[0].effluent.disabled=true; document.forms[0].gastellerD.disabled=false; document.forms[0].gastellerA.disabled=false; document.forms[0].AWFR1.disabled=true; document.forms[0].AWFR2.disabled=true; document.forms[0].uurtellerA.disabled=true; document.forms[0].uurtellerF.disabled=true; document.forms[0].volgnummerV.disabled=false; document.forms[0].volgnummerL.disabled=true; document.forms[0].volgnummerN.disabled=true; } if(ploeg == "laat"){ document.forms[0].EF1.disabled=true; document.forms[0].EF2.disabled=true; disableBlock2(false); document.forms[0].DeNOx.disabled=true; document.forms[0].ammoniak.disabled=true; document.forms[0].H2Oput.disabled=false; document.forms[0].H2Otank.disabled=false; disableBlock4(true); disableBlock5(false); disableBlock6(true); document.forms[0].effluent.disabled=false; document.forms[0].gastellerD.disabled=true; document.forms[0].gastellerA.disabled=true; document.forms[0].AWFR1.disabled=false; document.forms[0].AWFR2.disabled=false; document.forms[0].uurtellerA.disabled=true; document.forms[0].uurtellerF.disabled=true; document.forms[0].volgnummerV.disabled=true; document.forms[0].volgnummerL.disabled=false; document.forms[0].volgnummerN.disabled=true; } if(ploeg == "nacht"){ document.forms[0].EF1.disabled=true; document.forms[0].EF2.disabled=true; disableBlock2(true); document.forms[0].DeNOx.disabled=true; document.forms[0].ammoniak.disabled=true; document.forms[0].H2Oput.disabled=true; document.forms[0].H2Otank.disabled=true; disableBlock4(true); disableBlock5(true); disableBlock6(false); document.forms[0].effluent.disabled=true; document.forms[0].gastellerD.disabled=true; document.forms[0].gastellerA.disabled=true; document.forms[0].AWFR1.disabled=true; document.forms[0].AWFR2.disabled=true; document.forms[0].uurtellerA.disabled=false; document.forms[0].uurtellerF.disabled=false; document.forms[0].volgnummerV.disabled=true; document.forms[0].volgnummerL.disabled=true; document.forms[0].volgnummerN.disabled=false; } return true; }
-
No offense guys, but there are flaws in both of those scripts. In the first one the resizing on the Y axis is only done to maintain the ratio from the x axis resizing - there is no resizing to ensure the image fits in the 600 height limit. The 2nd function has a similar problem. If the image is just over the width restriction it will only resize based on the X axis even though the Y axis may be way above the height restriction - resulting ina an image that is still greater than 600 in height. I would also create a function that takes the height limit as a parameter so it will be reusable: <?php class ImageResize{ function resize($width, $height, $width_limit, $height_limit=600){ if($width > $width_limit || $height > $height_limit) { //Image is too big - need to resize $scale = ($width / $height); //Determine on which axis to resize on if ( ($width/$width_limit) > ($height/$height_limit) ) { //Scale on width $width = $width_limit; $height = round($width_limit/$scale); } else { //Scale on height $width = round($height_limit/$scale); $height = $height_limit; } } echo "width = '$width' height = '$height'"; } } ?>
-
That's not quite how I would implement it. If the user enters a value in a form and a value is not appropriate I typically will display an error message and presentt he user with the form with the previously entered values. With regard to $_POST['trainamount'] can it be any number (e.g. 2, 3.1459, -3, etc.)? Based upon the text I would assume it needs to be a positive integer (1, 2, 3, etc.). This has not been checked for errors and I didn't spend too much time validating the logic <?php include("templates/private_header.php"); $errors = false; if (isset($_POST['train'])) { //Validate the user entered values if (!is_int($_POST['trainamount']) || is_int($_POST['trainamount'])<1) { $errors[] = "Train amount must be a positive integer"; } if (!in_array($_POST['train'], array('Strength', 'Speed', 'Defense')) { $errors[] = "Training area is not a valid value"; } } if (isset($_POST['train'] && $errors==false) { $statupdate=($player->energy*$player->Awake)/100; $awakereduce=($player->Awake-5); $energyreduce=($_POST['trainamount']); //Check that player has Awake Left if ($player->Awake <= 0) { echo "You are too tired to train."; } //Check that player has energy Left else if ($player->energy <=0) { echo "You have no energy left"; } //check that player has paid taxes else if ($player->Taxes_Owed >0) { echo "<strong>You Deadbeat!</strong> <br>We already told you. You have to pay your property taxes before you can use our gym.<p>"; echo "You didn't think we knew that kind of stuff about you, did ya!<br>"; echo "Pay your taxes or we'll also put that video of you and the goat on youtube"; } //Perform the training else { switch($_POST["train"]) { case "Strength": $updatestrength = $db->execute("UPDATE `players` SET `strength`= `strength`+?, `Awake`=?, `energy`= `energy`-? WHERE `id`=?", array($statupdate, $awakereduce, $energyreduce, $player->id)); break; case "Speed": $updatestrength = $db->execute("UPDATE `players` SET `agility`= `agility`+?, `Awake`=?, `energy`= `energy`-? WHERE `id`=?", array($statupdate, $awakereduce, $energyreduce, $player->id)); break; case "Defense": $updatestrength = $db->execute("UPDATE `players` SET `vitality`= `vitality`+?, `Awake`=?, `energy`= `energy`-? WHERE `id`=?", array($statupdate, $awakereduce, $energyreduce, $player->id)); break; } echo "You just trained\n" . $energyreduce . "\ntimes" ; echo "Your stats are<p>"; $newstats=$db->execute("Select `strength`, `agility`, `vitality` from players Where `id`=?", array($player->id)); while ($newstats1 = $newstats->fetchrow()) { echo "Strength\n" . $newstats1['strength'] . "<p>"; echo "Defense\n" . $newstats1['vitality'] . "<p>"; echo "Speed\n" . $newstats1['agility'] . "<p>"; echo "Total Stats:\n" . ($newstats1['strength']+$newstats1['agility']+$newstats1['vitality']) . "<br>"; } } } else { echo "<h3>Welcome to the CAC World Gym!</h3><br>If you're a homeowner in our lovely city, all of our equipment is yours to use <strong>FREE!</strong><p>"; echo "The city just requires that you have all your property taxes paid in full<br>"; echo "You currently owe\n$<strong>" . $player->Taxes_Owed . "\n</strong>in taxes<p>"; echo "You can currently train\n<strong>" . $player->energy . "</strong>\ntimes<p>"; echo "<strong>How many times would you like to train</strong><p>"; if ($errors) { echo "The following errors occured:"; echo "<ul><li>" . implode('<li></li>', $errors) . '</li></ul>'; } echo "<form method=\"post\" action=\"gym.php\">"; echo "<input type=\"text\" name=\"trainamount\" value=\"$player->energy\"/><p> "; echo "<strong>What area would you like to work on?</strong><br>"; echo "<select name=\"train\"\n"; echo "<option value=\"Strength\">Strength</option>\n"; echo "<option value=\"Speed\">Speed</option>\n"; echo "<option value=\"Defense\">Defense</option\n"; echo "<input type=\"submit\" value=\"Train\" />\n"; } include("templates/private_footer.php"); ?>
-
how to disable textbox if the checkbox is not checked yet
Psycho replied to fredowinz23's topic in Javascript Help
That script is not complete. It will enable the text field when the checkbox is first checked, but will not disable the field when it is unchecked. I would also add functionality to clear the value in the text field when the checkbox is unchecked. Try this: <html> <head> <script type="text/javascript"> function enableText(checkBool, textID) { textFldObj = document.getElementById(textID); //Disable the text field textFldObj.disabled = !checkBool; //Clear value in the text field if (!checkBool) { textFldObj.value = ''; } } </script> </head> <body> <input id="myCheckBox" type="checkbox" onclick="enableText(this.checked, 'myTextArea');" /> <br /> <input id="myTextArea" type="text" disabled="disabled" /> </body> </html> -
[SOLVED] get the name of the column from a SQL database ?
Psycho replied to lonewolf217's topic in PHP Coding Help
mysql_fetch_field() http://us.php.net/manual/en/function.mysql-fetch-field.php -
Here is a class that allows youto work with excel files: http://phpclasses.ca/browse/package/86.html
-
I'm not sure what to tell you. The function rteCommand is someting outside of that function. That is most likely what is causing your problem. But, if I comment those lines out the function works fine. You need to look at your function for rteCommand() for the problem. <html> <head> <script type="text/javascript"> function insertLink(rte) { //function to insert link var linkt = prompt("Enter link text",""); var szURL = prompt("Enter a URL:", ""); try { //ignore error for blank urls // rteCommand(rte, "Unlink", null); document.getElementById(rte).value = linkt; // rteCommand(rte, "CreateLink", szURL); } catch (e) { //do nothing } } </script> </head> <body> Link output field: <input type="text" id="linkOutput" /> <br /><br /> <button onclick="insertLink('linkOutput');">Click for Prompt</button> </body> </html>
-
Although mysql_real_escape_string() will handle possible SQL injection, I would not say it is the only validation that would need to be done. With respect to the code you have above here are a few other things I would do: The value for $_POST['trainamount'] should be a number so you should add validation for that. It doesn't appear you are doing any math on this value, but that is always a concern as a non-number or a zero value can cause unrecoverable errors. For $_POST["train"] you are expecting a value from a fixed list. You should validate that the posted value is from that list. You should always, always assume the user is passing bad data and perform the necessary validations and have the proper error/warning conditions in place.