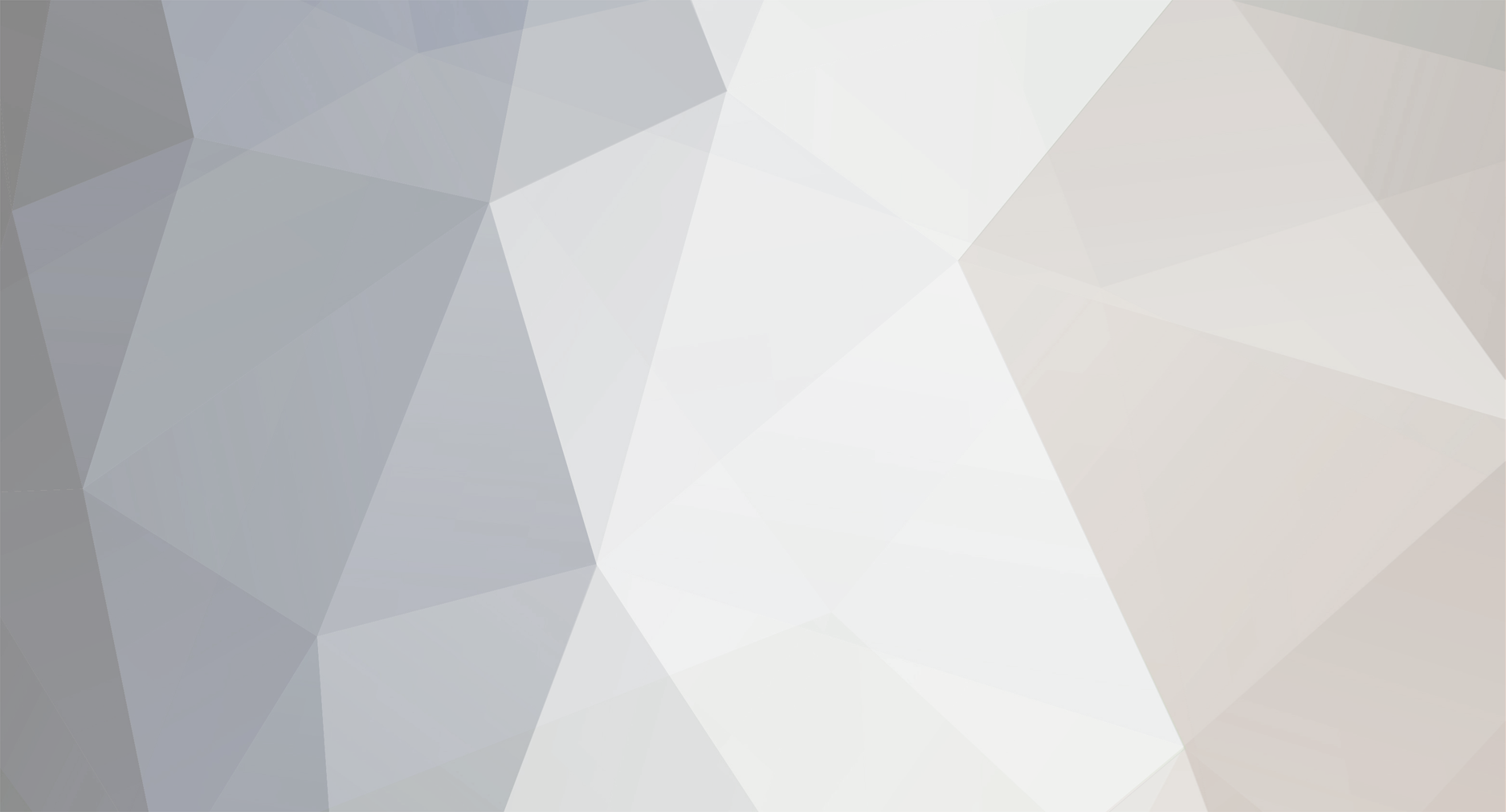
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Created right from the example in the manual for array_multisort(): http://us2.php.net/manual/en/function.array-multisort.php Assuming $data_array is your array: <?php // Create an array of just the mod values foreach ($data_array as $key => $row) { $mod[$key] = $row['mod']; } // Sort the data with mod ascending. Add $data_array // as the last parameter, to sort by the common key array_multisort($mod, SORT_ASC, $data_array); ?> Or you could also use usort(): http://us2.php.net/manual/en/function.usort.php <?php function sort_by_mod($a, $b) { if ($a['mod'] == $b['mod']) { return 0; } return ($a['mod'] < $b['mod']) ? -1 : 1; } usort($data_array, 'sort_by_mod'); ?>
-
[SOLVED] Code Cleaning... Giving me headaches
Psycho replied to monkeypaw201's topic in PHP Coding Help
As I said, I'm sure there are typos. Did you look at the source generated to see what might be wrong with the alert code? The problem was due to the \n's being interpteted in the PHP code and not being available in the javascript. There are two simple fixes. 1) Change the strings with \n's to be created with single-quotes, so the won't be interpreted, or 2) use \\n in the PHP strings and they will be created as \n in the Javavascript. This code will fix the issue: $onclick = "alert('"; $onclick .= 'Sorry, It looks like you are not meeting all the requirements to fly this route.'; $onclick .= 'The missing requirements are listed below. \n\n- ' . $qualified . '\n'; $onclick .= "');"; -
[SOLVED] Code Cleaning... Giving me headaches
Psycho replied to monkeypaw201's topic in PHP Coding Help
Well, you still didn't account for the problem regarding the broken logic around the international else clause - which I commented out in the code I posted. The code I posted that replaces much of your previous logic does exactly what you described. If the pilot's rank is greater than or equal to the aircraft rank, then they will link to 'file', if not they will link to 'details'. Anyway, based upon my understanding of your problem this is how I would do it (I'm sure there are some minor typos). There may be some slight logic errors based upon misunderstanding of the logic needed - which wouold just need some minor corrections. But, this presents a much cleaner implementation of what you need. I think it is always best to separate the logic from the presentation (i.e. don't put a lot of IF/ELSE statements within echo's. <?php if(isset($_SESSION['pilot_id'])) { $pilot = mysql_query("SELECT * FROM `users` WHERE `pilot_id` = '$_SESSION[pilot_id]'"); $row_pilot = mysql_fetch_array($pilot); $ranks = array('Regional', 'Short Haul', 'Medium Haul', 'Long Haul'); $pilot_rank = array_search($row_pilot['rank'], $ranks); $aircraft_rank = array_search($row_aircraft['rank'], $ranks); //Set qualified based upon aircraft/pilot match $qualified = ($pilot_rank >= $aircraft_rank)?true:'You Do Not Have A High Enough Rank'; //Check add'l qualification if intn'l if($row_route['category'] == "International") { $qualified = ($row_pilot['international']=="Yes")?true:'You Are Not Certified For International Flights'; $intl = true; } } else { $qualified = 'You Must Be Logged In'; } //Create variables for href and onlclick content if($qualified===true) { $href = "file.php?id={$row_timetable['id']}"; $onclick = "javascript:return confirm('Are you sure you want to place a bid on this flight?');"; } else { $href = "details.php?id={$_GET['id']}"; $onclick = "alert('"; $onclick .= "Sorry, It looks like you are not meeting all the requirements to fly this route.\n"; $onclick .= "The missing requirements are listed below. \n\n- {$qualified}\n"; $onclick .= "');"; } echo "<td>\n"; echo "<a href=\"$href\" onclick=\"{$onclick}\">"; echo "<img src=\"http://www.cormorantair.com/images/pirep.jpg\" hieght=\"15\" width=\"15\">"; echo "</a>\n"; echo "</td>\n"; ?> Oh yeah, you should really add some validation around any user input (e.g. GET['id'], and $_SESSION[pilot_id] if you don't validate before saving it) -
[SOLVED] Code Cleaning... Giving me headaches
Psycho replied to monkeypaw201's topic in PHP Coding Help
Well, first off the third elseif is unnecessary since that condition wouold be covered in the else if right before it. I *think* maybe the first part of that clause was to be Long Haul??? I also see another problem that I'm not sure what you meant to do. Int he long line of if/else's you set a value for the variable $file. But then before you use that variable you have another if/else for international in which you always reset the value of $file. So all the preceeding logic is wasted. You didn't explain any of the logic behind the code, but from looking at it I am assuming that there are four different values for aircraft and pilot (Regional, Short Haul, Medium Haul & Long haul). Also, if the pilot's rank is >= the aircraft rank then you set $file = "file"; and $rankreq = "1";. based upon that there is a much easier method. There are probably other changes I would make, but again I don't know all the issues around the logic: <?php if(isset($_SESSION['pilot_id'])) { $pilot = mysql_query("SELECT * FROM `users` WHERE `pilot_id` = '$_SESSION[pilot_id]'"); $row_pilot = mysql_fetch_array($pilot); $ranks = array('Regional', 'Short Haul', 'Medium Haul', 'Long Haul'); if (array_search($row_pilot['rank'], $ranks) >= array_search($row_aircraft['rank'], $ranks)) { $file = "file"; $rankreq = "1"; } else { $file = "details"; $rankreq = "0"; } if($row_route['category'] == "International") { if($row_pilot['international'] == "Yes") { $file = "file"; }else{ $file = "details"; $rankintl = "0"; } } // This logic always overwrites the first set of conditions ??? // else // { // $file = "details"; // } } else { $file = "details"; } ?> I would probably create an array with 0=>'details' and 1=>'file' instead of setting two values as they always seem to be in tandem. -
Because upon the first validation you are returning true. Don't "return" until you want to exit the function. function validate() { if(!document.form2.client.value) { alert('Fill in the Username before submitting'); return false; } if(!document.form2.project.value) { alert('Fill in the Password before submitting'); return false; } return true; }
-
Should the date really be associated with the items in the order, or just with the order. In other words, can you have items in a single order that are from two dates? You should probably have two different tables one for the orders and one for the items in the orders, but anyway... <?php $query = "SELECT * FROM table ORDER BY order_number, date"; $result = mysql_query($query) or die (mysql_error()); $current_order = ''; echo "<table>\n"; while ($record = mysql_fetch_assoc($result)) { //If first record for a new order display the header rows if ($current_order!=$record['order_number']) { $current_order = $record['order_number']; echo "<tr>\n"; echo "<td colspan=\"4\"><b>Order# $current_order:</b></td>\n"; echo "<td> </td><td>Item ID</td><td>Quantity</td><td>Date</td>\n"; echo "</tr>\n"; } //Display the record data echo "<tr>\n"; echo "<td> </td>\n"; echo "<td>" . $record['item_id'] . "</td>\n"; echo "<td>" . $record['quantity'] . "</td>\n"; echo "<td>" . $record['date'] . "</td>\n"; echo "</tr>\n"; } echo "</table>\n"; ?>
-
[SOLVED] Bug or simple solution?? Driving me crazy...
Psycho replied to lindm's topic in Javascript Help
Offhand I coundn't determine why it didn't work and didn't want to invest a lot of time since I thought there was a more efficinet way to accomplish the objective. -
[SOLVED] Bug or simple solution?? Driving me crazy...
Psycho replied to lindm's topic in Javascript Help
Why make it so difficult using parent.parent... Simply give an ID to each element and pass it in the function. Then you only need one function and it becomes much simpler. <html> <head> <style type="text/css" media="screen,print"> .checked {background-color: #F1F1F1;} </style> <script type="text/javascript"> function pl(fieldObj, elemID) { document.getElementById(elemID).className = (fieldObj.checked)?'checked':''; } </script> </head> <body> <form name="form1" method="post" action=""> <table border="1" cellspacing="0" cellpadding="0" class="" id="tbl1"> <tr> <td width="355">Tableclass 1 - not checked and no class. Works fine.</td> <td width="120"> </td> <td width="120"> </td> <td width="25"> <input type="checkbox" name="checkbox" id="checkbox" onclick="pl(this, 'tbl1')"> </td> </tr> <tr> <td> </td> <td> </td> <td> </td> <td> </td> </tr> </table> <br> <table border="1" cellspacing="0" cellpadding="0" class="checked" id="tbl2"> <tr> <td width="355">Tableclass 2 - checked and class set.</td> <td width="120"> </td> <td width="120"> </td> <td width="25"> <input name="checkbox" type="checkbox" id="checkbox" onclick="pl(this, 'tbl2')" checked> </td> </tr> <tr> <td><strong>PROBLEM!</strong> Why does not the background change??</td> <td> </td> <td> </td> <td> </td> </tr> </table> <br> <table border="1" cellspacing="0" cellpadding="0"> <tr class="" id="row1"> <td width="355">Rowclass 1 - not checked and no class. Works fine.</td> <td width="120"> </td> <td width="120"> </td> <td width="25"> <input type="checkbox" name="checkbox2" id="checkbox2" onclick="pl(this, 'row1')"> </td> </tr> <tr> <td> </td> <td> </td> <td> </td> <td> </td> </tr> </table> <br> <table border="1" cellspacing="0" cellpadding="0"> <tr class="checked" id="row2"> <td width="355">Row class 2 - checked and class set. Works fine.</td> <td width="120"> </td> <td width="120"> </td> <td width="25"> <input name="checkbox2" type="checkbox" id="checkbox2" onclick="pl(this, 'row2')" checked> </td> </tr> <tr> <td> </td> <td> </td> <td> </td> <td> </td> </tr> </table> <br> </form> </body> </html> -
It depends on WHAT you are really trying to test. For example this: $num2 = "0"; is really a string. Also, your results above show that this: $num5 = true; returns true for being a number. That doesn't look correct to me. Also your function doesn't take into account numbers that are not integers (e.g. 1.5). There are many built in functions for testing if a variable is a type of number: is_numeric() is_int() is_float() etc.
-
[SOLVED] decimal place formating within a table? help please
Psycho replied to HelpMe1985's topic in PHP Coding Help
echo "<td><center>£" . number_format($row['WeeklyCharge'], 2) . "</td>"; -
[SOLVED] possible OOP scope issue? - AJAX wrapper
Psycho replied to PC Nerd's topic in Javascript Help
Sorry, but I am no following you last question. After looking at the full code, I think the problem is still as I stated (perhaps poorly) before. When you call runQuery() that function makes the call to the server-side page. Then the very next line calls getData() to get the output of that request. The problem, I beleive, is that the time for the server call and response take more time than it takes before you run the getData() call. Let's say it take 1/10 of a second for the server call and response to complete. It probably take 1/100 of a second for the code to move from the runQuery() call to the getData() call. Thus, you are always getting the results of the last query - which is why the first one returns no data. I made an attempt at a quick modification that might work (have not tested it). Here is the basic concept: 1) Whenever you call runQuery it will set the object property this.information to false. 2) Then when the saveData function is run it will set this.information to the AJAX output. 3) When you call getData it will loop until this.information is not false. This will give the AJAX process time to complete before getData tries to return the value. I added two lines (both with // ***** NEW LINE *****)I don't consider this an efficinet solution due to the loop. Using onreadystatechange to trigger the response is better, but it would take longer for me to rewrite for that. function AJAX() { var self = this; var xmlProccessor; var xmlObj; var xmlProccessor; var proccessTag; var information; try { self.xmlObj = new XMLHttpRequest(); } catch(e) { try { self.xmlObj = new ActiveXObject("Msxml2.XMLHTTP"); } catch(e) { try { self.xmlObj = new ActiveXObject("Microsoft.XMLHTTP"); } catch(e) { alert("AJAX is not enabled in your browser. To complete this form you need to have full JavaScript capabilities enabled. Please email webmaster@battleages.com for more information."); return false; } } } this.xmlObj.onreadystatechange = function() { if(self.statusalert == true) { if(self.xmlObj.readyState == 0) { self.statusobject.innerHTML = "AJAX"; } else if(self.xmlObj.readyState == 2) { self.statusobject.innerHTML = "Request sent."; } else if(self.xmlObj.readyState == 3) { self.statusobject.innerHTML = "Receiving data."; } else if(self.xmlObj.readyState == 4) { self.statusobject.innerHTML = "Proccessing data."; } } if(self.xmlObj.readyState == 4) { self.xmlProccessor = new XML(self.xmlObj.responseXML); v = self.saveData(self.xmlProccessor.proccessOn(self.proccessTag)); //alert("V: "+v); if(v != true) { alert("Could not save data"); } else { //alert("Data Saved:\n\n "+self.information +"\n\n" + "Type: "+typeof self.information); } } } } AJAX.prototype.saveData = function(h) { this.information = h; if(this.information) { return true; } else {return false;} } AJAX.prototype.setStatusAlerts = function(a, b) { if(a == 1) { this.statusalert = true; this.statusobject = b; } else {this.statusalert = false;} } AJAX.prototype.runQuery = function(url, sendData, xmlTagName) { this.information = false // ***** NEW LINE ***** this.proccessTag = xmlTagName; this.xmlObj.open("POST", url+"?"+sendData); this.xmlObj.send(null); //xmlObj.send(sendData); //alert("Data: "+this.data); } AJAX.prototype.getData = function() { while (!this.information) {} // ***** NEW LINE ***** alert(this.information); if(this.information) { return(this.information); } else { return "EMPTY"; } } -
[SOLVED] possible OOP scope issue? - AJAX wrapper
Psycho replied to PC Nerd's topic in Javascript Help
Wihtout seeing all the code I can't be sure, but I think the problem is because you are not waiting for a status change. With AJAX there will be a delay between the time that the call is made and when the response is available. That delay can be very quick (a fraction of a second) to very long (many seconds). However you are calling getData right after runQuery and not giving it any time to do the query and return the data. So, when you say "my getData function only returns the data if i have previously gone" it is most likely returning data from the previous call. I just built an AJAX class for my own purposes (it's on my home PC so don't have access to it at the moment), but you will need to inject a delay to test for onreadystatechange. One option would be to modify the runQuery function to return the output once it is received and not use the getData. -
function validString(string) { var invalidChars = new RegExp(/[^\w ]/); return !invalidChars.test(string); } It's easier to check for invalid characters than to test for valid characters. \w = Match any alphanumeric character including the underscore. Equivalent to [a-zA-Z0-9_]. So using that and adding a space check for any character that is not in the approved "white" list.
-
The code I provided requires you to pass the ID of the field to move to. You didn't set IDs for the fields: <td>Home Phone: <td> <input type="hidden" name="homephone" id="homephone" /> (<input type="text" name="hphone1" size="3" maxlength="3" onkeyup="moveNext(this, 'hphone2');">)-<input type="text" name="hphone2" id="hphone2" size="3" maxlength="3" onkeyup="moveNext(this, 'hphone3');">-<input type="text" name="hphone3" id="hphone3" size="4" maxlength="4" onkeyup=""></td> </tr>
-
<html> <head> <script type="text/javascript"> function moveNext(fieldObj, nextFieldID) { if (fieldObj.value.length >= fieldObj.maxLength) { document.getElementById(nextFieldID).focus(); } } </script> </head> <body> Phone: (<input type="text" maxlength="3" size="2" id="area_code" name="area_code" onkeyup="moveNext(this, 'prefix');">) <input type="text" maxlength="3" size="2" id="prefix" name="prefix" onkeyup="moveNext(this, 'phone');"> - <input type="text" maxlength="4" size="3" id="phone" name="phone" onkeyup=""><br> </body> </html>
-
Adam, I'm not mening to bash you, but that is the exact opposite of how you should perform validation. The reason is that if the user does not have JS enabled they are unable to use the form at all. You should ALWAYS be validating on the server-side anyway. But, having validation client-side is a nice feature to have as well. You just don't weant to make your site unusable for non-js users. You can elegantly support both with the following method. Use a standard submit button, and in the FORM tag include validation through an onsubmit trigger such as onsubmit="return validate()". The validate() function should return false if validation fails and the form won't be submitted. That way if a user has JS enabled they will get the benefit of client-side validation. However, if a user does not have JS enabled the form will still submit and any errors will be taken care of in server-side validation.
-
You're going to need to provide a better explanation of what you want. Are you wanting to have the form "auto-tab" from one field to another (based upon what?), are you wanting to convert 8001234567 to 800-123-4567, or what?
-
Formula for redirecting users to a page 30% of the time
Psycho replied to katlis's topic in PHP Coding Help
I agree with the above comments as well. That method will result in 30% ratio in the long run. But, since you are tracking in a database and don't feel that is sufficinet, here is another method. When the page with the link in question loads, run a short script to get the percentage of times url 1 vs url 2 have been visited and set the link accordingly. This example makes several assumptions as I do not know the structure of your tables. <?php //Query the link hits by URL $query = "SELECT count(*) FROM table GROUP BY url ORDER BY url"; $result = mysql_query($query) or die (mysql_error()); //Set the count for each url from the query results //Assumes url1 comes before url2 in the results $url1_count = mysql_result($result, 0); $url2_count = mysql_result($result, 1); //Set the url for this page load $this_url = (($url1_count/$url2_count)<2.33)?'url1.htm':'url2.htm'; //Create the link echo "<a href=\"$this_url\">The Link</a>"; ?> If you are wondering why I used 2.33, it is mathmetically the equivalent to taking the count of link 2 and dividing by the total of link 1 and link 2. It is more efficient doing it that way as it involves less math operations (one division vs an addition and a division). Ex: Link 1 = 7 hits, link 2 = 3 hits 3 / (7 + 3) = .30 7 / 3 = 2.33 (repeating) -
[SOLVED] JavaScript and multidimensional arrays
Psycho replied to rhyspaterson's topic in Javascript Help
Here you go: var floor_array = [ ["ground", "Printer Status - Ground Floor", "Ground Floor"], ["one", "Printer Status - Level One", "First Floor"], ["two", "Printer Status - Level Two", "Second Floor"], ["three", "Printer Status - Level Three", "Thrid Floor"], ["four", "Printer Status - Level Four", "Fourth Floor"] ]; -
If I am understanding you correctly you are wanting to make a javascript call to a page which has JavaScript in it. I guess there are two possibilities on how you want that code run. 1) Have the code run in the external page to modify the results before being returned to the calling page. This makes little sense to me since you could do everything within the server-side code. Besides it won't work as the page is never actually loaded into the browser - and it is the browser that runs JavaScript. 2) You want to return some JavaScript tot he calling page to be used in that page. This is possible, but without more information on how you are implementing it I can't suggest much of a solution. There's alwasy the eval() function, but that's always a poor choice in my opinion.
-
The function before verify(), quick_date(), does not have a closing bracket. Add the closing bracket and it will work.
-
Yes, there is a foreach loop in PHP. But, for this you would want to use a while loop for displaying records from a database query. Example: <?php $query = "SELCT * FROM table ORDER BY dateposted"; $result = mysql_query($query) or die (mysql_error()); while ($post = mysql_fetch_assoc($result)) { echo $post['title'] . "<br>\n"; echo $post['content'] . "<br>\n"; echo "Posted: " . $post['dateposted'] . "<br>\n"; } ?>
-
Well, it worked for me. Of course, I had to strip out a lot of that code because you posted PHP code and I don't have the backend data to support it. When posting a JavaScript problem it is better, IMHO, if you only post the output - not the source data.
-
limit checkboxes in a form - boxes all differnent names
Psycho replied to scotchegg78's topic in Javascript Help
Well, I would first put the checkboxes in a div to group them. That way you can have other checkboxes on the form that are not part of the max count. Second, you could just disable the remaining checkboxes once the user hits the max count. <html> <head> <script type="text/javascript"> function setItems(checkObj) { var checkCount = 0; var maxChecks = 3; divObj = document.getElementById("checkGroup"); //Determine the number of checked boxes for (var i=0; i<divObj.childNodes.length; i++) { if (divObj.childNodes[i].type=='checkbox' && divObj.childNodes[i].checked) { checkCount++; } } //Enable/disable unchecked boxes for (var i=0; i<divObj.childNodes.length; i++) { if (divObj.childNodes[i].type=='checkbox' && !divObj.childNodes[i].checked) { divObj.childNodes[i].disabled = (checkCount==maxChecks); } } } </script> </head> <body> <form name=\"topfriends\"> <div id="checkGroup"> 1 <input type="checkbox" name="1" onclick="setItems(this.form);"><br> 2 <input type="checkbox" name="2" onclick="setItems(this.form);"><br> 3 <input type="checkbox" name="3" onclick="setItems(this.form);"><br> 4 <input type="checkbox" name="4" onclick="setItems(this.form);"><br> 5 <input type="checkbox" name="5" onclick="setItems(this.form);"><br> </div> </form> </body> </html> -
Extracting data from Multi dimensional array
Psycho replied to williamZanelli's topic in PHP Coding Help
Well a tpl file would lead me to believe it is a template. But, PHP doesn't care what a file's extension is. I will "assume" that the template file is being read/processed in a specific manner such that code is not parsed. Without knowing how the tpl file is used there is no way for me to provide any furhter assistance.