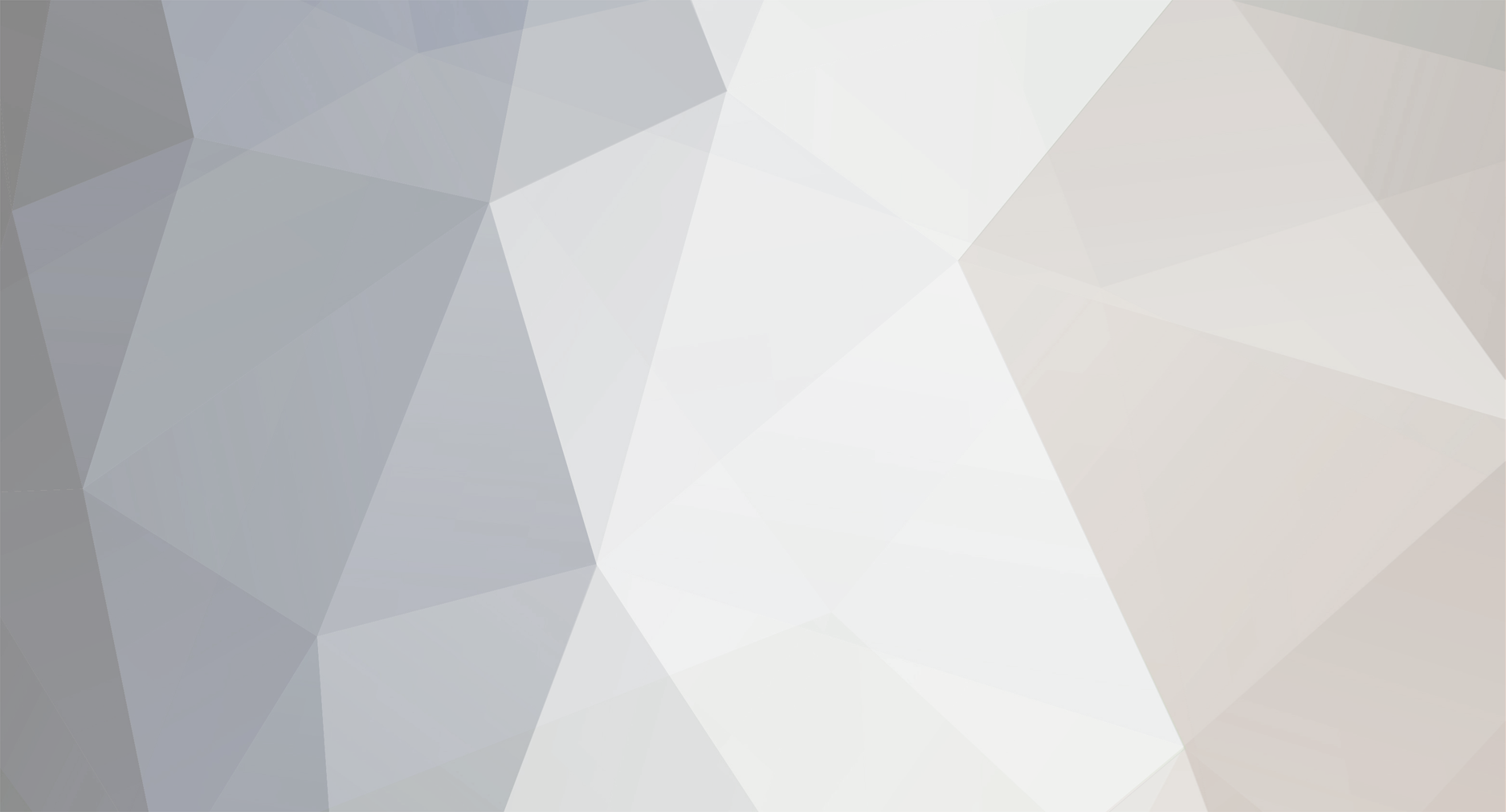
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
I saw your previous post the other day, and was going to respond. But the fact that you have an option group label in your select list complicates things. Is that really necessary if you are able to repopulate the list?
-
Just create a custom function: <?php function array_slice_from_index ($array, $index_value, $preserve_keys=false) { $return_array = array(); foreach ($array as $key => $value) { if ($key>=$index_value) { $new_key = ($preserve_keys)?$key:count($return_array); $return_array[$key] = $value; } } return $return_array; } ?> Use the perserve_keys parameter as needed.
-
Dude, this is a forum. I don't run an RSS feed to my computer 24/7 to answer a question the moment it is posted. Have you tried any debugging processes? move_uploaded_file() will return false if the file it is trying to upload is not valid. I would start will adding some debugging there. Change this move_uploaded_file($tempName, "upload/" . $fileName); To this if (move_uploaded_file($tempName, "upload/" . $fileName)) { echo "Stored in: upload/{$fileName}<br />"; } else { echo "Error uploading file {$tempName}<br />"; } Im guessing that the $tempfile name and location are not correct because of this: $tmpName = $_FILES['file']['tmpName']; Try this $tmpName = $_FILES['file']['tmp_name']; The reason your original code worked was that you never used the variables you created! You created the $tmpName variable, but then in the move_uploaded_file() function you used $_FILES["file"]["tmp_name"]. Why create the variable if you are not going to use it? This is just some constructive criticism, but you really need to plan out your code. As I stated about the HTML there were a lot of orphan tags, there are PHP variables that were created and not used, etc. As the programmer you need to know exactly what variables are created and where they are used.
-
How to distinguish between mouse click and dbl click
Psycho replied to praveenhotha's topic in Javascript Help
Yes I did. It works fine in IE, but not in FF (after further review). It was intended as a POC, not a final product. The logic is sound (the same as you proposed), just a small issue in the way it was interpreted. Apparently FF interprests a double click as 1-double click and 2-single clicks, whereas IE only interprest the first click as a single click and the subsequent click as a double only. Here is a slight modification that works in both IE & FF. I'm sure it could still be improved. <script type="text/javascript"> var clickedTwice = false; function clickOnce(firstRun) { if (firstRun) { document.getElementById('twice').innerHTML = ''; document.getElementById('once').innerHTML = ''; setTimeout('clickOnce(false)', 200); } else { if (!clickedTwice) { //Run the function normally document.getElementById('once').innerHTML = 'true'; document.getElementById('twice').innerHTML = 'false'; } } } function clickTwice() { clickedTwice = true; document.getElementById('twice').innerHTML = 'true'; document.getElementById('once').innerHTML = 'false'; setTimeout('resetClcikTwice()', 500); } function resetClcikTwice() { clickedTwice = false; } </script> -
Let's start with the form. Your first file element has the name "file" and the rest are "file2", "file3", etc. The first one should be called "file1". As I stated previously you should use a loop to create the form fields instead of the copy-paste method of programming. Your HTML has a lot of errors as well - many opening tags with no closing tags and vice-versa. You have a MAX_FILE_SIZE hidden filed, but it is not used anywhere. If you do want to use it you either need to declare it one, or if you need different values for each file, then you need to append a number to each one. No offense, but it is this kind of slopiness that makes it difficult to solve otherwise simple problems. Try this for your form: <form action="upload_file.php" method="POST" enctype="multipart/form-data"> <blockquote> <p align="center"> </p> <p> <label><strong>Id:</strong> <input name="id" type="text" id="id" size="2" maxlength="2"> </label> (Pick id number 1 through 10) <p><label><b>Make:</b></label> <input type="text" name="make" id="make" /></p> <p><label><b>Price:</b></label> <input type="text" name="price" id="price" /></p> <p><label></label> <label><b>Description:</b></label> <textarea name="description" id="description" cols="45" rows="5"></textarea /> </p> <?php for ($imgNum=1; $imgNum<=10; $imgNum++) { echo "<p><label for=\"file{$imgNum}\"><strong>Filename:</strong></label>\n"; echo "<input type=\"file\" name=\"file{$imgNum}\" id=\"file{$imgNum}\" />\n"; echo "<strong>(Note pictures must be sized to 182 X 124 pixels)</strong></p>\n"; } ?> <div align="center"><br /> <input type="submit" name="submit" value="Save" /> </p> </div> </form>
-
How to distinguish between mouse click and dbl click
Psycho replied to praveenhotha's topic in Javascript Help
Isn't that what I just demonstrated above (except that I used 500ms instead of 200ms)? -
How to distinguish between mouse click and dbl click
Psycho replied to praveenhotha's topic in Javascript Help
Well, I'm don't know the "whole picture" here. Do you have an event you want fired on a single click? If not, then just don't do anything for single clicks. Ok, so let's assume the worst-case scenario - that you have one event you want fired on single click and a different event to be fired on a double click. If that is the case, one solution I can think of would be to delay the single click function to wait and see if the double click takes place. Here is a quick test that works. However, it interprets more than two repetitive clicks as a single click. I'm sure that the code could be modified as appropriate. But, this works for single and double clicks only. <html> <head> <script type="text/javascript"> var clickedTwice = false; function clickOnce(firstRun) { if (firstRun) { setTimeout('clickOnce(false)', 500); } else { if (!clickedTwice) { //Run the function normally document.getElementById('once').innerHTML = 'true'; document.getElementById('twice').innerHTML = 'false'; } else { //Element was clicked twice, do not runsingle click //Reset double click trigger clickedTwice = false; } } } function clickTwice() { clickedTwice = true; document.getElementById('twice').innerHTML = 'true'; document.getElementById('once').innerHTML = 'false'; } </script> </head> <body> <a href="#" onclick="clickOnce(true)"; onDblClick="clickTwice();">Click Me!</a><br><br> Clicked Once: <span id="once"></span><br> Clicked Twice: <span id="twice"></span><br> </body> </html> -
[SOLVED] Java Dropdown Population Code Change
Psycho replied to mikemessiah's topic in Javascript Help
No it's definitely not the only way. I just didn't want to spend the time to learnt he code you posted to try and fix it when I already had a solution available. But, I think that the format which my code uses is much easier to maintain, IMO. -
Using a counter to retrieve records is a poor choice anyway. Use a while loop with one of the mysql_fetch_ functions. Also, no need to copy all the results into variables just to use those variables once. <?php include("checkloginstatus.php"); $host = $_SESSION['host']; $user = $_SESSION['user']; $passwd = $_SESSION['passwd']; $dbname = "ppss"; $cxn = mysqli_connect($host,$user,$passwd,$dbname) or die ("User name or password is wrong."); $query = "SELECT Given_Names, Family_Name, Roll_class, Date_Enrolled, T.ContactDate From studePE1 S, TIEnrolmentReview T WHERE S.Student_ID=T.StudentID ORDER BY 4 ASC"; $result = mysqli_query($cxn,$query) or die (mysql_error()); echo "<b><center>Database Output</center></b><br><br>"; while ($row = mysql_fetch_assoc($result)){ echo "<b> " . $row['Given_Names'] . " " . $row['Family_Name'] . " </b><br>" . $row['Roll_class'] . "<br>" . $row['Date_Enrolled'] . "<br>" . $row['ContactDate'] . "<hr><br>\n"; } ?>
-
Not that I'm aware of. I would think not because, if you think about it, if the browser was able to take control of the mouse cursor position that would be a security problem in my opinion. Just think about all the sites that would take control of your cursor to possibly do very malicious acts. Then again, I'm not 100% sure. But, if I ever visited a site that tried to take that kind of control - it would be the last time I would visit.
-
No offense, but is there some reason you couldn't check the manual? It'd sure be a lot quicker than posting on a forum. http://us.php.net/manual/en/function.hash-hmac.php
-
[SOLVED] Delayed actions in Internet Explorer problem
Psycho replied to Petty_Crim's topic in Javascript Help
This is actually one instance where IE gets it right. onchange is NOT supposed to be supported for a checkbox. -
Then you have done something wrong. If you copy and paste the code I have above in its entirety it works perfectly. I'm thinking that there is still a problem with the IDs or field names. Show your code it you want more help. If you are using this input for calculations, then I will assume that you are processing the code server-side with PHP or some other language. If that's the case, then you should still not use a secondary field. Just submit either the full text (and strip out the text for calculations) or just submit the number (and recreate the text for display purpose). By using two fields for essentially the same data you open yourself up to problems that can be difficult to debug. Youcould also make the value of the options something like this: large - $950|950 And then split the value on the server side to the text and the value parts.
-
I know you have maked this as solved, but now that you have posted the code properly I can now review it. Here is what I would do (may be some syntax errors): This would process all 10 images (I would also create a PHP loop for creating the form fields as well. Never copy-and-paste code that should be identical. <?php $imgTypes = array ( 'image/gif','image/jpeg', 'image/jpg', 'image/png', 'image/tif', 'image/psd', 'image/bmp', 'image/pct', 'image/pjpeg'); $for ($imgNum=1; $imgNum<=10; $imgNum++) { $image = $_FILES['file'+$imgNum]; $fileName = $image['name']; $tempName = $image['tmpName']; $fileSize = $image['size']; $fileType = $image['type']; $fileErr = $image['error']; if ($fileErr>0) { echo "Return Code: $fileErr\n"; } else { if (in_array($fileType, $imgTypes) && ($fileSize < 200000000000)) { echo "Upload: $fileName\n"; echo "Type: $fileType\n"; echo "Size: " . ($fileSize / 1024) . " Kb\n"; echo "Temp file: $tempName\n"; if (file_exists("upload/" . $fileName)) { echo "$fileName already exists.\n"; } else { move_uploaded_file($tempName, "upload/" . $fileName); echo "Stored in: " . "upload/" . $fileName; } } else { echo "Invalid file"; } } } ?>
-
Can't you just change the values of the options to fit your needs? I.e. <option value="950">large - $950</option> By the way, you should not have IDs for your options. Even if you did, you cannot duplicate the same ID on a page. You also had the wrong ID for the first "Cost" field. Anyway, here is some working code (although I would still go with having the appropriate value in the options to begin with) <head> <script type="text/javascript"> function getIPut(fieldObj) { var fieldID = fieldObj.id.substr(fieldObj.id.length-1 ,1); var boothObj = document.getElementById('Booth'+fieldID); var state = boothObj[boothObj.selectedIndex].value; var loca=''; if(state == 'large - $950') { loca = '950'; } else if (state == 'medium - $850') { loca = '850'; } else if(state == 'small - $750') { loca = '750'; } document.getElementById('Cost'+fieldID).value = loca; } </script> </head> <body> Booth type: <label> <select onchange="getIPut(this);" id="Booth1" name="Booth1"> <option value=""></option> <option value="large - $950">large - $950</option> <option value="medium - $850">medium - $850</option> <option value="small - $750">small - $750</option> </select> </label> <input id="Cost1" type="text" name="Cost1" /> <p /> Booth type: <label> <select onchange="getIPut(this);" id="Booth2" name="Booth2"> <option value=""></option> <option value="large - $950">large - $950</option> <option value="medium - $850">medium - $850</option> <option value="small - $750">small - $750</option> </select> </label> <input id="Cost2" type="text" name="Cost2" /> </body> </html> Or alternatively, you could use a regular expression to make it much simpler (assuming you always just want the numerical value from the option) function getIPut(fieldObj) { var fieldID = fieldObj.id.substr(fieldObj.id.length-1 ,1); var boothObj = document.getElementById('Booth'+fieldID); var loca = boothObj[boothObj.selectedIndex].value.replace(/[^\d]/g, ''); document.getElementById('Cost'+fieldID).value = loca; }
-
<div id="loginBox" onMouseOut="setTimeout('login(\'hide\')', 3000);"> http://www.elated.com/articles/javascript-timers-with-settimeout-and-setinterval/
-
Well, obviously the query is failing. But, you have no error handling to catch it. You should add " or die (mysql_error()): at the end of the query. The error in the query is due to the double quote marks at the end. But, you should also not have a looping query. Just grab all the records in one query: Sample code: <?php $tickedkValues = implode(',', $_POST['ticked']); $query = "SELECT status FROM branch_items WHERE drug_id IN ($tickedkValues) AND branch_id=".$_POST[branch_id][$row_value]; $result = mysql_query($query) or die ("Query:<br>$query<br>Error:<br>".mysql_error()); echo "<table>"; while ($row = mysql_fetch_array( $result2 )) { echo "<tr>"; echo "<td>".$_POST[drug_id][$row_value]."</td>"; echo "<td>".$_POST[branch_id][$row_value]."</td>"; echo "<td>".$row[status]."</td>"; echo "</tr>"; } echo "</table>"; ?> You also need to "clean" all the values before running a query on them
-
Well, do you honestly expect people to read through all that code (especially since you didn't even take the time to use CODE tags so it would be more legible)? Perhaps you should break down the code to certain parts and ask specific questions. The only advice I will offer is as follows: 1) You are doing the EXACT same thing for each image. So why wuould you want to write out all of that code 10 times. Create a loop. By "copying and pasting" code in that manner you are asking for trouble. By using the same code each time if there are any errors you only need to correct them once. Example: <?php for ($fileNum=1; $fileNum<=10; $fileNum++) { $currentFile = $_FILES['file9'.$fileNum]; //Now include all the processing using the array $currentFile } ?> 2) Instead of a bunch of OR's <?php if ((($_FILES["file10"]["type"] == "image/gif") || ($_FILES["file10"]["type"] == "image/jpeg") || ($_FILES["file10"]["type"] == "image/jpg") || ($_FILES["file10"]["type"] == "image/png") || ($_FILES["file10"]["type"] == "image/tif") || ($_FILES["file10"]["type"] == "image/psd") || ($_FILES["file10"]["type"] == "image/bmp") || ($_FILES["file10"]["type"] == "image/pct") || ($_FILES["file10"]["type"] == "image/pjpeg")) && ($_FILES["file10"]["size"] < 200000000000)) { ?> Do this: <?php //Define this outside the loop $imgTypes = array ( 'image/gif','image/jpeg', 'image/jpg', 'image/png', 'image/tif', 'image/psd', 'image/bmp', 'image/pct', 'image/pjpeg'); //Put this inside the loop if (in_array($currentFile["type"], $imgTypes) && ($currentFile["size"] < 200000000000)) { ?>
-
selecting from two tables where values not in a third table
Psycho replied to gnznroses's topic in PHP Coding Help
I prefer using JOINs. If you want to see which is faster - just test it. Put each one in a loop that runs n times and get the time before and after the loop. Although you can do much, much more with JOINs that you can't do by using the WHERE clause to join tables - for instance the LEFT JOIN that I used above. Yes, you need to add the WHERE clause as you have it, I didn't catch that requirement in your original post. -
selecting from two tables where values not in a third table
Psycho replied to gnznroses's topic in PHP Coding Help
You can absolutely do it in one query. I'm usually better with this when I have the appropriate tables to work with, but try the query below. The LEFT JOIN will ensure that all the records from the first two tables will be returned and the hated_users values will be returned for those records included in those tables, but for records that do not have matches to the hated_users table those values will be returned as NULL. So, for a user who does not exist in the hated_users table, that record will be returned with the column for hated_users.user_id, but it will be NULL and not equal to the actual user ID. Therefore, the last WHERE clause should filter out those users that are in the hated_users table. SELECT * FROM users u JOIN user_transaction ut ON u.id = ut.user_id LEFT JOIN hated_users hu ON u.id = hu.user_id WHERE u.active = 1 AND hu.user_id <> u.user_id EDIT: Scratch that, I think teng's solutin is more elegant: SELECT * FROM users u JOIN user_transaction ut ON u.id = ut.user_id WHERE u.active = 1 AND u.id NOT IN (SELECT user_id FROM hated_users) -
Maybe, but the process I identified above is the preferred approach.
-
Well, a NOSCRIPT tag would not prevent the above link from showing - it would just not work for users that don't have JS enabled. IMHO, the best process is to first build the page with no JavaScript. Then once it is complete find where you can add JS to make it more user-friendly. In this case, though I think there is an easy solution. Add an actual link to the href property. I would suggest linking back to the current page, but adding a parameter on the URL for the currency to be displayed (You would need to modify the page to accept that parameter and display the appropriate currency on reload). Then that link will work for users that do not have JS enabled. But, you don't want that link to refresh the page for users with JS enabled. So, just add a "return false;" to the onclick property and that will prevent the link from actually firing for JS users. <?php $changeCurrency = ($_SESSION['currency']=='EUR') ? 'Pounds.' : 'Euros.'; ?> <a href="<?php echo $_SERVER[php_SELF]?currency=$changeCurrency; ?>" onclick="doPrice('<?php echo $_SESSION['currency'] ?>');return false;"> Change to <?php echo $changeCurrency; ?> </a>
-
Change the function calls to the format (this, 'path/to/image.gif') <input type="image" src="images/button-addCart.gif" alt="Add to Cart" title="Add to Cart" name="cartButton" id="cartButton" onMouseOver="change_it(this, 'images/cartButton.gif')" onMouseOut="change_it(this, 'images/button-addCart.gif')"> function change_it(fieldObj, imgSrc) { if(document.images) { fieldObj.src = imgSrc; } }
-
[SOLVED] Java Dropdown Population Code Change
Psycho replied to mikemessiah's topic in Javascript Help
Here is some code I have that does the same thing, but is a bit more manageable (plus it uses the text as the value). You just need to change it for your values. <html> <head> <script type="text/javascript"> // State lists var states = new Array(); states['Canada'] = new Array('Alberta','British Columbia','Ontario'); states['Mexico'] = new Array('Baja California','Chihuahua','Jalisco'); states['United States'] = new Array('California','Florida','New York'); // City lists var cities = new Array(); cities['Canada'] = new Array(); cities['Canada']['Alberta'] = new Array('Edmonton','Calgary'); cities['Canada']['British Columbia'] = new Array('Victoria','Vancouver'); cities['Canada']['Ontario'] = new Array('Toronto','Hamilton'); cities['Mexico'] = new Array(); cities['Mexico']['Baja California'] = new Array('Tijauna','Mexicali'); cities['Mexico']['Chihuahua'] = new Array('Ciudad Juárez','Chihuahua'); cities['Mexico']['Jalisco'] = new Array('Guadalajara','Chapala'); cities['United States'] = new Array(); cities['United States']['California'] = new Array('Los Angeles','San Francisco'); cities['United States']['Florida'] = new Array('Miami','Orlando'); cities['United States']['New York'] = new Array('Buffalo','New York'); function setStates(){ cntrySel = document.getElementById('country'); stateSel = document.getElementById('state'); stateList = states[cntrySel.value]; changeSelect(stateSel, stateList); setCities(); } function setCities(){ cntrySel = document.getElementById('country'); stateSel = document.getElementById('state'); citySel = document.getElementById('city'); cityList = cities[cntrySel.value][stateSel.value]; changeSelect(citySel, cityList); } //**************************************************************************// // FUNCTION changeSelect(fieldObj, valuesAry, [optTextAry], [selectedVal]) // //**************************************************************************// function changeSelect(fieldObj, valuesAry, optTextAry, selectedValue) { //Clear the select list fieldObj.options.length = 0; //Set the option text to the values if not passed optTextAry = (optTextAry)?optTextAry:valuesAry; //Itterate through the list and create the options for (i in valuesAry) { selectFlag = (selectedValue && selectedValue==valuesAry[i])?true:false; fieldObj.options[fieldObj.length] = new Option(optTextAry[i], valuesAry[i], false, selectFlag); } } </script> </head> <body onload="setStates();"> <form name="test" method="POST" action="processingpage.php"> Country: <select name="country" id="country" onchange="setStates();"> <option value="Canada">Canada</option> <option value="Mexico">Mexico</option> <option value="United States">United States</option> </select> <br> State: <select name="state" id="state" onchange="setCities();"> <option value="">Please select a Country</option> </select> <br> City: <select name="city" id="city"> <option value="">Please select a Country</option> </select> </form> </body> </html> -
Again, that is not a valid statement. Let's say you have the super most uber encryption algorithym possible. I could still create something to run through possible combinations for passwords and create a rainbow table. So, in that respect no algorithym is better than another. The reason that passwords are "fairly" easy to crack from an MD5 (or anything else) is that passwords are typically restriced to a small number of characters (say 20). Plus, people tend to use proper words. So, creating a process to generate all the combinatins only takes time. However, if you take away those limitations, then there is no way to crack, unecrypt, or decypher an MD5 hash. This page explains why. MD5 is not collision resistent. That is bad news for some uses of MD5. But, that also means it is impossible to determine what was used to create an MD5 hash (unless you throw in restrictive limitations).