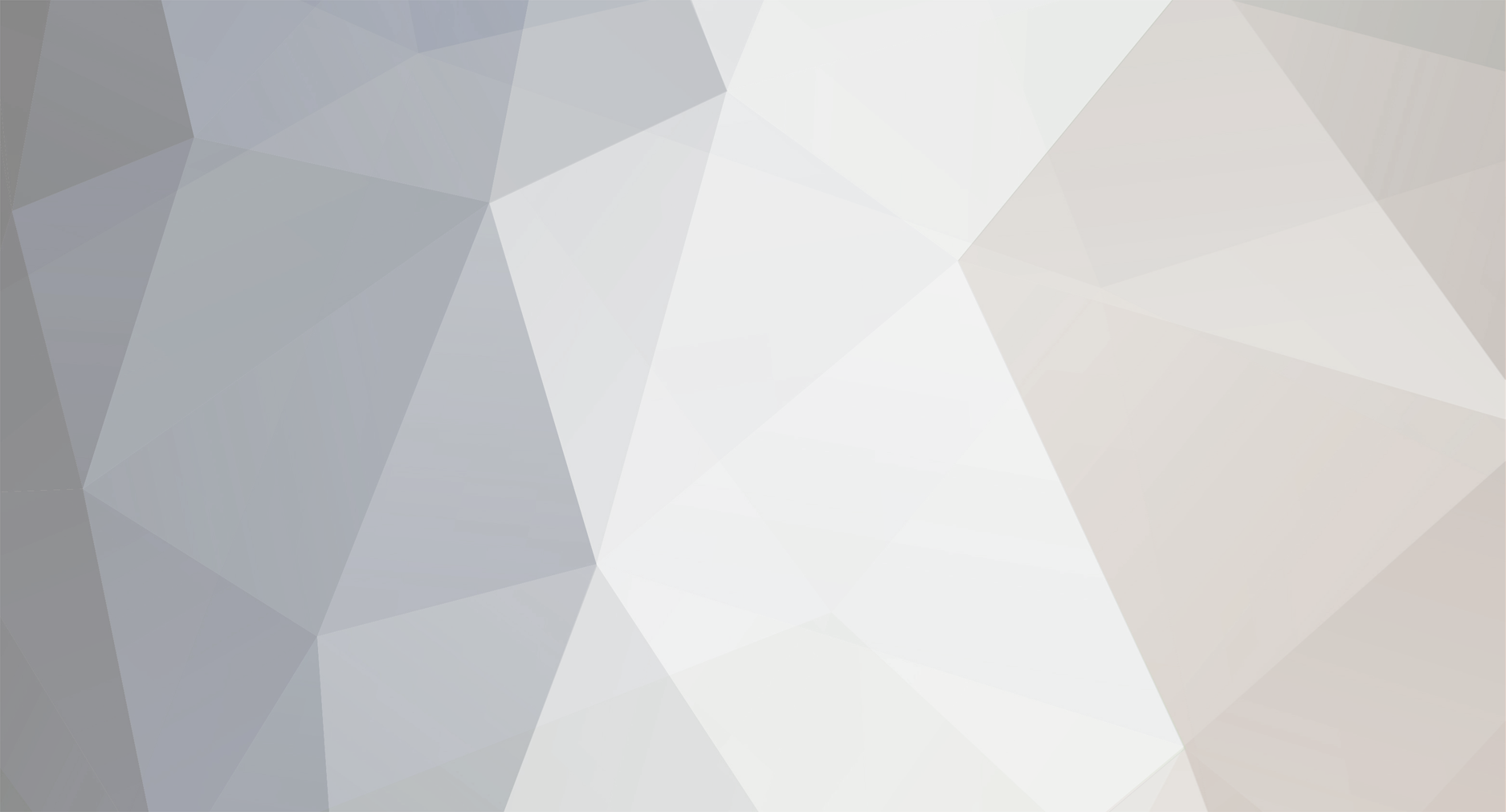
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
md5 = not much because it can be decrypted fairly easily and it also doesn't prevent data transfer issues as its still going from client to server unprotected. Well, I will disagree with MD5, but for the complete opposite reason. MD5 is not an encryption - it is a one-way hash. We are talking about protecting the password when being transmitted from the client to the server. So, what would you do use JavaScript to hash with MD5? That's not very user friendly. Then someone could simply "capture" the MD5 value and force their submission to send that value. As for being able to decrypt MD5, that is a misnomer. Yes, there are "lookup" tables where you can enter an MD5 value and it will return the value used to create that MD5 value. But, that is only because someone created that pair of values and entered it into the database. That would work to unencode/decrypt anything. Also, there is a finite number of MD5 values, but you can generate an MD5 values from an infinite number of source values. So, just because a lookup table says the hash was created from value x is not definitive.
-
Use https However, you need to consider the likelyhood of someone tryng to infiltrate your application and the value of that information. If it's just a gaming site there is no need for such security. If it's a banking site, it sure as hell better be using https. The most common method of gaining unauthorrized access is not through packet sniffing - it is through social engineering.
-
<html> <head></head> <body> <?php #set default values $one = 'watermellons'; $two = 'smelly'; $three = 'toilet'; $four = 'fanny'; $five = 'poop'; if (isset($_POST['submitted'])) { #define variables for the mad lib #disable magic quotes using the stripslashes function #remove left and right spaces if they are entered in the form using the trim function. $one = trim(stripslashes($_POST['1'])); $two = trim(stripslashes($_POST['2'])); $three = trim(stripslashes($_POST['3'])); $four = trim(stripslashes($_POST['4'])); $five = trim(stripslashes($_POST['5'])); #set error message if any fields in the form were left blank $errors = array(); if (empty($_POST['1'])) { $errors[] = "You didn't enter a plural noun!"; } if (empty($_POST['2'])) { $errors[] = "You didn't enter an adjective!"; } if (empty($_POST['3'])) { $errors[] = "You didn't enter the first noun!"; } if (empty($_POST['4'])) { $errors[] = "You didn't enter the second noun!"; } if (empty($_POST['5'])) { $errors[] = "You didn't enter the third noun!"; } #write an if statement to display the madlib if filled out correctly, but display an error message if any fields in the form were left blank. if (count($errors)) { foreach ($errors as $thisError) { echo "<span style=\"color:#ff0000;\">$thisError</span><br />";; } echo "<p style=\"color:#ff0000;\">Please fill out the form completely.</p>"; } else { #place the mad lib inside of a variable #include and concatenate the previous defined variables $madlib = "Cinderella had two step-" . $one .", who were very " . $two . " to her. So her fairy god" . $three . " turned her pumpkin into a big " . $four . ", and dragged her off to the " . $five . "."; echo $madlib; #print out a custom paragraph after the mad lib but do not print anything if one or more of the fields in the form were left blank. if (($one == "watermelons") && ($two == "smelly") && ($three == "toilet") && ($four == "fanny") && ($five == "poop")) { echo "<p><em>\"Hey! You copied off me!! And now, I shall kill you!!\"</em></p>"; } elseif (($one == "watermelons") || ($two == "smelly") || ($three == "toilet") || ($four == "fanny") || ($five == "poop")) { echo "<p><em>\"Still using some of my words... get your own!!\"</em></p>"; } elseif (($one == "sisters") && (($two == "mean") || ($two == "evil") || ($two == "nasty")) && ($three == "mother") && ($four == "carriage") && (($five == "ball") || ($five == "castle"))) { echo "<p><em>\"Oh, you did it the way it's supposed to go... that's not very funny now is it?\"</em></p>"; } else { echo "<p><em>\"Haha! Oh, how ruthlessly absurd.\"</em></p>"; } } } echo "<p>Stewie's Mad Lib</p> <p>Do the same Cinderella Mad Lib that Stewie did in Family Guy! The text boxes are already filled in with the words he used on the show, but you can change them to your own words if you like. Have fun!</p> <form action=\"$_SERVER[php_SELF]\" method = \"post\"> <table border=\"0\"> <tr><td>Enter a plural noun</td><td><input name=\"1\" type=\"text\" value=\"$one\" /></td></tr> <tr><td>Enter an adjective</td><td><input name=\"2\" type=\"text\" value=\"$two\" /></td></tr> <tr><td>Enter a noun</td><td><input name=\"3\" type=\"text\" value=\"$three\" /></td></tr> <tr><td>Enter another noun</td><td><input name=\"4\" type=\"text\" value=\"$four\" /></td></tr> <tr><td>Enter another noun</td><td><input name=\"5\" type=\"text\" value=\"$five\" /></td></tr> <tr colspan=\"2\"><td><input type=\"submit\" value=\"Let's see my Mad Lib!\" /></td></tr> <input type=\"hidden\" name=\"submitted\" value=\"TRUE\" /> </table> </form>"; ?> </body> </html>
-
Well, it would have been helpful if you had provided code. Since you didn't I will only offer some mock code to show one method. There may be a more efficient method based upon your existing code and functionality. <?php $field1value = (!empty(trim($_POST['field1']))) ? trim($_POST['field1']) : "Default for field 1"; ?> <input type="text" name="field1" value="<?php echo $field1value; ?>">
-
How do you make user acounts and user login on a website
Psycho replied to likwidmonster's topic in Javascript Help
Ok, here is a vert, very simple script that would work - although it would need a lot more work. Also, there are ready made classes that will allow you to use flat files just like a database. Create a text file called user_list.txt and enter the username and passwords like so: michael|testing robert|whatever Then create a PHP file such as this: <?php function validUser() { $uname = $_POST['uname']; $pword = $_POST['pword']; $accountFile = "user_list.txt"; $accounts = file($accountFile); foreach ($accounts as $userData) { list($username, $password) = explode('|', $userData); if (trim(strtolower($username))==trim(strtolower($uname))) { return (trim($password)==trim($pword)); } } return false; } $error = ''; if (isset($_POST['uname']) || isset($_POST['uname'])) { if (validUser()) { echo "<html><head></head><body>Log user in.</body></html>"; exit; } else { $error = "Username/password entered invalid"; } } ?> <html> <head></head> <body> <?php echo $error; ?> <form method="post"> Username: <input type="text" name="uname" value="<?php echo $_POST['uname']; ?>"><br> Username: <input type="password" name="pword"><br> <button type="submit">Submit</button> </form> </body> </html> Again this is only a basic example and I would not suggest using this code as is - but I'm not going to take the time to code all of this out. This only shows that it will work. -
How do you make user acounts and user login on a website
Psycho replied to likwidmonster's topic in Javascript Help
Well, you CAN'T do a login with JavaScript (at least not one that is of any value - anyone could log in by looking at the page source). You CAN do it with PHP (with or without a database - just use a flat file). There is no such thing as a "Linux sub-domain". The fact that the server is Linux vs. Windows or a sub-domain or a domain has nothing to do with any of this - nothing. Again, from the partial error message you posted (Which you still haven'tprovided full details for) it appears you can use PHP. If that's the case you can have a login function. If no database is availabel then you can use flat files. -
I think WMA is supported (it just doesn't stand out on that page). Under "Audio-Video formats" it states: ASF (ASF, Windows Media Audio, Windows Media Video)
-
This is going to be run on a Windows server. Perhaps a scheduled task? EDIT: A little searching answered my own question (and it does involved scheduled tasks) Configuring cron jobs on Windows
-
This works nicely: getID3()
-
Thanks, but that's appears to be a "complete" solution for accessing your MP3's. I'm building my own interface and am comfortable with all the functionality except for the process of scanning a large number of files. FYI: There's a really nice free PHP app for this called Jinzora which I want to use, but it seems to always have several areas that are broken (installed different versions on several different machines/environments). So instead of reengineering someone elses code I decided I would build my own. http://en.jinzora.com/
-
I am working on a project to make a web interface for my MP3 collection. It will include functionality to read the tags from the MP3 files and save them to a database. But, I'm at a loss as to how I should set up the scanning process since it will be doing a large number of files. I will most likely have the ability to scan newly added albums individually as they are added, but I will also need to have a full scan process to account for files that are moved or have had the tags changed. Each album has been ripped to a separate folder, so there are a few hundred folders and thousands of files. Timing out of the script will be an issue as well. I was thinking that utilizing AJAX would be a possible solution so I could have one request process a folder, when that completes a new request would process the next folder, etc. That would prevent timeout issues. But, how would I keep track between JS and PHP as to which folder is next? I was thinking of havng PHP do an initial scan of just the folders and then passing that back to the Javascript or saving to a text file. Then using that to monitor the next folder to process. But, I'm wondering if there is a more elegant solution. Any ideas or solutions are welcome.
-
How do you make user acounts and user login on a website
Psycho replied to likwidmonster's topic in Javascript Help
No I can read perfectly fine. Nowhere did you state what code you used that produced the error you posted on Reply#31 (in Reply#26 you stated that haku's code produced an error). So where, exactly, did you state that you used the code provided from lewis987? Why are you being so argumentative? For some reason you are hung up on the obsession that because your site is being run on a sub-domain that it would somehow function differently. That is just not the case. I'm not trying to be rude (unlike trying to question someone's reading ability), but you seem to ignore everything that everyone has stated. When asked a question by those trying to help you, you only respond with partial responses or change the subject entirely. If we ask you to try somethign and it doesn't work, just post back EXACTLY what did or did not work along with any error messages. We do not have access to your system so we rely upon you to give complete and accurate details. As haku stated, the error you recieved supports the fact that PHP is enabled for you. However, you didn't respond to his request to provide the full error which would explain the problem (which is in all likelyhood just a syntax error). Also, as he stated, your 'instructor' doesn't know what he is talking about - PHP does not require a database. You can create a PHP page that interacts with a database, but that is another matter. First let's get the PHP issue straitened out. -
Offhand, I can't think of any good solutions because of the same reasons listed above. In addition, the table of contents traditionally goes at the beginning of the document. There are two problems associated with that: 1) How to get the table of contents at the beginning of the content after it is created (since you need to create the content first to determine the pages - if possible, and 2) without knowing beforehand how long the table of contents is you could not determine what page the content starts on. And with most content types I can think of the pagination is determined by the rendering application. E.g. if you and I have different margins for Notepad we would have different page breaks for the same content. One "possible" solution would be to create the content in PDF form. I have not used PHPs PDF creation functions, but I used to work for a company that created dynamically generated PDFs through a web interface. However, in that environment we used PostScript programming to generate the PDFs and with PostScript the code can determine the exact position on the page as each line of text is "written" and the code is responsible for creating the page breaks - not the rendering application. If the PHP PDF functions have that capability it would be possible, but it would be more complex than it is now as you need to control the position of every piece of text - including line-breaks, pagination, etc. Even then, I'm not sure if it would be possible to append the table of contents to the beginning of the document after the content pages are generated. You might have to settle with putting the TOC at the end of the document.
-
How do you make user acounts and user login on a website
Psycho replied to likwidmonster's topic in Javascript Help
as I said, using a sub-domain doesn't mean anything. You probably just picked up the snippet of code that had the syntax error as identified on the previous page. -
With an RTF file, yes, I believe so. Assuming PHP could access the following: - The margins - The page size I don't see how PHP could determine where the page breaks are created in the document - and thus the number of pages and what content is on which page. The page breaks are "dynamic" in that they are determined by the document reading the file. They are not embedded in the file where they can be read by PHP.
-
PHP would have no way of knowing where the page breaks are when creating that type of dcoument.
-
Yes, especially since JavaScript can be disabled!
-
Well, for each user input you had an if statement to determine if you needed to append an OR first. So, you had those same two lines repeated over and over. Instead of doing that I first created an array "$queryAry". Then for each user input I kept the logic to determin whether or not the WHERE clause was need - if so, I added that clause to the array. At the end of the process you are left with an array of multiple clauses. Then the last step is to simply concatenate all of the clauses separated by OR's using the implode() command. So in essence it is replacing the two lines of code that is duplicated for every field with a single line.
-
Well, since you put the COED within QUOTE tags the links are being processed, so it is difficult to see how the links are being built. I would guess the problem is that you are passing all the WHERE clauses as POST variables, but the pagination script is using GET variables for the page to show. I would suggest taking all the WHERE paramaters and serializing it into a single string and appending that to the query string. Then simply check if there is POST data, if so then recreate the WHERE caluse and show page 1. If there is no POST data, but there is GET data then get the page and serialized WHERE clause and show the appropriate page. Also, this will trim down (and more importantly clean) the user input for the WHERE clause: <?php $queryAry = array(); if ($_POST[Amateur]<>"") { $queryAry[] = "jobsegment = '".mysql_real_escape_string($_POST[Amateur])."'"; } if ($_POST[Corporate]<>"") { $queryAry[] = "jobsegment = '".mysql_real_escape_string($_POST[Corporate])."'"; } if ($_POST[Facilities]<>"") { $queryAry[] = "jobsegment = '".mysql_real_escape_string($_POST[Facilities])."'"; } if ($_POST[Health]<>"") { $queryAry[] ="jobsegment = '".mysql_real_escape_string($_POST[Health])."'"; } if ($_POST[Professional]<>"") { $queryAry[] = "jobsegment = '".mysql_real_escape_string($_POST[Professional])."'"; } if ($_POST[sporting]<>"") { $queryAry[] = "jobsegment = '".mysql_real_escape_string($_POST[sporting])."'"; } if ($_POST[Location]="Region") { if ($_POST[West]<>"") { $queryAry[] = "jobregion = '".mysql_real_escape_string($_POST[West])."'"; } if ($_POST[Central]<>"") { $queryAry[] = ."jobregion = '".mysql_real_escape_string($_POST[Central])."'"; } if ($_POST[Northeast]<>"") { $queryAry[] = "jobregion = '".mysql_real_escape_string($_POST[Northeast])."'"; } if ($_POST[southeast]<>"") { $queryAry[] = "jobregion = '".mysql_real_escape_string($_POST[southeast])."'"; } if ($_POST[Canada]<>"") { $queryAry[] = "jobregion = '".mysql_real_escape_string($_POST[Canada])."'"; } } if ($_POST[Location]="State") { if ($_POST[state]<>"") { $queryAry[] = "jobstateabbrv = '".mysql_real_escape_string($_POST[state])."'"; } } $query = implode(' OR ', $queryAry);?>
-
How do you make user acounts and user login on a website
Psycho replied to likwidmonster's topic in Javascript Help
I guess I need to re-state what I stated on the previous page (with one addition): You are getting way too caught up on this "sub-domain" thing. If the server supoprts PHP, then it supports PHP. I create sub-domins all the time and, guess what?, those sub-domains support PHP without me having to do anything else. I'm sure there may be a way to disable PHP for a sub-diomain, but it would be the exception and not the norm. Did you even try any of the suggestions above to see if PHP is enabled? -
This is not a PHP problem, this is a problem in the differences between how some browsers interpret HTML code. Specifically the problem is that you are using the height & width parameters, but not giving them any values. IE is interpreting this as if you had set the values to 0. Just remove them, don't understand why you would include the parameters if you don't use them anyway.
-
Try this: $sql = "SELECT * FROM bocj WHERE id='".mysql_real_escape_string($_GET['id'])."'"; if (isset($_GET['instrument'])) { $sql .= " AND instrument = '".mysql_real_escape_string($_GET['instrument'])."'" }
-
Sessions have nothing to do with a database. And, what do you mean by "add lines to the end of a existing session[file]". While the data is stored in a file on the server, the data is created/accessed as an array - you don't have any control over how the data is stored.
-
No. PHP can be used to create browser-based applications. But, you are talking about porting a desktop application via the internet. What software are you trying to port?
-
Not with JavaScript. The closest thing I can think of would be Citrix, assuming you have a lot of spare cash handy.