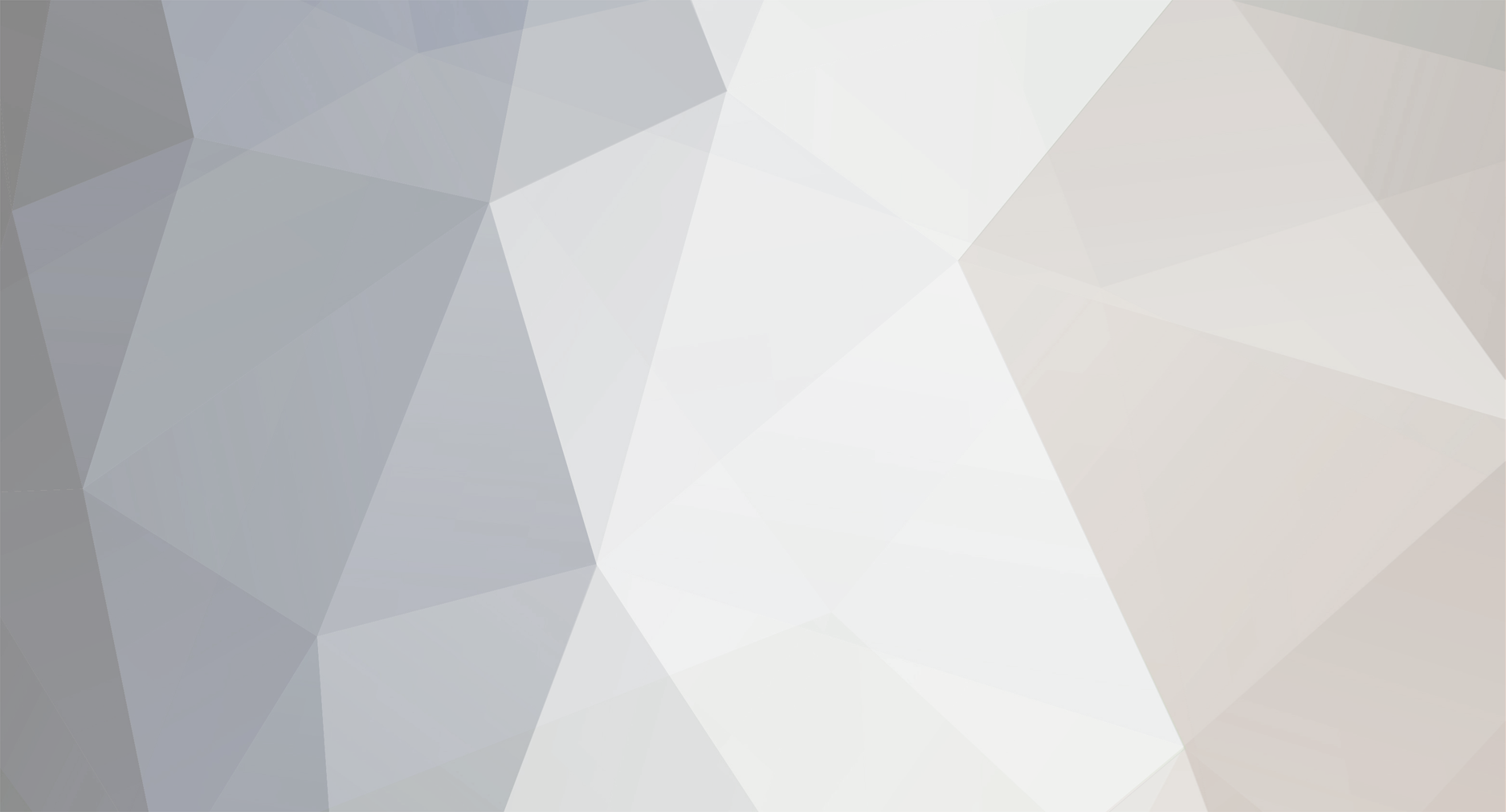
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Just because I like compact code: function toEntity($aa) { $bb = ''; for($i=0; $i<strlen($aa); $i++) { $bb .= (ord($aa[$i])>127)? ''.ord($aa[$i]).';' : $aa[$i] ; } return $bb; }
-
It could be because the code I provided used the variable $team whereas Barand's code uses the variable $teams. Make sure the variables are named the same.
-
[SOLVED] Need help Deleting multiple rows from mysql with php
Psycho replied to stopblackholes's topic in PHP Coding Help
This will be a little more efficient: <?php $deletelist= "'" . implode("', '", $_POST['checkbox']) . "'"; $query = "DELETE FROM posts WHERE post_id in ($deletelist)"; mysql_query($query)or die(mysql_error()."<br />QUERY: $query"); ?> -
$result = bb_db_query("SELECT * FROM bb_players"); while ($myrow=mysql_fetch_array($result)) { $team[myrow['team_id']]['name'] = $myrow['team']; $team[myrow['team_id']]['players'][] = $myrow['player']; }
-
[SOLVED] calling a function inside of another function
Psycho replied to iarp's topic in PHP Coding Help
Um, your query is only returning the value for the 'config_value' column and then you are trying to reference the 'site_location' value. change the query to return the value you actually want to use. <?php function links() { $query = "SELECT site_location FROM " . TBL_CONFIG . " WHERE config_name='site_location'"; $result = mysql_query($query); $row = mysql_fetch_array($result, MYSQL_ASSOC); echo "/" . $row['site_location'] . "/"; } ?> -
That is a shorthand version of an If/Else statement. I believe the correct name is the ternarry operator. Basically, that line states is the length of A is greater than the length of B return 1, else return -1. Here is a short article on it: http://php.codenewbie.com/articles/php/1480/The_Ternary_Operator-Page_1.html Personally, I woudl think the first example is more efficient, but I have not tested that hypothesis.
-
Here's another approach <?php $my_cars = array('chevy', 'chevys', 'dodge', 'dodges', 'lancer', 'mitsubishi', 'nissan'); if($my_cars){ foreach($my_cars as $key => $val){ $tmp_list[$val] = strlen($val); } asort($tmp_list); $my_cars = array_keys($tmp_list); } echo "<pre>\n"; print_r($my_cars); echo "</pre>\n"; ?>
-
Yes, it is possible. The problem is that you are using the string length as the array index. Since 'chevys' and 'lancer' have the same string length the index of [6] first gets defined as 'chevys', then it gets redefined as 'lancers'. Here's one solution: <?php function sortByLength($a, $b) { if (strlen($a)==strlen($b)) { //If length =, sort by alpha return strcmp($a, $b); } return (strlen($a)>strlen($b)) ? 1 : -1; } $my_cars = array('chevy', 'chevys', 'dodge', 'dodges', 'lancer', 'mitsubishi', 'nissan'); if($my_cars){ usort($my_cars, "sortByLength"); } echo "<pre>\n"; print_r($my_cars); echo "</pre>\n"; ?>
-
To help yourself, change this line $return = mysql_query($query); To this $return = mysql_query($query) or die ("Query:<br />$query<br />Error:<br />".mysql_error());
-
Just curious, what's the purpose of checking for a numeric value? mysql_real_escape_string() won't do anything to a number anyway, does it?
-
HELP with how to go about coding this function
Psycho replied to TaylorSandbek's topic in PHP Coding Help
And why would you use longtext? Were you planning to have a single record for each user with ALL of their firends listed in a specific field? Follow The Little Guy's example. You should have a single record for each friend relationship. Simple smallint fields should suffice. -
mysql_real_escape_string()
-
[SOLVED] Strange Problem - Should be a Simple Solution
Psycho replied to JSHINER's topic in PHP Coding Help
Or a more graphical representation 11 | 21 | 2 | 31 | 2 | 3 | 4 First pass Second pass Third pass Fourth pass -
Simple - don't do an include. Insead put the redirect in the "client" code based upon a response from the script on your server. Your page should be a sijmple PHP page that returns true or false. Here is some code (not tested) that should point you int he right direction: Script on Client Server: <?php $cp ="web"; $ht_us = urlencode($_SERVER['HTTP_USER_AGENT']); $ht_acc = urlencode($_SERVER['HTTP_ACCEPT']); $xw_prof = urlencode($_SERVER['HTTP_X_WAP_PROFILE']); $profile = urlencode($_SERVER['HTTP_PROFILE']); $mini = urlencode($_SERVER['X-OperaMini-Features']); $pix = urlencode($_SERVER['UA-pixels']); $source_page = "http://www.site.co.za/redirect/site_redirect.php?cp=$cp&ht_us=$ht_us&ht_acc=$ht_acc&xw_prof=$xw_prof&profile=$profile&mini=$mini&pix=$pix"; $mobile = file_get_contents($source_page); if ($mobile=='1') { header('Location: http://www.site.co.za'); } ?> The PHP page on you server: <?php $cp = $_GET['cp']; function detect_mobile_device(){ $ht_us = urldecode($_GET['ht_us']); $ht_acc = urldecode($_GET['ht_acc']); $xw_prof = urldecode($_GET['xw_prof']); $profile = urldecode($_GET['profile']); $mini = urldecode($_GET['mini']); $pix = urldecode($_GET['pix']); // check if the user agent gives away any tell tale signs // it's a mobile browser the code that goes here is working // perfectly because i have used it in tons of other places if (mobile) return true; } //Output 1 or 0 to indicate if it is a mobile device if(detect_mobile_device()) { echo '1'; } else { echo '0'; } ?>
-
Note that making the field a "password" field only masks the password on screen when enetered. It is still sent in plain text when submitted. You would need to hash/encrypt the password when recived before creating the DB record.
-
Maybe another set of eyes on this could help? While loop problems
Psycho replied to kb9yjg's topic in PHP Coding Help
<?php $columns = 4; $current_column = 1; while($info=mysql_fetch_array($query)){ //Start new row if ($current_column == 1) { echo "<tr>"; } //Display the current data echo "<td align=\"center\">"; echo "<img src=\"".$previewp.$info['itemno']."\" height=\"32\" width=\"32\">"; echo "<br><a href=\"iteminfo.php?itemno=".$info['itemno']."\" target=\"_blank\">"; echo "<span class=\"style2\">".$info['itemno']."</span></a><br>"; echo "<span class=\"style2\">".$info['pthick']."x".$info['pwidth']."</span></td>"; $current_column++ //Close the current row if last column if ($current_column > $columns) { echo "</tr>"; $current_column = 1; } } //Close out remaining columns (if needed) if ($current_column>1) { for ($col=$current_column; $col<=$columns; $col++) { echo "<td> </td>"; } echo "</tr>"; } ?> -
You seem to be asking for several different things in your post, but some of it is a little convoluted. You say you want a checkbox and a button for each product? Why do you need the checkbox if you are going to have a button next to each product? or, if you are saying you want only 1 button for the page, then what do you want to have happen if multiple product checkboxes are checked? The easiest (and most fool-proof) method is to simply open a new page with just the information you want printed. As for printing specific parts of a page, that can be accomplished with CSS. Just use classes within your page and then use the "media" type to specify whether certain content should be printed or not. <style> @media print { noprint { display: none; } } @media screen { noprint { display: inline; } } </style> ... <span class="print">This will be printed</span> <span class="noprint">This will NOT be printed</span>
-
Yes you can have POST values that are arrays - just give multiple fields the same array name. Here's an example of a form where an array would be returned: <input type="text" name="cost[]"> <input type="text" name="cost[]"> <input type="text" name="cost[]">
-
And what have you tried to debug the issue? Did you echo the query to the page to verify that the query is what you expect? Are there any results or just incorrect results? We have no idea what is in your database and what you expect to be returned. You never stated that the two values are supposed to come from the GET array, that was an assumption that thorpe made. Is that the case? Where are $GF and $Year supposed to be defined? In any event the logic in your code is horribly inefficient. As thorpe already stated having two IF statements makes no sense sice you are just using those values in the query anyway. The only prupose would be for validating that the values are within the proper range. And, if that's the case you only need one IF statement. You also don't need to have two sets of code for displaying the results. This won't "fix" whatevr the issue is with not gettng the correct results, but it will clean it up. But, I did add some debuggin code to help trace the problem. I also noticed that the code to write the results had some erros as well <?php //IF statement used just to validate the range of the values if ($Year = "2006" && ($GF = "1" || $GF = "2")) { $query = "SELECT reg_no, title1, incident_date, fault_rep_id, secr_recd, status FROM report WHERE GF = '" . $GF . "'AND year = '" . $Year . "'"; $result = mysql_query($query, $dblink); if ($result==false) { //Error with Query echo "The query:<br />$query<br />Produced the error:<br />".mysql_error()); } else if (mysql_num_rows($result)==0) { //No results echo "The query:<br />$query<br />Returned 0 records."; } else { //Query was successful & there were results while($myrow = mysql_fetch_assoc($result)) { echo "<tr>"; echo "<td align=\"center\"><span class=\"style2\">" . $myrow['reg_no'] . "</span></td>"; echo "<td align=\"center\"><span class=\"style2\">" . $myrow['title1'] . "</span></td>"; echo "<td align=\"center\"><span class=\"style2\">" . $myrow['incident_date'] . "</span></td>"; echo "<td><span class=\"style2\">" . $myrow['fault_rep_id'] . "</span></td>"; echo "<td align=\"center\"><span class=\"style2\">" . $myrow['secr_recd'] . "</span></td>"; echo "<td align=\"center\"><span class=\"style2\">" . $myrow['status'] . "</span></td>"; echo "</tr>"; } } } ?>
-
Another good question is why there is not error handling in that script, such as: $result = mysql_query($query, $dblink) or die ("Query:<br />$query<br />Error:<br />".mysql_error());
-
Se my post (reply #4) in this thread: http://www.phpfreaks.com/forums/index.php/topic,150765.0.html
-
That won't work since that code need to redirect in some instances. Don't use echos in that code. Simply save the responses to a variable (if there are any) and then echo that response below the form. <div class="login"> <?php if ($_SESSION['is_valid'] == false) { if (isset($_POST['login'])) { $user_name = mysql_real_escape_string($_POST["user_name"]); $user_password = mysql_real_escape_string($_POST["user_password"]); $cookiename = forumcookie; $verify_username = strlen($user_name); $verify_pass = strlen($user_password); if ($verify_pass > 0 && $verify_username > 0) { $userPswd = md5($user_password); $userpwsd = sha1($userPswd); $sql = "SELECT * FROM $user WHERE user_name='$user_name' AND user_password='$userpwsd' LIMIT 1;"; $result = mysql_query($sql) or die(mysql_error() . " in $sql"); if (mysql_num_rows($result) == 1) { $row = mysql_fetch_assoc($result); $s_userpass = serialize($userpass); $_SESSION['id_username'] = $row['user_name']; $_SESSION['id_user_password'] = $row['user_password']; $_SESSION['user_level'] = $row['userlevel']; $_SESSION['user_id'] = $row['user_id']; $_SESSION['is_valid'] = true; if (isset($_POST['remember'])) { $checked = "checked='checked'"; setcookie("uname", $_SESSION['id_username'], time() + 86400*100); setcookie("userpw", $user_password, time() + 86400*100); setcookie("check",$checked, time() + 86400*100); } header("Location:http://".$_SERVER[HTTP_HOST].$_SERVER[REQUEST_URI]); } else { $response = "Login failed. Username or Password are not correct."; } } else { $response = "<p><font style=\"font-size:small;\">You left required field(s) blank</p>"; } } $server = str_replace("?logout","",$_SERVER['PHP_SELF']); ?> <table class="log_in"><form action="<?php echo "$server"; ?>" method="post"> <th>Welcome</th> <tr><td align="center">Username:</td></tr><tr><td align="center"><input type="text" name="user_name" size="16" value="<?php echo $_COOKIE['uname']; ?>" /><br /></td></tr> <tr><td align="center">Password:</td></tr><tr><td align="center"> <input type="password" name="user_password" size="16" value="<?php echo $_COOKIE['userpw'] ?>" /><br /></td></tr> <tr><td align="center"><input type="hidden" name="login" value="true"><input type="submit" value="Submit"></td></tr> <tr><td align="centre"><input type="checkbox" name="remember" <?php echo $_COOKIE['check'] ?>> Remember Me </td></tr><tr><td align="center"><a href="register.php">[Register]</a></td></tr><tr><td align="center"><a href="forgot_password.php">[Forgot Password?]</a></td></tr></table> </form> </div> <?php echo $response; mysql_close(); } ?> </div>
-
You, as the developer, should know where your variables are coming from, what they "should" contain, and their format. So, why are you asking me? If they do not have the same number of elements then I don't have a clue what you are tring to accomplish in the code you posted - which is syntactically incorrect. It appeared to me you were trying to iterrate through both arrays simultaneously an run those queries. For example if the first array contains 1, 2, & 3 and the second array contains A, B, & C you would want queries using the combinations 1A, 2B, & 3C. However, if you want to do a matrix between the two arrays (1A, 1B, 1C, 2A, 2B, 2C, 3A, 3B, & 3C) then that is something different (which I think helraizer's code addresses). Since you didn't state what you are trying to do, I made a guess. I put this in my sig for a reason: The quality of the responses received is directly porportional to the quality of the question asked.
-
Do $niqid and $_POST['cost'] have the same number of elements? And are they numerically indexed consecutively from 0? <?php for ($i=0; $i<count($niqid); $i++) { $query = "INSERT INTO ctable (name, cost, date, post) VALUES ('".$niqid[$i]."', '".$_POST['cost'][$i]."', '$date', '$post')"; $sql = mysql_query($query) or die (mysql_error()); } ?>
-
If this is for developers then you should not need logic to parse the value based upon "unsupported" formats. The developers should simply read the documentation and program their apps accordingly. Just use two different delimeters. For example you could use a dash to delimit books and commas to delimit "bookname, chapter, verse" www.mydomain.com/index.php?ver=eng&books=genesis,1,5-matthew,1,10 In your code you could get the date like so <?php $version = $_GET_['ver']; $books = explode('-', $_GET['books']); foreach ($books as $book) { $bookData = explode(',', $book); $bookName = $bookData[0]; $bookChapt = $bookData[1]; $bookVerse = $bookData[2]; //Insert code to retrieve data from DB and do something with it } ?>