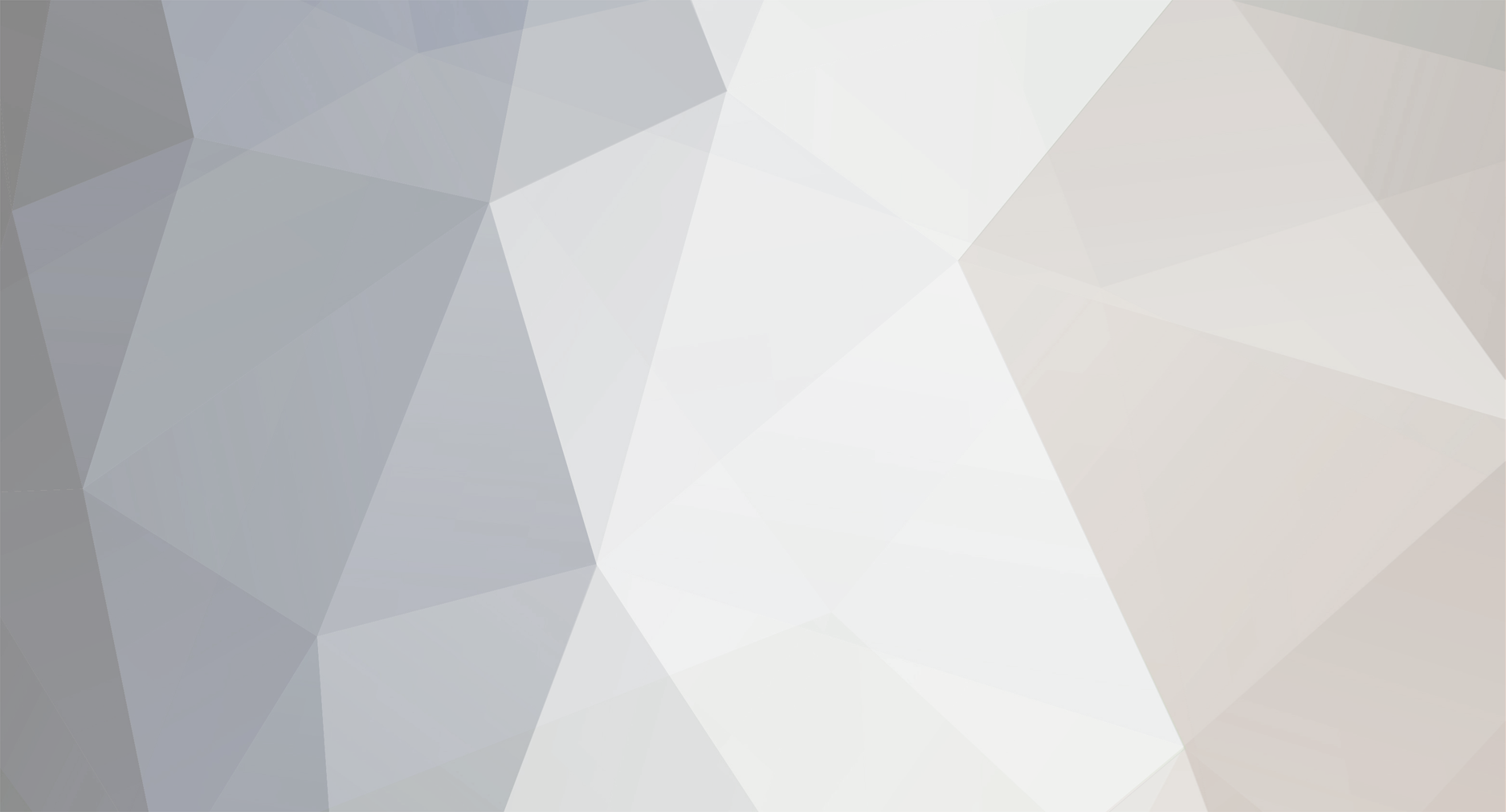
virtuexru
Members-
Posts
200 -
Joined
-
Last visited
Everything posted by virtuexru
-
not sure if i have coded my login system correctly?
virtuexru replied to yobo's topic in PHP Coding Help
First things first.. Do this in the beginning, take the $_POST, clear it, and assign variables.. It's safer and cleaner this way. $username = mysql_real_escape_string($_POST['username']); $password = mysql_real_escape_string($_POST['password']); Then, to error check, instead of doing <= 0, do this: $num_rows = mysql_num_rows($qry); if ($num_rows != '1') {} else {} Hope that helps, if not, let me know what the error is. -
Yea, down to the bottom.
-
OK. So the image/background image I want to stretch out is circled in red: Here is the code for this section in the CSS: .rightsub { background-image: url(img/rightsub.gif); min-height: 50px; } For the actual page file: <div id="navigation"> <div class="rightsub"> </div> </div> The navigation ID in question is: #navigation { float: left; width: 200px; }
-
Worked neo! I put it on top of the index, login and connect pages. Everything is perfect now, thank you.
-
Bump?!
-
Here's the connect.php file: <?php $connect = mysql_connect($dbhost, $dbuser, $dbpass); mysql_select_db($dbname) or die("Unable to select database"); ?>
-
That works but I still get the first error from the setcookie line 33.
-
Warning: Cannot modify header information - headers already sent by (output started at /home2/virtuex7/public_html/config/connect.php:4) in /home2/virtuex7/public_html/login.php on line 33 Warning: Cannot modify header information - headers already sent by (output started at /home2/virtuex7/public_html/config/connect.php:4) in /home2/virtuex7/public_html/login.php on line 47 LINE 33: setcookie ("member",$email,time()+172800,"/"); LINE 47: header("Location: profile.php");
-
Niether of those worked, still same error :|.
-
OK So I read the sticky, but I am still stuck.. any ideas? I'm logging in through index.php, before the header of index.php I have: <?php session_start(); if (isset($_POST['email']) && isset($_POST['password'])) { include('login.php'); } ?> This is my error: Warning: Cannot modify header information - headers already sent by (output started at /**/**/public_html/config/connect.php:6) in /**/**/public_html/login.php on line 36 Warning: Cannot modify header information - headers already sent by (output started at /**/**/public_html/config/connect.php:6) in /**/**/public_html/login.php on line 50 The connect.php is this: <?php $connect = mysql_connect($dbhost, $dbuser, $dbpass); mysql_select_db($dbname) or die("Unable to select database"); ?> and on the login.php is: setcookie ("member",$email,time()+172800,"/"); $member = $email; /* In-case cookies are turned off, set the session */ $_SESSION['member'] = true; /* Update login timer */ $qUpdate = "UPDATE xxx SET login = now() WHERE email='$email' AND password='$passwordencrypt' AND status='Y' "; $rsUpdate = mysql_query($qUpdate); if ($rsUpdate) { header("Location: profile.php"); }
-
Nevermind, fixed it. <? include 'config.php'; include 'opendb.php'; $username = mysql_real_escape_string($_POST['txtUserId']); $oldpw = mysql_real_escape_string($_POST['OldPassword']); $newpw = mysql_real_escape_string($_POST['NEWPW1']); $newpwv = mysql_real_escape_string($_POST['NEWPW2']); if (($newpw)!=($newpwv)) { echo "Password doesn't match. <a href=\"javascript: history.go(-1)\">Go Back</a>"; Die(); } $min_length = 6; if(strlen($newpw) < $min_length) { echo "Password not long enough, minimum 6 characters. <a href=\"javascript: history.go(-1)\">Go Back</a>"; Die(); } $sql = "SELECT user_id FROM tbl_auth_user WHERE user_id = '$username' AND user_password = OLD_PASSWORD('$oldpw')"; $result = mysql_query($sql) or die('Query failed. ' . mysql_error()); if (mysql_num_rows($result) == 1) { $encpass = sha1($newpw); $query = "UPDATE tbl_auth_user SET user_password = '$encpass' WHERE user_id = '$username'"; mysql_query($query) or die('Error, query failed'); echo "Update successful. <a href=\"http://www.-----.com/\">Home</a>."; } else { echo "Old password not correct or username does not exist. <a href=\"javascript: history.go(-1)\">Go Back</a>"; } include 'closedb.php'; ?>
-
how do I do that ? (stupid question).
-
I made a form for users to update new passwords. Any huge security flaws here? <? include 'config.php'; include 'opendb.php'; $username = $_POST['txtUserId']; $oldpw = $_POST['OldPassword']; $newpw = $_POST['NEWPW1']; $newpwv = $_POST['NEWPW2']; if (($newpw)!=($newpwv)) { echo "Password doesn't match."; Die(); } $min_length = 6; if(strlen($newpw) < $min_length) { echo "Password not long enough, minimum 6 characters."; Die(); } $sql = "SELECT user_id FROM tbl_auth_user WHERE user_id = '$username' AND user_password = OLD_PASSWORD('$oldpw')"; $result = mysql_query($sql) or die('Query failed. ' . mysql_error()); if (mysql_num_rows($result) == 1) { $newpassword = sha1($password); $query = "UPDATE tbl_auth_user SET user_password = '$newpassword' WHERE user_id = '$username'"; mysql_query($query) or die('Error, query failed'); echo "Update successful"; } else { echo "Old password not correct or username does not exist."; } include 'closedb.php'; ?>
-
OK. Well I decided to just have old users update their passwords by using a form. I'm going to be using SHA1() to encrypt the passwords now. Can you look at my update form let me know if there are any visible flaws? <? include 'config.php'; include 'opendb.php'; $username = $_POST['txtUserId']; $oldpw = $_POST['OldPassword']; $newpw = $_POST['NEWPW1']; $newpwv = $_POST['NEWPW2']; if (($newpw)!=($newpwv)) { echo "Password doesn't match."; Die(); } $min_length = 6; if(strlen($newpw) < $min_length) { echo "Password not long enough, minimum 6 characters."; Die(); } $sql = "SELECT user_id FROM tbl_auth_user WHERE user_id = '$username' AND user_password = OLD_PASSWORD('$oldpw')"; $result = mysql_query($sql) or die('Query failed. ' . mysql_error()); if (mysql_num_rows($result) == 1) { $newpassword = sha1($password); $query = "UPDATE tbl_auth_user SET user_password = '$newpassword' WHERE user_id = '$username'"; mysql_query($query) or die('Error, query failed'); echo "Update successful"; } else { echo "Old password not correct or username does not exist."; } include 'closedb.php'; ?>
-
OK. So I have a website and I recently updated to MySQL V5 from V4. My code for registering users was $request = "INSERT INTO tbl_auth_user values('$user_id',PASSWORD('$pass'),'$name')"; this way, the password would be hashed, and when logging in, I have image verification and pulling the PASSWORD('$pass') = $passwordinput code to check passwords. But now I have a problem... In MySQL 5, the PASSWORD() function creates a different type of hash, so when logging in, I can no longer use PASSWORD, I need to use OLD_PASSWORD(), so hence my new code: $request = "INSERT INTO tbl_auth_user values('$user_id',OLD_PASSWORD('$pass'),'$name')"; same for login.. My question is.. How would I go about updating the Database so that all user passwords are in the new hash format, or should I just create an if then statement when logging in to check what hash it is, how would I go about doing any of this?
-
So what am I supposed to do?
-
$request = "INSERT INTO userlist values('','$username',PASSWORD('$password'),'$email')";
-
OK. I know the username and password I'm entering is correct, I think its a problem with how the password is hashed or unhashed? Any luck with this? Here's my header code and the form code itself. Please help! <?php session_start(); $errorMessage = ''; if (isset($_POST['user_id_input']) && isset($_POST['user_pw_input'])) { include 'config/config.php'; include 'config/connect.php'; $userid = $_POST['user_id_input']; $userpw = $_POST['user_pw_input']; $authenticate = "SELECT username FROM userlist WHERE username = '$userid' AND password = PASSWORD('$userpw')"; $result = mysql_query($authenticate) or die('Query failed. ' . mysql_error()); if (mysql_num_rows($result) == 1) { // if the password/username matches, set the session $_SESSION['user_logged_in'] = true; // redirect to profile page header('Location: profile.php'); exit; } else { $errorMessage = 'Invalid Login Credentials'; } include 'config/close.php'; } ?> Form: <p/><form action="" method="post" name="Login" id="Login">Username: <input name="user_id_input" type="text" id="user_id_input" size="15"> Password: <input name="user_pw_input" type="password" id="user_pw_input" size="15"> <input name="Login" type="submit" id="Login" value="Login"></form> <p/> <?php if ($errorMessage != '') { ?> <b><font color="red"><?php echo $errorMessage; echo $result; ?></font></b> <?php } else { echo "Become a member today!"; } ?> | <a href="register.php">Register Now!</a>
-
OK. I'm getting this error: Warning: Cannot modify header information - headers already sent by (output started at /**/**/public_html/config/connect.php:7) in /**/**/public_html/config/register_user.php on line 84 For a registration page... connect.php : <?php $connect = mysql_connect($dbhost, $dbuser, $dbpass); @mysql_select_db($dbname) or die("Unable to select database"); ?> register_user.php line 84 : $request = "INSERT INTO table values('','$username',PASSWORD('$password'),'$email')"; $insert_confirm = mysql_query($request); if($insert_confirm) { $message = "<html><body>Please do not respond to this message. <p/>Congratulations, this is a confirmation of your registration submittal at PostMyDay.com. Welcome aboard!</body></html>"; mail( $email , "Registration for aaa.com Successful", $message, "From: aaa.com" ); $confirmation = "virtuexru@gmail.com"; mail ( $confirmation , "New aaa User", $username , "From: aaa.com" ); header ("Location: success.php"); Die(); } else { echo mysql_error(); echo $username; } mysql_close(); Die();
-
Great. Do you have a link to a list of these so I can check them out or any in particular? Googling didn't give me too many solid results.