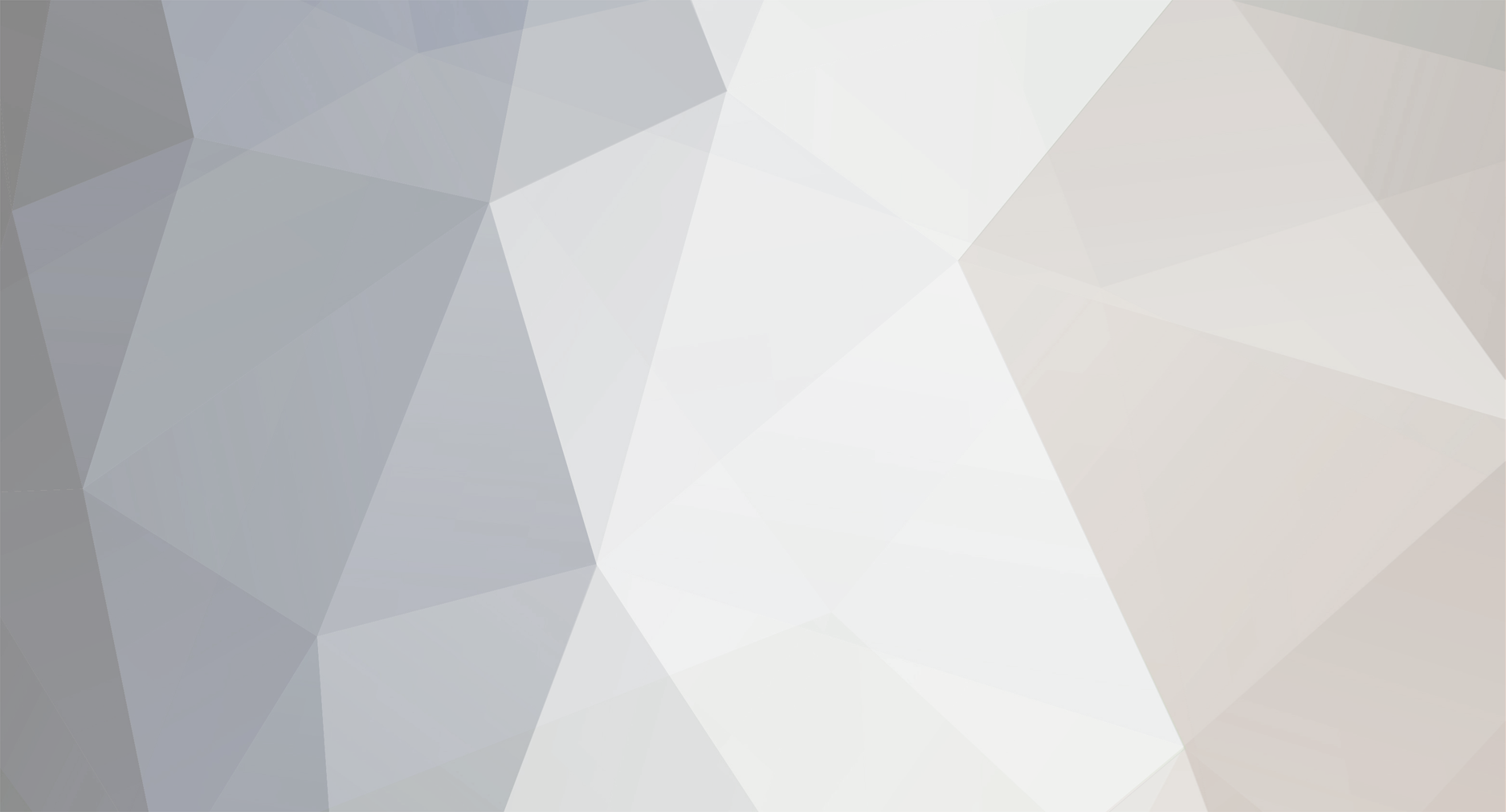
king arthur
Members-
Posts
335 -
Joined
-
Last visited
Never
Everything posted by king arthur
-
How to update 1 field in all records of db?
king arthur replied to stevens's topic in PHP Coding Help
How are the list of names defined? -
First you need to define how many rows you are going to display per page. [code] define ('ROWS_PER_PAGE', 20); [/code] for example. Then you need a page number. If we were on page two, for example, we would expect to display rows 20 - 39 (because we count from 0). So we set a start and end value. [code] $pagenum = 2; $start = ($pagenum - 1) * ROWS_PER_PAGE; $end = ROWS_PER_PAGE; [/code] Actually, $end here is just an offset. I have set the page num to two explicitly, you would need to be keeping track of it in some other way. Then in your query, you fetch only the rows you want by using a limit clause with the $start and $end values. E.g. [code] $query = "select * from blah where blahblah=something limit $start, $end"; [/code] Now you will get the rows returned that you want. Now you just display the next and previous links using the page num you have [code] echo "<a href=\"this.php?page=" . $pagenum - 1 . "\">Previous</a> | <a href=\"this.php?page=". $pagenum + 1 . "\">Next</a>"; [/code] Well, something like that anyway.
-
The other way you could get something like that to work would be to fill a javascript array with all the possible results from the query first and write some javascript along with the form, with a function that fills the textbox from the corresponding array element, which gets called from the select menu's onchange event.
-
Well, echo the three values you are testing in the if statement and see what they are.
-
Have you tried dumping the contents of the $_COOKIE array in the script where you are havig problems? E.g. print_r($_COOKIE)
-
Ah you didn't say you were running it on localhost!!! Cookies and sessions seem to behave strangely when you run scripts on localhost, I would never rely on them working properly until you test them on a remote server.
-
If $_COOKIE[$cookieName] returns blank then either there is no cookie with that name available to that script, or you have accidentally unset that element in the $_COOKIE array.
-
Sorry, try print_r($_SESSION) instead of the echo dump_array()!
-
Javascript doesn't insist on semi-colons after each statement, but the lack of the closing bracket would certainly prevent that code from running.
-
Well no. By accessing $_COOKIE[$cookieName], if $cookieName == "MyUnique" you are getting the value of a cookie found with the name "MyUnique" if there was one. The value isn't the same as the name. There will not be a corresponding key of that name in the $_SESSION array unless you set one there.
-
Try putting a line "<?php dump_array($_SESSION); ?>" between the body tags in admin.php and the same again in admin2.php and see what it outputs.
-
Where is $cookieName defined?
-
Try a left join.
-
Only thing I can think of is that by including admin.php in the login script you are calling session_start() twice but I don't know what effect that would actually have.
-
Use time() + 24 * 60 * 60
-
You can only do this if your column is a string type, and you append the values on the end of the string with some kind of separator character such as a comma or whatever else will work. But, you really should not ever be storing anything other than atomic values in table cells. It will make it impossible to search on that column. If you find the need to have multiple values in a cell, it means you have a many-to-many relationship and you should resolve this into two relationships, many-to-one and one-to-many, by creating another table (this is one of the database normalisation rules, I can't remember which one). So you should set up a new table which contains in each row, the other_user_id in one cell with the user_id of the person they relate to in another cell. Each relationship needs to use a separate row.
-
This isn't really a MySQL problem, it's down to your grasp of PHP programming. You need a while loop to loop through the results returned by the database query. First you don't need this line at all from what I can see: [code] $totalRows_Recordset1 = mysql_num_rows($Recordset1); [/code] Then you need to remove this line [code] $row_Recordset1 = mysql_fetch_assoc($Recordset1); [/code] and rework your code so it looks like this: [code] ?> Within the <body> tag: <?php echo "<table border='1'> <tr> <th>name</th> <th>desc</th> <th>price</th> <th>related1</th> <th>related2</th> <th>related3</th> </tr>"; while($row_Recordset1 = mysql_fetch_assoc($Recordset1)); { $name = $row_Recordset1['PDCT_NAME']; $desc = $row_Recordset1['PDCT_DES']; $price = $row_Recordset1['PDCT_PRICE']; $related1 = $row_Recordset1['RELATED1']; $related2 = $row_Recordset1['RELATED2']; $related3 = $row_Recordset1['RELATED3']; echo "<tr> <td>$name</td> <td>$desc</td> <td>$price</td> <td>$related1</td> <td>$related2</td> <td>$related3</td> </tr>"; } ?> [/code]
-
"SHOW TABLES" will return rows containing the table names in the database.
-
Think it may be this bit here [code] $sql2 = "SELECT * FROM shops WHERE owner = '$logged[username]'"; $result2 = mysql_query($sql2) or print ("Can't select table.<p>" . [/code] If the number of rows returned is zero, the rest of your code doesn't run. The number of rows returned may be zero because I think the query string is not interpolated in the way you want - instead try [code] $sql2 = "SELECT * FROM shops WHERE owner = '" . $logged[username] . "'"; $result2 = mysql_query($sql2) or print ("Can't select table.<p>" . [/code] Never sure about these but that's what I'd try!
-
If you already have the $start_of_month and $start_of_year variables defined then I guess: [code] $query = "select sum(total) as total_orders from order_sum where time>=$start_of_month and customer_id = ".$db->mySQLsafe($ccUserData[0]['customer_id']); $result=mysql_query($query) or die(mysql_error()); $month_total = mysql_result($result, 0); $query = "select sum(total) as total_orders from order_sum where time>=$start_of_year and customer_id = ".$db->mySQLsafe($ccUserData[0]['customer_id']); $result=mysql_query($query) or die(mysql_error()); $year_total = mysql_result($result, 0); $query = "select sum(total) as total_orders from order_sum where customer_id = ".$db->mySQLsafe($ccUserData[0]['customer_id']); $result=mysql_query($query) or die(mysql_error()); $whole_total = mysql_result($result, 0); [/code] Try that. The variables $month_total, $year_total and $whole_total should have the values you want, any typos notwithstanding as it is late on a Sunday here. BTW the use of mktime() is a little obtuse, but you can do all sorts with it. If you want total orders today, use mktime(0, 0, 0, $mon, $mday, $year). If you want everything since 9am May 17th 1995 for whatever reason, use mktime(9, 0, 0, 5, 17, 1995). The variables I used are from the keys extracted from the array made by getdate() which are $seconds, $minutes, $hours (self explanatory), $mday (day of month), $wday (day of week), $mon (month of year), $year, $yday (day of year).
-
Start of year would be [code] $start_of_year = mktime(0, 0, 0, 1, 1, $year); [/code] and all time, just leave out the where clause altogether in the query.
-
First get the components of the current time with [code] $date_array = getdate(); extract($date_array); [/code] This gives you lots of variables such as $mon, the number of the current month in the year, $mday the current day of the month etc. You can then use these to construct the date you want to count results from with mktime(). E.g. [code] $start_of_month = mktime(0, 0, 0, $mon, 1, $year); [/code] That will give you a timestamp for midnight on the first day of this month. Then query the database with this timestamp thus: [code] $query = "select sum(total) as total_orders from order_sum where time>=$start_of_month"; $result=mysql_query($query) or die(mysql_error()); $total = mysql_result($result, 0); [/code] $total is the total amount so far this month. HTH.
-
Well from the page in the manual from this very site: [quote] The argument structure of this function is a bit unusual, but flexible. The first argument has to be an array. Subsequently, each argument can be either an array or a sorting flag from the following lists. [/quote] so, although I've never used it, it would seem array_multisort() isn't quite going to do what you need.
-
Eliminating single apostraphe in echo statements?
king arthur replied to Allen4172's topic in PHP Coding Help
Where is the text coming from?