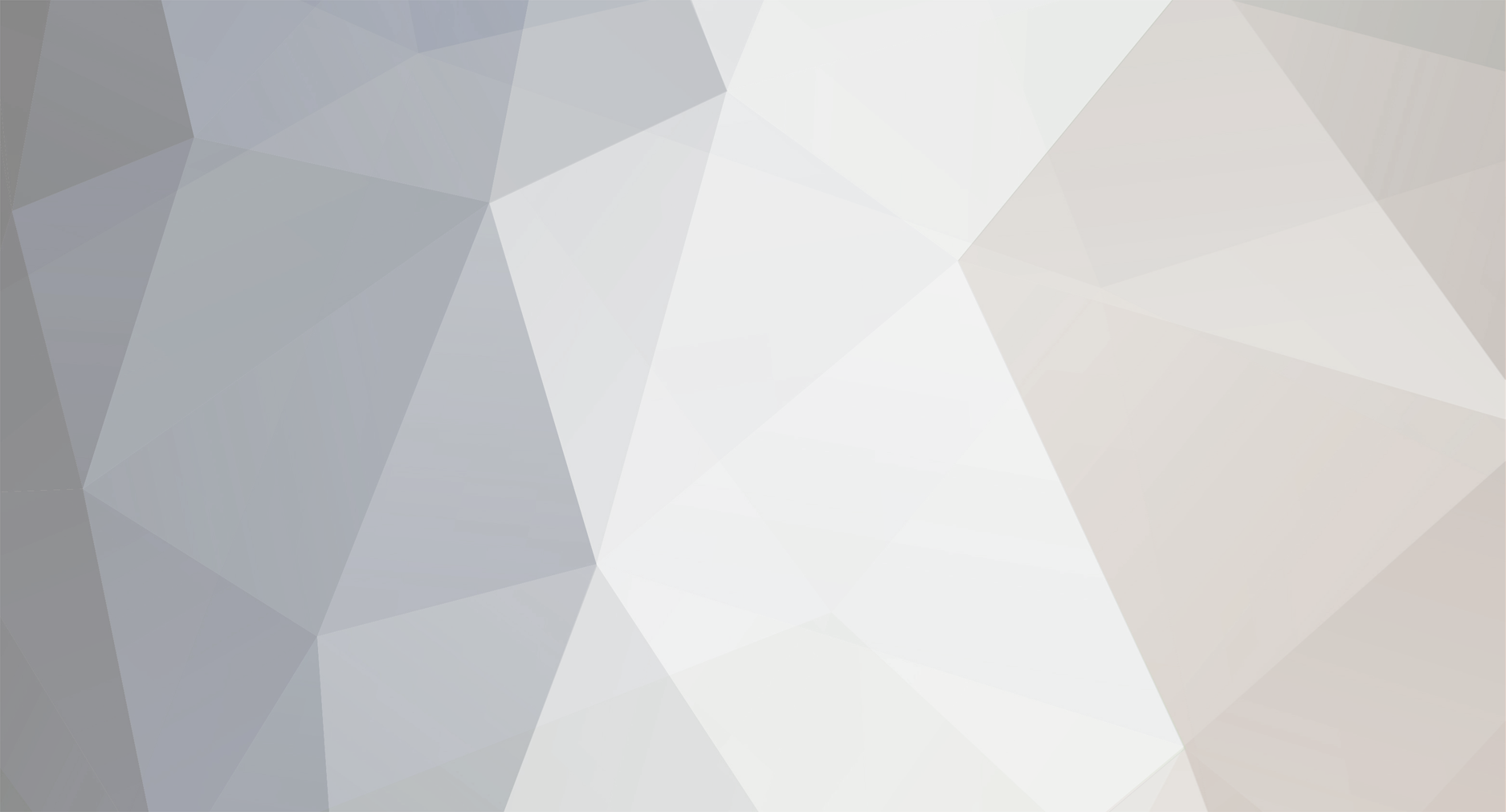
king arthur
Members-
Posts
335 -
Joined
-
Last visited
Never
Everything posted by king arthur
-
What I'm trying to establish is whether you want the browser to relocate to the script being clicked on. If so, that's easy: just add a query string on the end of the URL, e.g. [code] <a href="myscript.php?var1=1&var2=10&var3=20">My link</a> [/code] and you then get the values in the PHP script in the $_GET array, just like you get them from a form using the $_POST array. If you don't want to relocate the browser to the script, but just want to call it, there is a way to do it with Javascript (or even without the javascript but in a less useful way).
-
First, you might want to edit your post and remove the swearing before a moderator does it for you. Second, you're going to need to find a way to overcome PHP's lack of unions and pointers to convert this code. Also find out what type AnsiString is.
-
Which are the fields that do not get inserted?
-
What do you want the PHP script to actually do? Is it going to be echoing anything to the browser, or is it just updating a database? As, depending on what you want to do there is another solution, a little like Ajax but easier to do.
-
trouble comparing passwords for registration form [RESOLVED]
king arthur replied to AdRock's topic in PHP Coding Help
Actually, what's in your connection.php? Is it also using a variable named $password? -
trouble comparing passwords for registration form [RESOLVED]
king arthur replied to AdRock's topic in PHP Coding Help
Well the obvious thing to do is insert an echo to find out what the strings actually are at that point: [code] if ( $password <> $password2 ){ echo $password . "(" . strlen($password) . ")" . ", " . $password2 . "(" . strlen($password2) . ")"; $msg=$msg."Both passwords do not match<BR>"; $status= "NOTOK";} [/code] -
MySQL Error Has to do with PHP code. [Solved]
king arthur replied to dual_alliance's topic in PHP Coding Help
Looks like $conn, the connection resource, has not been set up properly. -
You'd be better off using an IF....ELSEIF construct.
-
Well, unless this is a typo and not your actual code: [code] $sizearray = count($invitearrary); [/code] you are getting the size of an array which is not defined, hence the result of zero!
-
I can never understand why people do not align their opening and closing braces! It makes it so much easier to spot errors. Example: [code] if($user == '') { $COerr_msgs->err_msgs('register1'); } else if($pass == '') { $COerr_msgs->err_msgs('register1'); } else { //compare username into database if($user_new == $user_old) { if($pass_new == $pass_old) { //check activated if set if set then proceed to authorized page. if(activate_sta == '1') { include "authorize_page.php"; } else { $COerr_msgs->err_msgs('login1'); } } else { $COerr_msgs->err_msgs('register2'); exit(); } } elseif($user_new != $user_old) { $COerr_msgs->err_msgs('activated2'); exit(); } } [/code] Identical to your code but so much easier to read! Well I think so anyway, YMMV.
-
Work out what the date will be one year from now (or one year from the time they sign up). Store that in the database. Then every time they log in, check to see if today's date is greater than the time you stored in the database. If it is, their subscription has run out.
-
Username and password are possibly reserved words in MySQL, try putting backticks around them: [code] $q = "select `password` from users where `username` = '$username'"; [/code]
-
How does the negation ~ operator work?
king arthur replied to praveenhotha's topic in PHP Coding Help
Because an unsigned 8 bit number can store up to 255. To put it another way, 2 to the power 8 is 256. It will work for any size integer. If we're using 16 bit numbers, then a signed 16 bit number goes from -32768 to +32767, and this time we are subtracting 65536 which is 2 to the power 16. Basically if you understand binary you will get it, if you don't you won't. -
No you only do that the first time you want to store it, that is the whole idea of having session variables. Do you have session_start() at the top of every page?
-
You have a call to mysql_numrows(), there's no such function. It's mysql_num_rows().
-
How does the negation ~ operator work?
king arthur replied to praveenhotha's topic in PHP Coding Help
Well not quite. A signed 8 bit number can represent values from -128 through to +127. This is because 127 is 01111111. Adding one more makes it 10000000, which now represents -128, because it is taken to mean 128 - 256. The 8th bit is the sign bit, if it is 0 then the number is positive, if it is 1 then it's negative and represents the number - 256. So if $a is 2, 00000010 in binary, and you take the NOT of that number (~ means logical NOT, or XOR with 11111111) you get 11111101 which is 253. 253 - 256 is -3. -
So basically you're saying it doesn't execute the die() function even if the passwords entered are different?
-
How to update 1 field in all records of db?
king arthur replied to stevens's topic in PHP Coding Help
Ok let's see if there is a problem with the queries, change this line [code] $result = mysql_query("SELECT id FROM details ORDER BY id"); [/code] to [code] $result = mysql_query("SELECT id FROM details ORDER BY id") or die(mysql_error()); [/code] and this line [code] $r = mysql_query("UPDATE details SET name='" . $word_array[$i++] . "' WHERE id=" . $row["id"]); [/code] to [code] $r = mysql_query("UPDATE details SET name='" . $word_array[$i++] . "' WHERE id=" . $row["id"]) or die(mysql_error()); [/code] and see what it says. -
How to update 1 field in all records of db?
king arthur replied to stevens's topic in PHP Coding Help
Couple of changes: [code] $words = $_POST['words']; $connection = mysql_connect($hostname, $user, $pass) or die ("Unable to connect!"); mysql_select_db($db, $connection); if ($words) { $word_array = explode(",", $words); $result = mysql_query("SELECT id FROM details ORDER BY id"); $i = 0; while($row = mysql_fetch_assoc($result)) { $r = mysql_query("UPDATE details SET name='" . $word_array[$i++] . "' WHERE id=" . $row["id"]); } } [/code] I forgot to increment $i and forgot the single quotes around the word. If your column in the details table is called "name" then it is "SET name='" in the query, not "SET word='". -
How to update 1 field in all records of db?
king arthur replied to stevens's topic in PHP Coding Help
[code] $names_array = explode(",", $names); $result = mysql_query("select id from mytable order by id"); $i = 0; while($row = mysql_fetch_assoc($result)) { $r = mysql_query("update mytable set name=" . $names_array[$i] . " where id=" . $row["id"]); } [/code] See if that works. Obviously change "mytable" to the name of your table. -
The problem is with your logic here [code] $result = MYSQL_QUERY("SELECT * from registration WHERE username='$username' and password='$password'") or die ("Name and password not found or not matched"); $worked = mysql_fetch_array($result); [/code] The query would only fail if there was an error, not if the matching name and password were not found. The query might easily work but not return any matched rows. So you need to count how many rows were returned, if it was none then the username and password have no match. [code] $result = MYSQL_QUERY("SELECT * from registration WHERE username='$username' and password='$password'") or die ("Name and password not found or not matched"); $numofrows = mysql_num_rows($result); $worked = mysql_fetch_array($result); if($numofrows == 0) { // no match found } [/code]
-
How to update 1 field in all records of db?
king arthur replied to stevens's topic in PHP Coding Help
Ok, well are all the id fields in contiguous order? Then you could do it as ToonMariner says, with a loop incrementing the id for each query. If not, then pull all the id's out first and then update the rows with names for each id you pulled out. -
And the error you are getting is?
-
Yes, but that would mean having to learn Ajax! The user need not see anything, it really all depends on how many options there are. If it was, say, only 10 or 20, it's not a big deal to write out a javascript array with those 10 or 20 elements.