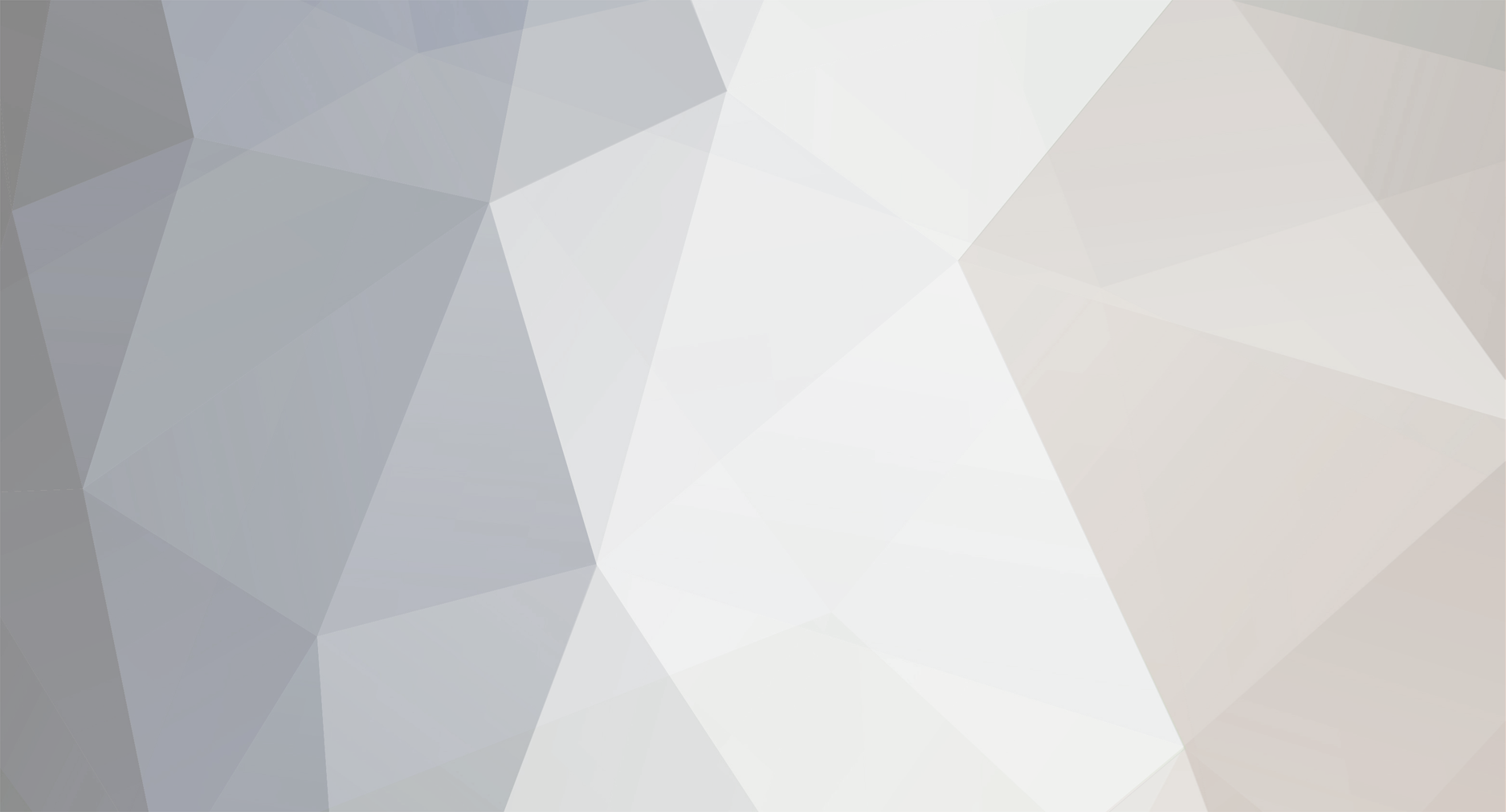
aebstract
-
Posts
1,105 -
Joined
-
Last visited
Posts posted by aebstract
-
-
The entire script is posted at the top, here it is with the most recent updates:
<?php // Copyright 2007, FedEx Corporation. All rights reserved. // Version 2.0.0 chdir('..'); require_once('library/fedex-common.php5'); $newline = "<br />"; ini_set("soap.wsdl_cache_enabled", "0"); $client = new SoapClient('http://www.berryequipment.net/wsdl/RateService_v2.wsdl', array('trace' => 1)); // replace with valid path to WSDL $request['WebAuthenticationDetail'] = array('UserCredential' => array('Key' => 'YXSO2j7gNqcJsOSs', 'Password' => 'zcuAEs7HNaI2j2Wnlaa1Nw3G2')); // Replace 'XXX' and 'YYY' with FedEx provided credentials $request['ClientDetail'] = array('AccountNumber' => '510087380', 'MeterNumber' => '1214194');// Replace 'XXX' with your account and meter number $request['TransactionDetail'] = array('CustomerTransactionId' => ' *** Rate Request v2 using PHP ***'); $request['Version'] = array('ServiceId' => 'crs', 'Major' => '2', 'Intermediate' => '0', Minor => '0'); $request['Origin'] = array('StreetLines' => array('6736 Cleveland Hwy'), // Origin details 'City' => 'Clermont', 'StateOrProvinceCode' => 'GA', 'PostalCode' => '30527', 'CountryCode' => 'US'); $request['Destination'] = array('StreetLines' => array('$street'), // Destination details 'City' => '$city', 'StateOrProvinceCode' => '$state', 'PostalCode' => '$zip', 'CountryCode' => 'US'); $request['Payment'] = array('PaymentType' => 'THIRD_PARTY'); // valid codes RECIPIENT, SENDER and THIRD_PARTY $request['DropoffType'] = 'REGULAR_PICKUP'; // valid codes BUSINESS_SERVICE_CENTER, DROP_BOX, REGULAR_PICKUP, REQUEST_COURIER and STATION $request['ServiceType'] = 'FEDEX_GROUND'; // valid codes STANDARD_OVERNIGHT, PRIORITY_OVERNIGHT, FEDEX_GROUND, ... $request['PackagingType'] = 'YOUR_PACKAGING'; // valid codes FEDEX_BOK, FEDEX_PAK, FEDEX_TUBE, YOUR_PACKAGING, ... $request['ShipDate'] = date('Y-m-d'); $request['RateRequestTypes'] = 'ACCOUNT'; // valid codes ACCOUNT, LIST $PassRateRequestPackageSummary = true; if ($PassRateRequestPackageSummary) { $request['RateRequestPackageSummary'] = array( 'TotalWeight' => array( 'Value' => 22.0, 'Units' => 'LB' ), 'TotalInsuredValue' => array( 'Amount' => $total, 'Currency' => 'USD' ), 'PieceCount' => $quan // there was an error here ); } else { $request['PackageCount'] = 1; $request['Packages'] = array( 0 => array( 'Weight' => array( 'Value' => 22.0, 'Units' => 'LB' ), 'InsuredValue' => array( 'Amount' => $total, 'Currency' => 'USD' // error here as well ) ) ); } try { $response = $client ->__soapCall("getRate", array('parameters' => $request)); if ($response -> HighestSeverity != 'FAILURE' && $response -> HighestSeverity != 'ERROR') { printRequestResponse($client); } else { echo 'Error in processing transaction.'. $newline. $newline; foreach ($response -> Notifications as $notification) { if(is_array($response -> Notifications)) { echo $notification -> Severity; echo ': '; echo $notification -> Message . $newline; } else { echo $notification . $newline; } } } writeToLog($client); // Write to log file } catch (SoapFault $exception) { printFault($exception, $client); } ?>
-
I've been trying.. many many times. Constantly throughout the day. I've gotten more help in an hour here than I have in several days of trying to contact them. I got a response back from a developer that said: "Are you rating dry ice?" I would much rather just get help here and get the code fixed. I understand there is a "3rd party" section to the forums, which hardly gets used. I looked and if I was to post this there, I would probably get a response once a day, meaning the problems would get solved in a week or two. Much better responses and response times here. If im not welcome here, okay but I hope that isn't the truth as I want really need to get this code working.
-
I agree with formatting it better, it was code from fedex developers ;( With that said, I'm kinda new to arrays and trying to work through their code but it's rather difficult for me.
Parse error: parse error, unexpected '{' in /home/virtual/site130/fst/var/www/html/Rate.php5 on line 67
After changing what you said, hitman. I am left with this error, line 67 beginning below
try { $response = $client ->__soapCall("getRate", array('parameters' => $request)); if ($response -> HighestSeverity != 'FAILURE' && $response -> HighestSeverity != 'ERROR') { printRequestResponse($client); } else { echo 'Error in processing transaction.'. $newline. $newline; foreach ($response -> Notifications as $notification) { if(is_array($response -> Notifications)) { echo $notification -> Severity; echo ': '; echo $notification -> Message . $newline; } else { echo $notification . $newline; } } } writeToLog($client); // Write to log file } catch (SoapFault $exception) { printFault($exception, $client); } ?>
-
-
Now it's:
Parse error: parse error, unexpected ';', expecting ')' in /home/virtual/site130/fst/var/www/html/Rate.php5 on line 51
-
Okay, now when I get those closed (correct me if I did it wrong) I'm getting an error about the next line:
} else { // Passing single piece shipment rate request $request['PackageCount'] = 1; // currently only one occurrence of RequestedPackage is supported $request['Packages'] = array(0 => array('Weight' => array('Value' => 22.0, 'Units' => 'LB'), // valid code LB and KG 'InsuredValue' => array('Amount' => $total, 'Currency' => 'USD'); }
Parse error: parse error, unexpected '}', expecting ')' in /home/virtual/site130/fst/var/www/html/Rate.php5 on line 52
Line 52 being the one with the }
If I remove the ; on 51, it gives me the error of expecting ; on 51 >.<
-
Parse error: parse error, unexpected '}', expecting ')' in /home/virtual/site130/fst/var/www/html/Rate.php5 on line 45
Here is all the code:
<?php // Copyright 2007, FedEx Corporation. All rights reserved. // Version 2.0.0 chdir('..'); require_once('library/fedex-common.php5'); $newline = "<br />"; ini_set("soap.wsdl_cache_enabled", "0"); $client = new SoapClient('http://www.berryequipment.net/wsdl/RateService_v2.wsdl', array('trace' => 1)); // replace with valid path to WSDL $request['WebAuthenticationDetail'] = array('UserCredential' => array('Key' => 'YXSO2j7gNqcJsOSs', 'Password' => 'zcuAEs7HNaI2j2Wnlaa1Nw3G2')); // Replace 'XXX' and 'YYY' with FedEx provided credentials $request['ClientDetail'] = array('AccountNumber' => '510087380', 'MeterNumber' => '1214194');// Replace 'XXX' with your account and meter number $request['TransactionDetail'] = array('CustomerTransactionId' => ' *** Rate Request v2 using PHP ***'); $request['Version'] = array('ServiceId' => 'crs', 'Major' => '2', 'Intermediate' => '0', Minor => '0'); $request['Origin'] = array('StreetLines' => array('6736 Cleveland Hwy'), // Origin details 'City' => 'Clermont', 'StateOrProvinceCode' => 'GA', 'PostalCode' => '30527', 'CountryCode' => 'US'); $request['Destination'] = array('StreetLines' => array('$street'), // Destination details 'City' => '$city', 'StateOrProvinceCode' => '$state', 'PostalCode' => '$zip', 'CountryCode' => 'US'); $request['Payment'] = array('PaymentType' => 'THIRD_PARTY'); // valid codes RECIPIENT, SENDER and THIRD_PARTY $request['DropoffType'] = 'REGULAR_PICKUP'; // valid codes BUSINESS_SERVICE_CENTER, DROP_BOX, REGULAR_PICKUP, REQUEST_COURIER and STATION $request['ServiceType'] = 'FEDEX_GROUND'; // valid codes STANDARD_OVERNIGHT, PRIORITY_OVERNIGHT, FEDEX_GROUND, ... $request['PackagingType'] = 'YOUR_PACKAGING'; // valid codes FEDEX_BOK, FEDEX_PAK, FEDEX_TUBE, YOUR_PACKAGING, ... $request['ShipDate'] = date('Y-m-d'); $request['RateRequestTypes'] = 'ACCOUNT'; // valid codes ACCOUNT, LIST $PassRateRequestPackageSummary = true; if ($PassRateRequestPackageSummary) { // Passing multi piece shipment rate request (by setting PieceCount > 1) $request['RateRequestPackageSummary'] = array('TotalWeight' => array('Value' => 22.0, 'Units' => 'LB'), // valid codes LB and KG 'TotalInsuredValue' => array('Amount' => $total, 'Currency' => 'USD'), 'PieceCount' => $quan, } else { // Passing single piece shipment rate request $request['PackageCount'] = 1; // currently only one occurrence of RequestedPackage is supported $request['Packages'] = array(0 => array('Weight' => array('Value' => 22.0, 'Units' => 'LB'), // valid code LB and KG 'InsuredValue' => array('Amount' => $total, 'Currency' => 'USD'), } try { $response = $client ->__soapCall("getRate", array('parameters' => $request)); if ($response -> HighestSeverity != 'FAILURE' && $response -> HighestSeverity != 'ERROR') { printRequestResponse($client); } else { echo 'Error in processing transaction.'. $newline. $newline; foreach ($response -> Notifications as $notification) { if(is_array($response -> Notifications)) { echo $notification -> Severity; echo ': '; echo $notification -> Message . $newline; } else { echo $notification . $newline; } } } writeToLog($client); // Write to log file } catch (SoapFault $exception) { printFault($exception, $client); } ?>
This part is where the line is and I'm sure the problem occurs:
if ($PassRateRequestPackageSummary) { // Passing multi piece shipment rate request (by setting PieceCount > 1) $request['RateRequestPackageSummary'] = array('TotalWeight' => array('Value' => 22.0, 'Units' => 'LB'), // valid codes LB and KG 'TotalInsuredValue' => array('Amount' => $total, 'Currency' => 'USD'), 'PieceCount' => $quan, } else { // Passing single piece shipment rate request $request['PackageCount'] = 1; // currently only one occurrence of RequestedPackage is supported $request['Packages'] = array(0 => array('Weight' => array('Value' => 22.0, 'Units' => 'LB'), // valid code LB and KG 'InsuredValue' => array('Amount' => $total, 'Currency' => 'USD'), } try {
This last bit of code starts on line 39
-
Two spots, can I not have a $weight.0? Since I am putting in a value of "##" and adding the .0 on?
-
This is from the sample Rate.php5 code that I have received from fedex. I have input my fields so that I can get the information they need, to them. I will show you where I include it in to my file, the error and the php file that is being included.
My include
include ('Rate.php5');
Error
Parse error: parse error, unexpected T_DNUMBER, expecting ')' in /home/virtual/site130/fst/var/www/html/Rate.php5 on line 42
Rate.php5
<?php // Copyright 2007, FedEx Corporation. All rights reserved. // Version 2.0.0 chdir('..'); require_once('library/fedex-common.php5'); $newline = "<br />"; ini_set("soap.wsdl_cache_enabled", "0"); $client = new SoapClient('wsdl/RateService_v2.wsdl', array('trace' => 1)); // replace with valid path to WSDL $request['WebAuthenticationDetail'] = array('UserCredential' => array('Key' => 'YXSO2j7gNqcJsOSs', 'Password' => 'zcuAEs7HNaI2j2Wnlaa1Nw3G2')); // Replace 'XXX' and 'YYY' with FedEx provided credentials $request['ClientDetail'] = array('AccountNumber' => '510087380', 'MeterNumber' => '1214194');// Replace 'XXX' with your account and meter number $request['TransactionDetail'] = array('CustomerTransactionId' => ' *** Rate Request v2 using PHP ***'); $request['Version'] = array('ServiceId' => 'crs', 'Major' => '2', 'Intermediate' => '0', Minor => '0'); $request['Origin'] = array('StreetLines' => array('6736 Cleveland Hwy'), // Origin details 'City' => 'Clermont', 'StateOrProvinceCode' => 'GA', 'PostalCode' => '30527', 'CountryCode' => 'US'); $request['Destination'] = array('StreetLines' => array('$street'), // Destination details 'City' => '$city', 'StateOrProvinceCode' => '$state', 'PostalCode' => '$zip', 'CountryCode' => 'US'); $request['Payment'] = array('PaymentType' => 'THIRD_PARTY'); // valid codes RECIPIENT, SENDER and THIRD_PARTY $request['DropoffType'] = 'REGULAR_PICKUP'; // valid codes BUSINESS_SERVICE_CENTER, DROP_BOX, REGULAR_PICKUP, REQUEST_COURIER and STATION $request['ServiceType'] = 'FEDEX_GROUND'; // valid codes STANDARD_OVERNIGHT, PRIORITY_OVERNIGHT, FEDEX_GROUND, ... $request['PackagingType'] = 'YOUR_PACKAGING'; // valid codes FEDEX_BOK, FEDEX_PAK, FEDEX_TUBE, YOUR_PACKAGING, ... $request['ShipDate'] = date('Y-m-d'); $request['RateRequestTypes'] = 'ACCOUNT'; // valid codes ACCOUNT, LIST $PassRateRequestPackageSummary = true; if ($PassRateRequestPackageSummary) { // Passing multi piece shipment rate request (by setting PieceCount > 1) $request['RateRequestPackageSummary'] = array('TotalWeight' => array('Value' => $weight.0, 'Units' => 'LB'), // valid codes LB and KG 'TotalInsuredValue' => array('Amount' => $total, 'Currency' => 'USD'), 'PieceCount' => $quan, } else { // Passing single piece shipment rate request $request['PackageCount'] = 1; // currently only one occurrence of RequestedPackage is supported $request['Packages'] = array(0 => array('Weight' => array('Value' => $weight.0, 'Units' => 'LB'), // valid code LB and KG 'InsuredValue' => array('Amount' => $total, 'Currency' => 'USD'), } try { $response = $client ->__soapCall("getRate", array('parameters' => $request)); if ($response -> HighestSeverity != 'FAILURE' && $response -> HighestSeverity != 'ERROR') { printRequestResponse($client); } else { echo 'Error in processing transaction.'. $newline. $newline; foreach ($response -> Notifications as $notification) { if(is_array($response -> Notifications)) { echo $notification -> Severity; echo ': '; echo $notification -> Message . $newline; } else { echo $notification . $newline; } } } writeToLog($client); // Write to log file } catch (SoapFault $exception) { printFault($exception, $client); } ?>
-
If you mean going to view > page source, that won't work since php doesn't show up. You'll have to have ftp access or access to a control panel to login and download the file you need.
-
header("Location:productsearch.php?var=productnumber&message=$message1");
If you are passing multiple variables in such a way, use an & sign instead of the ? between each variable. That should fix your problem.
-
This might just be what I need, I am going to try and get this working with my information and see if it solves my problem. Will update topic with either a response of help or topic solved. Thanks!
-
<table width=732 cellpadding=3><tr bgcolor=#8d8d8d cellspacing=6><td width=10 align=center>LOC</td><td width=100>Part Number</td><td width=330>Description</td><td>Price</td><td>QTY</td></tr><tr cellspacing=6><td align=center bgcolor="#c0c0c0">1</td><td bgcolor="#c0c0c0" width="100">APH-FR-9908</td><td bgcolor="#c0c0c0" width="330">SAW MOUNTING FRAME (L/R)</td><td bgcolor="#c0c0c0">5.99</td><td bgcolor="#c0c0c0">12</td></tr><tr cellspacing=6><td align=center bgcolor="#dddddd">2</td><td bgcolor="#dddddd" width="100">WBPH-FR-9921</td><td bgcolor="#dddddd" width="330">GUIDE BAR FRAME</td><td bgcolor="#dddddd">3.54</td><td bgcolor="#dddddd">67</td></tr><tr cellspacing=6><td align=center></td><td></td><td></td><td bgcolor=yellow>309.06</td><td></td></tr></table><br /><br /> <u>Tyson Foods - Clarksville, AR</u>, Terry Cantwell<br /> street<br /> City, STATE zip<br /> 770-770-770 <br /><br /> -770<br /><br /> 450545054505<br /> saturday<br />
This is exactly what the email looks like, obviously not as pretty as I was expecting!
$emailbody = " $parts<br /><br /> <u>$plantloc</u>, $name<br /> $address2<br /><br /> $telephone<br /><br /> $ponumber<br /> $shipping<br /> $notes "; mail('email@email.com', 'Order Sheet', $emailbody);
-
$_SESSION['telephone'] = "$_POST[phonenumber1]-$_POST[phonenumber2]-$_POST[phonenumber3]";
It is a string, is it not? I tried piecing it together with . ' ' . and didn't get a result. With the above, it isn't outputting anything.
-
Eh that makes sense, put a dash in between each instead of subtract?
-
-835
This is the output of me entering a phone number in to my 3 field area. It works if I echo each post variable, but when I try to echo my session it displays the above.
The form piece:
<input type=\"text\" size=\"3\" name=\"phonenumber1\" value=\"$_POST[phonenumber1]\" /> <input type=\"text\" size=\"3\" name=\"phonenumber2\" value=\"$_POST[phonenumber2]\" /> <input type=\"text\" size=\"3\" name=\"phonenumber3\" value=\"$_POST[phonenumber3]\" />
The session being set:
$_SESSION['telephone'] = $_POST['phonenumber1'] - $_POST['phonenumber2'] - $_POST['phonenumber3'];
-
MYSQL_QUERY("INSERT INTO users (client) WHERE username=$username". "VALUES ('$client')") or die (mysql_error());
Should work
-
wow... I swear I tried that like 3 times, but it worked.
-
for ($day = 1; $day <= $month_days; $day++) { echo "<font>$day/$month/$year</font>"; }
Updated code to go along with p2grace's code. If you put his first, this will do what you want.
-
for ($day = 1; $day <= 31; $day++) { echo "<font>$day/4/2009</font>"; } Something like that will work. To determine how many days it does, you change the 31. Need a little script that takes the set month and sets a variable for that 31 depending on the month.
-
$result2 = mysql_query("SELECT * FROM parts ORDER BY id ASC") or DIE(mysql_error()); while($r2=mysql_fetch_array($result2)) { $pid=$r2["id"]; $loc=$r2["LOC"]; $pn=$r2["PN"]; $desc=$r2["DESC"]; $price=$r2["PRICE"]; $row_color = ($row_count % 2) ? $color1 : $color2; $quan = ($_SESSION['cart'][$pid] < 1) ? "0" : $_SESSION['cart'][$pid]; if ($quan > 0){ $parts .= "<tr cellspacing=6><td align=center bgcolor=\"$row_color\">$loc</td><td bgcolor=\"$row_color\" width=\"100\">$pn</td><td bgcolor=\"$row_color\" width=\"330\">$desc</td><td bgcolor=\"$row_color\">$price</td><td bgcolor=\"$row_color\">$quan</td></tr>"; $total = "$total + ($price * $quan)"; } $row_count++; } $parts .= "<tr cellspacing=6><td align=center></td><td></td><td></td><td>$total</td><td></td></tr>"; $parts .= "</table><p align=right>check out</p></form>";
The equation in there should be taking the qty and multiplying it by the price for each row that has a qty > 0. Then add that amount to the amount of the next row that does the same thing.. etc etc all the way to the end which will result in a final price. Right now it displays like this:
+ (5.99 * 15) + (0 * 16) + (0 * 16) + (0 * 16) + (0 * 9) + (0 * 4) + (0 * 556)
instead of displaying the answer, any reasons for this? or better ways of approaching this even?
-
Perfect, that second way is definitely a better and cleaner way of doing it. Thanks for your help.
-
$sheet = $_GET['sheet']; mysql_connect("localhost","berryequipment","gU8Kso8Y") or die(mysql_error()); mysql_select_db("berryequipment_net"); $id = $_SESSION["id"]; $result = mysql_query("SELECT * FROM plants WHERE id='$id'") or DIE(mysql_error()); while($r=mysql_fetch_array($result)) { $id=$r["id"]; $plantloc=$r["plantloc"]; $address=$r["address"]; $ph=$r["PH"]; $fp=$r["FP"]; if (!$$sheet == 0) { header("Location: acchome.php"); exit; } }
In my db FP = 0 and PH = 1, if I go to order.php?sheet=PH it works, FP works too.. it isn't kicking me back to acchome.php
-
go to your forums and right click it > properties.
closing out an array? I think..
in PHP Coding Help
Posted
Parse error: parse error, unexpected '{' in /home/virtual/site130/fst/var/www/html/Rate.php5 on line 67