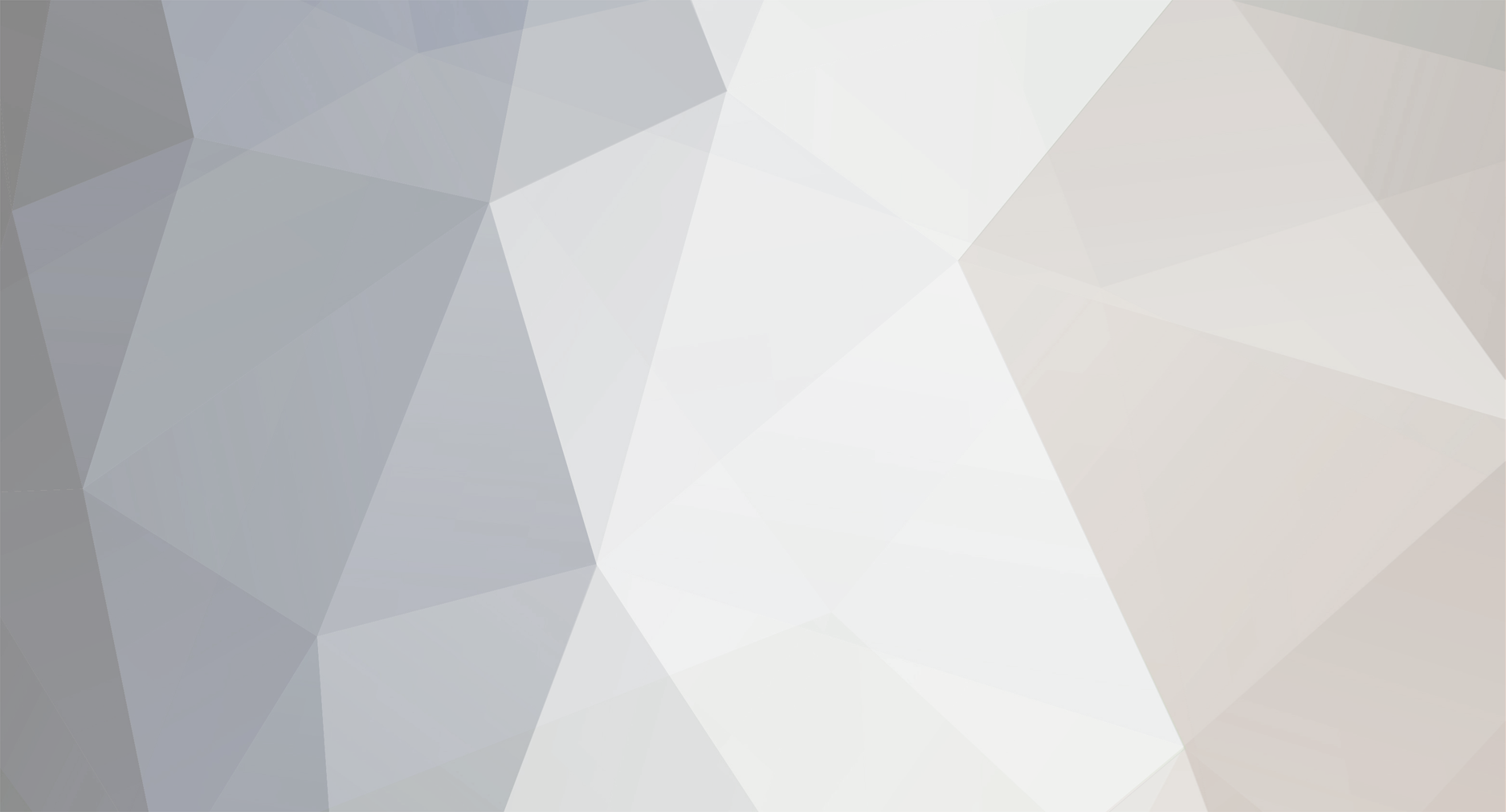
aebstract
-
Posts
1,105 -
Joined
-
Last visited
Posts posted by aebstract
-
-
I have been working around looking for the problem still and have come to a more solid conclusion that the id variable isn't being passed from that drop down to my other part of the script. I changed the error coding like you said, and it IS displaying the error of "password doesn't match". Which proves that the ID isn't passing correctly even more.
-
Still has an issue with logging in, was just putting an update on how it is displaying the drop down in case someone wanted to point the for loop as an issue.
Here is the code that is trying to connect and match up the variable, maybe it will help out:
$result = mysql_query("SELECT id, plantloc, password, address FROM plants WHERE id=('$_POST[id]') AND password=('".md5($_POST['password'])."')") or die ("error"); if (mysql_num_rows($result) == 0) { $echo = 'The pasword you entered did not match the plant location you chose. <br />'; } else { $worked = mysql_fetch_array($result); $_SESSION["id"] = $worked[id]; header("Location: acchome.php"); }
-
Update: I took out the 'for loop', it wasn't necessary and it was displaying multiples of each option. Now the drop down is displaying one of each row from the database.
-
Trying to a get login function to work. I have gone through several areas of the code to try and limit the problem to one specific area. I'm passing up the password fine, but I think the 'id' from the database isn't getting passed up from the drop down. I put an echo in the top to display the id and when I hit submit, nothing displays. ???
the code:
<select name="dropdown">'; mysql_connect("localhost","berryequipment","gU8Kso8Y") or die(mysql_error()); mysql_select_db("berryequipment_net"); $result = mysql_query("SELECT * FROM plants ORDER BY plantloc ASC") or DIE(mysql_error()); $allplants = mysql_num_rows($result); while ($r=mysql_fetch_array($result)) { $id=$r["id"]; $plantloc=$r["plantloc"]; for ($op = 1; $op <= $allplants; $op++) { $content .= "<option value=\"$id\">$plantloc</option>\n"; } } $content .= '</select>
-
Okay, sorry I keep having these issues. I think my whole drop down code is pretty messed up right now. So basically I should be able to login with this script, which is pulling the plant locations and then checking with what password they put in. I don't think my drop down is passing the information through when you hit submit. It needs to let me know what id is being chosen from the drop down and then I am going to compare that to that plants id/password in the database.
mysql_connect("localhost","berryequipment","gU8Kso8Y") or die(mysql_error()); mysql_select_db("berryequipment_net"); $result = mysql_query("SELECT * FROM plants ORDER BY plantloc ASC") or DIE(mysql_error()); $options=""; while ($r=mysql_fetch_array($result)) { $id=$r["id"]; $plantloc=$r["plantloc"]; $options .= "<OPTION VALUE=\"$id\">".$plantloc; } ?> <form action="account.php" method="post"> plant loc<br /> <SELECT NAME=dropdown> <OPTION VALUE=0> <?=$options?> </SELECT>
-
I'm not following you? I know how the drop down works, but I am trying to generate the content for the drop down from my mysql table. None of this information will be presented directly by me but rather directly from the database.
-
Alright, I am generating content for a drop down based on the information that I have stored in a database. It is very basic information, it is a table with "id", "plantloc", "password", and "address". What I want to do is generate a drop down that contains every plant. It should reference by id and should display as "plantloc". The plantloc is a nice looking description of the location, this way the user chooses their location and continues to login.
Here is what I have:
<?php mysql_connect("localhost","berryequipment","gU8Kso8Y") or die(mysql_error()); mysql_select_db("berryequipment_net"); $result = mysql_query("SELECT * FROM plants ORDER BY plantloc ASC") or DIE(mysql_error()); $options=""; while ($row=mysql_fetch_array($result)) { $id=$r["id"]; $plantloc=$r["plantloc"]; $options .= "<OPTION VALUE=\"$id\">".$plantloc; } ?> <form action="account.php?menu=login" method="post"> plant loc<br /> <SELECT NAME=dropdown> <OPTION VALUE=0> <?=$options?> </SELECT> <br /><br /> password <br /> <input type="password" maxlength="6" class="textfield" name="password" size="6" /><br /><br /> <input type="submit" name="submit" class="textfield" value="login" /> </form>
-
Got this problem solved. It turns out that the fact that we have our email server and web server set up on two different servers/accounts I had to get him to fix some settings on their end for it to actually send out. Thanks for the help and sorry for the bother!
-
I'm back on the phone right now, will ask and I found this in my php.ini:
[mail function] ; For Win32 only. SMTP = localhost ; For Win32 only. sendmail_from = me@localhost.com ; For Unix only. You may supply arguments as well (default: "sendmail -t -i"). sendmail_path = /usr/sbin/sendmail -t -i
I'm thinking it may be wrong "me@localhost.com" and I'm on a .net domain. So maybe it isn't sending out correctly? I'll come back with another update soon.
-
I didn't get connected to the same tech. but the guy I talked to had me go run a command line: "telnet berryequipment.net 25" which showed the result of:
220 hs25.order-vault.net ESMTP Sendmail 8.11.6/8.11.6; Thu, 17 Jan 2008 12:27:00
-0500
-
Alright I called in because I looked at my error log and had nothing dealing with mail in it. Couldn't find my php.ini file so he placed one in my html directory. I don't know anything about the php.ini file so I have no clue how to find what I need or check what it should be. He did a simple one line mail function test and said it was working so it may be my syntax?
-
Correct about that part, got the information inserting now. How about the email not sending? It still isn't (if it seems to look correct, I'm gonna call up my provider and see if sendmail is activated).
-
Hi again, back so soon! I put a else die on the end of my connecting to DB.. so I am 99% sure that isn't my problem here. No information is being placed in the database and no email is being sent out. Any ideas on why not?
<?php session_start(); header("Cache-control: private"); if (isset ($_POST['submit'])) { $problem = FALSE; if (empty ($_POST['plantloc'])) { $problem = TRUE; $error .= 'Must enter a plant location<br />'; } mysql_connect("*","*","*"); mysql_select_db("*"); if (!$problem) { $plantloc = $_POST['plantloc']; $length = 6; $password = ""; $possible = "0123456789bcdfghjkmnpqrstvwxyz"; $i = 0; while ($i < $length) { $char = substr($possible, mt_rand(0, strlen($possible)-1), 1); if (!strstr($password, $char)) { $password .= $char; $i++; } } $password2 = md5($_POST['password1']); $address = $_POST['address']; $result = MYSQL_QUERY("INSERT INTO plants (plantloc,password,address)". "VALUES ('$plantloc, '$password2', '$address,')"); $sendmail = " $password2 "; mail ('tcantwell@berryequipment.net', 'Thank You', $sendmail, 'From: aebstract@gmail.com'); } else { $content .= "$error"; } } ?>
-
thanks sorry I looked right past that.
-
? You didn't see this line?
Plant Location: <br /><input type="text" maxlength="100" class="textfield" name="username" size="40" value="' . $_POST[plantloc] . '" /><br /><br />
-
Hey guys, been awhile since I have been around these parts, but I am having a little trouble.
I got a form with two simple inputs. I put a check at the top of my page to see if the field has information entered or not. Well it is returning false when it has information and when it doesn't.
Here is what I have: (file - account2.php)
<?php session_start(); header("Cache-control: private"); if (isset ($_POST['submit'])) { $problem = FALSE; if (empty ($_POST['plantloc'])) { $problem = TRUE; $error .= 'Must enter a plant location<br />'; } mysql_connect("*","*","*"); mysql_select_db("*"); if (!$problem) { $plantloc = $_POST['plantloc']; $length = 6; $password = ""; $possible = "0123456789bcdfghjkmnpqrstvwxyz"; $i = 0; while ($i < $length) { $char = substr($possible, mt_rand(0, strlen($possible)-1), 1); if (!strstr($password, $char)) { $password .= $char; $i++; } } $password2 = md5($_POST['password1']); $address = $_POST['address']; $result = MYSQL_QUERY("INSERT INTO plants (plantloc,password,address)". "VALUES ('$plantloc, '$password2', '$address,')"); $sendmail = " $password2 "; mail ('tcantwell@berryequipment.net', 'Thank You', $sendmail, 'From: aebstract@gmail.com'); } else { $content .= "$error"; } } ?>
and the form area:
<?php $content .= '<form action="account2.php?menu=create" method="post"> <br /> Plant Location: <br /><input type="text" maxlength="100" class="textfield" name="username" size="40" value="' . $_POST[plantloc] . '" /><br /><br /> Address:<br /> <textarea cols="50" rows="4" name="address" value="' . $_POST[address] . '"></textarea> <br /><br /> <input type="submit" name="submit" value="submit" class="textfield" /></form>'; echo "$content"; ?>
-
<?php if (isset($work)) { echo " <img src=\"/print/$work.png\" /> "; } else { echo " <div id=\"thumbs\"> <a href=\"/print/1/\"><img src=\"/thumb.png\" border=\"0\" /></a><img src=\"/spacerw.png\" /><img src=\"/thumb.png\" /><img src=\"/spacerw.png\" /><img src=\"/thumb.png\" /><img src=\"/spacerw.png\" /><img src=\"/thumb.png\" /><img src=\"/spacerw.png\" /><img src=\"/thumb.png\" /><img src=\"/spacerw.png\" /><img src=\"/thumb.png\" /><br /><br /> <img src=\"/thumb.png\" /><img src=\"/spacerw.png\" /><img src=\"/thumb.png\" /><img src=\"/spacerw.png\" /><img src=\"/thumb.png\" /><img src=\"/spacerw.png\" /><img src=\"/thumb.png\" /><img src=\"/spacerw.png\" /><img src=\"/thumb.png\" /><img src=\"/spacerw.png\" /><img src=\"/thumb.png\" /><br /><br /> <img src=\"/thumb.png\" /><img src=\"/spacerw.png\" /><img src=\"/thumb.png\" /><img src=\"/spacerw.png\" /><img src=\"/thumb.png\" /><img src=\"/spacerw.png\" /><img src=\"/thumb.png\" /><img src=\"/spacerw.png\" /><img src=\"/thumb.png\" /><img src=\"/spacerw.png\" /><img src=\"/thumb.png\" /><br /><br /> </div> "; } ?>
This code is included in to an index.php file and whenever I go to /print/ it gives the forbidden error (that is the bottom half of the script) and whenever I go to /print/1/ the top half of the script works and it all displays correctly.
www.carbenco.com and click print, the first thumbnail is clickable (though it prob. won't show the page without hitting that error)
www.carbenco.com/print/ - problem
www.carbenco.com/print/1/ - no problem
Thanks!
-
Cool, I had forgot that was necessary in that part, the $page variable is set earlier in the script. That's why I mentioned isolating the problem down to this. Thank You!
-
Eh, I don't see a problem with it and I have isolated it down to this chunk of code, so maybe someone can see what is causing it. I know there are probably shorter ways to do this same code, but for now just help getting the error out if you don't mind. Thanks!
<?php if ($page == home) { echo '<div id="nav1"><a href="/print/"><img src="/printx.png" border="0" /></a></div> <div id="nav2"><a href="/identity/"><img src="/identityxx.png" border="0" /></a></div> <div id="nav3"><a href="/web/"><img src="/webx.png" border="0" /></a></div> <div id="nav4"><a href="/home/"><img src="/home.png" border="0" /></a></div>'; } elseif ($page == web) { echo '<div id="nav1"><a href="/print/"><img src="/printx.png" border="0" /></a></div> <div id="nav2"><a href="/identity/"><img src="/identityx.png" border="0" /></a></div> <div id="nav3"><a href="/web/"><img src="/web.png" border="0" /></a></div> <div id="nav4"><a href="/home/"><img src="/homex.png" border="0" /></a></div>'; } elseif ($page == identity) { echo '<div id="nav1"><a href="/print/"><img src="/printxx.png" border="0" /></a></div> <div id="nav2"><a href="/identity/"><img src="/identity.png" border="0" /></a></div> <div id="nav3"><a href="/web/"><img src="/webxx.png" border="0" /></a></div> <div id="nav4"><a href="/home/"><img src="/homexx.png" border="0" /></a></div>'; } elseif ($page == print) { echo '<div id="nav1"><a href="/print/"><img src="/print.png" border="0" /></a></div> <div id="nav2"><a href="/identity/"><img src="/identityxxx.png" border="0" /></a></div> <div id="nav3"><a href="/web/"><img src="/webxxx.png" border="0" /></a></div> <div id="nav4"><a href="/home/"><img src="/homexx.png" border="0" /></a></div>'; } else { echo '<div id="nav1"><a href="/print/"><img src="/printx.png" border="0" /></a></div> <div id="nav2"><a href="/identity/"><img src="/identityxx.png" border="0" /></a></div> <div id="nav3"><a href="/web/"><img src="/webx.png" border="0" /></a></div> <div id="nav4"><a href="/home/"><img src="/home.png" border="0" /></a></div>'; } ?>
-
Oh, right that is correct. Thanks a lot for all of your help
-
Okay that fixed that, and I think we are down to the very last problem.
If you go to the website, click home and then another link.. it'll do something like this:
http://carbenco.com/home/web/ which of course doesn't exist and errors.
Here is the code im using to do a link:
<a href="print/">
Do I need to put the entire url, like "http://www.carbenco.com/print/" ?
-
Hmm odd, I put that in and it's partially working. I can get it to display the variable "x".
The box is black and the font is black, but it'll display whatever number is there. So that part is working, however, whenever I go to a page like http://carbenco.com/home/ none of the page's images show up anymore, is that something to do with the htaccess bugging it up?
-
Put it in, index page loads fine, click a link and it 404's.
Just so we're on the same page with the index.php file, here is what I'm using in that php section:
<?php $page = (isset($_GET['page'])) ? $_GET['page'] : 'home'; $page_path = $_SERVER['DOCUMENT_ROOT'] . '/' . $page . '.php'; if(file_exists($page_path)) { include $page_path; } else { die("$page_path does not exist"); } ?>
EDIT:
Okay error was in my linking, had a / on the end, which I can easily put in to the .htaccess, what about the /1/ part, adding another variable to the url, is that hard?
-
somehow my index file just now got corrupted to:
+ADwAIQ-DOCTYPE html PUBLIC +ACI--//W3C//DTD XHTML 1.0 Strict//EN+ACI +ACI-http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd+ACIAPg +ADw-html xmlns+AD0AIg-http://www.w3.org/1999/xhtml+ACI xml:lang+AD0AIg-en+ACIAPg +ADw-head+AD4 +ADw-title+AD4-Carbenco Designs+ADw-/title+AD4 +ADw-link href+AD0AIg-/stylesheet.css+ACI rel+AD0AIg-stylesheet+ACI type+AD0AIg-text/css+ACI title+AD0AIg-default+ACI /+AD4 +ADw-/head+AD4 +ADw-body+AD4 +ADw-div id+AD0AIg-container+ACIAPg +ADw-div id+AD0AIg-top+ACIAPgA8-img src+AD0AIg-logo.png+ACI /+AD4APA-/div+AD4 +ADw-div id+AD0AIg-middle+ACIAPgA8-div id+AD0AIg-midleft+ACIAPgA8-img src+AD0AIg-midleft.png+ACI /+AD4APA-/div+AD4 +ADw-div id+AD0AIg-midmid+ACIAPg +ADw-div id+AD0AIg-midmidtop+ACIAPgA8-img src+AD0AIg-midtop.png+ACI /+AD4APA-/div+AD4 +ADw-div id+AD0AIg-midmidcontent+ACIAPg +ADw-div id+AD0AIg-nav+ACIAPg +ADw-div id+AD0AIg-nav1+ACIAPgA8-a href+AD0AIg-/print/+ACIAPgA8-img src+AD0AIg-print.png+ACI border+AD0AIg-0+ACI /+AD4APA-/a+AD4APA-/div+AD4 +ADw-div id+AD0AIg-nav2+ACIAPgA8-a href+AD0AIg-/indentity/+ACIAPgA8-img src+AD0AIg-identityxxx.png+ACI border+AD0AIg-0+ACI /+AD4APA-/a+AD4APA-/div+AD4 +ADw-div id+AD0AIg-nav3+ACIAPgA8-a href+AD0AIg-/web/+ACIAPgA8-img src+AD0AIg-webxxx.png+ACI border+AD0AIg-0+ACI /+AD4APA-/a+AD4APA-/div+AD4 +ADw-div id+AD0AIg-nav4+ACIAPgA8-a href+AD0AIg-/home/+ACIAPgA8-img src+AD0AIg-homexx.png+ACI border+AD0AIg-0+ACI /+AD4APA-/a+AD4APA-/div+AD4 +ADw-/div +ADw-div id+AD0AIg-mmiddle+ACIAPg +ADw-img src+AD0AIg-middle.png+ACI /+AD4 +ADw-/div+AD4 +ADw-div id+AD0AIg-art+ACIAPg hi +ADw-/div+AD4 +ADw-/div+AD4 +ADw-div id+AD0AIg-midmidbottom+ACIAPgA8-img src+AD0AIg-midbottom.png+ACI /+AD4APA-/div+AD4 +ADw-/div+AD4 +ADw-div id+AD0AIg-midright+ACIAPgA8-img src+AD0AIg-midright.png+ACI /+AD4APA-/div+AD4APA-/div+AD4 +ADw-div id+AD0AIg-bottom+ACIAPgA8-/div+AD4 +ADw-/div+AD4 +ADw-/body+AD4 +ADw-/html+AD4
What is up with that?
EDIT: I went back and redid/saved everything. I think it was transfering it over to a weird style when saving so I made it save and not change a thing, its working now. Could you explain the part I was wondering about doing something like carbenco.com/home/1/ having a second variable if I wanted? Thanks for your help!
EDIT2: Go to carbenco.com and look at the links, it's still bugging up. Not as bad, but a 404. So it isn't finding the variable or something?
[SOLVED] login script issue
in PHP Coding Help
Posted
It's all in one file, account.php. The form redirects back to the same page and if submit is hit that is when the top part goes in to play. I'll post all the php coding below so you can see. I have tried to echo out the id from the selection but it doesn't seem to be displaying a single thing. I really think the id isn't getting passed.
and the form area