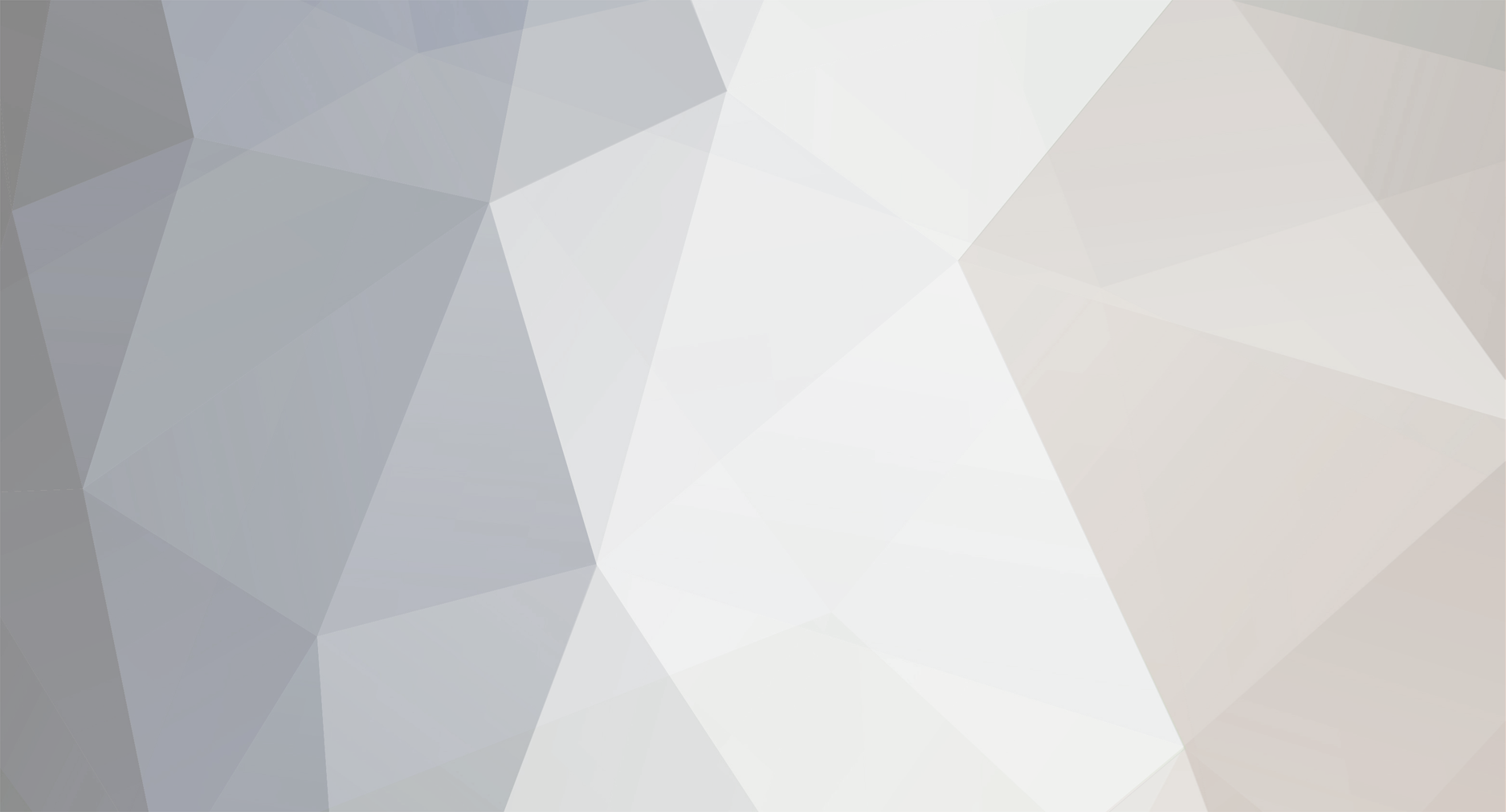
pocobueno1388
Members-
Posts
3,369 -
Joined
-
Last visited
Never
Everything posted by pocobueno1388
-
Okay, give this a shot. It's hard to tell if it's going to work when I can't test it on your server...but I think it will. <?php include("dbinfo.inc.php"); mysql_connect(mysql,$username,$password); @mysql_select_db($database) or die( "Unable to select database"); $default_event= array('event1', 'event2'); if(isset($_REQUEST['changed']) && count($_REQUEST['changed']) > 0 && !empty($_REQUEST['command'])) { $sql = ""; $insql = "IN('" . join("','",array_keys($_REQUEST['changed'])) . "')"; switch($_REQUEST['command']) { case "Deactivate": $sql = "UPDATE birthdays SET ACTIVE='0' WHERE id " . $insql; break; case "Activate": $sql = "UPDATE birthdays SET ACTIVE='1' WHERE id " . $insql; break; case "Delete": $sql = "DELETE FROM birthdays WHERE id " . $insql; break; } if(!empty($sql)) { mysql_query($sql); } } $where = $url = array(); if(!empty($_REQUEST['event'])) { $where[] = "Event='" . addslashes($_REQUEST['event']) . "'"; $url[] = "event=" . $_REQUEST['event']; } else { $num_of_events = count($default_event); for($i=0; $i<$num_of_events;$i++){ if ($i == 0) { $where_s = "(Event='$default_event[$i]'"; if ($num_of_events <= 1) $where_s .= ')'; $where[] = $where_s; } else { $stop = $i+1; $where[] $where_s = " OR Event='$default_event[$i]' "; if ($num_of_events == $stop) $where_s .= ')'; $where[] = $where_s; } } $_REQUEST['event'] = $default_event; } if(strlen($active) > 0) { $where[] = " AND Active='" . addslashes($_REQUEST['active']) . "'"; $url[] = "active=" . $_REQUEST['active']; switch($active) { case 1: $page_type = "ACTIVE"; break; case 0: $page_type = "INACTIVE"; break; } } else { $page_type = "ALL"; } $query="SELECT * FROM birthdays"; if(count($where) > 0) { $query .= " WHERE " . join(" ",$where); } $result=mysql_query($query)or die(mysql_error() . "<p>With Query:<br>$query"); $num=mysql_num_rows($result); mysql_close(); echo "<p><b>$query</b><p>"; //################## Query outputted on this line echo "<A name=\"top\"></A><b><center><H1>Display Records \"<I>$page_type $default_event records</I>\":</H1>"; ?>
-
EDIT: hold on...
-
So whats the problem? Is it when you click active it is showing inactive records?
-
Okay, I think this will solve the problem. I commented where I outputted the query, I would keep it there until this is solved though. <?php include("dbinfo.inc.php"); mysql_connect(mysql,$username,$password); @mysql_select_db($database) or die( "Unable to select database"); $default_event= array('event1', 'event2'); if(isset($_REQUEST['changed']) && count($_REQUEST['changed']) > 0 && !empty($_REQUEST['command'])) { $sql = ""; $insql = "IN('" . join("','",array_keys($_REQUEST['changed'])) . "')"; switch($_REQUEST['command']) { case "Deactivate": $sql = "UPDATE birthdays SET ACTIVE='0' WHERE id " . $insql; break; case "Activate": $sql = "UPDATE birthdays SET ACTIVE='1' WHERE id " . $insql; break; case "Delete": $sql = "DELETE FROM birthdays WHERE id " . $insql; break; } if(!empty($sql)) { mysql_query($sql); } } $where = $url = array(); if(!empty($_REQUEST['event'])) { $where[] = "Event='" . addslashes($_REQUEST['event']) . "'"; $url[] = "event=" . $_REQUEST['event']; } else { $num_of_events = count($default_event); for($i=0; $i<$num_of_events;$i++){ if ($i == 0) $where[] = "Event='$default_event[$i]'"; else $where[] = " OR Event='$default_event[$i]'"; } $_REQUEST['event'] = $default_event; } if(strlen($active) > 0) { $where[] = " AND Active='" . addslashes($_REQUEST['active']) . "'"; $url[] = "active=" . $_REQUEST['active']; switch($active) { case 1: $page_type = "ACTIVE"; break; case 0: $page_type = "INACTIVE"; break; } } else { $page_type = "ALL"; } $query="SELECT * FROM birthdays"; if(count($where) > 0) { $query .= " WHERE " . join(" ",$where); } $result=mysql_query($query)or die(mysql_error() . "<p>With Query:<br>$query"); $num=mysql_num_rows($result); mysql_close(); echo "<p><b>$query</b><p>"; //################## Query outputted on this line echo "<A name=\"top\"></A><b><center><H1>Display Records \"<I>$page_type $default_event records</I>\":</H1>"; ?>
-
Okay, I see a problem with that query. Try this <?php include("dbinfo.inc.php"); mysql_connect(mysql,$username,$password); @mysql_select_db($database) or die( "Unable to select database"); $default_event= array('event1', 'event2'); if(isset($_REQUEST['changed']) && count($_REQUEST['changed']) > 0 && !empty($_REQUEST['command'])) { $sql = ""; $insql = "IN('" . join("','",array_keys($_REQUEST['changed'])) . "')"; switch($_REQUEST['command']) { case "Deactivate": $sql = "UPDATE birthdays SET ACTIVE='0' WHERE id " . $insql; break; case "Activate": $sql = "UPDATE birthdays SET ACTIVE='1' WHERE id " . $insql; break; case "Delete": $sql = "DELETE FROM birthdays WHERE id " . $insql; break; } if(!empty($sql)) { mysql_query($sql); } } $where = $url = array(); if(!empty($_REQUEST['event'])) { $where[] = "Event='" . addslashes($_REQUEST['event']) . "'"; $url[] = "event=" . $_REQUEST['event']; } else { $num_of_events = count($default_event); for($i=0; $i<$num_of_events;$i++){ if ($i == 0) $where[] = "Event='$default_event[$i]'"; else $where[] = " OR Event='$default_event[$i]'"; } $_REQUEST['event'] = $default_event; } if(strlen($active) > 0) { $where[] = "Active='" . addslashes($_REQUEST['active']) . "'"; $url[] = "active=" . $_REQUEST['active']; switch($active) { case 1: $page_type = "ACTIVE"; break; case 0: $page_type = "INACTIVE"; break; } } else { $page_type = "ALL"; } $query="SELECT * FROM birthdays"; if(count($where) > 0) { $query .= " WHERE " . join(" ",$where); } $result=mysql_query($query)or die(mysql_error() . "<p>With Query:<br>$query"); $num=mysql_num_rows($result); mysql_close(); echo "<p><b>$query</b><p>"; echo "<A name=\"top\"></A><b><center><H1>Display Records \"<I>$page_type $default_event records</I>\":</H1>"; ?> Hopefully this gets it working. If not, post the new query it outputs.
-
How To Get Last 5 Day's Data Using PHP & MYSQL
pocobueno1388 replied to rohit_purohit's topic in PHP Coding Help
Since you didn't supply how you stored the date/time, I'm going to assume it is in yyyy/mm/dd format. So try this <?php $five_days_ago = date("Y-m-d", strtotime("- 5 DAY")); $query = mysql_query("SELECT * FROM table WHERE `date` < $five_days_ago")or die(mysql_error()); ?> -
Okay, lets try to echo the query out to make sure it looks okay. <?php include("dbinfo.inc.php"); mysql_connect(mysql,$username,$password); @mysql_select_db($database) or die( "Unable to select database"); $default_event= array('event1', 'event2'); if(isset($_REQUEST['changed']) && count($_REQUEST['changed']) > 0 && !empty($_REQUEST['command'])) { $sql = ""; $insql = "IN('" . join("','",array_keys($_REQUEST['changed'])) . "')"; switch($_REQUEST['command']) { case "Deactivate": $sql = "UPDATE birthdays SET ACTIVE='0' WHERE id " . $insql; break; case "Activate": $sql = "UPDATE birthdays SET ACTIVE='1' WHERE id " . $insql; break; case "Delete": $sql = "DELETE FROM birthdays WHERE id " . $insql; break; } if(!empty($sql)) { mysql_query($sql); } } $where = $url = array(); if(!empty($_REQUEST['event'])) { $where[] = "Event='" . addslashes($_REQUEST['event']) . "'"; $url[] = "event=" . $_REQUEST['event']; } else { $num_of_events = count($default_event); for($i=0; $i<$num_of_events;$i++){ if ($i == 0) $where[] = "Event='$default_event[$i]'"; else $where[] = " OR Event='$default_event[$i]'"; } $_REQUEST['event'] = $default_event; } if(strlen($active) > 0) { $where[] = "Active='" . addslashes($_REQUEST['active']) . "'"; $url[] = "active=" . $_REQUEST['active']; switch($active) { case 1: $page_type = "ACTIVE"; break; case 0: $page_type = "INACTIVE"; break; } } else { $page_type = "ALL"; } $query="SELECT * FROM birthdays"; if(count($where) > 0) { $query .= " WHERE " . join(" AND ",$where); } $result=mysql_query($query)or die(mysql_error() . "<p>With Query:<br>$query"); $num=mysql_num_rows($result); mysql_close(); echo "<p><b>$query</b><p>"; echo "<A name=\"top\"></A><b><center><H1>Display Records \"<I>$page_type $default_event records</I>\":</H1>"; ?> The query should come out bold on the page, so copy and paste whatever you see.
-
You have been here long enough to know the basic debugging procedures for a query. <?php if($_POST['journal']) { $query = "DELETE FROM vc_journal WHERE id='$id'"; $query2 = "DELETE FROM vc_jcomments WHERE jid='$id'"; $result = mysql_query($query)or die(mysql_error() . "<p>With Query:<br>$query<p>"); $result2 = mysql_query($query2)or die(mysql_error() . "<p>With Query:<br>$query2<p>"); echo "Your journal(s) have been deleted<br>"; ?>
-
Why would you want to avoid using an IF statement?
-
Can you post your code? Sounds like you need to use the return keyword.
-
Variables in a Multiple select drop down
pocobueno1388 replied to scrbowler's topic in PHP Coding Help
Just grab them straight from the array. From the HTML example I posted above, to get the first value you would use choices[0], and so on. Or you could use a foreach loop, it all depends on what your trying to do. So if this doesn't help you, explain more. -
EDIT: Nevermind
-
Take a look at this post: http://www.phpfreaks.com/forums/index.php/topic,95426.0.html
-
Variables in a Multiple select drop down
pocobueno1388 replied to scrbowler's topic in PHP Coding Help
You need to create an array for these values, so here is an example. HTML <form> <select name="choices[]" multiple="multiple"> <option>...</option> <option>...</option> <option>...</option> <option>...</option> <option>...</option> <option>...</option> </select> </form> Now when they submit the form, all the selected values will be in the array $_POST['choices'] -
SELECT MAX(ticket_number) as num FROM table
-
Have you checked the manual on number_format()? It shows you how to do it in one of the examples.
-
Well, the code I gave you will convert it to how you want, I'm not really sure what else your trying to do. Or did you get it to work?
-
[SOLVED] Why won't it insert the MySQL data?
pocobueno1388 replied to Shiny_Charizard's topic in PHP Coding Help
Ah, I can't believe I didn't see it before. You need to take the '*' out. -
That is called aliasing. It basically just shortens the table name for the query so you don't have to type it out all the time.
-
[SOLVED] Why won't it insert the MySQL data?
pocobueno1388 replied to Shiny_Charizard's topic in PHP Coding Help
Okay...do this and post what it gives you $addpost ="INSERT * INTO forum_posts ( author, title, post, showtime, realtime, lastposter, category_id ) VALUES ( '$name', '$subject', '$post', '$displaytime', '$thedate', '$name', '$ID' )"; $result = mysql_query($addpost)or die(mysql_error() . "<p>With Query<br>$addpost"); -
[SOLVED] Why won't it insert the MySQL data?
pocobueno1388 replied to Shiny_Charizard's topic in PHP Coding Help
Change your query to: $addpost =mysql_query("INSERT * INTO forum_posts ( author, title, post, showtime, realtime, lastposter, category_id ) VALUES ( '$name', '$subject', '$post', '$displaytime', '$thedate', '$name', '$ID' )") or die (mysql_error()); Post whatever error you get. -
[SOLVED] Inserting and Updating Arrays...
pocobueno1388 replied to briant's topic in PHP Coding Help
No problem -
[SOLVED] Is it possible to run multiple queries on one page
pocobueno1388 replied to affordit's topic in PHP Coding Help
Maybe instead of making a separate table for EVERY category, you can just add a field called "category" to your table and put ALL the items in it. So your fields would be auto heading picture description category And for category you can put "Toy", "Tool", "Garden", or whatever. That way it will be easy to sort out and grab exactly what you want. -
[SOLVED] Inserting and Updating Arrays...
pocobueno1388 replied to briant's topic in PHP Coding Help
Instead, why don't you just separate them by commas? That way you don't have to deal with the pain of selecting names in between two characters. Brian, Billy, Bob, etc -
[SOLVED] Is it possible to run multiple queries on one page
pocobueno1388 replied to affordit's topic in PHP Coding Help
Your going to have to give us details from a second table for us to help you link them. If you do that, make sure you tell us exactly what your wanting to select and do.