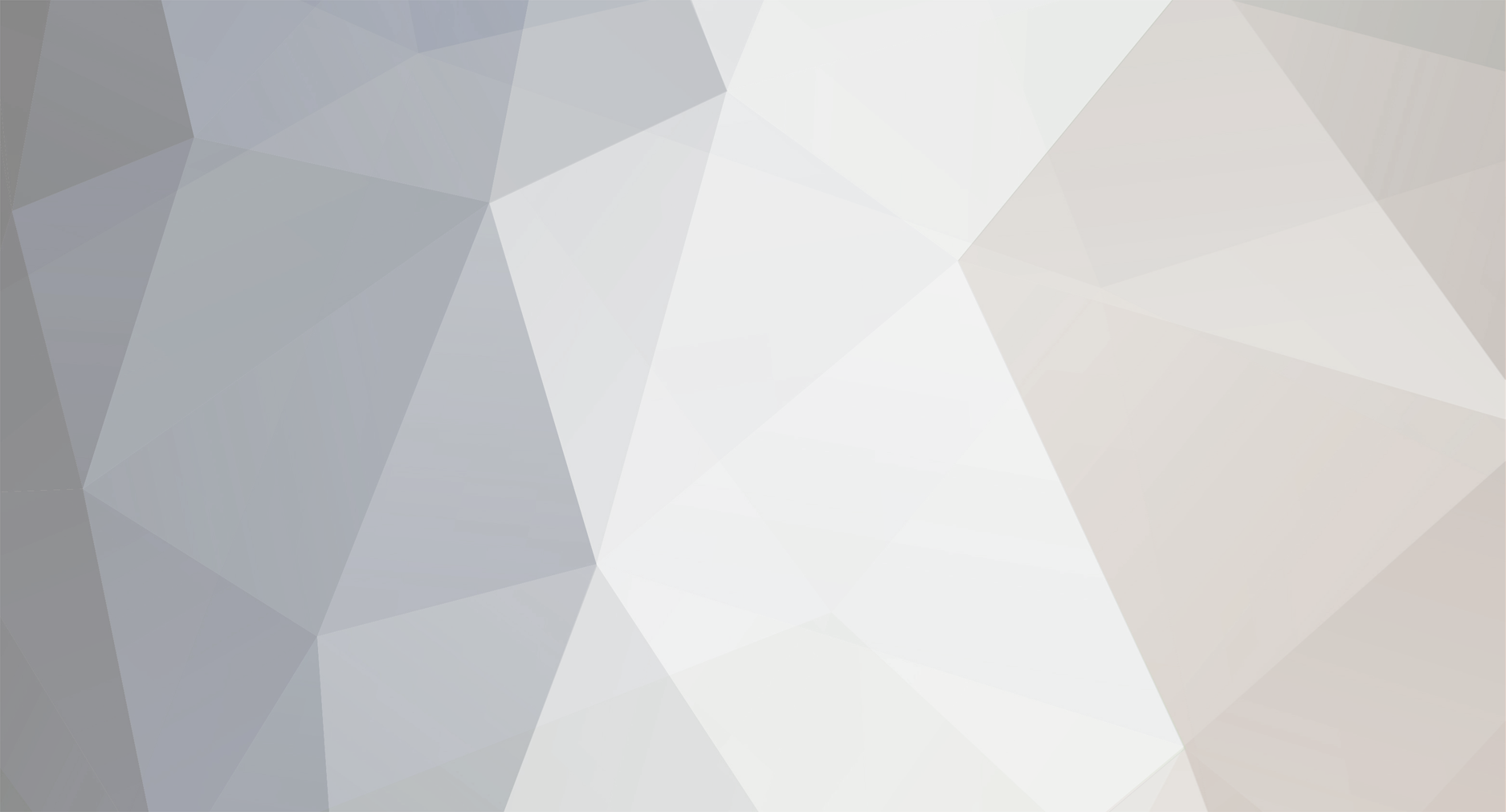
pocobueno1388
Members-
Posts
3,369 -
Joined
-
Last visited
Never
Everything posted by pocobueno1388
-
make it where users can't put < or leave form blank?
pocobueno1388 replied to A2xA's topic in PHP Coding Help
This will make sure they filled the dir out, and also strip any HTML tags. <?php //check if they filled it out if ((!isset($_POST['dir'])) || (empty($_POST['dir'])){ echo "You didn't fill the form out!"; } else { $dir = strip_tags($_POST['dir']); } ?> -
Just don't set the variable unless the price has a value. <?php if (!empty($row['price'])) $price = $row['price']; ?> It's kinda hard to give you a good example without seeing code.
-
[SOLVED] GROUP BY - getting weird results...
pocobueno1388 replied to acook's topic in PHP Coding Help
Hmm...I'm not familiar with using "odbc", so maybe it can't be used the way I tried to. -
Yes you can. You need to create the HTML form, when submitted you need to make sure they checked the "agree" box, if they did then display the store and set session. Here is a little snippet to look at <?php if (isset($_POST['agree'])){ //they agreed to terms, show the store and register session } else { //They did NOT agree, give error } <form action="url.com" method="post"> <input type="radio" name="agree"> <input type="submit"> </form>
-
[SOLVED] GROUP BY - getting weird results...
pocobueno1388 replied to acook's topic in PHP Coding Help
Your making it harder than it needs to be by creating your own array to store the values in. Try it this way <?php while($row = odbc_fetch_row($result)){ echo $row['num'] . ' - ' . $row['Assignee_Group_Counter'] . '<br>'; } ?> Your going to need to change your query to this SELECT COUNT(Incident_Id) as num, Assignee_Group_Counter FROM Incident_Management WHERE (Submitter = 'Me') GROUP BY Assignee_Group_Counter; -
You could store the information into sessions. So lets say they submit the form and one of the fields was their first name. $_SESSION['first_name'] = $_POST['first_name']; Now when they have to go back to the form, your form code should look like this. <input type="text" name="first_name" value="<?php if(isset($_SESSION['first_name'])) echo $_SESSION['first_name']; ?>">
-
You need to try to catch the error with die(). mysql_query($query)or die(mysql_error()."<p>With Query:<br>$query");
-
Are you sure your entering an email and lost password answer that exists in the database? If you are positive that you are, then echo your query out to make sure the variables are set right. <?php $query = "SELECT * FROM `login` WHERE `email` = '$email' and `lost_password_answer` = '$lost_password_answer'"; $result = mysql_query($query) or die (mysql_error()); $num = mysql_num_rows($result); echo "<p>$query<p>";
-
Everything in your switch statement has to be between a case or default. These lines inside your switch statement are not between a case <?php while ($link = mysql_fetch_array($query)) { $title = $link['title']; $address = $link['address']; ?> So you need to figure out a way to do this block of code differently <?php while ($link = mysql_fetch_array($query)) { $title = $link['title']; $address = $link['address']; case $title: include($address); break; }
-
<?php switch($page) { ############ NEED CASE HERE ##################################### while ($link = mysql_fetch_array($query) /* while $link has data, create each 'case' tag */ { $title = $link['title']; $address = $link['address']; case $link: /* NOTE : here is my case */ include($address); break; } default: /* NOTE : here is my default */ echo 'Welcome to the Education Trainer.'; break; } ?> I put a comment in the code where you need a case (it's hard to miss ;P). If you want that part of the code to show all the time, you need to bring it outside of the switch statement.
-
Here is a good one http://www.tizag.com/phpT/postget.php
-
On this line while ($link = mysql_fetch_array($query) Why do you have the semi-colon in there? Try changing it to while ($link = mysql_fetch_array($query)) Not sure if thats causing the error, but it could be.
-
You need to set up a cron job. You can usually do this through your hosts control panel, although some hosts don't offer this (usually they do if they support PHP/MySQL), you just have to check.
-
changing status of a record in a DB
pocobueno1388 replied to rnb_cassanova's topic in PHP Coding Help
Do an UPDATE query. UPDATE table SET status='new status' WHERE recordID='Record ID' -
Probably because your listing_id variable is a number. If you put quotes around it it would then become a string. So if you are searching for a number, don't use quotes.
-
You don't have quotes around your $_GET['listing_id'] var, that could be the problem. <?php $sql = "UPDATE chrislistings SET listing_address = '" . addslashes($_POST['listing_address']) . "', listing_overview = '" . addslashes($_POST['listing_overview']) . "', listing_price = '" . addslashes($_POST['listing_price']) . "', listing_location = '" . addslashes($_POST['listing_location']) . "', listing_style = '" . addslashes($_POST['listing_style']) . "', listing_sqrft = '" . addslashes($_POST['listing_sqrft']) . "', listing_lotwidth = '" . addslashes($_POST['listing_lotwidth']) . "', listing_lotlength = '" . addslashes($_POST['listing_lotlength']) . "', listing_year = '" . addslashes($_POST['listing_year']) . "', listing_taxes = '" . addslashes($_POST['listing_taxes']) . "', listing_parking = '" . addslashes($_POST['listing_parking']) . "', listing_exterior = '" . addslashes($_POST['listing_exterior']) . "', listing_roof = '" . addslashes($_POST['listing_roof']) . "', listing_heating = '" . addslashes($_POST['listing_heating']) . "', listing_ac = '" . addslashes($_POST['listing_ac']) . "', listing_fireplace = '" . addslashes($_POST['listing_fireplace']) . "', listing_school = '" . addslashes($_POST['listing_school']) . "', listing_transit = '" . addslashes($_POST['listing_transit']) . "', listing_bathrooms = '" . addslashes($_POST['listing_bathrooms']) . "', listing_bedrooms = '" . addslashes($_POST['listing_bedrooms']) . "' WHERE listing_id = '" . addslashes($_GET['listing_id']) . "'"; ?> Also, you should try echoing the query out in the die error, like so <?php $query = mysql_query($sql)or die(mysql_error() . "<p>With Query:<br>$sql"); ?>
-
I think you want to look into the exec() and system() functions. Take a look through this list of functions, I'm pretty sure what you need is there. http://nl2.php.net/manual/en/ref.exec.php
-
Why are you doing two of the same loops, just with different information in them? Combine them. <?php $poll_id_query = mysql_query("SELECT * FROM tblPollQuestions WHERE username = '$user' ORDER BY pollID DESC LIMIT 1"); $poll_id_array = mysql_fetch_array($poll_id_query); $poll_id = $poll_id_array['pollID']; $poll_question = $poll_id_array['question']; echo "<p>$poll_question</p><p>"; $poll_query = mysql_query("SELECT * FROM tblPollAnswers1 WHERE pollID = '$poll_id' "); while ($poll_array = mysql_fetch_array($poll_query)) { $amount = $poll_array['amount']; $total = $total + $amount; $answer = $poll_array['answer']; $amount = round((($amount * 100) / $total),1); echo "$answer - <img src='sections/line.jpg' width='14%' height='5'>"; } ?> See if that does what you want.
-
Instead of your IF statements looking like if ($tb1id != $s) { Do it like this if (!empty($tb1id)) {
-
Try this <?php echo "<a href='http://www.yourweblog.com/yourfile.html' onclick='window.open('http://wiicharged.com/hubchat/$hubid/chat.html','popup','width=500,height=500,scrollbars=no,resizable=no,toolbar=no,directories=no,location=no,menubar=no,status=no,left=0,top=0'); return false'>About</a>"; ?>
-
<?php echo "<a href='/home/wiicharg/public_html/hubchat/$dir/shout.php'>Link</a>"; ?>
-
Do this and see what it prints out. mysql_query($sql)or die("ERROR:<br>".mysql_error()."<p>With Query:<br>$sql");