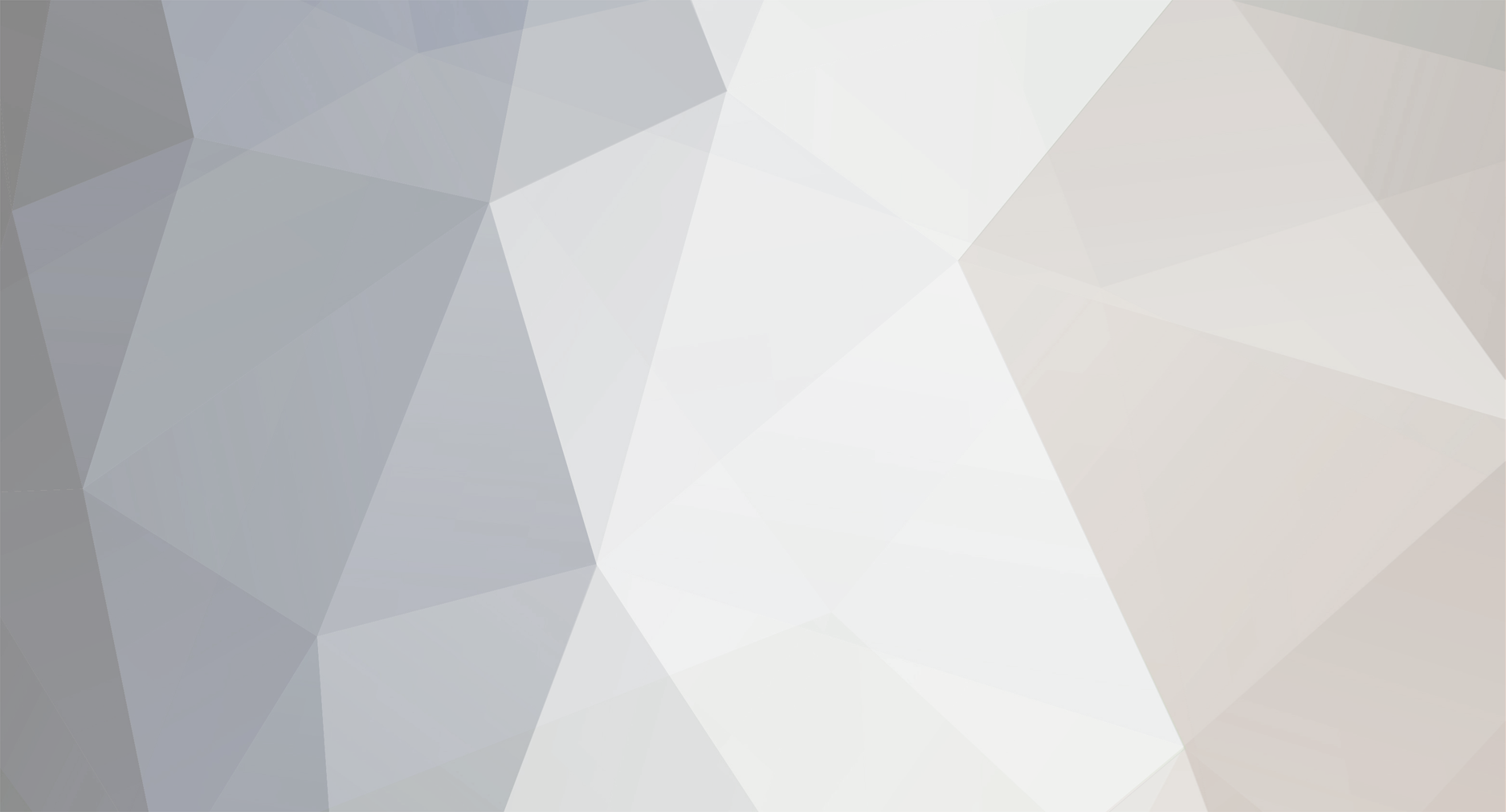
pocobueno1388
Members-
Posts
3,369 -
Joined
-
Last visited
Never
Everything posted by pocobueno1388
-
You need to use normal double quotes. <html> <head> <title>My Movie Site</title> </head> <body> <?php define("FAVMOVIE", "The Life of Brian"); echo "My favorite movie is "; echo FAVMOVIE; ?> </body> </html>
-
Whats the error your getting?
-
I'm not sure if the $_GET is case sensitive or not...but try to capitalize it and see if it works. $user_id = $_GET['user_id']; echo $_GET['user_id']; EDIT: Yeah, thats the problem. I just tested it.
-
Well, you need a submit button to submit the form...see if this gets you what you want. <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1" /> <title>Untitled Document</title> </head> <body> <?php if (isset($_POST['submit'])){ //connect to database include'db.php5'; //get parts of records $get_list_sql = "SELECT * FROM products WHERE Category = '".$_POST['select']."'"; $get_list_res = mysqli_query($mysqli, $get_list_sql) or die(mysqli_error($mysqli)); //create table with the selected data $display_block = "<p align = 'center'> <table class='sortable' border = '1' bordercolor = 'black' cellpadding= '0' cellspacing = '0'> <thead> <th bgcolor = 'orange'>Item Number</th> <th bgcolor = 'orange'>Manufacturer</th> <th bgcolor = 'orange'>Category</th> <th bgcolor = 'orange'>Description</th> <th bgcolor = 'orange'>Model</th> <th bgcolor = 'orange'>Quantity</th> <th bgcolor = 'orange'>Kw</th> <th bgcolor = 'orange'>Hours</th> <th bgcolor = 'orange'>Price</th> </thead>"; //if authorized, get the values while ($info = mysqli_fetch_array($result)) { $ItemNo = stripslashes($info['Item_No']); $Man = stripslashes($info['Manufacturer']); $Cat = stripslashes($info['Category']); $Des = stripslashes($info['Description']); $Model = stripslashes($info['Model']); $Qty = stripslashes($info['Qty']); $Kw = stripslashes($info['Kw']); $Hours = stripslashes($info['Hours']); $Price = stripslashes($info['Price']); //create display string $display_block .= " <tr> <td class = 'numeric'>".$ItemNo."</td> <td>".$Man."</td> <td>".$Cat."</td> <td>".$Des."</td> <td>".$Model."</td> <td>".$Qty."</td> <td>".$Kw."</td> <td>".$Hours."</td> <td>".$Price."</td> </tr>"; } $display_block .= "</table></p>"; //display selected data in a table //free result mysqli_free_result($get_list_res); } ?> <form id="form1" name="form1" method="post" action=""> <label> <div align="right">Display by Category: <select name="select"> <option>Diesal</option> <option>Engines</option> <option>Generators</option> </select> </div> </label> <input type="submit" name="submit" value="Submit"> </form> </body> </html>
-
Well, whats not working about the script? You need to tell us what the actual problem is before we can look for it. Also, it would help if you used [/code.][./code] without the dots.
-
[SOLVED] Quick easy code question...
pocobueno1388 replied to ShootingBlanks's topic in PHP Coding Help
Put this code on that page: echo '<pre>'; print_r($_SERVER); echo '</pre>'; That will make a list of $_SEVER variables and what they would print out if you used them. Just search the list for the URL you are looking for, then it will tell you what variable to use. -
Give this a try. <?php $file = $_GET['name']; $lines = file($file); foreach ($lines as $line) { echo $line.'<br>'; } ?>
-
SELECT * FROM tablename WHERE UserID != '$UserID'
-
Add fields to a form dynamically on the fly
pocobueno1388 replied to mrfusion's topic in PHP Coding Help
Are you meaning you want them to be able to click "Add Form" and a new form will appear without a page refresh? If thats the case, then you will need JavaScript. You could also have a page asking them how many forms they want, then when they press submit it would display as many forms as they asked for. It all depends on what you want to do... -
Why would you need mySQL for this? You would just treat it as a normal text file. <?php $file = $_GET['name']; $handle = fopen($file, "r"); ?>
-
[SOLVED] Need help putting together query.
pocobueno1388 replied to pocobueno1388's topic in MySQL Help
Here is the winning query, thanks to Travis xD SELECT clubID, vote_for FROM (SELECT clubID, vote_for, COUNT(*) as count1 FROM club_votes GROUP BY clubID, vote_for ORDER BY count1 DESC) as total GROUP BY clubID Thank you to all who helped, it was much appreciated. -
Where is your submit button? Make one of those, then put this on the top of your script. <?php if (isset($_POST['submit'])){ $id = mysql_real_escape_string($_POST['ID']); $name = mysql_real_escape_string($_POST['name']); $title = mysql_real_escape_string($_POST['Title']); //do this to the rest of them too $query = "UPDATE TableName SET name='$name', $title='$title' WHERE ID='$ID'"; $result = mysql_query($query)or die(mysql_error()); } ?>
-
Well, here are some tutorials to start reading: Working with Forms http://www.onlamp.com/pub/a/php/2003/03/13/php_foundations.html http://www.tizag.com/phpT/forms.php www.tizag.com has a lot of good tutorials, you might want to just start going from lesson to lesson on that site. Retrieving information from the DB http://www.redhat.com/magazine/010aug05/features/mysql/ http://www.php-mysql-tutorial.com/php-mysql-select.php Looping through rows in the DB (while loop) http://www.tizag.com/phpT/whileloop.php
-
[SOLVED] Question about psasing variables between scripts.
pocobueno1388 replied to bre's topic in PHP Coding Help
On your other server they probably had magic_quotes_gpc off. This means that you don't have to define your post/get data with $_POST or $_GET. On your new server they have them on, meaning you do have to define them like you are. In my opinion it is better to have to define them, that way you know exactly where the data is coming from. -
If this is your first lesson, you are going at a rate thats way too fast. You should build yourself up to being able to do this, that way when you get to this lesson, you will be able to do it without a problem. Here is what you will need to learn: -Retrieving/deleting information from the database with MySQL -Looping through the rows with a while loop -Working with forms and PHP -Using $_POST data, which goes along with forms. At least try to attempt it the best you can, then post the code you end up with. Before even doing that, I would suggest learning the very basics of PHP.
-
Ummm, no. They should have some primary key in the database that they will be able to identify which table to update with. They will have to pass this variable through a hidden input field.
-
Are you talking about updating the fields in the database? You would just use an update query. <?php $query = "UPDATE TableName SET col1='whatever', col2='whatever' WHERE condition"; $result = mysql_query($query)or die(mysql_error()); ?> If you posted the code to the form, it would be easier to help you. Sorry if I'm completely off, I didn't quite understand.
-
Well, you have two choices. You could use an Iframe, or you could put the code to the form into the table on your contact page. Could you post the code to the form page and the contact page?
-
Your going to have to explain a lot more than that.
-
[SOLVED] How would you do forum topic replies?
pocobueno1388 replied to DrRobot's topic in PHP Coding Help
I don't see how this has anything to do with OOP. You need to store all the replies in a separate table, set the table up like this: TABLE "replies" --------------- replyID (Primary Key) topicID (The topic they are posting the reply on) poster (Who is posting the topic) body (the actual reply) Then you can just make a simple query grabbing the replies for that topic. SELECT reply, poster FROM replies WHERE topicID='$topicID' Does that make enough sense? -
Whats the error your getting?
-
Theres probably a simple solution to this...
pocobueno1388 replied to sectachrome's topic in PHP Coding Help
Does it work when you echo out $row["ID"] somewhere else on the page? From a glance, I don't see any reason why it shouldn't be working. I would assume it has something to do with your database. Check for spelling and make sure your table has a row named "ID". -
Do you have a set size set for your input field? It may be cutting it off because the variable length is too long.
-
You need to comment the line out that is creating the errors, that way it will get down to the part that your trying to execute.
-
There is a problem with your query then, try catching the error: <?php $getCatStores = mysql_query("SELECT DISTINCT a.ownerid, u.store_name FROM probid_users u, probid_auctions a WHERE ".$subcatquery." a.listin!='auction' AND a.active='1' AND a.closed='0' AND a.deleted!='1' AND a.ownerid=u.id AND u.active='1' AND u.store_active='1' AND u.aboutpage_type='2'")or die(mysql_error()); ?>