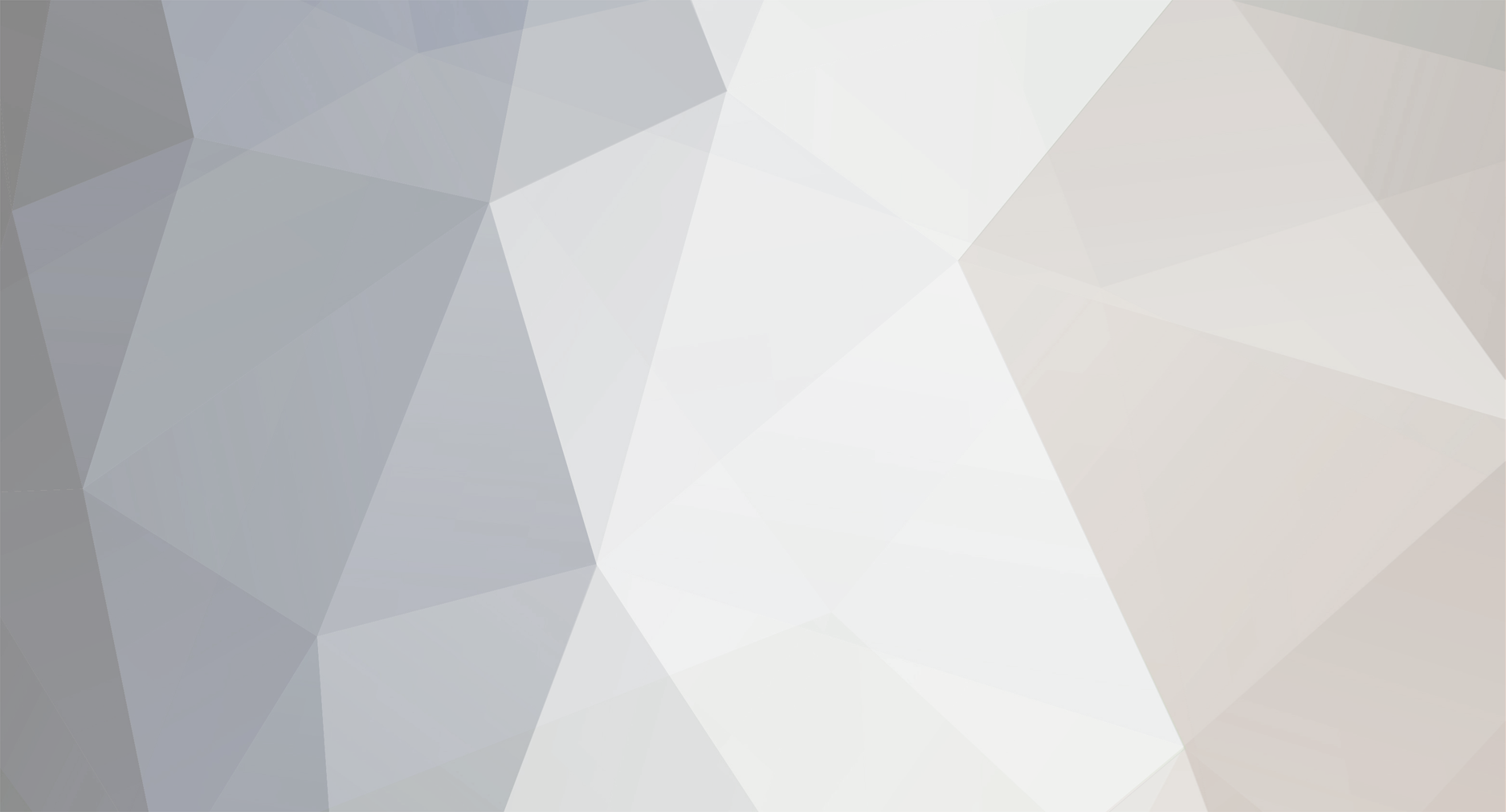
akitchin
Staff Alumni-
Posts
2,515 -
Joined
-
Last visited
Never
Everything posted by akitchin
-
Handling credit cards: point me in the right direction?
akitchin replied to mschrank's topic in PHP Coding Help
you don't even need to embed some header code in the customizable page. paypal notifies a file on your own server when a payment is made, and what the results of the payment are. you can then analyse this information and do whatever is appropriate for the results. no need to fiddle with e-mail notifications when they operate a system like this, the e-mails are just a handy record. -
i would suggest never EVER storing credit card or debit card information in a database unless you physically own and look after the server, and it is heavily protected. saving it anywhere on a shared server, VPS, or dedicated server that you aren't in control of directly is just asking for trouble.
-
for the record, even though using backticks works, i would suggest staying away from reserved keywords regardless just for good practice. also, the reason $_SESSION['user'] messes up your queries is likely that you're not including it properly. when you need to echo an array value within a string, use braces to enclose it: [code]<?php $_SESSION['name'] = 'Someone You Wish You Knew'; $hello = "Hi, I'm {$_SESSION['name']}."; ?>[/code]
-
another easy way of doing this is to use strrchr: [code]<?php $filename = 'something.zip'; $extension = strrchr($filename, '.'); ?>[/code] would yield '.zip' in $extension. use substr() if you want to remove the period as well.
-
Handling credit cards: point me in the right direction?
akitchin replied to mschrank's topic in PHP Coding Help
i think the others have avoided your question. paypal has features that allow a file on your server to process the results of the payment that was sent through them. this may take a while to learn the details of (it took me a little while), but the code samples on their website for IPN are a good ground setup which you can elaborate from. they have a service called Sandbox where you may test out your system without processing any actual payments. i'd download the manuals and get registered on Sandbox, as there are forums there where you can discuss what is going wrong with your setup. in short, paypal gives you all the tools you need to make integration and real-time processing possible. hope this helps. -
i don't know how your login system works specifically, but once a user has logged in successfully you should be able to assign their unique identifier (be it an actual ID, or their username, or whatever will identify them) to a $_SESSION variable which will propagate with them as they browse the site. you can then use this $_SESSION value in your work order builder (simply use the user's value in the query as you would any other piece of info). as to the second question, this is merely a matter of some looping with a query: [code]<?php $query = "SELECT id, name FROM techies ORDER BY name ASC"; $resource = mysql_query($query); if ($resource !== FALSE) { while ($tech_info = mysql_fetch_assoc($resource)) { echo '<option value="'.$tech_info['id'].'">'.$tech_info['name'].'</option>'; } } ?>[/code] that's a simple bit of code that you can pluck in between a <select></select> form element to echo one option in the dropdown box for each tech. if you just want to use their name, then there's no need to employ the "value" attribute in each <option> tag. don't forget to change the query, and feel free to fiddle with the code itself. hope this helps.
-
the easiest way to do this is to go one table at a time, one query for each table: [code]SELECT id, series, active FROM avatars WHERE active='1' ORDER BY series ASC[/code] i don't see any reason for you to try and grab all of the info in one query, as that only complicates everything. reserve your multiple table queries for queries that actually require the correlation of table values (ie. where one value is dependent upon a value from a different table). nonetheless, for the record, your problem is that you're not enunciating where each of those fields are to come from. put properly, your query should be: [code]$sql= "SELECT avatars.id, avatars.series, avatars.active, wallpapers.id, wallpapers.series, wallpapers.active, pngs.id, pngs.series, pngs.active, layouts.id, layouts.series, layouts.active FROM avatars, wallpapers, pngs, layouts WHERE avatars.active='1' ORDER BY `series` ASC";[/code] however, this query will not at all do what you want it to do.
-
i'm going to assume that you've got example@example.com replaced with a real e-mail address. perhaps try dropping the X- headers (in particular, the priority ones) and see if they go through.
-
well my naive suggestion would be a select box which you populate with all the directories available. are you asking how to get all the directories available, or do you already have those stored in a database? i'm not too clear on what information you've already got to work with.
-
then it's likely that it's being blocked from even reaching the junk box. try adding some headers such as "From: someone <somewhere@something.com>" and the reply-to header. the more legitimate headers you give your outgoing mail, the better the chance they have of reaching a user.
-
[b]EDIT: i've been robbed of my reply (thanks ken :)), so i'll just add the first and last paragraph from my original comment.[/b] first off, print_r() is for arrays only, not strings. there are a lot of FAQs and snippets and topics about simple MySQL data manipulation and handling, have a look and you'll find everything you need to know about it.
-
which table is "active" in? you need to be very specific with MySQL, otherwise your statement becomes ambiguous and impossible to accurately fetch. in the interest of properly solving this (and leaving you with useful knowledge), what exactly are you trying to pull and from which tables?
-
you have to label everything by table.field when you're selecting from multiple tables, otherwise SQL has no idea which table the field you're trying to pull is in.
-
out of curiosity, where have you met these clients? any site in particular that yields good clients/projects?
-
simply list as many tables as you need in the FROM segment: [code]SELECT table1.stuff, table2.something FROM table1, table2, table3, table4[/code]
-
no problem. for the record, $extension is the array of file types you deem acceptable, and is used in the if() immediately following $file's definition.
-
To "Array" or not to "Array".. THAT is the question
akitchin replied to dtyson2000's topic in PHP Coding Help
i believe he has a series of different "endings" that can be appended to a word, in which case it's likely easier putting them all in an array and picking/choosing which ones he wants to use. -
*SOLVED* Blank screen, What gives in my script?
akitchin replied to Leovenous's topic in PHP Coding Help
my best guess would be that the query is going through, but that one of the pages you're forwarding to (verifypitcher.php, etc) results in the blank page, not the processing script. for the last inserted id, another option is to use mysql_insert_id(), which returns the ID inserted in the last query performed. one way of checking where it is stalling is to use: [code]exit('<pre>'.print_r(get_defined_vars(), TRUE));[/code] this will cease the script wherever you put it and spit out any defined variables (with the local ones being at the bottom most often). then you can take a look at exactly what variables have what values. useful debugging line. hope this helps. -
the reason is exactly as it says - $extension is an array, as you can see in the line you were asking about. to get the right extension, you'd have to figure out what the file's extension is, most likely with strrchr: [code]$real_extension = strrchr($filename, '.');[/code] and replace $extension with $real_extension when you define $file. note that you'll also have to replace $filename with whatever contains the file's actual name.
-
haha i only just noticed that barand's status is "senile." probably because you've been here since the start, but just for that i'm gracing you. any man that old deserves good karma. [b]EDIT: i just noticed that that's a profile setting. barand, i think it takes courage to admit senility.[/b]
-
perhaps i can go ahead and fix the offending line for you: [code]print '<p>Congratulations '.$_POST['$fname'].' '.$_POST['lname'].'! /></p>';[/code] when exiting single quotes, you may include variables as you normally would, without parentheses. let me know how this works out.
-
the problem is that unless register_globals is on (which it shouldn't and needn't be), $fname and $lname will not be registered as local variables. try doing one of the following: 1) setting $fname = $_POST['fname'] and likewise for last name, or 2) using {$_POST['fname']} in place of $fname. i'd suggest the latter, as it is better practice in my opinion (rather than crowding the namespace with useless variables). note that the braces are needed when using array values, since otherwise the single quotes are taken as literals (as far as i remember). hope this helps.
-
i'll go ahead and add my few cents. i neither agree nor disagree with the system, but not many have asked the question "what users will actually pay attention the karma values?" back when i was getting answers here, i would take whatever solutions were presented me and decide (if there were more than one) which one i liked best. along the road, i'd find or make another solution to match my problem. that's the learning process. i never paid attention to who had the most posts, i just took what people were gracious enough to reply with. granted some of it was worthless (read: horse shit), but you get that in every forum. as i said, i'm a neutral party in terms of the system's workings/implications themselves - i simply stake my flag in the "why bother?" category.
-
not sure about DST, but the correct syntax is: DATE_ADD(NOW(), INTERVAL 5 HOUR) the interval type needs to be singular, which has always tripped me up.
-
Ask ONE QUESTION to determine if someone is a good programmer
akitchin replied to sharkyJay's topic in Miscellaneous
it appears saturday (june 17) is already up. neal, care to clue us in?