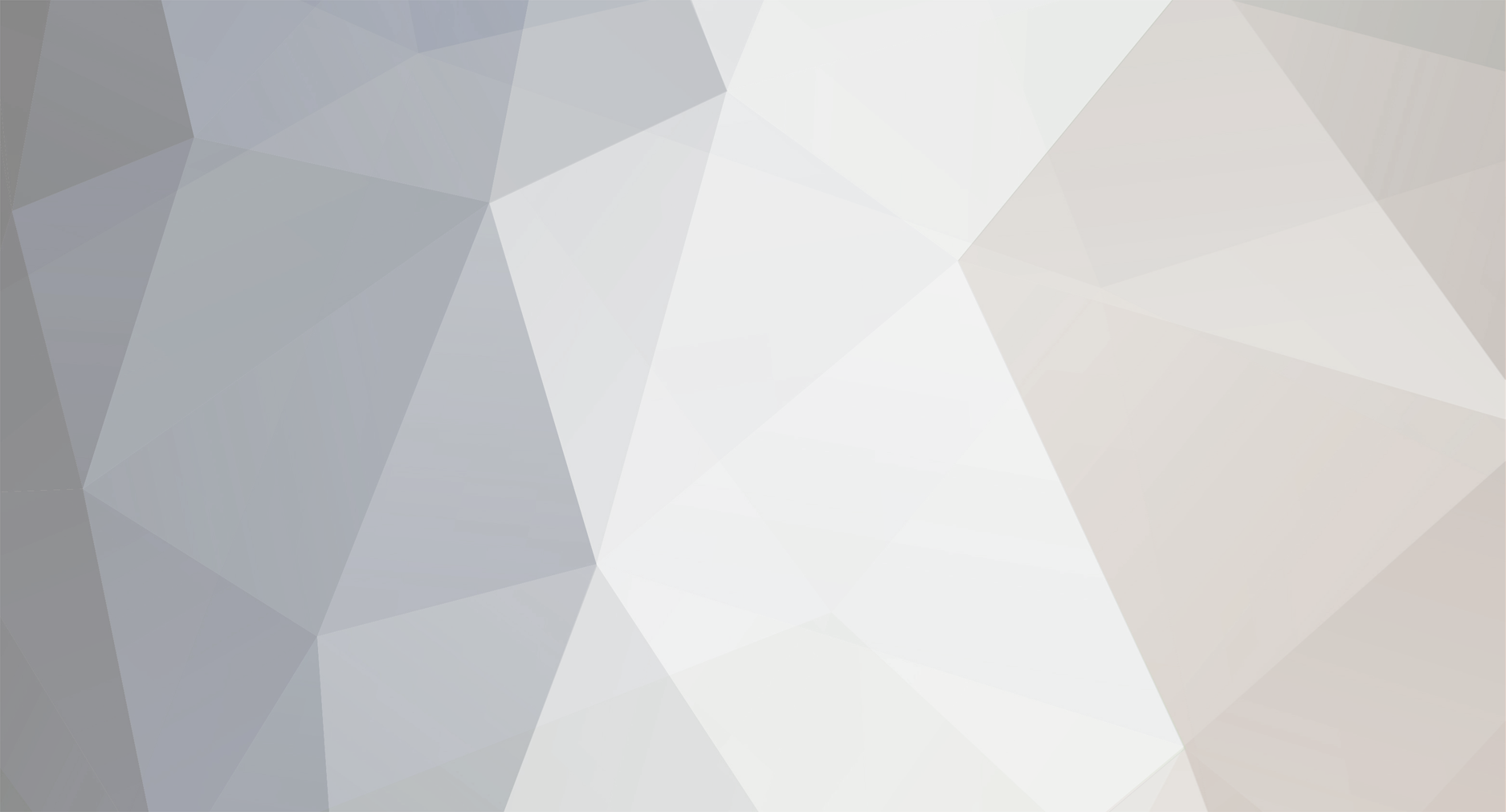
Daniel0
Staff Alumni-
Posts
11,885 -
Joined
-
Last visited
Everything posted by Daniel0
-
Take the first line. Start with the number 0. Each time you encounter a (, add 1, when you encounter ) subtract 1. If you don't end up with 0 again you are missing some
-
They work on the HTTP protocol only so if you run the cron job via HTTP, cookies will work.
-
[SOLVED] General C++ Questions (win32 API)
Daniel0 replied to Ninjakreborn's topic in Other Programming Languages
Do you know what the preprocessor is? The lines starting with hash marks are what's called preprocessor directives. The preprocessor is run before the compiler. The #define directive defines a constant, so when the preprocessor runs it will, in this case, go through the source code and everywhere it finds IDR_MYMENU it will replace it with 101. Then the compiler will run. -
You must include or require the file that contains the captcha class.
-
Which line?
-
I think Mr. Jobs is just talking out of his ass here.
-
[SOLVED] I need to search an array for a string
Daniel0 replied to refiking's topic in PHP Coding Help
So you want to check if it contains any of the words in the array? Then like this perhaps: $results = "I am testing this right now"; $options = array("am", "yes", "check"); $results = explode(' ', preg_replace('#[^a-z ]#', '', strtolower($results))); if (count(array_intersect($results, $options))) { echo 'Successful'; } Or like this: $results = "I am testing this right now"; $options = array("am", "yes", "check"); foreach ($options as $option) { if (preg_match('#\b' . preg_quote($option, '#') . '\b#i', $results)) { echo 'Successful'; break; } } -
Looks like something like this: #!/bin/bash find /home/***/conv/processed -mtime +1 -exec rm {} \; find /home/***/conv/uploaded -mtime +1 -exec rm {} \; find /home/***/conv/tmp -mtime +1 -exec rm {} \; find /home/***/public_html/tmpvidstore -mtime +1 -exec rm {} \; find /***/fetchmp3/public_html/convlog -mtime +1 -exec rm {} \; for DIR in `echo /home/***/public_html/vidcache/*` do find $DIR -mtime +7 -exec rm -rf {} \; done Would do the same.
-
AKA cloaking, a technique the big search engines would ban you for. It's explicitly prohibited in Google's webmaster guidelines.
-
Any reason why you don't just write it in bash?
-
Then you can use reflection. This should work: <?php function callFunction($callback, array $arguments = array()) { if (is_array($callback)) { if (count($callback) == 2 && (is_string($callback[0]) || is_object($callback[0]) && is_string($callback[1]))) { $class = is_object($callback[0]) ? get_class($callback[0]) : $callback[0]; $function = $callback[1]; $reflection = new ReflectionMethod($class, $function); } else { throw new InvalidArgumentException('Invalid callback'); } } else { $reflection = new ReflectionFunction($callback); } $params = $reflection->getParameters(); foreach ($params as $i => $param) { if (!$param->isOptional() && !isset($arguments[$i])) { throw new RuntimeException('Missing required argument ' . ($i + 1)); } } if (is_array($callback)) { if (!is_object($callback[0]) && !$reflection->isStatic()) { throw new RuntimeException('Cannot statically call a non-static method'); } else if (!is_object($callback[0])) { return call_user_func_array($callback, $arguments); } else { $return = $reflection->invokeArgs($callback[0], $arguments); } } else { $return = $reflection->invokeArgs($arguments); } return $return; } The $callback supports the regular callback format except the Class::method() notation. Exceptions are good because they can be caught. Errors cannot easily be caught.
-
Well yeah, but I just consolidated it into a single statement.
-
if (!mysql_num_rows(mysql_query("SELECT * FROM users WHERE username='admin' LIMIT 1"))) { // insert user }
-
Well, yeah just run a query selecting users with that username. If the result set is empty the user doesn't exist and you can run your insertion query.
-
[SOLVED] Problem with checking array & writing
Daniel0 replied to Tonic-_-'s topic in PHP Coding Help
Sorry, change it to $users = array_map('trim', file('users.txt')); Edit: Damn... beat me to it -
Need help with adding items from a folder to a dropdown list?
Daniel0 replied to SimonLewis's topic in PHP Coding Help
You need to add curly brackets around the thing in while. Simply indenting the code isn't sufficient. And then the <select> tag should be moved out of course. I.e.: if ($handle = opendir('/public_html/portraits/')){ echo "<select>"; while (false !== ($Images = readdir($handle))) { echo "<option name='brand'>"; echo "<value='$Images'>$Images</option>"; } echo "</select>"; closedir($handle); } -
[SOLVED] Problem with checking array & writing
Daniel0 replied to Tonic-_-'s topic in PHP Coding Help
Like this? $username = $_GET['user']; $users = file('users.txt'); if (!in_array($username, $users)) { $fp = fopen('users.txt', 'a'); fwrite($fp, $username . PHP_EOL); fclose($fp); } You might want to do some validation on the username though. Otherwise you could insert literally anything into the file. -
You could just not call it if it doesn't exist. $functionName = 'foo_bar_baz'; if (function_exists($functionName)) { $returnValue = call_user_func($functionName); } else { echo 'error'; }
-
Well, as always, there are many ways to do one thing, and which way you would want to use depends on the context.
-
I suppose you could change if (count($where)) { $query .= 'WHERE ' . join(' OR ', $where); } to if (count($where)) { $query .= 'WHERE ' . join(' OR ', $where); } else { $query .= 'WHERE 1=0'; } That would result in no results if all fields are left empty. If you regard all fields empty as an invalid search operation, why not give the user an error instead though?
-
What do you mean with "it doesn't work"? function Smiley($texttoreplace) { $smilies=array( '' => "<img src='images/smile.gif'>", ':blush' =>"<img src='images/blush.gif'>", ':angry' =>"<img src='images/angry.gif'>", ''=> "<img src='images/shocked.gif'>", 'fuck'=>"$#$%", 'Fuck'=>"&$#@" ); $texttoreplace = str_replace(array_keys($smilies), array_values($smilies), $texttoreplace); return $texttoreplace; } echo Smiley('foo bar '); Outputs: foo <img src='images/smile.gif'> bar <img src='images/shocked.gif'>