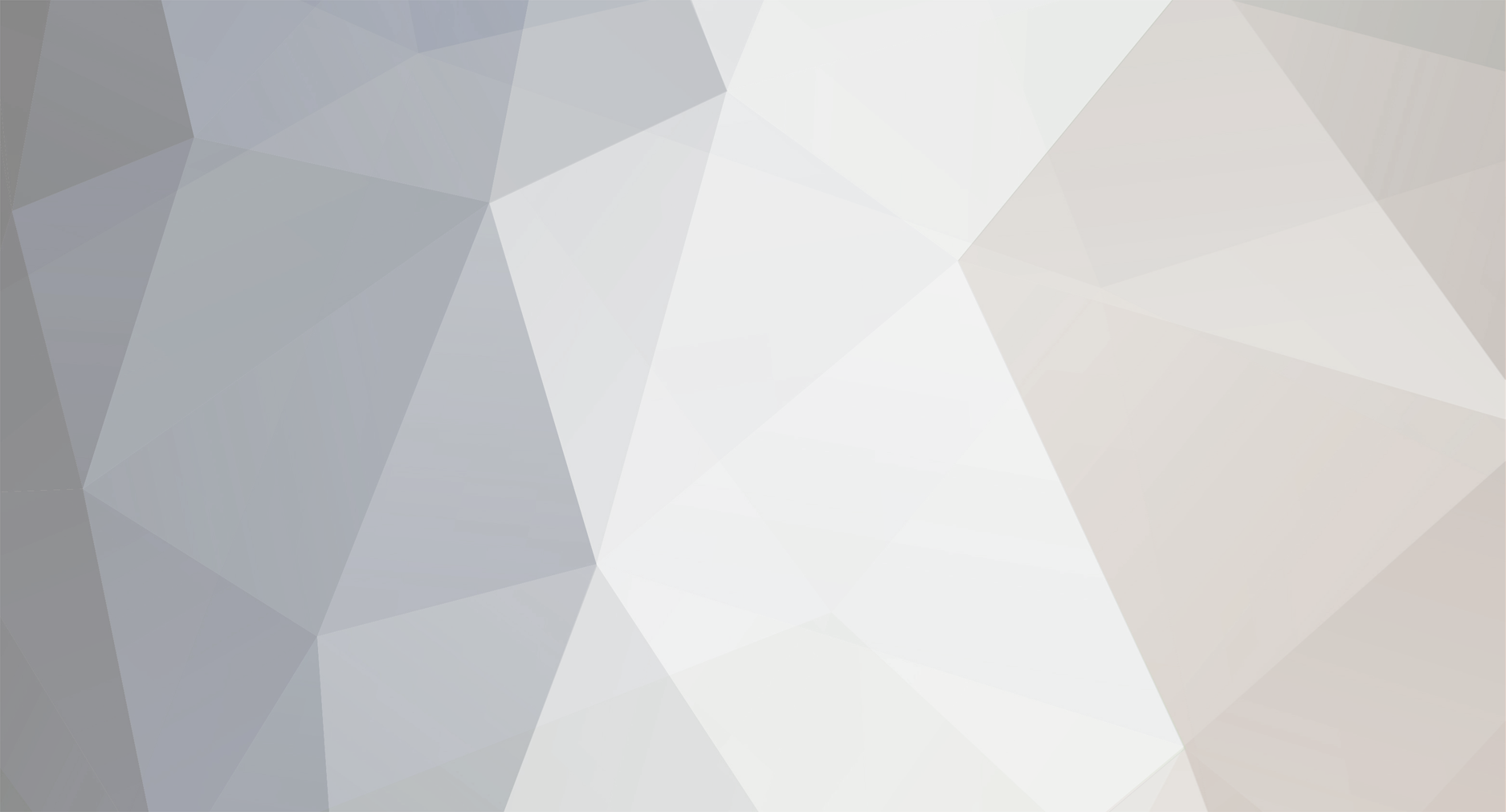
Daniel0
Staff Alumni-
Posts
11,885 -
Joined
-
Last visited
Everything posted by Daniel0
-
Hmm... $a = array( array('title' => 'ROOT 1', 'depth' => '0'), array('title' => 'CHILD 1 OF ROOT 1', 'depth' => '1'), array('title' => 'CHILD 2 OF ROOT 1', 'depth' => '1'), array('title' => 'CHILD 1 OF CHILD 2', 'depth' => '2'), array('title' => 'ROOT 2', 'depth' => '0'), ); $prevDepth = null; foreach ($a as $element) { if (null === $prevDepth || $element['depth'] > $prevDepth) { echo '<ul>'; } else if ($element['depth'] == $prevDepth) { echo '</li>'; } else if ($element['depth'] < $prevDepth) { echo str_repeat('</li></ul>', $prevDepth - $element['depth']) . '</li>'; } echo "<li>{$element['title']}"; $prevDepth = (int) $element['depth']; } echo str_repeat('</li></ul>', $prevDepth+1); Output: <ul><li>ROOT 1<ul><li>CHILD 1 OF ROOT 1</li><li>CHILD 2 OF ROOT 1<ul><li>CHILD 1 OF CHILD 2</li></ul></li></ul></li><li>ROOT 2</li></ul> which is valid (X)HTML.
-
You cannot close a user's window.
-
mvc what m stands for what v stands for what c stands for
Daniel0 replied to fantomel's topic in Miscellaneous
If you understand the theory in object oriented design, then you would know that no design pattern is set in stone. There can be many different implementations of a particular pattern and a specific implementation is not necessarily better or more "correct" than another. -
Amend it? You just do like this: That is literally the only thing you need to do... There is no need to write your own function.
-
No it is not (except you're misusing sessions), but as previously mentioned, why not use the built-in pathinfo when it's there?
-
Also, the explode() method is more expensive. Consider how you would implement explode() and strrpos() yourself. function myStrrpos($haystack, $needle, $offset = 0) { for ($i = strlen($haystack) - 1 - $offset; $i >= 0; $i--) { if ($haystack[$i] == $needle) { return $i; } } return false; } function myExplode($delimiter, $string, $limit = null) { $parts = array(); for ($i = 0, $j = 0, $length = strlen($string); $i < $length; $i++) { if ($string[$i] == $delimiter && ($limit === null || $limit > $j+1)) { $j++; continue; } if (!isset($parts[$j])) { $parts[$j] = $string[$i]; } else { $parts[$j] .= $string[$i]; } } return $parts; } It takes up more memory processing power to explode a string into parts by a delimiter than simply searching backwards for a particular character.
-
$i = strrpos($str,"."); This searches backwards in $str for a dot and stores the position in $i (the first character has position 0). See: strrpos. if (!$i) { return ""; } strrpos returns FALSE if no match is found, so if it's not the case that $i evaluates to true (i.e. it's false), an empty string is returned. $l = strlen($str) - $i; Here the the length of $str is found, and $i is subtracted, or in other words, $l now contains the length of the extension. See: strlen. $ext = substr($str,$i+1,$l); substr returns a substring, i.e. part of a string. The first argument is the string, the second is the starting position, and the third is the number of bytes to get (or the length if you wish). In this case the third argument (and thus the statement above this line) is irrelevant. File extensions are always on the end of the filename and substr goes until the end of the string by default. The result is stored in a variable called $ext. This could have been substr($str,$i+1). return $ext; The extension is returned. There is a function called pathinfo though, so you could just have done this: $ext = pathinfo($str, PATHINFO_EXTENSION); That won't work. What if it's called foo.bar.txt. Your function will return bar, but the extension is txt.
-
mvc what m stands for what v stands for what c stands for
Daniel0 replied to fantomel's topic in Miscellaneous
No offense, but if you don't understand the theory, how do you plan on creating a framework? It'll probably not end up being of very high quality and probably not be that usable for other people. You're better off using a good framework like ZF so you can learn how well-designed code looks and works while you're learning about the theory yourself too. When you have developed a solid understanding of application design and object oriented design you can perhaps make a framework yourself. -
One User -> Multiple Simultaneous Logins
Daniel0 replied to black.horizons's topic in Application Design
You needn't set a cookie as well. -
If you want to check that the page exist, just check that it returns a 200 status code.
-
One User -> Multiple Simultaneous Logins
Daniel0 replied to black.horizons's topic in Application Design
And how are you having trouble implementing that? It's fairly straightforward. Store the user ID using sessions. Fetch the user from the database that corresponds to the ID. If the ID is not set the user is logged out. -
Is there an easier way of doing this array loop
Daniel0 replied to cliftonbazaar's topic in PHP Coding Help
How does your array look? If it's an ordered list you can use binary search instead. That will run in logarithmic time instead of linear time, which will be faster. Edit: Here is an example of binary search: function binarySearch($needle, array $haystack) { $lowerBound = 0; $upperBound = sizeof($haystack) - 1; while ($lowerBound < $upperBound) { $mid = intval($lowerBound + (($upperBound - $lowerBound) / 2)); if ($haystack[$mid] < $needle) { $lowerBound = $mid + 1; } else { $upperBound = $mid; } } if ($haystack[$lowerBound] == $needle) { return $lowerBound; } else { return false; } } $haystack = range(1, 100000); $find = mt_rand(1, 100000); $pos = binarySearch($find, $haystack); echo "Find: {$find}\n"; echo "Pos: {$pos}\n"; echo "Value: {$haystack[$pos]}"; Here we have a problem size N=100000. Worst case scenario we will have to use ceil(log2(N))=17 steps because the problem size is halved on each step. Using linear search, which is what you were doing, worst case scenario is N steps. This only works if the lookup values are ordered though. -
There are a few tools that will make sprites easier for you. E.g. http://csssprites.com/ or http://spritegen.website-performance.org/
-
Help me format this php PDO statement properly
Daniel0 replied to cunoodle2's topic in PHP Coding Help
And my point is that statement is incorrect. Using his example: <?php $var="User', email='test"; $a=new PDO("mysql:host=localhost;dbname=database;","root",""); $b=$a->prepare("UPDATE `users` SET user=:var"); $b->bindParam(":var",$var); $b->execute(); ?> is the exact equivalent of <?php $var="User', email='test"; $a=new PDO("mysql:host=localhost;dbname=database;","root",""); $b=$a->prepare("UPDATE `users` SET user=:var"); $b->execute(array(':var' => $var)); ?> You can test it yourself if you want to. -
Should be if (!preg_match("/^.*\.(gif|jpg|png)$/i",$file)) { though. Otherwise e.g. foo.jpg.bar would match as well (note the added $.
-
Help me format this php PDO statement properly
Daniel0 replied to cunoodle2's topic in PHP Coding Help
Well, admin at wdfa dot co dot uk is only partially correct. He is not doing much else than stating the obvious. If you have $var = "User', email='test"; then his string passed to PDO::prepare() evaluates to UPDATE `users` SET user='User', email='test' because string variable interpolation takes place before the string is passed as argument to a function or method. Again, that is covered in the manual: http://php.net/manual/en/language.types.string.php The manual entry for PDOStatement::execute() explicitly says that you can either pass an array or bind them manually using PDOStatement::bindParam(). Do remember that the comments can be submitted by anyone and the comments are not an authority. The manual entries on the other hand are authoritative. -
That is not what he wanted though. He wanted entries on a particular day, not entries within a 24 hour interval. If you want the entries for e.g. May 23rd, but the time is 11:00 AM, then -24 hours up until now would be May 22nd 11:00 AM to May 23rd 11:00 AM. This means you, incorrectly, will get entries from the yesterday as well. What you can do is something like this: $timestamp = time(); $start = mktime(0, 0, 0, date('n', $timestamp), date('j', $timestamp), date('Y', $timestamp)); $end = mktime(23, 59, 59, date('n', $timestamp), date('j', $timestamp), date('Y', $timestamp)); $getcount = "SELECT * FROM `signatures` WHERE `timestamp` BETWEEN {$start} AND {$END}";
-
Help me format this php PDO statement properly
Daniel0 replied to cunoodle2's topic in PHP Coding Help
Yet better than posting false information I would argue. If you would read the manual (sorry, chaking) you would see that PDOStatement::execute() returns a boolean value. Scalar values do not have any methods, only objects do. Further, if you read the pages Axeia linked to you would see that errorCode() is a member of PDOStatement, so it's $stmt->errorCode() and not $user_info->errorCode(). -
Help me format this php PDO statement properly
Daniel0 replied to cunoodle2's topic in PHP Coding Help
Maybe if all of you would bother to use the manual for its intended purposes it would all become clear: http://php.net/manual/en/pdostatement.execute.php No. -
Okay so I take it it's a UNIX timestamp. You can just check if the timestamp is within range of the possible UNIX timestamps for that particular day.
-
I think it can make a difference. On the recent templates you sent me* I cut off about 20 HTTP requests. That's quite a lot considering that it's 20 requests less on each page view. It's 20 times less the browser will have to check with the server if there is anything new going on. Around 80% of the load time is network activity and execution on the on the client side, so performance wise that is where optimization efforts should be primarily focused at. _________ * Sorry those of you who don't know what I'm talking about. I suppose you'll find out soon enough.
-
What type is your timestamp field?
-
One User -> Multiple Simultaneous Logins
Daniel0 replied to black.horizons's topic in Application Design
Well, cookies can't be relied on in the same way that the keys to your doors can't be relied on to protect you from burglary. Whenever someone has the keys/cookies they have access to whatever the keys/cookies grant access to. Cookies are merely a way of persisting data across multiple requests. Sessions are a layer on top of the cookies. -
One User -> Multiple Simultaneous Logins
Daniel0 replied to black.horizons's topic in Application Design
No, browsers employ a same-origin policy to prevent exactly that scenario. Otherwise badsite.com would be able to read the cookies set for goodsite.com. -
"It" (HTML/JSON/XML/whatever) is sent whenever there is something to send.