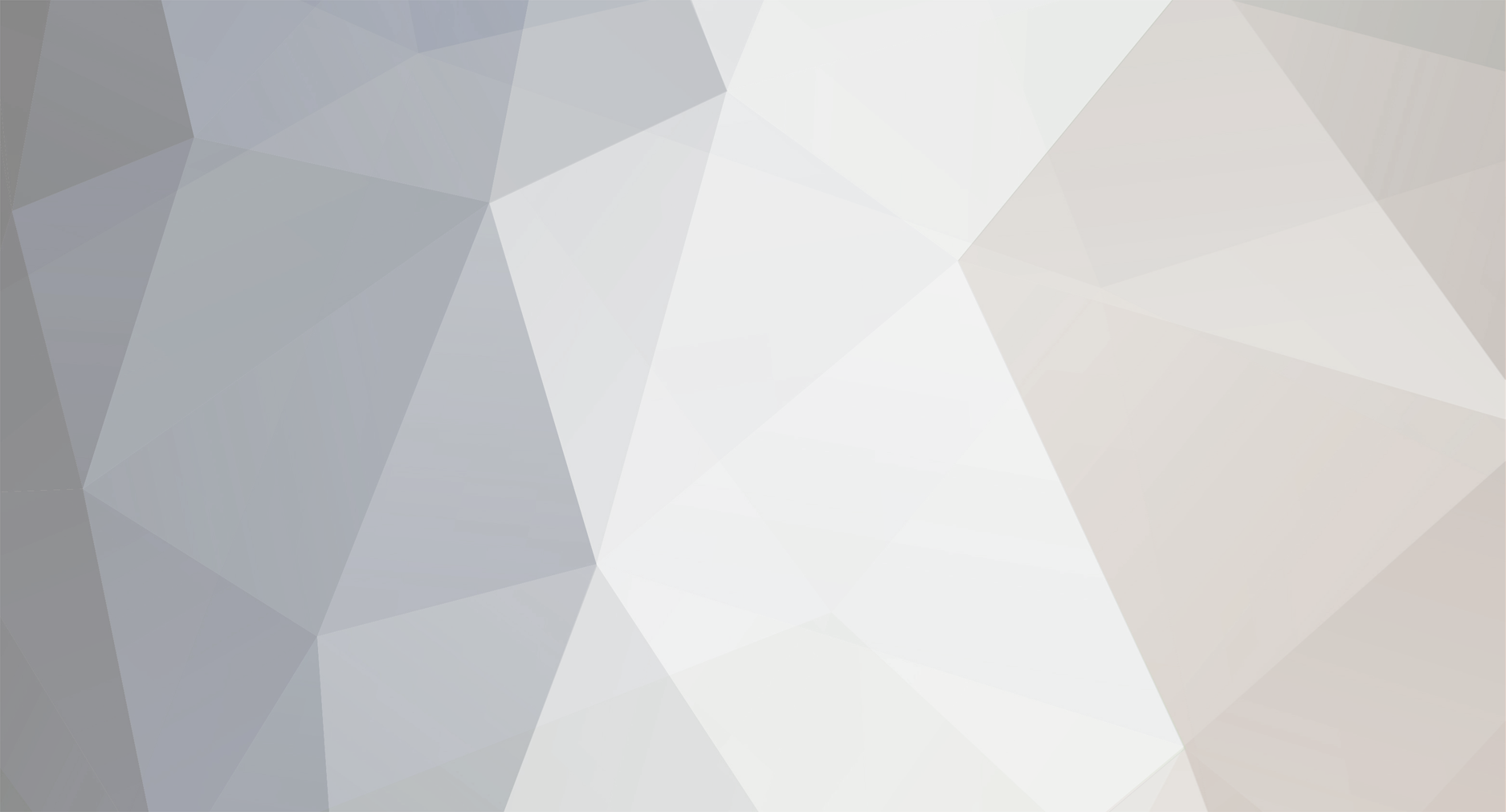
Daniel0
Staff Alumni-
Posts
11,885 -
Joined
-
Last visited
Everything posted by Daniel0
-
Make sure you have an MTA installed and configured correctly on your computer.
-
If you don't already own a Vista license, don't bother with it. Stick with XP until 7 is released later this year. Vista is a fine OS though, and it's what I primarily use.
-
How about this: $str = <<<EOF foo bar <br /><br/><br> <BR>hello<br>test EOF; $str = preg_replace('#(<br ?/?>\s*)+#i', '<br>', $str); echo $str; That will output: foo bar <br>hello<br>test No, \s means any whitespace character (line break included). If you just used \n then it wouldn't work if there were e.g. spaces or tabs in between.
-
Try this then: $str = preg_replace('#(<br ?/?>){2,}\s*#i', '', $str); \s means any whitespace character.
-
$str = preg_replace('#(<br ?/?>){2,}#i', '', $str); Should work. Jack, the quantifier in your pattern is the the closing >. You need to group it in parenthesis to have it work on multiple characters.
-
There are some problems. First of all, you access a property like $this->name and not like this->$name. The latter is actually syntactically incorrect, so it wouldn't even parse. Secondly, you declared employee as abstract, but in page2.php you're trying to instantiate it. That's not allowed either. It also seems like many of the properties are not relevant for all of your classes. E.g. workingdays seems to be only applicable for parttime employees. In that case it should belong in that class. Here is an example of how you might do it: abstract class DomainObject { /** * Name of the table. * * @var string */ protected $_tableName; /** * Name of primary key. * * @var string */ protected $_pk; /** * Array of field names. * * @var array */ protected $_fields; /** * Array of changed fields. * * @var array */ protected $_dirty = array(); /** * Array of current data. * * @var array */ protected $_data = array(); private $_disableDirty = false; /** * Array of original data. * * @var array */ protected $_originalData = array(); public function __construct(array $data = array()) { if (!isset($this->_tableName)) { throw new RuntimeException('Table name is not set.'); } if (!isset($this->_fields) || count($this->_fields) == 0) { throw new RuntimeException('No fields are set.'); } if (!isset($this->_pk)) { throw new RuntimeException('Primary key is not set.'); } else if (!in_array($this->_pk, $this->_fields)) { throw new RuntimeException('Primary key is not in the field list.'); } $this->_disableDirty(true); foreach ($data as $field => $value) { $this->setValue($field, $value); } $this->_disableDirty(false); } /** * Saves dirty fields to database. */ public function save() { if (count($this->_dirty) == 0) { // nothing to do return; } $sql = 'UPDATE ' . $this->_tableName . ' SET '; $updates = array(); foreach ($this->_dirty as $fieldName) { $updates = $fieldName . " = '" . mysql_real_escape_string($this->_data[$fieldName]) . "'"; } $sql .= join(', ', $updates) . ' WHERE ' . $this->_pk . " = '" . mysql_real_escape_string($this->_data[$this->_pk]) . "';"; mysql_query($sql); $this->_dirty = array(); } public function setValue($field, $value) { if (!in_array($field, $this->_fields)) { throw new OutOfBoundsException("Invalid field '{$field}'."); } $this->_data[$field] = $value; if (!$this->_disableDirty && !in_array($field, $this->_dirty)) { $this->_dirty[] = $field; } return $this; } public function getValue($field) { if (!in_array($field, $this->_fields)) { throw new OutOfBoundsException("Invalid field '{$field}'."); } return $this->_data[$field]; } public function __get($name, $value) { $this->getValue($name, $value); } public function __set($name) { $this->setValue($name); } protected function _disableDirty($state = true) { $this->_disableDirty = (bool) $state; return $this; } } class Employee extends DomainObject { protected $_tableName = 'employees'; protected $_pk = 'employee_id'; protected $_fields = array( 'employee_id', 'name', 'salary', ); } $john = new Employee(array('employee_id' => 1234, 'name' => 'John Doe', 'salary' => 43)); $john->salary = 40; // sorry john $john->save(); You could then write some (perhaps static) methods that are responsible for fetching data so you don't have to pass it manually to the constructor. You might also want to have some way to get an instance of e.g. MySQLi or PDO (or your own wrapper around these). Please note that I didn't test any of that code.
-
It's not just that page, but rather all pages. I didn't read that page nor some of the others actually, but perhaps some of their other content is "theft-worthy". I don't know.
-
Haha... that's pretty retarded. Try to get a screen reader to read that
-
The pattern <[\s]*title[\s]*>([^<]+)<[\s]*/[\s]*title[\s]*> Means this: < Match < [\s]* Match a whitespace character 0 or more times title Match title [\s]* Match a whitespace character 0 or more times > Match > ([^<]+) Match any character 1 or more times which is not < and store these in backreference 1 < Match < [\s]* Match a whitespace character 0 or more times / Match / [\s]* Match a whitespace character 0 or more times title Match title [\s]* Match a whitespace character 0 or more times > Match >
-
[SOLVED] Working out 10% of a number always increasing
Daniel0 replied to killah's topic in PHP Coding Help
$number * .05 Learn math before PHP (and learn to post in the correct boards). -
Sort of like this? Adjust column names. You can probably sort using MySQL as well. function sortByCustomer(array $a, array $b) { if ($a['customer_id'] == $b['customer_id']) { return 0; } return ($a['customer_id'] < $b['customer_id']) ? -1 : 1; } $data = array( array( 'item_name' => 'Foo bar test', 'price' => 48, 'qty' => 2, 'customer_name' => 'John Doe', 'customer_id' => 5, ), array( 'item_name' => 'Foo bar test', 'price' => 48, 'qty' => 3, 'customer_name' => 'Daniel Egeberg', 'customer_id' => 2, ), array( 'item_name' => 'Hello world', 'price' => 52, 'qty' => 1, 'customer_name' => 'Daniel Egeberg', 'customer_id' => 2, ), array( 'item_name' => 'Test Item', 'price' => 15, 'qty' => 4, 'customer_name' => 'John Doe', 'customer_id' => 5, ), ); $totals = array(); usort($data, 'sortByCustomer'); $currentName = $data[0]['customer_name']; $newCustomer = true; echo "Item name:\tPrice:\tQty:\tTotal:\n"; foreach ($data as $i => $purchase) { if ($newCustomer) { echo "\n" . $purchase['customer_name'] . ":\n"; $newCustomer = false; } $total = $purchase['qty'] * $purchase['price']; printf("%s\t\$%.2f\t%d\t\$%.2f\n", $purchase['item_name'], $purchase['price'], $purchase['qty'], $total); $totals[$purchase['customer_id']] = isset($totals[$purchase['customer_id']]) ? $totals[$purchase['customer_id']] + $total : $total; if (!isset($data[$i+1]) || $data[$i+1]['customer_id'] != $purchase['customer_id']) { printf("Total: \$%.2f\n", $totals[$purchase['customer_id']]); $newCustomer = true; } } printf("\n\nTotal (all customers): \$%.2f", array_sum($totals));
-
Yes, you can, but if you don't have any programming experience then don't bother. Find an easier project to start with. Ebay is a pretty complex website.
-
Why can't you use auto increment?
-
Query: post reply in PHP Freelancing?
Daniel0 replied to rupam_jaiswal's topic in PHPFreaks.com Website Feedback
Rumor has it that sticky topics contain important and/or useful information: http://www.phpfreaks.com/forums/index.php/topic,167887.0.html -
Inheritance can be seen as an "is a" relationship. So unless your timesheet is an employee it shouldn't be a descendant. Like a poodle is a dog, but a dog isn't a poodle, and your poodle's collar isn't a poodle. You could think about OO inheritance as being sort of the same as the taxonomy used in biology for living organism, if that helps you. Sort of overall you have "life" and then within life you have domains, then kingdoms, etc. So a poodle shares all the common traits that dogs have, but it also has some specific traits that that dogs in general do not have. Your poodle, let's call it Fluffy, would be an instance of the object Poodle. Fluffy's collar wouldn't be an instance of Poodle, it isn't even a dog or even a living being. It's an entirely different thing, so it Poodle and Collar don't even share common ancestor classes. This type of relationship could be described as "has a", or more precisely aggregation. Fluffy also has a heart, among other things, but this wouldn't be aggregation, but rather composition. Let's try to explain it in PHP: abstract class Dog { protected $_name; protected $_collar; protected $_heart; public function __construct($name) { $this->_name = $name; $this->_heart = new Heart(); } public function getName() { return $this->_name; } public function setCollar(Collar $collar) { $this->_collar = $collar; return $this; } /** * Each kind of dog barks in a different manner, * so we might want to declare the bark() method * as abstract. */ abstract public function bark(); } class Poodle extends Dog { public function bark() { // implementation here... } // more poodle specific stuff here... } class Collar { // class members here } class Heart { // class members here } $fluffy = new Poodle('Fluffy'); $fluffy->setCollar(new Collar()); $fluffy->bark(); The difference between aggregation and composition is essentially that with aggregation, objects persist after their container object has been destroyed. Fluffy's collar doesn't somehow cease to exist just because Fluffy dies. If your company closes, your employees wouldn't immediately die. Conversely, if Fluffy dies then the heart would as well. Yes, except I don't like the part where you call it "main object". The idea of attributes is correct though. Yes and no. Creating an employee would equate to creating a new instance of the employee class (e.g. new Employee();). When you have an instance of a particular employee, editing it would perhaps be modifying its attributes somehow, and you could perhaps then have a save() method. Your employee class shouldn't have a method that would display details about it, but rather expose the data via an logical interface such that the information can be retrieved, but handled in various ways by other objects. I hope this clears up some of the stuff about OOP. At any rate, OOP is a far too big topic to cover in a topic on a forum, so what I would suggest you do is to get some good books (search the forums for recommendations). You are of course then very welcome to ask about any particular problems you might run into.
-
$left = $leftOrig = (int) $_POST['num1']; $right = $rightOrig = (int) $_POST['num2']; $c = $r = 0; for ($t = PHP_INT_MAX; (bool) $t; $t >>= 1) { $r <<= 1; $r |= ($left ^ $right ^ $c) & 1; $c = (($left | $right) & $c | $left & $right) & 1; $left >>= 1; $right >>= 1; } for ($t = PHP_INT_MAX, $c = ~$t; (bool) $t; $t >>= 1) { $c <<= 1; $c |= $r & 1; $r >>= 1; } echo "{$leftOrig} + {$rightOrig} = {$c}";
-
Yeah that was essentially the problem in my post as well. If the monitor fails, who monitors the monitor?
-
Which log did you check? You need to check PHP's and not Apache's. Also, you need to ensure that error logging is turned on, obviously.
-
I don't like the PHP hello world script that much. It's too short. Take a look at the C hello world program for instance: #include <stdio.h> int main(int argc, char** argv) { printf("Hello World"); } There are a lot of more things you can talk about: The preprocessor and its directives, header files, datatypes (int, char, etc.), functions, function signatures, library functions, pointers, strings (as arrays of chars), arrays as pointers, command line arguments.
-
'position-1' is a string literal whereas position-1 is the field name called position subtracted by 1.
-
Heh... that'll work too
-
Select them and recalculate their position values according to their order. SELECT id FROM foo WHERE bar='something' ORDER BY position ASC; Now loop through them. Start with position=1 and increment it on each iteration. Then save to database.
-
Really? Your not supposed to do that? That's really interesting, and I never knew that. Is there a reason why, or is it just a bad practice? What could happen to the date if it was stored in a varchar? It's bad practice and it prevents you from using comparison operators and date functions efficiently on them using MySQL. You could use UNIX timestamps, but MySQL DATE(TIME) fields can store large ranges of dates.
-
Use IDs for selecting using Javascript instead.
-
Nope, not in the way I laid it out. Say you got three "thumbs": 2 up = 2*1 = 2 1 down = 1*0 = 0 2 + 0 = 2, so it's got like 2 "points", but there were 3 votes. So 2/3 = .67, i.e. 67% liked it (67% gave thumbs up, the rest down). Had all of the three votes been thumb down, then it would be 0/3=0%, and had all of them given thumbs up then it would be 3/3=100%.