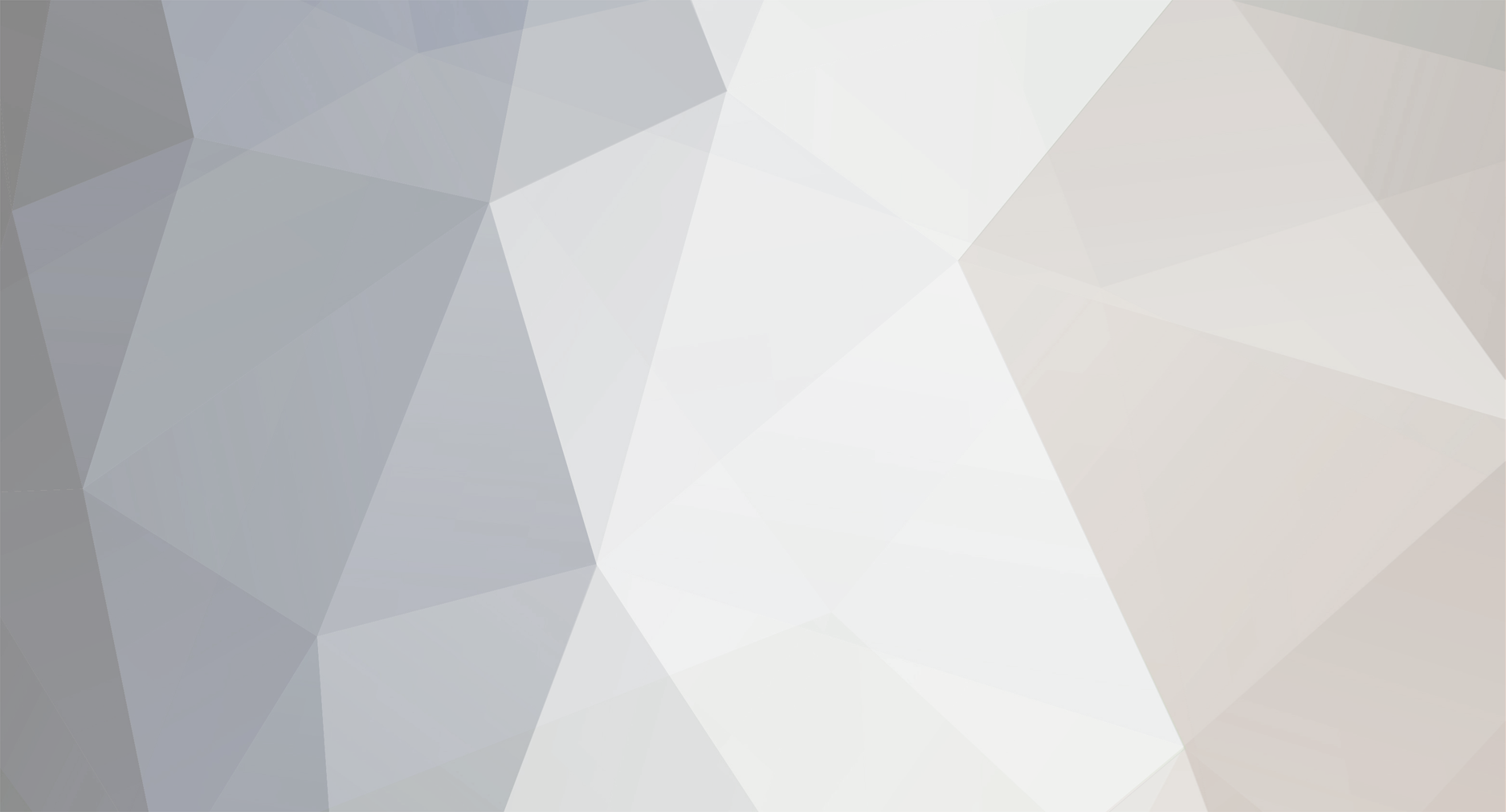
Daniel0
Staff Alumni-
Posts
11,885 -
Joined
-
Last visited
Everything posted by Daniel0
-
I don't think the protocol (FTP vs. HTTP) should matter. Disk I/O on both ends and network throughput should be the only real limiting factors.
-
[SOLVED] topic solved suggestion
Daniel0 replied to shadiadiph's topic in PHPFreaks.com Website Feedback
Clicking the topic solved button should take you back to the topic. From there you can just enter your post in the quick reply box (assuming you've turned it on) and hit "Post". You can also configure it such that you are returned to the topic when you reply to it. -
[SOLVED] "MESSAGES[1]", but no messages
Daniel0 replied to Maq's topic in PHPFreaks.com Website Feedback
I've changed it manually using the MySQL console. -
OOP Databases Singletons and Registries
Daniel0 replied to black.horizons's topic in Application Design
Copy data from one server to another. Read data from an external server without disconnecting from local server. As I said, what you need right now doesn't matter in this instance because you can't know what you need in the future. For that reason you need to design a system that doesn't lock you in. Even then there is still the coupling issue that needs to be taken into account. -
OOP Databases Singletons and Registries
Daniel0 replied to black.horizons's topic in Application Design
A database wrapper, though often used as an example, is in my opinion not a good candidate for using the singleton pattern. What if you later find that you need to open another connection? If that's the case then you're out of luck as you've designed your system to only support one connection. You've coded to an interface for the singleton and you would have to go through all your code to migrate to another interface. That could turn out to be very time consuming. You can't say now that you probably won't need it unless you have the ability to look into the future. One of the strengths of OOP is that you are making your code modular and reusable, but by using the singleton pattern you are enforcing a particular type of usage, which locks you in, and which couples you very tightly. Consider the differences between these methods: public function something() { $db = Database::getInstance(); // using singleton $db->doStuff(); } public function something(Database $db) // passed by argument and using type hinting { $db->doStuff(); } There is one fundamental difference between these two methods even though they do the same. The first one is limited to always using a Database. The second one can use descendants of Database. If you have another class CustomDatabase that extends Database then you could use that one in variant two, but not easily in the first variant. One of the most important design principles is that you should code to an interface, not to an implementation. The first variant of the method fails to obey this principle because it automatically assumes that it will be specifically a Database and not potentially any descendant of that. The first one can only be changed by going through the code and changing it, i.e. it's determined at compile time. The second one can be changed dynamically at run time, which design wise is much better because it means it will have a looser coupling. The singleton pattern is in my opinion way too overused. I suppose it's because it's conceptually pretty easy to comprehend and it's also easy to implement and it gives you easy access. This easy access has several quite serious drawbacks though. Just because it's a pattern doesn't mean it's a good pattern nor that you should use it. I can't think of even one good instance where a singleton would be a better option than passing an object by argument. I'm not too much of a fan of the registry pattern either. It's mainly because it's stores elements globally. It's difficult to ensure the integrity of global objects. From within a class you can't know who have touched it, and you can't know if it contains the correct value anymore. In most registry implementations people don't check values that are put in the registry. Most people just create a registry that takes all possible data. I don't like the idea of having one big hole where you dump everything into. Again, I think it's a much better idea to pass by argument. There will always be a way of writing OO code without using neither a singleton nor registry, and I think it would be better to do that. -
is this css to round edges ? It is in Gecko based browsers. Neither -moz-border-radius nor -webkit-border-radius are valid CSS attributes though. There is an attribute in CSS3 called border-radius.
-
You mean the one called "FTP Programs" in the board you just posted in? Locked.
-
There is no best language. You could do it in Perl, Python, Ruby, PHP, etc. The most secure would be to store the files above the document root. In that way you would never be able to access it directly through a browser. You can then serve it dynamically using PHP, or you could send it as an email attachment. I don't know if there is any readily available application that will do what you want, but you can see an example of dynamically serving a file here, and various library classes such as Zend_Mail or the ones you would find in PEAR will enable you to easily send MIME multi-part messages (which is what you need for attachments). Strictly speaking you don't need any information other than whether the transaction was successful and a way to identify customers. Ideally you would store it in a database. Most shopping cart systems would ship with integration with a couple of CC processors. All the usual stuff should suffice. See this tutorial for instance: http://www.phpfreaks.com/tutorial/php-security I'm not sure what kind of advice you want, but make sure you read the following chapters of the manual: "Language Reference", "Features" and "Security". You'd probably want to check out the existing shopping carts that are available. It's not the easiest type of application to start out with for a beginning programmer. Not at all. Nobody has an innate knowledge in any fields. The annoying ones are the ones who don't bother to research themselves. Clearly you've done that.
-
FYI wildteen, the curly bracket notation is deprecated as of PHP 6, so it would be better to use the square bracket like you do with arrays. See: http://www.php.net/manual/en/language.types.string.php#language.types.string.substr
-
I hate the term megapixel. It's the single thing most people look for when buying a camera. "My phone has like got a 234234234324 MP camera!!11one!". Yeah, but it probably has a crap lens, so it doesn't really matter. GTFO.
-
Security Test - American Gangsters
Daniel0 replied to DeanWhitehouse's topic in Beta Test Your Stuff!
Okay then, if you're too don't want to check for yourself. strip_tags removes HTML tags from a string. htmlentities converts characters to their respective HTML entities (e.g. © -> ©) filter_var with FILTER_SANITIZE_STRING removes HTML tags plus some extra optional stuff. This has already been done. trim removes whitespace from both ends. That's fine. stripslashes strips slashes. How do you know that's needed? mysql_real_escape_string escapes things for MySQL queries. This is the only thing you needed here. You shouldn't escape things like HTML entities before inserting it into the database, but rather do it before you output it. You should store the raw data in the database. Better yet, just use prepared statements and your strings will automatically be sanitized. darkfreaks just listed a lot of functions for sanitation, most of which were irrelevant to that problem, and you just slammed all of them in one big function. -
Security Test - American Gangsters
Daniel0 replied to DeanWhitehouse's topic in Beta Test Your Stuff!
Sorry, my last post was meant to say that your function sucks, and that reading the relevant manual pages should make it clear why... -
Pre-coding Pre-installation Help NOOB
Daniel0 replied to Tr4mpldUndrfooT's topic in Application Design
You'll really only need one database. As for the host, I'd be inclined to recommend buying a VPS, but considering you're new to this stuff, you probably don't know how to administrate a server yourself either. There is a sticky topic in the misc. forum with a lot of web host suggestions. -
Security Test - American Gangsters
Daniel0 replied to DeanWhitehouse's topic in Beta Test Your Stuff!
Please read the individual manual pages for the functions to see what they do... -
This is how I think I would do it (sort of): topics topic_id (PK) forum_id (FK -> forums) updated_at (DATETIME - gets updated with NOW() when somebody posts in that topic, or the topic, or posts therein, are edited) etc. forums forum_id (PK) etc. log_topics_read user_id (FK -> users) topic_id (FK -> users) read_at (DATETIME) (make user_id and topic_id a combined unique index) users user_id (PK) etc. Okay, so when a logged in user user_id=123 views a topic with topic_id=321 you would run the following query: INSERT INTO log_topics_read (user_id, topic_id, read_at) VALUES (123, 321, NOW()) ON DUPLICATE read_at=NOW(); When you are viewing the topic listing you would simply left join the log_read table with the topics table (and possibly other tables if you wish). So when you've done that you can check if the user has read it. If the left joined read_at value is NULL, then the user hasn't read it at all, and if the timestamp is earlier than the topic.updated_at then there have been some sort of update to that topic since the user last viewed it. If you are concerned about the size of the database then you can just automatically mark topics that haven't been updated for X days as read and prune log_read entries that are over X days old.
-
Security Test - American Gangsters
Daniel0 replied to DeanWhitehouse's topic in Beta Test Your Stuff!
You want a warning for trolling -
I think it'll be more efficient storing what they have read instead of what they haven't read. Imagine you want to store read/unread information for a month and assume anything older is just automatically marked as read. This forum, which is rather large, but not huge, had 25,117 posts in April. So, when a member registers you using your method you would also create that many new rows in the table. In April there was an average of 43.5 registrations each day, so that would mean 1,092,589.5 new rows to the unread table for just those people. The table would very quickly become enormous, and it's unlikely these people are going to read all those posts within a month. It would be much more efficient storing what they have read.
-
You can imagine functions as mini-programs within your program that are readily executable at any time. You can pass information to these via arguments and you can return information back from that function. A function can also have what's called a side-effect, which could be things like printing output to the screen, creating a file, etc.
-
Won't work. Chrome doesn't know how to interpret PHP.
-
It works for me. Install a web server on your computer though.
-
It's probably just a str_replace() somewhere in the file that's responsible for sending the email.
-
Store it in a config file?
-
Correction: PHP outputs plaintext. PHP usually outputs plaintext to a browser. A browser interprets it as HTML by default. PHP can output anything you want.
-
I mean in general. Doing that or die() trick is a horrible way of doing error handling. It can't be caught or logged, and it can't be hidden in a production environment.
-
You'll probably have to elaborate on how it doesn't work. You're just multiplying two numbers. I don't see how that can fail unless you enter a huge number so it overflows.