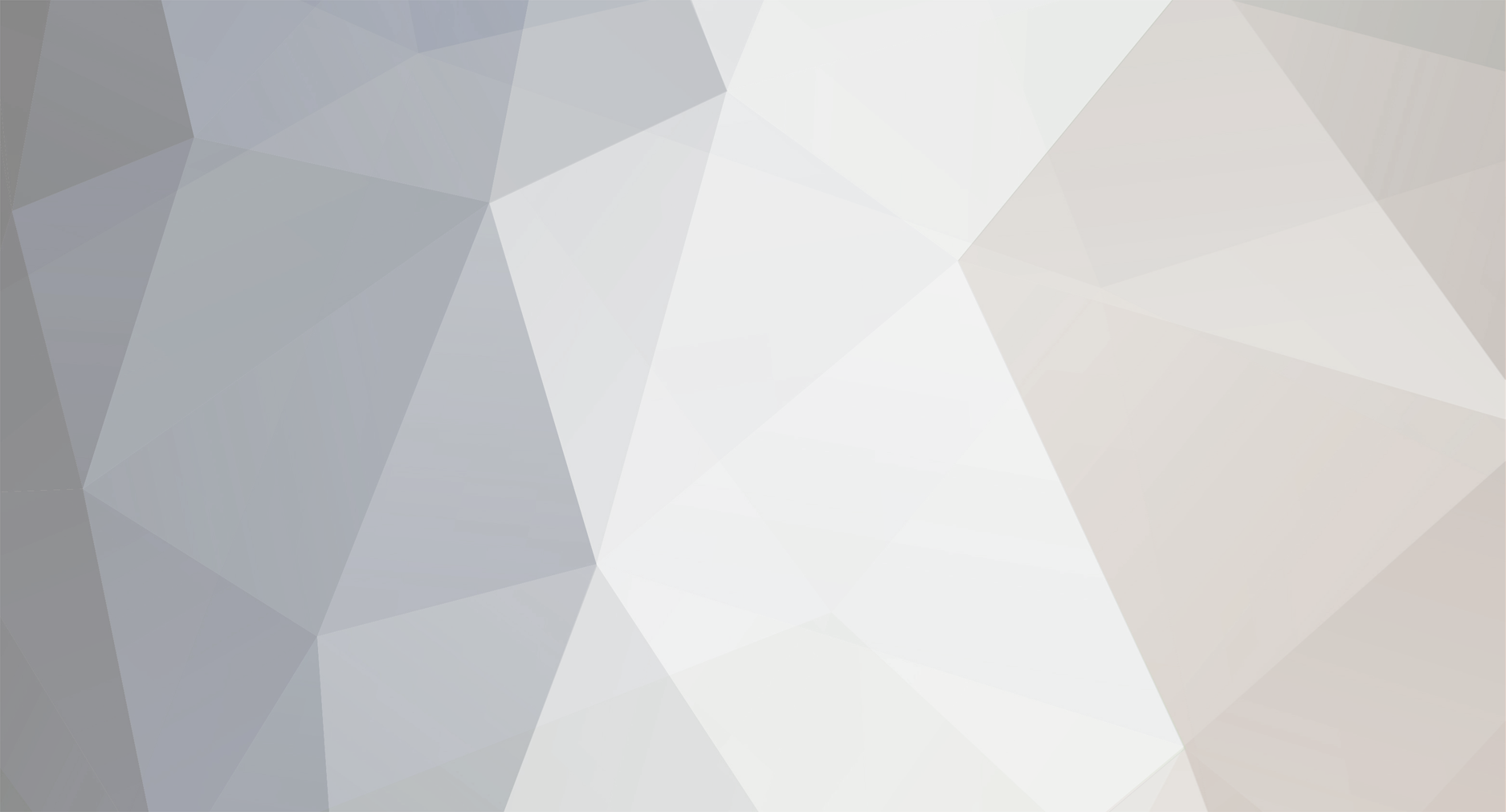
Eiolon
Members-
Posts
358 -
Joined
-
Last visited
Everything posted by Eiolon
-
I am trying to query for a code and want it to return a result only if it was used within the last 30 minutes. mysql> SELECT code, last_used FROM codes WHERE code = '234-246-248' AND DATE_SUB(CURDATE(),INTERVAL 30 MINUTE) <= last_used; +-------------+---------------------+ | code | last_used | +-------------+---------------------+ | 234-246-248 | 2009-03-13 06:46:05 | +-------------+---------------------+ 1 row in set (0.00 sec) It's been well over an hour since the code was last used but it is still returning a result. Any clues?
-
Is there any way I should be storing special characters in a database? For example, is there a problem putting this in: ñ from Jalapeño Also, symbols such as & Thanks!
-
[SOLVED] Data not updating even though it was successful
Eiolon replied to Eiolon's topic in PHP Coding Help
I found the problem. It was: <?php echo $_SERVER['PHP_SELF']; ?> in the form. I took that out and it works. -
[SOLVED] Data not updating even though it was successful
Eiolon replied to Eiolon's topic in PHP Coding Help
This is weird, I use or die(mysql_error()); and it still is saying: "Cannot retrieve user information" even though it's nowhere to be found in the page! Is it possible something could be cached? -
[SOLVED] Data not updating even though it was successful
Eiolon replied to Eiolon's topic in PHP Coding Help
Okay, I changed to what you recommended and still get the error: Cannot retrieve user information. I don't understand how I am getting the QUERY error when I am UPDATING. -
[SOLVED] Data not updating even though it was successful
Eiolon replied to Eiolon's topic in PHP Coding Help
What is the short way then? -
[SOLVED] Data not updating even though it was successful
Eiolon replied to Eiolon's topic in PHP Coding Help
Okay, here is what I have done. I have cut almost all the unneccessary code to make this work. I also limited it so only 1 field is being called up and updated. When I press the submit button, it gives me the error: Cannot retrieve user information. That is the error that is given if it cannot query the user information but it is clearly doing that. If it is not updating, then it should give this error: Could not edit the user. So here is the barebones code so if you can review and see why it's not saving the changes: <?php require_once('../mysql.php'); $query_users = "SELECT id, phone1 FROM users WHERE id = ".$_GET['id'].""; $users = mysql_query($query_users) OR die ('Cannot retrieve user information.'); $row_users = mysql_fetch_assoc($users); if (isset($_POST['submit'])) { $update = "UPDATE users SET phone1='".$_POST['phone1']."' WHERE id = ".$_GET['id'].""; $result = mysql_query($update) OR die ('Could not edit the user.'); } ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Edit Contact Information</title> </head> <body> <h2>Edit Contact Information</h2> <form id="edit" action="<?php echo $_SERVER['PHP_SELF']; ?>" method="post"> Phone Number: <td><input type="text" name="phone1" style="width:200px" maxlength="40" value="<?php echo $row_users['phone1']; ?>" /></td> <input type="submit" name="submit" value="Save Changes" /> </form> </body> </html> -
[SOLVED] Data not updating even though it was successful
Eiolon replied to Eiolon's topic in PHP Coding Help
I replaced the line and took out the header. Now when I update the info it gives me a blank page with no errors. -
[SOLVED] Data not updating even though it was successful
Eiolon replied to Eiolon's topic in PHP Coding Help
I am updating data. It is a user profile. When I pull up the info and make a change, it goes through but the data is not actually changed. The user should only be directed to user_edited.php if $update has been successfully. And since it did not DIE, then it should have updated the info. Instead, it is saying that it updated and redirecting, even though didn't actually change any of the data. -
I called up the record, which it did fine. When I make a change to any of the fields, it says its successful and directs me to the confirmation page instead of erroring out. However, the information is not saved. Any ideas? <?php session_start(); require_once('../mysql.php'); $id = (int) $_GET['id']; function escape_data ($data) { global $dbc; if (ini_get('magic_quotes_gpc')) { $data = stripslashes($data); } return mysql_real_escape_string (htmlspecialchars(trim(strip_tags($data))), $dbc); } $query_users = "SELECT username, first_name, last_name, phone1, phone2, email, admin FROM users WHERE id = '$id'"; $users = mysql_query($query_users) OR die ('Cannot retrieve a list of users.'); $row_users = mysql_fetch_assoc($users); if (isset($_POST['submit'])) { $errors = array(); if (empty($_POST['first_name'])) { $errors[] = 'You must enter a first name.'; } if ($_POST['first_name']) { $fn = escape_data($_POST['first_name']); } if (empty($_POST['last_name'])) { $errors[] = 'You must enter a last name.'; } if ($_POST['last_name']) { $ln = escape_data($_POST['last_name']); } if (empty($_POST['phone1'])) { $errors[] = 'You must enter a phone number.'; } if ($_POST['phone1']) { $p1 = escape_data($_POST['phone1']); } if ($_POST['phone2']) { $p2 = escape_data($_POST['phone2']); } if (empty($_POST['email'])) { $errors[] = 'You must enter an e-mail address.'; } if ($_POST['email']) { $e = escape_data($_POST['email']); } if ($_POST['admin']) { $a = escape_data($_POST['admin']); } if (empty($errors)) { $update = "UPDATE users SET first_name='$fn', last_name='$ln', phone1='$p1', phone2='$p2', email='$e', admin='$a' WHERE id = '$id'"; $result = mysql_query($update) OR die ('Could not update the user.'); if ($update) { header('Location: user_edited.php'); exit; } } } ?> <form id="edit_user" action="<?php echo $_SERVER['PHP_SELF']; ?>" method="post"> <table border="0" cellpadding="2" cellspacing="2" width="100%"> <tr> <td width="125">First Name:</td> <td><input type="text" name="first_name" style="width:200px" maxlength="20" value="<?php echo $row_users['first_name']; ?>" /></td> </tr> <tr> <td>Last Name:</td> <td><input type="text" name="last_name" style="width:200px" maxlength="20" value="<?php echo $row_users['last_name']; ?>" /></td> </tr> <tr> <td>Phone:</td> <td><input type="text" name="phone1" style="width:200px" maxlength="20" value="<?php echo $row_users['phone1']; ?>" /></td> </tr> <tr> <td>Additional Phone:</td> <td><input type="text" name="phone2" style="width:200px" maxlength="20" value="<?php echo $row_users['phone2']; ?>" /> (optional)</td> </tr> <tr> <td>E-mail:</td> <td><input type="text" name="email" style="width:200px" maxlength="20" value="<?php echo $row_users['email']; ?>" /></td> </tr> </table> <p><input <?php if (!(strcmp($row_users['admin'],1))) {echo "checked=\"checked\"";} ?> name="admin" type="checkbox" id="admin" value="1" /> Check to make this user an administrator.</p> <input type="submit" name="submit" value="Save Changes" /> </form>
-
This appears to have fixed it. Thanks.
-
I have my username and password boxes set as the same size. I recently installed updates to IE7 and afterwards the password box appears shorter than the username box. In Firefox 3 it appears the same length. Any ideas? <input type="text" name="username" size="25" maxlength="20" /> <input type="password" name="password" size="25" maxlength="40" autocomplete="off" />
-
Any ideas on how to turn off auto complete in a compliant manner? Last time I checked the above was not compliant.
-
Thanks
-
http://www.intravising.com/test/login.php http://www.intravising.com/test/admin/index.php is the secure page I just have a login page and a secure page up. Nothing fancy, I'm just looking for holes in the php script. Working account if needed: username: test password: qaz123 thanks!
-
I have a header file that will be included on each page. Will I have problems if I put all the session information in the header file instead of each individual page? I am trying to not have to query user permissions in each page. I figured I would query for the user and its permissions in the header file and on the individual pages check to see if the user has permission to be on it. Also, would that mean I still need to declare session_start(); at the top of each page or just the header include file? Thanks.
-
I have a global settings section where they can control the site name (which is shown on the title bar and footer) and URL as to where the app is installed. This way they can change things on the fly. I am wondering if there is a better way to do it. Currently, I have a query on each page that calls for the settings and I am not sure if this is effecient to do. Example: $query_global_settings = "SELECT * FROM global_settings"; $global_settings = mysql_query($query_global_settings) OR die ('Cannot retrieve global settings.'); $row_global_settings = mysql_fetch_assoc($global_settings); <title><?php echo $row_global_settings['site_name']; ?> - User Management</title> Any suggestions on how to approach this or is what I am doing okay?
-
There is a lot to consider for security, MySQL is only a small portion of your concern. It doesn't matter if you make MySQL itself unhackable, you still have to consider PHP security, Apache or IIS security, operating system security and then the front-end code security. All these things interact with MySQL and if someone finds an exploit in just one of those they can make MySQL think it's being legitamently accessed. My advice is, if you don't want to put in the time to learn all of that, and since you are dealing with sensitive information, you should hire a professional.
-
[SOLVED] Dynamic drop down menu but with an item always listed first
Eiolon replied to Eiolon's topic in PHP Coding Help
Wow, that was easy. Works fine. Thanks! -
I have a dynamic drop down menu that is pull categories from a database. I have everything listed in alphabetical order however, I want one item to always be listed as the first thing in the box even though its not at the beginning of the alphabet. $query_categories = "SELECT * FROM categories ORDER BY category ASC"; $categories = mysql_query($query_categories) OR die ('Cannot retrieve a list of categories.'); <select name="category" id="category" style="width:250px"> <?php while($row_categories = mysql_fetch_array($categories)) { print '<option value="' . $row_categories['category_id'] . '">' . $row_categories['category'] . '</option>'; } ?> </select> That's the code I have now. Not sure what to do about the moving of the item to the top. Thanks for your help!
-
Try to use the source command rather than the restore command.
-
I would approach this by combining the USERS and PROFILES tables (unless there is some reason why you are having them separated). TABLE 1 = Users ==================== user_id username password firstName lastName TABLE 2 = Friends ==================== user_id friend_id The next thing to note in your query is to not query by someone's name. You can have multiple "Joe's" in your database. Exactly which "Joe" are you trying to query? Query by the user_id instead. SELECT users.user_id, firstName, lastName FROM users JOIN friends ON users.user_id = friends.friend_id WHERE friends.user_id = 1;
-
I have a page that generates a list of names from a database. There is a list of 300+ names so I am trying to make it easier for people to see if a name is on the list. Currently, they press CTRL+F (or Edit > Find) and they type the name in to see if it's on there. I'd like to embed a box that is similiar on the page so they do not need to press CTRL+F to bring the prompt up. The other nice thing is it highlights the names as it finds them which would be nice. Is there a javascript solution for this? I know it could be done in PHP by making a search box and query the database but I was trying to avoid that.
-
I want to the most recent entries in a table but I want to have a cutoff date of 2 weeks. Entries that are more than 2 weeks old won't be listed. All entries are stored with a mysql datetime column: record_added datetime not null default '0000-00-00 00:00:00' Any idea on how I could do this? Thanks!
-
I have a script that queries a database of barcodes. The barcodes always start with 29971 and have an additional 9 numbers after it. I need a way to make sure anything that does not start with 29971 gets rejected. Any ideas?