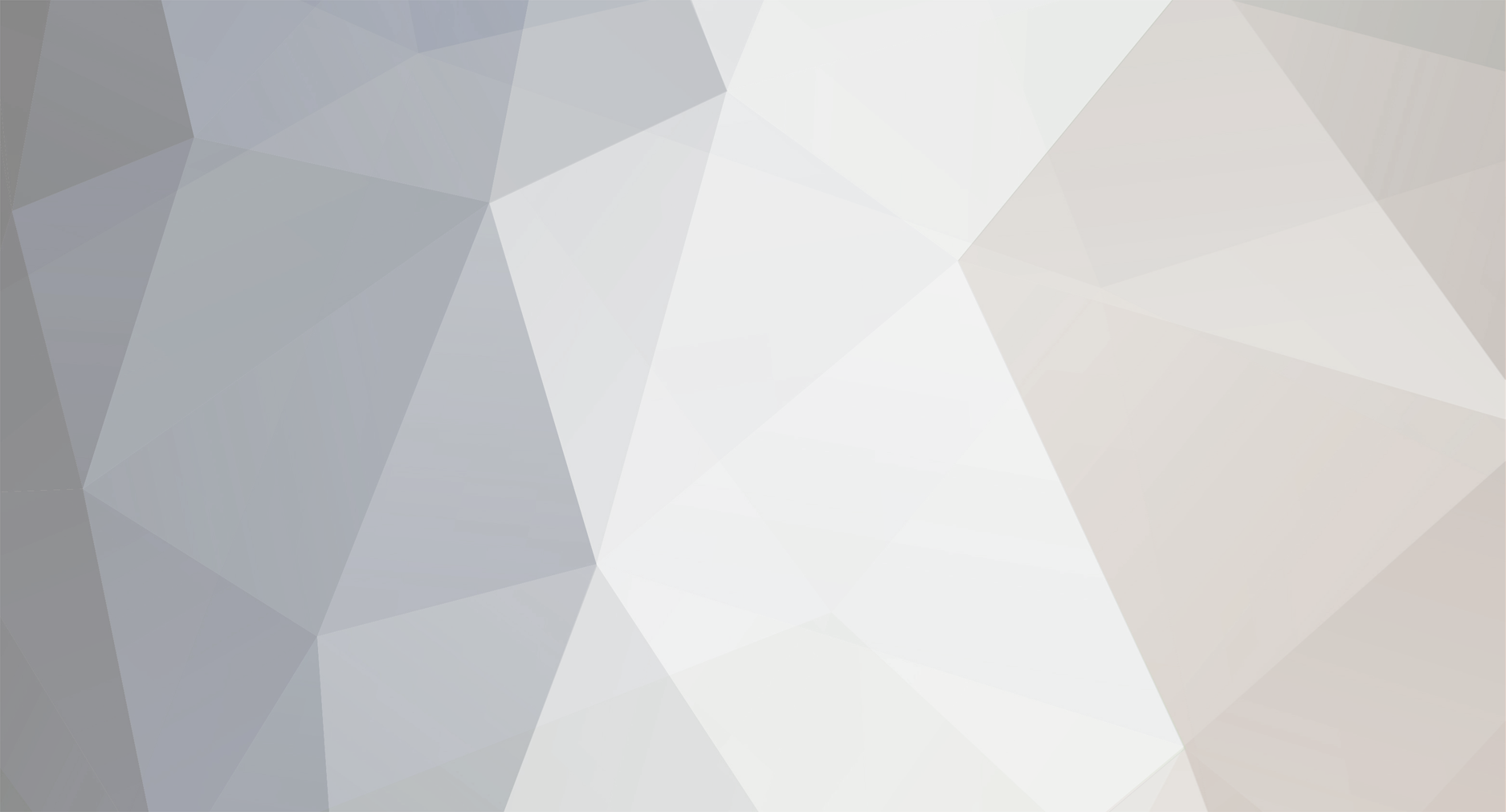
Eiolon
Members-
Posts
358 -
Joined
-
Last visited
Everything posted by Eiolon
-
Nevermind, I am a retard. Forgot to insert a column in the actual database.
-
Nevermind, found a solution
-
Thank you!
-
I have a field that asks for a number. Any number is being accepted except for zero. It will accept double zeros but not a single zero. Here is my validation: if (empty($_POST['seats'])) { $errors[] = 'Enter the number of seats available'; } if (!ctype_digit($_POST['seats'])) { $errors[] = 'The number of seats must be a digit'; } if ($_POST['seats']) { $s = escape_data($_POST['seats']); } My escape data function function escape_data ($data) { global $dbc; if (ini_get('magic_quotes_gpc')) { $data = stripslashes($data); } return mysql_real_escape_string (htmlspecialchars(trim(strip_tags($data))), $dbc); }
-
I have a page with a series of check boxes. If one in particular is checked, it will disable the other ones. There are other fields on the page. When the fields are validated and an error occurs, the check box remains checked but it does not keep the other ones disabled. Here is what I am using for my code: <?php if ($_POST['no_registration_required'] == "1") { echo "<input name='no_registration_required' type='checkbox' id='no_registration_required' value='1' onclick='this.form.allow_online_registration.disabled = this.checked; this.form.ask_age.disabled = this.checked; this.form.ask_address.disabled = this.checked; this.form.ask_source.disabled = this.checked; this.form.fee_required.disabled = this.checked;' checked='checked' />"; } else { echo "<input name='no_registration_required' type='checkbox' id='no_registration_required' value='1' onclick='this.form.allow_online_registration.disabled = this.checked; this.form.ask_age.disabled = this.checked; this.form.ask_address.disabled = this.checked; this.form.ask_source.disabled = this.checked; this.form.fee_required.disabled = this.checked;' />"; } ?>
-
In IE, if I disable a radio button or check box it turns grey. If I disable a text field or text area, it stays white but the content turns grey. If there is no text in the box, the user does not see the box become disabled. Is there a way in CSS to change the field color when they select something that would disable a field?
-
I have use a select menu for people to choose a date. When it is inserted into the database, it is stored in DATE format (ex. 2009-12-31). I accomplished it by doing: $month = $_POST['month']; $day = $_POST['day']; $year = $_POST['year']; $dp = $year.'-'.$month.'-'.$day; Are there any tutorials on how to make it so when a user edits the record they can once again use a select menu to change the date? I don't know how to separate them back out and call them back up. Thanks!
-
Oh, thanks very much Working!
-
Thanks, but the value is still not being passed to company.php. In the address bar it is showing: http://localhost/contacts/company.php?id=$id Instead of: http://localhost/contacts/company.php?id=1
-
I am trying to make it so when a record is created the user will be redirected to that record once submitted. It is redirecting to the proper page but it is not pulling up the record. I'm not sure how to get the $id to pass through using the header('Location') redirect. if (empty($errors)) { $insert = "INSERT INTO companies (name, phone1, phone2, fax, website, customer, contract) VALUES ('$n','$p1','$p2','$f','$w','$cu','$co')"; $result = mysql_query($insert) OR die ('Could not add the company to the database.'); $id = mysql_result((mysql_query("SELECT * FROM companies WHERE id = '$id'")), 0, 'id'); if ($insert) { header('Location: company.php?id=".$id."'); exit; } }
-
Just create a permissions table and have one of the options be if they are allowed to view other peoples tickets, it be a 1 if not, then a 0. Go into the ticket.php file and throw in some php to validate if they have permission or not based on the permissions table.
-
I am creating an app that will have statistics generated for users and their activity. I know of at least two ways to get the statistics (if there are other more practical methods please tell) and am looking for advice for the best one to use. Method 1: Query the database using joins between the tables for the info I need Method 2: When inserting records into the tables, I would also insert into a statistics table the info that I would be calling up Thanks!
-
I agree that it's a bit dull. It could be used for the presenter as a note card but it does nothing different than a tutorial in a book does. In an era where everything is YouTube'd and zip zooming in your face with sound and graphics, it doesn't really do anything to keep attention.
-
Yeah, the easiest way to do this is to insert the user into the phpBB users table and then call on that table when logging into the game.
-
I have an application for event registration. Users log into their account at a website so they can register for an event. It also keeps track of events they previously attended and other records. Now, I want to make it so I can register people for the event but without needing to create a user account. Call it a "one-time registration". The users would not be able to log into the website to check for reminders, etc. Any suggestions on how I can design the app for one-time registration?
-
if (isset($_POST['go'])) { $insert = "INSERT INTO user (username, password, email) VALUES ('$username',MD5('$password'),'$email')"; $result = mysql_query($insert) OR die ('Could not add the user to the database.'); } I reworked it a little. I test this on my own system and it works.
-
$query = mysql_query("INSERT INTO user (username, password, email) VALUES ('$username',MD5('$password'),'$email')"; Your query was part INSERT and part UPDATE. Also, you need to escape data.
-
Encryption and hashing are different things. I don't see you doing either of those in that script. Perhaps its in the register.php that is in your form action?
-
What you are asking to do is very basic, it's just a matter of putting all the pieces together. You will need to learn some basic database design, how PHP handles forms with POST and GET and sessions. Once you have learned those pieces, you can put it together and make what you'd like. I suggest reading PHP and MySQL for Dynamic Websites by Larry Ullman. He gives you scenarios with visual examples to follow along by. This is how I started to learn PHP. Once I have started to understand the basics of PHP from the book's help, I was able to understand the terminology of the PHP manual much easier.
-
All I want to do is have the results shown on the same page as the search box. I don't want it to be directed to a second page that would have the results. As it stands, if I run the query nothing is displayed. I can get it to work if I leave out: if (isset($_GET['submit'])) { } but I need it to error out of a row is not returned and I don't want the error to always be shown until a correct query has been executed. <?php define ('DB_USER', 'root'); define ('DB_PASS', ''); define ('DB_HOST', 'localhost'); define ('DB_NAME', 'trailers'); $dbc = mysql_connect (DB_HOST, DB_USER, DB_PASS) OR die ('Cannot connect to MySQL server.'); mysql_select_db (DB_NAME) OR die ('Cannot connect to database.'); $barcode = $_GET['barcode']; if (isset($_GET['submit'])) { $query_trailer = "SELECT * FROM trailers WHERE barcode = '$barcode'"; $trailer = mysql_query($query_trailer) OR die ('Cannot retrieve trailer information.'); $row_trailer = mysql_fetch_assoc($trailer); $total = mysql_num_rows($trailer); if ($total == 0) { echo 'Nothing found'; } } ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <script> <!-- function focus(){document.search.barcode.focus();} // --> </script> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <meta http-equiv="refresh" content="210;url=http://library/trailers" /> <title>Trailer</title> </head> <body onLoad=focus()> <p><?php echo $row_trailer['title']; ?> (<?php echo $row_trailer['year']; ?>)</p> <object width="<?php echo $row_trailer['width']; ?>" height="<?php echo $row_trailer['height']; ?>" classid="clsid:02BF25D5-8C17-4B23-BC80-D3488ABDDC6B" codebase="http://www.apple.com/qtactivex/qtplugin.cab"> <param name="src" value="http://library/trailers/<?php echo $row_trailer['filename']; ?>.mov"> <param name="autoplay" value="true"> <param name="controller" value="false"> <embed src="<?php echo $row_trailer['filename']; ?>.mov" width="<?php echo $row_trailer['width']; ?>" height="<?php echo $row_trailer['height']; ?>" autoplay="true" controller="false" pluginspage="http://www.apple.com/quicktime/download/"> </embed> </object> <form id="search" name="search" method="get" action="<?php echo $_SERVER['PHP_SELF']; ?>"> <p style="font-size: 30px; font-family: arial; font-weight: bold;">SCAN DVD BARCODE</p> <input name="barcode" type="text" id="barcode" style="font-size: 30px; height:30px; width: 400px;"/> <input type="submit" name="submit" id="submit" value="Search" /> </form> </body> </html>
-
I noticed when viewing websites in Vista the style of the text fields and buttons are different compared to viewing them on XP. Is there any way to make it so people viewing with XP see the same as Vista users?
-
Thanks for explaining that to me. I will work on better validation. Howver, it is interesting that the description field was not susceptible to any of the attacks.
-
Out of 14605 tests, it failed 15: Server Status Code: 302 Moved Temporarily Tested value: %31%27%20%4F%52%20%27%31%27%3D%27%31 Server Status Code: 302 Moved Temporarily Tested value: 1 UNI/**/ON SELECT ALL FROM WHERE Server Status Code: 302 Moved Temporarily Tested value: 1 UNION ALL SELECT 1,2,3,4,5,6,name FROM sysObjects WHERE xtype = 'U' -- Server Status Code: 302 Moved Temporarily Tested value: 1 AND ASCII(LOWER(SUBSTRING((SELECT TOP 1 name FROM sysobjects WHERE xtype='U'), 1, 1))) > 116 Server Status Code: 302 Moved Temporarily Tested value: ' OR username IS NOT NULL OR username = ' Server Status Code: 302 Moved Temporarily Tested value: 1' AND non_existant_table = '1 Server Status Code: 302 Moved Temporarily Tested value: 1'1 Server Status Code: 302 Moved Temporarily Tested value: '; DESC users; -- Server Status Code: 302 Moved Temporarily Tested value: 1 AND USER_NAME() = 'dbo' Server Status Code: 302 Moved Temporarily Tested value: 1' AND 1=(SELECT COUNT(*) FROM tablenames); -- Server Status Code: 302 Moved Temporarily Tested value: 1 AND 1=1 Server Status Code: 302 Moved Temporarily Tested value: 1 EXEC XP_ Server Status Code: 302 Moved Temporarily Tested value: 1'1 Server Status Code: 302 Moved Temporarily Tested value: 1' OR '1'='1 Server Status Code: 302 Moved Temporarily Tested value: 1 OR 1=1
-
I was running my project through SQL Inject Me and stumbled upon a page that was vulnerable. It only has 2 fields, and interestingly, only 1 of the fields is vulnerable even through they are the exact same type of field and both are being escaped the same way. It is the NAME field that is giving me problems, the DESCRIPTION field seems to be okay. Any ideas? <?php session_start(); function escape_data ($data) { global $dbc; if (ini_get('magic_quotes_gpc')) { $data = stripslashes($data); } return mysql_real_escape_string (htmlspecialchars(trim(strip_tags($data))), $dbc); } require_once('../includes/mysql.php'); if (isset($_POST['submit'])) { $errors = array(); if (empty($_POST['name'])) { $errors[] = 'Group name must be entered'; } if ($_POST['name']) { $n = escape_data($_POST['name']); } if ($_POST['description']) { $d = escape_data($_POST['description']); } if (empty($errors)) { $insert = "INSERT INTO groups (name, description) VALUES ('$n','$d')"; $result = mysql_query($insert) OR die ('Could not add the group to the database.'); if ($insert) { header('Location: group_added.php'); exit; } } } ?>
-
It seems to be working, though the color scheme throughout the site is enough to make me want to put a fork in my eyes. Can you do anything once the character is created?