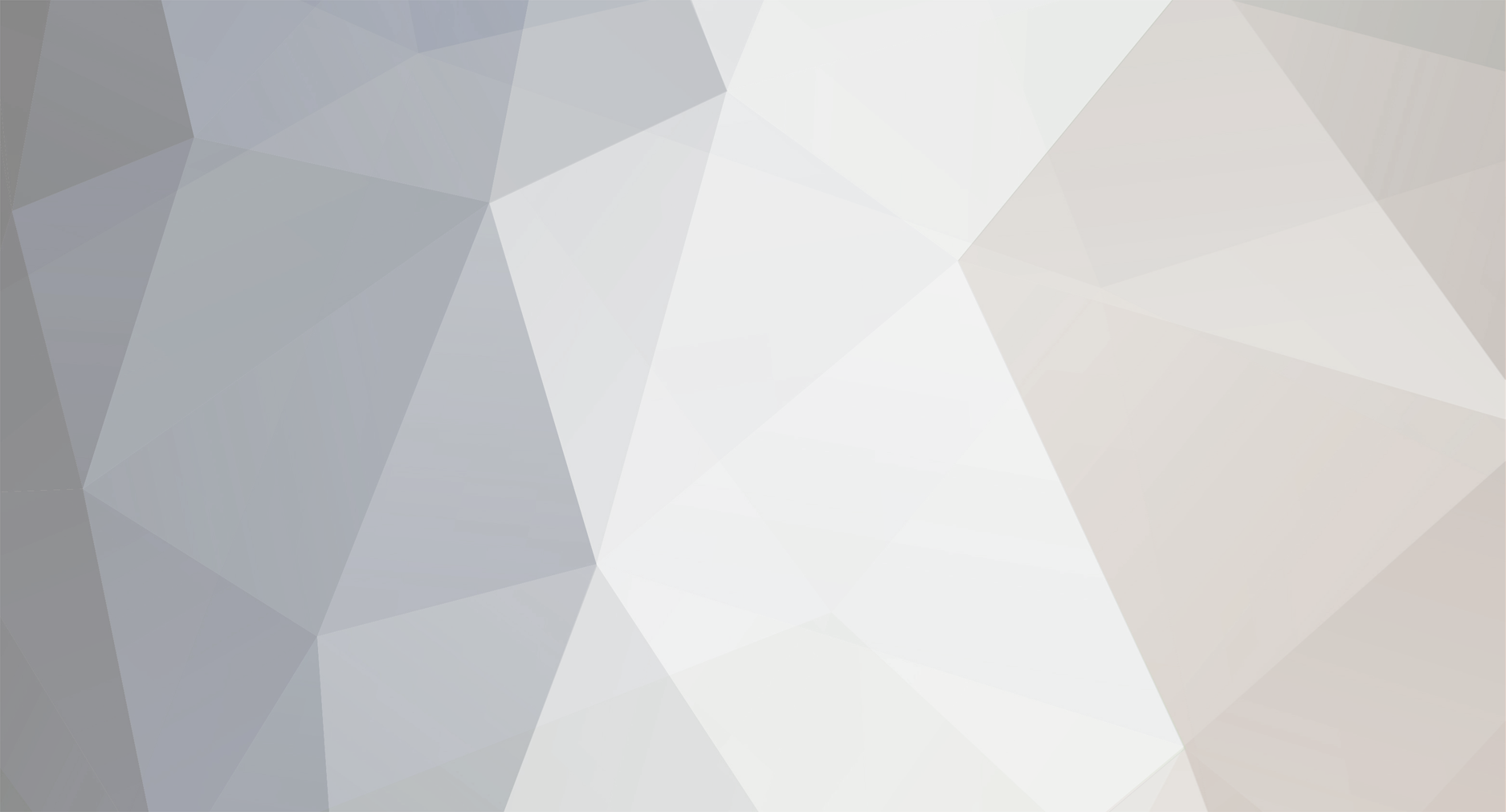
Eiolon
-
Posts
358 -
Joined
-
Last visited
Posts posted by Eiolon
-
-
Any data submitted through POST or GET should be considered possibly malicious and must be properly validated and sanitized. Just as a user can enter data on the URL they may also create their own forms to post data.
Yes, this is what I was referring to. Is there any information on how to "validate and sanitize" the data?
-
So wait, you are saying I should use POST to RETRIEVE data? How would I delete a record by using POST? I thought I need to call the record id in order to delete it, hence I used GET.
-
Are there any tutorials on this? Basically, I want to make it so people can't type in the URL to delete a record and whatnot.
Also, what are your thoughts on using $_SESSION instead of $_GET to navigate records? For example, use $_GET to set the session and use the session thereafter to do the queries.
-
I have an error message that appears if the username or password field has not been filled out. The problem is it always appears in the upper left hand corner of the screen. How would I make it so I can control where in the layout the message appears?
<?php // Verify that the username has been entered. if (empty($_POST['username'])) { $u = FALSE; echo 'You must enter a username.<br />'; } else { $u = escape_data($_POST['username']); } // Verify that the password has been entered. if (empty($_POST['password'])) { $p = FALSE; echo 'You must enter a password.<br />'; } else { $p = escape_data($_POST['password']); } ?>
Thanks!
-
Take a look here for changing the auto_increment:
-
Sorry, I am a novice. When you mean variable interpolation, what do you mean? Like this?
// Query the database for topic and reply information. $query_topics = "SELECT t.*, r.*, DATE_FORMAT(t.topic_last_reply, '%c/%e/%Y %l:%i %p') AS convdate FROM topics t LEFT JOIN replies r ON (t.topic_id = r.reply_topic) WHERE t.topic_forum = ".$_GET['forum_id']." GROUP BY t.topic_subject ORDER BY t.topic_last_reply DESC"; $topics = mysql_query($query_topics) OR die ('Cannot retrieve a list of topics.'); $row_topics = mysql_fetch_array($topics);
-
I made a forum and it works for the most part. The problem I have is when I query for the list of topics in the forum, anything with 0 replies does not get shown. Once I have a reply to that topic it gets shown. Here is the query:
SELECT t.*, r.*, DATE_FORMAT(t.topic_last_reply, '%c/%e/%Y %l:%i %p') AS convdate FROM topics t LEFT JOIN replies r ON (t.topic_id = r.reply_topic) WHERE t.topic_forum = ".$_GET['forum_id']." GROUP BY t.topic_subject HAVING t.topic_num_replies >= 0 ORDER BY t.topic_last_reply DESC
Thanks!
-
Hello,
I am making a column called "Last Post" much like the one used on this forum. It will display the date, time and user of the lastest reply to a thread. The problem I am having is it's querying the very first reply.
Query:
// Query the database for topic and reply information. $query_topics = "SELECT t.*, r.* FROM topics t JOIN replies r ON (t.topic_id = r.reply_topic) WHERE topic_forum = ".$_GET['forum_id']." GROUP BY t.topic_subject ORDER BY t.topic_date DESC"; $topics = mysql_query($query_topics) OR die ('Cannot retrieve a list of topics.'); $row_topics = mysql_fetch_array($topics);
PHP:
<table width="100%" border="0" cellspacing="1" cellpadding="6" bgcolor="#CCCCCC"> <tr bgcolor="#E5E5E5"> <td><strong>Topic</strong></td> <td width="75"><div align="center"><strong>Replies</strong></div></td> <td width="250"><div align="center"><strong>Author</strong></div></td> <td width="250"><div align="center"><strong>Last Post</strong></div></td> </tr> <?php do { ?> <tr bgcolor="#FFFFFF"> <td><a href="topic.php?topic_id=<?php echo $row_topics['topic_id'] ?>"><?php echo $row_topics['topic_subject'] ?></a> </td> <td width="75"><div align="center"><?php echo $row_topics['topic_num_replies'] ?></div></td> <td width="250"><div align="center"><?php echo $row_topics['topic_author'] ?></div></td> <td width="250"><div align="center"><?php echo $row_topics['reply_date'] ?> by <?php echo $row_topics['reply_author'] ?></div></td> </tr> <?php } while ($row_topics = mysql_fetch_array($topics)); ?> </table>
Many thanks!
-
Thanks very much
-
When I created a table I accidentally forgot to make the id a primary key and auto_increment. Can I go back and make it this way or do I need to recreate the table?
WANTED
reply_id int not null primary key auto_increment
ENDED UP WITH
reply_id int not null default '0'
Thanks!
-
Are you looking for a wysiwyg editor?
http://www.hotscripts.com/PHP/Scripts_and_Programs/WYSIWYG_Editors/index.html
Also, you can reverse engineer code from free forum software like phpbb and punbb.
-
Thanks very much! Works like a charm!
-
Correct, it's actually just like this forum. On the main page you see the name, desc, topics created in the forum and total posts in the forum.
-
The above query does get rid of the duplicate forum. The problem that occurs with removing the topic_num_replies is I am doing math in the do-while statement to give me the total posts. It adds forum_num_topics + topic_num_replies to get the total posts.
So if I were to add another column, forum_num_posts, how would I modify my above insert query for adding a reply? Currently I have it updating the topics table upon a successful insert. Is it possible to update two tables upon successful insert?
Thanks again for all your help on this!
-
Let me ask you this, would it be better to make a new column in the forums table called "forum_num_posts" and whenever a new topic/reply is made update that column? It would stop me from having to do a join but then again I am not sure if that is the best choice or not since I am new to this stuff.
-
Looks to be the same output.
mysql> SELECT DISTINCT f.forum_id, f.forum_name, f.forum_desc, f.forum_num_topics, t.topic_num_replies, t.topic_forum FROM forums f JOIN topics t ON (f.forum_id = t.topic_forum); +----------+--------------------+---------------------------------------------------+------------------+-------------------+-------------+ | forum_id | forum_name | forum_desc | forum_num_topics | topic_num_replies | topic_forum | +----------+--------------------+---------------------------------------------------+------------------+-------------------+-------------+ | 5 | The Lounge | Chat about anything not related to the library. | 2 | 11 | 5 | | 3 | General Discussion | Talk about general library business, issues, etc. | 1 | 1 | 3 | | 4 | Library Expansion | Discuss the library expansion project. | 1 | 1 | 4 | | 5 | The Lounge | Chat about anything not related to the library. | 2 | 0 | 5 | +----------+--------------------+---------------------------------------------------+------------------+-------------------+-------------+ 4 rows in set (0.00 sec)
-
Certainly, here you go:
mysql> SELECT topic_subject,topic_forum from topics; +-----------------------------+-------------+ | topic_subject | topic_forum | +-----------------------------+-------------+ | Welcome to the forums! | 5 | | use of others library cards | 3 | | The new room | 4 | | South Wing | 5 | +-----------------------------+-------------+ 4 rows in set (0.00 sec)
mysql> SELECT forum_id,forum_desc,forum_num_topics from forums; +----------+---------------------------------------------------+------------------+ | forum_id | forum_desc | forum_num_topics | +----------+---------------------------------------------------+------------------+ | 3 | Talk about general library business, issues, etc. | 1 | | 4 | Discuss the library expansion project. | 1 | | 5 | Chat about anything not related to the library. | 2 | +----------+---------------------------------------------------+------------------+ 3 rows in set (0.00 sec)
-
No luck, same result :'(
-
Insert query for adding a topic:
$insert = "INSERT INTO topics (topic_subject, topic_author, topic_body, topic_date, topic_forum) VALUES ('".$_POST['topic_subject']."','".$_POST['topic_author']."','".$_POST['topic_body']."',now(),'".$_GET['forum_id']."')"; $result = mysql_query($insert) OR die ('Could not add topic to forum.'); if ($insert) { $update = "UPDATE forums SET forum_num_topics = '$topics', forum_num_posts = '$posts' WHERE forum_id = ".$_GET['forum_id'].""; $update_result = mysql_query($update) OR die ('Could not add to topic total.'); header('Location: topic_added.php'); exit; }
Insert query for adding a reply:
$insert = "INSERT INTO replies (reply_author, reply_body, reply_date, reply_topic) VALUES ('".$_POST['reply_author']."','".$_POST['reply_body']."',now(),'".$_GET['topic_id']."')"; $result = mysql_query($insert) OR die ('Could not add reply to topic.'); if ($insert) { $update = "UPDATE topics SET topic_num_replies = '$replies' WHERE topic_id = ".$_GET['topic_id'].""; $update_result = mysql_query($update) OR die ('Could not add to reply total.'); header('Location: reply_added.php'); exit; }
I just want to be clear that it's not actually inserting the duplicate forums into the database. It appears that it's the join making the output duplicate, but I have tried every join that I could find and still no resolution.
-
I created a small forum which seems to be working with one exception. Whenever more than 1 topic is created in a forum it duplicates the forum on the main page and also gives the duplicate the wrong post count.
Query:
$query_both = "SELECT f.forum_id, f.forum_name, f.forum_desc, f.forum_num_topics, t.topic_num_replies, t.topic_forum FROM forums f JOIN topics t ON (f.forum_id = t.topic_forum)"; $both = mysql_query($query_both) OR die ('Cannot retrieve forum information.'); $row_both = mysql_fetch_array($both);
Table:
<table width="100%" border="0" cellspacing="1" cellpadding="6" bgcolor="#CCCCCC"> <tr bgcolor="#E5E5E5"> <td><strong>Forum</strong></td> <td width="75"><div align="center"><strong>Topics</strong></div></td> <td width="75"><div align="center"><strong>Posts</strong></div></td> </tr> <?php do { $number1 = $row_both['forum_num_topics']; $number2 = $row_both['topic_num_replies']; $posts = $number1 + $number2; ?> <tr bgcolor="#FFFFFF"> <td><a href="forum.php?forum_id=<?php echo $row_both['forum_id'] ?>"><?php echo $row_both['forum_name'] ?></a> - <?php echo $row_both['forum_desc'] ?></td> <td width="75"><div align="center"><?php echo $row_both['forum_num_topics'] ?></div></td> <td width="75"><div align="center"><?php echo $posts ?></div></td> </tr> <?php } while ($row_both = mysql_fetch_array($both)); ?> </table>
Result after more than 1 topic is created in the forum (duplicate in red square):
Thanks for your help!
-
EDIT: NVM solved. Thanks to all!
-
Hmmm... this is the result I am getting:
37 years 461 months
The date is 1998-09-01.
<?php $started = strtotime($row_employee['hire_date']); $now = time(); $dif = $now-$started; echo floor($dif/(60*60*24*365))." years ".floor($dif/(60*60*24*30))." months"; ?>
-
I have each employees hire date stored in date format in MySQL (YYYY-MM-DD) and would like to calculate how many years and months of service they have. The output would be like:
Years of Service: 7 yr and 2 mo
How would I go about doing this calculation?
Thanks!
-
Ah, my mistake. Thanks!
Query all columns except one
in MySQL Help
Posted
I'd like to query all columns in my users table except the password column. Is there an easy way to do this without having to type out all of the fields and excluding the password column?