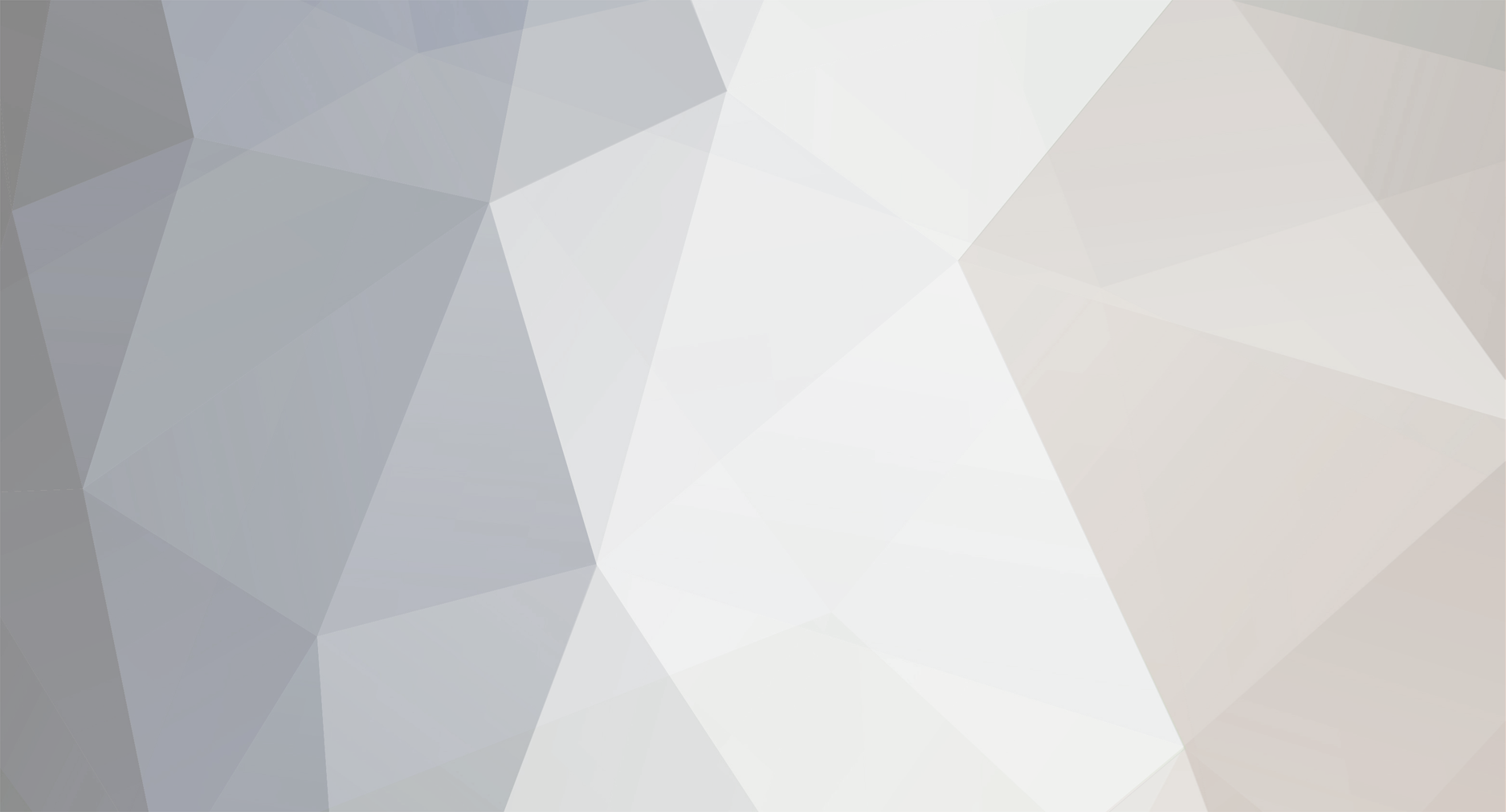
Eiolon
-
Posts
358 -
Joined
-
Last visited
Posts posted by Eiolon
-
-
So I am creating a list of events. There will be a drop down menu to change between months. I only want the months to be listed if an event during that month exists.
So if I have events listed for September and November, list only September and November, skipping October. Any idea on how to approach this or if there are any functions for such a thing?
-
Ha ha! Oh my I didn't even notice that. Thanks very much!
-
I am getting this error:
Warning: mysql_fetch_assoc() expects parameter 1 to be resource, array given in C:\wamp\www\purchase.php on line 52Line 52 in question:
<?php } while ($items = mysql_fetch_assoc($items)); ?>
My query:
$query_items = "SELECT id, qty, description, price, total FROM items WHERE purchase_id = 8"; $items = mysql_query($query_items) OR die ('Cannot retrieve a list of line items.'); $row_items = mysql_fetch_assoc($items);
<table class="data_grid"> <tr> <th width="50">QTY</th> <th>DESCRIPTION</th> <th width="125">PRICE</th> <th width="125">TOTAL</th> <th width="50"></th> </tr> <?php do { ?> <tr> <td width="50"><div align="center"><?php echo $row_items['qty']; ?></div></td> <td><?php echo $row_items['description']; ?></td> <td width="125"><div align="center">$<?php echo $row_items['price']; ?></div></td> <td width="125"><div align="center">$<?php echo $row_items['total']; ?></div></td> <td width="50"><div align="center"><img src="images/delete.png" alt="Delete Line"/> <img src="images/edit.png" alt="Edit Line"/></div></td> </tr> <?php } while ($items = mysql_fetch_assoc($items)); ?>
I have 4 entries for the purchase_id. It is displaying the first row twice, and the other 3 arent shown.
-
I've been kind of overwhelmed with Javascript or AJAX. For some reason it doesn't sink in to me like PHP does. I decided to use PHP for some client-side functionality and I am wondering if it's even a good idea to do so in the first place.
For example, if I want a DIV shown, instead of Javascript, I use a POST to show the div. I will set the variable:
$showDIV = '0'
for when the page loads
Then when the link is clicked to show the div, the page will POST and change $showDiv to equal 1. If showDIV equals 1 then show the div.
I am just looking for input if I should continue down this path or not.
Thanks!
-
I have a form that is displayed through an echo.
Since it is already inside PHP tags, I am trying to figure out how to make the value of the form field echo:
<?php if (isset($_POST['category'])) echo $_POST['category']; ?>
so if there is an error it does not lose the value submitted.
Here is my form:
<?php if ($show_form == 1) { echo ' <form id="add_category" name="add_category" method="post" action="" class="form"> <input type="text" id="category" name="category" style="width:400px" maxlength="100" title="Enter a new category name here" value="" /> <input type="submit" id="submit" name="submit" class="submit" value="Submit"/> </form> '; } ?>
-
In the background, I want to send the ID but I want the name displayed for the user.
-
I have this semi-working. I have the name being put in the TO field but I do not have the actual record ID of the name being sent to it.
My code:
<a href="#" onclick="window.opener.document.add.employee.value=window.opener.document.add.employee.value+'<?php echo $row_employees['name']; ?>; ';"><?php echo $row_employees['name']; ?></a>
If I change:
<?php echo $row_employees['name']; ?>
to
<?php echo $row_employees['id']; ?>
it simply sends the ID to the text box but not the name.
-
I am not sure what this is called so I can't find anything on Google about it.
But as an example, in some webmail systems, a user can click the TO link and a popup window with a list of their contacts will appear. They can then select the contacts via check box and then have it applied to the text field in the TO field on the parent window.
Any ideas on how to go about doing that?
-
I have a DIV that I split into two columns.
Left column has a fixed width of 500px.
Right column needs to expand 100% to fill the screen regardless of resolution.
What is happening is the right column is aligning itself to the right and there is tons of space in the middle.
If I tell the right column to be 100% it drops the column below the left column.
Attached is a screenshot of what is happening and what I am trying to do.
My code:
CSS
#wrapper { border: 1px solid #000000; } #left { width:500px; float:left; border: 1px solid #000000; } #right { float:right; border: 1px solid #000000; width: 100%; }
HTML
<div id="wrapper"> <div id="left"> <div class="program"> <label for="name">Name</label> <input class="textbox" type="text" name="name" id="name" maxlength="100" title="What is the name of the program?" value="" /> </div> <div class="program"> <label for="start">Date & Time</label> <input class="textbox" type="text" name="start" id="start" maxlength="100" title="What date and time does it occur?" value="" /> </div> <div class="program"> <label for="location">Location</label> <input class="textbox" type="text" name="location" id="location" maxlength="100" title="Where is the program being held?" value="" /> </div> <div class="program"> <label for="coordinator">Coordinator</label> <input class="textbox" type="text" name="coordinator" id="coordinator" maxlength="100" title="Who is creating this program?" value="" /> </div> <div class="program"> <label for="seats">Seats</label> <input class="textbox" type="text" name="seats" id="seats" maxlength="3" title="How many seats are available?" value="" /> </div> </div> <div id="right"> <div class="program"> <label for="name">Name</label> <input class="textbox" type="text" name="name" id="name" maxlength="100" title="What is the name of the program?" value="" /> </div> <div class="program"> <label for="start">Date & Time</label> <input class="textbox" type="text" name="start" id="start" maxlength="100" title="What date and time does it occur?" value="" /> </div> <div class="program"> <label for="start">Date & Time</label> <input class="textbox" type="text" name="start" id="start" maxlength="100" title="What date and time does it occur?" value="" /> </div> </div> </div>
[attachment deleted by admin]
-
Very nicely done. Makes me almost want to sell my account since it would be more anonymous, but I don't want to give up all my time spent since I've been playing since day one
-
I was wondering how to scale a textarea so that it fits the text in terms of rows. For example, if the textarea by default has 5 rows, and when a user types in 8 rows of text and saves it, it will increase the rows to 8 when the record is pulled back up for updating.
This isn't something that needs to be done in real-time. It only needs to scale when the record is retrieved. Thanks!
-
Nevermind, I got it working. Stupid \'s
-
No, that didn't work. =(
-
I am not sure if it is my PHP or Javascript that is causing this to not work. The first select gets populated successfully but the second doesn't get populated when something is selected from the first:
<?php require_once('../includes/support.php'); require_once('../includes/functions.php'); @$cat=$_GET['cat']; if(strlen($cat) > 0 and !is_numeric($cat)) { echo "Error"; exit; } $query_categories = mysql_query("SELECT DISTINCT id, category FROM categories ORDER BY category ASC"); if(isset($cat) and strlen($cat) > 0){ $query_equipment = mysql_query("SELECT DISTINCT name, description FROM equipment WHERE category_id=$cat"); } else { $query_equipment = mysql_query("SELECT DISTINCT name, description FROM equipment WHERE category_id=0"); } ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Support</title> <link href="../includes/style.css" rel="stylesheet" type="text/css"> <SCRIPT language=JavaScript> function reload(form) { var val=form.cat.options[form.cat.options.selectedIndex].value; self.location='index2.php?cat=' + val ; } </script> </head> <body> <?php include_once('../includes/menu.php'); ?> <div id="headers"> <img src="../images/support.png" class="icon" alt="Support" /><h1>Support</h1> </div> <div id="content"> <form method="post" name="add" action=""> <label>Category:</label> <select name="cat" id="cat" onchange=\"reload(this.form)\"> <option value=''>SELECT</option> <?php while($category = mysql_fetch_array($query_categories)) { if($category['id']==@$cat) { echo "<option selected value='$category[id]'>$category[category]</option>"."<br>"; } else { echo "<option value='$category[id]'>$category[category]</option>"; } } ?> </select> <label>Equipment:</label> <select name="equipment_id" id="equipment_id"> <option value=''>SELECT</option> <?php while($equipment = mysql_fetch_array($query_equipment)) { print '<option value="' . $equipment['name'] . '</option>';}?> </select> <input type="submit" value="Submit"> </form> </div> </body> </html>
Thanks for your assistance!
-
That gets information about the server. I believe the OP is asking for information about the remote computer,i.e. the users PC. To get that information, something has to executed on the PC and the information can be sent back to the PHP script via AJAX.
Ken
This is correct. I have seen various web based inventory products do this so I thought it could be pulled off with out executing something on each client. Thanks.
-
I am looking to make my inventory system remotely grab PC info on the network such as the processor, OS, RAM, etc. Can PHP do this or is there something else I would need to use?
-
It still do not get a record returned when doing that.
-
I need to be able to search by items that have symbols in them. When it is passed through the URL it looks like its not retaining its value. I can query within mySQL fine but not with my PHP script. For example, i need to be able to query this:
A 1.34/6:
My PHP code for it:
$callno = $_GET['call_number']; $query_call = "SELECT * FROM documents WHERE call_number = '$callno'"; $call = mysql_query($query_call) OR die ('Cannot retrieve record information.'); $row_call = mysql_fetch_assoc($call);
So when I submit the above call number it is passed through the URL as:
http://library/govdocs/call_number.php?callno=A+1.34%2F6%3A
Which is obviously not retaining its value. Any ideas?
-
I am able to retain the value a user has entered into a text box in the event of an error. How would I do this with a select menu?
Here is my select menu:
<select name="department_id" id="department_id" style="width:406px;"><option>SELECT</option> <?php while($row_departments = mysql_fetch_array($departments)) { print '<option value="' . $row_departments['id'] . '">' . $row_departments['name'] . '</option>';}?> </select>
I am not sure where to echo the selection at.
<?php if (isset($_POST[department_id])) echo $_POST[department_id]; ?>
-
I am working on a script to insert multiple rows into a table. The the front end, I have a drop down menu called "CODE". It shows for the first row but not for the other rows:
Screenshot: http://www.intravising.com/code.jpg
How do I make it so the CODE is displayed for each row?
Thanks!
Here is my PHP/HTML:
$query_codes = "SELECT id, code FROM codes ORDER BY code ASC"; $codes = mysql_query($query_codes) OR die ('Cannot retrieve a list of codes.'); <table border="0" cellpadding="2" cellspacing="2" width="100%"> <tr> <th width="50">CODE</th> <th width="100">CALL NUMBER</th> <th>DESCRIPTION</th> <th width="75">ACQUIRED</th> </tr> <tr> <td width="50"><div align="center"><select name="code_id[]"><?php while($row_codes = mysql_fetch_array($codes)) { print '<option value="' . $row_codes['id'] . '">' . $row_codes['code'] . '</option>';}?></select></div></td> <td width="100"><div align="center"><input name="call_number[]" type="text" style="width:100px" maxlength="50" /></div></td> <td><div align="center"><input name="description[]" type="text" style="width:500px" /></div></td> <td width="75"><div align="center"><input name="acquired[]" type="text" style="width:100px" maxlength="10" /></div></td> </tr> <tr> <td width="50"><div align="center"><select name="code_id[]"><?php while($row_codes = mysql_fetch_array($codes)) { print '<option value="' . $row_codes['id'] . '">' . $row_codes['code'] . '</option>';}?></select></div></td> <td width="100"><div align="center"><input name="call_number[]" type="text" style="width:100px" maxlength="50" /></div></td> <td><div align="center"><input name="description[]" type="text" style="width:500px" /></div></td> <td width="75"><div align="center"><input name="acquired[]" type="text" style="width:100px" maxlength="10" /></div></td> </tr> <tr> <td width="50"><div align="center"><select name="code_id[]"><?php while($row_codes = mysql_fetch_array($codes)) { print '<option value="' . $row_codes['id'] . '">' . $row_codes['code'] . '</option>';}?></select></div></td> <td width="100"><div align="center"><input name="call_number[]" type="text" style="width:100px" maxlength="50" /></div></td> <td><div align="center"><input name="description[]" type="text" style="width:500px" /></div></td> <td width="75"><div align="center"><input name="acquired[]" type="text" style="width:100px" maxlength="10" /></div></td> </tr> <tr> <td width="50"><div align="center"><select name="code_id[]"><?php while($row_codes = mysql_fetch_array($codes)) { print '<option value="' . $row_codes['id'] . '">' . $row_codes['code'] . '</option>';}?></select></div></td> <td width="100"><div align="center"><input name="call_number[]" type="text" style="width:100px" maxlength="50" /></div></td> <td><div align="center"><input name="description[]" type="text" style="width:500px" /></div></td> <td width="75"><div align="center"><input name="acquired[]" type="text" style="width:100px" maxlength="10" /></div></td> </tr> <tr> <td width="50"><div align="center"><select name="code_id[]"><?php while($row_codes = mysql_fetch_array($codes)) { print '<option value="' . $row_codes['id'] . '">' . $row_codes['code'] . '</option>';}?></select></div></td> <td width="100"><div align="center"><input name="call_number[]" type="text" style="width:100px" maxlength="50" /></div></td> <td><div align="center"><input name="description[]" type="text" style="width:500px" /></div></td> <td width="75"><div align="center"><input name="acquired[]" type="text" style="width:100px" maxlength="10" /></div></td> </tr> </table>
-
I want to make it show that a card has expired if it's after the expiration date. Dates are stored in DATETIME format in the database so this is what I have done:
<?php $today = date("Y-m-d"); ?> Status: <?php if ($ExpirationDate < $Today) { echo 'The card has expired.'; } else { echo 'Active.'; } ?>
It seems that my card is never expired even though I set the date in the database to be: 2010-02-26 00:00:00
Thanks!
-
I am working on a server status script using fsockopen. When the server is online, it displays online fine. However, when it is offline, it lists the server offline but gives me an fosckopen error. How do I make it so the PHP error is not shown and just the offline is shown?
<?php function server_check ($hport,$hdomain) { $fp = fsockopen($hdomain, $hport, $errno, $errstr, 4); if (!$fp){ $data = "<font color=red>OFFLINE</font>"; } else { $data = "<font color=green>ONLINE</font>"; fclose($fp); } return $data; } ?> <?php echo "<table width=200 border=0 cellspacing=1 cellpadding=6 bgcolor=#CCCCCC>"; echo "<tr bgcolor=#E5E5E5><td width=100><strong>Service</strong></td>"; echo "<td width=100><div align=center><strong>Status</strong></div></td></tr>"; echo "<tr bgcolor=#FFFFFF><td width=100>Polaris</td>"; echo "<td width=100><div align=center>".server_check("3389","192.168.19.42")."</div></td></tr>"; echo "<tr bgcolor=#FFFFFF><td width=100>SIP</td>"; echo "<td width=100><div align=center>".server_check("5000","192.168.19.31")."</div></td></tr></table>"; ?>
Here is the error that is being shown:
Warning: fsockopen() [function.fsockopen]: unable to connect to 192.168.19.31:5000 (A connection attempt failed because the connected party did not properly respond after a period of time, or established connection failed because connected host has failed to respond. ) in C:\wamp\www\includes\functions.php on line 16 -
bump, anyone?
-
When users click the refresh button, I want the records from the Access database to move to the MySQL database.
It semi-working except I am only getting the first record from the Access database transferred over. Any ideas on how to get the other records?
<?php $conn=odbc_connect('pcres','',''); $sql="SELECT PC_Id, Stop_Time FROM usage"; $rs=odbc_exec($conn,$sql); require_once('includes/mysql.php'); $pc = odbc_result($rs,"PC_Id"); $stop = odbc_result($rs,"Stop_Time"); $query_users = "SELECT pc, stop FROM pcres ORDER BY stop ASC"; $users = mysql_query($query_users) OR die ('Cannot retrieve a list of current users.'); if (isset($_POST['refresh'])) { $delete_old = "DELETE FROM pcres"; $result_old = mysql_query($delete_old) OR die ('Could not delete old data.'); if ($delete_old) { $insert = "INSERT INTO pcres (pc, stop) VALUES ('$pc','$stop')"; $result = mysql_query($insert) OR die ('Could not insert into database.'); if ($insert) { header('Location: index.php'); exit; } } } ?> <form id="add" name="add" method="post" action=""><input type="submit" name="refresh" id="button" value="REFRESH" /></form> <table class="data" border="1"> <tr> <th><div align="left">PC</div></th> <th><div align="left">Stop Time</div></th> </tr> <?php do { ?> <tr onmouseover="this.bgColor='#EBFAFF';" onmouseout="this.bgColor='#FFFFFF';"> <td><?php echo $row_users['pc']; ?></td> <td><?php echo $row_users['stop']; ?></td> </tr> <?php } while ($row_users = mysql_fetch_assoc($users)); ?> </table>
Run php script from URL instead of form.
in PHP Coding Help
Posted
Did you try to put the URL in the <form> tag?
<form action="URL" method="post">