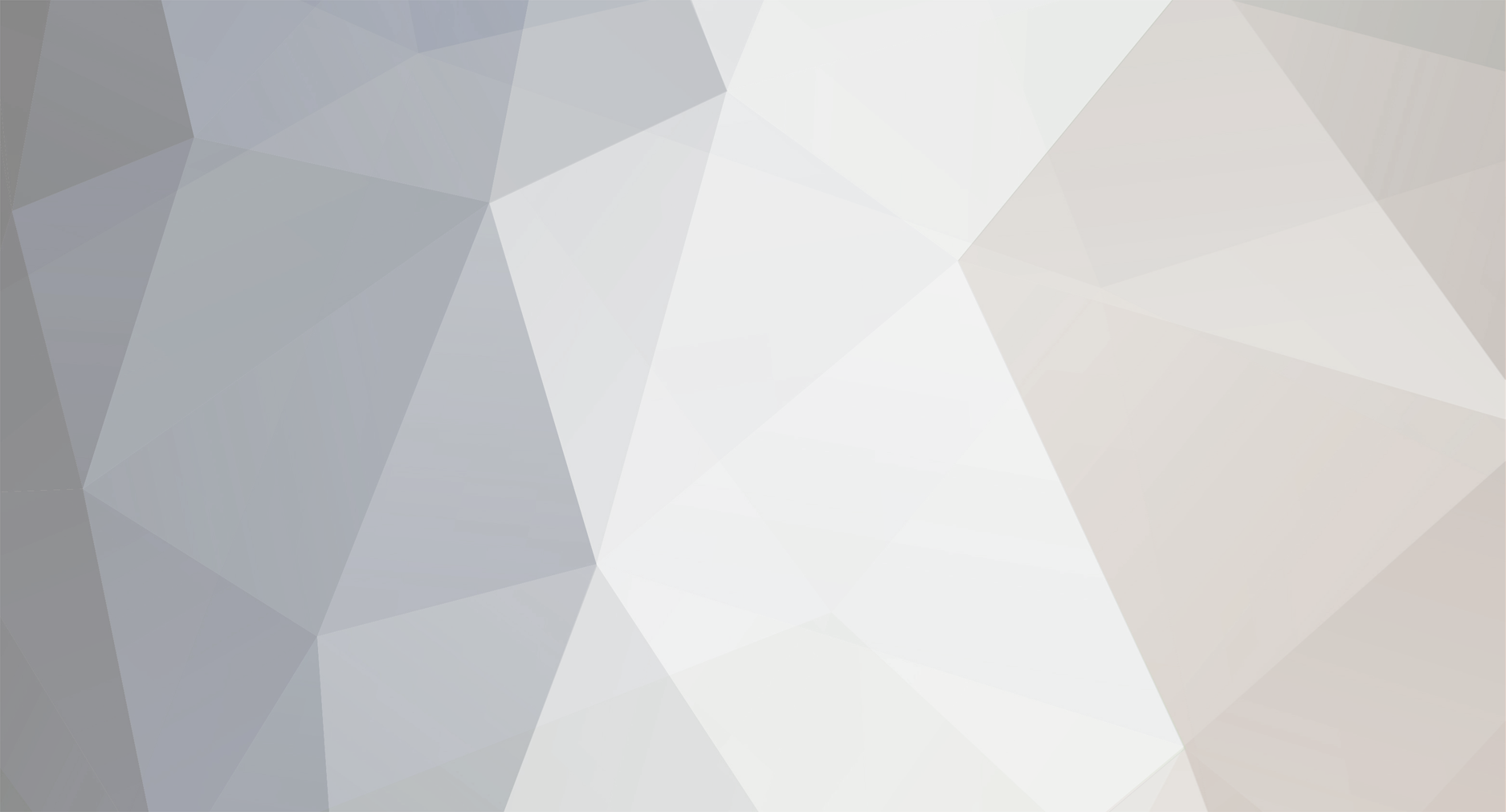
HeyRay2
Members-
Posts
223 -
Joined
-
Last visited
Never
Everything posted by HeyRay2
-
The method posted by [b]bltesar[/b] will work if you make change to your query: [code=php:0] $query_count = "SELECT count(*) AS rowcount FROM gallery"; [/code] and change the [b]$totalrows[/b] to read this: [code=php:0] $totalrows=$row['rowcount']; [/code] so your query code would read like this: [code=php:0] $query_count = "SELECT count(*) AS rowcount FROM gallery"; $result_count = mysql_query($query_count); $row = mysql_fetch_array($result_count, MYSQL_ASSOC); $totalrows = $row['rowcount']; [/code] also, your code for printing a [b]Prev[/b] link will not work, because you have a [b]&[/b] where you need a [b]?[/b]. Change this line: [code=php:0] echo ("<a href=\"$PHP_SELF&page=".$pageprev."\">PREV".$limit."</a> "); [/code] to this: [code=php:0] echo ("<a href=\"$PHP_SELF?page=".$pageprev."\">PREV".$limit."</a> "); [/code]
-
Good catch, [b]king arthur[/b]. Make these changes to the code I posted: [code=php:0] $pageprev = $page--; $pagenext = $page++; [/code] to this: [code=php:0] $pageprev = $page - 1; $pagenext = $page + 1; [/code] and you should be good to go... ;)
-
Try these changes. I changed some [b]echo()[/b] formatting, added some page validation checking, and removed some redundant code. [code=php:0] $numofpages = ceil($totalrows / $limit); // Check that the page requested is a valid page number if($page < 1){ $page = 1; } if($page > $numofpages){ $page = $numofpages; } if($page > 1){ $pageprev = $page--; echo ("<a href=\"$PHP_SELF&page=".$pageprev."\">PREV".$limit."</a> "); }else{ echo ("PREV".$limit." "); } for($i = 1; $i <= $numofpages; $i++){ if($i == $page){ echo($i." "); }else{ echo("<a href=\"$PHP_SELF?page=".$i."\">$i</a> "); } } if($page < $numofpages){ $pagenext = $page++; echo("<a href=\"$PHP_SELF?page=".$pagenext."\">NEXT".$limit."</a>"); }else{ echo("NEXT".$limit); } [/code] Let us know how this works.
-
Okay, so you're not getting your Prev and Next to show up as links, and you're not getting links to your pages printed out. I tested your page by adding "?page=<pagenumber>" to the URL, but I'm not seeing the results change. Can you post the code you are using to define your [b]$page[/b], [b]$totalrows[/b], and [b]$limit[/b] variables?
-
Add this code: [code=php:0] // Set the maximum width and height $max_width = 400; $max_height = 20; // Check if either dimension is over the maximum if (($height > $max_height) || ($width > $max_width)) { // Set a size factor variable, depending on which // dimension is greater if ($height > $width) { $sizefactor = (double) ($max_height / $height); } else { $sizefactor = (double) ($max_width / $width) ; } $newwidth = (int) ($image_width * $sizefactor); $newheight = (int) ($image_height * $sizefactor); } [/code] After this line: [code=php:0] //FINDS SIZE OF THE OLD FILE list($width, $height) = getimagesize($filename); [/code] Let us know if that works... ;)
-
That sounds like a caching problem. Try this: [code=php:0] <?php header("Cache-Control: no-cache, must-revalidate"); // HTTP/1.1 header("Expires: Mon, 26 Jul 1997 05:00:00 GMT"); // Date in the past ?> [/code] More information on cache control can be found here: http://us2.php.net/manual/en/function.header.php
-
Do a header relocation: [code=php:0] //Update character zone $sql = "UPDATE " . RPG_CHARACTERS_TABLE . " SET character_area = '$destination_id' WHERE character_id = '$user_id' "; if( !($result = $db->sql_query($sql)) ){ // Zoning failed $zoned = false; } else { $zoned = true; } // go back to zone page Header("Location: zone.php?zoned=".$zoned); // Stop further page execution exit; [/code] and on your zone.php, where you show the current zone info: [code=php:0] if($_GET['zoned'] == true){ // Zone was successful } else { // Zoning failed } [/code]
-
HELP!!!!! Passing Parameters in the URL from website to website!
HeyRay2 replied to 420blaze's topic in PHP Coding Help
The first thing you have to do is authenticate the user information on your end, so below is a basic form to do that: [b]login.php[/b] [code] <?php // Check if the login form was submitted if($_POST['login']){ // Get the username and password from the form submission $username = $_POST['username']; $password = $_POST['password']; // Query the database $sql = "SELECT * FROM 'users' WHERE 'username' = '$username' AND 'password' = '$password' LIMIT 1"; $result = mysql_query($sql) or die("Error Retreiving Users: ".mysql_error()); $row = mysql_fetch_array($result); // Check if any results were returned if($row > 0){ // If a match for the user information was found, // start a session and register the username and password in the session session_start(); $_SESSION['username'] = $username; $_SESSION['password'] = $password; // Define the iframe $iframe_loc = "http://www.remotesite.aspx" . "?username=" . $_SESSION['username'] . "&password=" . $_SESSION['password']; // Start showing the web page echo '<html> <body>'; /// Show the iframe echo '<iframe src="'.$iframe_loc.'">error messege for if the browser cant display it</iframe>'; // Close the page echo '</body> </html>'; } else { // If no matches were found for the user information provided // Go back to the login page with an error set Header("Location: login.php?error=1"); } } else { // Start the page echo '<html> <body>'; // Show any errors if($_GET['error'] = 1){ echo '<strong><font color="red">There was an error with your login information</font></strong>'; // Show the login form: echo '<h1>Login</h1> <form action="login.php" method="POST"> <input type="text" name="username"><br /> <input type="password" name="password"><br /> <input type="submit" name="login" value="Login!"> </form>'; // Close the page echo "</body> </html>"; } ?> [/code] [b]NOTE: The code above is aimed to be a simple example only, I highly recommend you encrypt the password before you check it using md5() or similar[/b]. Once you've have the login information for the current user, then you can take that information and pass it to the remote site in the following line from above: [code] // Define the iframe $iframe_loc = "http://www.remotesite.aspx" . "?username=" . $_SESSION['username'] . "&password=" . $_SESSION['password']; [/code] For this to work, the remote site has to accept login information through the $_GET variable array, and you will need to be sure that you're passing it variable names that it understands. The only alternative would be to configure the remote site to query [b]YOUR[/b] database to check the login information. -
anyone know any tutorials on the net for my problem!?
HeyRay2 replied to dalemorson's topic in PHP Coding Help
[b]tomfmason[/b] points to a good tutorial. You could take the basic idea of that tutorial and make the user profile information viewable by everyone, but only editable by that user. -
How to forward all variables to another URL ?
HeyRay2 replied to adrianTNT's topic in PHP Coding Help
I don't really understand why you couldn't process the variables passed to your site directly from 2co.com, but if you have to redirect payment information, I would most certainly avoid passing that information through a URL. How many servers are involved in this process, just the 2co.com and your web server? If so, you could create a session and register the information you received in the session, redirect to the new URL and grab the information on the new page in the $_SESSION array. If you have a database that you can save the information to, create a table to hold your sales transactions, and give each transaction a unique ID number, and the you could pass just that ID number through a URL, and then pull the information from the database at the new location. Barring sessions or a database, you could save the information to a file on your server, and give the file name a randomly generated name, pass that random name through the URL and then pull the information back out of the file for processing. However, the best method would be to find a way to process that information right after receiving it from 2co.com. Good luck. -
[code=php:0] //select the entry that matches this userID $userID=$_SESSION['userid']; $query="SELECT * FROM users WHERE userID='$userID'"; [/code]
-
Let's say, for example, that you have 16 results, at 3 results per page. If you use this code: [code] $totalpages = $totalpages / $limit; $totalpages = round($totalpages,0); [/code] You will get a result of [b]5.33[/b] pages, which will get rounded down to [b]5[/b] with [b]round()[/b]. Change this line in your code: [code]$totalpages = $totalpages / $limit;[/code] to this: [code]$totalpages = ceil($totalpages / $limit);[/code] and remove this line: [code]$totalpages = round($totalpages,0);[/code] That will give you [b]6[/b] pages as your result in my example, which should fix your issue.
-
[b]nethnet[/b], I looked for an advanced tutorial, but I was unable to locate it. Normally those are the first things I link to when replying to code questions. Do you have a link to that tutorial? I would be ineterested to see the method that was used.
-
There are some great tutorials on this site for basic pagination, however [b]mcmuney[/b] wanted something a little more advanced.
-
Did you try this? [code=php:0] <?php $text = $status[2]; include("/blahblah/myinclude.php") ?> [/code] If not, try it out and let us know if it works.
-
But your include file is already in PHP mode: [b]/blahblah/myinclude.php[/b] [code] <?php echo "$text"; // Text Inc ?> [/code] Which translates to bring read as: [code] echo '<? echo $status[2] ?>'; [/code]
-
Okay, here we go. I updated your [b]getPageingLine[/b] function for the pagination you requested, but you can add this to your other paging functions as well if you want similar functionality. [code=php:0] function getPageingLine($PageURL,$PageNo,$numPages,$frmname="form1",$class="dark_blue"){ // Set a variable to limit how many links are shown $maxLinks = 10; // Cut our max links in half, so we can get an even number of // page links before and after the current page $linksPerSide = floor($maxLinks / 2); // Determine the start and end pages, set variables accordingly // Start by checking if we need to limit our results. We determine this // by checking if there are more results pages than max links if($numPages < $maxLinks){ // We have less pages than max links, so show them all $startPage = 1; $endPage = $numPages; } else { // If we have more results than max links can show at one time, then we need // to limit what links are printed. // // Start by checking if we are currently less than half of our max links away // from the first page of results if(($PageNo - $linksPerSide) <= 1){ // If we are less than half our max links to the first page, // set our first link to print out as page 1 $startPage = 1; // Add the number of max links to the start page to get the end page $endPage = $startPage + $maxLinks; } elseif(($PageNo + $linksPerSide) >= $numPages){ // If we are less than half our max links to the last page, // set our last page as the number of total pages $endPage = $numPages; // Subtract the number of max links from the end page to get the start page $startPage = $endPage - $maxLinks; } else { // If we have more than half max links between the first and last pages of results // set our current page in the middle of the links we show $startPage = $PageNo - $linksPerSide; $endPage = $PageNo + $linksPerSide; } } //For MultiLinagual $strFirst = "First"; $strNext = "Next"; $strLast = "Last"; $strPrevious = "Previous"; if ($PageNo == 1) // this is the first page - there is no previous page echo $strFirst . " | "; else echo "<a onclick=\"javascript:".$frmname.".page.value=1;".$frmname.".formSubmit.click();\" href=\"$PageURL&page=1\" class=$class>".$strFirst."</a> | "; if ($PageNo == 1) echo $strPrevious; else echo "<a onclick=\"javascript:".$frmname.".page.value=".($PageNo-1).";".$frmname.".formSubmit.click();\" href=\"$PageURL&page=" . ($PageNo - 1) . "\" class=$class>".$strPrevious."</a>"; // Here we will print out our page links for($i = $startPage;$i <= $endPage;$i++){ // If we are printing out the current page number, don't make it a links if($i == $PageNo){ echo $i; } else { // Otherwise, print a link for the page echo " <a onclick=\"javascript:".$frmname.".page.value=".($i).";".$frmname.".formSubmit.click();\" href=\"$PageURL&page=" . ($i) . "\" class=$class> ".$i."</a>"; } } if ($PageNo == $numPages) echo " | " . $strNext; else echo " | <a onclick=\"javascript:".$frmname.".page.value=".($PageNo+1).";".$frmname.".formSubmit.click();\" href=\"$PageURL&page=" . ($PageNo + 1) . "\" class=$class> ".$strNext."</a>"; if ($PageNo == $numPages) echo " | ". $strLast; else echo " | <a onclick=\"javascript:".$frmname.".page.value=".$numPages.";".$frmname.".formSubmit.click();\" href=\"$PageURL&page=" . $numPages . "\" class=$class> ".$strLast."</a>"; } [/code] Regarding the boxes that you see on the link you provided, that's all formatting. You can change the look of your pagination however you wise, and the function will still be the same. Let me know how this works for you.
-
So you want to show links to [b]all[/b] pages, or something like I mentioned?
-
When posting to a help forum, I would highly recommend that you be as specific as possible on the problem you are having or the functionality you are looking for. This will ensure that people will quickly have the information they need to provide you assistance. It's not wise to make others have to dig around to discern what your question is. Onto your question, after looking deeper the pagination on the page you link, I think I believe what type of functionality you are looking for. You would like your pagiation to have a sort of "result page" limit, showing only a certain number of links to other pages around your current page. For examle, say you're on page 50 of 100, you want your links to look something like: [b]<< Prev 47 48 49[/b] 50 [b]51 52 53 Next >>[/b] To accomplish this, you'll want to set something of a "maxlinks" variable. Let me ponder on this for a moment, and I"ll post back with some sample code.
-
Why are you putting tags around this? [code=php:0] <? echo $status[2] ?> [/code] You are already in PHP mode with this code block: [code=php:0] <?php $text = '<? echo $status[2] ?>'; include("/blahblah/myinclude.php") ?> [/code] Try this: [code=php:0] <?php $text = $status[2]; include("/blahblah/myinclude.php") ?> [/code]
-
Lots and lots of animated badgers on your login page. That will keep those hackers at bay! ... ;D Seriously though, it sounds like you are on the right track. Just be sure to validate all input your get from the user, and keep tabs on where they go and what they do. Good luck!
-
[quote] [code] // Write the queries $sql = "INSERT INTO make(make_id, make) VALUES ('$make_id','$make')"; $sql2 = "INSERT INTO model (model_id, model) VALUES ('$model_id','$model')"; $sql3 = "INSERT INTO submodel(model_id, submodel) VALUES ('$model_id','$submodel')"; // Execute the queries mysql_query($sql) or die(mysql_error()); mysql_query($sql2) or die(mysql_error()); mysql_query($sql3) or die(mysql_error()); [/code] [/quote] LOL, that code looks familiar. Let me clarify what you are asking. Are you wanting to populate your input text boxes with values that already exist in the database, or are you wanting to know what "[b]name[/b]" each input box should have in order to run these queries? [code] // Write the queries $sql = "INSERT INTO make(make_id, make) VALUES ('$make_id','$make')"; $sql2 = "INSERT INTO model (model_id, model) VALUES ('$model_id','$model')"; $sql3 = "INSERT INTO submodel(model_id, submodel) VALUES ('$model_id','$submodel')"; [/code]
-
It sounds like you already have the queries written and ready to go. As long as the variables you mention in them exist, you can run a unlimited amount of queries. So, why not just do this: [code=php:0] // Write the queries $sql = "INSERT INTO make(make_id, make) VALUES ('$make_id','$make')"; $sql2 = "INSERT INTO model (model_id, model) VALUES ('$model_id','$model')"; $sql3 = "INSERT INTO submodel(model_id, submodel) VALUES ('$model_id','$submodell')"; // Execute the queries mysql_query($sql) or die(mysql_error()); mysql_query($sql2) or die(mysql_error()); mysql_query($sql3) or die(mysql_error()); [/code]
-
[code] <script language="javascript"> <!--- static=0; function Increment() { static++; } //--> </script> <form> <input type=button name=button value="CLICK" onClick="Increment()"> </form> [/code]
-
Let's save you some database calls and move things around a bit: [code=php:0] <?php // Fetch favorites for the current user, place them in an enumerated array $sqlfavc = mysql_query("SELECT favid FROM favorites WHERE username='$session->username'") or die("Error Retrieving User Favorites: ".mysql_error()); $user_favs = array_values(mysql_fetch_array($sqlfavc)); // Fetch all favorites $sqltwo1 = mysql_query("SELECT * FROM ".TBL_LAYOUTS." ORDER BY views DESC LIMIT 3") or die("Error Retrieving Favorites: ".mysql_error()); // Loop through all favorites while ($r=mysql_fetch_array($sqltwo1)) { $hl_id=$r["id"]; $hl_subcat=$r["subcat"]; $hl_username=$r["username"]; $hl_lname=$r["lname"]; $hl_thumb=$r["thumb"]; $hl_views=$r["views"]; $hl_views++; $sqltwo2 = "UPDATE ".TBL_LAYOUTS." SET views='$hl_views' WHERE id='$hl_id'"; $result = mysql_query($sqltwo2); //top 3 layouts table is right here.. add/delete to favorites images are within the table. // Check if the user already has this favorite if(in_array($hl_id, $user_favs)){ //show delete favorite image } else { //show add favorite image } } ?> [/code]