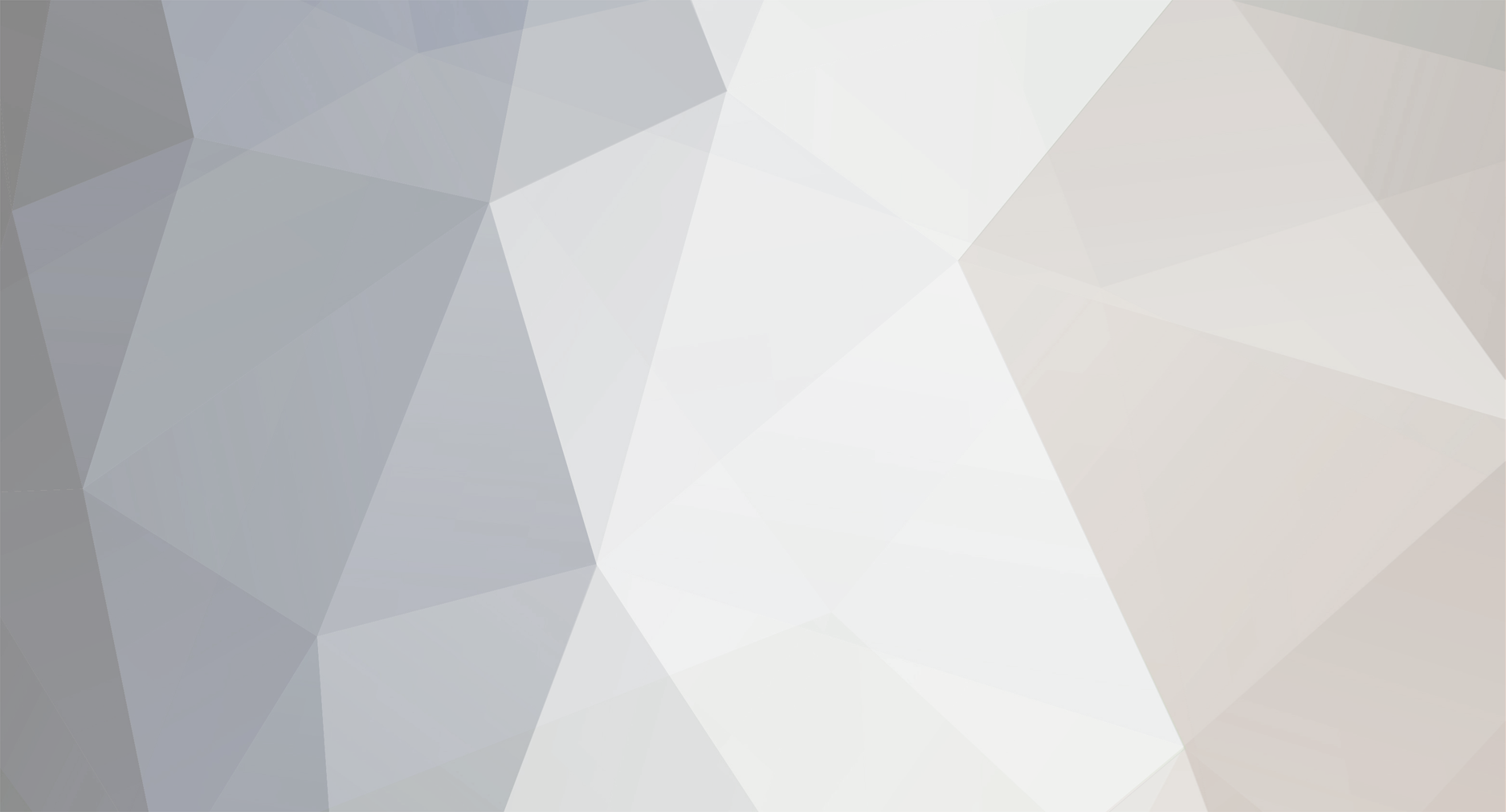
Jenk
-
Posts
778 -
Joined
-
Last visited
Never
Posts posted by Jenk
-
-
It's because you have it encompassed in braces.
Valid formats:
[code]<?php
$string = "blah$array[var]blah" . "blah${array['var']}blah" . "blah{$array['var']}blah"
?>[/code] -
[code]<?php
$p = '';
if (!empty($_GET['p'])) $p = $_GET['p'];
switch ($p) {
case 'cat_1':
include $p . '.php';
break;
/* snip.. */
default:
include 'default.php';
}
//etc.[/code] -
Just for the sake of it, try $row['lam_number'] instead of $row[lam_number]
-
Glad to be of service. :)
-
Actually, I forgot about that. Scrap OSCommerce from my list as it is now very much a dated application and does infact break in most instances off the shelf, requiring several user contributions to be installed (awkwardly in some cases) post main installation which is nothing shy of a PITA.
-
XCart
Actinic
Google 'PHP shopping cart'
OSCommerce
Monster Cart (ASP)
The list is long. -
I'm going to guess you are having problems with the insert and receiving a mysql error message?
Question (and hopefully you'll be able to figure out the problem from this..)
Where are your variables defined? -
don't echo/print anything until all scripting has completed.
-
take heed, PHP_SELF can be tainted. Use $_SERVER['SCRIPT_NAME'] instead.
-
You could use PHP to execute a ping request or similar, and you could even script it to SSH to the box to verify the webserver is running, but over HTTP you will not be able to request the page without it registering a hit - UNLESS the 'hit scoring' system has an exceptions list and you can add a domain/ip/mac-address/login/authentication/etc. clause.
-
are you session_start()'ing at the top of every page? and before any and all output? (error messages can also be a problem as they are output before _anything_ else is.)
-
no, just use explicit declarations/definitions such as:
[code]<?php
$products = $_POST['products'];
?>[/code]
or just go straight for:
[code]<?php
foreach ($_POST['products'] as $product) {
//etc
?>[/code]
but remember to sanitise your input for its relevant purpose, and to check if there is any input in the first place. -
Urgency is something that a project this large does not accomodate, this is not a quick fix/job and you will need to spend considerable time planning.
Start with: Everytime something needs retrieving, adding or modifying to your database tables, you'll need to generate and execute an SQL statement. -
use file system strings, not http:// urls.
Relative path's can also be used.
e.g. if your includes are in "/var/www/includes/" but your document root is "/var/www/docroot/" and you want to use an include file in your main index, you can use:
[code]<?php
include '../includes/ClassFile.php';
//etc.
?>[/code]
I prefer to use realpath() to get the absolute path (also to verify the path exists) before including however. -
no, you can't have multiple docroots :)
just move all your tutorial files into the docroot or modify them. -
depends on what you mean by user posting. If it's the same user who just posted, yes, as above.
But if you mean refresh when [i]another[/i] user posts, no. Not with PHP alone. -
It will work providing the value is there.
Have you tried echoing $_POST['tarriff_name'] to see if the value is present?
What value does $img hold? (echo it) -
Yes they do.
register_globals is now set to Off by default and will be removed completely from php6 onwards.
It is also bad practice to rely upon register_globals.
also, even if you are relying on register_globals, are they even defined in the $_GET, $_POST, $_COOKIE, $_SERVER or $_FILES superglobal arrays? -
include does not work in that way.
include is just like copying and pasting the code into your script manually.
a.php:[code]<?php
echo $a;
?>[/code]
b.php:
[code]<?php
$a = 'Hello World!';
include 'a.php';
?>[/code]
output:
[code]Hello World![/code] -
none of your variables are defined before you try to obtain their supposed values.
e.g. $products doesn't exist until you try to foreach() it. -
What's the exact problem? No session data? Session not starting? Session collisions?
Also, using globals in classes is frowned upon. have a look into designing a session handler object. (NOT related to session_set_save_handler(), but the design pattern "session handler") -
you'll need to either change your document root directive in httpd.conf and php.ini or change all the files.. choice is yours.
and re: include syntax:
[code]<?php include_once '<file>'; ?>[/code] is the correct syntax as mentioned in the php manual. It's a construct, not a function.[/code] -
use checkboxes like so:
[code]<?php
if (empty($_POST['checkbox']) {
?>
<form action="" method="post">
<input type="checkbox" name="checkbox[]" value="check1" />
<input type="checkbox" name="checkbox[]" value="check2" />
<input type="checkbox" name="checkbox[]" value="check3" />
<input type="checkbox" name="checkbox[]" value="check4" />
<input type="checkbox" name="checkbox[]" value="check5" />
<input type="submit" value="Submit" />
</form>
<?php
} else {
foreach ($_POST['checkbox'] as $box) {
echo "<p>Check box: {$box} was checked!</p>\n";
}
}
?>[/code] -
[code]<?php
$query = "INSERT INTO `table` VALUES ('" . mysql_real_escape_string($_POST['textarea']) . "')";
echo '<textarea>' . nl2br(htmlentities($_POST['textarea'])) . '</textarea>';
?>[/code]
btw, nl2br() is the equivalent of [code]<?php
function nl2br ($string)
{
return str_replace("\n", "<br />\n", $string);
}
?>[/code]
typo? misprint?
in PHP Coding Help
Posted
you have to specify indices by type and when you use braces, you are extrapolating the variable they encompass. Whe using braces, this reverts back to how you must use them on the php scope, as you should be able to see in your example, all three will throw a notice error because PHP will think you are specifying the Constant called 'variable' and not specifying the string value of 'variable'
e.g.:
[code]<?php
$array['var'] = 'foo'; //indice is a String, as denoted by the apostrophies.
$string = "blah $array[var] blah"; //works - 'var' is still a string here, because the variable is within a string.
?>[/code]