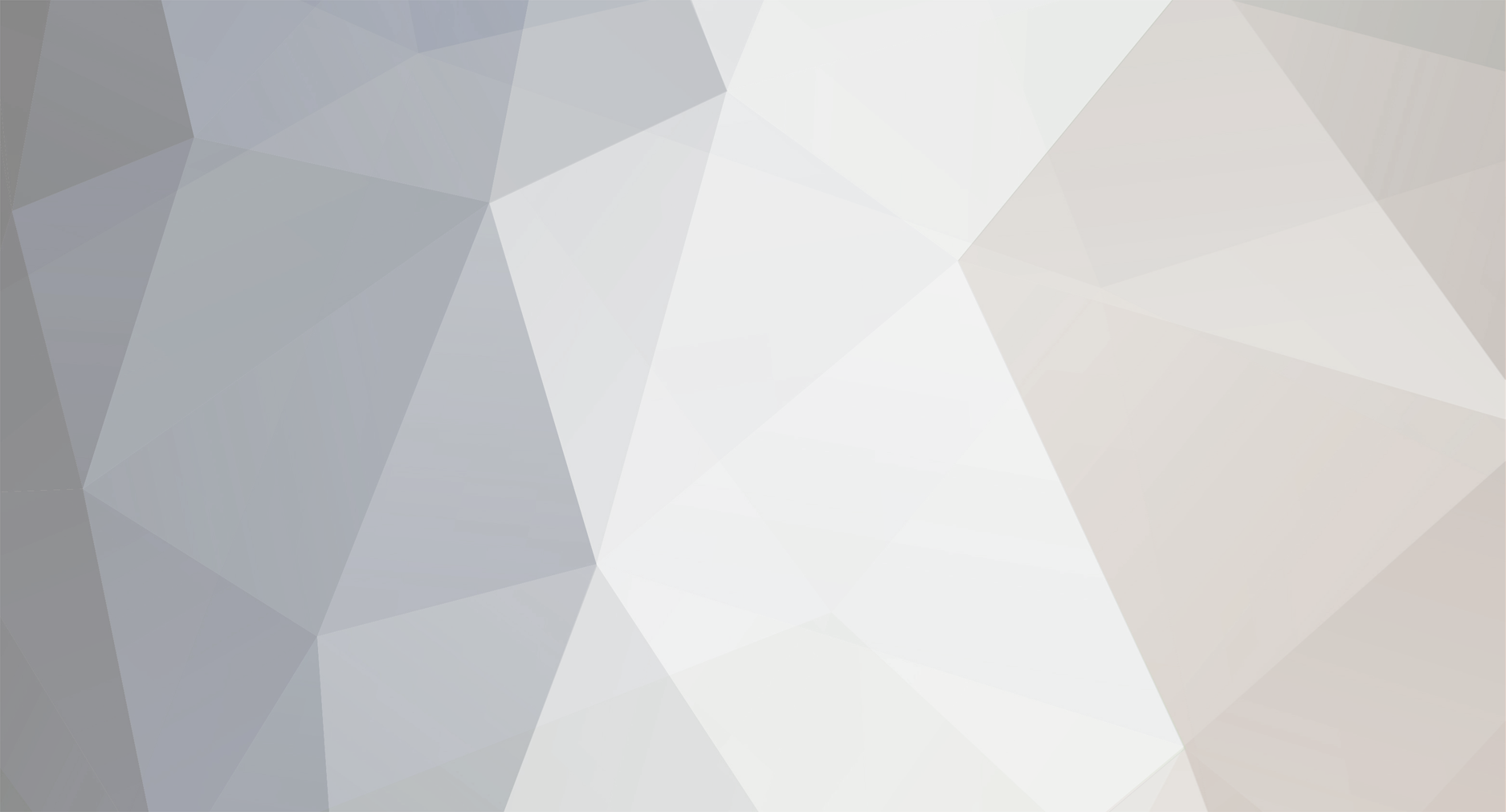
Jenk
-
Posts
778 -
Joined
-
Last visited
Never
Posts posted by Jenk
-
-
[code=php:0]foreach($array as $key => $val) {
echo "<option $sel value=\"".htmlentities($val)."\">".htmlentities($key).'. '.htmlentities($val)."</option>";
}[/code] -
reliance on the user agent string is not bullet proof, because it is of course dependant on the user agent broadcasting it in the first place.
FireFox allows the user to change their user agent string quite easily for example.
In other words.. make your site accessible to all browsers.
Should also use a keyword search rather than standard comparison:
[code]<?php
if (stripos('mozilla', $_SERVER['HTTP_USER_AGENT']) === false) {
die('Get firefox.');
}
?>[/code]
-
an example of how you make your input 'safe'
[code]$username = mysql_real_escape_string($_POST['username']);[/code] -
[code]<?php
$days = array(
'Sundays text',
'Mondays text,
/* etc */
'Saturdays text'
);
echo $days[array_rand($days)];
?>[/code] -
or you can just be sensible and not use the session ID as the key on your basket table.. if the user accidentaly disconnects after adding a large number of items to a cart they are going to be mighty annoyed to find it all lost when they return..
-
can you clarify what (and why?) you want to ping a server?
-
read up on arrays on http://php.net/arrays
look at array_diff and array_merge. -
Which template engine you using? (I'm guessing Smarty?)
Anyway, to get you started..
[code]<?php
$queued = mysql_query("SELECT * FROM links WHERE links_status = 'queued'");
if (!mysql_num_rows($queued)) {
$quededs_link_html = array();
while ($row = mysql_fetch_assoc($queued)) {
$quededs_link_html[] = array(
'href' => $row['links_url'],
'img' => $row['links_image'],
);
}
$tpl->assign('quededs_link_html', $quededs_link_html);
}
?>[/code]
and if it is Smarty, your template can now use the following to display the above:
[code]<table>
{if isset($links)}
{section name=link loop=$links}
<tr><td><a href="{$links[link].href}"><img src="{$links[link].img}"></a></td></tr>
{/section}
{else}
<tr><td width="100%" bgcolor="#FFFFFF" class="style7">There are 0 links waiting in queue</td></tr>
{/if}
</table>[/code]
btw.. don't hardcode HTML into you app.. that's why you are using templates in the first place. -
[code]<?php echo htmlentities('<a href="http://www.example.com/">link</a>'); ?>[/code]
-
everytime user clicks 'add to basket,' add it to the session data and/or table.
everytime user clicks 'remove from basket,' remove it from the session data and/or table.
Baskets are no different from your standard Order tables/pages. -
but do you get any output?
-
do you get any output when you run it manually? (in command prompt)
-
can also do[code]<?php
$var += 4;
?>[/code] -
check encoding too, at a guess ezpdf will need UTF-8 - finding any docs for eZ is a pain so I can't verify.
-
[code]<?php
$cmd = "soxmix tmp/audio1.wma tmp/audio2.wma tmp/final.wma";
echo shell_exec($cmd);
?>[/code]
output? -
PHP_SELF has vulnerabilities.. use SCRIPT_NAME instead, and to be fully compat, use full URI not just relative:
[code]<?php
echo '<form action="http://' . $_SERVER['HTTP_HOST'] . $_SERVER['SCRIPT_NAME'] . '" method="post">';
?>[/code] -
sanitise user input.
-
read the manual on getcwd() - namely the return type.
-
try swapping to \r\n, and if still problematic try with just \r.
-
just a note: php is by far [b]not[/b] the best solution for this activity.
-
have you got a die/exit immediately after that?
-
[quote author=Barand link=topic=106321.msg425561#msg425561 date=1157053325]
This the approach I had in mind
[code]
<?php
session_start();
if (isset($_POST['usernum'])) {
if ($_SESSION['randomnumber']==$_POST['usernum']) {
unset($_SESSION['randomnumber']);
session_regenerate_id(true);
// process form data here
echo "<p>Number OK<br>Processing</p>";
// redirect to other page
echo "<p>Redirecting</p>";
exit;
}
else {
echo "<p>Invalid input</p>";
}
}
else {
//generate random code
$_SESSION['randomnumber'] = rand(11111,99999);
}
echo "Enter this number : {$_SESSION['randomnumber']} ";
?>
<FORM method='POST'>
Code <input type="text" name="usernum" size="5"><br>
<input type="submit" name="submit" value="Submit">
</FORM>[/code]
[/quote]added unset() and session_regenerate_id()
and simply echoing it is a bad idea.. spam bots can just 'copy and paste' it, which is why most captcha's use images - but I get that the above is just 'pseudo' -
have read up on mod_rewrite :)
-
also for a slightly more helpful contribution:
[code]<?php
$result = @mssql_query($theQuery) die('Error with Query');
?>[/code]
PHP file renaming script
in PHP Coding Help
Posted
$dir = '/path/to/old/dir';
$handle = opendir($dir);
while(($file = readdir($handle)) !== false)
{
if (preg_match('/' . preg_quote('\/ : * ? " < > |', '/') . '/', $file))
{
$path = $dir . DIRECTORY_SEPARATOR . $file;
if (!in_array($file, array('..', '.')) && is_file($path))
{
$contents = file_get_contents($path);
$newPath = $dir . DIRECTORY_SEPARATOR . preg_replace('/' . preg_quote('\/ : * ? " < > |', '/') . '/', '_', $file);
if (file_put_contents($newPath, $contents) !== false)
{
$unlink($path); //delete old file only if able to create new
}
}
}
}
?>[/code]