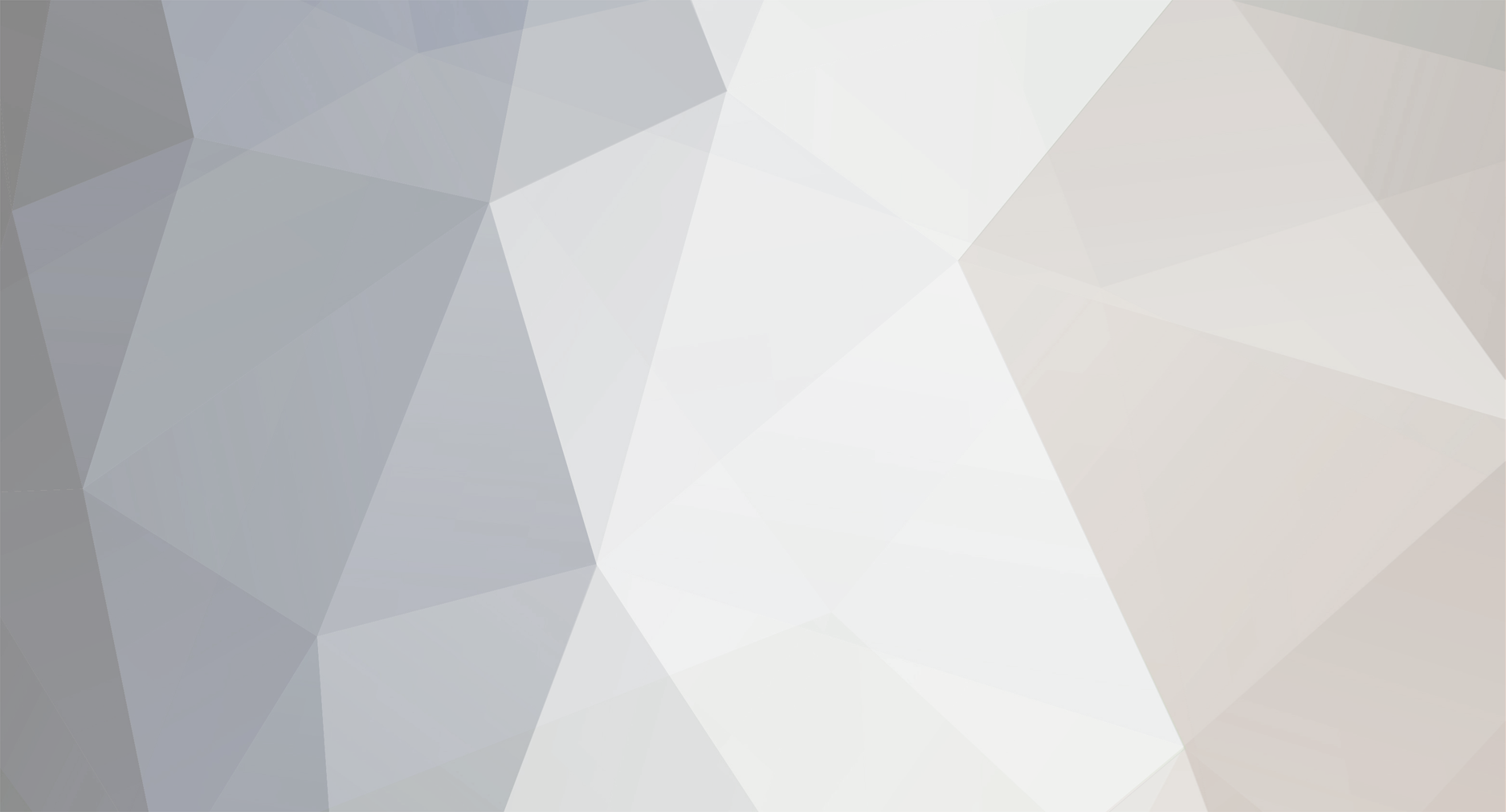
HuggieBear
Members-
Posts
1,899 -
Joined
-
Last visited
Everything posted by HuggieBear
-
Need help sorting an array - please help
HuggieBear replied to simboski19's topic in PHP Coding Help
You can't pass in additional arguments to this function as it's a callback of uasort(), but you could use global variables. See the example below. I've added two new variables, sort order and sort key. I've also added quantity to the product array as a new key to sort on. <?php // Setup product array (You already have yours) $products = array( 'Bike' => array('price' => '199.99','quantity' => '1'), 'Apple' => array('price' => '0.87','quantity' => '2'), 'Car' => array('price' => '5999.00','quantity' => '3') ); // *NEW* Specify either ASC or DESC $sortorder = 'DESC'; // *NEW* Specify the key you'd like the array sorted on $sortkey = 'quantity'; // Comparison function *NOTE* You now change the sort order with the global variable, not by swaping the return values around function compare($x, $y){ global $sortorder; global $sortkey; if ( $x[$sortkey] == $y[$sortkey] ){ $return = 0; } if ($sortorder == 'ASC'){ return ($x[$sortkey] < $y[$sortkey]) ? -1 : 1; } else { return ($x[$sortkey] < $y[$sortkey]) ? 1 : -1; } } uasort($products, 'compare'); // Output the products echo '<pre>'; print_r($products); echo '</pre>'; ?> -
No, it will work, but as far as I'm aware it can't be done in the same page as you need to output different header types. It's easier if just the image path is stored in the database. I think you'll need a page that displays the html, with the image source links pointing to something like getimage.php?imageid=1
-
Where's your code that does the insert, we'll let you know.
-
You can still use the same principle, just a variable to keep count.
-
How to get weekly time e.g from thursday to thursday???
HuggieBear replied to hmdnawaz's topic in PHP Coding Help
OK, so is it safe to assume that if Friday is the 13th of the month and you run the script you want events from the database between Thursday 12th and Thursday 19th? -
I'm well thanks, if you don't get any joy after reading that then just shout.
-
How to get weekly time e.g from thursday to thursday???
HuggieBear replied to hmdnawaz's topic in PHP Coding Help
You realise that this will only send you an email when you run the page in the browser right? -
Memorable word - How to ask for three random letters
HuggieBear replied to bb90's topic in PHP Coding Help
This should get you started // Set secret word $word = 'mysecretword'; // How many letters must they provide $required_letters = 3; // Populate array with letters keyed on their position $letters = array(); while (count($letters) < $required_letters){ $k = rand(1, strlen($word)); $letters[$k] = substr($word, $k-1, 1); } // Sort if you want them to enter the letters in order ksort($letters); // Print array echo '<pre>'; print_r($letters); echo '</pre>'; -
How to get weekly time e.g from thursday to thursday???
HuggieBear replied to hmdnawaz's topic in PHP Coding Help
So are you going to invoke the script every Friday morning or something? -
How to get weekly time e.g from thursday to thursday???
HuggieBear replied to hmdnawaz's topic in PHP Coding Help
How is this script going to be invoked? Via the browser, or via a cron job? -
Take a look at the cURL library
-
Hey Stu, you still hanging around on here. You could take a look at the is_dir() Rich
-
Are you storing the actual image in the database or just the path to the image?
-
Try this for returning the award // Query the database for active awards $res = mysql_query("SELECT code, award FROM awards WHERE active = '1'"); // Populate the codes array $codes = array(); while ($row = mysql_fetch_assoc($res)){ $codes[$row['code']] = $row['award']; } // Hardcoded userid, yours probably comes from a form or something $userid = '1'; // Query the database for user awards $res = mysql_query("SELECT * FROM log WHERE userid = '$userid'"); // Print the award details (I'm assuming this will only be a single row) $row = mysql_fetch_assoc($res); foreach ($codes as $code => $award){ if ($row[$code] == 1){ echo $award . "<br />\n"; } } As for the id, you can just use a simple count variable. Do you need the id to output as an HTML id, or do you just need it for layout of the awards?
-
It's not very good database design. What's the reason for not wanting multiple rows for each user? Like I said, it can be done the way you want but I wouldn't advise it: // Query the database for active awards $res = mysql_query("SELECT code FROM awards WHERE active = '1'"); // Populate the codes array $codes = array(); while ($row = mysql_fetch_row($res)){ $codes[] = $row[0]; } // Hardcoded userid, yours probably comes from a form or something $userid = '1'; // Query the database for user awards $res = mysql_query("SELECT * FROM log WHERE userid = '$userid'"); // Print the award details (I'm assuming this will only be a single row) $row = mysql_fetch_assoc($res); foreach ($codes as $award){ if ($row[$award] == 1){ echo $award . "<br />\n"; } } This is untested, but I expect it to be pretty close to the mark.
-
Can you change the database structure? If so, that would be better. awards awardid award code active userawards userid awardid You have a row in the userawards table for each award that each user has. Then a simple join could retrieve the results you're after. If you can't change the structure then it can still be done, but changing the structure would be better.
-
You didn't attach anything. Also, paste a description of the problem here.
-
It makes perfect sense, but I'm sure there's a better way to do it. Here's an interim solution: <?php // Declare totals as an array $totals = array(); // This is the format of your array (I assume posted from your form) notice some duplicates $submitted[] = array('1', '12113'); $submitted[] = array('2', '21200'); $submitted[] = array('4', '10200'); $submitted[] = array('1', 'yyyy'); $submitted[] = array('20', 'xxxxx'); $submitted[] = array('5', '21200'); $submitted[] = array('7', '21200'); // This sticks it into a new array keyed on product id with quantity as a value foreach ($submitted as $k => $a){ if (!array_key_exists($submitted[$k][1], $totals)){ $totals[$submitted[$k][1]] = $submitted[$k][0]; } else { $totals[$submitted[$k][1]] = $totals[$submitted[$k][1]] + $submitted[$k][0]; } } // Print the array and total quantities echo '<pre>'; print_r($totals); echo '</pre>'; ?>
-
How is this array constructed? Is it provided for you like that, or have you built it up like that?
-
You must have specified a column called 'blah blah' somewhere, or that isn't the exact error.
-
You probably want to cURL library to submit data to web services.
-
This is a PHP forum, the link you posted is a tutorial relating to a JavaScript API. Did you have a PHP related question about it?
-
OK, in that case can you post your full code. You must have 'blah blah' specified somewhere.
-
Can you actually paste the full error?