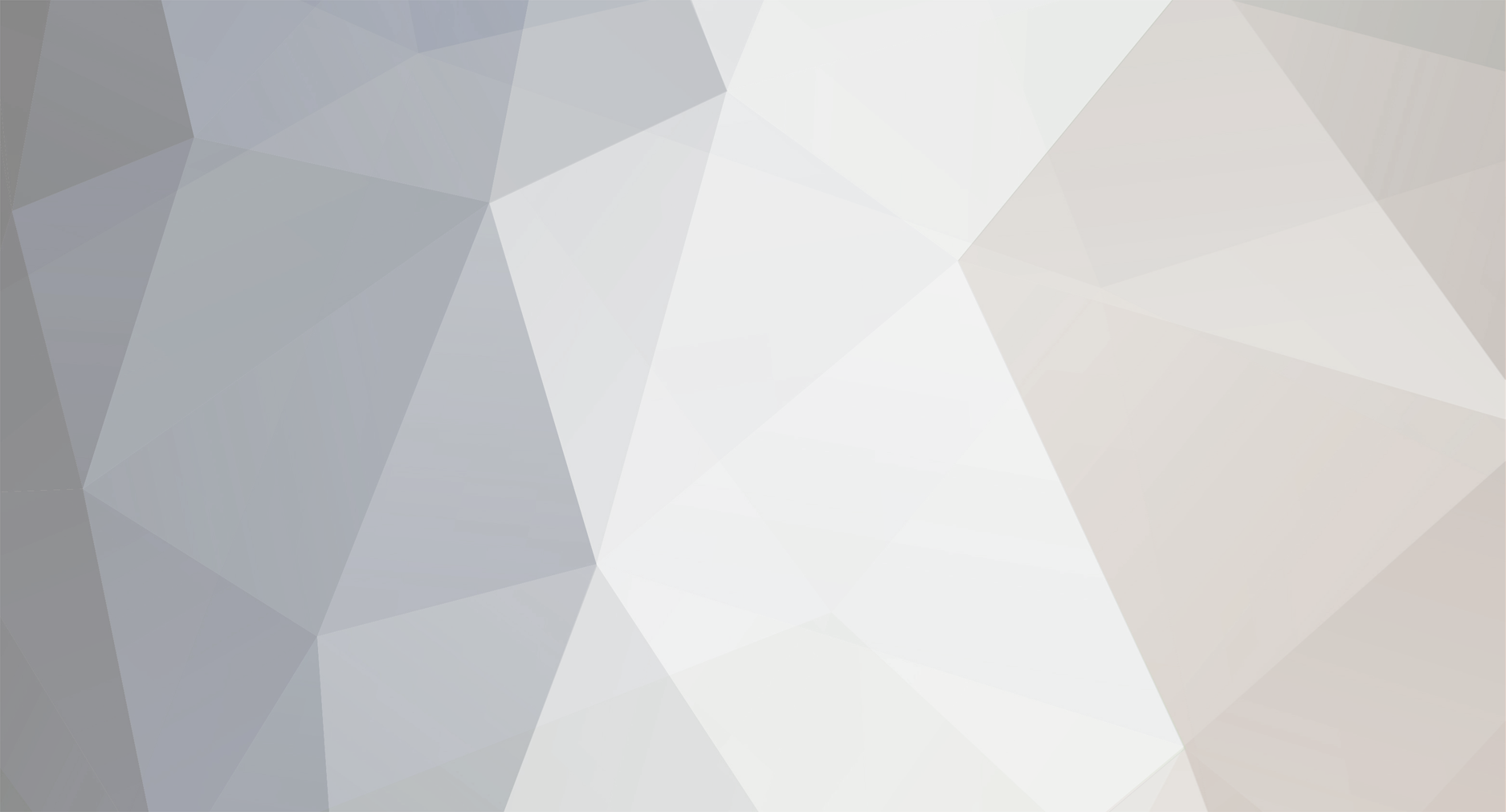
HuggieBear
Members-
Posts
1,899 -
Joined
-
Last visited
Everything posted by HuggieBear
-
[quote] The array is built with [code=php:0]$pic_code = mysql_fetch_array($result);[/code] and accessing it like [code=php:0]$pic_code[0]; $pic_code[1];[/code]... doesn't work [/quote] Have you checked you have a valid result... Try adding this to your [code=php:0]mysql_query()[/code] statement: [code] <?php $result = mysql_query($sql) or die ("Can't execute $sql: " . mysql_error()); // Will echo if the query itself is failing $pic_code = mysql_fetch_array($result); var_dump($pic_code); // Will echo the results returned from the DB ?> [/code] This should allow you to debug it a little easier. Regards Huggie
-
Guys, Just a quick one... If I insert a row into my database using mysql_query() it returns TRUE if successful and FALSE if not. I'm assigning this value to $insert... [code=php:0]$insert = mysql_query($sql) or die ("Couldn't execute $sql: " . mysql_error()); [/code] Now how do I check if $insert is true? Do I just need to include it in my if statement like so... [code=php:0]if ($insert){ // my code...;} [/code] I figured that even if $insert was FALSE, the fact that it's defined would still make the above statement true, is that correct? Regards Hungover Huggie
-
[quote] Unless you use something like ajax to check. [/quote] I didn't want to explain that route, am a little too hungover for that one :D Regards Huggie
-
You're going to need to allow the form to submit anyway. Once it checks the database to see if the user exists, it either registers them or it spits out an error, returning them to the page, the fields can be pre-filled with the values they already entered. Regards Huggie
-
Looks good, I'd correct one thing though... If you look at this page: http://pluswomen.thefreestuffvault.com/clothing-9245.htm You'll see that the <<Previous 1 2 3 4 Next>> aren't quite evenly spaced. It's only a tiny thing, but I'm anal, and it seems a shame after after all this hard work to not have it 'Perfect'. Regards Huggie
-
Make them both the same type... [code] <?php if (strtolower($userpanname) == strtolower($_POST['recip'])){ // output here } ?> [/code] Regards Huggie
-
No problem, any more questions before I become a bedtime bear? Regards Huggie
-
Try specifying the column names.... [code] $query = "INSERT INTO link (name, address, description, keywords) VALUES ('$siteName', '$url', '$description', '$keywords')"; [/code] Obviously I've made the column names up, but should help. Also, notice how I haven't escaped the variables at all? That's because you don't need to, when dealing with simple variables between double quotes " ", php reads the value of the variable, not the literal string. Regards Huggie
-
ok, if you don't want to display a default category, then maybe something like this at the top of the page... [code] <?php if (empty($_GET['cat'])){ header("Location: index.php"); } ?> [/code] This will redirect them to index.php if they don't provide a category, and we can do something else for if they provide a category, but there's no items in it. At the top of your page, insert this: [code] if ($num_records == 0){ echo "There are no records to display in the selected category, please select another\n"; exit; } [/code] Between these lines of code: [code] $num_records = mysql_result($result,0,0); <--- Insert here // Set maximum number of rows and columns [/code] This isn't very tidy or helpful, but it gives you the bare bones to add the flesh too ;) Regards Huggie
-
You still have a category field and a band field in the products table, but they only contain ID's, ID's that are linked to the primary key in both the band and categories table. It's called normalization. Try searcing on Google for it. You'd do well to spend just 15 minutes reading about it or maybe taking a tutorial on it. It seems like a boring step now, but it will save you so much time and coding in the future, and that's a promise! Regards Huggie
-
Not at all, just paste this... [code] // If there's more than one page, show links if ($total_pages > 1){ echo <<<HTML <table> <tr> <td align="center">Please select a page</td> </tr> <tr> <td align="center"> HTML; // Build the previous link if ($page > 1){ $prev = ($page -1); echo "<a href=\"{$_SERVER['PHP_SELF']}?cat=$cat&page=$prev\"><< Previous</a> "; } // Build the page numbers for($i = 1; $i <= $total_pages; $i++){ if($page == $i){ echo "$i "; } else { echo "<a href=\"{$_SERVER['PHP_SELF']}?cat=$cat&page=$i\">$i</a> "; } } // Build the next link if ($page < $total_pages){ $next = ($page +1); echo "<a href=\"{$_SERVER['PHP_SELF']}?cat=$cat&page=$prev\">Next >></a>"; } // Close off the links echo <<<HTML </td> </tr> </table> HTML; } [/code] Between these two line... [code] $num_rows = ceil(mysql_num_rows($result_main)/$num_columns); <---- Here // Lets start creating tables and echoing code [/code] Regards Huggie
-
Yes, that sounds about right... Regards Huggie
-
[quote author=alpine link=topic=109840.msg443897#msg443897 date=1159556474] my misreading - thought it was about the categories table [/quote] :D I do it all the time. Doesn't have a categories table yet I don't think, that's the point, so we'll see what we can do. Huggie
-
ok, try changing this... [code] // increment counter - if counter = max columns, reset counter and close row if(++$c == $max_columns){ echo "</tr>"; $c = 0; } } // clean up table - makes your code valid! if($c < $max_columns){ for($e = $c; $e < $max_columns; $e++){ echo " "; } } [/code] To this: [code] // increment counter - if counter = max columns, reset counter and close row if(++$c == $max_num_columns){ echo "</tr>"; $c = 0; } } // clean up table - makes your code valid! if($c < $max_num_columns){ for($e = $c; $e < $max_num_columns; $e++){ echo " "; } } [/code] Did you see that by adding in a default page and category, you can go direct to http://pluswomen.thefreestuffvault.com/testcolumns2.html without any variables in the url and still get a valid page up. Regards Huggie
-
[quote] well, using distinct would prevent more than one category ever to show up - thats unlightly what you want as a result. [/quote] That's exactly what he's after in that scenario! Did you read the previous post? [quote] I'm getting 2 "T-Shirt" links on my left menu!! The good news is the T-shirt link does show both the T-shirt products added, but obviously I don't want to add a new Category link on my left navigation every time I add a product that already is under an existing category [/quote] Regards Huggie
-
An easy fix is to change the sql code that gets the categories... You probably have something like: [code=php:0]$sql = "SELECT category FROM products"; [/code] Change to: [code=php:0]$sql = "SELECT DISTINCT category FROM products"; [/code] In the long run you'd probably be better changing your table structure if possible, having a seperate category table with a link to the products table. Imagine this if you will... A product table contains the 1 million products, each product fits into one of 10 categories, that's listed in the column called category in the product table. To 'SELECT DISTINCT' categories, MySQL has to scan 1 million rows looking for distinct values and then return the 10 that it's found. Now how much quicker would it be if MySQL went to a categories table and did a 'SELECT ALL' from that table, it only has 10 rows in it, but each of those rows is linked by a unique ID back to the product table :D Regards Huggie
-
It's not beacuse of the curly braces that it's not inserting. It's probably due to the functions you're trying to insert! Do any processing outside of the query... [code=php:0]$username = get_current_username(); $sql = "INSERT INTO table (username) VALUES ('$username')"; [/code] Not like this: [code=php:0]$sql = "INSERT INTO table (username) VALUES ('.get_current_username().')"; [/code] You could even use some SQL commands to save even more time... [code=php:0]$sql = "INSERT INTO table (date_last_modified) VALUES (curdate())"; [/code] Regards Huggie
-
Maybe you've hit the nail on the head... [quote] People ridicule me and all that I've worked for because I did something my way (and it functions the way I want it to) [/quote] If you're thinking about selling it, maybe you should be thinking about how THEY want it to work, what features THEY want in it. Why not offer a free version and when people make suggestions for new features they want, if you think they're simple and easy, put them in the free version, earn brownie points for offering their requirements for nothing, but if they're advanced features, put them in the 'Pro' version. That way, they'd actually pay for something worthy that they want. Regards Huggie
-
[quote author=tleisher link=topic=109993.msg443847#msg443847 date=1159553137] I don't want people coming back to me saying "this is too simple, I could have done this myself" [/quote] If that was the case then they'd have done it themselves, if they've purchased your code, then they're past this stage. As to whether or not they're alarmed by that fact post-purchase, is based on what you charge. If your pricing scale reflects the product properly, then they won't moan, if you charge way over the odds, then they will. If you don't try to fleece people (which I'm sure you're not) then you'll probably be fine. Regards Huggie
-
[quote author=OldManRiver link=topic=109870.msg443834#msg443834 date=1159551629] Now trying to get it to transfer to the secondary pages. Not having much luck with that. Do I need to add a "globals" for the vars to pass? [/quote] No, there's no need for 'globals' you should just be able to use any of those $_SESSION variables on any of your pages where you've started the session. Regards Huggie
-
Sorry, typo! Remove the $ from the front of [b][color=red]$[/color][/b][color=green]mysql_fetch_array(...[/color] Regards Huggie
-
Give this a try and let me know how you get on. It was written on the fly and as such is untested, so don't dispair if it doesn't work, just let me know what the error is... There's a commented line in there where I need you to add a default category. [code] <?php include("config.php"); // Sort products by Name as default //if(!$sortby){ $sortby="ProductName"; } // Commented out as it's not used anywhere, it was incorrect in your links // Select total results for pagination $result = mysql_query("SELECT count(*) FROM womensproducts WHERE CategoryID = '$cat'"); $num_records = mysql_result($result,0,0); // Set maximum number of rows and columns $max_num_rows = 3; $max_num_columns = 3; $per_page = $max_num_columns * $max_num_rows; // Work out how many pages there are $total_pages = ceil($num_records / $per_page); // Get the current page number if (isset($_GET['page'])) $page = $_GET['page']; else $page = 1; // Get the category or assign a defailt if (isset($_GET['cat'])) $cat = $_GET['cat']; else $cat = "default"; // enter a default category here // Work out the limit offset $start = ($page - 1) * $per_page; // Run your query with the limit and offset in place $result_main = mysql_query("SELECT * FROM womensproducts WHERE CategoryID='$cat' limit $start, $per_page") or die(mysql_error()); $num_columns = ceil(mysql_num_rows($result_main)/$max_num_rows); $num_rows = ceil(mysql_num_rows($result_main)/$num_columns); // Lets start creating tables and echoing code echo "<table>\n"; $c = 0; while($row = mysql_fetch_array($result_main)){ // make the variables easy to deal with extract($row); // open row if counter is zero if($c == 0){ echo "<tr>"; } // echo the individual cells echo <<<HTML <td align=center><table class="quick" width="100%" height="350" border="0" align=center cellspacing="0" cellpadding="0"> <tr> <td align=center><a href="http://pluswomen.thefreestuffvault.com/product-{$ProductID}.htm"> <img src="{$BigImage}" border="0" height=180 alt="{$Name}"></a></td> </tr> <tr> <td align=center><p id="productname"><a href="http://pluswomen.thefreestuffvault.com/product-{$ProductID}.htm">{$ProductName}</A></p></td> </tr> <tr> <td align=center><font size="2">Price: {$prodPrice}</font><font color=red> Now: ${$prodSale}</td> </tr> </table></td> HTML; // increment counter - if counter = max columns, reset counter and close row if(++$c == $max_columns){ echo "</tr>"; $c = 0; } } // clean up table - makes your code valid! if($c < $max_columns){ for($e = $c; $e < $max_columns; $e++){ echo " "; } } // If there's more than one page, show links if ($total_pages > 1){ echo <<<HTML <table> <tr> <td align="center">Please select a page</td> </tr> <tr> <td align="center"> HTML; // Build the previous link if ($page > 1){ $prev = ($page -1); echo "<a href=\"{$_SERVER['PHP_SELF']}?cat=$cat&page=$prev\"><< Previous</a> "; } // Build the page numbers for($i = 1; $i <= $total_pages; $i++){ if($page == $i){ echo "$i "; } else { echo "<a href=\"{$_SERVER['PHP_SELF']}?cat=$cat&page=$i\">$i</a> "; } } // Build the next link if ($page < $total_pages){ $next = ($page +1); echo "<a href=\"{$_SERVER['PHP_SELF']}?cat=$cat&page=$prev\">Next >></a>"; } // Close off the links echo <<<HTML </td> </tr> </table> HTML; } ?> [/code] Regards Huggie
-
I've almost finished the code and it looks good, but I have a question, why if you want three rows of three (nine in total) does your $perpage variable show a value of 7... lol I'd recoment changing $perpage to a value of max_row * max_col, and I'll include that in my code. Also, I'm trying a few different methods, can you possibly let me know the order your columns are in in the database? Regards Huggie
-
Why not give this a try? I removed the '@' sign from the query, but if it was meant to be there (if you're searcing for a string followed by an @ sign) then just add it in before the last % in the query. I've changed the query too, there's no need to escape normal variables when using double quotes " " as php translates them. [code] <?php $searchtype=$_POST['searchtype']; $searchterm=$_POST['searchterm']; $searchterm= trim($searchterm); if (!$searchtype || !$searchterm) { echo 'You have not entered search details. Please go back and try again.'; exit; } if (!get_magic_quotes_gpc()) { $searchtype = addslashes($searchtype); $searchterm = addslashes($searchterm); } $db = mysqli_connect('*', '*', '*', '*'); if (mysqli_connect_errno()) { echo 'Error: Could not connect to database. Please try again later.'; exit; } $query = "select * from link where "$searchtype" like '%$searchterm%'"; $result = $db->query($query); $num_results = $result->num_rows; echo "<p>Numbers of Links found $num_results</p>"; for ($i=0; $i <$num_results; $i++) { $row = $result->fetch_assoc(); echo '<p><b>'.($i+1).'. Site Name: '; echo htmlspecialchars(stripslashes($row['siteName'])); echo '</b><br>URL: '; echo stripslashes($row['url']); echo '<br>Discription: '; echo stripslashes($row['discription']); echo '<br>Keywords: '; echo stripslashes($row['keywords']); echo '<br>Date Added: '; echo stripslashes($row['dateAdded']); } mysqli_free_result($result); $db->close(); ?>[/code] If it doesn't work then post the errors back here. Regards Huggie
-
Correct, your details page would look something like this I guess. You'd just need to get the category from the URL and use it in the SQL query. [code] <?php include('connect.php') // Initiate your database connection $cat = $_GET['id']; $sql = "SELECT name, image, description FROM products WHERE category = '$cat'"; $result = mysql_query($sql); while ($product = $mysql_fetch_array($result, MYSQL_ASSOC)){ echo <<<HTML <table> <tr> <td><img src="{$product['image']}" alt="{$product['name']}"></td> </tr> <tr> <td>{$product['name']}</td> </tr> <tr> <td>{$product['description']}</td> </tr> </table> <br><br> HTML; } ?> [/code] Regards Huggie