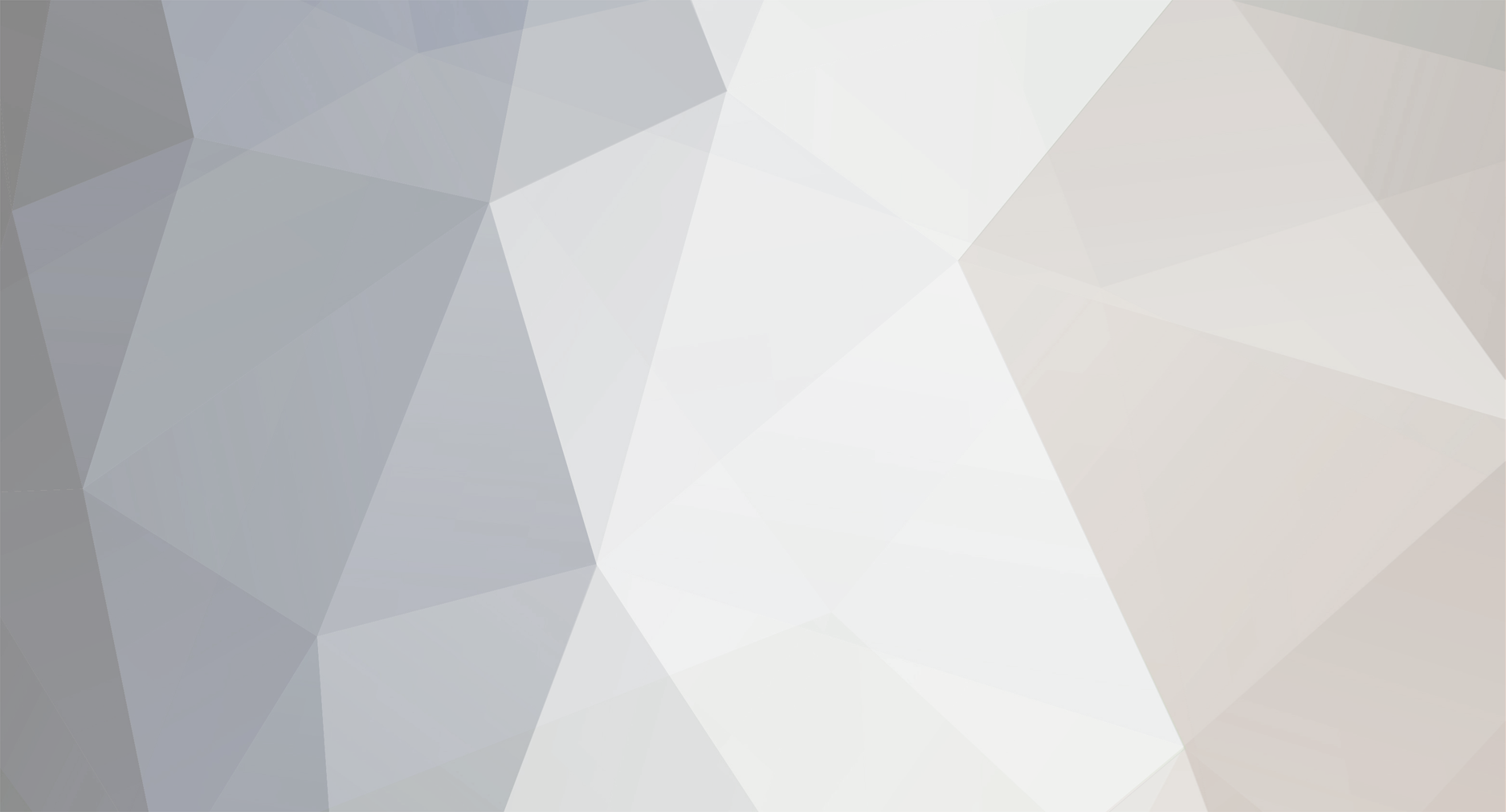
Wintergreen
Members-
Posts
107 -
Joined
-
Last visited
Never
Everything posted by Wintergreen
-
You'll have to send them an e-mail with an new temporary password as well as change their password in the DB to the new one. Then have them log in and change it
-
[code] $numcol = 0; $numpics = 7; // this is however many pictures total you want displayed for($i = 0; $i < $numpics; $i++) { if($numcol == 0) { echo "<tr>"; } echo "<td width=\"162\" bgcolor=\"#e6e6e6\" valign=\"top\"><p align=\"center\">"; echo "<img border=\"0\" src=\"images/somepic.jpg\" width=\"150\" height=\"113\"><br>"; echo "<span class=\"text1\"><b>some text</b><br>some text<br>some text<br></span>"; echo "</p></td>"; $numcol++; if($numcol == 3) { echo "</tr>"; $numcol = 0; } } if($numcol != 0) { echo "</tr>"; } [/code] So each time it displays a pic, $numcol goes up one. It starts out at 0, meaning it will put a <tr> in. When it reaches 4 (after having displayed 4 pics) it will put a </tr> and reset to 0. Then, if there are still more pics to be displayed, it will post another <tr> and then continue going. The last thing I do is check the value of $numcol when it's done with the for loop. If it is anything but 0 it means that there needs to be a </tr> echoed.
-
If you're getting an error, post it for us
-
Just thinking, not fully sure how you'd implement it, but you could create another column in your users table along the lines of is_banned, making it DATETIME format (0000-00-00), the default being empty. Then if you want to ban someone you can make yourself a form allowing you to ban for however many days or whatever you'd like, and then use date functions to get the current date and add your ban time to it. Then make a check when users log in to see if they're banned by comparing the current date to the is_banned row, and if their ban time isn't up just exit out of the login or print a message saying what's up.
-
Is this possible within PHP? If not - what technology do I need?
Wintergreen replied to Anidazen's topic in PHP Coding Help
My only advice would be to find some way of caching the sites, unless they are being updated very quickly and you have to have the latest info from them. Then you can just recache them every few hours or something along those lines. -
http://www.php.net/manual/en/function.mkdir.php
-
What I'm saying is, you don't ever have to explicitly say 'don't insert into the DB'. It happens automatically with the IF ELSE statements. You just have to learn to set them up correctly [code] if( (empty($_POST['username']) || (empty($_POST['password']) || (empty($_POST['confirmPass']) || ($_POST['password'] != $_POST['confirmPass']) || (empty($_POST['firstname']) || (empty($_POST['lastname']) || (empty($_POST['email']) || (empty($_POST['business']) || (empty($_POST['title']) || (empty($_POST['address']) || (empty($_POST['city']) || (empty($_POST['zip']) || (empty($_POST['phone']) || (empty($_POST['fax']) || (empty($_POST['mobile']) || (empty($_POST['comments']) || (empty($_POST['specialties']) || (empty($_POST['photo']) ) { Here's where you check the values and create your messages, like 'you must have a username', 'your passwords don't match'. So as an example, if (empty($_POST['username']) { $eg_error['username'] = "You need a username."; if ($_POST['password'] != $_POST['confirmPass']) { $eg_error['confirmPass'] = "Your passwords do not match."; } } [/code] Now, in this format, ALL fields are required to have data or the form will not go into the else loop that does your second round of checks. See how this works? If you want to make some of the fields optional, then take their respective check out of that if statement.
-
My code that I posted is an example from something I made, you would have to modify it to fit with your variable names etc. I'm not quite sure how !empty($_POST) works, I haven't ever used it, but for that first IF statement, you're really trying to see if there IS anything empty, right? Again I can't comment on using something like empty($_POST), but either way, you're trying to see if they're empty, not if they're full, so take the ! out of the first IF statement. As for your setup: So the first big IF statement is just checking to see if the variables are empty or not. If they are empty, it creates the error message. If none of them are empty, you're wanting it to go into the second big IF, do a few more validation things and then if those turn out okay, insert to the DB. So this would be the basic layout you'd want to use then: [code] if(any of the $_POST vars are empty) { check each one individually, if one is empty create the error message $header="form.php"; // or whatever page your original form is on } else { // So this else statement is only entered if all of the $_POST variables you checked above have something in them Here do your other validation statements for file size, etc. if(the validation turned out okay) { Insert info into the DB $header="success.php"; // Name of whatever page you want them to go to if everything is good } else { Something was wrong with the validation, so set any error message you want to display here $header="form.php"; // Again, take them back to the original page and display any problems } } header('location:$header'); [/code] So in the ELSE statement, there is another IF ELSE set. It only enters here if the $_POST variables have datat in them. Then it does the other validation you wanted. If those turn out well, then it writes to the DB, if something is wrong, it enters the else loop. Does this make more sense?
-
Look at the two main if statements you have, they're the same... So if it goes into one, won't it also go into the other? Why do you have an empty else statement sitting there? Take a look at the first post I made in here. The base is if (any of the post variables are empty go here) else (do what's in here)
-
strtolower() Then use your function you found, ucwords
-
You're going to have to show your code, otherwise we have nothing to go on
-
http://www.php.net/manual/en/function.strtoupper.php
-
You only use echo when you want something displayed on the screen. When you're declaring a new variable and making it equal to the post ones, you just do variable = $_POST['whatever'];
-
Well, I assume that Flash has variables? $blah denotes a PHP variable. Flash isn't PHP, so using the $ wouldn't work. Again, I don't know actionscript but there has to be a way to send the score with a link like blah.php?score=variable. Getting the variable once you've sent it is easy. So you probably need to find someone who can do Flash
-
determine variable names for dynamic variables *RESOLVED*
Wintergreen replied to markbett's topic in PHP Coding Help
How are they specifying the amount? If they're doing it by a small text-area, just give it a name and then it's easy to do. You can just access the number of people coming with them with $_POST['guests'] or whatever you choose to name the element. Then just do [code] if(!empty($_POST['guests'])) { $guests = $_POST['guests']; $_SESSION['guests'] = $guests; for($i = 0; $i < $guests; $i++) { echo "Guest " . $i . "<br />"; echo "<input type=text size=25 name=" . $i . "><br />"; } } [/code] The session var is just so you know how many names you'll be checking on the next page. -
whats teh best way to carry data accross multiple forms?
Wintergreen replied to markbett's topic in PHP Coding Help
Probably faster and easier -
php.net seems to be down right now, but the function you can use to check and see if it is numeric is is_numeric()
-
Post your errors, that helps us know what to look for. For starters, I think if you're comparing a variable to a string you need to put it in quotes, either '' or "", so try putting monkey in quotes. Other than that, from what I see there you're not accessing any data from anywhere. If you're using forms and the method is post, you use the value from the form by $_POST['variable_name'].
-
You need session_start() at the top of each page
-
Here's the tutorial: http://www.phpfreaks.com/tutorials/65/0.php You'll have to change some of the table to fit what you need, which will in turn make it so you need to change some other stuff as well, but it won't be hard. Since you're doing a site, I doubt you'll have people use their real names, so you don't need a first name or last name, just a username. Things like that, they're not too hard to watch out for. Also, you don't need to bother with the marketing stuff, just the membership system.
-
There's a login tutorial on this site that works fine. As for making sure they're logged in to view any pages, all you do is make a file called logincheck.php or something, and put this inside [code] <? session_start(); if(empty($_SESSION['username'])) { header("location: index.php"); } ?> [/code] Then at the top of each page (Except index.php), put this [code] <? session_start(); include 'logincheck.php'; ?> [/code] This works in conjunction with the login tutorial on the site.
-
You name each form element so that you can check its value. Here's an example [code]<form name=entry method=post action=makeentry.php> <table width=390 cellspacing=0 cellpadding=0> <tr><td>Entry Title</td></tr> <tr><td width=100%><input name=posttitle type=text size=45></td></tr> <tr><td>Entry type</td></tr> <tr><td><input type=radio name=posttype value=0> General Update<br /> <input type=radio name=posttype value=3> Journal Update<br /> <input type=radio name=posttype value=2> Art Update<br /> </td></tr> <tr><td>Body</td></tr> <tr><td width=100%><textarea name=bodytext type=text rows=20 cols=34></textarea></td></tr> <tr><td><input name=submit type=submit value=submit></td></tr> </table> </form>[/code] Then I check the values, first I see if they're empty, and if they aren't, it starts checking values and then inputs. [code] <? session_start(); if( !empty($_POST['posttitle']) && !empty($_POST['bodytext']) && !empty($_SESSION['user_level'])) { include 'db.php'; $posttitle = mysql_real_escape_string(strip_tags($_POST['posttitle'])); $post_body = ereg_replace("\n", "", $_POST['bodytext']); $post_body = ereg_replace("\r", "", $post_body); $post_body = mysql_real_escape_string(strip_tags($post_body, '<img><a>')); $poster = $_SESSION['screenname']; $posttype = $_POST['posttype']; mysql_query("INSERT INTO posts (title, post_body, post_time, post_type, poster_name) VALUES('$posttitle','$post_body','$post_time','$posttype','$poster')") or die(mysql_error()); } header("location: index.php"); exit(); ?> [/code] So as you can see, if the variables have stuff in them it goes in, does a few things and then inputs it into the DB, otherwise it skips right to the header, which sends them (in this case) to index.php
-
admitedly I've never dealt with actionscript. But I'm assuming you know how to get from one page to the other, and you can pass the variable in the value area. So with PHP variables looking like $value, the link would look like page.php?score=$value